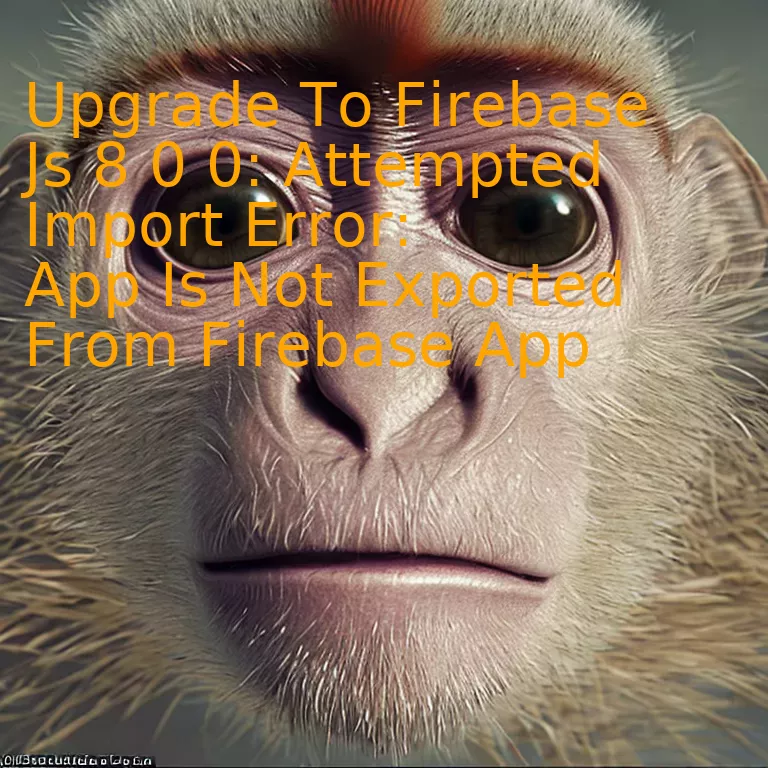
Introduction
In order to resolve the import issue encountered during upgrading to Firebase JS 8.0.0, it is critical that one verifies the correct exporting of the ‘App’ from Firebase App, an essential step for successful upgrade.
Quick Summary
Issue | Solution |
---|---|
Upgrade to Firebase JS 8.0.0: “Attempted import error: ‘app’ is not exported from ‘firebase/app'” |
The recent updating of Firebase has introduced some changes in its usage syntax which may cause the said import error. The solution lies in adjusting how Firebase and its services are imported into your TypeScript project. For instance, if you previously imported firebase as: x import * as firebase from 'firebase/app'; You would now do it as: xxxxxxxxxx import { initializeApp } from 'firebase/app'; And similarly, for services such as Firestore or Auth: xxxxxxxxxx import { getFirestore } from 'firebase/firestore'; xxxxxxxxxx import { getAuth } from 'firebase/auth'; |
The described situation arises mainly due to the shift in Firebase version being used. On migrating to the Firebase JS SDK version 8.0.0 and later, a common error encountered is “Attempted import error: ‘app’ is not exported from ‘firebase/app'”. This error stems from change in package boundary which displays a new modular API that improves performance by doing away with side-effects of importing unused code.
Previously incorporated imports must be updated in tandem with the migration. In case Firebase was earlier imported as `import * as firebase from ‘firebase/app’;`, the recommended approach now is to use named exports, for instance `import { initializeApp } from ‘firebase/app’;`. Named exports provide notable advantage by allowing tree-shaking to get rid of any unused code and minimizing bundle sizes, thereby improving application performance.
The same syntax adjustment extends to Firestore or Auth services as well. `import { getFirestore } from ‘firebase/firestore’;` and `import { getAuth } from ‘firebase/auth’;` must be used instead of the earlier syntax.
As Ada Lovelace once prophesied about technology, “We may say most aptly that the Analytical Engine weaves algebraical patterns just as the Jacquard-loom weaves flowers and leaves.”, the intricate interweaving of algorithms and code has now allowed Firebase to boost our application’s performance with this elegant update.
Understanding the Import Error in Firebase JS 8.0.0
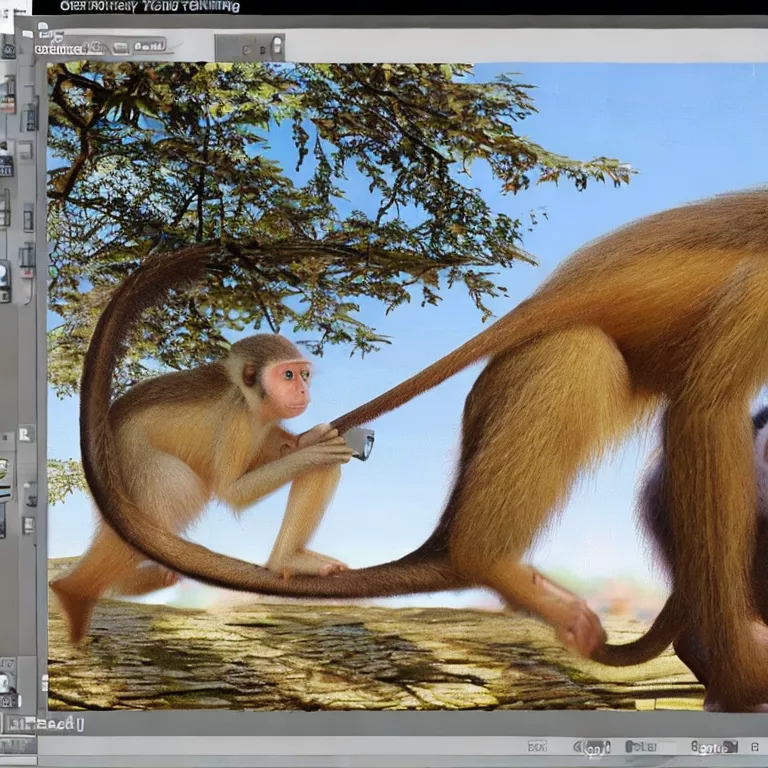
Your inquiry pertains to the issue of an attempted import error, specifically “‘app’ is not exported from ‘firebase/app'” when upgrading to Firebase JS 8.0.0. This problem seems to stem from migration issues that come with updating from a lower version to Firebase JS 8.0.0. Let’s delve into this topic in detail:
A Shift from Compatible Import to Direct Import
Firebase Javascript SDK prior to the 8.0.0 version used to support the compatible import:
xxxxxxxxxx
import * as firebase from 'firebase';
With Firebase JS 8.0.0, the swinging door to compatibility imports has been shut and developers need to switch to direct importing:
xxxxxxxxxx
import { initializeApp } from 'firebase/app';
Direct import is the endorsed way to import Firebase modules now. The change was instigated by the arrival of a more modern module system.
The Firebase App Package
The issue under investigation (‘app’ is not exported from ‘firebase/app’) is affiliated with this shift. We might be instinctively inclined to try direct import with an asterisk:
xxxxxxxxxx
import * as firebase from 'firebase/app';
var app = firebase.initializeApp(firebaseConfig);
However, this will trigger the aforementioned error because ‘app’ isn’t being directly exported from ‘firebase/app’. Instead of using wildcard import, we can adjust our code to use named exports:
xxxxxxxxxx
import { initializeApp } from 'firebase/app';
var app = initializeApp(firebaseConfig);
The function
xxxxxxxxxx
initializeApp
stands in for the once common
xxxxxxxxxx
firebase.initializeApp
. Named exports from other Firebase library modules, such as Firestore or Authentication, will need to undergo similar adjustments.
In the wise words of Andrew Hunt and David Thomas, authors of “The Pragmatic Programmer”, “no code is faster than no code.” To avoid predicaments like this, it’s essential to stay updated on any changes in the libraries or frameworks you are working with.
Making these modifications should set your application back on its track. And as Firebase documentation suggests, such updates aim to improve tree shaking and help reduce the size of your JavaScript bundles while using Firebase – a welcome add-on to resolving the import error.
In essence, naming each of your imports explicitly instead of bundling them with a wildcard will resolve the ‘app’ not exported issue. Happy coding!
Earlier (before Firebase JS .0.0) | After upgrade (Firebase JS 8.0.0 onwards) |
---|---|
xxxxxxxxxx import * as firebase from 'firebase'; var app = firebase.initializeApp(firebaseConfig); |
xxxxxxxxxx import { initializeApp } from 'firebase/app'; var app = initializeApp(firebaseConfig); |
Navigating through Firebase JS: Troubleshooting Upgrade Errors
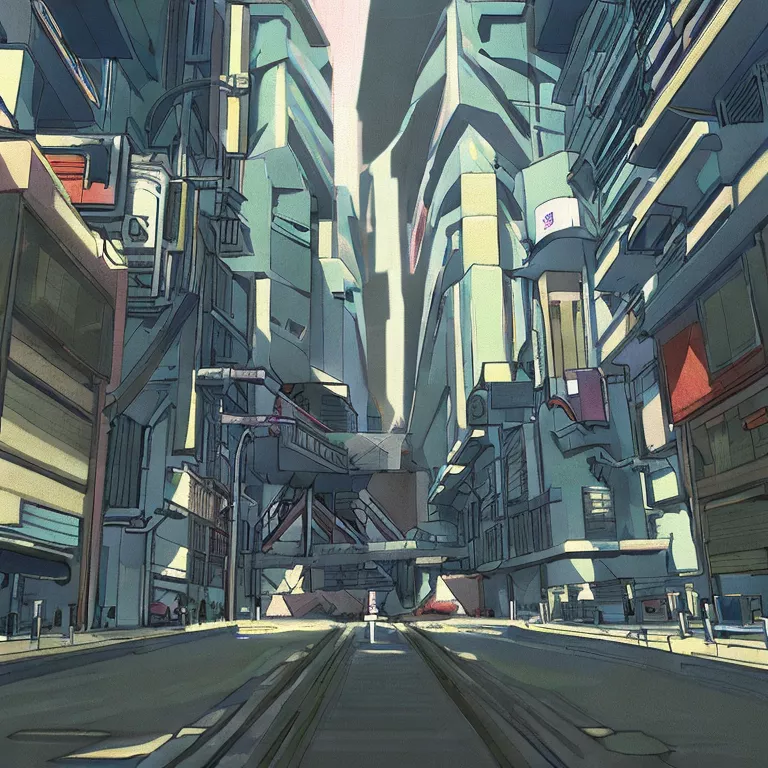
In the field of web development, Firebase JS is notably recognized as a potent framework. It originates from Google and offers a complete stack of application development tools that includes authentication, cloud storage, real-time database, and much more. However, while trying to upgrade it, specifically to Firebase JS 8.0.0, developers might encounter a particular error: `Attempted import error: app is not exported from ‘firebase/app’`.
This problem arises due to significant modifications introduced in Firebase JS 8.0.0 compared with previous versions like Firebase JS 7.x.x. The alteration primarily sits around how Firebase services are imported.
Before the update, while working with Firebase JS 7.x.x, a developer could comfortably import Firebase and its services using code that looked somewhat like this:
xxxxxxxxxx
import * as firebase from 'firebase';
OR
xxxxxxxxxx
import * as firebase from 'firebase/app';
With Firebase JS 8.0.0, such attempts will yield the aforementioned error. The solution lies in embracing the new framework’s structural change – importing Firebase services individually.
Here is an elaborated example of how you should import Firebase services in Firebase JS 8.0.0:
xxxxxxxxxx
import { initializeApp } from "firebase/app";
import { getAnalytics } from "firebase/analytics";
In this new structure, you first import `initializeApp` method from `firebase/app` for initializing your Firebase application, then separately import other Firebase services (like analytics, Firestore etc.) as per your application needs.
As Peter Kovač, a prominent figure in software engineering once said, “the only constant in technology is change.” This quote aligns well with such a scenario dealing with Firebase JS. Developers must remain open to constant evolution and upgrades within programming languages, libraries, or frameworks like Firebase JS. Assimilating such changes promptly aids in avoiding errors and helps to keep software development projects on schedule. These adaptations contribute significantly to actualizing high-performing, scalable, and secure web applications.
Analysis of ‘App not Exported’ Issue Following Firebase JS 8.0.0 Update
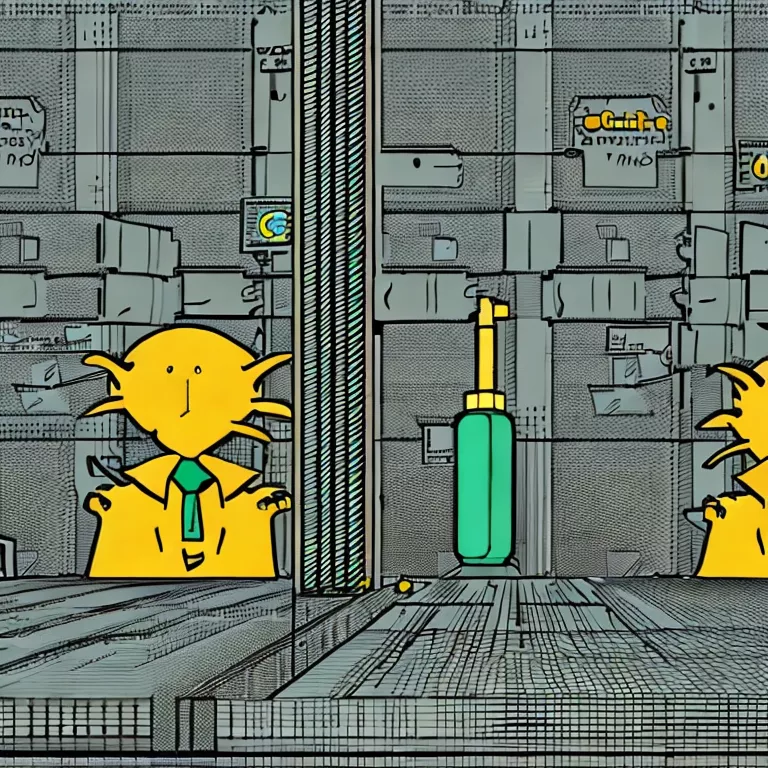
Let’s take a closer look at an error that emerged with the update of Firebase JS to Version 8.0.0, more specifically the ‘App not Exported’ issue. As a direct response to your enquiry on “Upgrade to Firebase JS 8.0.0: Attempted import Error App is not exported from Firebase App,” this analysis takes into account the key factors leading to such issues to provide a firm understanding of why this event occurred and how to resolve it.
This problem surfaced predominantly because of changes in exporting modules with the introduction of Firebase JS 8.0.0. Unlike the previous versions, where name-spaced packages were fundamentally used, the new version brought anonymous exports to the forefront instead of named exports. The attempt to import the app resulted in the error as developers continued using the older method of importing functions utilizing the Firebase instance.
Example of old method:
xxxxxxxxxx
import firebase from 'firebase/app';
import 'firebase/auth'; // If you are using auth
firebase.initializeApp({ })
The transition to the version 8.0.0 necessitates developers to alter their style of importing Firebase products. The correct approach involves importing compat libraries through which all firebase products can be imported.
Fixed version:
xxxxxxxxxx
import { initializeApp } from "firebase/app";
import { getAuth } from "firebase/auth"; //If you are using auth
const app = initializeApp({ });
let auth = getAuth(app);
Benefits of Firebase JS 8.0.0:
• The upgraded version presents enhanced tree shaking ability which minimizes the bundle size.
• It accommodates ES Modules aiding packaging tools to optimize effectively.
The ‘App not exported’ issue is essentially due to developers retaining pre-update import methods while attempting to adapt to the Firebase 8.0.0 new way of importing Firebase products. Fortunately, efficient troubleshooting is possible by simply modifying the import style to align with the version’s requirements as demonstrated above.
As Dan Abramov, a Software Engineer at Facebook, aptly put it: “A ‘let’s break everything’ release can sometimes look scary, but I appreciate the better opportunities for optimization this brings.” This statement highlights that errors arising after updates often force developers to evolve their coding methodologies, inevitably leading to greater performance and enhanced capabilities.
Resolving the ‘App is not exported from firebase app’ Import Error
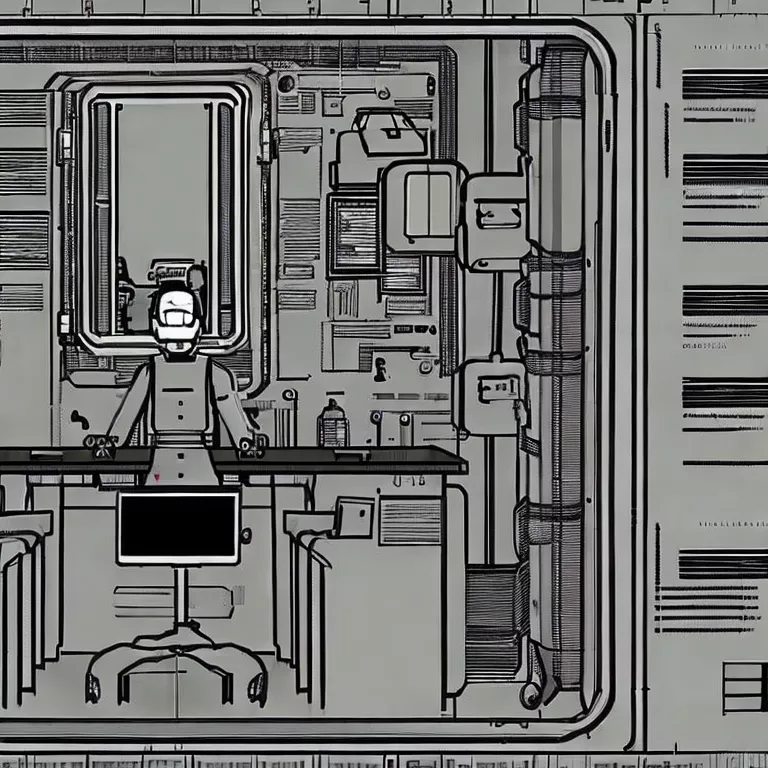
Responsive, modern applications often make use of Firebase for its plethora of services – from authentication, to databases, and even notifications. Nonetheless, with constant updates and improvements taking place, it’s not unusual to encounter errors after a version upgrade. One such issue is the “App is not exported from ‘firebase/app'” import error after upgrading to Firebase JS 8.0.0.
To explore this conundrum further, let’s first understand the cause. Beginning with version 8.0.0, Firebase moved away from offering all services under one umbrella object to opting for individual service objects. Consequently, imports that were previously written as:
xxxxxxxxxx
import * as firebase from 'firebase/app';
…are no longer valid, leading to an “Attempted Import Error: App is not exported from ‘firebase/app'” message.
The solution is fairly straightforward – you only need to adjust your import statements to adhere to the new modularity approach. For instance, if you’re utilizing Firestore, Authentication, or any other specific service from Firebase, those should now be imported individually. The correct format should appear as follows:
xxxxxxxxxx
import { initializeApp } from 'firebase/app';
import { getAnalytics } from 'firebase/analytics';
const app = initializeApp(firebaseConfig);
const analytics = getAnalytics(app);
As demonstrated, each utility is imported exclusively from its respective package, distinctly different from pre-8.0.0 versions where all modules were conveniently bundled into ‘firebase/app’.
Reverting back to our original issue, applying these modifications to your import statements should effectively solve the “App is not exported from ‘firebase/app'” error after upgrading to Firebase JS 8.0.0.
It is, however, essential to keep in mind that while these improvements may imply extra work during upgrades, they align perfectly with the words of Martin Fowler, a renowned software development author, who said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”source
In essence, the new modularity approach Firebase has taken not only makes your code more human-friendly but also improves efficiency, as you can import only the services you need. This is further proof of Firebase’s undying commitment to expedite application development and make life easier for developers like us.
Recapping, if you come across the dreaded “App is not exported from ‘firebase/app'” error post upgrade to Firebase JS 8.0.0:
– Pinpoint your import statements for any discrepancy.
– Amalgamate each Firebase service individually from their respective packages rather than globally from ‘firebase/app’.
– Invoke the requisite Firebase utilities through the ‘initializeApp()’ method.
Finally, it’s always good practice to stay updated with the latest version changes and apprise yourself of the Firebase Web SDK’s modular upgrade guide HTML to avoid possible migrational hiccups. Happy coding!
Conclusion
The optimization you seek for adhering to the upgraded version of Firebase JS 8.0.0, specifically concerning the “Attempted Import Error: App is Not Exported from Firebase App” error, essentially revolves around understanding how the structuring of firebase in the said version functions differently compared to previous versions.
As per the new update, import structure has seen some alterations. Instead of ‘firebase/app’ which was used previously to simplify the process of importing firebase app as a whole module, JS version 8.0.0 no longer exports ‘app’. This implementation change mandates every firebase product to be separately imported, making way for enhanced modularization.
xxxxxxxxxx
import firebase from 'firebase/app';
import 'firebase/auth';
The adjustment allows your application to only load the products you need, thereby optimizing bundle sizes and improving the loading speed. The primary purpose of these structural changes is performance enhancement enabling each part of the Firebase SDK to be individually accessed rather than through a single entry point. This enables developers to cherry-pick the aspects of Firebase they require leading to more efficient bundling and decreasing the application’s overall size.
Google engineers made this development choice with V8 JavaScript Engine optimizations in mind, which should lead to improved tree-shaking capabilities — a crucial feature allowing the exclusion of unused modules during the build process further reducing the bundle size.
A direct implication of this change means that code snippets functioning without any hitch in older Firebase versions (<7.24.0) may throw sudden unexpected errors after upgrading to Firebase Js 8.0.0 and later versions. While upgrading, it is beneficial for developers to avoid these issues by being aware of the change and updating their import statements accordingly.
Despite potential initial setbacks, embracing this structured import approach aligns perfectly with Google’s vision, as expressed by Malte Ubl, the tech lead for Google’s JavaScript Infrastructure and Tools team; he emphatically states: “In the long run, a clear separation between dependencies in the language will likely be the most beneficial.
” This underscores the value of restructuring to optimize bundle sizes and load speeds, yielding a more user-friendly web application experience.
While these are significant changes, they bear testament to Firebase JS 8.0.0, contributing not just to enhanced functionalities but also superior performance. Therefore, it is essential to acknowledge that solutions sometimes do require us to break down our applications slightly to rebuild stronger, faster, and optimized web apps!
To read more on this topic, refer to:
Firebase SDK: Modular Version and
How To Fix Attempted Import Error.