Introduction
To efficiently execute TypeScript with a module import and module defined, Ts-Node offers a compelling solution, enhancing your project productivity by simplifying the integration of various code modules.
Quick Summary
With TypeScript’s
module system, developers are able to organize their code into separate files and folders, making the codebase more maintainable and easier to understand. With TS-Node, you have a solution that allows you to directly execute TypeScript code with module imports and module definitions, without needing to transpile it to JavaScript first.
Here’s an informative table dissecting the process:
Aspect | Description |
---|---|
TS-Node | This is a runtime environment and REPL for TypeScript – allowing execution of TypeScript codes via Node.js. |
Module Import in TypeScript | Allows the inclusion of external modules or scripts within a TypeScript file using the
import keyword. |
Module Definition in TypeScript | The process of creating a reusable block of code that encapsulates related functions, variables, and types, and can be exported for use in other modules using the
export keyword. |
When writing TypeScript programs that use modern ES6 style imports where you would typically use an import statement like
import express from 'express';
, the program must be executed in a specific way if utilizing TS-Node. Using the
-r ts-node/register
options when launching the process, the TypeScript files can be directly executed. However, since
ts-node
is not an ES6 runtime, the
--experimental-modules
flag is needed.
An execution command might look as follows:
node -r ts-node/register --experimental-modules index.ts
This command will launch Node.js, registering the ts-node module, then execute the ‘index.ts’ TypeScript file with all it’s module imports and exports properly handled.
As Douglas Crockford, a well-known figure in the JavaScript community once said, “Programming is not about typing… it’s about thinking.” Understanding how to structure and import modules in your TypeScript projects goes a long way towards improving both the maintainability and scalability of your application.
Understanding the Basics of TypeScript with Module Import and Module Defined
TypeScript, a strongly-typed superset of JavaScript brought us features like static typing and modules. Executive Typescript with module import and module defined using Ts-Node is an essential part of understanding the fundamentals of TypeScript development.
TypeScript Modules
Modules in TypeScript are designed to work with libraries, yet they seamlessly fit into most JavaScript environments. They allow you to export and import functionalities, types, or values between different components.
A module can be defined in TypeScript as follows:
// greeter.ts export function greet(message: string) { return "Hello, " + message; }
The function
greet
has been exported, which means it’s now accessible from other parts of your application.
Importing Modules
With the module defined, we can then import it into another components. To import the above module (greeter.ts), you’d use the following code:
// main.ts import { greet } from './greeter'; console.log(greet('World'));
Here, the previously defined module ‘greeter’ has been imported in ‘main.ts’. As a result, the function
greet
is accessible to be utilized in ‘main.ts’.
Executing TypeScript using TS Node
Ts-Node is a development tool that allows developers to run TypeScript files (.ts) directly without the need for separate compilation step. This is extremely useful, particularly when executing TypeScript with module import and module defined, as in our case.
To execute the TypeScript file, we use the following command in console:
$ npx ts-node main.ts
If everything went perfectly, this will log “Hello, World” in the console.
It’s important to note that Ts-Node is not intended for production use. In production, you should ideally compile your TypeScript to JavaScript ahead of time.
As Steve Jobs once said, “Deciding what not to do is as important as deciding what to do.” This quote encapsulates the importance of understanding the right tools and methods to use in any given context, be it in regards to using TypeScript modules or understanding when or where to use a tool like Ts-Node.
Understanding these basic features of TypeScript, including defining and importing modules, as well as executing them directly with tools like Ts-Node, provides the foundation required to develop complex projects in TypeScript effectively.
For more information or further elaboration, refer to the official TypeScript Documentation on Modules or the Ts-Node GitHub page.
Advanced Utilization of Ts-Node in Executing TypeScript
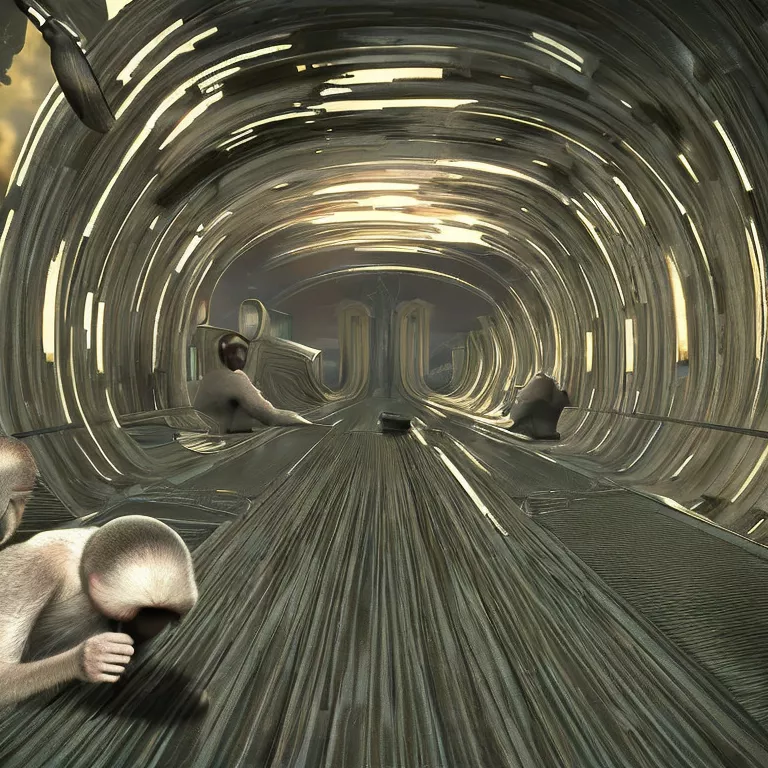
Ts-Node is an invaluable tool for runtime TypeScript transpilation, which essentially converts the TypeScript code into readable JavaScript. When incorporating module imports and module definitions, there are good practices that streamline the development process.
Module Importing and Definition with Ts-Node
CommonJS Module Pattern:
In Node.js, and therefore also in ts-node, the most widely used pattern for modularization is CommonJS. While writing TypeScript, you can adhere to its counterpart using
import
statement.
import * as express from 'express'; const app = express();
Always remember that while writing TypeScript which will eventually run on Node.js runtime (via ts-node or compiled JavaScript), you need to stick with the CommonJS pattern.
Import Versus Require:
Although TypeScript allows both
import
and
require
syntax, there’s a crucial difference between them that one should be aware of. In TypeScript, the
import
keyword on its own does not necessarily copy the whole exported list of objects; instead, it creates a type reference. Hence, calling methods on imported object may not work as expected.
// Expected behaviour const fs = require('fs'); fs.readFileSync('package.json'); // This will work // Unexpected behaviour import * as fs from 'fs'; fs.readFileSync('package.json'); // Error: fs.readFileSync is not a function
Using
require
ensures that the fs module in the above example works correctly, even though the newer
import
might appear more favorable in TypeScript development.
Compile-time and Runtime Type Assertion:
To make your TypeScript modules co-operate with existing JavaScript code, occasional use of type assertion (
as any
) can be extremely useful. A statement like
import * as fs from 'fs'
tells TypeScript to assert the type of fs during runtime, according to your definitions or those found in available @types/node package.
import * as untypedFs from 'fs'; const fs = untypedFs as any; // Now you can use fs like a JS variable fs.readFileSync('package.json');
The example above enables the use of all fs methods through compile-time and runtime type assertions. It’s a powerful mechanism for transitioning from a JavaScript codebase to TypeScript.
In the larger context of software development, it’s worth reminding ourselves that we’re not just writing programs for machines to understand, but also to communicate ideas between humans. As programming luminary Martin Fowler so aptly put it: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
As such, integrating ts-node, TypeScript, and module systems may require negotiating trade-offs between idealized practices and reality—but ultimately, the goal should always be enhancing readability and maintainability of our code.
Diving Deeper: Working with Imports and Modules in TypeScript
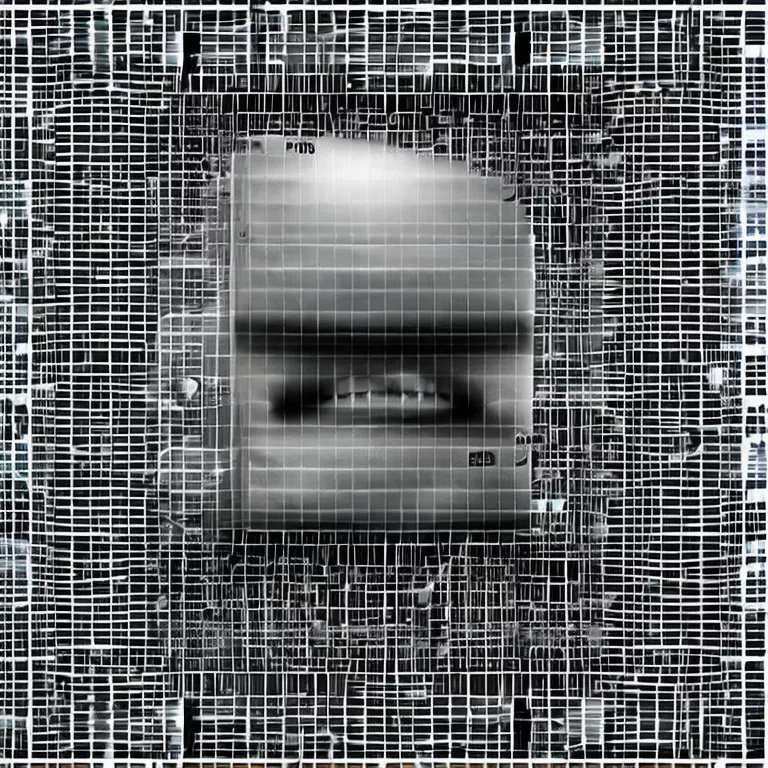
Working with imports and modules in TypeScript, specifically when using the ts-node module to execute TypeScript scripts directly, entails a keen understanding of how TypeScript structures its codebases into modules.
Understanding Imports and Modules in TypeScript
TypeScript utilizes two kinds of module systems: external and internal. However, ES6 style ‘import’/’export’ modules (commonly referred to as “external” modules) are largely favored by the community for their scalability, controllability, and simplicity.
To import a module in TypeScript, we use the
import
keyword. For instance:
import { Component } from '@angular/core';
Here, we are pulling the ‘Component’ entity from an external module (@angular/core).
Working with ts-node Module
ts-node is an npm package that allows for seamless TypeScript compilation and execution. It ensures you can use TypeScript to write scripts without the need to manually compile them to JavaScript first.
To start using ts-node, it must be installed globally or as part of your project dependencies as follows:
npm install -g ts-node
Or locally:
npm install --save-dev ts-node
Once installed, you can now execute TypeScript directly by simply doing:
npx ts-node script.ts
Your TypeScript files should utilize ES6 style import/export syntax to operate with ts-node. If they’re not, TypeScript configurations could be adjusted to accommodate the desired module type.
Dealing With Module Resolution Issues
Occasionally you may encounter issues revolving around module resolution. These issues typically stem from TypeScript struggling to locate a module specified within an import statement. To ensure smooth compilation, consider these tips:
* Ensure your file paths are correct.
* Use relative file paths for local modules and absolute paths for node_modules.
* Leverage TypeScript’s path mapping feature, which allows you to specify paths and baseUrl within tsconfig.json.
As said by Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Just as Linus suggests fun in programming, effective handling of imports and modules can supercharge one’s enjoyment while writing TypeScript thanks to the cleaner and leaner codebase.
Practical Use Cases: Application of Ts-Node Execution & Module Definitions in Real World Scenarios
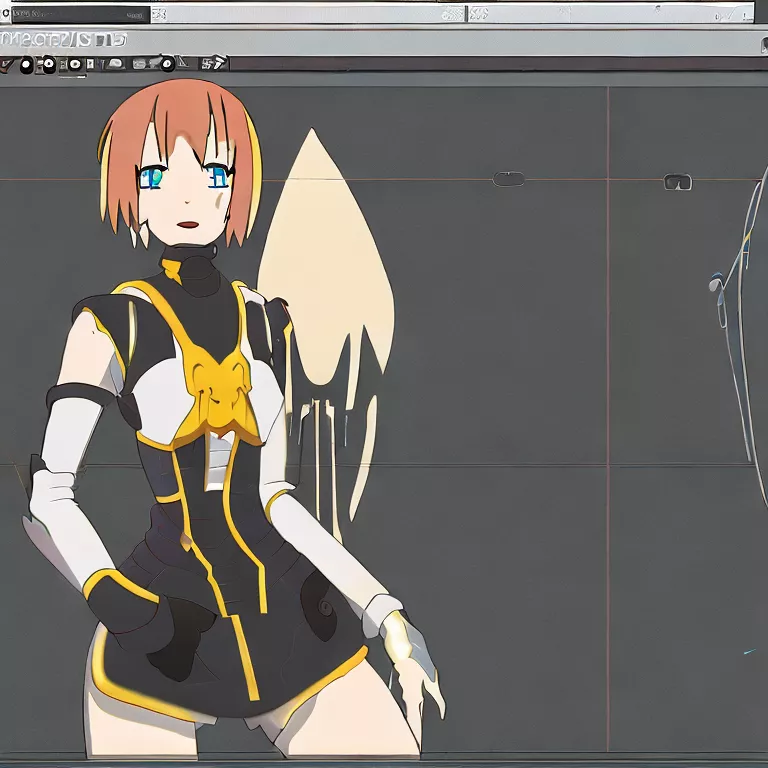
In real-world scenarios, the execution of TypeScript using Ts-Node encompasses several practical use cases including module importation, and definition that are impossible to ignore given their central role in development workflows.
To begin with, Ts-Node offers the feasibility to execute TypeScript code directly without first having to compile it down to JavaScript. This is particularly important in developing environments that require frequent manipulation of codes such as testing and prototyping. Here’s a simple example:
import { sayHello } from "./greeting"; sayHello("Typescript");
The above script can be run directly, assuming there’s a “greeting” module defined in the project:
export function sayHello(name: string) { console.log(`Hello, ${name}!`); }
Secondly, Ts-Node finds its application in interpreting TypeScript files in runtime—for instance, launching applications, running scripts, or publishing packages. Fundamentally, when you choose to launch your whole application with Ts-Node, it will interpret any imported “.ts” file on-the-fly.
Finally, Ts-Node allows for the definition of modules—an aspect that paves the way for advanced TypeScript functionalities. Ts-Node provides mechanisms to make sure the modules written in TypeScript are executed perfectly. In particular, it supports ECMAScript 2015 (ES6) syntax for importing and exporting modules, synergizing well with cutting-edge frontend technologies like React and Vue.
As Dr. Venkat Subramaniam, founder of Agile Developer, Inc., once said; “Code is like humor. When you have to explain it, it’s bad.” So, to apply these concepts practically, ensure that you structure your code in a self-explanatory manner—your modules should strictly adhere to single responsibility principle, which promotes maintainability and ease of comprehension. For best practices, checkout TypeScript official documentation. Remember, Ts-Node can help you streamline your development process but effectively utilizing its features requires practice and deep understanding of TypeScript.
Conclusion
Applying the power of TypeScript through ts-node offers numerous benefits- from enabling development in a statically-typed environment to providing advanced debugging tools. Injecting this into our Node.js runtime facilitates an enrichened development process, enhancing code reliability for robust and scalable applications.
Let’s spark an exploration of how ts-node enables us to execute TypeScript scripts by supporting module import and module definitions:
TypeScript Execution with Ts-Node
The key strength of ts-node lies in its inherent ability to execute TypeScript – optimal for running ‘on-the-fly’ tests during development and debugging stages. This comes as an upgrade to manually compiling TypeScript files into JavaScript before execution.
Module Import Support
Node.js relies heavily on modules – reusable pieces of code that allow functionality to be added from different files or modules. TypeScript also utilizes these concepts, albeit with some differences. Through ts-node, common patterns such as ES6 style module imports are adopted, offering seamless transitions between the two languages.
import { helloWorld } from './module'; console.log(helloWorld);
With the above script executed using ts-node, we get an efficient marriage of TypeScript static typing with Node.js dynamic functionality.
Module Definitions
Another intriguing aspect is Module Declaration or ‘ambient’ modules in TypeScript, allowing us to define custom modules. Defining modules not written in TypeScript (e.g., JSON) makes it a perfect fit for Node.js, known for its diverse module support:
declare module "*.json" { const value: any; export = value; }
Executing such a TypeScript script via ts-node emits no errors, seamlessly blending TypeScript definitions into Node.js runtime.
Accomplishing TypeScript execution using ts-node integrates flawlessly with Node.js, proffering a robust development experience. As software architect Robert C. Martin once [said](https://www.goodreads.com/quotes/8366870-i-m-not-a-great-programmer-i-m-just-a-good-programmer), “The only way to go fast is to go well.” It seems the combination of TypeScript’s static type-checking and ts-node’s platform integration has us heading ‘fast’ towards well-drafted applications.
All technologies have their strengths and weaknesses, but the synergy offered by combining TypeScript with ts-node makes for an effective tool in creating robust, efficient, and scalable runtime environments.