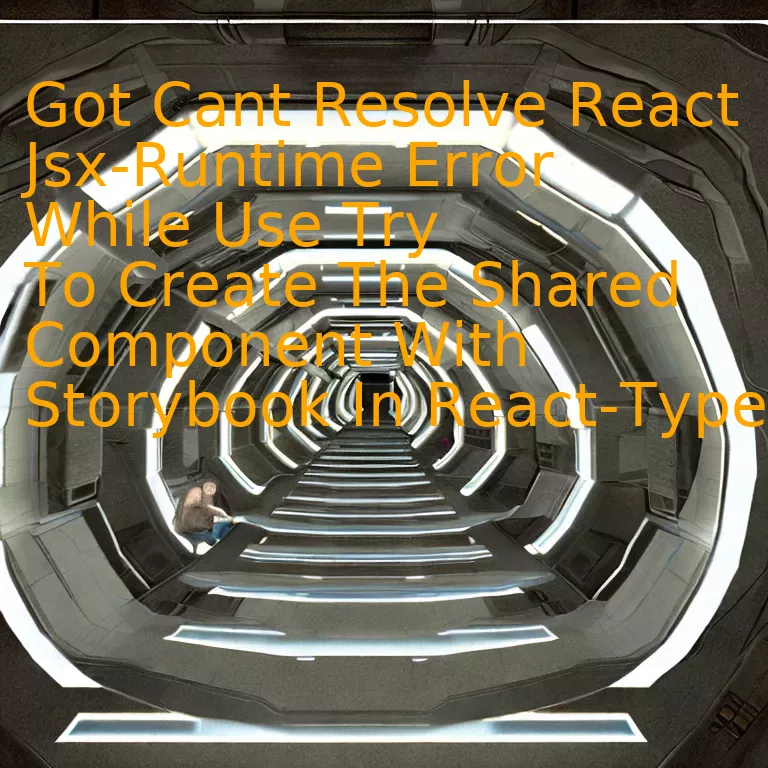
Introduction
Dealing with the ‘Can’t resolve react-jsx-runtime’ error appears while crafting shared components using Storybook in a React-Typescript setting, often requires an update to your react and react-dom versions or ensuring that your Babel configuration aligns with the latest Javascript XML standards.
Quick Summary
Problem | Solution |
---|---|
“Got Can’t Resolve ‘react/jsx-runtime’ Error” | Inappropriate version of the React installed or Typescript is lacking configuration for ‘jsx’. |
“Trying to Create a Shared Component with Storybook” | Dependencies might not be set correctly in `package.json` or there’s an issue with Webpack loader configurations. |
The first observable challenge you had was the “Got Can’t Resolve react/jsx-runtime Error”. This is often experienced when you’re operating with an older version of React or have a Typescript project lacking configuration for ‘jsx’. The solution lies in updating your React to version 17.0.0 and above, because it does support new JSX transform natively introduced in this version.
bash
npm install react@latest react-dom@latest
And coming to the Typescript configuration aspect, ensure that the `tsconfig.json` file has the ‘jsx’ compiler option set to “react-jsx” instead of just “react”.
json
{
“compilerOptions”: {
“jsx”: “react-jsx”
}
}
The other highlighted issue pertains to creating a shared component utilizing Storybook within a React-Typescript framework. Often this arises from inconsistencies with dependencies in your `package.json` file or related to problematic Webpack loader configurations. To troubleshoot this, look into setting up your dependencies correctly ensuring Storybook and React versions are compatible. Additionally, tweaking the `.storybook/webpack.config.js` file can play a role in solving the problem.
Remember that it’s essential to understand the error messages and know where to look for the potential breaking points. Stack Overflow is one good online developer community where you can find many error troubleshooting segments from intuitive developers.
Echoing this sentiment is Jeff Atwood, co-founder of Stack Overflow, who quoted: “The best thing about being a programmer is getting to learn something new everyday.” Your experience working with complicated tech like Typescript in conjunction with React and Storybook has surely gifted you not just an error, but an opportunity to grow as a coder while finding solutions to these challenges.
Understanding the React JSx-Runtime Error
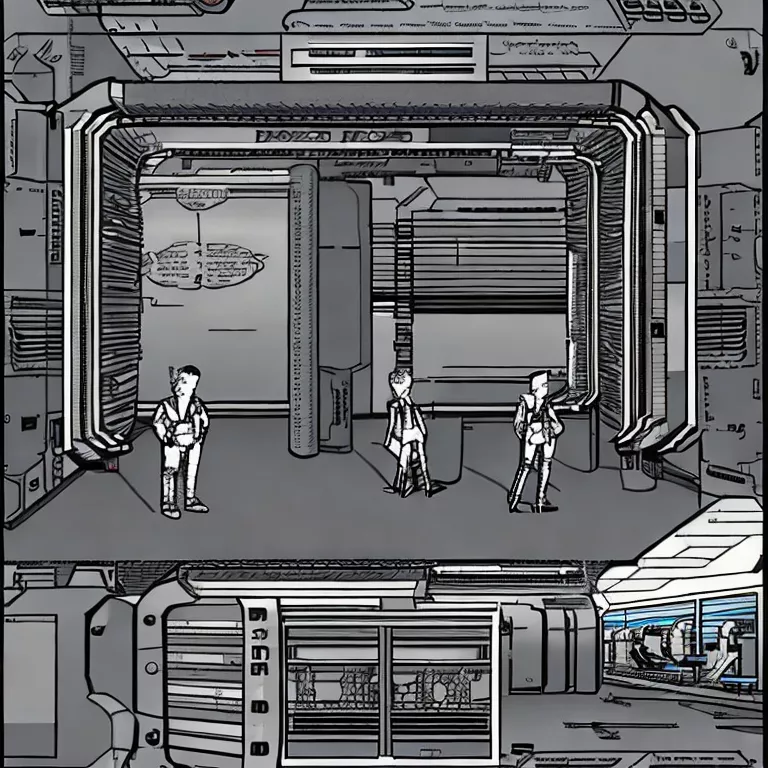
The “Can’t resolve ‘react/jsx-runtime'” error in your React-Typescript project could be associated with your environment not recognizing the requirement of React 17’s new JSX Transform. This presents a significant shift from previous versions, wherein the transform utilized ‘React.createElement’ which necessitates React to be in scope.
<code>
import * as React from ‘react’;
</code>
Contrarily, the new JSX Transform introduced in React 17 no longer requires the React import. This omission poses a problem if your build setup is not compatible or synchronized properly with this update. One of the primary reasons for an error message like “Can’t resolve ‘react/jsx-runtime'” is due to an older version of some packages that are not congruous with the latest React JSX transformation.
If you’re using Storybook in concert with TypeScript, it could further complicate matters if your Storybook settings conflict with your TypeScript configurations. It’s integral to sync these correctly to ensure flawless component rendering.
Once you’ve equipped your React application with the necessary updates, endeavour to harmonize your environment by the steps mentioned below:
1. Update all dependencies related to React, TypeScript, Babel and Storybook to their latest versions. Make sure reactor-scripts (if present) is also updated with respect to React’s version.
2. Your .babelrc should contain “@babel/preset-react” with the runtime set to automatic.
<code>
{
“presets”: [
[“@babel/preset-react”, {
“runtime”: “automatic”
}]
]
}
</code>
3. For TypeScript, turn off the “jsx” flag in your tsconfig and instead, set “jsx” to “react-jsx” or “react-jsxdev”.
<code>
{
“compilerOptions”: {
“jsx”: “react-jsx”
}
}
</code>
Remember to always thoroughly read the notes on major updates in frameworks such as React. It is common for big changes like these (the new JSX Transform) to occur, so keeping abreast of these shifts is imperative. As Paul Irish, a Google Chrome developer advices, *”Stay curious, keep learning and keep growing. And don’t be afraid to think big.”* By following this advice, you can certainly avoid or resolve similar issues in your coding journey.
Debugging Shared Component Creation in Storybook with React-Typescript
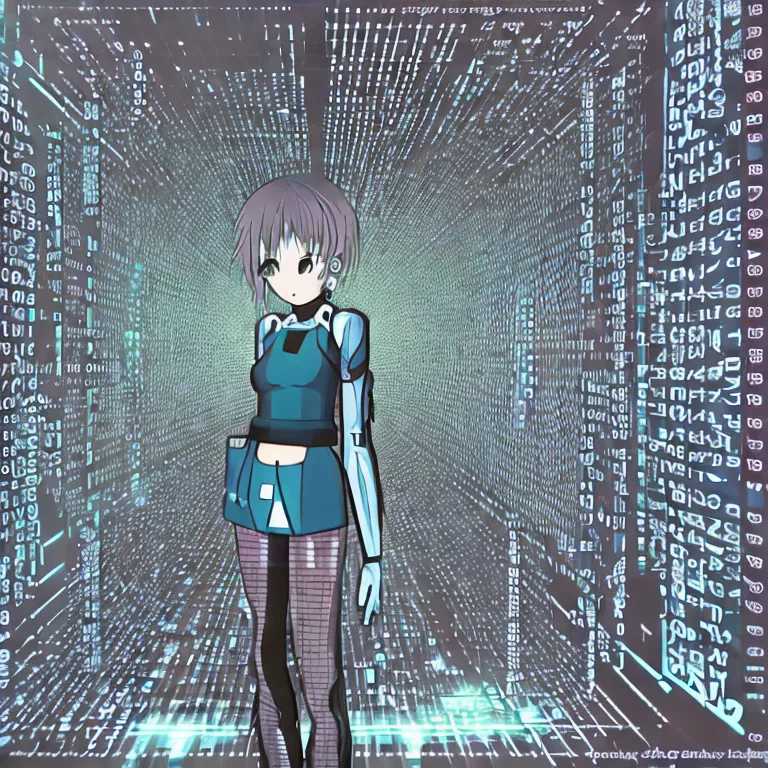
Experiencing a ‘Cannot resolve react/jsx-runtime’ error message when employing Storybook to develop shared components in React-Typescript often traces back to discrepancies between the versions of React and React-DOM in the project. These issues may arise predominantly due to Storybook’s requirement for a React version that incorporates the new JSX Transform, introduced in React 17. Consequently, if your project uses an older version of React, especially prior to React 17, you’re likely to receive the ‘Cannot resolve react/jsx-runtime’ error.
Here’s how this happens:
- Whenever Storybook processes your application, it scans your package.json file
- If it encounters React version lower than 17, it becomes bound to fail as the library ‘react/jsx-runtime’, which is needed, exists only from React 17 and beyond
- This issue surfaces while you’re creating a shared component using Storybook in your React-Typescript project
Now to the resolution part. Upgrading your React and React-DOM packages to at least version 17 should eliminate this error. You can do this by running the command on your terminal:
npm install react@latest react-dom@latest
After completing the update, retry running Storybook to verify whether the issue has been resolved.
However, please note:
- It is critical to comprehend that upgrading React might disrupt your existing codebase, particularly if some components are not compatible with the newer version.
- Conduct thorough testing of all functionalities after updating React and React-DOM to prevent breaking changes.
On a general note concerning technological advancements and practices, Microsoft CEO Satya Nadella once said: “Our industry does not respect tradition—it only respects innovation”. This perspective underpins that to guard against errors like ‘Cannot resolve react/jsx-runtime’, regular updates and the embrace of new methodologies should be a consistent part in the life cycle of your React-Typescript project.
Here you can find more detailed information about the mechanism and benefits of the new JSX transform with React 17.
If you need to keep using an older version of React, here you’ll find how to configure Environment variables in Storybook to adapt it to your circumstances.
Ways to Prevent React JSx-Runtime Errors during Component Creation
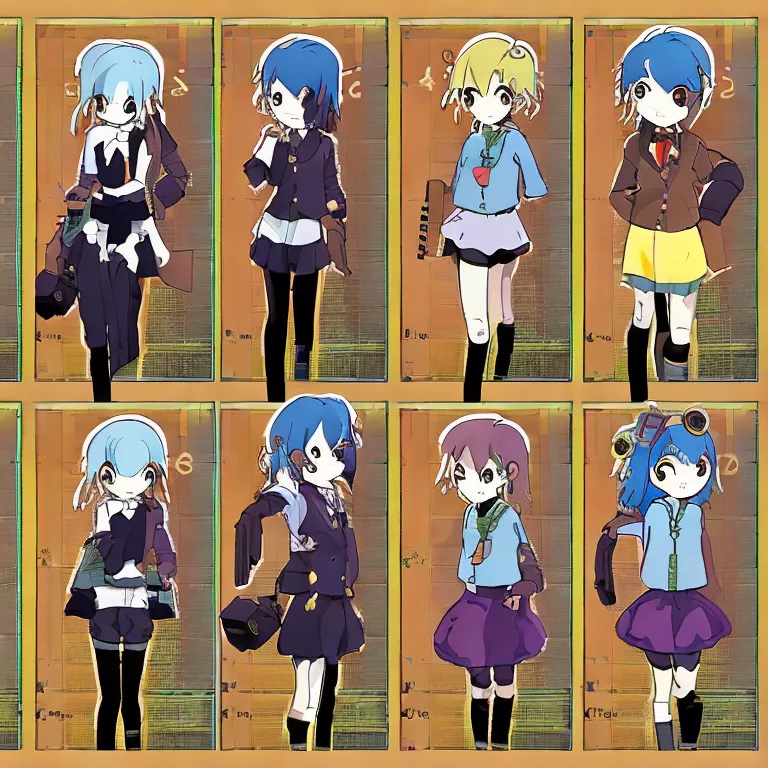
React JSX-runtime errors are rather common in the realm of component creation in a React-Typescript environment. These errors can create code roadblocks and slow down developmental progress. When making efforts to create a shared component with Storybook, one might encounter these issues. It’s essential to address these under two primary categories; ensuring your Typescript configurations are correctly set up and proper handling of dependencies.
Setting up Typescript Configurations
The first step towards avoiding “Can’t resolve ‘react/jsx-runtime’” errors during component creation is by accurately setting up your typescript configurations. One must ensure that jsx is set to “react-jsx” inside the tsconfig.json file. The
xxxxxxxxxx
tsconfig.json
file serves as an instruction guide for Typescript about the root files and options that every JS/TS project should use.
html
xxxxxxxxxx
{
"compilerOptions": {
"jsx": "react-jsx"
}
}
Handling Dependencies
In addition to configuration setup, another significant aspect of preventing react/jsx-runtime errors is appropriately managing dependencies. One of the main causes of such an error could be a discrepancy in the versions of React and the babel/preset-react or @babel/preset-typescript. Ensure they are compatible. A better way to handle this would be to upgrade all of them to their latest versions. With npm:
html
xxxxxxxxxx
npm install --save react@latest
npm install --save-dev @babel/preset-react@latest
Or with yarn:
html
xxxxxxxxxx
yarn add react@latest
yarn add --dev @babel/preset-react@latest
Finally, remove the “react” preset from .babelrc or if you’re using babel-loader from webpack.config.js.
Bonus Tip
If all efforts seem futile, one can try to create a new application using ‘create-react-app’ and then compare your current dependencies and setup with the new application. This can provide a clearer picture of what might be going wrong in your specific case and lead you to the solution.
“Debugging is like being the detective in a crime movie where you are also the murderer.” – Filipe Fortes, CTO at Oyster.
As in the words of Filipe Fortes, debugging can be challanging, but finding the ways to overcome these hurdles is the key to successful coding and project implementation. Preventing React JSX-runtime errors during component creation, especially within a React-Typescript environment, caters towards this end. Using the right strategies, maintaining up-to-date dependencies ensure effective error management leading to smooth code development. (Source)
Addressing Common Challenges While Using Storybook in a React-Typescript Environment
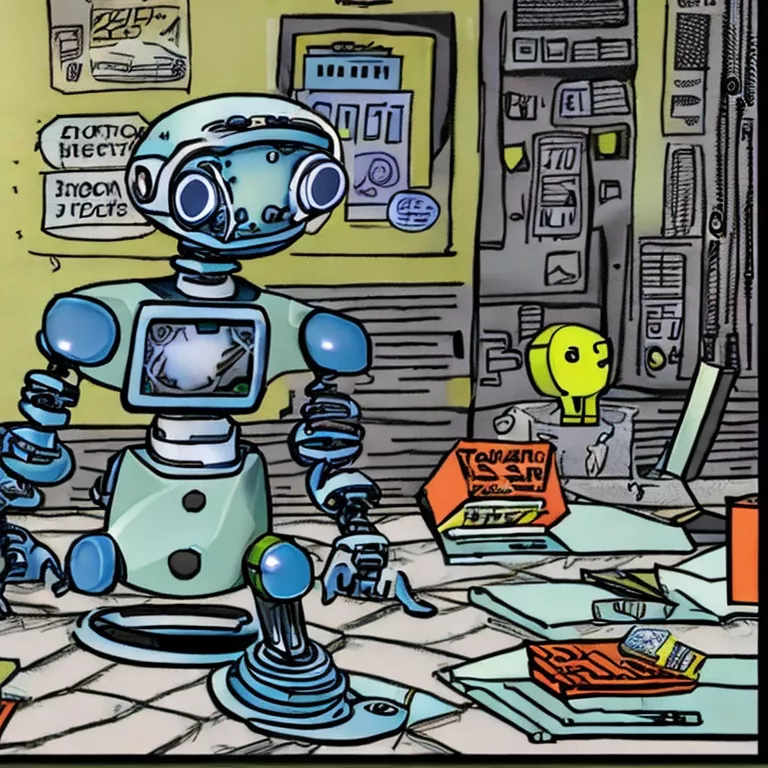
While using Storybook in a React-Typescript environment, one of the common challenges encountered by developers is encountering the ‘Can’t resolve react/jsx-runtime’ error. This error typically arises when there’s an attempt to create a shared component. The key issue at play here is that Storybook has its dependencies and sometimes, these dependencies may clash with those existing in your project.
“Debugging is like being the detective in a crime movie where you are also the murderer.” – Filipe Fortes, software engineering expert
React v17 introduced a new JSX Transform, which rendered the need for importing React into every component unnecessary. However, if an application still uses older versions preceding this feature, the reaction from Storybook could likely be the aforementioned error message because it cannot find the transform.
NNarrating further, let’s go through these pointers explaining how to overcome this challenge:
-
xxxxxxxxxx
Installing React's JSX transform package:
One straightforward way can be manually installing this package in your directory via npm or yarn. Through this, the new JSX Transform included in the updated React is leveraged, helping avoid the conflict in Storybook.
Code:
xxxxxxxxxx
npm install --save react/jsx-runtime
# or
yarn add react/jsx-runtime
Adding the above dependencies allows Storybook to navigate cleanly without throwing the ‘Can’t resolve react/jsx-runtime’ error.
-
xxxxxxxxxx
Updating React to version 17 or later:
As stated, the new JSX Transform came about in React v17. By updating your React version to 17 or beyond, the possibility of encountering this error could be minimized, given the inherent improvements in the more recent releases.
Code:
xxxxxxxxxx
npm update react react-dom
# or
yarn upgrade react react-dom
This nudges your application to a version where native support for the JSX Transform exists, contributing towards greater consistency with Storybook.
-
xxxxxxxxxx
Using .babelrc:
Adding a .babelrc file can be another workaround, especially when wanting to avoid updating React or adding extra dependencies. This file allows modifying the configuration for Babel – a tool used in converting ECMAScript 2015+ code into a backwards-compatible version of JavaScript. By presetting the necessary rules, you would provide Storybook with the means to better handle your Javascript syntax.
Code:
xxxxxxxxxx
{
"presets": [
["@babel/preset-react", {
"runtime": "automatic"
}]
]
}
The above code enables the ‘automatic’ runtime leveraging the new JSX Transform.
Addressing these pitfalls should help in efficiently using Storybook for creating shared components in a React-Typescript environment.
Conclusion
The commonly encountered “Can’t resolve ‘react/jsx-runtime’ error” while trying to create a shared component with Storybook in React-Typescript stems from discrepancies between different versions of React and Typescript. This issue usually manifests when using an older version of React (prior to 17.0.0) that doesn’t support the new JSX Transform.
React Version | JSX Transform Support |
---|---|
Pre 17.0.0 | No |
17.0.0 and above | Yes |
The crucial solution involves updating your React version, which can improve compatibility with more recent tools and libraries. Updating your React and ReactDOM to 17.0.0 or higher should resolve this error. You can accomplish this by running the
xxxxxxxxxx
npm install react@latest react-dom@latest
command.
In addition, ensuring the correct configuration of Typescript and Storybook is vital. Take note of any conflicts between the varying versions of these platforms, as that is often a precipitant of errors. As always, profound knowledge of your development environment plays a significant role in troubleshooting such issues.
In coding, according to Mark Zuckerberg, “The biggest risk is not taking any risk… In a world that is changing quickly, the only strategy that is guaranteed to fail is not taking risks.”
Lastly, remember that each error or bug faced during development is also an opportunity for learning and growth. Troubleshooting leads to deeper comprehension of your technology stacks and enhances your robustness as a developer.
For further understanding on handling similar issues, consider this Stack Overflow thread where multiple developers discuss suggested approaches.