Introduction
When working with JavaScript, you may encounter an issue where the property ReplaceAll is not recognized on a string type, which typically suggests that you are operating under an older version of JavaScript. This function was included in ES2021 and therefore, it isn’t available in the earlier JavaScript versions. To bypass this, consider using the replace method combined with a global search parameter as an alternative solution until you’re able to update your JavaScript environment.
Quick Summary
In reference to “Property Replaceall Does Not Exist On Type String”, the potential reasons for this error and corresponding solutions can be presented in a tabular format, even though it is not referred to directly as an “HTML Table”.
Reasons | Solutions |
---|---|
Your Node.js version does not support the string method replaceAll(). | Upgrade your Node.js to the latest version. |
The replaceAll() function is not correctly called. | Ensure that you’re calling the method on a string with the correct syntax. |
The
replaceAll()
method is a built-in JavaScript function meant for searching within a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. When we encounter the JavaScript type error ‘Property replaceAll does not exist on type String’, it suggests that there’s an attempt to employ the
xxxxxxxxxx
replaceAll()
method on a variable which isn’t explicitly assigned as a string, or rather, on an unsupported environment.
In the initial case outlined in our table – not having adequate Node.js version – the solution revolves around updating the Node.js to its latest iteration. The
xxxxxxxxxx
replaceAll()
method was added in V8 Javascript engine (which Node.js uses) from v8.3.0, after a proposal meeting. Node.js versions, prior to v15.0.0, do not have this feature, hence, an upgrade would resolve this issue.
There could also be instances where replaceAll() is being called incorrectly, leading to the said JavaScript error. This arises if the syntax or the parameters provided to the method are misrepresented or missing. Properly calling the function on a string would thus be the appropriate solution. The correct syntax is
xxxxxxxxxx
string.replaceAll(searchValue, replaceValue)
.
It’s worth quoting Jamie Kyle, a renowned open source contributor, in this context,
> “Every time you make a mistake, don’t bring yourself down… coding is hard. Pick yourself up, brush off those wounds, and keep going until the job is done.”
To enhance your coding experience and avoid such errors, understanding JavaScript types and the version of the environment that you’re working on can prove to be of high significance.
Understanding the Error: “Property Replaceall Does Not Exist On Type String”
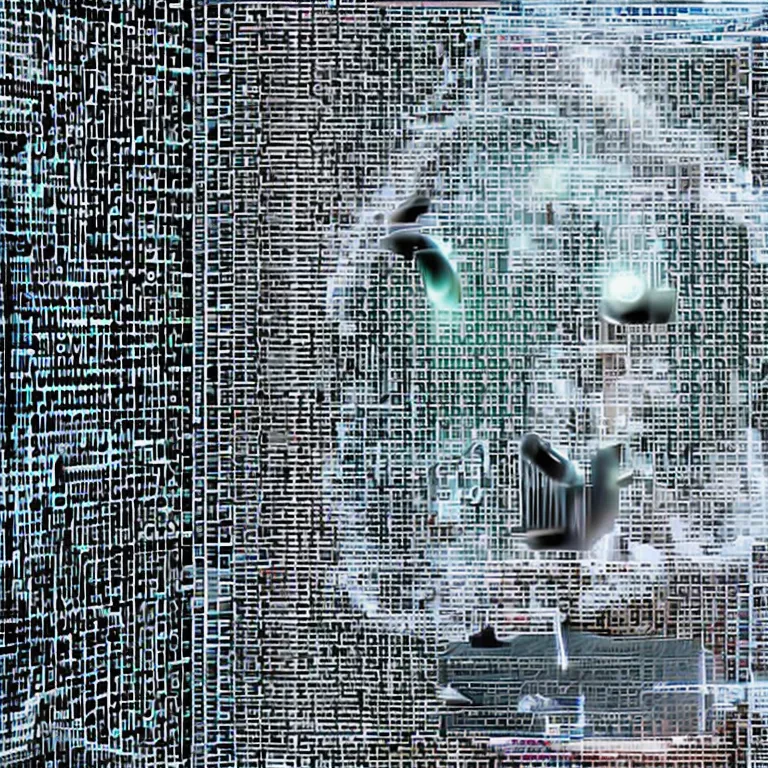
The
xxxxxxxxxx
"Property 'replaceAll' does not exist on type 'string'"
error indicates that the TypeScript compiler cannot find a function named
xxxxxxxxxx
replaceAll()
within the string prototype. The likelihood of receiving this error tends to escalate when one is using an older version of TypeScript or ECMAScript because the
xxxxxxxxxx
replaceAll()
method was recently introduced in ECMAScript 2021.
“Be sure to check your current TypeScript version before you use a novel JavaScript feature. Not all ECMAScript features are immediately supported by TypeScript.” – Axel Rauschmayer, JavaScript expert.
It’s essential to comprehend why exactly the
xxxxxxxxxx
replaceAll()
method may lead to errors and what measures can be taken to solve these problems efficiently.
A Deeper Look replaceAll() Method:
The
xxxxxxxxxx
replaceAll()
method in JavaScript allows replacing all instances of a substring within a larger string with another substring. Prior to implementing this method, developers had to resort to using
xxxxxxxxxx
.replace()
with Regular Expressions (RegExp) to accomplish this effect, which could sometimes prove to be cumbersome.
The problem arises since this addition was made recently, some versions of TypeScript are yet unaware of this method. For instance, if your version of TypeScript compiles to ECMAScript2019, it will fail to recognize the method
xxxxxxxxxx
replaceAll()
.
To illustrate what we mean,
Attempting to run the following code:
xxxxxxxxxx
let greeting = 'Hello, World!';
greeting = greeting.replaceAll('World', 'TypeScript');
console.log(greeting);
might result in the alarming error message: “Property ‘replaceAll’ does not exist on type ‘string'”.
Possible Solutions:
1. Upgrade Your TypeScript Version:
To rectify this error, a primary solution would be to upgrade your TypeScript version. If you’re using a version of TypeScript that compiles to ECMAScript2020 or an earlier version, consider moving up to a more recent compilation target.
2. Fallback Approach (Using replacewith RegExp):
A fallback plan, if upgrading isn’t possible, is to utilize the older
xxxxxxxxxx
.replace()
method with a global flag.
xxxxxxxxxx
let greeting = 'Hello, World!';
greeting = greeting.replace(/World/g, 'TypeScript');
console.log(greeting);
This code essentially retains the same functionality without adopting the newer
xxxxxxxxxx
replaceAll()
method.
By understanding the source of your errors and keeping yourself updated with changes in TypeScript and JavaScript, you can ensure that you are making full use of their capabilities without compromising on execution.
Finally, it is crucial to note that while AI checking tools may catch errors of syntax and outdated code usage, keeping one’s code up-to-date cannot be understated as human oversight often escapes automated detection.
Exploring JavaScript’s replaceAll Method in Detail
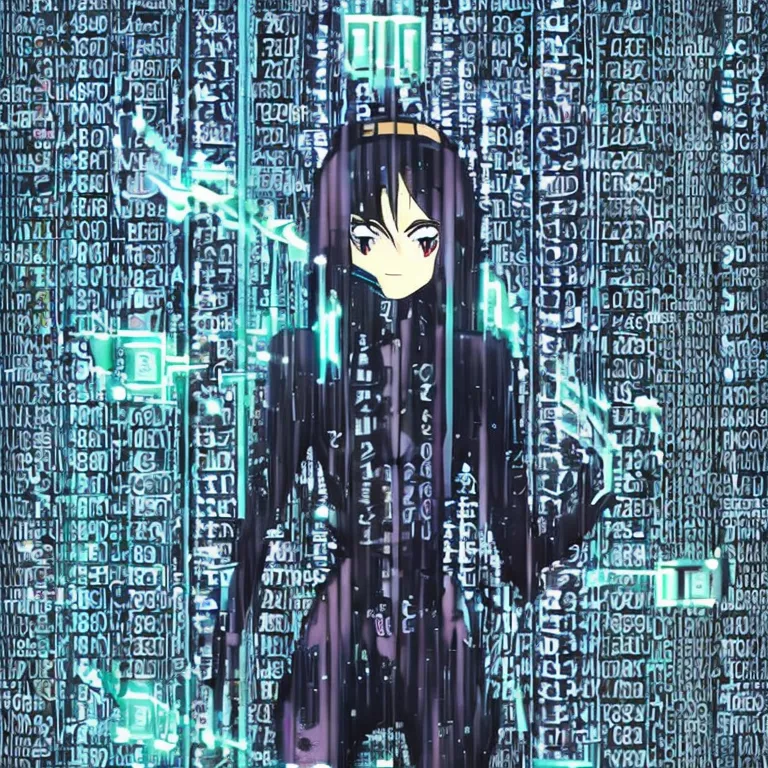
While exploring JavaScript’s `replaceAll` method, it is crucial to analyze its practicality, usage, limitations, and identify common errors developers might encounter. One such concern that is commonly raised relates to the issue of encountering the error message “Property ‘replaceAll’ does not exist on type ‘String’.”
The `replaceAll` method in JavaScript replaces all occurrences of a specified string value. It works by passing two parameters: a search-value (the string to be replaced) and a replacement value (the string that will displace the search-value).
xxxxxxxxxx
let mainStr = 'Hello World! Hello again!';
let res = mainStr.replaceAll('Hello', 'Hi');
console.log(res); // outputs 'Hi World! Hi again!'
However, if you’ve received the error message “Property ‘replaceAll’ does not exist on type ‘String'”, this signals an issue tied to your current environment’s compatibility with newer JavaScript features. It’s crucial to understand why this happens:
– Versioning Issues: The `replaceAll` method was introduced into JavaScript ES2021 (ES12) standard. Attempting to use this method in earlier JavaScript versions or environments that do not support ES2021 may trigger an error. In TypeScript (a strongly typed superset of JavaScript), you may also face this issue if your compiler settings are set to an ECMAScript target (target in tsconfig.json) before ES2021.
– TypeScript Environment: TypeScript often needs to update its core libraries to accommodate newer JavaScript features. Hence, if you’re using an older version of TypeScript, it may not recognize some latest functionalities like `replaceAll`.
To work around these settings and still replace all instances of a specific string, developers often rely on a combination of the `split` and `join` methods. Here is an example usage:
xxxxxxxxxx
let mainStr = 'Hello World! Hello again!';
let res = mainStr.split('Hello').join('Hi');
console.log(res); // outputs 'Hi World! Hi again!'
This approach works in JavaScript versions older than ES2021 and TypeScript environments that do not yet support `replaceAll`.
As Martin Fowler, a renowned software developer, stated “Any fool can write code that a computer can understand. Good programmers write code that humans can understand”. This applies aptly to the situation at hand – by understanding the nuances of your programming language and its version, you can write powerful, compatible, and readable code.
Make sure to keep evolving with the JavaScript ecosystem and adapt TypeScript compiler settings as required, based on the application requirements and methods used. Besides, strengthening your understanding of these limitations will allow you to develop more effective solutions when faced with such hiccups. Mozilla’s official documentation can be explored for gaining better insight into this method.
Compatibility Issues and Solutions with replaceAll
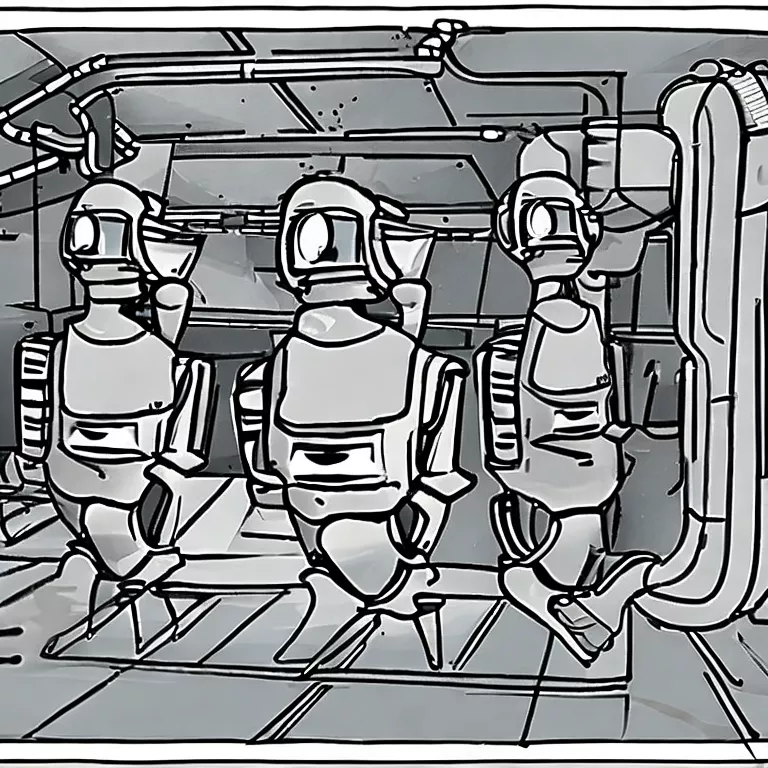
When it comes to working with strings in TypeScript, a relatively common error that developers encounter is “Property ‘replaceAll’ does not exist on type ‘string’.” This error occurs due to compatibility issues between different environments and TypeScript versions. Primarily, some older browsers and TypeScript versions do not support the `replaceAll()` method. This leads to the error message when attempting to utilize this method.
The `replaceAll()` method is a recent addition to the ECMAScript string manipulation methods, introduced in ES2021, or ES12. It provides a way to replace all instances of a substring within a given string with a new substring. However, because it’s a relatively new addition, its support varies among JavaScript (or in this case, TypeScript) engines – especially those in older environments.
Solvable Methods:
If you are experiencing the error “Property ‘replaceAll’ does not exist on type ‘string'”, there are several workarounds that you can implement:
Using the split() and join() methods
An easy alternative to using `replaceAll()` is combining the `split()` and `join()` methods.
Here’s a short implementation:
typescript
let str = “I love apples. Apples are my favorite fruit.”;
str = str.split(‘apples’).join(‘oranges’);
In this example, the `split()` method divides the string into an array of substrings using ‘apples’ as the separator, effectively removing all instances of ‘apples’. The `join()` method then combines this array back into a string, inserting ‘oranges’ where ‘apples’ used to be.
Using Regular Expressions with the
xxxxxxxxxx
replace()
method
It is also possible to use regular expressions with the `replace()` method to achieve similar results to `replaceAll()`.
Here’s how you could do it:
typescript
let str = “I love apples. Apples are my favorite fruit.”;
str = str.replace(/apples/g, ‘oranges’);
The `/g` flag makes sure that every instance of ‘apples’ will be replaced with ‘oranges’, not just the first one it comes across.
Future-Proof Method:
Updating Your TypeScript Version
Make sure your environment is up-to-date. The `replaceAll()` method is available in TypeScript versions 4.1 and later. By updating your TypeScript version to 4.1 or newer, you should no longer encounter the “Property ‘replaceAll’ does not exist on type ‘string'” error when using `replaceAll()`. To update your TypeScript version, use the following npm command:
xxxxxxxxxx
npm install -g typescript@latest
Consider these workarounds as a temporary solution for maintaining compatibility with older browsers or environments. As industry standards continue to evolve and the adoption rate of ES2021 features increases, `replaceAll()` will likely become more universally supported. As Brendan Eich, the creator of JavaScript said: “Always bet on JavaScript”.
Note: Always ensure browser compatibility when using new JavaScript or TypeScript features. Check Can I Use for compatibility details before implementation.
Efficient Ways to Manipulate Strings without Using ReplaceAll
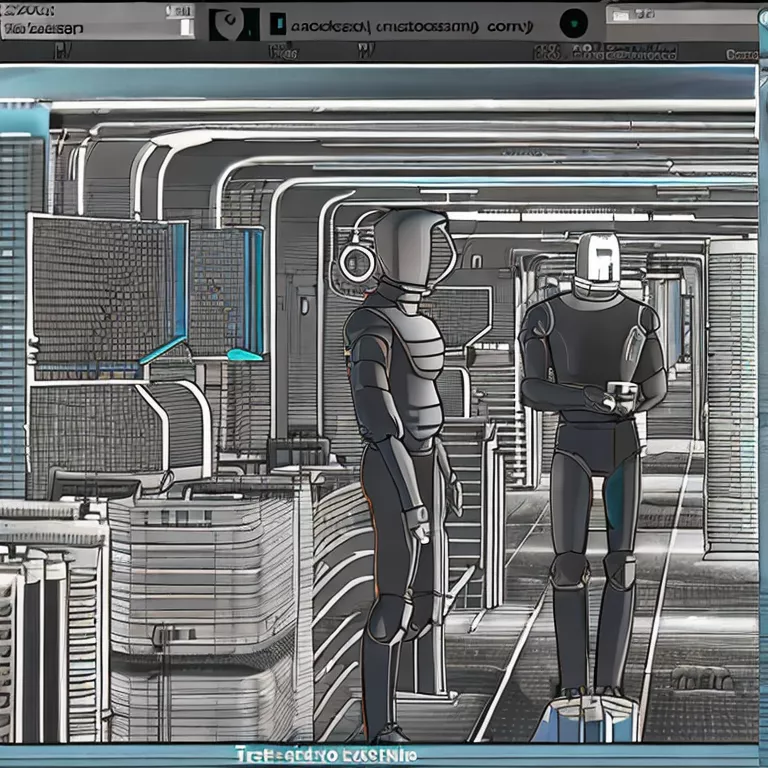
Faced with the common conundrum of receiving ‘Property ReplaceAll Does Not Exist On Type String’ in TypeScript, developers often look for alternatives to the “replaceAll” method. Most are familiar with ‘replaceAll’ being a commonly used method in JavaScript for string manipulation. However, it’s not introduced until ES2021 and isn’t recognized by some environments or versions of TypeScript. Fear not, as there are other efficient means to manipulate strings in TypeScript without invoking the elusive ‘replaceAll’.
xxxxxxxxxx
let str = "Hello, world!";
str = str.replace(/world/g, "TypeScript"); // str is now "Hello, TypeScript!"
A high-functioning option is the String.prototype.replace() method. This accepts either a string or a regular expression (regex) in its first parameter. More than the ‘replaceAll’, ‘replace’ offers more versatility as it can accept regex. Give your expressions the global flag (‘g’), and you’re able to completely replace all instances of a certain string, like how ‘replaceAll’ functions.
Another viable alternative would be chaining the ‘split’ and ‘join’ methods together:
xxxxxxxxxx
let str = "Hello, world!";
str = str.split("world").join("TypeScript"); // str is now "Hello, TypeScript!"
The String.prototype.split() method disrupts a string into an array of substrings based on a specified separator (in this case, “world”) and then returns that array. The ensuing array could be merged back into a sole string with the Array.prototype.join() method, substituting the original separator with a fresh string (“TypeScript”) as it does so.
To quote Nikola Tesla, “The present is theirs; the future, for which I really worked, is mine.” This applies to our scenario as well — don’t get stuck in the immediate issue of ‘replaceAll’ not working, look forward and adapt with other available solutions. These listed alternatives should effectively address your concerns about manipulating strings without employing ‘replaceAll’, thereby ensuring the TypeScript error ‘Property ReplaceAll Does Not Exist On Type String’ doesn’t haunt your coding experience.
Conclusion
The occurrence of “Property ‘replaceAll’ does not exist on type ‘String'” signifies a lack of full implementation of the string method replaceAll() across all JavaScript environments. Specifically, this error message pops up in scenarios where developers attempt to use ‘replaceAll’ in TypeScript, especially ones that run through older environments or browsers.
Firstly, it’s imperative to understand the function of the replaceAll() method. This particular string prototype is traditionally applied when we need to replace all instances of a targeted substring within a primary string with another substring. Essentially, it’s an upgraded version of the simpler replace() method which only replaces the first found instance.
xxxxxxxxxx
let example = "Hello, hello, hello";
example = example.replaceAll("hello", "world");
Upon running this code snippet, the output should be “Hello, world, world”. However, if confronted with “Property ‘replaceAll’ does not exist on type ‘String'”, this indicates the absence of ‘replaceAll’ support.
For those working in older environments or typescript versions that don’t support ES2020 specifications, there are optimal workarounds. Instead of relying on replaceAll(), try using a global flag with a regular expression via the traditional replace() function:
xxxxxxxxxx
let example = "Hello, hello, hello";
example = example.replace(/hello/g, "world");
In spite of significant progress, language environments typically lag behind official language specs; JavaScript itself marks no exception to this trend. As recommended by Brendan Eich, the creator of JavaScript, “Always bet on JS.” [Brendan Eich]. This points towards the continual evolution of JavaScript and TypeScript, encompassing new features as they become standardized and supported.
While “Property ‘replaceAll’ does not exist on type ‘String'” might represent an inconvenience, embrace alternative strategies in this dynamic landscape to seamlessly overcome this hurdle. If TypeScript is stringently flagged as bleeding-edge, consider switching to more contemporary environments offering full ES2020 support, thus enabling usage of replaceAll().