Introduction
When building TypeScript, moving non-TS files to Dist is an integral process that requires a systematic approach. To do this efficiently: first, use ‘gulp’ or ‘webpack’, tools known for their nifty ability to handle file transfers dynamically during creation procedures; secondly, ensure your configuration is set up correctly to automate the copying of non-TypicalScript (non-TS) files into your Distribution (Dist) directory, thereby enhancing the effectiveness of the build process.
Quick Summary
With TypeScript, the process of building often involves generating a
dist
folder where all compiled JavaScript files reside. This folder contains the very essence of an application’s functionality after going through transpilation from TypeScript to JavaScript. However, non-TypeScript (non-TS) files, such as JSON or image files, are typically not included in this process. Therefore, we have to manually copy these non-TS files into the dist folder during or after the build process.
Task | Explanation |
---|---|
xxxxxxxxxx Adding file-copy scripts in package.json |
For copying non-TS files manually, consider adding a script in
xxxxxxxxxx package.json file which uses an npm package like xxxxxxxxxx "cpx" or shell commands like xxxxxxxxxx cp (UNIX)/ xxxxxxxxxx copy (Windows). |
xxxxxxxxxx Usage of gulp |
Gulp, a task runner, could be used to handle file operations and integrate it with TypeScript’s compile flow. It needs a gulpfile to specify tasks. |
xxxxxxxxxx Pipeline methodology using Webpack/Parcel/Rollup |
Webpack, Parcel, Rollup – all these support copying static files/folders directly to the build directory. |
The first strategy outlined is to use an npm package like
xxxxxxxxxx
cpx
or operating system-specific commands such as
xxxxxxxxxx
cp
for UNIX or
xxxxxxxxxx
copy
for Windows. Here, we manually add a “file-copy” script in the
xxxxxxxxxx
package.json
file that copies the non-TS files to the target directory. Here is a reference to cpx on npmjs.com.
xxxxxxxxxx
"scripts": {
"copy-files": "cpx \"src/**/*.{json,jpg,png}\" dist"
}
The second strategy involves using much celebrated task runners like Gulp for handling file operations and integrating them with TypeScript’s compile flow. A gulpfile specifies tasks, defining a `copy` task would tell Gulp to match source files’ patterns and pipe them to the destination directory.
xxxxxxxxxx
gulp.task('copy', function () {
return gulp.src('./src/**/*.json')
.pipe(gulp.dest('./dist'));
});
Lastly, we have bundlers/module loaders like Webpack, Parcel, or Rollup. These tools include extended capabilities such as copying static files/folders directly to the build directory by utilizing plugins like `copy-webpack-plugin` for Webpack, which takes care of copying over non-TS files during the bundling process.
xxxxxxxxxx
new CopyWebpackPlugin([
{from:'src/images',to:'images'}
]),
“As operations grow more complex, they tend to hide some really useful features in their tool belts.” – Addy Osmani (Engineering Manager at Google), underlining the power of development tools like build scripts and task runners.
Understanding the Process of Building TypeScript
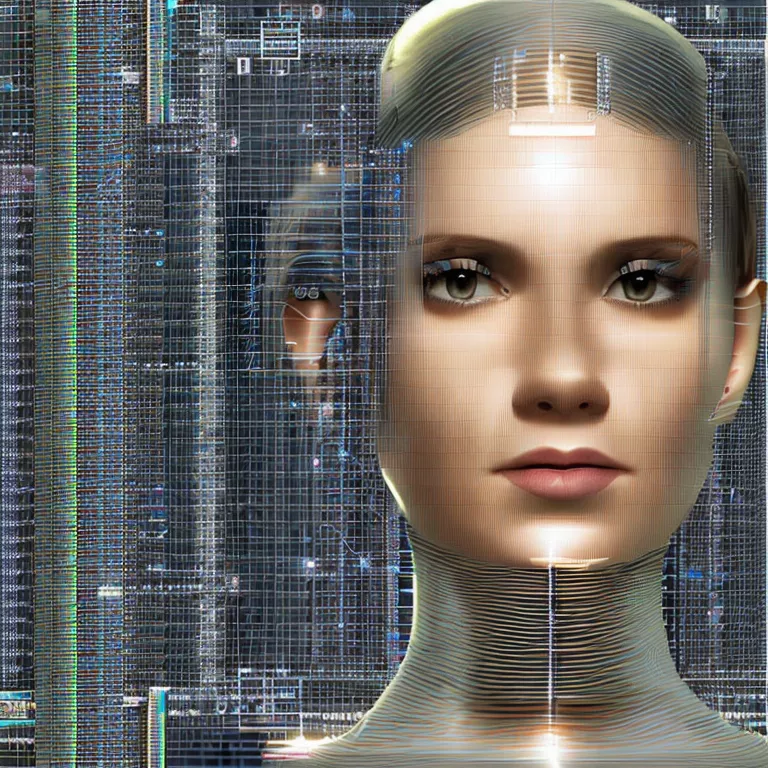
Building a TypeScript project involves a number of steps and considerations. As a developer, it’s crucial to appreciate not just the construction of .ts files but also their non-TypeScript counterparts. The proceedings will primarily focus on how you can seamlessly integrate the process of copying non-TypeScript (.json, .html, etc) files during your build process to the dist/ directory.
When writing real-world applications with TypeScript, you are not limited to working only with .ts files. There may be many supporting files such as JSON configurations, HTML templates, or other raw data files that don’t get transpiled by TypeScript compiler (tsc). To make sure these files get into the distribution or output folder alongside the transpiled JavaScript files, you have to employ a method to copy them.
At the heart of the solution for copying non-TypeScript files when building TypeScript lies the useful npm package: copyfiles. You would utilize this package within your scripts section in your package.json file in order to automate the process of copying such files during TypeScript build.
To illustrate this let’s consider a scenario where you need to include a configuration file (config.json) in your built assets:
javascript
// First, we install the “copyfiles” package
npm install –save-dev copyfiles
Then, you designate a script in your package.json file:
xxxxxxxxxx
"scripts": {
"prebuild": "copyfiles -u 1 src/*.json dist",
"build": "tsc"
}
The prebuild script here ensures that all JSON files at the root of your src/ directory are copied into the dist/ directory as part of the build process.
Never forget what James Gosling, the father of Java said – “One of the interesting things about the computer science field is that we’re constantly looking for new things to learn.” Whenever you are building TypeScript applications, remember to consider how to handle non-TS files for a smooth and uninterrupted development experience.
Copying Non-TS Files during TypeScript Compilation: An Insight
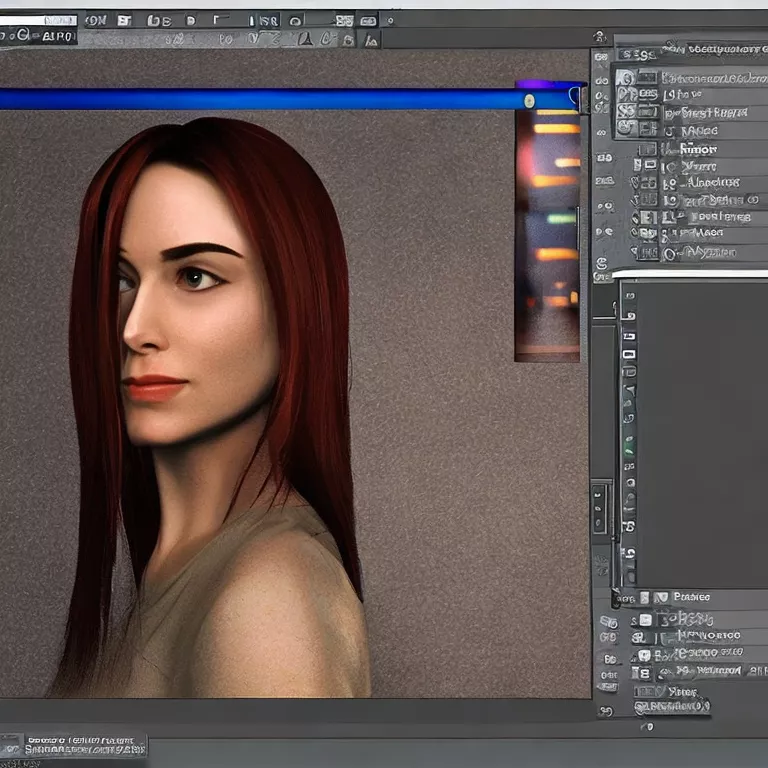
When building TypeScript compilations, one common challenge encountered by developers involves the process of copying non-TS files into the distribution directory (dist). These files could range from static assets like pictures and JSON files to configuration files or even HTML templates. By default, the TypeScript compiler (“tsc”) only processes .ts files, but in practice, a real-world application will often require more than just TypeScript.
Unfortunately, the TypeScript Compiler does not inherently provide for this functionality. The “outDir” option tells the compiler where to place the generated JavaScript files, but files other than that are not considered. Nevertheless, there are practical workarounds that can be utilized to address this issue:
Copy-webpack-plugin:
This solution hinges on using Copy-webpack-plugin along with Webpack, a popular module bundler, and task runner. Webpack has out-of-the-box TypeScript support and Copy-webpack-plugin allows you to copy individual non-TS files or entire directories to the build directory.
Example:
xxxxxxxxxx
const CopyWebpackPlugin = require('copy-webpack-plugin');
module.exports = {
entry: './src/index.ts',
plugins: [
new CopyWebpackPlugin({
patterns:[
{from:'source', to:'dest'}
]
})
]
};
Gulp task runne/r:
Gulp is another powerful alternative focused on handling such tasks. It is an open-source JavaScript toolkit used as a streaming build system to automate repetitive tasks like modifying files.
An example of how Gulp could be used to copy non-TS files during TypeScript compilation:
xxxxxxxxxx
var gulp = require('gulp');
gulp.task('copy-files', function() {
gulp.src(['./source/**/*', '!./source/**/*.ts'])
.pipe(gulp.dest('./dist'));
});
Npm script:
One can also utilize an npm script to copy these non-TS files. This crude method is applicable in situations where installing and setting up another tool might seem excessive.
Example:
xxxxxxxxxx
"scripts": {
"build": "tsc && cp src/*.json dist"
}
Each of the above-mentioned approaches has its advantages and disadvantages, as well as differing levels of complacency with various project scenarios. Therefore, it’s important to gauge which approach fits your project requirements best before employing them.
“There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies. The first method is far more difficult.” – C.A.R Hoare
Proper Techniques for Transferring Non-Ts files to Dist During Build In TypeScript
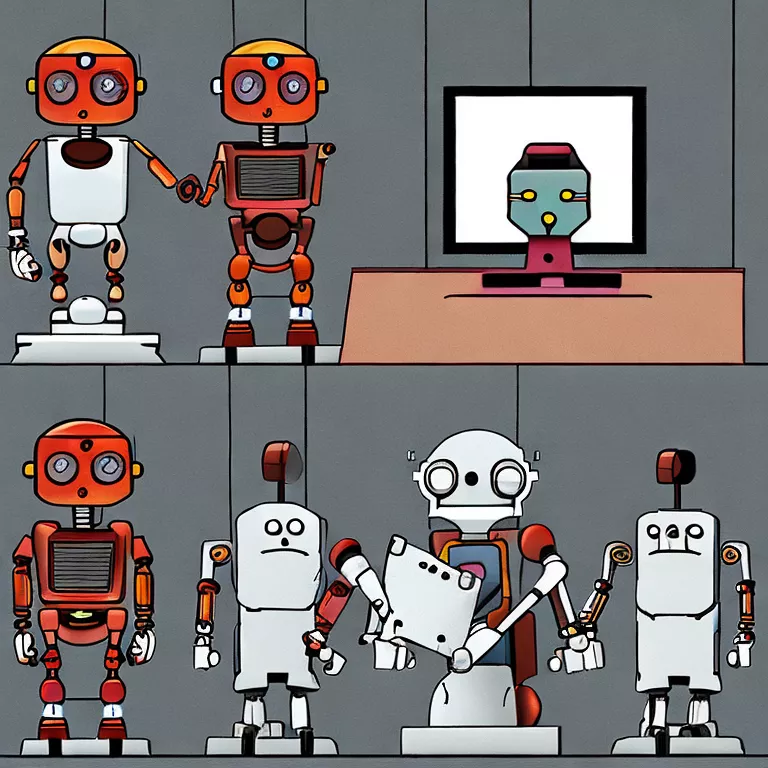
When developing Typescript applications, a common need is to transfer non-TS files (like JSON, images, etc.) to the distribution folder (`dist`) during build. The challenge, however, lies in the ability of the TypeScript compiler (`tsc`), to only process and transpose TypeScript files (.ts) into JavaScript files, leaving out non-ts assets.
For creation of production-ready applications, seamlessly transferring such non-TS files becomes essential. Let’s delve into techniques that help achieve this.
Manual Copy
During development, copying these non-TS files manually each time you compile may seem viable, but it is not recommended for larger projects or continuous deployments due to the complexity and consistency issues.
Example, consider there is a file named `config.json`.
You can manually copy this file from `src` directory to `dist` directory.
xxxxxxxxxx
cp src/config.json dist
Consider implementing an automated approach if files are numerous or frequently updated.
Utilizing npm scripts
npm scripts provide a platform-independent approach towards file management during builds. By introducing the `copyfiles` package, one can move non-ts files conveniently in a cross-platform manner on `prebuild` or `postbuild`.
This is how the “scripts” part of your package.json might look:
xxxxxxxxxx
{
"scripts": {
"build": "tsc",
"prebuild": "copyfiles -u 1 src/**/*.json dist"
}
}
Here, `copyfiles -u 1 src/**/*.json dist` will copy all .json files found within src to the dist directory while maintaining the original directory structure.
Using Gulp
Gulp is a powerful tool that automates time-consuming tasks in your development workflow. It can be used alongside TypeScript to transfer non-TS files during build.
Example, a typical setup in `gulpfile.js` will look like:
xxxxxxxxxx
const gulp = require('gulp');
const ts = require('gulp-typescript');
gulp.task('scripts', function () {
const tsResult = gulp.src('src/**/*.ts')
.pipe(ts({outDir: 'dist'}));
return tsResult.js.pipe(gulp.dest('dist'));
});
gulp.task('assets', function() {
return gulp.src('src/**/*.json')
.pipe(gulp.dest('dist'));
});
gulp.task('default', gulp.series('scripts', 'assets'));
Here, the `scripts` task handles the transposition of Typescript files, while the `assets` task copies all JSON files.
Do choose an approach that aligns best with your project’s requirements and consistency. It can often be a balancing act between simplicity and flexibility.
Transferring non-TS files at build time is crucial for TypeScript projects. As Tony Hoare states, “There are two ways to perform software design: one way is to make it so simple that there are obviously no deficiencies; another is make it so complicated that you can’t see the deficiencies”. Let’s work on achieving the former – simple yet effective solutions for complex problems.[source]
Best Practices and Approach when Copying Non-TS Files During Typescript Building
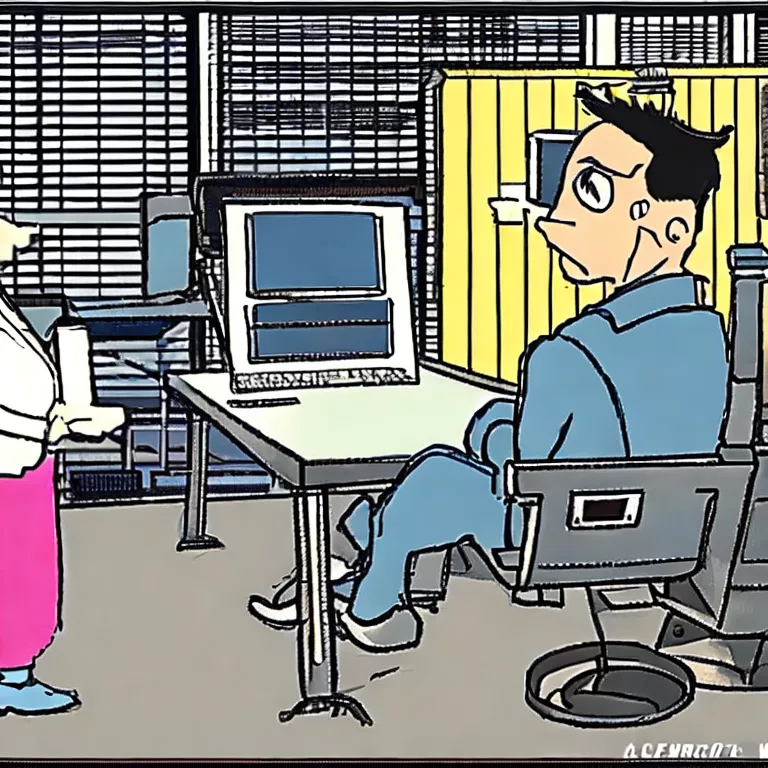
When working with TypeScript, it’s important to have an efficient and effective approach to copying non-TS files during the build process. These files could include images, JSON configuration files, or even text documents, all of which may be crucial to the operation of your application. Here are some key practices to keep in mind:
1. Using a Build Tool:
A common method used by developers is leveraging build tools, like Webpack. It’s widely adopted in the community due to its extensive capabilities including copying static files from one location to another. For instance, you can utilize the ‘copy-webpack-plugin’. An example of how to configure Webpack to do so is:
xxxxxxxxxx
// webpack.config.js
const CopyWebpackPlugin = require('copy-webpack-plugin')
module.exports = {
// ... other configurations
plugins: [
new CopyWebpackPlugin({
patterns: [{from: 'src/assets', to: 'dist/assets'}],
}),
],
}
This notifies Webpack to copy everything within the ‘src/assets’ directory into ‘dist/assets’ directory whenever a build is initiated.
2. Npm Scripts:
If you prefer using npm scripts over build tools, consider incorporating ‘shx’ or ‘cpx’ npm packages. They provide cross-platform Unix shell commands for Node.js respectively. In this way, you can seamlessly copy non-TS files as part of your build process. A snippet would look like:
xxxxxxxxxx
{
"scripts": {
"copy": "shx cp -r src/assets/. dist/assets",
"build": "tsc && npm run copy"
}
}
This technique works by initially running the TypeScript compiler to generate JavaScript code followed by executing the copy script.
3. Typescript 2.0+ :
If you are working with TypeScript 2.0 or above, using ‘include’ and ‘exclude’ in your tsconfig.json file can help simplify the process. Unfortunately, this method doesn’t directly copy non-TS files to the ‘dist’ during build but includes them in the compilation context meaning they won’t trigger a compilation error:
xxxxxxxxxx
{
"include": ["src/**/*.ts", "src/assets/**/*"],
"exclude": ["node_modules"],
}
Jeff Whelpley, a renowned developer and contributor to the Angular community once said, “The smaller the dot, the bigger the problem,” hinting at the importance of paying attention to these seemingly minute details. While deploying applications manually or automating through CI/CD systems, ensure that all relevant non-typescript files are also appropriately included so that the application runs without hitches after deployment.
In essence, the best practice resonates with aligning your build methods according to the requirements of the project framework, the size and complexity of the project, and your team’s expertise with these tools.
Conclusion
The process of copying non-Ts files to dist when building TypeScript, is integral for a range of development tasks. We delved into the core considerations:
– Appreciating that TypeScript transpiler by default only works with .ts (TypeScript) files, and not .json or any other non-TypeScript files. This constitutes a challenge when there is a requirement to bring along other file formats in the build step.
– Recognizing that there are several reliable techniques to address this challenge. These methods include manual copying, using ‘tsconfig’ properties, or employing external tools such as webpack or gulp.
To demonstrate one particular approach, we discussed the use of Gulp – a toolkit to automate and enhance your workflow:
xxxxxxxxxx
const gulp = require('gulp');
gulp.task('copy-files', function(){
return gulp.src(['src/**/*.json']).pipe(gulp.dest('dist/'));
});
As per the code above, it simply identifies all .json files under the src directory and makes copies of them into the destination (‘dist’) folder.
Sustaining the understanding of these processes will both ensure more resilient and sturdy builds along with fostering increased fluidity for often arduous development sequences. “The act of coding is a conversation between thoughts and results,” stated Turing Award recipient Alan Kay; never is that more evident than when navigating the intricacies of incorporating non-Ts files into the TypeScript’s build procedures. The willingness to tap into the versatility tools like Gulp provide can significantly streamline routine operations.