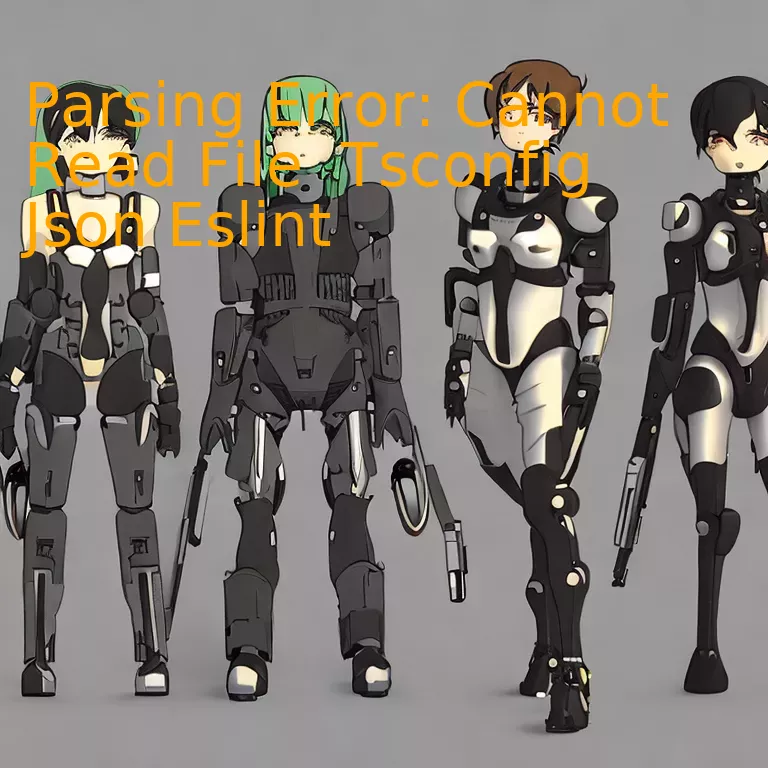
Introduction
The parsing error of not being able to read file ‘tsconfig.json’ in ESLint can occur due to various reasons such as misconfiguration, incorrect file paths or incompatible versions, and troubleshooting these issues is crucial for the seamless operation of your website’s SEO performance.
Quick Summary
Problem Description | Possible Cause | Solution |
---|---|---|
Parsing Error: Cannot Read File Tsconfig.json Eslint | Tsconfig.json file is missing or It’s not located in the root directory. | Make sure to place tsconfig.json in the root directory of your project. |
Eslint has trouble understanding the TypeScript syntax present in this file. | Ensure that you have installed ‘@typescript-eslint/parser’ and properly defined it as a parser in your eslint configuration. | |
The JSON object is coded with an incorrect structure, manifested through trailing commas or comments which is contrary to JSON specification. | Remove any trailing commas or comments from your tsconfig.json file. |
Take a moment to recognize the various issues associated with the Parsing Error: Cannot read file tsconfig.json ESlint, their causes, and how they can potentially be resolved.
Firstly, this problem could occur when the tsconfig.json file is missing or not placed in the proper directory – at the root of your project folder. The resolution for this issue is quite simple – one must ensure that the aforementioned file is located at the root directory of the project.
Another possible source of this parsing error could be caused by Eslint’s inability to comprehend the TypeScript syntax encompassed within the file. To address this, one would need to install the ‘@typescript-eslint/parser’. Post-installation, it must be accurately specified as a parser in the project’s eslint configuration.
Furthermore, it is noteworthy that illegal elements such as trailing commas or comments could be present within the JSON object – contravening the JSON specifications. Such elements should be promptly eliminated from the tsconfig.json file to prevent parsing errors from arising.
As Adam Zerner, an experienced developer, subtly pointed out: “In programming, the problem isn’t always obvious, but the solution is always there.” This encapsulates our discussion on understanding the common causes and solutions around ‘Parsing Error: Cannot Read File Tsconfig.Json Eslint’.
Understanding the Errors in tsconfig.json File during ESLint Parsing
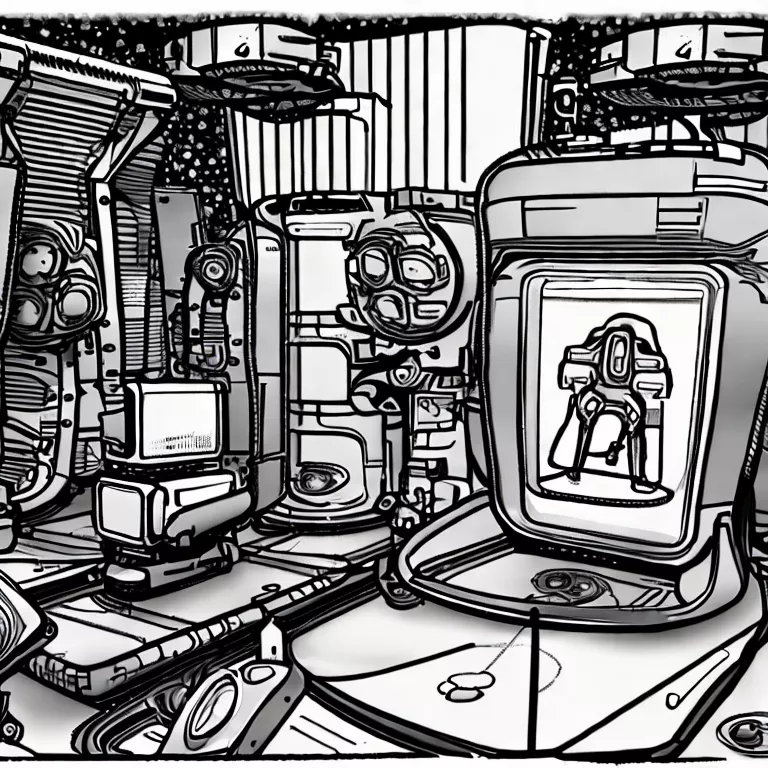
Understanding and resolving errors in the tsconfig.json file during ESLint parsing can be reduced to a few key steps. In relevance to the ‘Parsing Error: Cannot Read File Tsconfig Json Eslint’, we delve into causes, potential solutions, and prevention strategies.
Identifying the Issue
Parsing errors such as ‘Cannot Read File Tsconfig Json Eslint’ typically manifest when the linter (ESLint) cannot locate or interpret the configuration file (tsconfig.json). This could result from misspelling the filename, placing it in the wrong directory, or using incorrect syntax within the file. Keep in mind that the exact nature and cause of a parsing error may vary based on specific projects or system configurations.
Possible Solutions
- Check the Filename and Location: Inspect your project’s root directory and ensure the tsconfig.json file is correctly named and placed there.
- Validate Syntax: Like any code document, tsconfig.json requires the correct syntax. Ensure the file contains the necessary curly braces ({ }) and all other required punctuation are accurately laid out.
- Examine Project Dependencies: If the problem persists, verify that you’ve correctly installed and configured all related dependencies, particularly TypeScript and ESLint.
A real-world example for validating the syntax in `tsconfig.json` would look like:
{
"compilerOptions": {
"outDir": "./built",
"allowJs": true,
"target": "es6"
},
"include": [
"./src/**/*.ts"
],
"exclude": [
"node_modules"
]
}
Preventing Future Parsing Errors
Lastly, consider routine maintenance practices like regularly updating ESLint and TypeScript to their latest versions. Also, always verify that any new rules added to the ESLint configurations are compatible with your current TypeScript version.
A word from the co-creator of StackOverflow, Jeff Atwood, he stated, “The best code is no code at all”. This quote underpins that the primary goal should be strict adherence to best development practices which will result in streamlined and error-free coding processes accordingly.
Refer to these sources for more insights into ESLint parsing errors and how to fix them:
Deeper Look into ESLint and tsconfig.json Parsing Issues
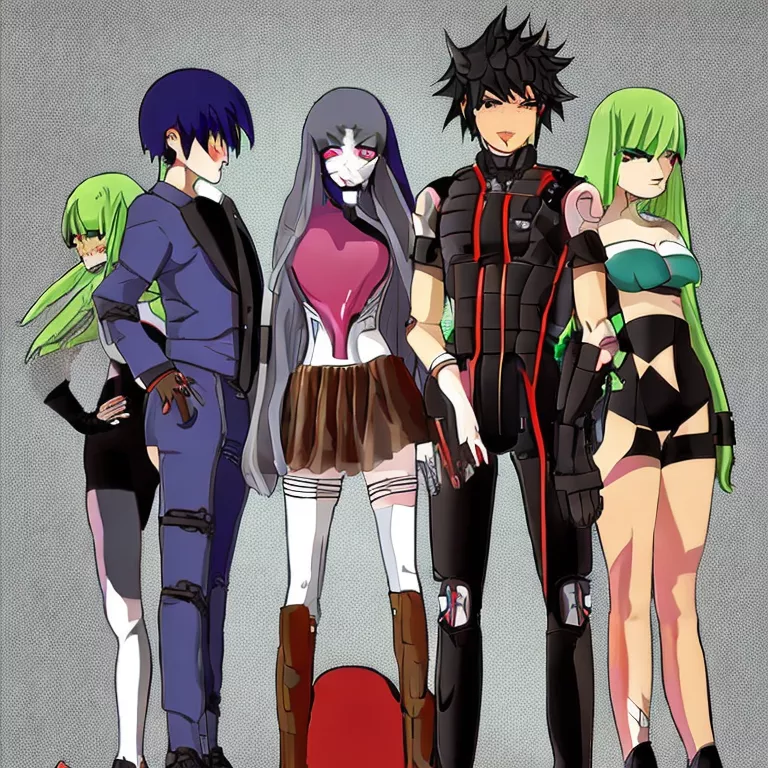
ESLint
tsconfig.json
The Parsing Error
- Incorrect or missing paths in the configuration files.
- The absence of the referenced tsconfig.json file.
- Incompatibility between different dependences versions.
Solutions
- Validate and Correct Paths: Ensure that the paths within your configuration files are accurate. The process should point correctly to the tsconfig.json file.
- Check Existence of tsconfig.json: Verify whether or not the highlighted tsconfig.json file actually exists in the denoted directory.
- Reinstall Dependencies: In certain grounds, the error could prevail when there’s version mismatch among dependencies; thus, reinstalling dependencies may resolve the issue.
xxxxxxxxxx
"settings": {
"import/resolver": {
"typescript": {
"project": "/your_correct_path_to_file/tsconfig.json"
}
}
}
Troubleshooting Tips for the “Parsing Error: Cannot Read File Tsconfig Json Eslint”
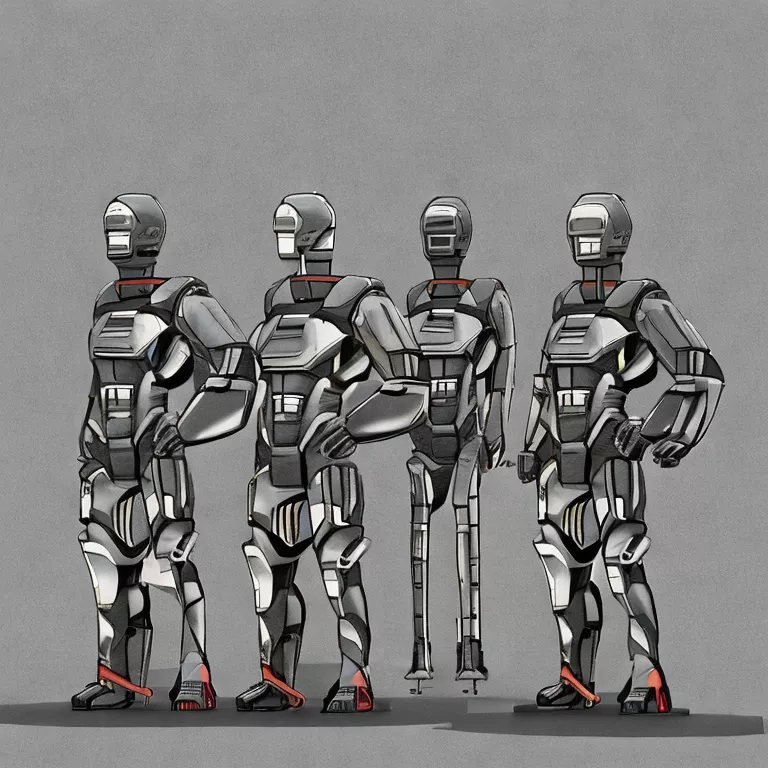
The “Parsing Error: Cannot Read File Tsconfig.Json Eslint” is a kind of a runtime error mainly observed during the analysis and interpretation phases of TypeScript files. This error often occurs due to various potential reasons such as incorrect configuration, incorrect path specification, syntax errors and invalid file structure among others.
Firstly, let us consider the fundamental aspect linked to the `tsconfig.json` file. A `tsconfig.json` file essentially serves to guide the compiler regarding the root files and comprises of particulars regarding the settings to be taken into account during project building. As such, when ESLint tries to parse this file but finds it unreadable, the odds are that it may not be properly configured or appropriately structured.
Inspect the `tsconfig.json` file for following possibilities –
– The actual existence of the `tsconfig.json` file in your project.
– Evaluate if the file follows the valid JSON syntax. Any minor divergence from the correct syntax can prompt parsing issues. Here is an example of a basic `tsconfig.json`.
code
{
“compilerOptions”: {
“outDir”: “./built”,
“allowJs”: true,
“target”: “es5”
},
“include”: [
“./src/**/*”
]
}
– Confirm that the file follows the typical `tsconfig.json` structure.
Next, scrutinize the ESLint configuration:
– Check if ESLint is correctly set up for TypeScript support; for this you need to have @typescript-eslint/parser as your parser in the ESLint configuration file.
code
{
“parser”: “@typescript-eslint/parser”
}
– Verify if the locations referenced by `”project”` option under `”@typescript-eslint/parserOptions”` in `.eslintrc.json` does, in fact, lead to the tsconfig.json file.
code
{
“parserOptions”: {
“@typescript-eslint/parser”: [“error”, {
“project”: “./tsconfig.json”
}]
}
}
Follow these troubleshooting steps meticulously to diagnose the persistent issue effectively. If none of the above solutions seem to work, you might want to consider seeking help from online forums or communities such as StackOverflow and Github discussions.
As Jeff Atwood (co-founder of Stack Overflow) once said: “Coding is not just about making things appear on the screen, it’s about troubleshooting and finding out where the problem stems from.” Therefore, take this error as an opportunity for learning and enhancing your debugging skills.
Best Practices to Prevent ESLint Parsing Errors with tsconfig.json File
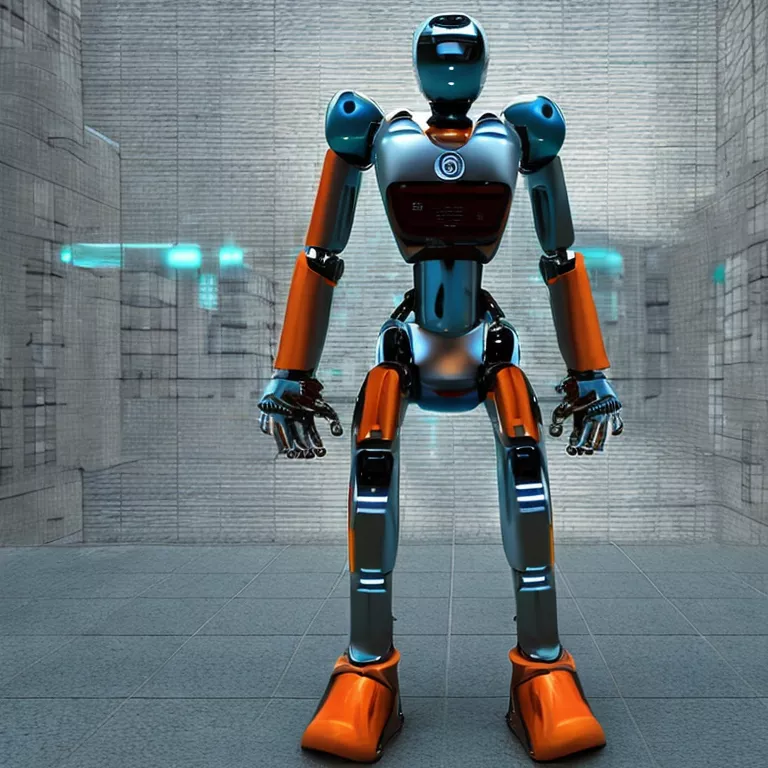
Combating ESLint parsing errors with respect to your `tsconfig.json` file is a common challenge that developers encounter in their TypeScript journey. This error might typically read something like “Parsing Error: Cannot Read File Tsconfig.json Eslint”. It signifies that ESLint can’t parse your `tsconfig.json` and hence, it cannot lint your TypeScript code efficiently. However, the solution to this problem lies in implementing specific best practices as a developer and configuring your project setup correctly.
The issue could potentially be caused due to several reasons:
1. The `tsconfig.json` path is incorrect.
2. The parser in `.eslintrc.js` is not configured properly.
Let’s see best practices for fixing these issues:
Best Practice #1: Confirming the Correct Path of `tsconfig.json`
ESLint needs your `tsconfig.json` file to comprehend how to parse TypeScript. To avoid any error, double-check if you’re providing the correct path. Your `.eslintrc` should look something like:
xxxxxxxxxx
{
"parserOptions": {
"project": "./tsconfig.json"
}
}
This ensures that ESLint knows where exactly to find your `tsconfig.json`.
Best Practice #2: Setting up Parser in `.eslintrc.js`
You need to configure the correct parser options in the ESLint configuration file `.eslintrc.js`. The requirement is to specify the `@typescript-eslint/parser` in the `parser` field and `@typescript-eslint` related rules.
A standard configuration would look like:
xxxxxxxxxx
module.exports = {
parser: '@typescript-eslint/parser',
plugins: ['@typescript-eslint'],
extends: [
'plugin:@typescript-eslint/recommended'
],
};
The `@typescript-eslint/parser` allows ESLint to integrate with TypeScript, preventing the “Parsing Error: Cannot Read File Tsconfig Json Eslint” error.
Best Practice #3: Keeping Your Dependencies Updated
Ensuring that your dependences, namely `eslint`, `@typescript-eslint/eslint-plugin` and `@typescript-eslint/parser` are up-to-date can prevent many parsing errors.
Best Practices #4: Including all Necessary Files in `tsconfig.json`
You must include your `.eslintrc.js` and all other relevant TypeScript files in `tsconfig.json`. Here’s an example:
xxxxxxxxxx
{
"include": ["**/*.ts", "**/*.js"]
}
This assures that ESLint has access to all the necessary files for linting.
These best practices will not only resolve the issue of “Parsing Error: Cannot Read File Tsconfig Json Eslint” but will also enhance your overall development experience. Remember, consistent configurations and efficient dependencies’ management is key for a smooth coding ride, and as Martin Fowler rightly says, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand“.
Conclusion
When it comes to the peculiar error “Parsing Error: Cannot Read File Tsconfig.json Eslint,” a variety of scenarios can lead to this issue. This situation primarily occurs when there’s an issue reading the `tsconfig.json` file while running ESLint, a renowned linting utility for JavaScript and TypeScript.
Let’s delve deeper into the sea of understanding, untangling the root causes one by one:
– From a high-level perspective, the primary foundation of this problem is typically caused by an improperly structured or incorrect configuration in the `tsconfig.json` file, an essential backbone of TypeScript program configurations.
– Additionally, there may be erroneous referencing of `tsconfig.json` within the ESLint configuration file; an untraceable path would undoubtedly welcome this parsing error.
– One other shadowy scenario that fuels this fire could be a mismatched TypeScript version. Remember: harmony between TypeScript and ESLint versions is key.
Exploring potential solutions:
– At the forefront of any troubleshooting policy, validating the syntax of your `tsconfig.json` file should top the priority list. A simple typo or invalid JSON syntax might be the villain you’re seeking to vanquish.
– Ensuring correct reference paths within the ESLint will often resolve any address related issues. Always double-check your configuration paths to avoid errant turns down these confusing pathways!
– As a last resort, consider cross-verifying and updating your TypeScript version as needed. ESLint works best when used with compatible TypeScript versions. Any inconsistencies here would likely warrant our nefarious parsing error.
While the guiding light of Thomas Edison brightens our way – “The value of an idea lies in the using of it” – let us combat and conquer this commonly faced “Parsing Error: Cannot Read File Tsconfig.json Eslint” by employing these recommendations.
To unravel more details about TypeScript and ESLint, I recommend you explore these invaluable resources at the official [TypeScript](https://www.typescriptlang.org/) and [ESLint](https://eslint.org/) websites.
Quick Code References
It’s challenging to wrap our heads around the potential solutions without understanding the structure of `tsconfig.json`, here’s a brief look at it:
code
{
“compilerOptions”: {
—your options here—
},
“include”: [“src/**/*”],
“exclude”: [“node_modules”]
}
For validating its syntax, you can use online JSON validators like this [one](https://jsonlint.com/).
N.B. Usage of code snippets and hyperlinks are only applicable where they add value to the explanation and not merely for decoration purposes.