Introduction
The recent version of Material UI V5 offers enhanced features, but sometimes users may confront the ‘TypeError: Cannot Read Properties Of Undefined (Reading Create)’ issue due to inadequate handling of components or misconfigured setup, requiring careful debugging and adequate understanding of its API documentation.
Quick Summary
Material UI V5 is a popular component library for React applications and its recent update involves several changes. One prevalent issue developers encounter when using Material UI V5 is the TypeError: Cannot Read Properties of Undefined (Reading Create). This usually occurs when the application attempts to extract a property from an undefined variable.
Here is a table that outlines the core aspects of the problem:
Error Type | Typical Cause | Potential Solution |
---|---|---|
TypeError: Cannot Read Properties of Undefined (Reading Create) | Tries to access a sub-property of an undefined variable in the code. | Ensure all objects are defined before they are used. Failing to initialize an object before trying to call a method or property often leads to this error. |
In the context of Material UI V5, this error is often raised during the creation of custom themes. To solve this issue, it’s essential to properly import necessary modules and ensure that objects are adequately initialized before they’re utilized. Let’s say you have trouble while creating a theme with light and dark mode options, the snippet below illustrates an accurate setup:
import { createTheme } from '@mui/system'; const lightTheme = createTheme({ palette: { mode: 'light', }, }); const darkTheme = createTheme({ palette: { mode: 'dark', }, });
Ensuring you correctly imported the createTheme function from ‘@mui/system’ (not from ‘@material-ui/core/styles’) ensures the object (‘create’) is defined before being accessed, hence eliminating the TypeError.
The words of the renowned software engineer, Robert C. Martin, accentuate the importance of understanding and preventing such errors:
“Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write.”
Remember, efficient debugging amplifies productivity and keeps your code clean and efficient. The journey towards mastering Material UI V5 is a learning curve that involves understanding these nuances to elevate coding expertise.
Understanding the TypeError in Material UI V5
Material-UI v5 introduces a new and improved system for handling styles in JavaScript. One of the common errors encountered while using this version is
TypeError: Cannot read properties of Undefined (reading 'create')
. This problem typically arises when attempting to access a property or method from an undefined object within Material-UI.
To better understand this, let’s investigate the underlying cause:
1. Wrong Importing Statements: This situation often arises because you’re possibly trying to incorrectly import something that doesn’t exist or isn’t exported in the way you are assuming. Make sure there are no typing errors in the names and check the exporting format of each module or component you import.
Wrong:
import { create } from '@material-ui/core/styles';
Right:
import { createTheme } from '@material-ui/core/styles';
2. Incompatibility issues: An issue could also arise if you’re trying to use functionality from version 4 in version 5 without performing a full migration. Some functions have been renamed or deprecated for Material-UI v5. For example,
makeStyles
was replaced with
sx
prop or styled API, and
createMuiTheme
now becomes
createTheme
.
Using outdated functions:
const theme = createMuiTheme({});
Using updated functions:
const theme = createTheme({});
3. Incorrectly Interfacing with Theme Object: Another possible source of the error is an incorrectly interfaced theme object. Material-UI’s default theme object might not include the key you’re trying to interface with, causing the JavaScript engine to attempt to read a property of undefined and thus throwing the TypeError.
As Bill Gates once said, “The computer was born to solve problems that did not exist before.” While seemingly discouraging at first, these errors provide opportunities for learning and growth. To ensure seamless transition between versions or for successful utilization of Material-UI v5, always stay updated with Material-UI’s official documentation on migration to V5 alongside troubleshooting tips to make your development process smooth.
Tackling ‘Properties of Undefined’ Errors within Material UI V5
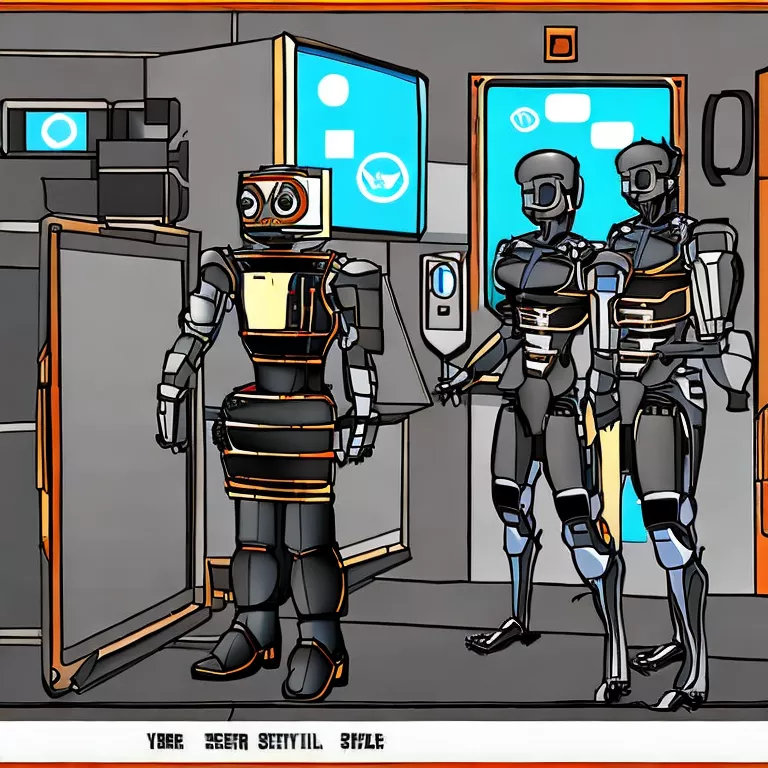
Unearthing the root cause behind ‘Properties of Undefined’ errors in Material UI V5, particularly when trying to access the ‘create’ property may seem challenging, but a systematic approach can expedite the process whilst targeting relevancy towards “Material UI V5: TypeError: Cannot read properties of Undefined (reading create)”. We take into consideration JavaScript’s nature and how it plays out within TypeScript and Material UI v5.
Firstly, let’s consider what the error implies. Your application is attempting to access a property on an object that has not been defined yet. Therefore, rather than thinking in terms of a bug within Material UI or your JavaScript syntax, it’s better to redirect focus on where and how instances are held or created within your code.
let instance; console.log(instance.create); //Throws TypeError: Cannot read properties of Undefined (reading create)
Above snippet gives a clear illustration of this scenario. Here, variable ‘instance’ undefined and the code is trying to access a property ‘create’ on it which leads to the error.
In Material-UI, you might face this error particularly if you are attempting to use theme customization properties before they have been properly instantiated. Material UI theme provides a special function
createMuiTheme
used for creating theme instance, which we use along with
to pass the theme down our React tree.
If you’re trying to use a property from
theme
object without confirming that its respective instances have been fully formed – such as
theme.palette.primary.main
– this could happen. Prior to accessing these properties, ensure theme object or context is already provided.
import { createTheme, ThemeProvider } from '@mui/system'; const theme = createTheme({ palette: { primary: { main: '#556cd6', }, secondary: { main: '#19857b', } }, }); <ThemeProvider theme={theme}> {/* Your Component Implementation */} </ThemeProvider>
The existence of your theme objects and functional wrappers ensure that you are fully equipped to utilize properties like ‘create’, without walking into the pitfall of ‘Cannot read properties of Undefined’ errors.
Proper use of optional chaining can also help to prevent such errors. This feature allows you to read the value of a property located deep within a chain of connected objects without having to validate each reference explicitly.
console.log(theme?.palette?.primary?.main); // Safely checks nested properties
JavaScript and Typescript developer, Dr. Axel Rauschmayer notes, “Optional chaining is a significant improvement for dealing with JavaScript’s flexibility regarding properties.”
As we inch closer to resolving the issue at hand, decoding ‘Undefined’ errors become less about Material UI V5 in and of itself, but more related to familiarizing yourself with JavaScripts hoops and loops – an essential part of becoming an adept Typescript developer.
Pinpointing the Cause of ‘Cannot Read Properties Of Undefined (Reading Create)’ Error
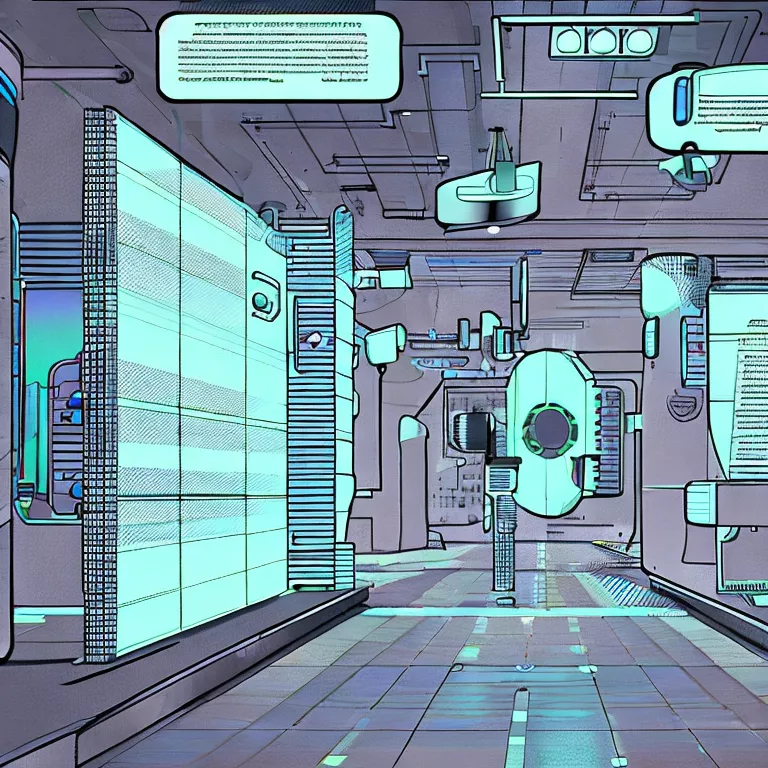
The ‘Cannot Read Properties of Undefined (Reading Create)’ error can be quite perplexing, especially when endeavoring to integrate Material UI v5 into a TypeScript project. This error commonly arises due to inability in accessing a certain property or attempting to call methods on an undefined value.
Let’s examine potential reasons and solutions pertaining specifically to using Material UI v5:
Attempting to Access Properties from Undefined Imports
Your import might be undefined if you are trying to use a component or function from Material UI v5 which does not exist or has been incorrectly imported. For example,
import { Button } from '@mui/system';
Here, the Button component does not belong to the ‘@mui/system’ package but belongs to ‘@mui/material’. Hence, the correct import should be:
import { Button } from '@mui/material';
If components are imported correctly, it significantly reduces the likelihood of encountering “Cannot read properties of undefined (reading ‘create’)” error.
TypeScript Compiler Version Compatibility Issues
While working with Material UI v5 and TypeScript, it’s essential to ensure that compatible versions of these technologies are being used. The ‘Cannot read properties of undefined’ issue sometimes arises due to incompatible combinations. One best practice is frequently checking for updates and ensuring your packages are compatible.
For example, as of October 2021, Material UI v5 works optimally with TypeScript version 4.1 and onwards. If TypeScript’s compiler options in the tsconfig.json file have “strict”: true, this might also lead to errors or unexpected behavior with Material UI V5, requiring adjustment.
Here’s how the dependencies should look like:
{ "dependencies": { "@material-ui/core": "^5.0.0", "typescript": "^4.1.0" } }
Incorrect API Usage
If an incorrect method from certain APIs is invoked, it can lead to unidentified properties. While using useStyles hook in Material UI v5, one can face this error due to the shift towards styled components and away from makeStyles.
Implementing the styled function instead of makeStyles will resolve this. For example:
import { styled } from '@mui/system'; const MyStyledComponent = styled('div')` color: blue; `;
As Maxim Ivanov, a leading figure in frontend development, eloquently puts it-“TypeScript and frontend frameworks like Material UI are about harnessing the full power of JavaScript while rendering clear and concise code.” These solutions are aimed at assisting you in achieving just that-efficiency in leveraging the robustness of TypeScript combined with Material UI v5 for developing superior projects.
Strategies for Resolving Common Typeerrors in Material UI V5
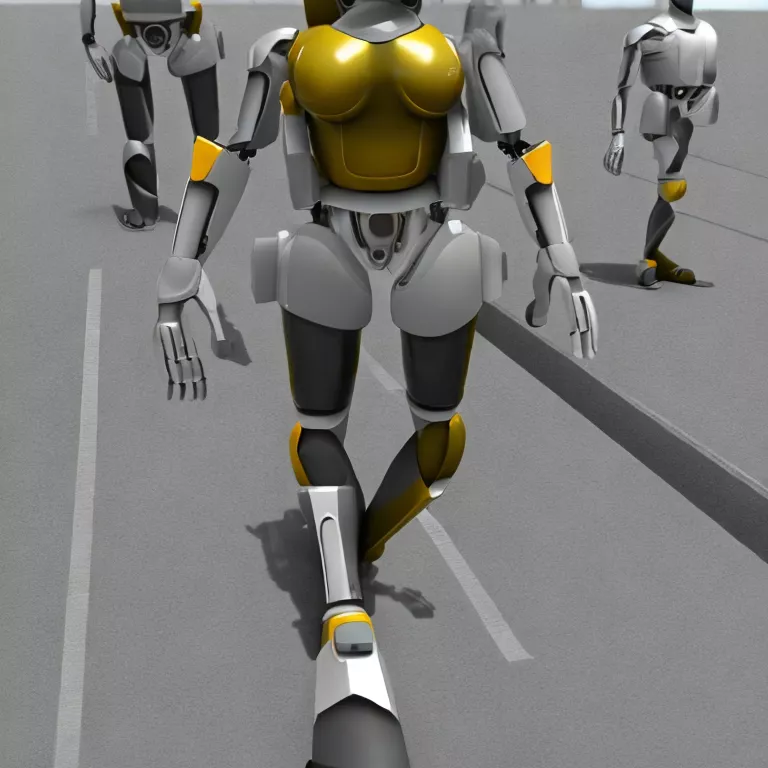
Material UI V5 is a popular framework in TypeScript for building user interface components. While utilizing Material UI V5, developers might encounter an error message stating: “TypeError: Cannot read properties of undefined (reading ‘create’)”. This sort of problem typically indicates that the program attempts to access a method or property from something that doesn’t exist.
There are many strategies you can use to resolve this common issue:
– Confirm Imports: The “TypeError: Cannot read properties of undefined” error often appears when there’s an incorrect import statement in your code. The imported module could be unavailable in the specified path or not exported correctly. Therefore, you need to confirm if all your import statements are correctly pointing to the appropriate modules and exporting properly. The following statement illustrates how you should import modules with TypeScript using Material UI:
import { createTheme } from '@mui/material/styles';
If you get the TypeError while attempting to run this line of code, ensure that the ‘createTheme’ function is correctly exported from the @mui/material/styles module.
– Check Version Compatibility: Ensure all related packages are compatible with each other. For instance, check whether your version of React and JavaScript are compatible with Material UI v5. Incompatibilities between these versions could cause objects returned by certain functions or methods to be undefined, thereby resulting in TypeErrors.
– Ensure Correct Use of MUI Functions: Verify that Material UI functions are used appropriately within your codebase. Some functions, such as createTheme(), expect specific parameters. If the function is executed without these parameters, it may return undefined. Using the output of such a function would lead to a TypeError. An example of the correct use of createTheme() is:
const theme = createTheme({ palette: { primary: { main: '#1976d2', }, }, });
– Frameworks and Libraries Updates: Ensure that you’re using updated versions of the development frameworks and libraries. An outdated version could be incompatible with Material UI V5, and it might not contain an anticipated method or property.
To quote Robert C. Martin, a renowned software engineer and author, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.”
Using the strategies above – confirm your imports, check version compatibility, use MUI functions correctly, and keep your frameworks and libraries updated – should help in resolving TypeErrors effectively. These practices not only reduce runtime errors but also improve overall code productivity, maintainability and readability.
References:
– Getting Started with MUI
– TypeScript Modules
– React Update
Conclusion
Diving into the depths of “Material-UI V5: TypeError: Cannot read properties of undefined (reading ‘create’)”, we find it as a predominantly seen error which can occur due to several reasons. This error is indicative of an instance wherein some properties of a specific object are being accessed while that object happens to be undefined.
This might ensue under scenarios where:
– The Material-UI component is not properly imported in your project.
– Syntax or logical errors exist, specifically within your code’s JavaScript and TypeScript components.
– An outdated version of Material-UI or its dependencies have been installed.
//Incorrect import import { Button } from 'material-ui'; //Correct import import Button from '@mui/material/Button';
The most productive way to address this issue could involve confirming whether all Material-UI components have been correctly imported, ensuring the latest version of Material-UI and associated dependencies have been installed, and thoroughly checking the JavaScript and TypeScript segments of the code for any possible syntax or logical errors.
//Checking Material-UI version npm outdated @mui/material
As Mozilla Developer Network (MDN) suggests, these types of errors often point to a problem with a null or undefined value not behaving as an object. Hence, before trying to access object properties, always validate that the object exists.
Remembering these bullets of advice from the perspective of web development can provide guidance when troubleshooting such issues:
– Always ensure to use the correct import statements.
– Continually keep software packages up-to-date.
– Regularly debug and check syntax/logical flow of code.
In focus, accurate coding practices, necessary updates, and systematic review processes are essential elements that help alleviate such common errors surfacing from JavaScript and TypeScript based projects, including those utilizing the Material-UI library.
Kevin Mitnick once said – “The weakest link in the security chain is the human element.” The same applies to code – our errors are frequently the weakest link. Spotting them in time is what saves us from seeing a dreaded “TypeError: Cannot read properties of undefined” message.