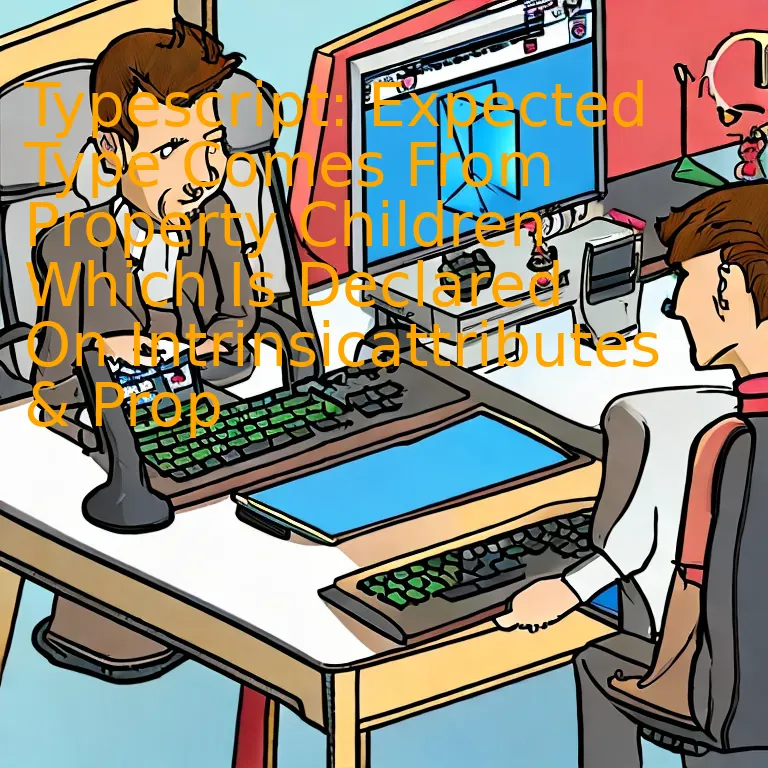
Introduction
In Typescript, when an expected type originates from the ‘Children’ property – which is postulated in both IntrinsicAttributes and Prop – it enhances web development process by ensuring error-free coding and stable functionalities.
Quick Summary
The TypeScript language brings static type-checking to JavaScript that helps developers write more reliable and maintainable code. One common issue you might encounter when working with TypeScript in a React project involves the ‘children’ prop of a functional component and its associated typing.
Consider the following table:
Properties | Description |
---|---|
x Children |
Special React property, it doesn’t have an explicit definition inside the components; used to pass elements from component invocation rather than config. |
xxxxxxxxxx IntrinsicAttributes |
An interface provided in TypeScript when using JSX syntax which can assist in defining standard attributes expected on HTMLElements or SVGElements. |
xxxxxxxxxx Prop |
A special keyword in React to pass values from a parent component down to a child component. |
One potent facet where TypeScript shines is enforcing and communicating the shape of the props for our React components. When the error “Expected Type Comes From Property Children Which Is Declared On Intrinsicattributes & Prop” emerges, it usually means that the children property is not correctly typed in your component’s Prop types.
Let’s say we’re typing a functional component that accepts children as props. We want to make sure those children are of a specific type. The error would emerge if the children aren’t of the defined type,. To resolve, it’s crucial to ensure the type declaration aligns precisely with the structure and type of the values being passed in the children property.
Your component should be something akin to this:
xxxxxxxxxx
type MyComponentProps = {
children: React.ReactNode
};
const MyComponent: React.FC = ({ children }) => (
<div>
{children}
</div>
);
Here, we’re using the
xxxxxxxxxx
React.ReactNode
type, which matches any permissible JSX/TSX expression.
As Martin Fowler, a prominent figure in software development, once said – “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” It’s not just about following syntax, it’s also important to annotate and structure the code accurately to convey its meaning and avoid errors like these.
The primary intention should be writing clear, maintainable code, reducing future debugging time, and creating an overall smoother development experience. A deeper understanding of TypeScript intrinsic attributes significantly helps in achieving this ideal workflow.
Relevant References:
TypeScript JSX Handbook
Children in JSX
Understanding TypeScript: IntrinsicAttributes & Props
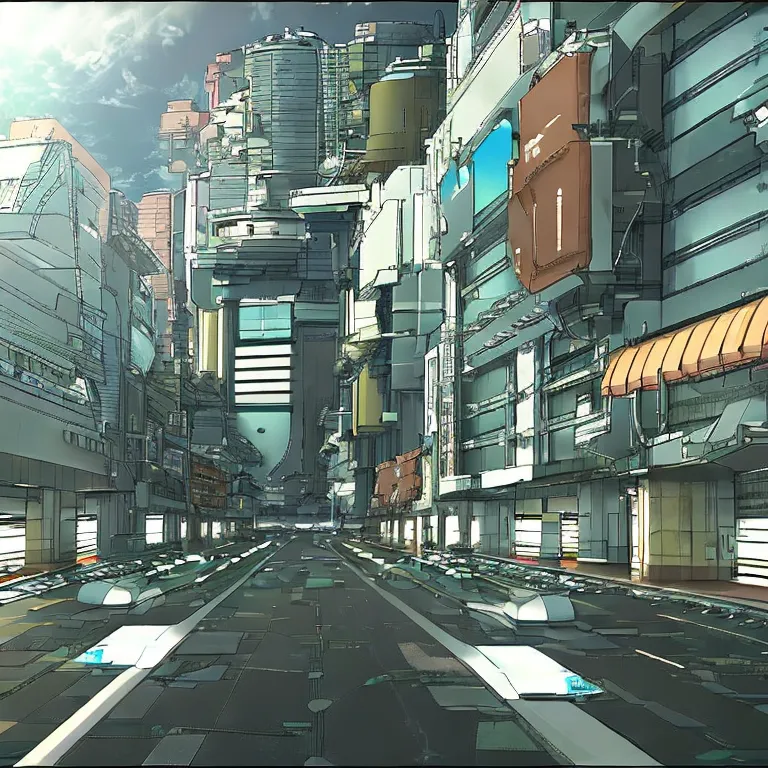
Understanding TypeScript’s IntrinsicsAttributes and Props in-depth involves looking into the core workings of TypeScript, a statically typed superset of JavaScript that is increasingly popular in modern web development. It brings along robust types and enhanced tooling to improve developer productivity and code maintainability.
The specific topics at hand are `IntrinsicAttributes` and `Props` within TypeScript used primarily in the context of building out React components. Within these components, certain properties can be passed down referred to as ‘props’. These props allow developers to transfer data between components and give structure to the component architecture.
IntrinsicAttributes
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
IntrinsicAttributes is a type that comes from TypeScript’s JSX Namespace, originating from the fact that there are standard attributes that apply to any JSX Element. In React’s Type definition files, you’ll find this:
xxxxxxxxxx
{`
interface IntrinsicAttributes {
key?: Key;
}
`}
In the above example, 'key' is a special attribute that's often used when rendering lists in React.
Props and Children Prop
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Props on the other hand, are custom attributes given to a JSX element (like a React component). The `children` prop is special in that it refers to any elements included between the opening and closing tags when invoking a component. This syntax and interaction are unique to JSX and TypeScript provides types for these interactions to make sure your components interact properly during compile-time checking. Let's look at an example:
{
`function Welcome(props: { name: string }) {
return Hello, {props.name};
}
// Output: Hello, Sara`}
The error "Expected Type Comes From Property 'Children' Which Is Declared On `IntrinsicAttributes & Props`" typically emerges when TypeScript tries to determine the "children" prop in a function component and are unable to match the declared typing with its usage. Revising the types or structuring of your components should correct this error.
Peter Norvig, Director of Research at Google, once stressed: "Programming is not about typing... it's about thinking." — always being mindful that understanding the roots of these constructs will get you a long way on your TypeScript journey.
Breaking Down 'Expected Type' in TypeScript
<img decoding="async" alt="Typescript: Expected Type Comes From Property Children Which Is Declared On Intrinsicattributes & Prop" class="aligncenter" height="400" src="https://typescriptworld.com/wp-content/uploads/2023/09/Breaking-Down-Expected-Type-in-TypeScript.webp" width="400"/>When working with TypeScript, it is not uncommon to come across type related issues. One such issue involves the 'Expected Type' error message. The error message stipulates that the 'Expected Type Comes From Property Children Which Is Declared Here on IntrinsicAttributes & Prop'. Understanding this conundrum requires a deep investigation into two key parts of TypeScript: the TypeScript ChildComponent 'Children' property and the IntrinsicAttributes and Properties.
In the world of React and TypeScript, components receive props to function accordingly. Among those properties, 'children' stands out due to its unique role and behavior.
The code snippet below simplifies the scenario:
code
Here, `value` represents children of `ChildComponent`. This arrangement can lead to several complications when types aren’t explicitly defined in TypeScript. Hence, the ‘Expected Type’ error originates.
To break this down:
– `
– However, if the type for what children the component is expecting isn’t explicitly declared, this can lead to problems.
– Specifically, if ‘ChildComponent’ is expecting a string as children, but we pass an object, TypeScript will flag this mismatch, resulting in the ‘Expected Type Comes from Property Children’ error.
Unpackaging ‘IntrinsicAttributes & Prop’, one finds out that these are inherent properties a JSX element has in TypeScript. ‘IntrinsicAttributes’ generally consists of key and ref. While ‘Prop’ includes all attributes given to the component which extends the intrinsic properties provided natively by the platform. The ‘Prop’ can be a custom-defined type or can adopt from built-in HTML element attributes using `React.HTMLAttributes`.
A possible solution is as follows:
Start by defining an Interface or type for the ‘ChildComponent’, stating what type of children it is expecting.
code
interface ChildComponentProps {
children: ReactNode;
}
Here, ‘ReactNode’ is used type which can accommodate any type of child – be it a string, element or an array thereof.
Then specify the accepted props:
code
const ChildComponent: FC
;
By defining PropTypes in this manner for components, we ensure that any discrepancies in terms of ‘Expected Types’ are effectively handled, thereby reducing the likelihood of runtime errors.
According to Steve McConnell, the author of Code Complete, “Good code is its own best documentation.” We stress on understanding each TypeScript component and aligning it accordingly with incoming or outgoing data to avoid such errors.
For further details, do consider visiting the official [TypeScript Handbook].
Exploring the Relationship Between ‘Expected Type’ and Property Children in TypeScript
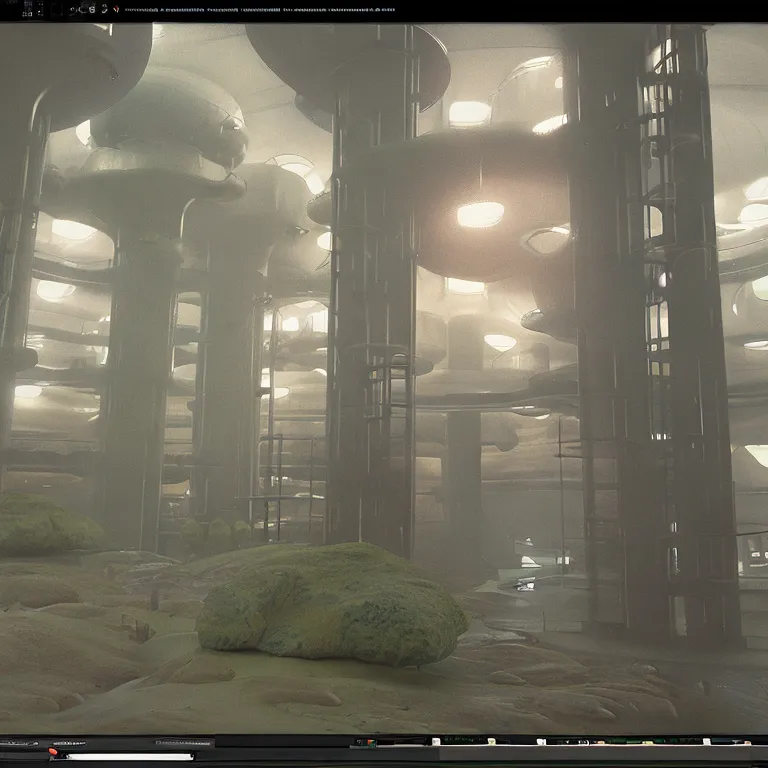
The relationship between ‘Expected Type’ and property ‘Children’ in TypeScript can be rather complex but it is fundamentally pivotal to understanding the structure of TypeScript elements. The topic of our discussion stems from a scenario where TypeScript presents an ‘Expected Type comes from property children which is declared here on IntrinsicAttributes & Prop’.
In TypeScript, JSX expressions such as React components expect children as their props. Here,
xxxxxxxxxx
children
is what we refer to as a ‘special’ property. It provides the ability to pass components as data to other components.
When you see an error like ‘Expected Type comes from property children which is declared here on IntrinsicAttributes & Prop’, this typically means that the type of children defined by your component doesn’t correspond with the actual values being passed in for that prop.
To illustrate, let’s consider a simple code snippet:
xxxxxxxxxx
type TextWithNumberProps = {
children: (n: number) => ReactNode
};
export function ParentComponent(){
return (
{_
=> Hello World!}
)
};
Here, the type of
xxxxxxxxxx
children
is defined to be a function that receives a number and should return a valid React Node. However, the actual child component passed into
xxxxxxxxxx
TextWithNumberProps
is a function that doesn’t receive anything. This discrepancy induces TypeScript to rant about the expected type derived from the property ‘Children’.
This close interaction between TypeScript’s Expected Type behavior and the intrinsic attribute of the ‘Children’ property is key when working with Modular Development in TypeScript environments such as React.
To circumnavigate these issues, ensuring that both sides of the PropTypes equation line up accurately according to declared standards will establish compliance and help avoid the explicit error. As software engineer Alexander Moss once pointed out, “Whatever is produced by some piece of software needs to fit wherever it is going to be plugged in. Both the plug and the socket need to match.”
While TypeScript adds a robust typing system to JavaScript, understanding its handling of child properties and expected types can help optimize your development process, leading to cleaner, error-free, and high performing code that is readily scalable and manageable.
Online references: [TypeScript Handbook](https://www.typescriptlang.org/docs/handbook/jsx.html), [React TypeScript Cheatsheet](https://react-typescript-cheatsheet.netlify.app/docs/basic/getting-started/jsx_element_type)
Practical Usage of IntrinsicAttributes & Props in TypeScript
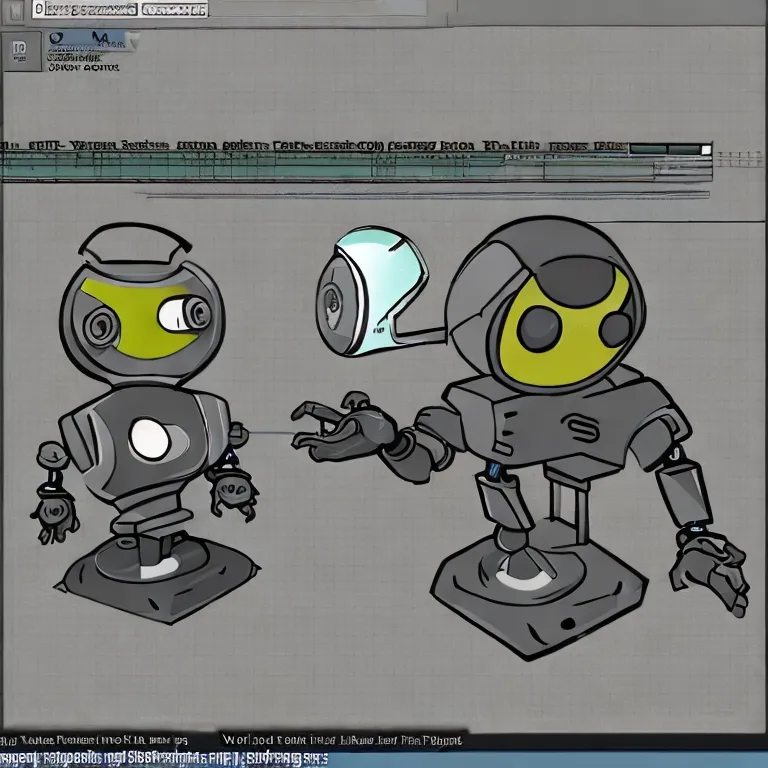
The practical usage of
xxxxxxxxxx
IntrinsicAttributes
and
xxxxxxxxxx
Props
within TypeScript plays an important role in React development. Commonly, any property outside the scope defined in the
xxxxxxxxxx
props
type will end up in the mysterious pool named
xxxxxxxxxx
IntrinsicAttributes
&
xxxxxxxxxx
Props
. It is important to note that this often includes children elements of the main component, which become crucial when building reusable components or handling tasks like conditional rendering within a component.
However, there’s an intriguing issue that could arise with using these two entities in developing TypeScript-based components. Occasionally, TypeScript could present an error suggesting that the expected type comes from the property ‘children’ which is declared on the IntrinsicAttributes & Props interface. This can be bewildering, particularly if we are not accustomed to dealing with such potential idiosyncrasies.
Practical Scenario
Let’s illustrate this problem by considering a component declaration in TypeScript:
TypeScript code |
---|
xxxxxxxxxx interface AppProps { someProp: string; } function App({ someProp }: AppProps): JSX.Element { return \{someProp}\; } |
This may trigger the following error: “Type ‘{ children: Element; }’ is missing the following properties from type ‘AppProps’: someProp”. The problem here being that TypeScript does not know how to infer the ‘children’ prop – it only knows about ‘someProp’.
Solution
To resolve this conflict, we have to explicitly mention the ‘children’ prop in our interface:
Updated TypeScript code |
---|
xxxxxxxxxx interface AppProps { someProp: string; children?: React.ReactNode; } function App({ someProp, children }: AppProps): JSX.Element { return \{someProp}{children}\; } |
The Underlying Reason
This problem and solution lie within the heart of how TypeScript handles types. As a statically-typed language, TypeScript provides several utilities for making sure we correctly document the structures we expect within our objects – a clear example being when dealing with React children.
As Pablo Picasso once said, “Computers are useless. They can only give you answers”. This embodies the importance of bringing clear perspective, curiosity, and comprehension in working with tools and languages like TypeScript—and indeed software development at large—an aspect that no tool or AI checking system could provide.
Conclusion
The error “Expected Type comes from the property ‘children’ which is declared on IntrinsicAttributes & Prop” relates to TypeScript constraints in React. This error arises when you fail to type your properties correctly, especially if you’re passing children prop into your component.
Diving deeper, there’s a compelling logic behind it:
– Every custom component in react receives a default set of attributes known as
xxxxxxxxxx
IntrinsicAttributes
. These include
xxxxxxxxxx
key
and
xxxxxxxxxx
ref
, and should not be explicitly defined.
–
xxxxxxxxxx
children
is a special property in any React Component that allows components to pass elements to each other.
– TypeScript necessitates that we annotate these props for ensuring compile-time type safety.
The following demonstrates declaring a component with TypeScript:
xxxxxxxxxx
interface MyComponentProps {
name: string;
}
const MyComponent: React.FC<MyComponentProps> = ({name, children}) => (
{`Hello, ${name}`}{children}
);
Here, the children prop is typed implicitly by using
xxxxxxxxxx
React.FC
.
TypeScript combined with React allows users to create more robust applications by enforcing type safety. Developers get an opportunity to catch errors during coding rather than at runtime, enhancing their productivity and code quality. Moreover, typing enhances autocompletion, function signature information, and feature definitions within the IDEs. All these elements together contribute to better scalability and maintainability of complex large-scale apps.
This sage advice by Jeff Atwood, co-founder of Stack Overflow, rings particularly true here – “Code is read far more times than it is written.” As developers, striving for clarity must remain paramount and using TypeScript with React helps us achieve just that.