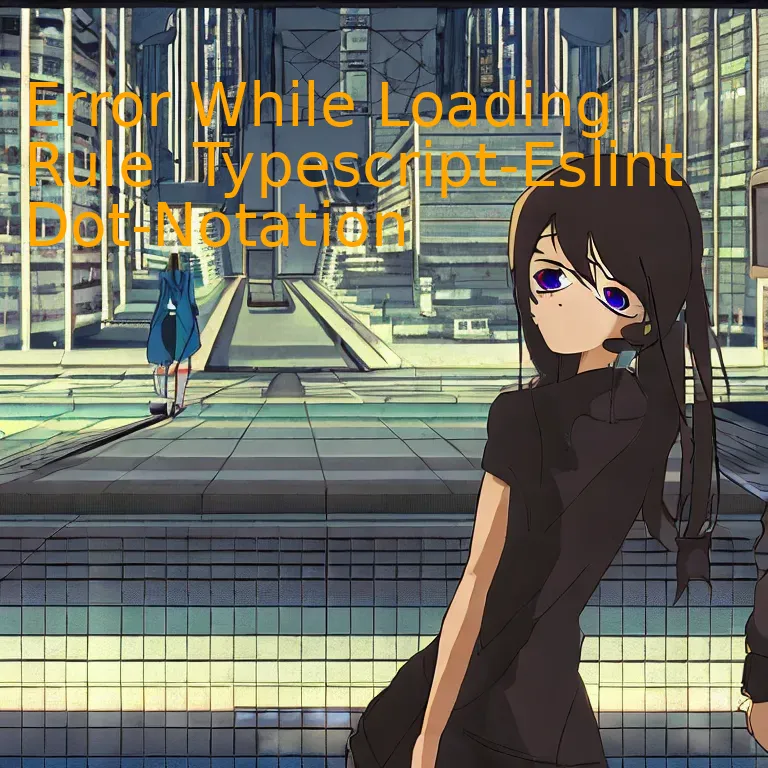
Introduction
Delving into the facet of Typescript-Eslint Dot-Notation, it’s noted that occasional hitches related to “Error While Loading Rule” can potentially arise from incorrect setup or incompatible versions requiring prompt debug and proper configuration for seamless operational efficiency in the world of coding.
Quick Summary
The Error While Loading Rule Typescript-Eslint Dot-Notation is a common issue developers might encounter when working with TypeScript and ESLint together. This error generally reflects a configuration problem between these technologies.
Error Component | Description |
---|---|
x Typescript-Eslint |
A set of ecosystem plugins designed to maximize compatibility between TypeScript and ESLint. |
xxxxxxxxxx Dot-Notation |
A rule in the ESLint system, advising that dot notation be employed whenever it’s possible. |
xxxxxxxxxx Error While Loading Rule |
An indicator that the specified rule (in this case, Dot-Notation) could not be correctly loaded or applied to the TypeScript environment. |
Understanding individually,
xxxxxxxxxx
Typescript-Eslint
serves as an intermediary for TypeScript (a statically typed superset of JavaScript) and ESLint (a tool to identify and report patterns found in ECMAScript/JavaScript code). Through a series of plugins, it enables TypeScript and ESLint to work together accurately.
Meanwhile,
xxxxxxxxxx
Dot-Notation
is a specific rule within ESLint that advises developers to use dot notation (e.g., object.value) instead of bracket notation (i.e., object[‘value’]) where feasible. It enhances readability and understanding of code.
When you encounter the
xxxxxxxxxx
Error While Loading Rule
, it signifies an issue with the application of this rule to the TypeScript environment. The core reason can vary; however, it often stems from incorrect configurations in your settings, such as erroneous ESLint set up, wrong version of dependencies, or use of incompatible plugins.
To rectify this issue, it’s important to check your TypeScript and ESLint integration, reassure that you’re using compatible versions of software/packages, and ensure the rule isn’t being unevenly applied in your ESLint configuration file (.eslintrc).
Lastly, as suggested by Sir Tony Hoare, focus on simplicity and clarity in problem-solving. Following these principles ingrained in his quote, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies.” should guide your resolution process in debugging and correcting such errors efficiently.
Understanding the Essence of Typescript-Eslint Dot-Notation Error
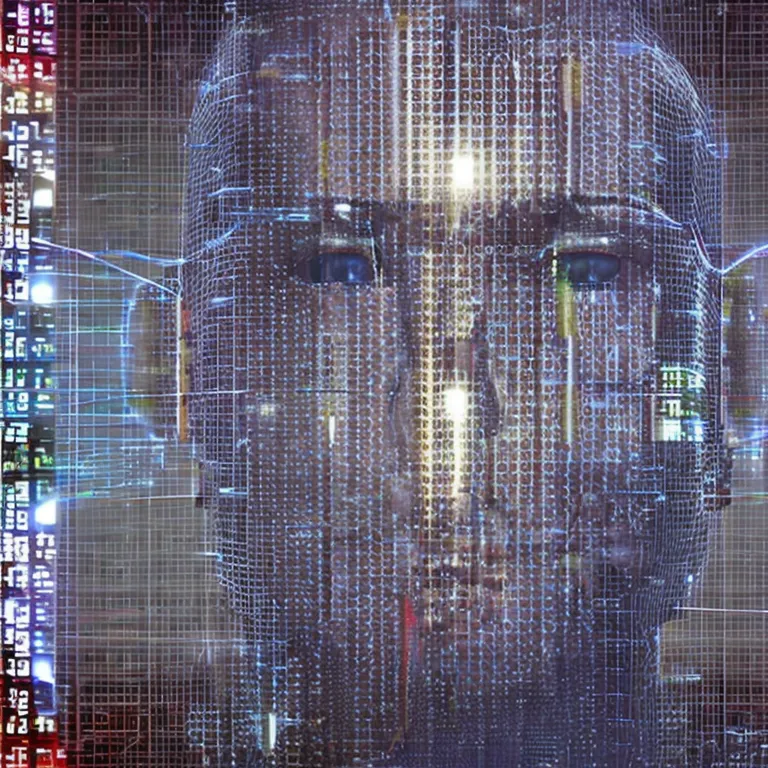
When working with TypeScript coupled with ESLint, often one can encounter certain kinds of errors that may disrupt the smooth functioning of their code. One such glitch one might run into is the “Error while loading rule ‘typescript-eslint/dot-notation'”. This particular error, although a common hiccup encountered, could be a bit challenging to troubleshoot if not equipped with a proper understanding of its essence.
-
xxxxxxxxxx
Error while loading rule 'typescript-eslint/dot-notation'
typically indicates a conflict with your ESLint setup, where it’s struggling to correctly enforce the dot notation usage in your Typescript language
- The primary intent of ‘typescript-eslint/dot-notation’ is to enforce the consistent use of dot notation whenever possible in your code. It’s designed to catch and flag any instance where bracket notation is used to access properties when the property name doesn’t require it
- If you encounter this error, it usually points towards an issue with your ESLint configuration or potentially a version mismatch between various packages that power the eslint-typescript functionality
Possible Reasons | Solutions |
---|---|
Mismatched versions | Check if all your ESLint, TypeScript, and typescript-eslint packages are updated and match each other in terms of compatibility. |
Incorrect Configuration | Audit your .eslintrc configuration files to ensure correct syntax and usage. |
Inappropriate Rule Usage | Reassess how and where you’re applying the ‘typescript-eslint/dot-notation’ rule within your code. |
If the error persists, you might want to consider reinstalling those packages or seek help from different community forums by posting your problem there.
Here’s a basic example of implementing dot notation in your code which works perfectly fine with the eslint dot-notation rule:
xxxxxxxxxx
{" "}
let user= {
name: 'John'
};
console.log(user.name);
“Computers are incredibly fast, accurate and stupid; humans are incredibly slow, inaccurate and brilliant; together they are powerful beyond imagination.” – Albert Einstein. Bear this quote in mind as you work with TypeScript and ESLint. Technology is here to be our tool, not master. Understand it, harness its power, and when you hit roadblocks like these, know that there’s always a solution right around the corner.
Decoding Errors: Comprehensive Insight into Typescript-Eslint Dot-Notation
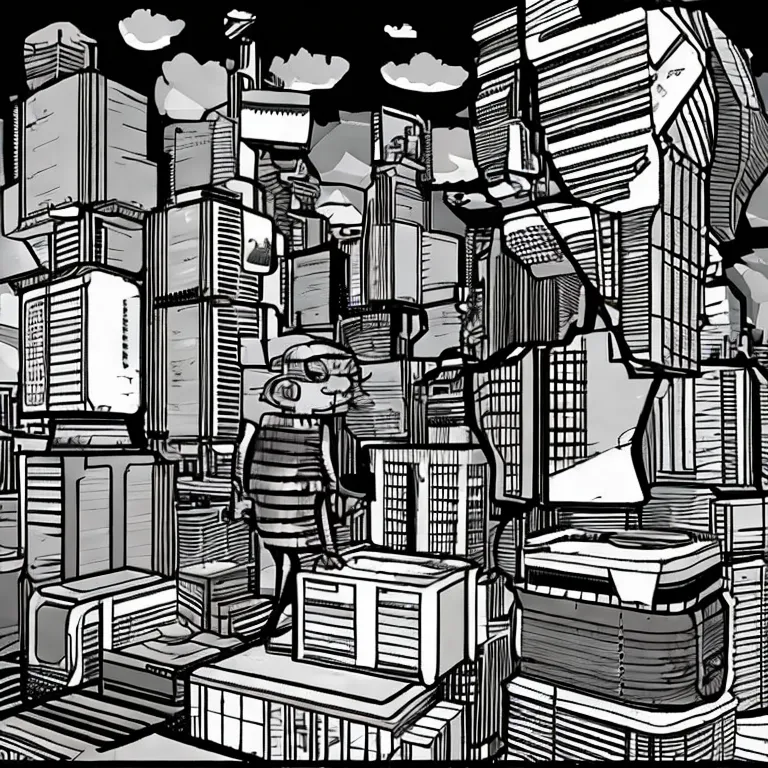
Understanding the specifics of error decoding, with a primary focus on “Error While Loading Rule `@typescript-eslint/dot-notation`” can essentially help us better navigate coding mishaps within the TypeScript environment. Here in this write-up, we delve further into this error and unravel the effective ways to navigate it while working on TypeScript projects.
To commence with a basic understanding, TypeScript is an open-source programming language developed by Microsoft. It’s often considered a superset of JavaScript, adding static types to the language, thereby improving code quality and readability.
Error While Loading Rule `@typescript-eslint/dot-notation`: A Comprehensive Insight
The stated error usually pops up when there is either a discrepancy in the correct installation or some configuration issues with your ESLint extension, specifically related to the rule ‘@typescript-eslint/dot-notation’. Let’s further understand the issue:
- ESLint, an open-source JavaScript linting utility, assists developers to find potential errors in their JavaScript code without executing it.
- The @typescript-eslint/dot-notation is one of the many rules offered by ESLint to maintain code consistency. Particularly, this rule urges the usage of dot notation whenever possible over bracket notation for property access in JavaScript objects.
- If you encounter ‘Error While Loading Rule’ warning, likely, ESLint cannot load the ‘@typescript-eslint/dot-notation’ due to incorrect installation or missing dependency.
xxxxxxxxxx
let value = object.property;
(Preferred over:
xxxxxxxxxx
let value = object['property'];)
Standard procedure for resolving these TypeError includes two essential steps:
1. Verifying the proper installation of ‘@typescript-eslint/eslint-plugin’ package, since the ‘@typescript-eslint/dot-notation’ belongs to this package.
xxxxxxxxxx
npm install --save-dev @typescript-eslint/eslint-plugin
2. Ensuring that you have correctly configured the .eslintrc configuration file, which guides how ESLint applies rules in your coding project.
Advanced Troubleshooting
If the error continues to persist after following above steps, it might require a deeper dig into:
- The compatibility between various versions of TypeScript, ESLint and their related packages.
- Any conflicts with other plugins or rules specified in your ESLint configuration
- The possibility of a bug in the ESLint plugin itself, which may necessitate an issue filed in the ‘@typescript-eslint’ GitHub repository
This broad-looking comprehension of TypeScript-ESLint dot-notation and the associated errors ensures effective error handling which eventually accentuates a streamlined coding experience. As famously stated by Linus Torvalds, creator of Linux and Git, “Talk is cheap. Show me the code.“, such knowledge certainly sweeps away potential roadblocks on our path to showing great code in TypeScript.
Troubleshooting Techniques for Typescript-Eslint Dot-Notation Error
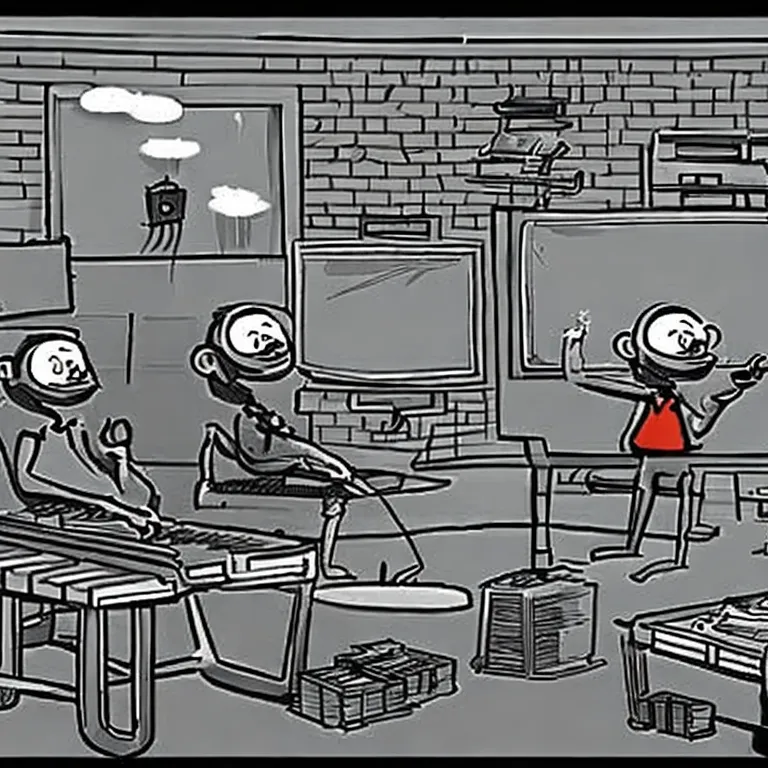
Diagnosing and Resolving the Typescript-Eslint Dot-Notation Error
The Typescript-Eslint dot-notation error typically occurs when there’s a discrepancy between the recommended dot notation syntax and the actual way you’ve utilized bracket notation in your code. Diagnosing and resolving this issue necessitates an understanding of the ECMAScript/JavaScript programming conventions, as well as the specific rule configurations in ESLint.
➤ Firstly, consider the difference between dot notation and bracket notation. Essentially, dot notation is often favored for simplicity:
xxxxxxxxxx
// Correct usage of dot notation
let value = obj.property;
While bracket notation might be causing the error:
xxxxxxxxxx
// Potentially incorrect usage leading to ESLint error
let value = obj['property'];
Incorporating them appropriately can ensure your code follows JavaScript best practices and aligns with ESLint rules.
➤ By defining rules in your .eslintrc or equivalent configuration file, TypeScript-ESLint enforces improved error-handling. Should an element not adhere, like in the error case “Error While Loading Rule ‘typescript-eslint/dot-notation'”, this signifies that ESLint encountered an unexpected token when reading through bracket notation properties.
To rectify this trouble, customize the rules in your ESLint configuration file. According to the ESLint documentation, the following configuration allows bracket notation explicitly:
xxxxxxxxxx
{
"rules": {
"dot-notation": ["error", { "allowPattern": "^[a-z]+(_[a-z]+)+$" }]
}}
This adjustment permits the use of bracket notation for properties that match the regular expression pattern, thus avoiding the aforementioned TypeScript-ESLint error.
Moreover, TypeScript’s type assertion functionality might be advantageous in some situations. Consider the following:
xxxxxxxxxx
(window as any)['property'] = value;
Remember though, having a clear understanding and proper application of these language features is key.
As Steve Jobs once said, “Everybody in this country should learn how to program a computer… because it teaches you how to think”. Hence, comprehending these particulars not only elevates your coding skills but also enhances your logical thinking abilities. Therefore, debugging of the TypeScript-ESLint-dot notation error becomes a pathway towards programming proficiency.
Effective Solutions to Resolve Typescript-Eslint Dot-Notation Issues
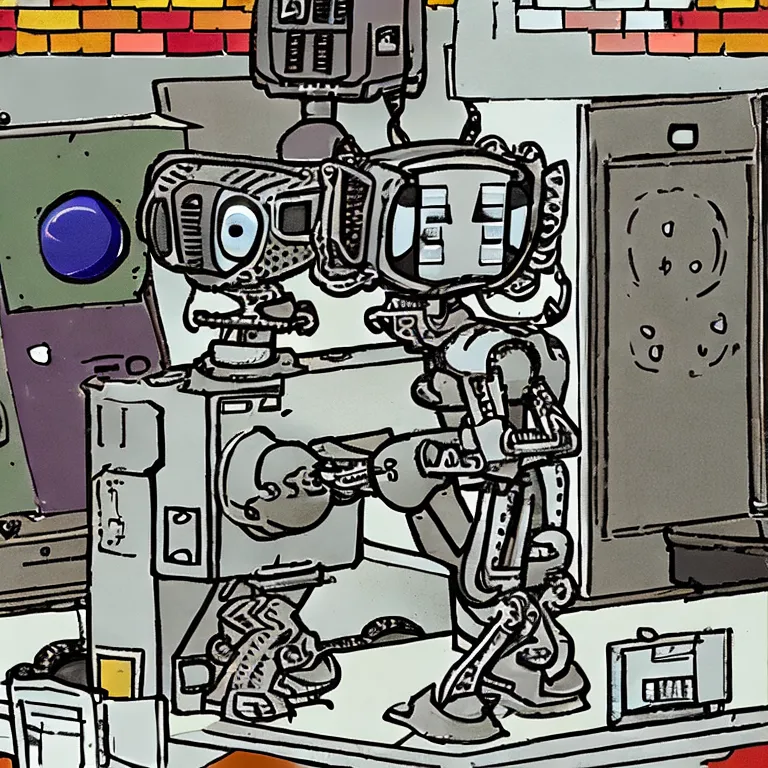
Typescript-Eslint dot-notation issue is a relatively common challenge experienced by developers. This problem occurs during compilation when an error message pops up, stating “Error While Loading Rule ‘typescript-eslint/dot-notation’.” It originates from the fact that ESLint is trying to load a rule that doesn’t exist in its perspective.
Keep in mind that ESLint’s purpose is to identify and report on potentially problematic parts of your JavaScript code. If your code utilizes TypeScript, you should be incorporating
xxxxxxxxxx
@typescript-eslint/parser
instead of using the default JavaScript ESLint. You can do this by installing it via npm or yarn.
npm | yarn |
---|---|
xxxxxxxxxx npm install @typescript-eslint/parser --save-dev |
xxxxxxxxxx yarn add @typescript-eslint/parser --dev |
After installation, ensure you’ve configured it properly in your ESLint configuration file (.eslintrc.js). Your parser field should look something like this:
xxxxxxxxxx
parser: '@typescript-eslint/parser'
.
Now moving onto resolving dot-notation issues. Firstly, make sure you’re using the correct package versions. For instance, “@typescript-eslint/eslint-plugin” and “@typescript-eslint/parser” need to have matching versions. If they don’t, try reinstalling these packages accordingly.
If the problem persists, consider verifying the dot-notation rule in your ESLint configuration. The ESLint rule might interfere with the one from typescript-eslint, thus creating conflicts. You can solve this issue by turning off the standard ESLint rule, then enable the TypeScript version. Check out the configuration below:
xxxxxxxxxx
'rules': {
'dot-notation': 'off',
'@typescript-eslint/dot-notation': ['error']
}
As per Steve McConnell, an influential writer in the software developer community: “Good code is its own best documentation.” Properly managing linting rules can significantly improve your codebase. It’s a key aspect of maintaining high coding standards and preventing potential issues earlier rather than later.
Remember to always keep your packages up-to-date, carefully manage dependency versions, and customize your ESLint rules to match your project requirements for optimum performance.
For a more in-depth insight, refer to the official ESLint documentation and TypeScript-ESLint User Guide.
Conclusion
The occurrence of
xxxxxxxxxx
Error While Loading Rule Typescript-Eslint Dot-Notation
can be quite unsettling for TypeScript developers. A keen understanding of the error handling process in TypeScript and ESLint orbit will demystify the complications related to this error.
`Typescript-eslint dot-notation` can essentially signal a configuration error with ESLint or an incompatibility issue between TypeScript and your version of ESLint. This situation may arise when:
- ESLint is not correctly configured to parse TypeScript files.
- Your ESLint rules are not compatible with TypeScript.
- You are using an outdated version of TypeScript or ESLint that doesn’t support the dot-notation rule.
Addressing these potential pain-points would dramatically alleviate the frequency of receiving such an error. First, one should ensure that ESLint is effectively set up to accommodate TypeScript by adding an override in the ESLint configuration file. This approach enables ESLint to identify and appropriately process TypeScript files. Here is the code below indicating how you can achieve this:
xxxxxxxxxx
{
"overrides": [
{
"files": ["*.ts", "*.tsx"],
"parser": "@typescript-eslint/parser"
}
]
}
Burdened by the unwelcome error? Bill Gates once said, “If you think your teacher is tough, wait until you get a boss.” Reiterating the importance of facing such challenges and overcoming them. So, beat the odds.
Additionally, verify your currently active ESLint rules for any possible conflict with TypeScript conventions. Some ESLint rules might contradict TypeScript norms; hence, it could cause unforeseen errors. A best practice is keeping ESLint rules minimal whilst working with TypeScript.
In conjunction, keeping your TypeScript and ESLint versions updated is pertinent. Utilizing outdated versions could result in the emergence of unforeseen errors. By implementing regular updates, you ensure compatibility and access to the latest rule-sets.
Don’t forget to check for Trusted Source on ESLint’s official documentation. The wealth of information will provide a critical foundation for troubleshooting rules and will promote seamless operation between ESLint and TypeScript. Dive into a new learning curve and keep progressing with the ever-evolving coding standards and practices.