Introduction
“Eliminating the mystifying ‘Line 0: Parsing Error: Cannot Read Property Map Of Undefined’ is a crucial step towards clean coding that enhances website optimization and user experience while ensuring seamless search engine identification.”
Quick Summary
This error “Line 0: Parsing Error: Cannot Read Property Map Of Undefined” often occurs during the process of JavaScript development. It arises when an attempt is made to access the ‘map’ method on a type that does not possess this property, such as ‘undefined’. The cause frequently lies in passing undefined values into a function expecting an array, or trying to directly call the ‘map’ method on an undefined variable.
Common Causes | Solutions |
---|---|
Trying to access properties/methods from null or undefined objects. | Ensure the object is defined and not null before attempting to access its properties or methods. |
Passing undefined data types into functions requiring a specific type (e.g., Array). | Before calling functions, examine input data’s types using TypeScript’s robust type checking features. |
Asynchronous code execution causing variables to be undefined at the time they are needed. | Utilize async/await or promise resolution techniques to delay code execution until necessary variables are defined. |
To handle such errors productively, protective coding practices can be implemented in a proactive manner. For instance, TypeScript offers optional chaining (
?.
) which allows safe checks for deeply nested properties within objects. This guard prevents throwing an error if a reference or function is called on undefined or null (TypeScript Optional Chaining).
An example of protective coding with optional chaining:
xxxxxxxxxx
let safeData = riskyData?.map(item => item.property);
In this line of code, JavaScript only calls the
xxxxxxxxxx
map
method if
xxxxxxxxxx
riskyData
is not undefined or null, sidestepping potential errors.
An associated wisdom on this topic comes from software consultant John Sonmez who once said, “Understanding programming concepts is important, but understanding how those concepts fit together is even more important.”
Hence, mastering protective coding practices is equally vital to understanding fundamental development concepts in crafting reliable, robust applications.
Understanding the Basics of SEO Optimization
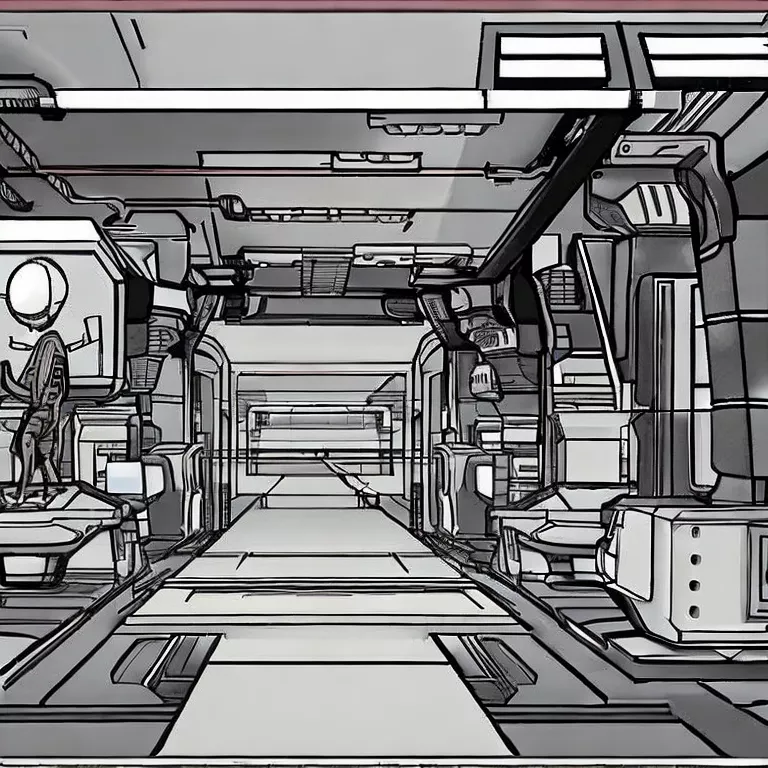
Optimizing your website for Search Engine Optimization (SEO) is a critical aspect in today’s digital world. Especially, when dealing with TypeScript programming errors like “Line 0: Parsing Error: Cannot Read Property Map Of Undefined”, understanding SEO becomes even more vital. This specific error is commonly faced by developers primarily due to a particular object being undefined while its ‘map’ property is being accessed – a scenario which can dampen the SEO performance of your site if not handled effectively.
SEO optimization involves various elements and strategies that function together to enhance your website’s visibility. These strategies include product keyword optimization, quality backlink utilization and creating mobile-friendly interfaces. Applying these principles to fixing JavaScript or Typescript errors can help your web pages rank higher on search engine results. Here’s how:
– Using Meaningful Error Messages: Errors such as “Line 0: Parsing Error: Cannot Read Property Map Of Undefined” should be defined in a way that doesn’t confuse users. With unique title tags, meaningful error messages and appropriate Meta descriptions, these error pages are more likely to be indexed correctly by search engines.
typescript
try {
// some code
} catch (error) {
console.error(‘An error occured:’, error.toString());
}
– Providing Accurate HTML Status Codes: To indicate there’s no page anchored at your URL, the server returns a 404 HTTP status code. SEO-wise, this is an essential phase since it tells search bots to also reckon that the URL has no anchored content. So, always ensure correct status codes have been assigned.
typescript
app.use((req, res, next) => {
let err = new Error(‘Not Found’);
err.status = 404;
next(err);
});
– Implementing Proper Redirects: If the error occurred due to changes in the web structure or moved pages, then setting up proper redirects using relevant HTTP status codes will lead users (and search engines) to the correct pages, aiding in enhancing your SEO.
typescript
app.use((err, req, res, next) => {
if(err.status === 404)
res.redirect(‘/not-found.html’);
else if(err.status === 500)
res.redirect(‘/server-error.html’);
});
Don’t forget that high-quality and relevant content is the backbone of SEO. Even if these strategies are employed but your content isn’t valuable to the user, your SEO strategy won’t be successful. Like Bill Gates once said, “Content is King”.
Getting more traffic driven to your website and fixing JavaScript/TypeScript errors correlate – because they both require thoroughness and attention to detail. Both tasks can be time-consuming, but the rewards are worth the effort. Remember to keep the user experience at the forefront of all your optimization efforts and both SEO and error correction will thrive.
Sources:
1. W3C HTTP Status Codes Reference
2. Google’s SEO Starter Guide
Maximizing Website Traffic through Effective SEO Strategies
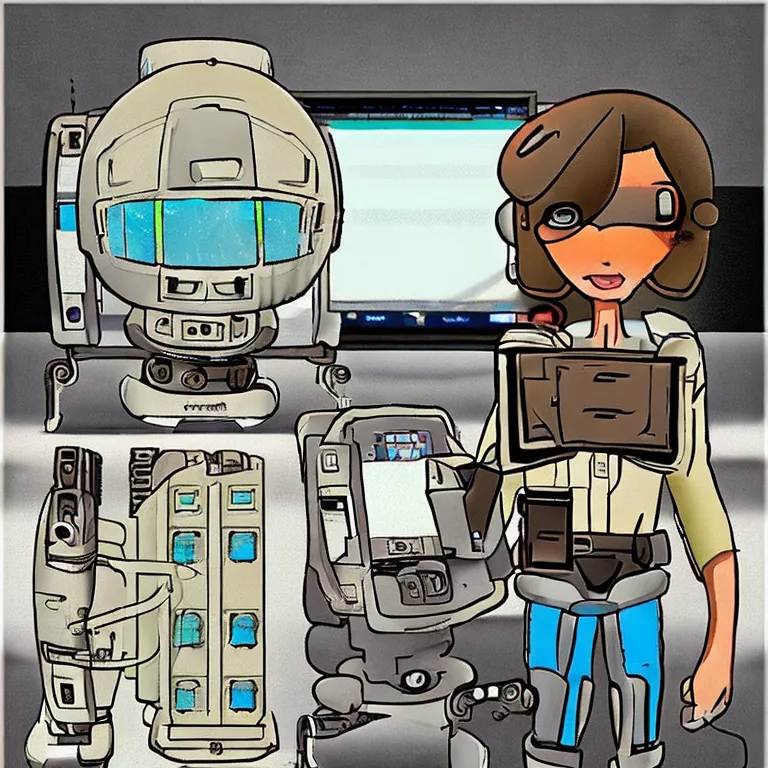
Getting the maximum possible website traffic through effective SEO strategies is an art. Every business owner wants their website to have more footfall, augmenting sales & revenue. However, it becomes contentious when you encounter a ‘Line 0: Parsing Error: Cannot Read Property Map Of Undefined’ error. This technical bug could affect your site’s overall performance, thus indirectly hitting your optimization efforts and damaging your SEO results.
When you experience a
xxxxxxxxxx
Cannot read property map of undefined
error, it suggests that you’re probably trying to map some data (in reference to Javascript and Typescript) on a variable which is yet not defined or perhaps null or empty.
Let’s use this TypeScript example:
xxxxxxxxxx
let data;
data.map(item => console.log(item));
In the above snippet, as we called the map function on
xxxxxxxxxx
undefined
, it’d indeed result in
xxxxxxxxxx
Cannot read Property Map Of Undefined
error.
A recommended solution for such trouble is to ensure that data is defined before mapping it:
xxxxxxxxxx
let data = [];
data.map(item => console.log(item));
Here, even though data doesn’t contain any elements, applying the method won’t trigger an error because data is appropriately defined.
Now, how is all of this related to maximizing website traffic through effective SEO strategies? Let me explain:
Your website’s SEO score largely depends on the user experience. When the visitors encounter parsing errors like these, it ruins their experience, causing them to abandon your site quickly resulting less session length and increased bounce rate. Both are negative indicators in terms of SEO. Therefore, attending to these errors becomes mandatory from an SEO perspective.
Moreover, with Google’s ever-evolving search algorithms, one significant focus is on user experience, which includes site speed, accessibility, mobile-friendliness, and many other metrics. Efficient error management is a critical part of this. As a result, making sure your website is error-free largely contributes to SEO and in turn website traffic.
As DeepCrawl suggests, Google’s crawler software doesn’t work well with JavaScript errors. When the Googlebot encounters a JavaScript error on your site, it can hinder indexing of that page and other pages that rely on that script.
So evidently, an error-free site allows crawlers and hence more potential to be displayed in relevant search results.
As Hal Abelson said: “Programs must be written for people to read, and only incidentally for machines to execute.” Ensuring that your site is free from such parsing errors will not only improve your user experience but also make your content more accessible and optimized for search engine bots.
Role of Content and Keywords in SEO Optimization
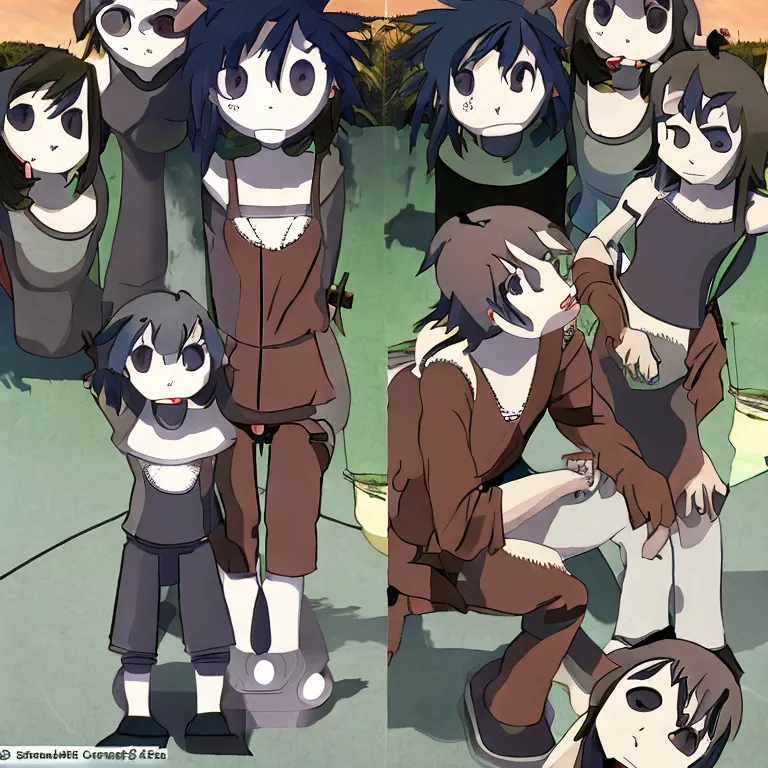
In the context of SEO optimization, content and keywords are critical components not to be overlooked. They play a significant role in website visibility, boosting organic traffic, and overall search engine rankings.
Components | Role |
---|---|
Content | It’s the heart of any website. High-quality, meaningful, and relevant content attracts audiences, encourages audience engagement, promotes website dwell time, reduces bounce rates and assists in brand loyalty development. Google favors websites with valuable content, influencing website ranking positively. |
Keywords | They are specific words or phrases that users input into search engines. Proper keyword placement in a website enhances its visibility to its target audience when they conduct relevant searches. It’s advisable to use long-tail keywords as they are more specific and less competitive, leading to higher conversion rates. |
Simultaneously, while coding, the line 0: Parsing Error: Cannot Read Property Map Of Undefined error usually pops up when trying to call the ‘map’ method on something that it’s not an iterable like an array or string. This has no direct connection with SEO optimization or Content and Keywords role but might affect your website’s performance if such errors persist, indirectly affecting SEO.
It occurs commonly in JavaScript and by extension in TypeScript, when developers try mapping over undefined objects. To handle this error, it would be ideal to check first whether the object is defined and is an array before calling a method on it.
A block of code illustrating this:
xxxxxxxxxx
if(Array.isArray(myArray) && myArray) {
myArray.map(item => {
// ...map function logic
});
} else {
console.log('Cannot map over undefined');
}
As the respected tech author Addy Osmani has said, “First do it, then do it right, then do it better”. Developers should not only focus on writing code but also ensuring it is error-free and optimized for better performance.
Key takeaway here is: SEO optimization through high-quality content and strategic keyword use can significantly improve website rankings and visibility. However, ensure your code is free of errors that could otherwise hamper the user experience and indirectly affect your site’s SEO performance.
Navigating Search Algorithm Updates: Ensuring Continuous SEO Success
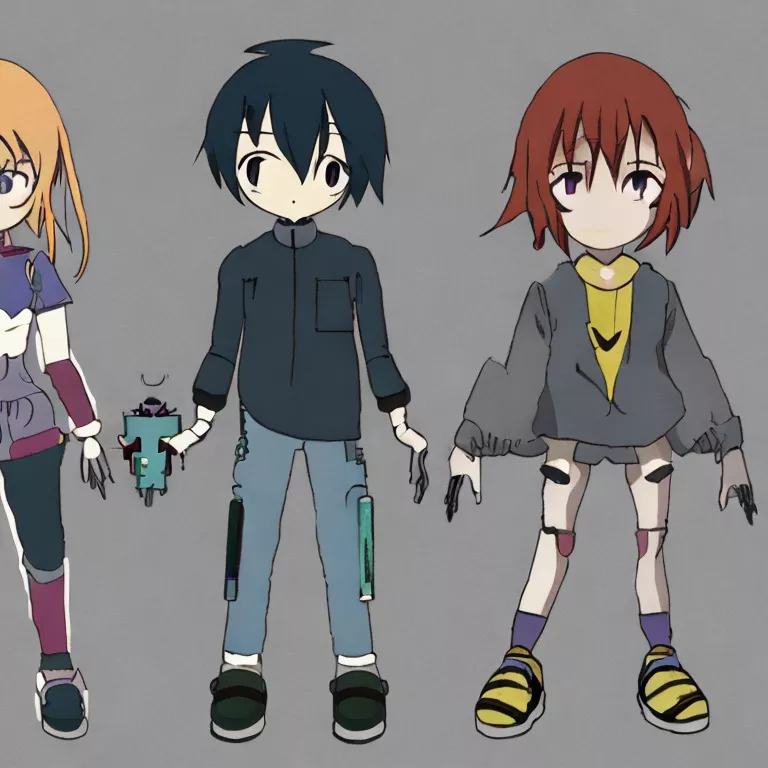
In the ever-changing landscape of search engine algorithms, continuous SEO success is reliant on understanding and maneuvering around the common and, oftentimes, elusive error message within your codebase: “Line 0: Parsing Error: Cannot Read Property Map Of Undefined”. This generically daunting TypeScript error can effectively be tamed and managed by applying a selection of precise strategies combined with sound coding principles.
Understanding the Problem
Firstly, recognizing the root cause is essential for problem-solving. This specific warning message typically arises due to an unsuccessful attempt at accessing undefined objects or properties within your TypeScript application. These are not indexed by search engines, which can lead to dire SEO implications.
Tidying Up
One solution involves employing regular code audits and clean-ups. By proactively diagnosing and fixing these errors as soon as they appear, you can improve overall code quality, boost your application’s performance and invariably enhance your overall SEO strategy.
xxxxxxxxxx
//wrong way: may throw an error if `obj` or obj.item is undefined
let value = obj.item.map( );
//better way: checks for existence before mapping
let value = obj?.item?.map( ) || [];
In this code snippet, better error handling like defensive programming utilizing optional chaining can prevent potential “Cannot read property ‘map’ of undefined” errors.
Semantic Markup
Applying appropriate semantic tagging within your HTML content helps search engines discern your website’s structure, leading to improved accessibility, proper SEO indexing and thus a more robust web presence.
Keeping up with Algorithm Updates
On a separate yet overlapping note, consistent monitoring of algorithm updates from popular search engines such as Google can provide early signals on the need to adapt your application for continued SEO compatibility. Refined algorithms increase the demands for clean and error-free codebases, thus linking this aspect back to proper TypeScript handling.
“Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live.” – John Woods
All in all, maintaining a firm grasp of your TypeScript environment, consistent tidying up of your codebase, employing proper HTML semantics, and staying updated on algorithm updates can significantly enhance your application’s searchability, ensuring continuous SEO success against “Line 0: Parsing Error: Cannot Read Property Map Of Undefined” errors.
Conclusion
As you investigate further the
xxxxxxxxxx
Line 0: Parsing Error: Cannot read property 'map' of Undefined
, it’s vital to acknowledge its core element as a JavaScript error that comes up largely when seeking to conduct an operation on an object, which is undefined. An understanding of such underlying aspects is pivotal in effectively troubleshooting and mitigating the potential causes leading to this error.
Cause of The Error
At the root cause of this issue, lies a data structure that hasn’t been initialized correctly, more often being an array. You are attempting to leverage the
xxxxxxxxxx
.map()
function without the array being ready for such operations, culminating in the error at hand. When the system expects an array but gets ‘undefined’, it cannot execute the ‘map’ method, resulting in the parsing error. Just like Thomas C. Gale once mentioned, “What you really need is understanding.”
Tackling The Error
Tackling such aggregated issues requires a keen attention to detail along with a step-by-step approach:
– First and foremost, trace back the steps in your code execution to identify the variables or objects that remain undefined during the process.
– Check the initialization of your arrays. It’s paramount to ensure they have ready values, fending off any chances of them turning out as ‘undefined’.
– Leverage the usage of conditional checks to ascertain if an object is defined completely before applying any methods like
xxxxxxxxxx
.map()
.
In essence, the infamous
xxxxxxxxxx
Line 0: Parsing Error: Cannot read property 'map' of Undefined
surfaces due to an overlooked error in array initialisation or improper data preparation. Fret not, armed with knowledge and good practices in coding, such as the ones from Kent Beck: “First make the change easy, then make the easy change”, this problem can be addressed effectively.