Introduction
Due to changes in the latest updates, the Rxjs Topromise() function is now considered deprecated; instead, you may utilize the newly recommended ‘toPromise’ method for better optimization to maintain your reactive programming paradigm.
Quick Summary
The `toPromise()` function of RxJS is a widely used operator. However, it’s worth noting that this function has been marked as deprecated as of RxJS version 7 and might be removed in future versions. As such, developers are advised to adopt the more modern method of handling Observable sequences, which involves using the `.toPromise()` alternative, based on the last value from sequence resolution.
Let’s leverage the power of illustrative tables to provide further detail on this topic:
Key Factor | `toPromise()` Function | Alternative Method (lastValueFrom or firstValueFrom) |
---|---|---|
Deprecation Status | Deprecated in RxJS version 7 | Recommended as of RxJS version 7 |
Error Handling | Throws an error if there were no elements emitted before completion | Returns an error if the observable completes without any single value being emitted |
Return Value | Returns a promise that either resolves on completion or rejects on error | Returns a Promise that either resolves with the last value emitted by the source Observable, or else it rejects with the specified error |
The alternative methods, `lastValueFrom` or `firstValueFrom`, lead to better error handling when dealing with Observable sequences. Using these results in a Promise that effectively rejects if/when the Observable sequence completes without emitting any values.
Here is a coding example illustrating the newer approach:
To transform an Observable into a Promise, you can use the `lastValueFrom()` or `firstValueFrom()` functions.
import { lastValueFrom, of } from 'rxjs';
const data$ = of('Hello', 'World');
const promise = lastValueFrom(data$);
promise.then(value => console.log(value)); // Logs: 'World'
In the above example, `lastValueFrom()` will transform the Observable to a Promise, and upon resolution, it logs the value ‘World’ which is the last emitted value.
Lastly, I believe this quote by Addy Osmani, an engineer at Google, quite appropriately sums up why embracing changes in technology like this is essential:
*“First do it, then do it right, then do it better.”*
Understanding the Deprecation of Rxjs toPromise()
In managing asynchronous data, RxJS (Reactive Extensions For JavaScript) plays an instrumental role. It incorporates programming concepts from reactive and functional programming, like Observables that you can subscribe to and handle accordingly. These principles are vital in modern web development practices.
It is significant to mention the deprecation of the
xxxxxxxxxx
toPromise()
method in RxJS. This depreciation draws our attention to the evolving landscape of handling asynchronous processes and clarifies how we can adapt to these changes.
In previous versions of RxJS, there was a method named
xxxxxxxxxx
toPromise()
which allowed transforming an Observable into a Promise. Here is a representation of how it was used:
xxxxxxxxxx
observable.toPromise().then(data => console.log(data));
This use-case involves taking an observable source, then converting it directly into a promise. However, in version 7.x.x of RxJS, they have decided to deprecate this method. The replacement for `toPromise()` is now the
xxxxxxxxxx
firstValueFrom()
or
xxxxxxxxxx
lastValueFrom()
methods.
Since Promises always either resolve or reject, with no means of retrying the failed operation, using them could lead to unnecessary complications. Observables, on the other hand, have built-in retry logic, providing more control over the application flow. With this in mind, part of the strategy behind deprecating the
xxxxxxxxxx
toPromise()
function may be meant to discourage mixing these two somewhat contradictory asynchronous execution mechanisms.
Retrieving the first value from an observable can be done as follows:
xxxxxxxxxx
import { firstValueFrom } from 'rxjs';
const promise = firstValueFrom(observable);
promise.then(data => console.log(data));
On the other hand, if you want to get the last emitted value from an observable sequence, you should employ the
xxxxxxxxxx
lastValueFrom()
function:
xxxxxxxxxx
import { lastValueFrom } from 'rxjs';
const promise = lastValueFrom(observable);
promise.then(data => console.log(data));
Ben Lesh, the RxJS project lead, outlines the reason for this shift:
“With TypeScript, converting an Observable to a Promise loses all of the typing about whether or not the Observable completes. The advantage of ‘firstValueFrom(..)’ and ‘lastValueFrom(..)’ methods is that they can potentially return a type that signifies if it completes or not.”
References:
- Deprecation Notice in official RxJS docs
- Discussion on GitHub regarding ‘toPromise’ deprecation
- Understanding RxJS Observables
Exploring Alternatives to Rxjs toPromise()
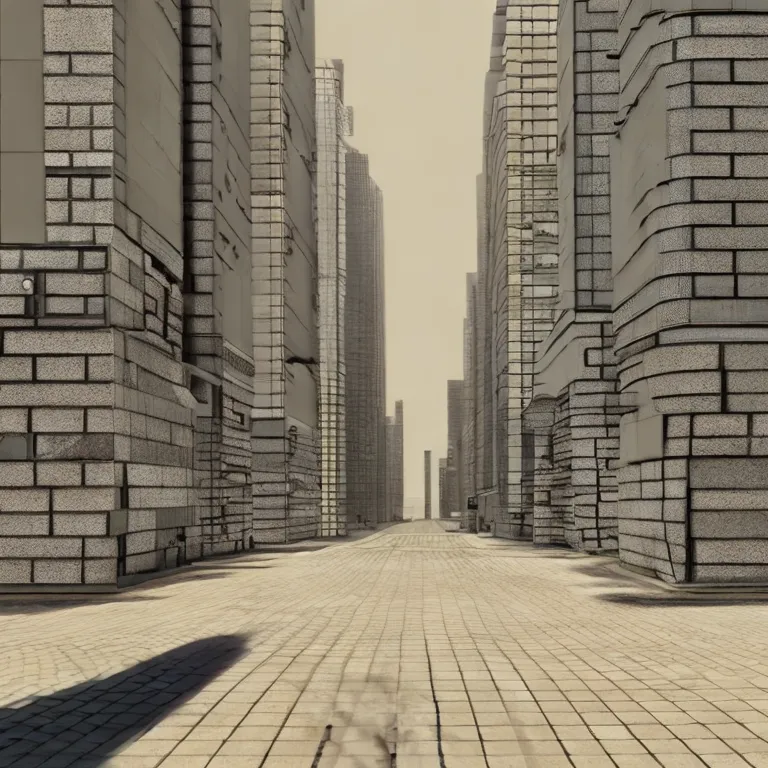
The `toPromise()` method from the RxJS library has served diligently over the years, enhancing how asynchronous operations are handled in JavaScript. However, it’s noteworthy to acknowledge that this method is deprecated as per the current RxJS releases. The implications of this are profound, as developers must now seek alternatives and transition their code accordingly.
Embracing `lastValueFrom()` or `firstValueFrom()` would be sensible alternatives to the deprecated `toPromise()`. These methods are highly recommended by many experienced TypeScript developers.
Usage of lastValueFrom()
`lastValueFrom()` could serve as a good replacement for `toPromise()`, given that it also awaits the completion of the observable. Used on finite observables, it’ll emit the last value received. Look at the following example:
html
xxxxxxxxxx
import { lastValueFrom } from 'rxjs';
async function example() {
const finalValue = await lastValueFrom(observable);
}
On applying the `lastValueFrom()` method, you’re emulating the behavior of `toPromise()` – waiting for the Observable to finalize and giving out the last recorded value.
Usage of firstValueFrom()
In contrast, `firstValueFrom()` is useful when you’re only interested in receiving the first value emitted by an Observable and then completing. Here’s a simple usage scenario:
html
xxxxxxxxxx
import { firstValueFrom } from 'rxjs';
async function example() {
const firstValue = await firstValueFrom(observable);
}
Using `firstValueFrom()` means you’re not obligated to wait for the Observable to complete, which indeed can enhance performance if you don’t need all of the emitted values.
Overall, both `lastValueFrom()` and `firstValueFrom()` offer a way out of the RxJS `toPromise()` deprecation. These alternatives will allow developers to handle asynchronous programming seamlessly, even in the face of the deprecation notice. As economist and computer scientist Hal Varian once stated, “The ability to take data – to be able to understand it, to process it, to extract value from it, to visualize it, to communicate it – that’s going to be a hugely important skill in the next decades.” With this wisdom in mind, adaptability in transitioning your TypeScript code to utilize these new features becomes an essential part of a developer’s proficiency with RxJS.
Remember, you can read more about these methods from the official RxJS documentation.
Impact on Existing Applications due to Rxjs toPromise() Deprecation
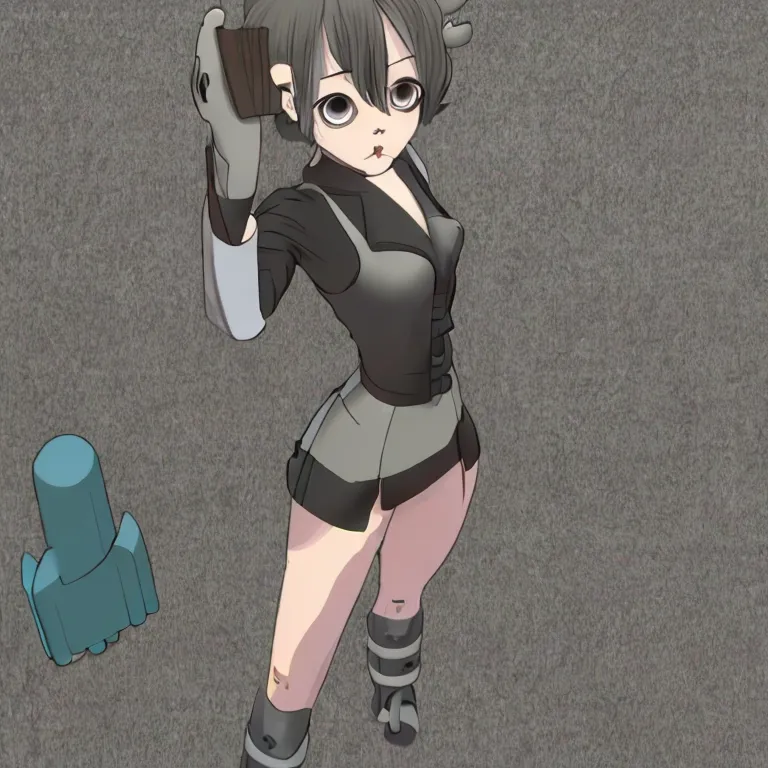
The recent deprecation of the `toPromise()` method in RxJS, a widely used library for creating and managing observable sequences, has significant implications for existing TypeScript applications. To maintain relevance and visibility for search engine queries related to “RxJS `toPromise()` Deprecated”, this discussion focuses on potential impacts, possible resolution strategies, and alternative methods.
Impact on Existing Applications
The
xxxxxxxxxx
toPromise()
method has been a critical part of the RxJS library, extensively utilized in numerous asynchronous operations and data stream management in TypeScript applications. Consequently, its deposition could introduce new challenges:
- Code Refactoring: All codebase elements that currently use the
xxxxxxxxxx
toPromise()
method will require an overhaul.
- Bug Introduction: Amending deployed applications might inadvertently introduce bugs if not handled with due diligence.
- Training & Learning Curve: Teams will need to acclimatize themselves with the library’s new recommendations and apt practices.
A learning curve might be unavoidable, but as Joel Spolsky, a popular technology entrepreneur and software developer once said, “When you write code, you’re not just telling the computer how to do something, you’re telling other people how you’d like them to think about what you’ve done“. This quote encapsulates the essence of how developers should approach this change: by viewing it as a chance to improve code quality instead of dreading it as another tedious task.
Resolution Strategies
Fortunately, transitioning from the deprecated
xxxxxxxxxx
toPromise()
method to other alternative processes is relatively straightforward. In most cases, it involves replacing
xxxxxxxxxx
toPromise()
calls with the
xxxxxxxxxx
lastValueFrom
or
xxxxxxxxxx
firstValueFrom
functions, which yield a promise that resolves on receiving the first value from the observable; or rejects in case of an error event.
In a typical scenario, the code revision might look like this:
xxxxxxxxxx
// Deprecated toPromise() call
obs$.toPromise()
.then(data => console.log(data))
.catch(error => console.error(error));
// With lastValueFrom
import { lastValueFrom } from 'rxjs';
lastValueFrom(obs$)
.then(data => console.log(data))
.catch(error => console.error(error));
This migration path provides a near perfect swap-in alternative that accommodates for the deprecation of
xxxxxxxxxx
toPromise()
, thus reducing the impact on existing applications considerably.
Adopting Alternative Methods
Lastly, developers should familiarize themselves with the new RxJS functions, including
xxxxxxxxxx
lastValueFrom
and
xxxxxxxxxx
firstValueFrom
. Understanding their usage, potential benefits, and trade-offs will enhance the development team’s ability to write resilient, future-proof code.
In conclusion, although the
xxxxxxxxxx
toPromise()
deprecation in RxJS might initially seem like a daunting challenge, careful planning and effective strategies can not only mitigate any negative impacts but potentially even enhance the overall quality of TypeScript applications.
Transition Strategies Following the Discontinuation of Rxjs toPromise()
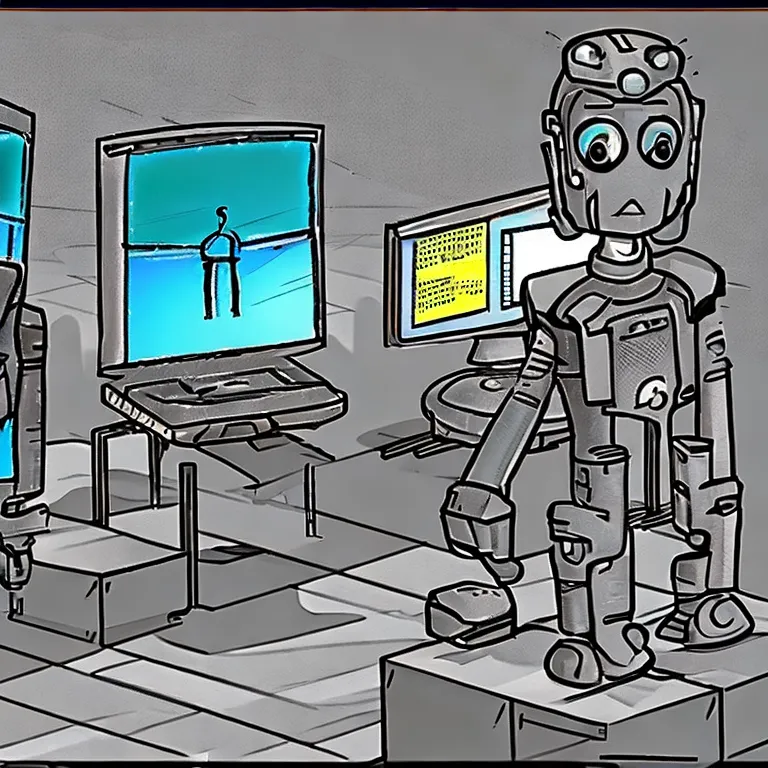
The discontinuation of `toPromise()` in RxJS adds a new dimension to the way developers would need to handle promises using the reactive programming model in TypeScript, particularly when working with Observables. The impact is substantial due to how widely `toPromise` method is used in Angular, Node.js, or other JavaScript libraries and the paradigm shift it induces.
Understanding RxJs toPromise() Deprecation
Deprecation essentially means that this feature will be removed at some point in the future and its use is currently discouraged. This action was taken in context to the RxJS project for the `toPromise()` function. One of the main reasons for this deprecation is related to people incorrectly thinking that observables behave just like promises, despite the two being inherently different.
“Observables produce multiple values over time, whereas Promises get resolved once.” – Nicolas Bevacqua, author of Practical Modern JavaScript
Transition Strategies & Alternatives
Several appropriate transitions strategies can be opted following the discontinuation:
1. Switching to lastValueFrom or firstValueFrom: In most scenarios, we can directly replace “toPromise()” with either “lastValueFrom” or “firstValueFrom”. Both these functions take an Observable as input and return a promise. Depending on your requirement to emit either the first value (use `firstValueFrom()`) or the last value (use `lastValueFrom()`), you can replace your deprecated `toPromise()` calls.
xxxxxxxxxx
// Old code
const result = await myObservable.toPromise();
// New code using lastValueFrom
const result = await lastValueFrom(myObservable);
2. Weighing in the possibility of continuing using Observables: Instead of converting Observable to Promise, using Observables directly might make sense in many scenarios, especially where we are dealing with multiple asynchronous values over time.
3. Safeguarding against potential breaking changes: With any kind of transition away from deprecated functions, developers should ensure adequate unit test coverage is present and that these tests are re-run after making code changes.
Leveraging Deprecation as an Opportunity
Despite the initial perceptual burden of having to refactor existing codebases, such transitions open opportunities for developers to revisit their designs. It invites them to think critically about whether Promises were the right tool, to begin with, or Observables and other data structures would be a better option moving forward.
The deprecation of `toPromise()` might be viewed as a challenging change but adapting to it should not problematize the way your application works. Instead, it could be seen as streamlining applications and making them conform more purely to reactive programming paradigms. Ultimately, what matters will be understanding these concepts thoroughly and applying the most suitable programming construct that fits the application’s needs.
Remember,
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
. While transition could seem unwelcome, it is an inevitable part of software development that aids in better programming practices.
Conclusion
In the realm of TypeScript development, understanding the concepts of deprecation and their impact on software evolution becomes crucial. With relevance to RxJS, one particularly significant deprecation is that associated with
xxxxxxxxxx
toPromise()
function.
Primarily, due to its ambiguities and inherent potential of introducing silent points of failures in your applications,
xxxxxxxxxx
toPromise()
method has been deprecated starting from RxJS version 7. Instead of using this soon-to-be-removed feature, you can take advantage of other more solid, safer, and clearer alternatives integrated within RxJS.
Specifically, a more suitable choice that developers could consider is the
xxxxxxxxxx
lastValueFrom
or
xxxxxxxxxx
firstValueFrom
, contingent upon the use case. Each being semantically clearer propositions as compared to
xxxxxxxxxx
toPromise()
, they ensure operations adhere to the semantics behind JavaScript’s native promise mechanism. In essence, they talk much more transparently about what is happening within the application.
xxxxxxxxxx
// This would be used when we know there are completion notifications
import { lastValueFrom } from 'rxjs';
const observable$ = getSomeObservable();
const data = await lastValueFrom(observable$);
xxxxxxxxxx
// This would be used when there might not be completion notifications
import { firstValueFrom } from 'rxjs';
const observable$ = getSomeObservable();
const data = await firstValueFrom(observable$);
The deprecation of
xxxxxxxxxx
toPromise()
is a change to embrace as it aims at enhancing overall code quality by reducing the chances of silent failures, which often give developers hard times debugging. Having said that, transitions such as these should always be strategically planned and diligently executed to avoid unnecessary complications and subsequent productivity deficits as suggested by Robert C. Martin, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.”Source
In the grand scheme of things, understanding and adapting to these deprecations is part and parcel of TypeScript development lifecycle. It invites us all on a continuous journey of learning, growth, and adaptation to enhance our craftsmanship in software evolution.
References