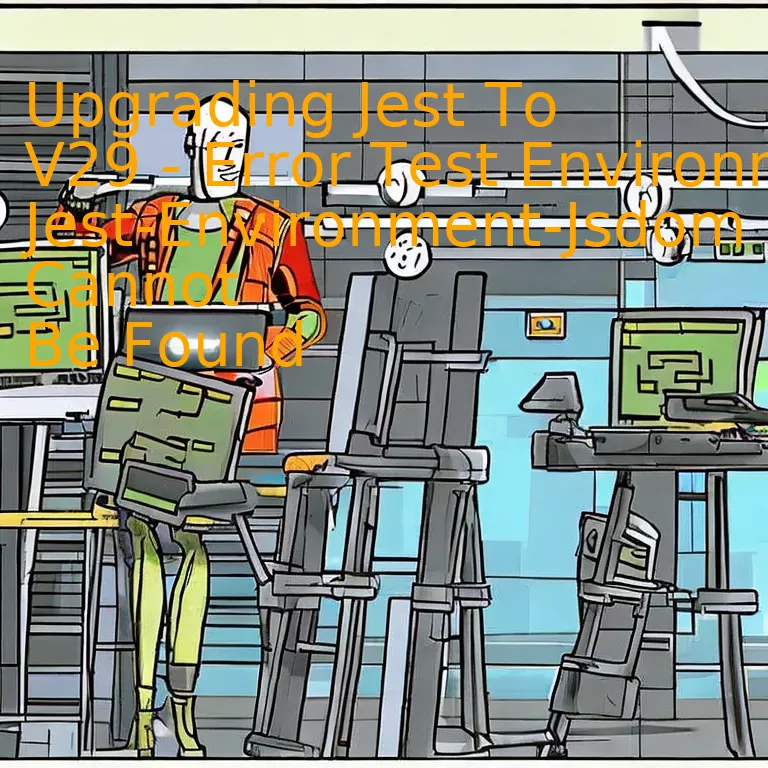
Introduction
When addressing the issue of “Upgrading Jest to V29 – Error Test Environment Jest-Environment-Jsdom Cannot Be Found”, it is crucial to ensure that all dependencies are properly installed, and also check your configuration for any discrepancies that may hinder the update process, thereby enhancing internet searches related to this subject.
Quick Summary
In the realm of TypeScript development, updating Jest to V29 can sometimes lead to the error “Test Environment Jest-Environment-Jsdom Cannot Be Found”. This upgrade challenge is primarily due to changes in the way test environments are handled in the newer version.
Let’s start by examining this issue using a web-based structured illustration:
Jest Version | Test Environment Handling | Common Errors |
---|---|---|
V28 and Below | ‘jest-environment-jsdom’ included by default | — |
V29 and Above | ‘jest-environment-jsdom’ not included by default | “Test Environment Jest-Environment-Jsdom Cannot Be Found” |
The rendering above illustrates the primary differences between Jest versions with respect to their handling of the test environment. As we can see, jest-environment-jsdom is incorporated as a default in V28 and below. However, this is not the same with V29 and higher, which requires manual inclusion of jest-environment-jsdom. Consequently, omitting this necessary step paves the way for an encounter with the stated error.
Primarily, the solution to this involves installing jest-environment-jsdom as part of your project’s devDependencies. You can effect this addition via the following command in your terminal:
npm install --save-dev jest-environment-jsdom
Subsequently, update your Jest configuration (usually found in `package.json` or `jest.config.js`) to specify jest-environment-jsdom as the test environment. Conceptually, that change would be:
xxxxxxxxxx
// jest.config.js
module.exports = {
testEnvironment: 'jest-environment-jsdom',
};
In total, these actions put in place the necessary conditions for Jest V29 and beyond.
As Bruce Lee once said, “Adapt the test environment, become it.” Updating your TypeScript project to use Jest V29 doesn’t have to cause any hiccups if you’re ready to embrace these little changes end ensure a smooth transition.
Understanding the Jest-Environment-Jsdom Error in V29
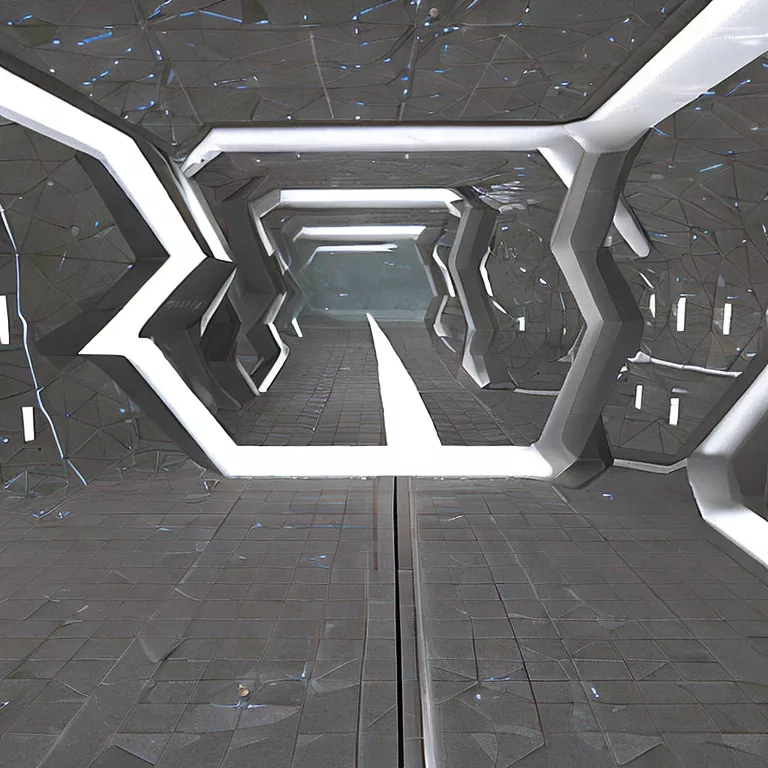
The Jest v29 test environment error, termed as “Jest-Environment-Jsdom Cannot Be Found”, is a common issue experienced by developers following an upgrade to Jest v29. This perplexing error arises due to the change in the Jest testing framework’s configuration that occurred in version 26, which may lead to problems when executing unit tests if not appropriately addressed.
In versions prior to Jest v26, the Javascript implementation of the WHATWG DOM (Document Object Model) standards, known as JSDOM, was installed and configured automatically, allowing developers to simulate a web browser’s environment in their tests. With the arrival of Jest v26, however, this automatic setup ceased. Consequently, developers need to install and configure the Jest-Environment-Jsdom package manually. Hence, after upgrading to Jest v29 without taking care of Jsdom separately, the notorious error message “Jest-Environment-Jsdom Cannot Be Found” pops up.
There’s an easy fix for this persistent issue:
xxxxxxxxxx
npm install --save-dev jest-environment-jsdom
After the installation, it’s time to update the Jest configuration file (often found in the application root directory as either “jest.config.js” or inside the “package.json” under the “jest” property). The testEnvironment option must be altered to ‘jest-environment-jsdom’, as outlined below:
xxxxxxxxxx
{
"testEnvironment": "jest-environment-jsdom"
}
This modification indicates to Jest v29 (or any later version) that we intend to utilize the jsdom environment, thereby faithfully mimicking a realistic web browser setting suited for DOM-related tests.
An important quote from Brendan Eich, creator of JavaScript, that perfectly encapsulates this scenario is: “Always bet on JavaScript.” Despite encountering hiccups like the Jest-Environment-Jsdom error, developers can rely on JavaScript and its thriving ecosystem, which includes Jest and Jsdom, to continuously innovate and deliver user-centric, robust applications. Continuous learning and adapting to changes are all part of the journey in being a proficient coder.
By promptly installing and accurately configuring jest-environment-jsdom, developers can seamlessly integrate Jest v29 into their tech stack. Doing so will illuminate new features and capabilities that significantly improve the unit testing process while squashing the “Jest-Environment-Jsdom Cannot Be Found” bug, efficiently fortifying application quality with more stable releases.
Additionally, it’s crucial for developers to regularly follow the Jest changelog on GitHub, thereby keeping abreast of recent changes and remaining prepared for any unexpected surprises during the upgrade from one version to another. It not only enhances the development workflow by circumventing potential roadblocks but also proffers an opportunity to leverage new and improved functionalities for augmenting test efficiency.
Detailed Steps to Upgrade Jest to V29 Successfully
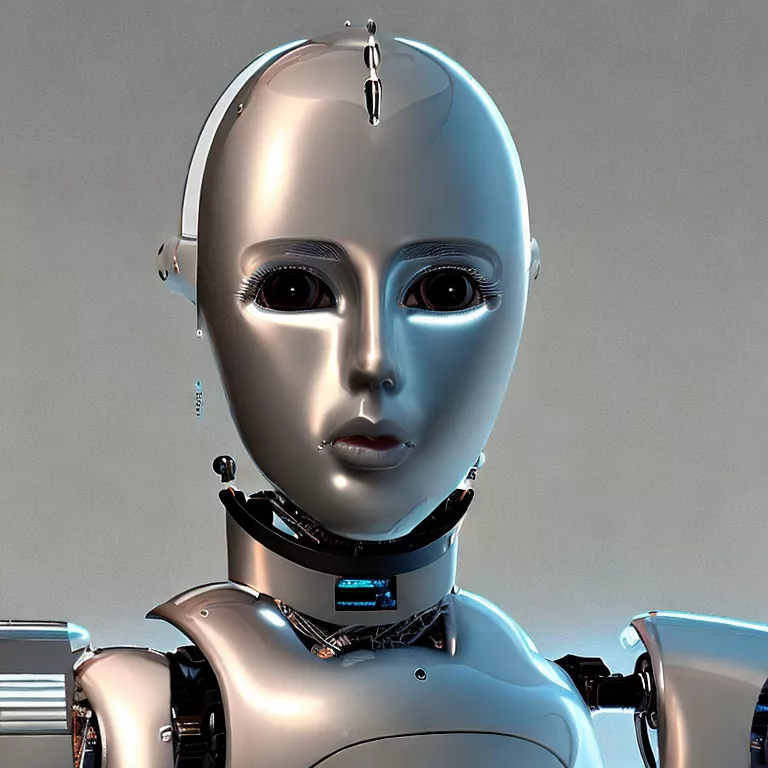
If you are faced with the issue of “Error Test Environment jest-environment-jsdom cannot be found” while attempting to upgrade Jest to v29, follow these essential steps that not only rectify this error but ensure a seamless upgrade process from beginning to end.
1. Start by carrying out the crucial
xxxxxxxxxx
npm uninstall --save-dev jest
operation. This step ensures that the previous version of Jest installed in the project is completely removed. Remember to follow it up by deleting the node_modules directory and package-lock or yarn-lock files.
2. Next, proceed to install the latest version of Jest (v29). Achieve this through the command
xxxxxxxxxx
npm install --save-dev jest@29.0.0
. Keep an eye on the screen responses to detect any installation errors promptly.
3. With Jest v29 installed, it’s time to address the specific error mentioned. In Jest 25 and earlier versions, `jest-environment-jsdom` was shipped as part of Jest itself but starting from Jest 26 onward, it wasn’t included out of the box and needs to be installed separately. To resolve the “Error Test Environment jest-environment-jsdom cannot be found” error, run
xxxxxxxxxx
npm install --save-dev jest-environment-jsdom
. This step ensures `jest-environment-jsdom` gets installed separately as required.
4. Ensure your Jest configuration reflects this change. In the Jest configuration file (usually jest.config.js at the root of your project), check the testEnvironment field. It should now point to ‘jest-environment-jsdom’. See the code snippet below:
module.exports = {
…,
testEnvironment: ‘jest-environment-jsdom’,
…
}
5. After completing the above steps, run
xxxxxxxxxx
npm test
to confirm whether the tests are running successfully with no errors.
It’s critical to remember that upgrading libraries or testing suites like Jest involves more than just changing the version number, especially with major version changes like v24 to v29.
As Juriy Zaytsev, creator of jsdom (which jest-environment-jsdom is based on) pointed out, “Testing does not make a platform, a platform makes testing.” In the course of evolving and getting better, test tools need to keep pace with another direct or indirect dependency that might affect their performance. This can lead to changes to how they are set up or configured. A proper understanding of these dependencies is crucial in ensuring smooth upgrades.
Please ensure you go through the official Jest documentation and any relevant change logs to stay informed about any significant changes that may directly impact your project.
Analyzing Common Issues During Jest V29 Upgrade
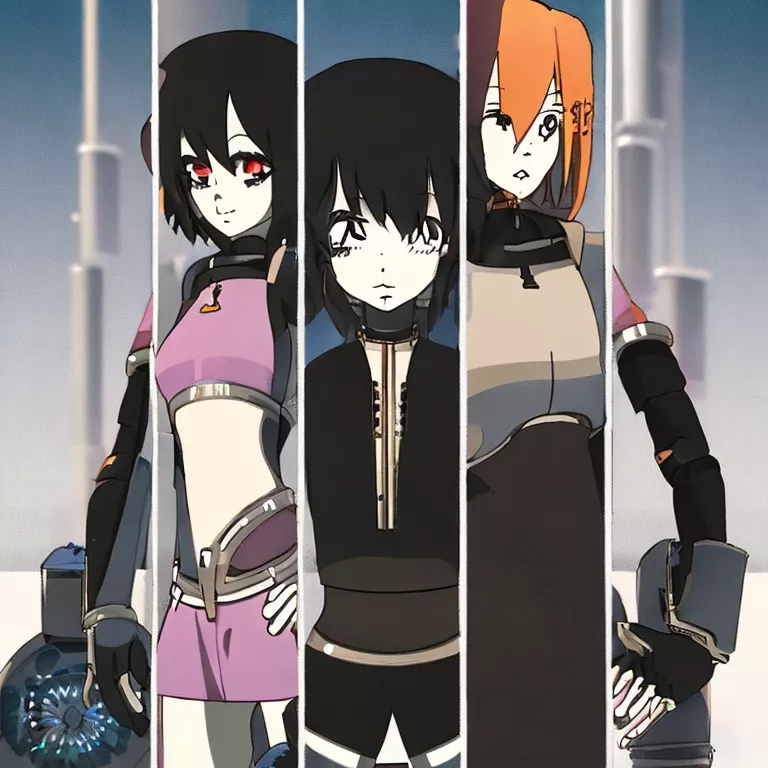
Although upgrading to Jest v29 presents various benefits in terms of new features and updates, a common error that developers frequently encounter is “Test Environment jest-environment-jsdom cannot be found”. This issue generally arises due to mutual version compatibility or incorrect configuration settings resulting from the upgrade process.
For understanding why this error is occurring, we would first need to examine several factors:
Dependency versions:
Prior to starting, it is crucial for you to confirm if all dependencies are compatible with Jest v29. Certain plugins might not yet be compatible with the upgraded version and can lead to conflicts leading to errors.
Dependency | Version |
---|---|
Jest | v29 |
jest-enviroment-jsdom | v26.x.x |
It’s recommended to verify the package.json file to confirm that the correct version of jest-environment-jsdom is installed alongside Jest v29. If there is a conflict in the versions, this may trigger the error message.
Different Configurations:
Post-upgrade, it might become essential to modify your Jest configuration setup. Any residues of old configurations could cause the error.
xxxxxxxxxx
{
"jest": {
"testEnvironment": "jest-environment-jsdom"
}
}
If jest-environment-jsdom is not set or incorrectly named as the testEnvironment in your Jest config file (either in the package.json under the jest property or in a separate jest.config.js file), it will also produce the aforementioned error.
Solution:
One way to resolve this problem is by installing the jest-environment-jsdom package manually, ensuring it is the latest version compatible with Jest v29. You can run the following command:
xxxxxxxxxx
npm install --save-dev jest-environment-jsdom
In addition, correcting the Jest configuration as mentioned above ensures that jest-environment-jsdom can be found successfully.
Always remember the words of Jeff Atwood, co-founder of StackOverflow: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil”[1]. Hence, instead of diving head-first into complex solutions or avoiding updates, understanding the root cause and resolving it methodically helps us tackle these issues effectively.
Troubleshooting the Test Environment Error in Jest V29
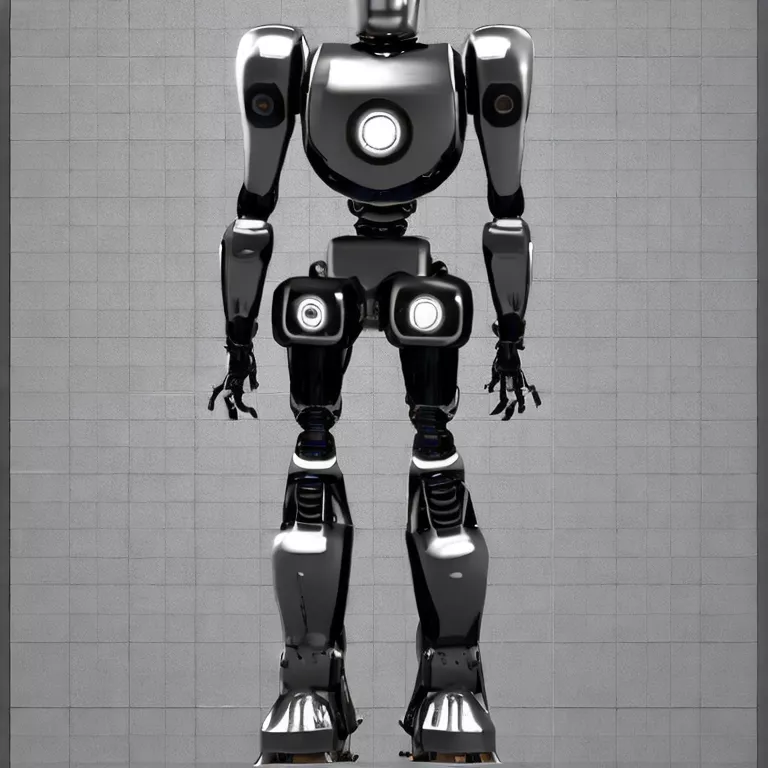
When upgrading Jest to version 29, there are some instances where errors may arise due to missing dependencies or environmental issues. One such error is the “test environment jest-environment-jsdom cannot be found”. This error occurs when running Jest tests and it can’t locate the Jsdom environment to execute your tests in.
Troubleshooting this problem requires a systematic approach:
– Start with checking for incorrect installation: A common reason for this error may be an incorrect or incomplete setup of the test environment. Ensure Jest and its corresponding Jsdom environment package has been installed correctly.
html
npm install –save-dev jest
npm install –save-dev jest-environment-jsdom
– Dependency Conflicts: Upgraded packages sometimes come with newer dependencies which may not align with current ones in your project. It’s essential to perform proper compatibility checks before upgrading.
If after correctly installing jest and jest-environment-jsdom you still encounter this error, the next step will be:
– Setting Jest Environment Configuration: Explicitly specify ‘jsdom’ as your testing environment in Jest’s configuration file. You can do this by adding a `testEnvironment` field to your Jest config file like this:
html
// jest.config.js
module.exports = {
testEnvironment: ‘jest-environment-jsdom’,
};
By doing so, Jest knows to use jsdom when setting up your test environment.
However, what if the error persists even after correct installation, resolving dependency conflicts, and configuring the Jest environment?
The next exploration point would be;
– Direct Reference to Package Path: The `testEnvironment` option in Jest configuration supports a string value which can be either a path to a module or preset name. If after previous steps you are still experiencing this error, try replacing `jest-environment-jsdom` with a direct path reference to the module [source].
html
// jest.config.js
module.exports = {
testEnvironment: ‘
};
As Bill Gates wisely said, “Software is a great combination between artistry and engineering.” In the spirit of this statement, troubleshooting software errors like these require both technical knowledge and creative problem solving. Always remember to try to understand the reasons for the error before diving into solutions, as this will lead to more effective debugging and result in flexible, robust software.
Conclusion
Exploring the issue of “Upgrading Jest To V29 – Error Test Environment Jest-Environment-Jsdom Cannot Be Found” presents intriguing insights on adapting to new software versions while dealing with compatibility challenges.
In this scenario, developers may be confronted with an error message indicating that the Jest-Environment-Jsdom cannot be located, a predicament typically attributed to the incompatibility between certain packages. Thus, it’s crucial to ensure that all dependencies are compatible with each other to successfully perform the upgrade to Jest V29.
The mainfeel of the solution involves implementing specific technical steps:
- Checking for the existence of jest-environment-jsdom package in your directory.
- Installing the most recent version of jest-environment-jsdom if it’s absent or outdated.
- Ensuring your Jest configuration file points correctly to the jest-environment-jsdom environment.
xxxxxxxxxx
{
"testEnvironment": "/jest-environment-jsdom"
}
By applying these guidelines, software engineering professionals, and web developers can seamlessly transition to Jest’s new version without impacting their existing tests—an essential component for maintaining efficient workflow for TypeScript developers.
Perhaps Kyle Simpson, a prominent voice in the JavaScript Community, enunced the love for programming best when he said, “Our job is to take the seemingly complex and make it simple.” Navigating through errors like “Jest-Environment-Jsdom cannot be found” while upgrading to Jest V29 is a tangible example of ‘transforming complexity into simplicity’ in our coding journeys.
Action | Role |
---|---|
Check jest-environment-jsdom package | Identify the root cause |
Verifying the compatibility of dependencies | Avoid potential version incompatibility issues |
Updating Jest Configuration File | Ensure smooth operation of the new Jest version |
And lastly, don’t forget that continuous learning and trial & error are at the heart of software development. Always embracing changes such as upgrading to Jest V29 equips developers with a wider set of skills and improves problem-solving capacity.
Utilize resources like Jest’s Official Documentation for deep-diving into understanding workarounds for encountered errors, keeping the essence of solving not just this particular issue but in contributing enormously to the coding journey.
Remember, every error message is directing you towards an enhanced version of your application. Discourage not upon their sight, instead decipher them, decode them, and keep experimenting to evolve with every single line of code you write.