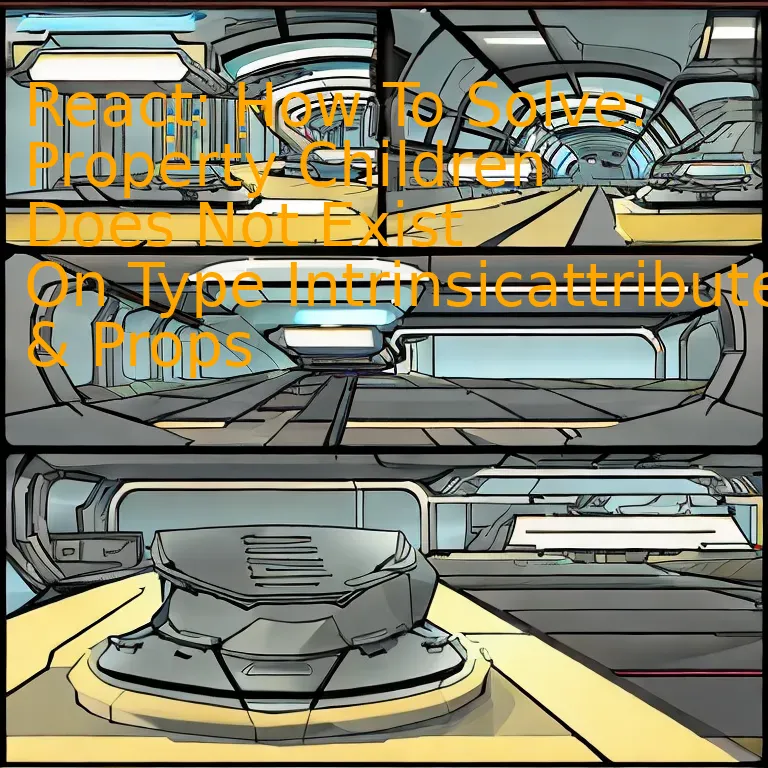
Introduction
Enhancing your React development, resolving the error: ‘Property Children does not exist on type IntrinsicAttributes & Props’, often involves a thorough assessment of component declarations ensuring correct property allocations which could drastically optimize web application performance.
Quick Summary
Let us begin by decoding the error “Property ‘children’ does not exist on type ‘IntrinsicAttributes & Props’ “. In essence, this error is emanating because TypeScript is unable to identify the props that are being passed down to a component – in this case, it’s the ‘children’ prop.
Problem | Solution |
---|---|
TypeScript cannot identify ‘children’ prop being passed down | Include ‘children’ in your type definitions explicitly or implicitly via React’s built-in types. |
To further elaborate on the solution, TypeScript needs to be aware of all possible props that a component can take. When it encounters a property that hasn’t been defined in the type declaration for the prop object, such as ‘children’, it throws the above-mentioned error.
One way to bring clarity to TypeScript about these props is to specifically include ‘children’ in your prop type definitions. An example would look like this:
type MyComponentProps = { someProp: string; children?: React.ReactNode; } const MyComponent: React.FC= ({ someProp, children }) => { ... };
Here we are explicitly including ‘children’ in our prop definitions so that TypeScript knows what to expect.
Alternatively, one could employ the built-in types provided by the React library itself. The type `React.FunctionComponent` (or `React.FC` for short) is a predefined, generic type in React which implicitly includes the ‘children’ prop of type `ReactNode`. By defining our component as a `React.FC`, we let TypeScript know that our component may have children, and those children could be any valid React node. This can be seen in the example above as well.
As Martin Fowler once stated, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” It is important to remember that the core goal when utilizing TypeScript with React is to improve readability and prevent runtime errors.
Also, while custom prop typing and use of `React.FC` both have their advantages, the choice largely depends on the specific requirements and complexity of your project. Some projects might treat ‘children’ as an exceptional case and may find it convenient to explicitly declare; others may prefer to stick to built-in mechanisms for ease of use and standardization.
Remember, our ultimate objective is clean, readable and bug-free code. Therefore, make your decision wisely based on your sense of judgement and project needs.
Understanding the Problem: ‘Property Children Does Not Exist on Type IntrinsicAttributes & Props’
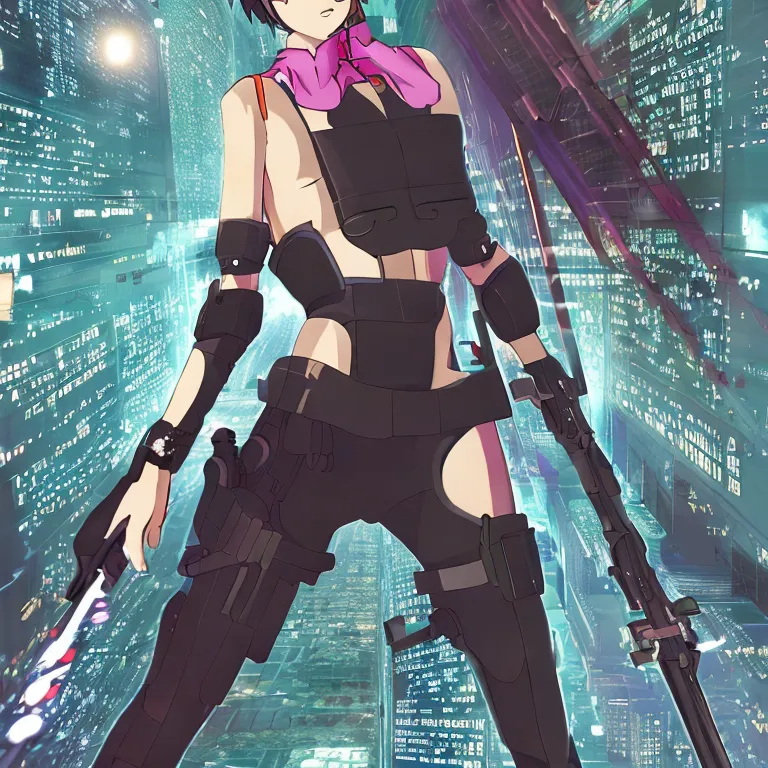
Understanding the Problem: ‘Property Children Does Not Exist on Type IntrinsicAttributes & Props’ in React
1. Introduction to the Problem:
The error message ‘Property children does not exist on type IntrinsicAttributes & Props’ typically arises when using TypeScript with the React.js library. This error suggests that your component method is unable to recognize or access the
children
property of the element being passed as a prop.
In essence, the heart of this issue lies within the scope of TypeScript’s understanding of your components and their props structure. For TypeScript to understand and interpret the properties correctly, explicit definitions must be declared for each property associated with the components. Without an explicitly declared interface, any properties not defined within the default React Component types’ interfaces (including
IntrinsicAttributes & IntrinsicClassAttributes
) would throw an error.
2. Possible Solution:
One effective solution to this problem involves creating a type definition, or interface, for your props. This interface should explicitly include the
children
property to ensure it’s acknowledged by TypeScript. Here’s a basic example:
interface IProps { children: React.ReactNode; } const MyComponent = ({children}: IProps) => <div>{children}</div>;
This code block tells TypeScript that our
MyComponent
accepts a prop called “children” which is of type
React.ReactNode
, thereby instructing TypeScript to expect any child elements to be passed to this component.
3. Deeper Considerations:
– Keep in mind that specifying the interface for your component isn’t about restricting its usage, but rather, it enhances your development experience by providing an extra layer of validation at compile-time.
– Defining interfaces for your props not only resolves this error but also improves the clarity of your code, making it easier for other developers to understand the requirements of your components.
– Always consider using React’s built-in types and interfaces (for instance,
React.ReactNode
), as they are designed to work seamlessly with common patterns in the library.
As software engineer Kent C. Dodds eloquently puts it: “Code, like a good joke, doesn’t need to be explained.” The clearer and more intuitive we can make our codes, the better it is for our peers and the health of the application development process. Key resources for further reading include insights on JSX in TypeScript and React TypeScript cheatsheets.
Diving Deeper into React and Its Key Components
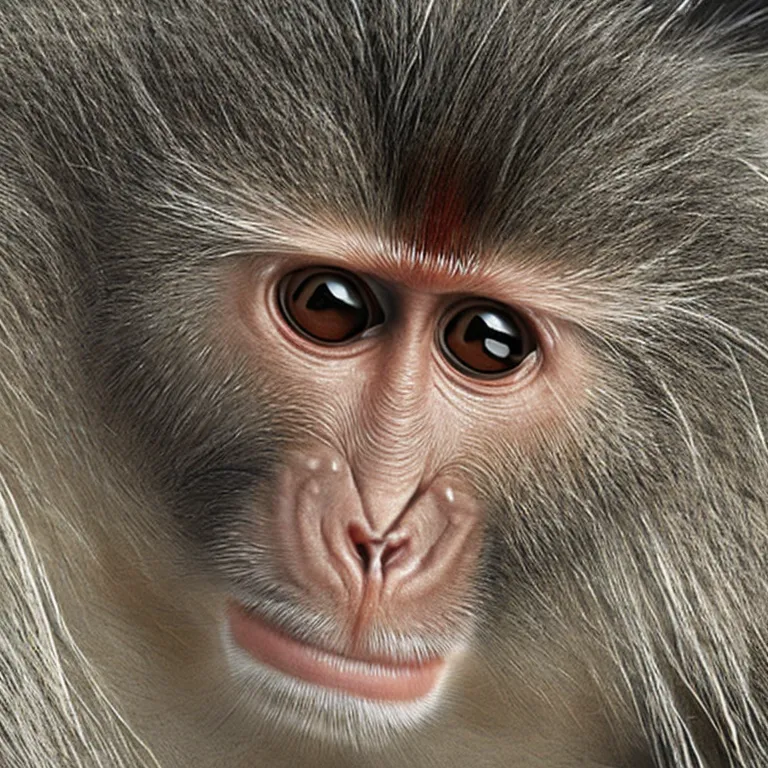
React, a Javascript library developed by Facebook, is commonly used for building user interfaces, especially for single page applications. It allows developers to create large web applications that can change data without reloading the page.
A critical feature of a good React component is its ability to accept “props” from parent components and use these “props” to render HTML or other React components. Among the most essential “props” in React is “children”, which is often used to integrate the contents between opening and closing tags when invoking a component.
However, a common issue that many developers encounter while working with this powerful library is the TypeScript-related error: “Property ‘children’ does not exist on type ‘IntrinsicAttributes & Props.” A key source of confusion in dealing with this problem derives from TypeScript’s static nature versus JavaScript’s dynamic nature. Despite React being primarily built with JavaScript in mind, it can add a new layer of complexity when integrating with TypeScript – a statically typed superset of JavaScript designed to scale JavaScript projects.
The typical cause of the “Property ‘children’ does not exist on type ‘IntrinsicAttributes & Props'” error lies in explicitly defined component types which do not include the children prop. By default, TypeScript won’t recognize the ‘children’ attribute as a valid prop unless specifically included in its Props interface. This phenomenon is entirely in line with TypeScript’s goal: ensuring adhering to strongly-typed paradigms to minimize runtime errors that JavaScript might overlook.
Taking this into account, rectifying this error becomes a straightforward affair. We have to ensure that our Props definition includes the children property explicitly if our React component intends to use it. Here’s an example:
Before fixing:
interface MyProps { name: string; } function MyComponent({name}: MyProps) { return{name}; }
After fixing:
interface MyProps { name: string; children?: ReactNode; // The children prop is included now! } function MyComponent({name, children}: MyProps) { return{name}{children}; }
In the revised approach, we’ve added the ‘children’ prop into the Props definition for our component. Now, TypeScript does not only recognize the ‘children’ prop but also ensures its content aligns with possible node elements within a React Component.
As Dan Abramov, one of the core team members of React said, “Code is like humor. When you have to explain it, it’s bad.” Integrating React and TypeScript might seem challenging initially, but as shown, solving such hurdles can lead to cleaner, more type-safe codebases that mitigate many potential errors inherent in large-scale JavaScript projects. As with any other technology adoption, the right mix of theory, practice, and understanding of underlying principles results in better design and implementation.
Remember, TypeScript isn’t a language you read – it’s a language you write. And adding ‘children’ explicitly into your type definitions is a small but significant step towards writing better, safer TypeScript codes in your React applications.
Exploring Solutions: Rectifying ‘Property Children Does Not Exist’ Error in React
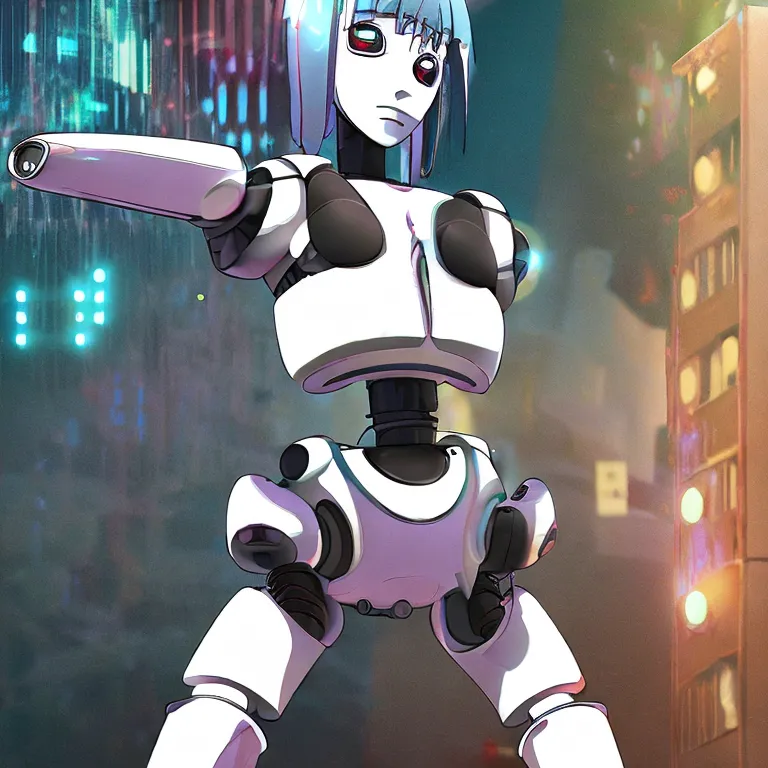
When leveraging the power of the modern React frameworks, developers may occasionally encounter problematic issues. One such issue that has been reported frequently is the ‘Property Children Does Not Exist On Type IntrinsicAttributes & Props’ error. When faced with this problem, one must not panic, as solutions are available.
First and foremost, it’s crucial to understand the cause of the problem. This specific error typically occurs when you’re trying to access the `children` prop in a TypeScript based project but haven’t actually defined that you expect to receive children. Since TypeScript exists to confirm types at compile time, when using React components with children props without disclosing this information to TypeScript, it can lead to such errors being highlighted.
To mitigate this troublesome scenario, it would be necessary to enhance the component’s Prop type to accept children. For instance:
interface IProps {
...
children?: React.ReactNode;
}
const MyComponent: React.FC= ({ children }) => (...)
Here `ReactNode` includes all possible return values for a component. Including these changes allows useState and useEffect hooks to be correctly typed as well.
Another solution could be to define your component using `React.FC` which implicitly adds the children props for you:
const MyComponent: React.FC = ({ children }) =>
As stated by Kent C. Dodds, a renowned software engineer, “Tools not used to their full capacity can turn out to be tripwires rather than escalators.” Hence, it is essential to gauge the depth of the tools we are using and leverage them to our advantage. Mitigating such problems can therefore help developers in ensuring a seamless coding journey when working with TypeScript and React.
Remember that accessing props in TypeScript requires careful attention. Leveraging the power of TypeScript in React projects significantly enhances your coding experience, improving the reliability and robustness of your codebase.
More about React/TypeScript typing can be found here.
Case Study: Practical Applications of Solutions to Common Errors
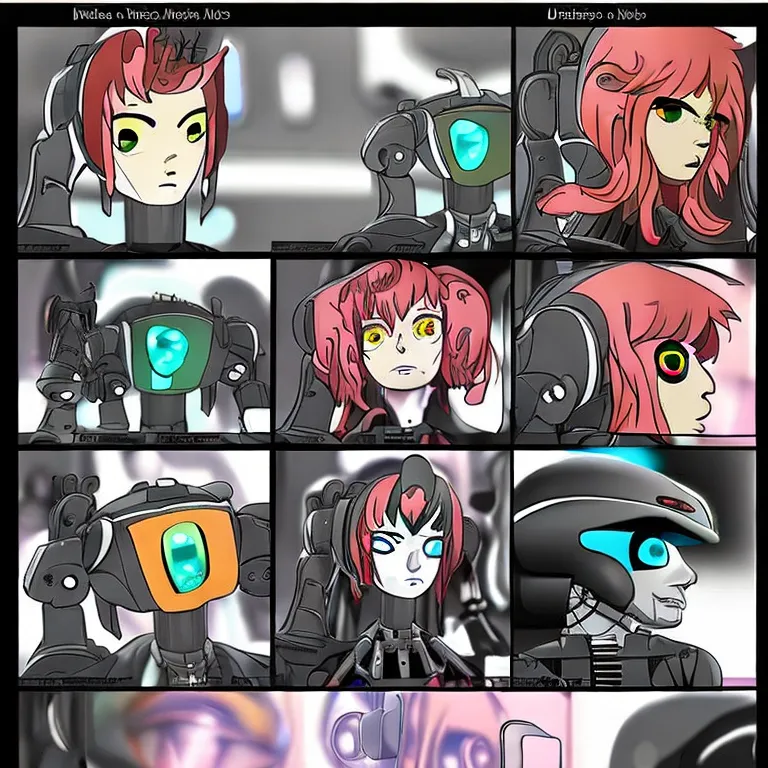
In the context of React, the error “Property ‘Children’ does not exist on type…” is a common one which happens when TypeScript is trying to type-check your JSX templates. This happens because when you create new components, TypeScript checks if the props match what’s defined in your types/interfaces. However, TypeScript doesn’t inherently know about the ‘children’ prop that React acknowledges.
For instance, let’s consider this scenario:
type AppProps = {} ; function App({}: AppProps) { return (//...some code ); }
When you try to use it in this manner:
Here's some content
You are bound to come across the aforementioned issue. To understand why this occurs, we must delve into understanding specific aspects, i.e., Intrinsic Attributes and Children Props.
Intrinsic Attributes:
These are attributes universally accepted by all JSX elements. ‘Key’ and ‘ref’ are intrinsic attribute examples React employs.
Children Prop:
This concept in React offers enormous versatility and expressiveness, allowing us to insert child elements directly into component outputs.
Therefore, to resolve this TypeScript error, the application needs to specify that a given component accepts children:
type AppProps = { children: React.ReactNode } ; function App({ children }: AppProps) { return ({children} ); }
Adding the children prop within our AppProps type allows TypeScript to acknowledge and check against this.
Following this approach will enable developers to avoid such prevalent errors, thus enhancing the practicality of TypeScript in working collaboratively with React. Discussion along these lines would ensure an efficient development environment.
As Frederick P. Brooks famously said, “The programmer, like the poet, works only slightly removed from pure thought-stuff. He builds his castles in the air, from air, creating by exertion of the imagination.”[1] Thus, effectively mitigating such errors enables programmers to realize their imaginative constructs more smoothly with React and TypeScript.
Conclusion
The React error, “Property ‘children’ does not exist on ‘IntrinsicAttributes & Props'”, can manifest as a somewhat perplexing assertion for TypeScript developers. This cryptic message is often indicative of a definitions file which is failing to recognize ‘children’ as a valid property under the existing TSX element.
Exploring and resolving this issue involves understanding the fundamental principles of TypeScript when dealing with React. This encompasses recognizing that there are two categories of JSX components in TypeScript: Function Components (React.FC) and Class Components. The ‘children’ property is typically implied in the type definition of Function Components, however, it may not be explicitly defined within Class Components. TypeScript has a somewhat stringent compilation process requiring explicit type definitions, hence the discord.
Luckily, mitigating this challenge isn’t an arduous journey but rather a swift intervention of declaring the ‘children’ property in your class component props interface.
interface YourComponentProps { children?: React.ReactNode; }
The above code snippet denotes how you could specify ‘children’ within your props, thereby satisfying the TypeScript compiler’s requirements. React’s ‘ReactNode’ type is being used here mainly because it is designed to represent any node that can be rendered in React, making it an ideal candidate to designate our ‘children’ prop.
TypeScript avails a golden opportunity for JavaScript developers to write robust and bug-resistant code. Nevertheless, there exists various quirks and caveats which could prove daunting at times, especially when venturing into JSX/TSX scenarios. Understanding these will make your overall journey towards TypeScript mastery more straightforward and less demanding.
As Edsger W. Dijkstra once said, “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” Grasping the interaction between TypeScript and React enables one to minimize the number of these bugs from inception – the true essence of effective programming.