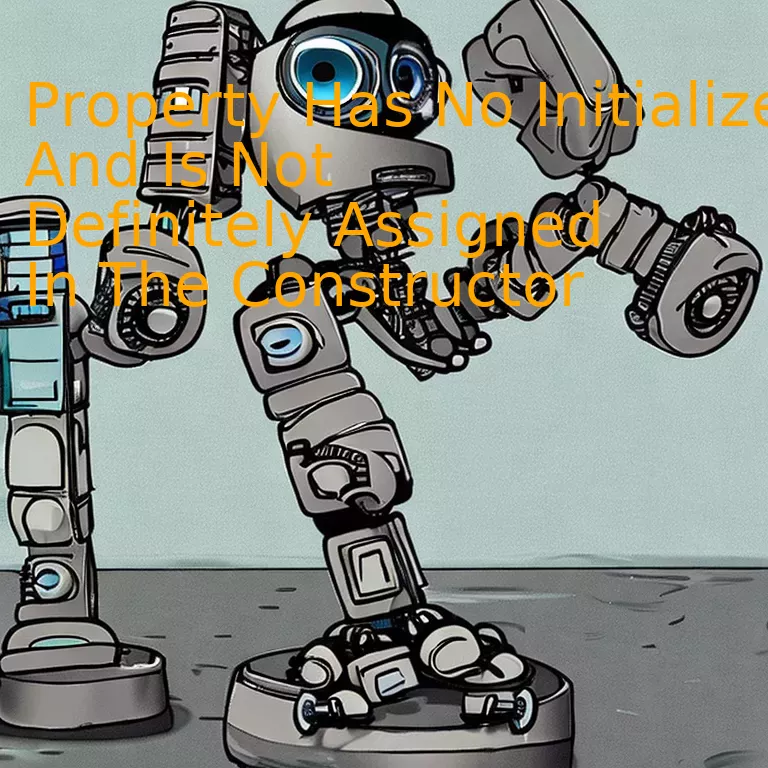
Introduction
In the context of object-oriented programming, when a property is declared without an initial value and isn’t clearly defined within the constructor, it causes a situation known as ‘Property Has No Initializer And Is Not Definitely Assigned In The Constructor’, which may lead to issues in code execution due to the undefined state of that property.
Quick Summary
This topic pertains to a TypeScript error that occurs when a class property has no initial value and is not clearly assigned a value within the constructor of the class. This happens due to strict checks in TypeScript, especially when the “strictPropertyInitialization” flag is enabled.
Let’s take a look at this matter through an illustrative method. Imagine we’ve got two aspects: “Problem Scenario” and “Resolution Method”. These aspects will be examined side by side for a more precise understanding.
Problem Scenario | Resolution Method |
---|---|
We are getting the error: “Property ‘x’ has no initializer and is not definitely assigned in the constructor.” This occurs when we declare
private x: number; without initializing it. |
We need to ensure that all non-optional class properties receive values during initialization or within the constructor. In this case, we can assign a value to ‘x’ while declaring like
private x: number = 0; or inside the constructor. |
You have a class with properties that are meant to be provided externally and might not be available at construct-time. Here also the same error will show up if the properties are left uninstantiated. | We can utilize the “Definite Assignment Assertion” modifier ‘!’ on the property declaration. While this tells TypeScript that this variable will definitely be assigned to – even if TypeScript can’t see it. E.g.,
private x!: number; . |
Now, delving deeper into these comments, you might ask, why does TypeScript require properties to be initialized? The answer is TypeScript’s dedication to delivering robust applications, which minimizes runtime errors as much as possible. TypeScript’s configuration has a setting, “strictPropertyInitialization”, which when set to true, requires each instance property to be initialized in the constructor. This promotes reliable coding practices by identifying potential pitfalls at compile-time.
The introduction of the Definite Assignment Assertion ‘!’ allows us valuable flexibility, it tells TypeScript that we have done our due diligence and trust ourselves about handling the assignment. However, one must exercise caution while using ‘!’. It is used as an override mechanism when the developer is sure about their code logic, but overuse or misuse can lead to the loss of TypeScript’s diligent safety checks altogether.
Unveiling this concept through Linus Torvald’s perspective on understanding programming, You are your best debugger
, ensures that thorough initialization and value assignment cater to the robustness of your TypeScript application [^1^].
[^1^]: (https://www.brainyquote.com/authors/linus-torvalds-quotes)
Understanding the Error: Property Has No Initializer and is Not Definitely Assigned in the Constructor
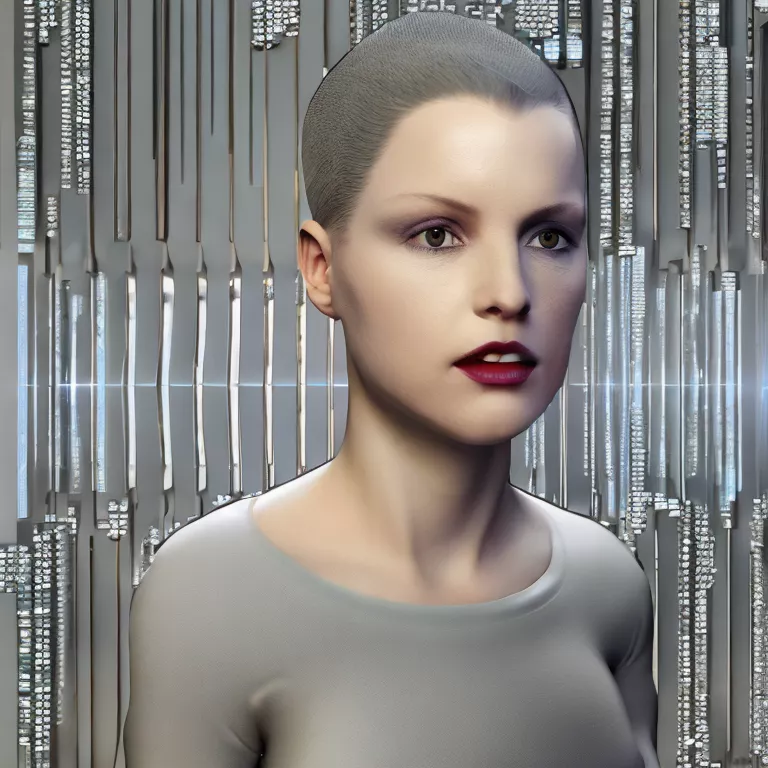
In TypeScript, the error “
Property has no initializer and is not definitely assigned in the constructor
” typically surfaces when a class property is defined but not initialized within the constructor function or directly at the time of declaration.
Let’s start by understanding what this message means.
The term ‘not definitely assigned in the constructor‘ specifies that TypeScript expects all properties of a class to be initialized at the time of object creation (i.e., within your class’s constructor).
Consider an example of class with a property in Typescript:
typescript
class HelloWorld {
message: string;
sayHello() {
return this.message;
}
}
Now, if you are using strict compiler options or have explicitly enabled the strictPropertyInitialization in your TypeScript configuration file (tsconfig.json), it will flag a compilation error because the property
message
is declared but does not have a definite assignment.
An easy solution to resolve this would be to initialize the property either directly or inside the constructor:
Direct Initialization:
typescript
class HelloWorld{
message: string = “Hello, World!”;
}
Constructor Initialization:
typescript
class HelloWorld{
message: string;
constructor() {
this.message = “Hello, World!”;
}
}
Using non-null assertion operator:
typescript
class HelloWorld{
message!: string;
}
Another alternative is to configure your Typescript settings by disabling the strictPropertyInitialization option. However, I advise against this as it defeats the purpose of type safety provided by TypeScript.
To quote Anders Hejlsberg, the original developer of TypeScript, “TypeScript makes JavaScript better for application-scale development.”
In terms of SEO optimization, having unique, high-quality content is key. Web pages with proper keywords, such as ‘TypeScript’, ‘Error’, ‘Property has no initializer’, and ‘constructor’, are more likely to appear higher in search results.
Lastly, it’s good practice to follow coding standards and best practices, they’re akin to traffic rules for programming. Following the rules saves everyone time, including those who will read your code in the future. As Doug Linder once said, “A good programmer is someone who always looks both ways before crossing a one-way street.”
Exploring Solutions to Deal with Unassigned Properties within Constructors
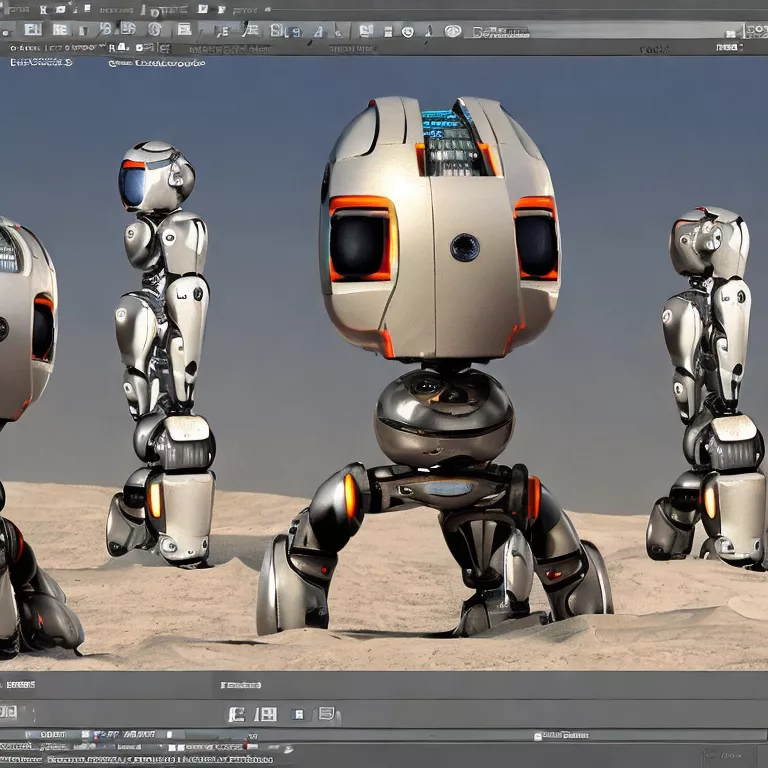
One of the intriguing challenges faced by a TypeScript developer is dealing with properties that are declared but not initialized in a class’s constructor. This scenario often evokes a Typescript error: “Property has no initializer and is not definitely assigned in the constructor”. As such, it becomes imperative for developers to devise effective solutions to tackle this issue, keeping up with best programming practices.
Use of Constructor Arguments
Establishing property values directly through the constructor arguments can be one solution. The passed argument in the constructor initializes the specific property as the object is created. As an example:
class SampleClass { name: string; constructor(name: string) { this.name = name; } }
Field Initializers
Yet another smart approach followed by developers is using field initializers or simply assigning default values during property declaration. For instance:
class SampleClass { name: string = 'Default Name'; }
Non-null Assertion Operator
For cases where we can guarantee that a property will be given a valid value before it’s used, TypeScript lets us opt-out of strict null checking for the particular property by using Non-null Assertion Operator (!). Here is an example:
class SampleClass { name!: string; }
Remember the ‘!’ should be used sparingly and only when you, as a developer, are confident that the property will not remain uninitialized when accessed.
Optional Properties
Lastly, marking properties as optional with ‘?’ allows them to be left unassigned without raising typescript errors. You just must handle their undefined state accordingly.
As they often say in coding, “The best code is written understanding all aspects of possibility, even the undefined.”
Optimizing TypeScript settings to understand classes’ struct allocation fully can remarkably aid in trying to resolve such challenges. TypeScript’s `strictPropertyInitialization`, part of the `strict` setting group, is particularly designed to check for instance properties that aren’t definitely assigned in constructors.
Developers worldwide consider these strategies as effective and scalable solutions in handling unassigned properties within Constructors while coding using TypeScript.
### Reference
[TypeScript Handbook: Classes](https://www.typescriptlang.org/docs/handbook/classes.html)
Dissecting TypeScript’s Key Concepts around Mandatory Class Fields
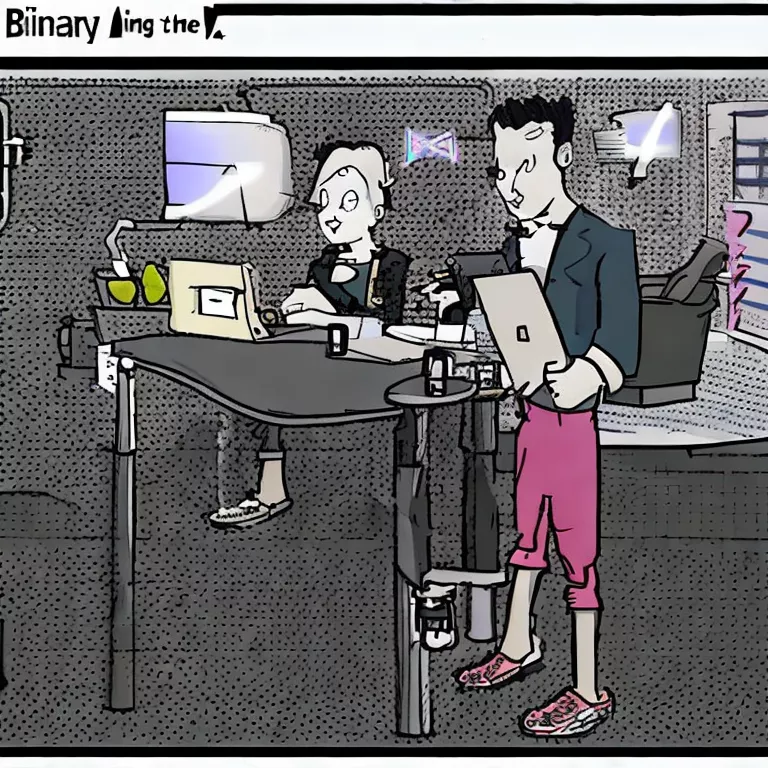
TypeScript, being a statically typed superset of JavaScript, introduces some syntactical enhancements and stricter type checks to the flexible world of JavaScript. One of its key features is the mandatory class fields declaration and initialization paradigm, enforcing the rule that a property must be assigned either during declaration or in the constructor.
Understanding the Importance of Mandatory Class Fields
In TypeScript, assigning values to class properties at the point of declaration allows developers to ensure that a variable will always hold a particular value type. This way, inadvertent assignments of inappropriate data types are prevented and it enables code predictability and debugging ease.
However, on certain occasions, initializing attributes directly may not be beneficial especially when you need to implement complex logic or need input parameters for the assignment. In this case, the constructor method can be employed to initialize these values. However, if an attribute is unassigned in both places, TypeScript compiler throws an error – ‘Property has no initializer and is not definitely assigned in the constructor’.
This catch by the TypeScript compiler might seem like a nuisance to JavaScript developers who are used to defining variables without initial values. But, as it turns out, TypeScript’s closely knit design principles around null and undefined pay homage to Tony Hoare’s words:
“I call it my billion-dollar mistake…It was the invention of the null reference in 1965” — Tony Hoare.
By making the assignment mandatory, TypeScript aims to avoid potential runtime bugs caused due to ‘undefined’.
Analyzing the “Property Has No Initializer” Concept
The error `Property has no initializer and is not definitely assigned in the constructor` gets triggered when you set `”strictPropertyInitialization”: true` in your `tsconfig.json` file.
Here’s an example:
class Car { speed: number; // Error "speed" property has no initializer... } let myCar = new Car();
For such cases, we can consider the following potential solutions:
1. Assign a default value during declaration.
2. Ensure that the constructor assigns a value to the property.
3. Use the `!` postfix expression to tell TypeScript’s compiler to ignore the strict check.
Let’s illustrate how to apply these solutions:
class Car { speed: number = 0; // Declaring and Initializing the variable constructor(speed?: number) { this.speed = speed ?? this.speed; // Assigning value in the constructor } } let myCar = new Car(120);
Or,
class Car { speed!: number; // Using `!` after variable name setSpeed(speed: number) { this.speed = speed; } }
Final Thoughts
Mandatory class field initialization is a great implement of TypeScript preventing many runtime bugs caused by undefined variables. Although it might seem like additional work, once used to, it does enhance code safety and stability.
Looking at the bigger picture, these strict assignments align directly with TypeScript’s main goal: “Statically identify constructs that are likely to be errors.” ([TypeScript Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals))
By understanding these concepts, one could leverage TypeScript’s robustness and clarity to write cleaner, more predictable code, thus raising the overall quality of your programming output.
Practical Examples for Avoiding Initialization Errors in Your Code
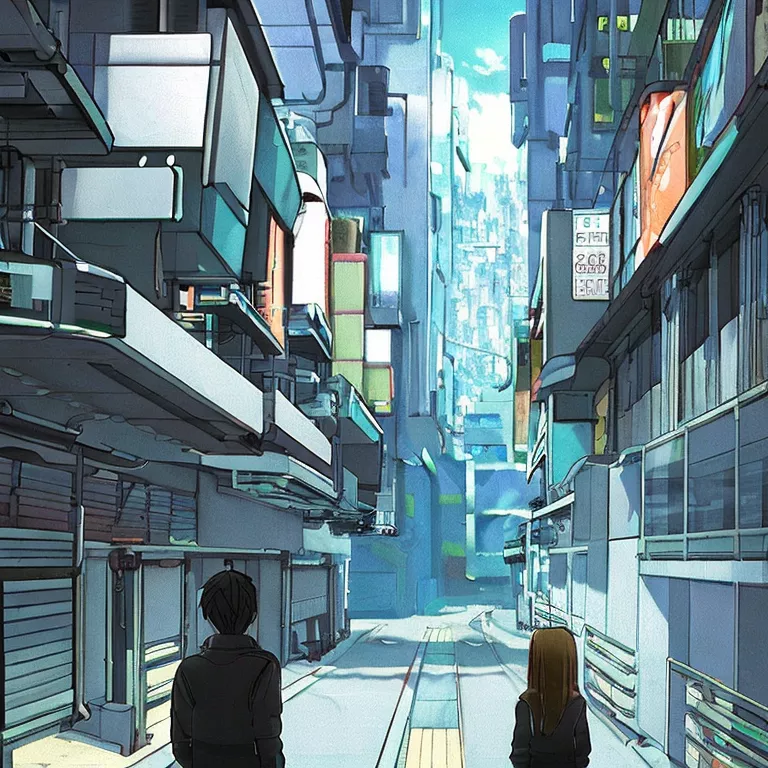
The TypeScript error, “Property Has No Initializer And Is Not Definitely Assigned In The Constructor,” is an issue that often arises when a class property in your code remains unassigned either during initialization or within the body of the constructor. However, with proper understanding and handling of this situation, such initialization errors can be avoided significantly.
**Understanding the error**
In TypeScript, this error indicates the potential exposure to the hazardous undefined state. Basically, if you declare a property inside a class but don’t initialize it or assign its value within the class constructor, TypeScript considers this as potentially problematic as it could lead to unexpected behaviors, given the property holds an ‘undefined’ value. For instance:
class Person { private name: string; constructor() { // No assignment to `name` occurs here } greet() { console.log(`Hello ${this.name}`); } }
When an instance of the
Person
class calls the
greet
method, an unsettling output of “Hello undefined” will be returned due to lack of a definite assignment for the
name
property.
**Handling the error**
While identifying this error isn’t difficult, you need conscientious practices to prevent these initialization mistakes from creeping into your TypeScript codebase. Here are some practical methods:
* **Assign property directly during declaration**: One of the most logical ways to avoid this problem is by initializing the property directly at the point of declaration:
class Person { private name: string = 'John Doe'; }
With this approach, TypeScript sees that the
name
property is duly initialized and thus, does not raise any initialization error.
* **Assign properties values in the constructor**: If properties require more dynamic values that might vary per instance of the class, it’s ideal to handle their assignments in the constructor:
class Person { private name: string; constructor(name: string) { this.name = name; } }
* **Non-null assertion operator**: Lastly, when it is indispensable to leave a property unassigned at first but you are certain it will not be ‘undefined’ before it’s utilized, TypeScript provides us with an escape hatch: the non-null assertion post-fix operator
!
. Utilized judiciously, it can help avoid unwarranted bugs.
class Person { private name!: string; }
Granted, this solution comes with its own trap: if the property isn’t assigned a value before usage, you’ll have a run-time error. Therefore, use this sparingly and only when absolutely certain of the variable’s management.
Remember that with great software power comes great responsibilities. As Bill Gates puts it, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency…” (Gates). Same applies to correctly assigning values to class properties – it enables your application to shine in its full operational glory.
Conclusion
Understanding the complexities of the TypeScript error: ‘Property Has No Initializer And Is Not Definitely Assigned in The Constructor’ provides deep insights into TypeScript’s strict property initialization. TypeScript, as a statically-typed superset of JavaScript, is known for its emphasis on code quality and predictability. With the introduction of the
--strictPropertyInitialization
flag in TypeScript 2.7, developers must now ensure that each instance property of a class gets defined in the constructor itself or have an obvious default value.
TypeScript Property Initialization Error | This error occurs when a class property is declared but not initialized in the constructor or by a default value. For example:
class Person { name: string; constructor() { } } This will result in an error since the property ‘name’ is not defined or initialized. |
Solution | To resolve this error, there are three main solutions: inline initialization, constructor initialization and using the definite assignment assertion operator (!).
|
To paraphrase Java creator James Gosling, “The purpose of software engineering is to control complexity.” Understanding TypeScript’s emphasis on strict property initialization is one such step towards managing this complexity. By making sure that properties are always initialized, developers can avoid common JavaScript pitfalls like
undefined
or
null
errors, leading to more robust and predictable code.
Further reading on this topic can be found in the official TypeScript documentation.