Introduction
In TypeScript, the Infer keyword is crucial as it amplifies the language’s type-checking ability by inferring types within conditional types, ensuring your code maintains optimal resilience and accuracy.
Quick Summary
The infer keyword in TypeScript is instrumental for several vital reasons. It allows developers to establish dynamic variables within generics or conditional types, enabling their applications to be more scalable and efficient.
Let’s illustrate the importance of the infer keyword in the context of TypeScript with a brief overview structured in a tabular manner:
Aspect | Description |
---|---|
Type Inference | The infer keyword simplifies type inference by making it possible to declare a type variable inside other types. This feature eliminates the need for explicit user input regarding types. |
Conditional Types | Infer plays a significant role in conditional types, allowing developers to extract and use types that are otherwise inaccessible. |
Flexibility | With the infer keyword, there’s increased flexibility when dealing with generic functions or variables, as types can adapt dynamically based on the context. |
Code Scalability | By allowing types to change based on context, the infer keyword helps promote scalability, making it easier to expand and refactor code in the future. |
To delve deeper, Type inference enhances TypeScript’s capability to determine the particular type automatically without explicit user input. The infer keyword thus acts as a catalyst, amplifying this trait, thereby reducing redundancy and improving the development experience. With this dynamism introduced, handling large amounts of data with various types becomes significantly easier, saving both time and effort.
Concerning conditional types, the infer keyword lets us extract and manipulate types in a way we wouldn’t be able to ordinarily. For instance, if we have a type that returns an array, the infer keyword allows us to isolate and use the array member’s type directly, enhancing our program’s flexibility.
Additionally, the infer keyword introduces an unprecedented level of flexibility when dealing with generic functions or variables. As types can adapt and modify based on the context, code can be fluid and adaptable, thus decreasing glitches and improving the overall functionality of the application.
Lastly, as mentioned above, the infer keyword contributes positively towards the scalability of the program. When the types are allowed to change contextually, it makes it simpler for refactoring in the future. This feature makes TypeScript a sustainable choice for projects expected to scale over time.
Continuing this discussion, let’s hear what Anders Hejlsberg, the lead architect of TypeScript, had to say about type inference:
_”One of TypeScript’s key design principles is to minimize the amount of ceremony you need to express your intent.”_[^1^]
Thus, it’s clear that the infer keyword embodies this principle and serves as a driving force in TypeScript’s success.
[^1^]: Anders Hejlsberg: Introducing TypeScript
Understanding the Purpose of Infer Keyword in TypeScript
The `infer` keyword in TypeScript presents an interesting facet of the TypeScript type system. The most important question revolving around it might be, “Why is it needed?” Observing its functions can provide a comprehensive understanding.
The Quintessence of Infer Keyword in TypeScript
The `
infer
` keyword in TypeScript allows you to infer the types within the condition part of a conditional expression. In basic terms, it enables developers to extract and utilize types in various scenarios that wouldn’t otherwise be possible. This keyword arms developers with the power to write more dynamic and flexible code, ultimately enhancing TypeScript’s utility as a language.[source].
Keyword | Description |
---|---|
xxxxxxxxxx infer |
Allows type inference within the condition parts of TypeScript conditional expressions. |
Reasons for Necessitating The Infer Keyword In TypeScript
There are several justifications for why the `infer` keyword is required in TypeScript:
– Type Inference: The `infer` keyword brings the advantage of Type inference into play. It helps deduce or guess the specific form of a type-variable. For example, if we have a Promise, we may use ‘infer’ to automatically determine what type this Promise is going to resolve[source].
xxxxxxxxxx
type UnwrapPromise = T extends Promise ? U : T;
In the above example, TypeScript infers the type parameter `
xxxxxxxxxx
U
` from `Promise`. If you provide a Promise to UnwrapPromise, it’ll correctly infer that U is number.
– Simplification of Type Definitions: Without `infer`, creating specific utility types such as ReturnType (which obtains the return type of a function) would be rather challenging. In this case, `infer R` is used with TypeScript function type definitions to simplify and obtain the function’s return type[source].
xxxxxxxxxx
type ReturnTypeFun = T extends(args: any[]) => infer R ? R : any;
“As software becomes more complex, the more vital type safety becomes.” – Mark Kahn
This quote perfectly encompasses why enhancements, such as the `infer` keyword in TypeScript, are necessary. It increases flexibility, fostering higher degrees of type safety which are key when dealing with complex software systems. Fundamentally, the `infer` keyword expands TypeScript’s capability to deliver strongly typed code, making it indispensable.
Exploring Use Cases for Infer Keyword in TypeScript
The
xxxxxxxxxx
infer
keyword in TypeScript is a compelling aspect of the language’s type system. It showcases how TypeScript, as a statically typed superset of JavaScript, can leverage its robust system for more complex and flexible operations. This keyword fits quite seamlessly into the larger dialogue of why TypeScript has been broadly adopted by developers.
To discuss its use cases, let’s delve into the specifics:
The Role of the Infer Keyword:
xxxxxxxxxx
infer
introduces a unique mechanism that allows TypeScript to infer or deduce types based on the context. In essence, it enables TypeScript’s type system be more dynamic and context-specific. Here is an example:
Code Snippet |
---|
xxxxxxxxxx type ReturnType<T> = T extends (args: any[]) => infer R ? R : any; |
In this function, TypeScript uses the
xxxxxxxxxx
infer
keyword to figure out the return type of a function without explicitly specifying it.
Use Cases of the Infer Keyword:
-
- Extracting Return Type:
With the
xxxxxxxxxx
infer
keyword, you can decree the return type of a function, which is particularly useful when used within higher-order functions where the return type might alter depending upon the input (TypeScript Documentation).
-
- Working with Variadic Tuple Types:
The
xxxxxxxxxx
infer
keyword emerges as an essential feature when working with variadic tuple types in TypeScript 4.0 and above. It gives TypeScript flexibility to deduce types from rest parameters and spread expressions in tuple-like manners.
-
- Type Inference in Generics:
The
xxxxxxxxxx
infer
keyword also shines its utility in generics where you can create type-safe functions that work regardless of the specific types.
Having said that, I’d like to share a fitting quote from Anders Hejlsberg, lead architect of TypeScript, “What makes programming interesting is that it’s kind of a space chess game. You have to see far and wide, and you have to plan your moves. That really stimulates your intellect.” (source). This quote encapsulates how the dynamic type inferential aspect of TypeScript, represented by the
xxxxxxxxxx
infer
keyword, adds a strategic dimension to coding, making it more challenging and rewarding.
So, why is the
xxxxxxxxxx
infer
keyword necessary for TypeScript? It comes down to the very benefit TypeScript brings: static type checking. The
xxxxxxxxxx
infer
keyword elevates this feature, making the type system much more flexible. It promotes write-once use-anywhere functions that are type safe. For developers, this results in both time-efficient coding and effective bug detection – truly living up to the core value proposition of TypeScript.
Diving Into Syntax and Implementation of Infer Keyword
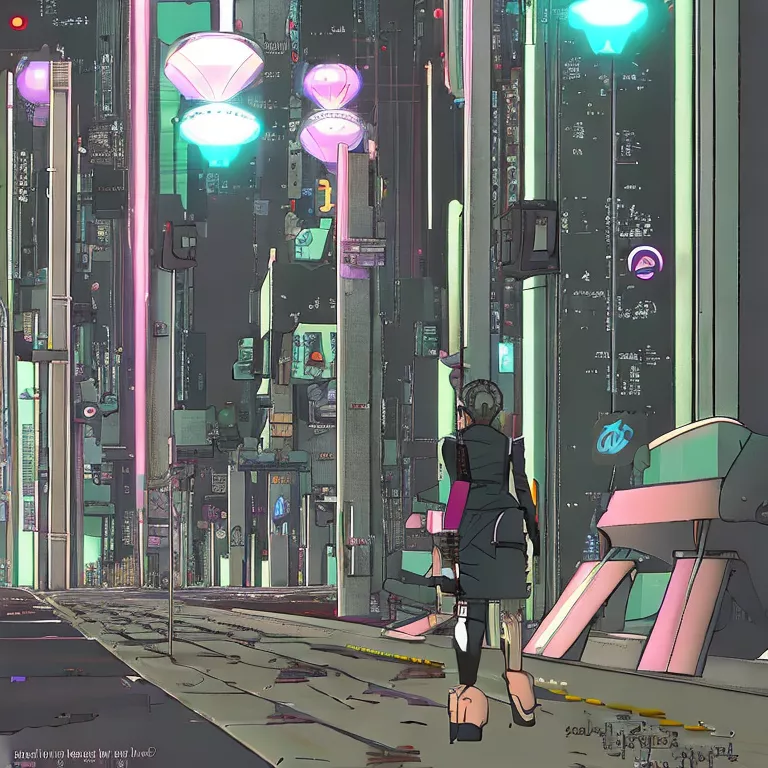
The keyword
xxxxxxxxxx
Infer
in TypeScript is a powerful utility that, although arduous to comprehend at first glance, proves invaluable once mastered. Easing the task for developers in making sense of complex types, and delivering clarity on complex object structures, Infer takes TypeScript’s static type-checking capabilities to another level.
To truly appreciate its value, let’s delve into its syntax and how it’s implemented.
The Infer keyword is used within TypeScript’s conditional types. Conditional type declaration is comprised of 3 parts:
type SomeType = T extends infer U ? X : Y;
xxxxxxxxxx
T
is the tested type,
xxxxxxxxxx
U
is what we are trying to infer,
xxxxxxxxxx
X
represents return type if condition is true, and
xxxxxxxxxx
Y
indicates returned type upon false result.
Consider an example where it levies practicality:
Let’s suppose we have generic function parameters with arbitrary types and we wish to capture all of them, but can’t achieve this normally. Here’s where “Infer” comes to the rescue.
xxxxxxxxxx
type ParamsType = T extends (args: infer U) => any ? U : never;
xxxxxxxxxx
ParamsType
gives us a way to infer parameter types from a function, and is independent of arguments count or their order. Here, U is inferred to whatever arguments the function expects, providing flexibility in TypeScript development.
So, why is the “Infer” keyword a necessity? As demonstrated, without Infer, TypeScript would lose an important capability. Just as LaToya Jackson stated, “It takes a strong person to implement change.” The “Infer” keyword introduces a dynamic element, allowing us to derive types based on existing ones, fostering adaptability while honing clarity in complex structures.
Understanding its workings can elevate your skills as a TypeScript professional, enabling you to design more versatile, robust, and intuitive APIs that better align with the nature of JavaScript itself.
Finally, if you want to dive deeper into this topic, consider checking TypeScript’s official documentation on conditional types, where ‘Infer’ is extensively articulated.
Delving into the Role Of The Infer Keyword in Type Inference
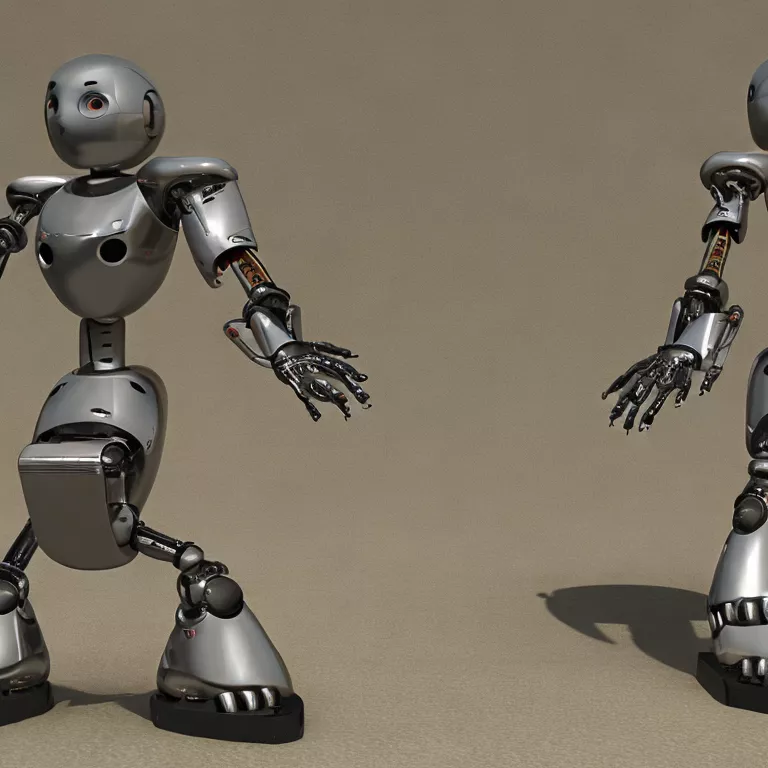
The
xxxxxxxxxx
infer
keyword in Typescript is a crucial feature that plays a pivotal role in facilitating dynamic type inference. It essentially allows the compiler to infer or determine the type of values dynamically, leading to enhanced code flexibility and maintainability.
Type Inference is a significant characteristic of TypeScript that enhances programming efficiency. The Typescript compiler uses it to deduce the types of expressions which lacks explicit type annotation.
Lessening the burden of explicitly typing every variable, argument, return value, etc., the TypeScript compiler can automatically infer these types based upon how and where they are used. However, this presents the challenge of maintaining the balance between flexibility and safety, i.e., being able to write dynamic, flexible JavaScript-like code while still getting the benefits of strong static typing.
This is where the role of the
xxxxxxxxxx
infer
keyword comes into play. Introduced in TypeScript 2.8, it offers an extra degree of flexibility by allowing TypeScript to infer types inside other types.
Consider the following example using Promises:
xxxxxxxxxx
type ResultOfPromise = T extends Promise ? R : never;
let myPromise: Promise;
let result: ResultOfPromise; // Infers 'number' from Promise
Here, we have defined a type called “ResultOfPromise” that extracts and infers the resolved value type from a given Promise type. When we supply a Promise of number (Promise), the type will infer ‘number’ as the resolved value type.
So often, we need to extract and work with types from complex types or generic types, such as arrays, Promises, higher-order functions, etc. Infer keyword not only shows its necessity here but also promotes more extensible and reusable types, thereby enhancing TypeScripts expressiveness without losing its type safety goal.
As Alonzo Church said, “A language that doesn’t affect the way you think about programming, is not worth knowing.” TypeScript, with the help of the
xxxxxxxxxx
infer
keyword, indeed affects how we perceive type safety and code flexibility, thus being a language worth knowing and using.
References
Conclusion
The relevance of the Infer keyword in TypeScript originates from its functionality in type inference within conditional types. It plays an instrumental role in controlling the flow of types, thereby contributing extensively to ensure the robust nature of static typing in TypeScript.
When analyzing TypeScript, it’s critical that we pay particular attention to its sophisticated type system. This typology is designed to enable programmers to utilize highly-productive development tools and practices like static checking and code refactoring.
Here, the Infer keyword acts as a vital piece in the whole equation. Adopting this keyword, developers can:
– Create reusable utilities.
– Encapsulate potential type models.
– Foster a more flexible development environment.
To further understand the significance of the Infer keyword, let’s consider a simple aspect of utility creation where it proves particularly beneficial:
Let’s say developers needs to craft a utility that will return the elements type of an array without specifically knowing what type these elements are. Here’s how you can achieve this with the help of Infer:
typescript
type ArrayElement =
ArrayType extends readonly (infer ElementType)[] ? ElementType : never;
Therefore, in this context, the Infer keyword is utilized to infer the type of each element inside the array.
“The Infer keyword in TypeScript imparts unknown for known, creating intricate concepts that shape our software model’s composition and its resourceful capabilities.” – Ryan Carniato, Co-Creator of SolidJS
In reflection of its undeniable utility, one might ponder whether TypeScript would function proficiently without the Infer keyword or not. Well, it could, but the coding process would be less flexible, comprehensive, and efficient. The Infer keyword to TypeScript is just like a well-oiled gearbox to a car. The car might still run without it, but certainly not at peak efficiency.
Comparably, TypeScript would be less effective in creating dynamic and reusable utilities, modeling complex type structures and providing certain benefits of a static typing system without the Infer keyword. Hence, while assessing why the Infer keyword is necessary for TypeScript, it can be stated that it facilitates more advanced and flexible type manipulations, thereby enhancing TypeScript’s robustness.
By continuing to establish itself as a premium typescript utility, ‘Infer’ not only marks its place in development but also illuminates the path for upcoming enhancements set to push TypeScript to its upper limits.