Introduction
Understanding the usage of Typescript React.Fc can often seem confusing but with proper learning and utilization, it becomes a vital tool in enhancing your web development proficiency, ensuring you design more accurate and efficient applications.
Quick Summary
React.Fc in Typescript refers to Function Component with props. It’s a subtle but important concept for developers engaged in producing scalable, maintainable React components with Typescript.
To better analyze the concept, let’s take a look at a visual representation:
Element | Description |
---|---|
React.Fc | This is a type definition that represents Function Components in React. |
<Props> | This signifies a generic parameter, effectively allowing us to specify the shape of the props this component should accept. |
Deconstructing a typical usage of `React.Fc` might look as follows:
);
interface MyComponentProps {
someProperty: string;
}
const MyComponent: React.FC = ({ someProperty }) => (
Here we are defining a functional component named “MyComponent”. The `React.FC` defines the function as a React Function Component and expects an object of MyComponentProps. The `{ someProperty }` inside the parentheses harnesses the power of ES6 destructuring to extract properties directly from an object. Essentially, it’s syntactic sugar over props.someProperty.
This approach can lead to confusion because of expectations from conventional communication between parent-child components. In traditional Javascript or even class-based components in React, one would typically expect passing and receiving props via the ‘this.props’ pattern.
Switching to Typescript may complicate the transition due to these added types and their associated demands. Thus, understanding how to harness the power of `React.Fc` becomes integral to leveraging Typescript and React together.
Bill Joy, one of the co-founders of Sun Microsystems, once insightfully said, “After learning to code in high-level languages, there’s always some irritation in dealing with assembly language.” This sentiment can sometimes resonate with developers transitioning from ES6-based React to more strictly-typed approaches like Typescript. But remember, it’s all part of the constant evolution and adaptability inherent in technology.
Here is an online reference for detailed guide about Function Component & Props in TypeScript and React.
Understanding the Basics of TypeScript React.Fc
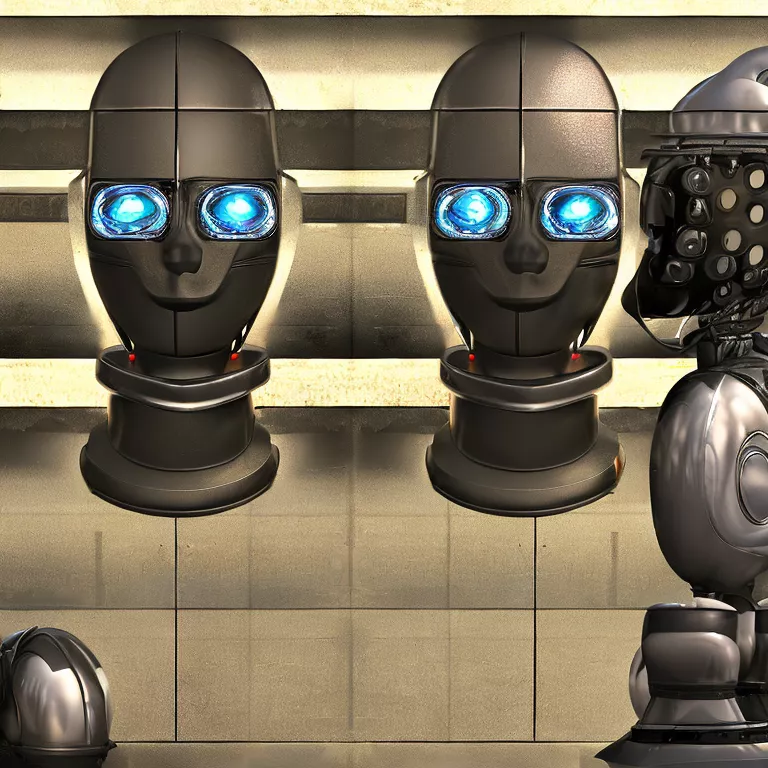
The TypeScript “(a.k.a. Functional Component) in combination with “ is a key aspect of working with React and TypeScript. Notably, it deciphers the complexities involved in defining components, their properties, and types in an unambiguous manner. Nonetheless, confusion might arise, especially for those new to this notion, primarily because TypeScript isn’t native to JavaScript or React.
Breakdown of `<react.fc`</react.fc
-
xxxxxxxxxx
React.FC
stands for React’s ‘Functional Component’. FC is a type defined in the React library which represents a function that returns an element. Essentially,
xxxxxxxxxx
React.FC
is just a TypeScript type that describes a functional component.
-
xxxxxxxxxx
Props
, on the other hand, signifies properties (parametric types) passed down to a function or component – a way to allow data flow from parent components to child components.
Let’s consider an example:
;
xxxxxxxxxx
type AppProps = {
message: string;
};
const App: React.FC = ({ message }) =>
This shows the usage of a simple `React.FC` with Props, where `AppProps` object specifies the type structure, and `React.FC` uses the structure to accept an appropriately shaped props argument.
The
xxxxxxxxxx
React.Fc<Props>
Confusion
Understanding and using proper typings is vital in a TypeScript environment as this ensures application reliability. The confounding factor could be around understanding the role of TypeScript within this context. TypeScript brings static typing to JavaScript, enhancing editor productivity and catching errors while writing rather than during runtime. Thus, to reduce confusion:
- The role of TypeScript should be clear, i.e., it adds static types to JavaScript.
- Understanding the importance of
xxxxxxxxxx
React.FC
‘s and Props will offer better clarity on usage. It’s all about type checking of the components and their properties, ensuring they adhere to the defined structure only.
To refer to the TypeScript Handbook for understanding generic typings like “ can be worthwhile [link]
As per Eric Elliott, a renowned JavaScript expert, “JavaScript developers have spent a lot of time inventing ways to scheme around these problems instead of adopting simpler, easier to manage alternatives — alternatives which have been in plain sight for years: just use static typing”. Although he implies generally towards static typing in JavaScript, it also highlights the value proposition of TypeScript within a React ecosystem.
Remember that adapting to concepts like `React.Fc` requires time. The important takeaway is their function: assist in defining and enforcing correct software constructs during development.
Function Component in React Using TypeScript: A Closer Look
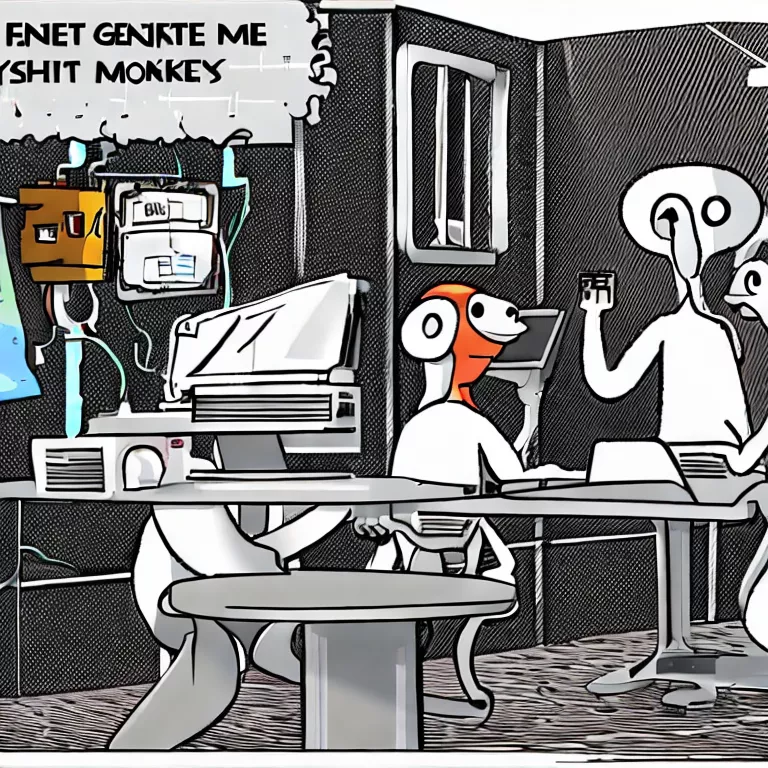
Function components in React and TypeScript have become vastly popular today due to their simplicity and ease of use. A common source of confusion for many TypeScript developers is the utilization of
xxxxxxxxxx
React.Fc<Props>
. To put it simply,
xxxxxxxxxx
React.Fc<Props>
is a type definition that describes a functional component in React with properties of type ‘Props’.
Let’s dive a bit deeper into this concept:
In a functional component, you define the functionality and render specifications using TypeScript. The
xxxxxxxxxx
React.Fc<Props>
(short for function component) comes with an extended type definition and makes your component ready to receive properties.
Here’s some sample usage to demonstrate how it might be used in a real-world scenario.
html
interface Props {
name: string;
}
const Greeting: React.FC<Props> = ({ name }) => <h1>Hello, {name}!</h1>;
//You can now use the Greeting component with a ‘name’ prop.
<Greeting name=”John” />
In this example, the Greeting component is declared as a Function Component (`
xxxxxxxxxx
FC
`) receiving a `
xxxxxxxxxx
props
` object of type ‘~Props’. By using
xxxxxxxxxx
React.Fc<Props>
, you gain access to several additional properties such as `defaultProps` or `propTypes`.
However, using
xxxxxxxxxx
React.Fc<Props>
doesn’t come without pitfalls. Even though it suggests you are defining a function component, it doesn’t guarantee you are following the rules-of-hooks inside the component. It also unnecessarily extends the component’s props to include children even when they are not needed. Hence, some developers opt to directly use the function signature instead:
html
interface Props {
name: string;
}
const Greeting = ({ name }: Props) => <h1>Hello, {name}!</h1>;
As TypeScript continues to evolve, debates around how best to type React components will persist. But as Martin Fowler, one of the leading voices in software engineering hinted, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This suggests that readability and maintainability should be at the forefront when deciding which approach to use in your projects.
Pros and Cons: Utilizing TypeScript Props with React.FC
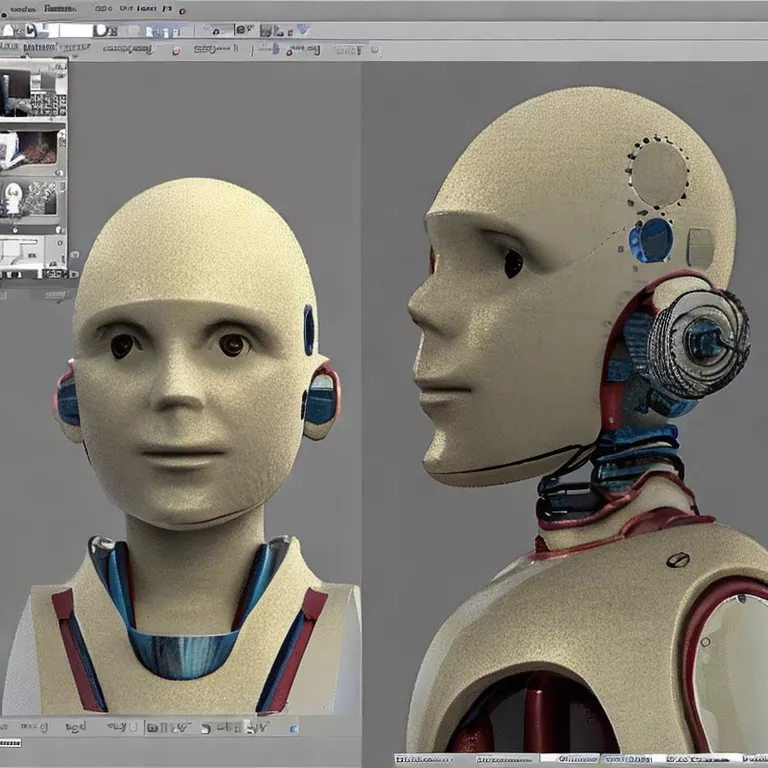
Pros:
Static Type Checking | The primary advantage of using TypeScript Props with React.FC is static type checking. It reduces typographical errors by preempting them during the development process itself. |
Autocompletion & Intellisense | In many IDEs such as VS Code, TypeScript provides autocompletion and improved Intellisense for prop types in components. This improves developer productivity and reduces the learning curve. |
Easy Maintenance | TypeScript and its Types system can make JavaScript applications easier to understand and maintain, especially when working in large codebases or teams. |
Cons:
Verbose Syntax | One downside is TypeScript’s syntax which can sometimes become verbose, especially with complex types and generics utilized within React.FC component props declaration. |
Learning Curve | There exists a steep learning curve for developers who have never used strongly typed languages before or have little experience in between the interplay of TypeScript and React. |
Limits Flexibility | TypeScript might seem limiting to those accustomed to Javascript’s dynamic typing, as it introduces constraints that some developers might find restrictive. |
From a coding point of view, when you declare a functional component with React.Fc<Props>, you’re pointing out to TypeScript that your function is a specific type of entity: a React Functional Component. An example could be:
xxxxxxxxxx
type AppProps = { message: string };
const App: React.FC<AppProps> = ({ message }) => <div>{message}</div>;
Diving deeper into this, there’s often confusion about whether or not it’s necessary or beneficial to use React.FC (or formerly known as React.FunctionComponent) when defining components. It’s quite common to see the type definitions for props passed in like normal arguments instead of using React.FC.
In the words of Jeff Atwood, co-founder of StackOverflow, “Code is like humor. When you have to explain it, it’s bad.” Hence, if developers find themselves constantly trying to explain or grapple with understanding React.FC and its implications, then perhaps it may not be the most elegant solution to the problem.
Apart from these points, the decision to use TypeScript Props with React.FC or not depends on the requirements of the project, the team’s comfort level with TypeScript, and the complexity of the codebase. Therefore, it is not a one-size-fits-all solution, but rather a technological choice that needs deliberate assessment and evaluation.
Breaking Down Common Mistakes and Confusions with Typescript React.Fc
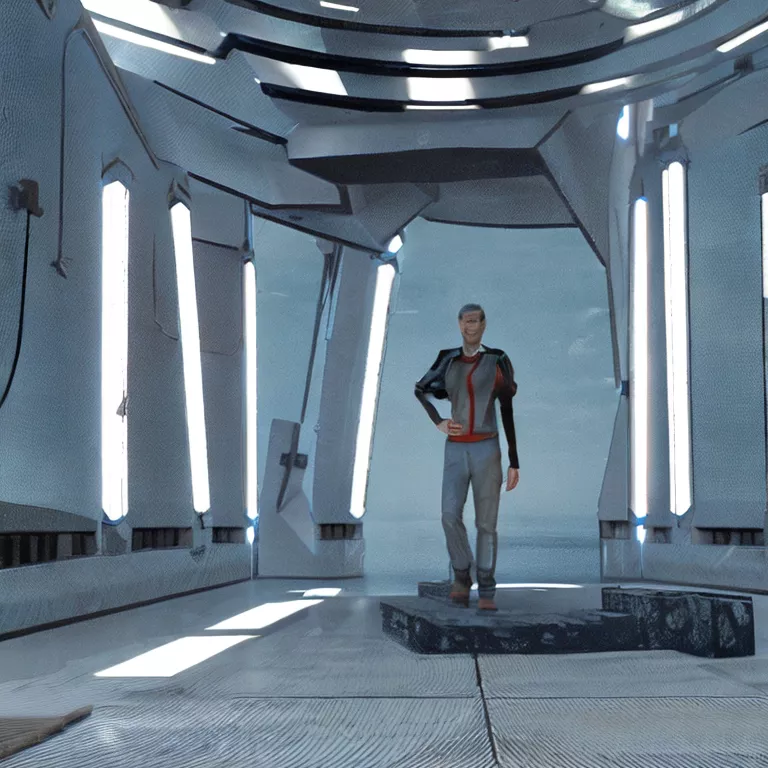
While TypeScript and React continue to gain popularity among web developers, many often encounter confusing aspects when working with TypeScript’s `React.FC` in both beginner and advanced stages. These confusions often lead to frequent errors. Here we will analyze common issues and pragmatically delve into optimum solutions.
When using TypeScript with React, one of the most misinterpreted concepts is the “ generics used in `React.FC`. This construct denotes a functional component that accepts an object of type Props. Misunderstandings often ensue when there are incorrect assumptions about what exactly `Props` represent.
Firstly, some developers assume that `Props` should always reflect every single prop their component uses. In reality, while `Props` should indeed describe the properties the component takes, not all props necessarily need to be specified here if they’re being consumed by higher-order components or are part of the inherent attributes like ‘className’ or ‘children’.
For instance, consider this component:
; }
xxxxxxxxxx
type MyComponentProps = {
message: string;
}
const MyComponent: React.FC = ({ message }) => {
return
The `MyComponentProps` describes the `message` prop, but does not have to include other default HTML attributes div might accept, such as `className`. This misconception frequently contributes to bloated and hard-to-maintain prop definitions.
Secondly, many developers confuse the necessity of defining children within `React.FC`. When using this construct, `children` is implicitly added to your props. Some developers, unaware of this, explicitly add `children` into their prop types which leads to redundancy and potential confusion.
Consider:
; }
xxxxxxxxxx
type MyComponentProps = {
children: React.ReactNode;
message: string;
}
const MyComponent: React.FC = ({ children, message }) => {
return
In this case, specifying `children` within `MyComponentProps` is unnecessary since `React.FC` already inherently contains it. Removing such redundancies simplifies the code and keeps it streamlined.
Finally, a popular discussion within the developer community is whether to use `React.FC` in the first place. While it does provide automatic inclusion of `children` and correct type checking for the return value, it can arguably lead to redundant prop definitions and additional complexity[^1^]. In some cases, directly typing the function may offer simplicity and clarity.
For instance:
; }
xxxxxxxxxx
type MyComponentProps = {
message: string;
}
function MyComponent({ message }: MyComponentProps ) {
return
Overall, being aware of these common misconceptions and understanding the precise workings, benefits, and drawbacks of `React.FC`, helps create cleaner, more manageable TypeScript React code.
[^1^]: [Why I Don’t Use React.FC<>](https://fettblog.eu/typescript-react-why-i-dont-use-react-fc/)
Conclusion
Exploring the topic of ‘Typescript React.Fc Confusion’, one can effectively decode crucial factors which often can become confusing to many TypeScript developers. To delve deep into this topic, it is significant first to comprehend what precisely Typescript and React.Fc are. In terms of coding languages, while
xxxxxxxxxx
TypeScript
is a statically typed superset of JavaScript that compiles to plain JavaScript,
xxxxxxxxxx
React.Fc<Props>
is a particular type of React component where Fc denotes function component and Props refers to the properties passed to the component.
The confusion around
xxxxxxxxxx
TypeScript React.Fc<Props>
generally arises when defining types for function components in TypeScript with React. Programmers often muddle up when deciding whether to use a functional component as a regular function or an arrow function. Adding to this confoundment, it’s also seen some perplexity among programmers about declaring props with or without React’s built-in `React.FC` type. Given is an example of code for better understanding:
xxxxxxxxxx
type AppProps = { message: string };
const App: React.FC<AppProps> = ({ message }) => <p>{message}</p>;
This example illustrates a Function Component using TypeScript with Props. The syntax may look elusive to beginners, however, with practice, the constraint becomes seamless to work with. It’s worth noting that TypeScript enhances the ability of a developer to write error-prone codes with its static typing feature.
As Linus Torvalds, creator of Linux, once wisely said, “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.” Relating to this quote, when viewing the confusion revolving around
xxxxxxxxxx
Typescript React.Fc<Props>
, at its essence, it’s about understanding and utilizing data structures and their relationships effectively. A strong comprehension of same aids in alleviating any confusion associated with code.
In the vast world of coders, to hone TypeScript skills, it’s advisable to thoroughly understand how it functions. Invariably the knack lies in comprehending its structure, analyzing its alignment and correlation with React and its components, and hence applying these coding strategies meticulously. If well-practiced, over time, developers can overcome the
xxxxxxxxxx
Typescript React.Fc<Props>
confusion and skirt any subsequent challenges met during coding processes.
Let’s promote continuous learning in technology to evolve as a better coder each day!