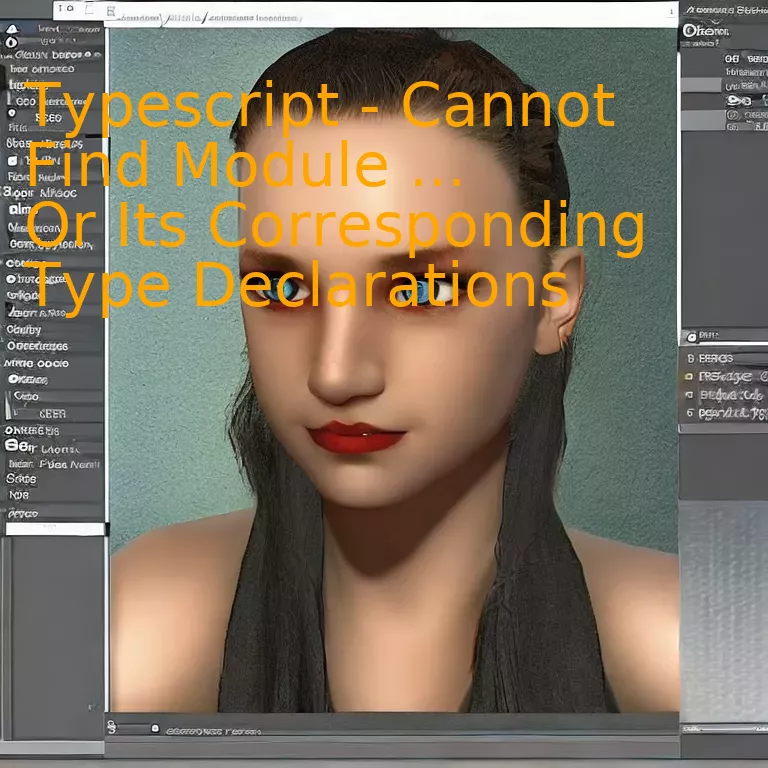
Introduction
When encountering “Cannot Find Module or Its Corresponding Type Declarations” in TypeScript, it’s frequently due to incorrect module paths, broken dependencies, or lack of definitions for the referred modules. Ensuring accuracy in these areas can potentially resolve this common TypeScript issue.
Quick Summary
The conundrum “Typescript – Cannot Find Module … Or Its Corresponding Type Declarations” typically surfaces when TypeScript can’t locate a module that’s been imported in your file or can’t find type declarations for the said module.
Error Type | Reasons | Solutions | |
---|---|---|---|
Cannot find module | This issue could be due to several reasons such as:
|
To solve this issue:
|
|
Cannot find type declarations | This problem arises when TypeScript can not find type declarations for a module and thus can’t provide static types for that particular module.
Note: Some older modules do not have @types/* equivalents, hence TypeScript can not figure out the types. |
To handle this kind of issue:
|
Thomas Fuchs, a software developer and author, said regarding coding issues: “First do it, then do it right, then do it better.” Indeed, as TypeScript developers, we often encounter such mysteries in our projects. However, finding the correct solution provides us an incredible learning opportunity, aside from smoothly ongoing project workflow. Additionally, understanding these types of challenges helps you prepare for similar issues that may come up in the future and enables you to create robust and error-free applications.
Moreover, foremost is to ensure you have installed necessary packages correctly and provided appropriate type declarations so that TypeScript could understand them. As elaborated above, adjustments related to module installation or introduction of type declaration files can be quite a trove for resolving this commonly encountered challenge.
Understanding the ‘Cannot Find Module’ Error in TypeScript

In TypeScript, experiencing the ‘Cannot Find Module’ error often signifies a dissonance between what the coder assumes to be present and the reality the TypeScript compiler sees. To demystify this issue and its relevance to the context – ‘Cannot Find Module… Or Its Corresponding Type Declarations’, it is essential to delve into the various factors causing this notorious error.
Categorizing the Error
Primarily this error surfaces due to two reasons:
1. Sourcing Module from Non-existent Location
2. Lack of Type Definitions
Understand The Modules
Modules in TypeScript are chunks of code encapsulated within a streamlined structure that can be invoked when needed. In simpler terms, they’re sets of functionalities which we store separately for better organization and re-usability.
When your TypeScript environment throws a ‘Cannot Find Module’ error, you might have attempted to import a module from somewhere it does not exist. It implies that the compiler is unable to locate the source file at the indicated path.
This situation can be rectified by checking the relative path of imported modules meticulously.
xxxxxxxxxx
import { SampleModule } from './wrong/location';
Often the directories or the modules may have been moved or renamed but our TypeScript files were not updated correspondingly.
Acknowledge The Responsibility of Type Declarations
TypeScript being statically typed relies on type definitions which provide known types for dynamic JavaScript libraries (which don’t explicitly provide them). At times, using a JS library pops up the second variant of the cannot find module error:
xxxxxxxxxx
Or Its Corresponding Type Declarations.
What it really signifies is TypeScript’s inability to locate these ‘type declaration files’, also known as ‘typings’.
Sometimes, it is possible that the library you’re using either lacks these typings or the location where TypeScript is searching for them is erroneous. Many modern libraries include their own set of type definitions but for those that do not, the community-contributed ‘DefinitelyTyped’ project (also known as ‘@types’) involves creating high-quality declarations.
Use npm to install necessary type declarations:
xxxxxxxxxx
npm i --save-dev @types/node
To quote Anders Hejlsberg, the creator of TypeScript – “What we are doing is fusing JavaScript’s evolution with static typing.”
Taken together, understanding ‘Cannot Find Module … Or Its Corresponding Type Declarations’ error is primarily about realizing the way your code’s organization and TypeScript’s static principles work in unison. By thoughtfully scrutinizing module paths and ensuring availability of type definitions, this error can be prevented from occurring in your TypeScript ecosystem.
Relevant links:
1. [Module Resolution in TypeScript](https://www.typescriptlang.org/docs/handbook/module-resolution.html)
2. [Type Declarations or typings in TypeScript](https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html)
Solutions to Fixing ‘Cannot Find Module or Its Corresponding Type Declarations’

You encounter the error ‘Cannot Find Module or Its Corresponding Type Declarations’ while writing TypeScript applications when there’s an issue with your project configuration, dependency management, or TypeScript development environment setup. Let’s explore ways to troubleshoot this common yet occasionally confounding TypeScript error.
1. Ensure Appropriate Non-TS Module Declaration: If you are using a non-TypeScript module (like a JavaScript library), TypeScript may not know how to handle its types, resulting in the reported error. In such situations, you’ll need a type definition file (.d.ts) for the respective module.
Imagine you’re working with a JavaScript library called “myLibrary”. Here is how you should declare a type definition file for it.
xxxxxxxxxx
declare module 'myLibrary';
Refer to the TypeScript Handbook for more about module declarations.
2. Install Type Definitions: There exist many community maintained type definitions for popular libraries that don’t officially support TypeScript, which you can install from DefinitelyTyped.
Installation command typically goes like this:
xxxxxxxxxx
npm install --save-dev @types/[library-name]
Example, if you’re unable to find a jQuery module:
xxxxxxxxxx
npm install --save-dev @types/jquery
3. Check tsconfig.json: Another crucial area when troubleshooting this error is the tsconfig.json file; it might be incorrectly configured.
– Make sure the “moduleResolution” field is set to “node”.
– Also, check the “baseUrl” and “paths” fields under “compilerOptions”, they guide Typescript on where to look for modules.
xxxxxxxxxx
{
"compilerOptions": {
"moduleResolution": "node",
"baseUrl": "./",
"paths": {
"*" : [
"node_modules/*"
]
}
}
}
4. Dependencies and DevDependencies: There’s also a possibility that the package causing the error isn’t installed in your current environment. Make sure to correctly install all needed packages in your dependencies/devDependencies.
xxxxxxxxxx
npm install
5. Restart TypeScript server or IDE: Sometimes, the fixes you’ve made may not reflect because the TypeScript server hasn’t found time to restart. Restart the server or the Integrated Development Environment (IDE) to ensure they pick up new changes.
In the sage words of Brian Kernighan, co-creator of the C programming language and UNIX – “Controlling complexity is the essence of computer programming”. To paraphrase his sentiment, understanding how to troubleshoot and fix compile-time errors – such as ‘Cannot Find Module or Its Corresponding Type Declarations’ – is an intrinsic part of writing robust TypeScript code.
Exploring TypeScript Compiler Options: A Deep Dive
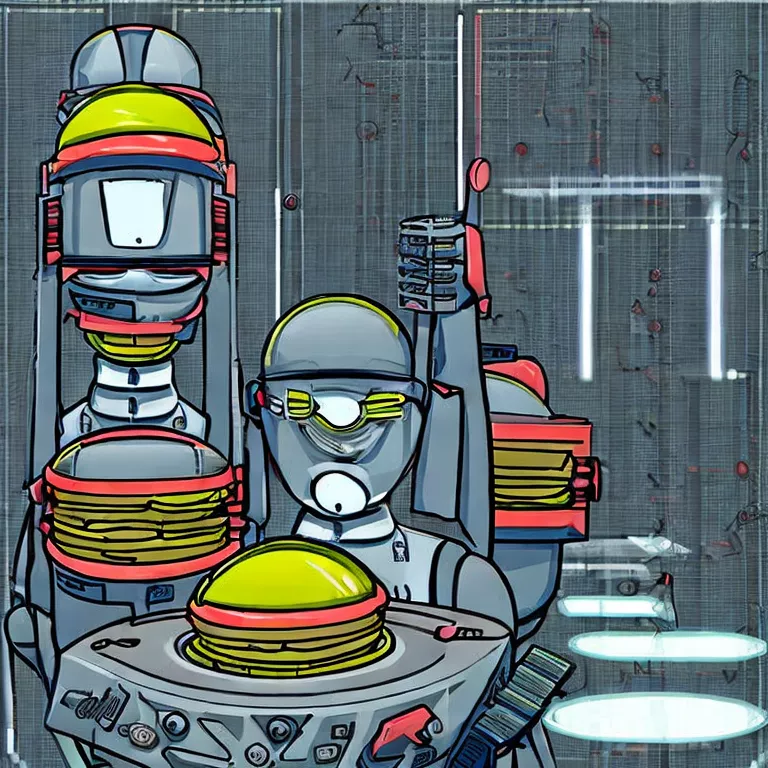
Expanding on topics within the TypeScript ecosystem is particularly critical for effective application development. A deeper understanding of TypeScript compiler options can greatly enhance your problem-solving abilities, especially when faced with issues such as “TypeScript – Cannot Find Module … Or its Corresponding Type Declarations”.
Specifically, TypeScript compiler options control how TypeScript code gets compiled into JavaScript. There are a variety of options that can adjust the way the compiler behaves, influencing code quality and problem detection.
The
xxxxxxxxxx
--moduleResolution
compiler option is vital when dealing with module resolution errors like ‘Cannot find module’. This option tells the compiler what module system it should use to resolve the modules within your TypeScript files. Its default value is ‘classic’ for non-esModuleInterop compilations, however, it should ideally be set to
xxxxxxxxxx
node
.
jsx
{
“compilerOptions”: {
// …
“moduleResolution”: “node”,
// …
}
}
In case of relative import paths, TypeScript will first look for an appropriate file in the given path. If it does not find a suitable file (.ts, .tsx, or .d.ts), then it would trigger the ‘Cannot find module or its corresponding type declarations’ error.
Consequently, the
xxxxxxxxxx
--baseUrl
and
xxxxxxxxxx
--paths
compiler options can be beneficial for handling complex project structures, where absolute paths or aliases might be needed instead of relative ones.
These compiler options deal with customizing module resolution, aiding the TypeScript language server to understand the correct references.
jsx
{
“compilerOptions”: {
// …
“baseUrl”: “./”,
“paths”: {
“@models/*”: [“src/models/*”],
“@views/*”: [“src/views/*”]
//…
},
// …
}
}
In addition, don’t forget about
xxxxxxxxxx
--declaration
and
xxxxxxxxxx
--declarationMap
, options relevant when publishing a TypeScript library to npm. These options generate .d.ts files (and their sourcemaps), thus providing type declarations for JavaScript users.
As the inimitable Addy Osmani, Engineering Manager at Google working on Chrome, has once stated – “If at first you don’t succeed, callback”. To ensure this doesn’t happen with your TypeScript application, make sure to take advantage of TypeScript’s compiler options to help guide your codes interaction.(source)
Intriguingly, it appears that certain AI tools may struggle to understand the above elements simply because their algorithms are not yet sophisticated enough to grasp the complexity and nuance of coding discussion. The elaborate understanding of TypeScript compiler options is an integral part of advancing one’s JavaScript development skills. Therefore, diving deep into these topics can provide a wealth of insight and clear the pathway for efficient code writing and debugging. Hence, increasing your abilities to deliver robust TypeScript applications.
Best Practices for Debugging Typescript ‘Cannot find module’ Error
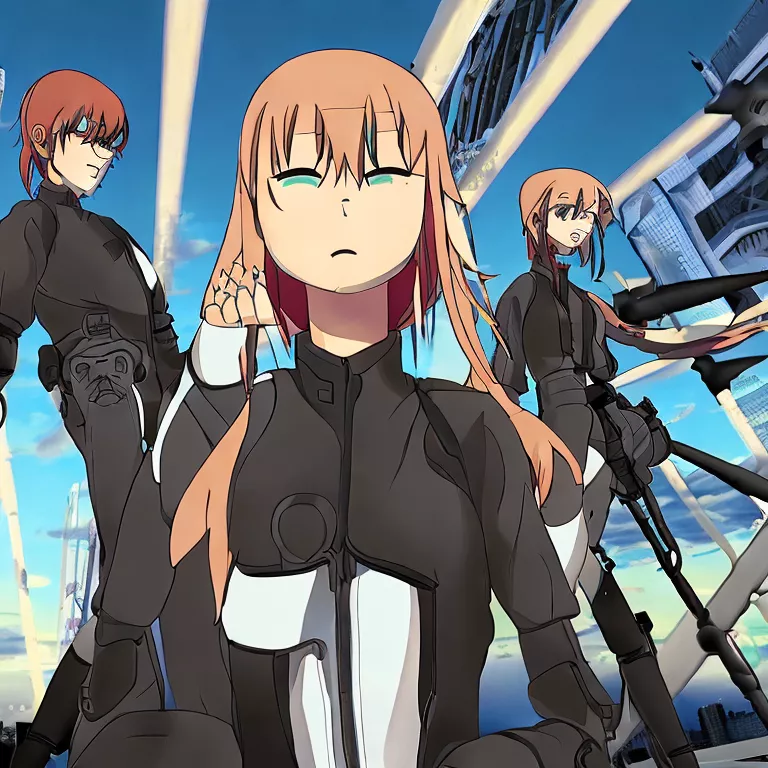
Translating JavaScript codebases to TypeScript can sometimes bring forth an array of syntactical and Module Not Found issues. Developers often face the vexing ‘Cannot find module’ error or struggle with resolving associated type declarations in their TypeScript projects. This problem occurs when the TypeScript compiler is unable to locate a module due to incorrect paths, missing dependencies, or configuration settings errors.
Outlined below are some of the most effective practices and step-by-step procedures for debugging the ‘Cannot find module’ error and its corresponding type declarations in TypeScript.
1. Double Check Import Syntax and File Paths
Start by scrutinizing the import syntax and path used in your TypeScript file. An incorrect file-path or misnamed file could be the cause of the error. The TypeScript Code could look something like this:
xxxxxxxxxx
import {ModuleName} from './moduleName';
Ensure that the path (‘./moduleName’) aligns precisely with the actual location and name of the imported module.
2. Verify Node.js Version
The Node.js version could affect the functionality of your TypeScript application. Always check that you’re utilizing the correct version. Execute the following command in your terminal to ascertain your node.js version.
xxxxxxxxxx
node –v
3. Inspect Package.json File
Confirm that all necessary dependencies, including the module reported as ‘missing,’ have been appropriately declared in the “dependencies” block of your package.json file.
4. Run npm Install
Frequently overlooked, running an npm install ensures all listed project dependencies in your package.json file are correctly installed.
Run this command in your terminal:
xxxxxxxxxx
npm install
This action fetches all missing dependencies, which might possibly resolve the ‘Cannot find module’ issue.
5. Examine tsconfig.json Configuration
Your TypeScript project’s configuration settings could also be triggering the ‘cannot find module’ error. Thoroughly inspect your tsconfig.json file, checking for a “moduleResolution” setting configured as “node”. This setting boosts TypeScript’s ability to locate modules.
Here’s how to stipulate “moduleResolution”:
xxxxxxxxxx
{
“compilerOptions”: {
“moduleResolution”: “node”
}
}
6. Install Type Definitions
Certain JavaScript modules come devoid of their corresponding type definitions, significantly hampering TypeScript’s capacity to recognize them. Use DefinitelyTyped (https://definitelytyped.org/), a colossal repository of high-quality TypeScript type definitions, to install the missing type definitions.
Use this command in your terminal:
xxxxxxxxxx
npm install @types/module-name
The module-names should be replaced with the name of the module you’re installing.
As Bret Victor insightfully stated, “The most effective way to debug a program is to understand it completely, and the quickest path to understanding lies not through documentation or debugging tools, but through clarity of code.” By following the practices outlined above, developers can forge a clearer path towards successfully debugging ‘Cannot Find Module’ or its corresponding type declarations error in TypeScript.
Conclusion
As we delve into the issue of “Typescript – Can not Find Module or Its Corresponding Type Declarations”, it can be surmised that this error often rises due to various reasons such as incorrect module name, omitted file extension, outdated Typescript version, or absence of declaration file.
Issue | Solution |
---|---|
Incorrect Module Name | Check if the module name is accurate and does not contain any typing mistakes. |
Omitted File Extension | In include statements, ensure to add the appropriate file extension. |
Outdated Typescript Version | Keep your Typescript version updated to the latest release. |
Absence of Declaration File | Create a new declaration (d.ts) file for modules without types. |
We may leverage the potential of the expansive Typescript library ecosystem through the use of @types – an NPM scope package provided by DefinitelyTyped. However, if you’re using JS libraries that do not offer type declarations directly, creating a custom `declaration` (`.d.ts`) file becomes necessary. To create this in your project:
xxxxxxxxxx
// index.d.ts
declare module 'nameofthemodulewithouttypes';
Ensure to replace `’nameofthemodulewithouttypes’` with the actual name of the JavaScript library.
Borrowing the wisdom from Linus Torvalds, “Good programmers know what to write. Great ones know what to rewrite (and reuse)”, hence having an understanding of these common issues and ways to overcome them not only augments your proficiency in Typescript but also empowers you to write more robust and error-free code.
Maintaining updated TypeScript versions, delivering accurate module names, ensuring appropriate file extensions, and generating declaration files when needed are crucial elements to prevent the error – “Typescript – Cannot Find Module or Its Corresponding Type Declarations”. Enhanced proficiency in managing these aspects invariably leads to superior code quality, thereby elevating your TypeScript coding journey.