
Introduction
The error, ‘TypeError: Cannot Read Properties Of Undefined (Reading Id)’, generally surfaces when you try to access an ID attribute from an object that isn’t completely defined in your code, a situation that can be resolved by ensuring the object’s correct initiation before referencing its properties.
Quick Summary
Understanding the
Typeerror: Cannot Read Properties Of Undefined (Reading Id)
can be simplified by viewing it in a tabular format. For instance:
Error Occurrence | Reason | Solution |
---|---|---|
Typeerror: Cannot read properties of undefined | The error signifies that JavaScript is attempting to access a property on a value that is currently undefined, hence cannot find the said property. | Ensure that values are defined before accessing their properties |
(Reading ‘id’) | This usually indicates that you’re trying to fetch an ‘id’ property from an object that is not available or non-existent. | Implement safety checks to confirm the existence of objects before manipulating their properties |
This table offers a distilled view of the occurrence, reason, and solution for the Typeerror under discussion.
Digging deeper beyond the tables content, the error—
xxxxxxxxxx
Typeerror: Cannot read properties of undefined (reading id)
— is a common stumbling block for developers working with JavaScript and TypeScript. It indicates a problem in your code whereby you try to access properties of a variable or an object that has not been defined yet or does not exist.
JavaScript is a loosely-typed language with dynamic tendencies. Attempting to fetch a property from an undefined variable creates this type of error. Why? Because in JavaScript universe, `undefined` represents the absence of a value or the absence of a defined state. So if JavaScript finds `undefined` where it anticipates an actual value, it throws a TypeError.
For example:
typescript
let obj;
console.log(obj.id);
This will cause
xxxxxxxxxx
TypeError: Cannot read properties of undefined (reading 'id')
because `obj` is undefined when we attempt to get its `id`.
How do you address this error? Prioritizing safety in your code can help prevent these kinds of issues. Always validate that your variables or objects exist before trying to access their properties. You could use an if-statement for such checks:
typescript
let obj;
if(obj){
console.log(obj.id); // This won’t cause an error now
}
The error under discussion ties into one of the best practices in coding. As Edsger W. Dijkstra quotes, “Programming without an extremely heavy use of console.log / System.out.print etc debugging assistance is akin to a doctor diagnosing patients without CAT scans.” It reminds us of the importance of using built-in functionality for debugging and managing potential errors and issues.
Interestingly, TypeScript, offers powerful tools to handle these situations. One key tool offered by TypeScript is Optional chaining (`?.`). The optional chain operator allows you to read the value of a property located deep within a chain of connected objects without having to expressly validate that each reference in the chain is valid.
Consider an example:
typescript
let person;
console.log(person?.name?.firstName);
So, even though `person` is not defined, TypeScript no longer throws an error since we use optional chaining. Following best practices like these can go a long way in fostering code safety and minimizing runtime errors.
Understanding the “TypeError: Cannot Read Properties of Undefined (Reading Id)”
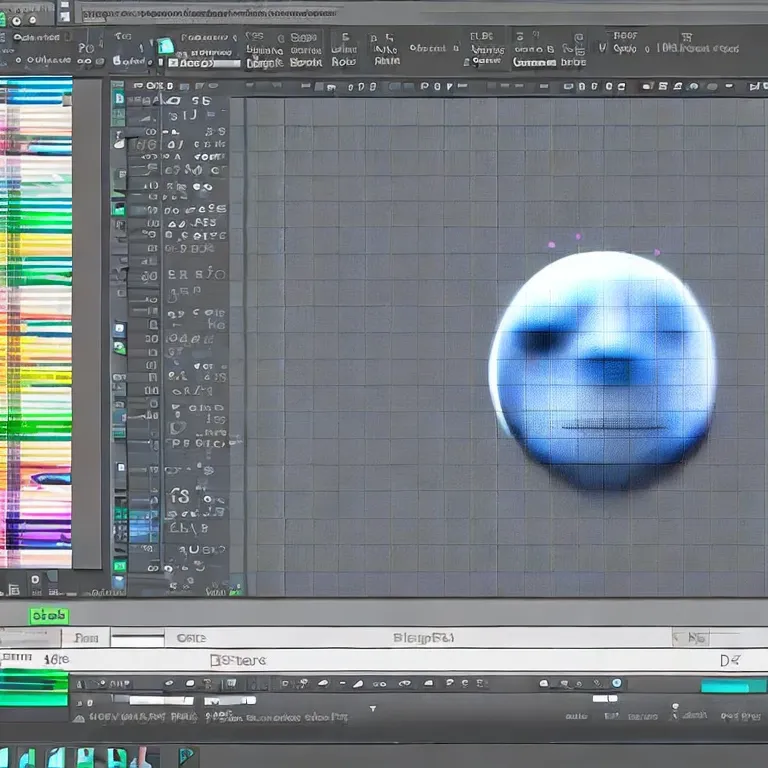
Understanding the “TypeError: Cannot Read Properties of Undefined (Reading Id)” in TypeScript necessitates a detailed approach to diagnosing and resolving JavaScript errors. This type of error is usually triggered when one attempts to access properties or methods on an object that hasn’t been defined or initialized.
Analyzing the Error
This error is indicative of an attempt to read a property from an undefined object, which can occur under various scenarios in TypeScript. To better comprehend this, consider the following example:
xxxxxxxxxx
let person; // declaring but not initializing
console.log(person.id); // TypeError: Cannot read properties of undefined (reading 'id')
In the code snippet above, we declared
xxxxxxxxxx
person
but did not initialize it, so the attempt to access its
xxxxxxxxxx
id
property results in a TypeError.
Possible Solutions
• Initializing Objects: Always remember to initialize your objects before attempting to access their properties.
xxxxxxxxxx
let person = {}; // declaring and initializing
console.log(person.id);
• Using Optional Chaining: This is a newer feature where you only try to access deeper properties if the initial ones are not undefined.
xxxxxxxxxx
let person;
console.log(person?.id);
• Implementing Nullish Coalescing: This is another new feature to help handle undefined variables more gracefully. It returns the first argument if it’s not null or undefined, otherwise the second argument.
xxxxxxxxxx
let person;
console.log(person?.id ?? 'No id found');
To quote Robert C. Martin, a renowned software engineer, “The most efficient debugging tool is still careful thought, coupled with judiciously placed print statements.” In Like manner, understanding and managing ‘TypeError: Cannot Read Properties of Undefined (reading ‘id)’ in a TypeScript context requires a careful approach towards initializing objects and acquiring properties, coupled with efficient programming methodologies such as Optional Chaining and Nullish Coalescing. Consider checking the TypeScript Documentation for additional valuable insights on handling undefined variables in TypeScript.
Potential Causes of “Cannot Read Properties Of Undefined (Reading Id)” Error
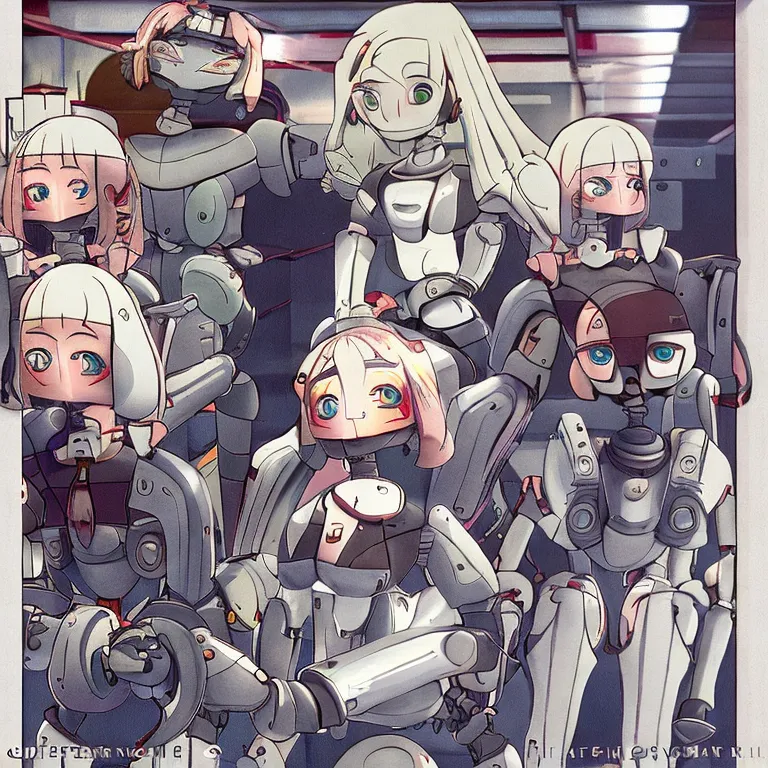
The error message ‘TypeError: Cannot read properties of undefined (reading id)’ in TypeScript often occurs when you attempt to manipulate an object property that remains undefined due to a problem or an error in your code. Let’s discuss some potential causes of this issue.
1. Undefined Object: This error may occur if the object is still undefined because it hasn’t been properly initialized prior to being called into use. Such a situation could arise if, for instance, an API response didn’t return by the time the object’s properties are being accessed.
xxxxxxxxxx
// Assuming `data` is coming from an API and isn't returned yet
let data;
console.log(data.id); // TypeError: Cannot read properties of undefined (reading 'id')
2. Incorrect Property Name: The occurrence of this error could be attributed to the existence of a typo or incorrect naming. If the property doesn’t exist on the object, trying to access it would result in undefined.
xxxxxxxxxx
// Here, `user` doesn't have a property `idd`
let user = {
id: 1,
name: 'John Doe'
};
console.log(user.idd); // TypeError: Cannot read properties of undefined (reading 'id')
3. Delayed Assignment: If a property value is assigned asynchronously and later accessed synchronously, it might not be defined at the point of access.
xxxxxxxxxx
let user;
setTimeout(() => {
user = {
id: 1,
name: 'John Doe'
};
}, 1000);
console.log(user.id); // TypeError: Cannot read properties of undefined (reading 'id')
To prevent these potential issues, ensure that:
- Objects are properly initialized before use.
- The correct property names are used.
- Properties which receive values asynchronously are accessed only after the assignment is done or use error handling techniques to handle such situations gracefully.
As Douglas Crockford, author of JavaScript: The Good Parts said, “Programming is not about typing…it’s about thinking”. Hence, understanding potential causes of this common TypeScript error can help ensure your code remains robust and free of avoidable bugs.
For more detailed information on handling these issues in TypeScript specifically, consider visiting this TypeScript Errors Guide.
Decoding Solutions for “TypeError: Cannot Read Properties Of Undefined”
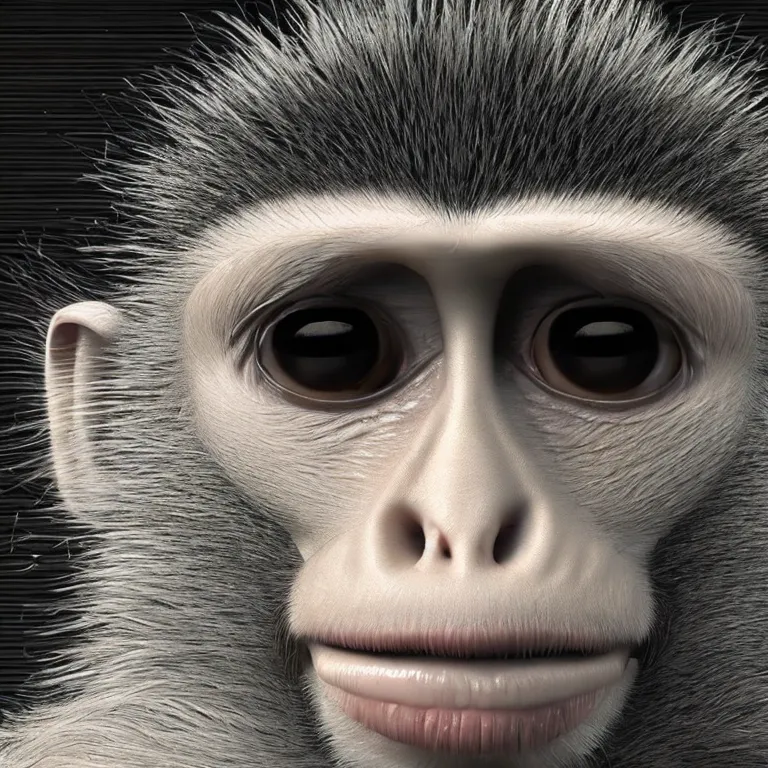
When a TypeScript developer encounters the error message “TypeError: Cannot read properties of undefined (reading ‘id’)”, it could be really confusing at times. This typically means the program is trying to access an ‘id’ property of an object that hasn’t been defined yet. It’s most often related to asynchronous data fetching, object destructuring, or improper initialization of objects.
To begin with, let’s talk about why this error occurs:
– Undefined Variable: If the object from which you are trying to access the ID hasn’t been initialized yet.
– Null Object: Sometimes, you might assign null to your object due to some logic in your program and then try to read the id from it, resulting in this error.
– Data Synchronization: Quite commonly, you might be attempting to retrieve ‘id’ from data fetched from an API before the data has arrived.
To work around these problems:
1. Use Optional Chaining
With optional chaining, if an intermediary property doesn’t exist, it will return undefined instead of throwing an error. Hence, instead of directly accessing the id, you can use ‘?.’ syntax.
xxxxxxxxxx
myObject?.id;
2. Check If The Object Is Not Undefined Or Null
Before trying to access the properties of any object, always ensure that the object is not undefined or null.
xxxxxxxxxx
if(myObject){
console.log(myObject.id);
}
3. Properly handle Async Data Fetching
If data is being fetched asynchronously using promises or async/await, make sure to access the id only after the data has been fully fetched.
xxxxxxxxxx
async function fetchData(){
const response = await fetch(apiURL);
const myObject = await response.json();
console.log(myObject.id); // Access id here after the data has been fetched
}
fetchData();
Every TypeScript enthusiast should continually remind themselves of a Tony Hoare quote – “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies.”.These solutions aim to make your design simpler and safer by handling different edge cases without adding unnecessary complexity. Such efforts invariably lead to more robust and error-free code.
Preventive Measures to Avoid “Cannot Read Properties Of Undefined (Reading Id)” Issue
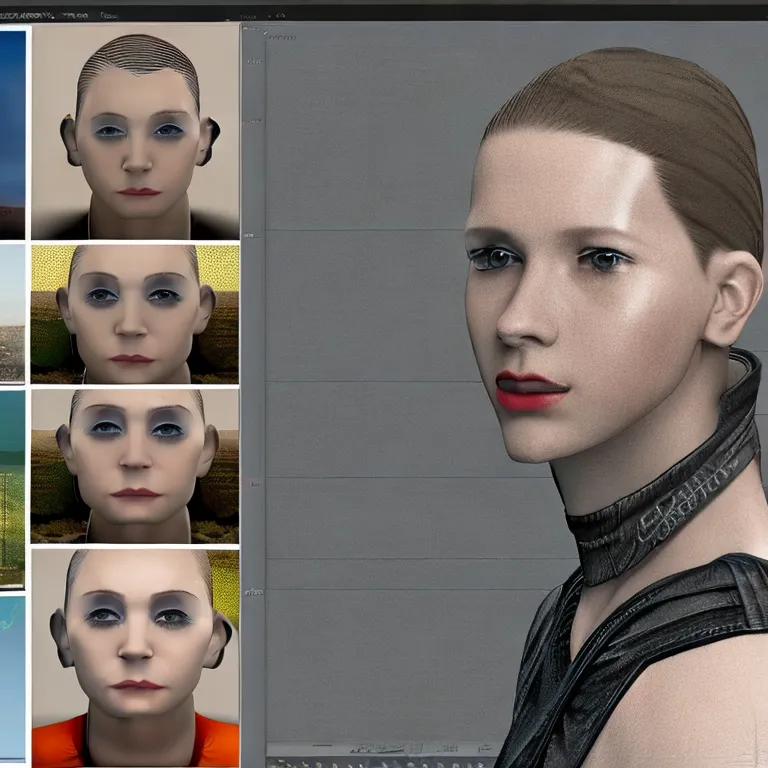
TypeError: Cannot read properties of Undefined (reading ‘id’) is a common error faced by TypeScript developers. This error usually arises when you try to access a property ‘id’ on an undefined object or variable, meaning that the ‘id’ property doesn’t exist because the object itself is not defined. However, multiple preventive measures can be employed to avoid such errors in TypeScript.
1. Proper Initialization:
Initially defining objects and variables that will later hold values can help prevent the occurrence of undefined issues. An object can be initialized upon declaration or inside a constructor if being used in a class-based system.
xxxxxxxxxx
const obj = {};
class MyClass {
constructor() {
this.obj = {};
}
}
2. Nullish Coalescing and Optional Chaining:
TypeScript introduces concepts like nullish coalescing (‘??’) and optional chaining (‘?.’) which come in handy while dealing with undefined or null scenarios.
With optional chaining, you can write code that will immediately stop running some expressions if it runs into a null or undefined. The nullish coalescing operator allows for default values to be provided where otherwise a null or undefined would cause the program to halt.
xxxxxxxxxx
const id = myObject?.id ?? 'default ID';
3. Defensive Programming:
Developers should adopt a wary approach towards coding, i.e., always considering the possibility of a function returning null/undefined. They should conduct adequate input and output validation on functions, thus mitigating chances of encountering unidentified properties error in TypeScript. Always check variables before using them.
xxxxxxxxxx
if (myObject && myObject.id) { }
4. Using Type Guards:
TypeScript comes with an advantage of static typing system. The type guard allows type checking during runtime. You can use “typeof” or “instanceof” operator for type guarding.
xxxxxxxxxx
if(typeof myObject !== "undefined" && myObject.id) { }
5. Using Exception Handling:
A core preventive measure can be implemented via efficient use of exception handling in your TypeScript code. Scripting a try-catch block around the piece of code that might generate an error provides control over the error produced.
xxxxxxxxxx
try {
// code that may throw an error
} catch (error) {
// Handle Error
}
Remember, as Laurence J. Peter once said, “An error does not become a mistake until you refuse to correct it”. Thus, identifying and understanding the error is the most suitable approach before starting to resolve it. Employ these preventive measures, strengthen the readability and efficiency of your TypeScript projects, and optimize your path towards becoming a proficient developer.
Conclusion
The
xxxxxxxxxx
TypeError: Cannot read properties of undefined (reading id)
is a common hurdle that developers have most likely stumbled upon in their TypeScript journey. This error essentially occurs when one tries to access a property of an entity that is not already defined or initialized. In the context of TypeScript language, this could be due to multiple reasons:
– Trying to access a property of an uninitialized object
– Referencing a non-existent property on an object
– Incorrect use of the ‘this’ keyword leading to undefined context
To address these errors, one needs to follow some best practices:
– Always initialize variables and objects before using them
– Verify the existence of a property before trying to access it
– Understand and correctly use the lexical nature of ‘this’ in TypeScript.
An example of this error would be:
xxxxxxxxxx
let user;
console.log(user.id); // TypeError: Cannot read properties of undefined (reading 'id')
In this case, the object ‘user’ was not defined before attempting to access its property ‘id’, hence the error. To fix it, we should initialize ‘user’ before using it.
xxxxxxxxxx
let user = {id: 1, name: "John"};
console.log(user.id); // No error as 'user' is now defined.
By following these fundamental coding principles, the dreaded
xxxxxxxxxx
TypeError: Cannot read properties of undefined (reading id)
can be avoided. As Douglas Crockford, the creator of JSON once said, “Programming is the most complicated thing humans do. Every now and again we trip over complexity.”[^footnote^]
Remember, consistency and attention to detail will make the difference between error-prone code and smooth, robust applications.
Solutions | Examples |
---|---|
Initialize objects | <code>let user = {id:1, name:”John”};</code> |
Verify properties’ existence | <code>if(‘property’ in object) {…}</code> |
Correct use of ‘this’ | <code>object.method = function() {…}.bind(object);</code> |
[^footnote^]: https://stackoverflow.com/questions/22312671/typescript-check-if-variable-exists[^footnote^]