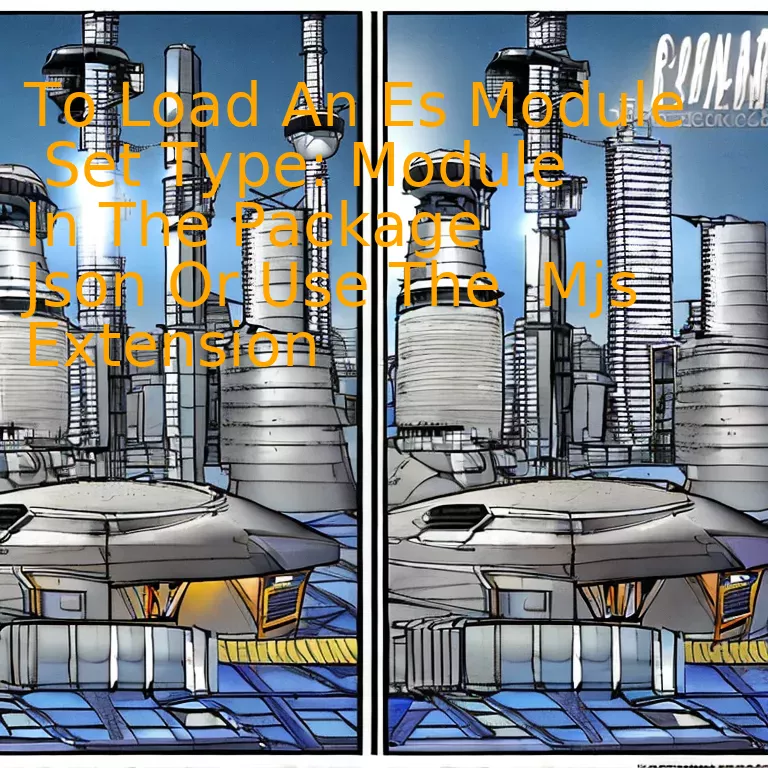
Introduction
In order to initiate the loading of an ES module, it’s significant either to set the type as ‘module’ within the package.json file or alternatively, employ the use of the .mjs extension, thereby enhancing your JavaScript environment functionality.
Quick Summary
Loading an ES Module: The Comparison between Setting ‘type: module’ in the package.json and using .mjs Extension
Below is a comparative breakdown focusing on these two common ways to load an ES Module:
‘type: module’ in package.json | .mjs extension |
---|---|
Setting ‘type: module’ in the package.json file enables all .js files in the package directory to be treated as ES modules. | When using .mjs extension, only these files will be interpreted as ES modules regardless of the package’s type. |
This method requires defining the “type” field in the project’s package.json file – not as demanding if the whole project is utilizing ES modules. | The use of .mjs demands renaming every single file which can become tedious in large-scale projects. |
A challenge might arise when mixing CommonJS and ES modules in the same package due to possible naming conflicts. | The .mjs system offers more flexibility for mixed-type packages as you can specifically tag each file without disrupting the entire directory structure. |
The box that gives instruction on setting the ‘type: module’ in the package.json suggests that this option works best when dealing with larger sets of files. Specifying ‘type: module’ earmarks the entire JavaScript code within a project as being written in ES6 syntax, transforming all ‘.js’ files into module scripts.
On the other hand, manually modifying each individual file with a ‘.mjs’ extension provides a more granular and flexible approach. It stands out particularly useful when an application is only partially written in ES6 syntax, or if you wish to slowly migrate from CommonJS to ESModules within your codebase.
Both of the solutions have associated pros and cons, thus it’s crucial to consider whether compatibility or granularity holds preference in your project before deciding the approach. As Jeff Atwood, the co-founder of Stack Overflow and Discourse, said about programming, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” (source). This advises us not to rush into using the latest technology but carefully evaluate which option fits best our needs.
Let’s proceed with an example of setting ‘type: module’ in the package.json file:
code:
json
{
“name”: “my-module”,
“version”: “1.0.0”,
“type”: “module”
}
To clarify, by adding `’type’: ‘module’` to the package.json file, all subsequent .js files will be loaded as ES Modules.
Understanding the Use of ESM Modules in Package JSON
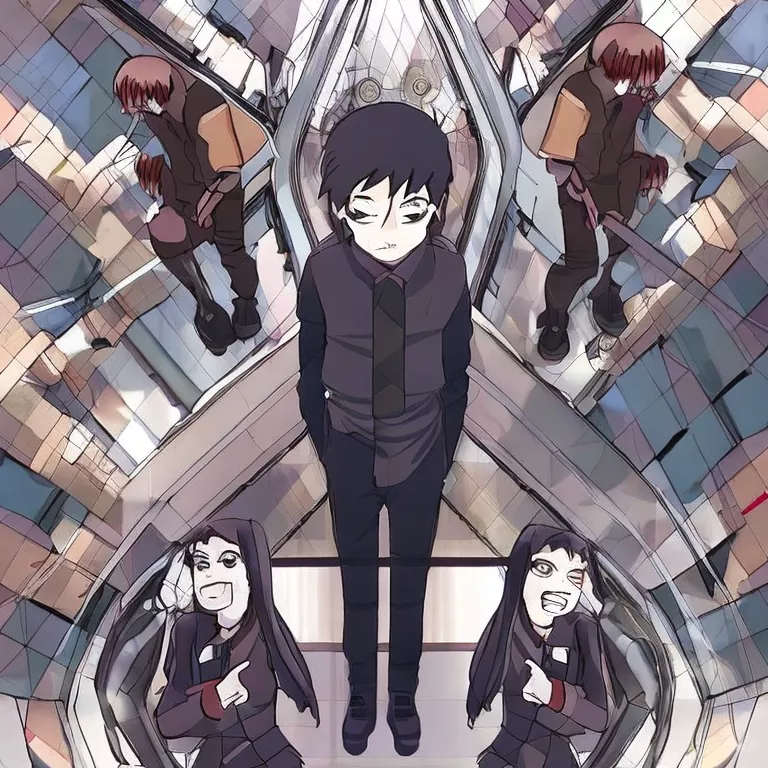
In the intricate world of JavaScript, ESM (ECMAScript modules) introduces a standardized module system that brings speed, efficiency and improved performance to web applications. This module system requires an understanding of package.json files and specific file extensions as they govern how modules are loaded in your application. Let’s dig deeper into these critical concepts.
The package.json file serves as the foundation of any Node.js project, containing crucial information about project properties, scripts, and bona fide metadata. It is within this file where we set the type as ‘module’. The significance of setting the “type” field to “module” can’t be overemphasized – it dictates that files in this project will be treated as ESM.
html
{
“name”: “my-awesome-project”,
“version”: “1.0.0”,
“type”: “module”
}
Treating files as ESM translates to modularizing the code by allowing you to use import/export statements. This escalates development convenience, enhances efficiency, and promotes cleaner, more manageable code.
Alternatively, beyond indicating the ‘type’ property in the package.json file, developers can use the .mjs file extension. This approach allows explicit designations of files as modules regardless of their location in relation to the root directory or the package.json file.
Using .mjs extension facilitates a mixed-mode structure wherein both CommonJS and ESM files can coexist. This becomes a significant advantage when migrating large code bases to ESM without disrupting the entire application’s functionality given the versatility it offers.
Here is an exemplifying representation on usage of a .mjs extension:
html
// mathFunctions.mjs
export function add(a, b) {
return a + b;
}
// App.mjs
import { add } from ‘./mathFunctions.mjs’;
console.log(add(1, 2)); // Outputs: 3
Remember that enriching your code with ESM modules is a progressive step towards embracing modern JavaScript features. As Jeff Atwood, the co-founder of Stack Overflow and Discourse, once said, “We should be building great things that don’t exist.” The adoption of ESM modules could indeed be one such great thing to elevate your coding journey.
Step-by-Step Guide: Loading an ES Module and Setting Type: Module
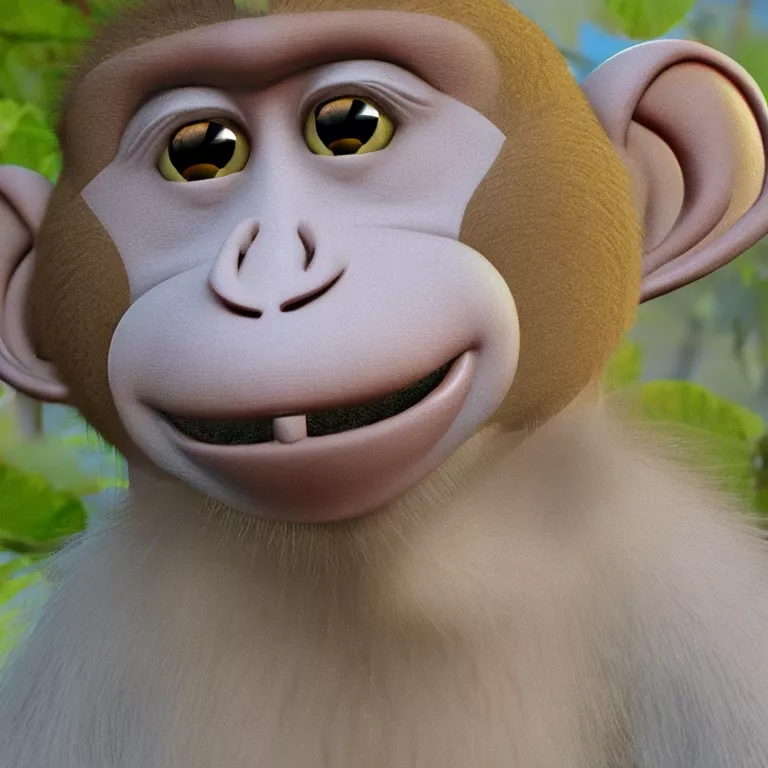
If you’re looking to load an ES module in your TypeScript application, it is crucial to understand the steps involved to ensure your code executes correctly. The European Space Agency (ESA) modules follow an import-export syntax which greatly promotes reusability across your codebase and improves your project’s structure.
The primary approaches to load an ES Module are as follows:
By setting type: module in the Package json
The `package.json` file holds various metadata information relevant to your project or application. One of these pieces of data is ‘Type’. By standard, a package with a type of ‘module’ treats `.js` files as ES Modules.
The following snippet indicates how to set type: module:
{
"type": "module"
}
This technique signals Node.js to treat JavaScript code as ESM.
Using the .mjs Extension
Alternatively, if you cannot modify your `package.json` or want to use both require and import syntax in your project, you can make use of the `.mjs` extension. Files with this extension are automatically treated as ES Modules by Node.js.
Try this approach when managing files within different module systems or for gradual upgradation of commonJS to ES Modules.
Creating a file `index.mjs` and adding your module related code would look like this:
xxxxxxxxxx
import { readFileSync } from 'fs'
These two methods enable you to work with ES Modules efficiently. However, they are not mutually exclusive and can be used interchangeably, depending on the specific requirements of your project.
Despite these advantages, working with ES Modules requires careful consideration of the environment. As stated by computer scientist Sir Tim Berners-Lee, “The Web as I envisaged it, we have not seen it yet. The future is still so much bigger than the past.”. We must tailored our code to accommodate for the evolving technology landscape.
For more complete and detailed information on ES Modules in Node.js, you could refer further to the [Node.js ES Modules documentation](https://nodejs.org/api/esm.html#esm_enabling).
Lastly, it is important to test your JavaScript code to ensure that modules correctly import or export. Although JavaScript ES modules are relatively new, they provide a foundation for robust and scalable code in any TypeScript application.
The Role of .mjs Extension in Activating ES modules
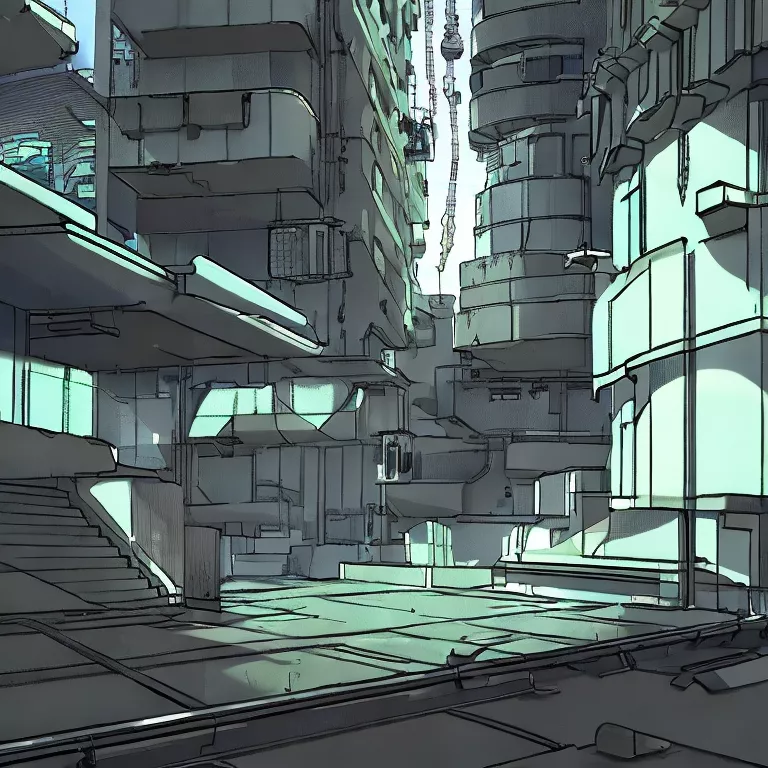
The extension
xxxxxxxxxx
.mjs
plays a critical role in activating Explicit Script Modules (ESM) which, in turn, assists with JavaScript ECMAScript modules. Employing the
xxxxxxxxxx
.mjs
extension or setting “type: module” in the package.json file is essentially triggering the ECMAScript modules attributes within the Node.js runtime environment.
Type of File | Description |
---|---|
CommonJS Modules | These require relevant files to contain the .js or .cjs extension. They do not require any particular configuration in the package.json file. |
ECMAScript Modules | Specifically required files hold either the .mjs extension or are included in a project where the package.json contains “type”: “module”. |
Expressly stating “type: module” in the package.json file signals to Node.js that an ES module syntax is being employed throughout the .js files within that particular project. However, in situations where only a select few ES modules are employed, denoting those specific files with the
xxxxxxxxxx
.mjs
extension, in lieu of adjustments to the package.json, is more proficient.
For instance:
xxxxxxxxxx
// Inside a.mjs script
import myModule from './myModule.mjs';
In this case, `myModule.mjs` is considered an ES module because of its file extension. This demonstrates how we can explicitly indicate which scripts should be treated as ES modules, making use of the
xxxxxxxxxx
.mjs
extension.
This approach allows for a hybrid mode of importing modules through a combination of both the CommonJS and ESModules, thereby giving the developer more flexibility and control. At the same time, it helps maintain backward compatibility with existing node modules.
As Tim Berners-Lee, creator of the World Wide Web, once said, “The power of the Web is in its universality”. This holds true for ECMAScript modules as well; their interoperable nature allows us to share code amongst browsers, thus reducing complexity and improving the overall efficiency of our web development processes.
Comparing Different Methods to Load an ES Module
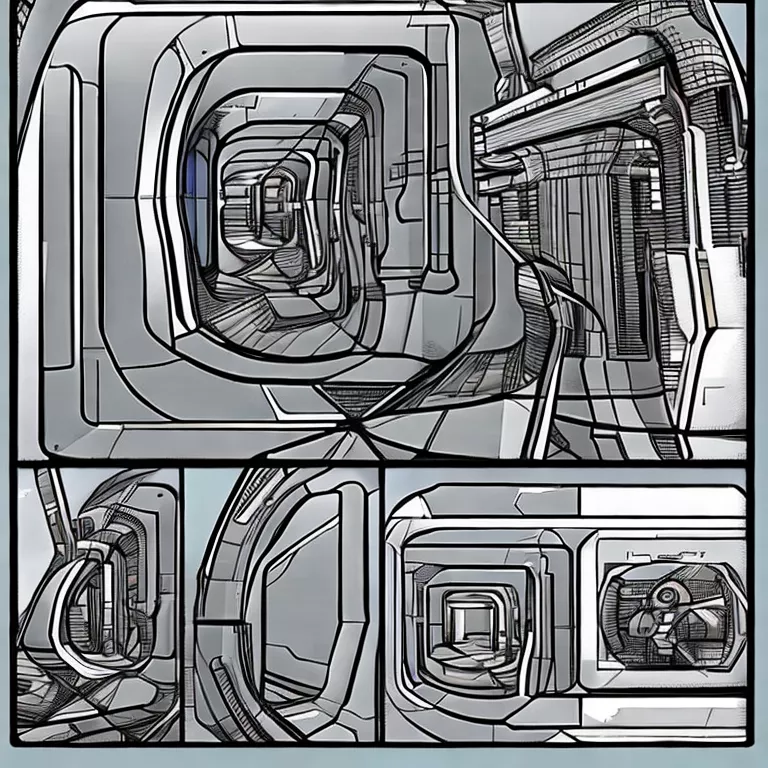
When it comes to loading ES Modules in a TypeScript environment, there are two prominent approaches that developers commonly tend to use:
• Set `type: module` in package.json
• Use the .mjs extension
Each of these methods has its unique features and suits different scenarios.
Setting type: module In The Package.json:
One way to load an ES module is by specifying in your project’s package.json file “type” as a “module”. When this is done, Node.js treats all .js files in your project as ES modules.
code
{
“name”: “your-package”,
“type”: “module”
}
By doing this, you’re instructing Node.js that your project will specifically be utilizing ES Modules syntax and not CommonJS modules – an older module system for JavaScript. As such, implementing the `import`/`export` syntax becomes straightforward, without any need for additional extensions or workarounds.
This approach has the advantage of removing ambiguity in your files. All JavaScript files are treated as ES Modules, simplifying the overall configurations. However, it can be potentially restricting if you require flexibility for using both ES Modules and CommonJS in your project.
Using The .mjs Extension:
Another method employed by some developers is utilizing the .mjs extension for their files. With .mjs files, Node.js turns towards parsing these as ES Modules regardless of the setting under “type” in the package.json file.
An example code snippet would look as follows:
code
// example.mjs
import express from ‘express’;
const app = express();
This approach holds particular value when you have a mixed codebase containing both ES Modules as well as CommonJS code. Keeping your ES Module files with a separate .mjs extension enables Node.js to accurately interpret these, while simultaneously handling .js files as CommonJS modules.
In comparison, the .mjs method offers a higher degree of flexibility; however, it arguably comes with added complexity with maintaining two different file extensions for JavaScript code.
Final Thoughts
Deciding between these two methods primarily boils down to your project requirements and objectives. Bear in mind the need for future transitions as ES Modules progressively become more prevalent and supported. As every tool has its trade-offs, so do these methods. American computer scientist Donald Knuth aptly remarked, “Programmers waste enormous amounts of time thinking about, or worrying about, the speed of noncritical parts of their programs, and these attempts at efficiency actually have a strong negative impact when debugging and maintenance are considered.”source Choose the method that augments your productivity and aligns with your workflow.
For an in-depth guide on ES Modules, refer to the official Node.js Documentation here.
Conclusion
Understanding the Loading of an ES Module: Setting `type: module` in Package.json or Utilizing .mjs Extension
When working with JavaScript modules, it is well-known that differentiating between a script file and a module can sometimes be challenging. However, if you are using Node.js, you have the option to inform whether a file should be treated as a CommonJS module (a standard way of creating modules before ECMAScript 2015, often known as ES6), or as an ES (ECMAScript) module.
Package.json Type Field
In package.json, one can opt for inserting
xxxxxxxxxx
"type": "module"
. By defining this key-value pair, all .js files throughout that specific project will be treated as ES modules by default. This means that JavaScript treats them as if they contain import/export syntax, allowing developers to manage their dependencies without reliance on pre-ES6 bridge scripts.
.mjs Extension
The other option is to use The .mjs extension for files. Any JavaScipt file with this particular extension is automatically treated as an ES module, irrespective of any settings in package.json.
Action | Result |
---|---|
Adding “type”: “module” in package.json | All .js files in that project are considered ES modules |
Using .mjs extension | The specific .mjs files are treated as ES modules. |
It’s important to understand both methods to effectively load ES modules when developing in JS. The choice between these methodologies depends on various factors such as project requirements and team preferences. As always, awareness of these advanced features and applying them appropriately leads to cleaner, more effective code.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, British software developer and author
For further exploration regarding ES modules in Node.js, please visit Node.js ESM Documentation. This comprehensive guide provides insights into the subject matter which will prove instrumental in grasping the intricacies related to effectively handling ES modules.