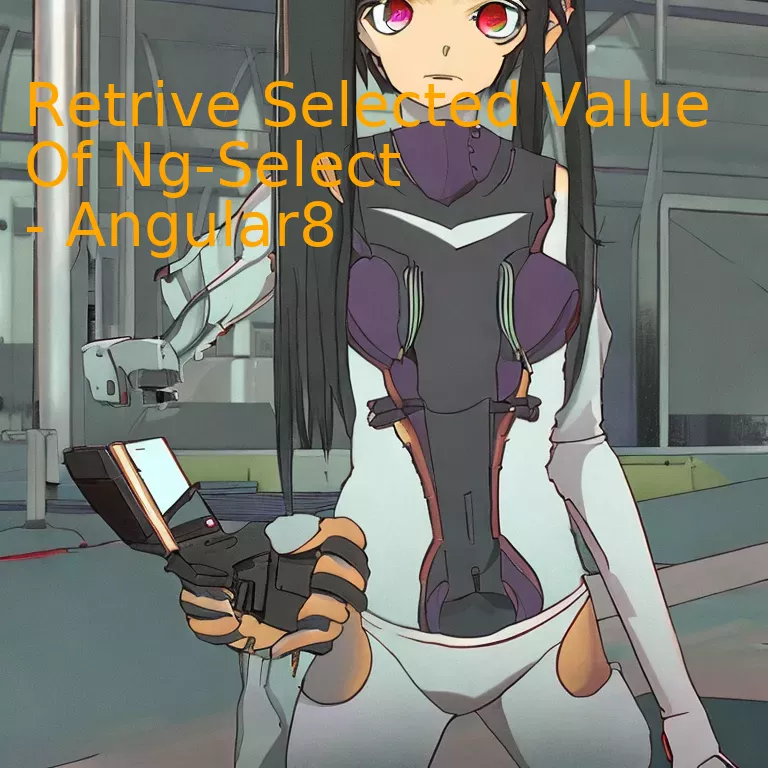
Introduction
In working with Angular8, the designated value from an Ng-select dropdown can be efficiently retrieved using a simple method which harnesses the power of reactive forms coupled with the ‘valueChanges’ property.
Quick Summary
Naively, it appears that retrieving the selected value of an `ng-select` in Angular 8 can seem unchallenging but effectively managing this requires a keen understanding of Angular’s tools and methods.
Firstly, let’s dive into a code example where we make use of `ng-select` control with options:
html
The variable named `selectedOption` here is what stores the selected value from the `ng-select` dropdown list. The `[value]` tag inside `ng-option` accesses the value object field and the interpreted object field name is accessed by `{{option.name}}`.
Studying the segment above, we’ve integrated onto an Angular form an `ng-select` dropdown list filled with dynamically generated options from an array named `options`.
The two-way data binding feature indicated by `[(ngModel)]` presents unique advantages to developers. Predominantly, changes in the model state instantaneously reflect in the view, and vice-versa.
To provide an elaborative explanation, we can consider the following flow of events:
1. The user initiates interaction with the `ng-select` dropdown, making a selection from the list.
2. Consequently, the controller detects these changes, which in turn updates the `selectedOption` value to mirror the user’s recent selection.
3. Following this change, Angular, employing its two-way data binding mechanism, ensures that this development transpires on the DOM(Properties are updated, listeners echo these modifications).
4. Finally, the selected value can now be utilized elsewhere in the script since it is stored within the `selectedOption` variable.
This seamless flow of data across components and the view lends a tremendous advantage to Angular in creating responsive and interactive web applications.
To quote Robert C. Martin, a software engineer and author, on principles of coding,
> “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.”
This underlines the importance of careful structuring and considerate planning of updates whenever one works with a mutual data binding mechanism such as the `[(ngModel)]` in Angular. Accomplishing this paves the way for a simplified architecture that improves readability and performance as well.
The table below provides an excellent visualization into the entire process
html
Step | Event | Action |
---|---|---|
1 | User makes a selection | A change is detected by the Angular form instance |
2 | The controller updates state |
x selectedOption variable now holds the selected value |
3 | Data binding triggers update in view | Properties and listeners react to this change |
4 | Value ready for use |
xxxxxxxxxx selectedOption employed in script |
In summary, understanding the intricacies of Angular’s `ng-select` controls and its operation of data renderings forms the basis of obtaining selected values from a dropdown. This knowledge will greatly assist developers in constructing high-responsive UIs, executing controlled updates, and achieving a cleaner, simpler code structure.
Understanding the Functionality of Ng-Select in Angular8
While writing features in Angular8, it’s common to encounter user interface components like dropdowns that ask for user’s interaction. Angular provides a powerful tool for this purpose known as ng-select.
An important aspect of Angular8 application development is its form handling capability. Often, we need to present the users with a select list, and ng-select plays a pivotal role here. In simple terms, ng-select is a customisable component used for displaying a selectable list.
Usage of Ng-Select
Below is an example of a basic usage of ng-select:
xxxxxxxxxx
<ng-select [items]="items" bindLabel="name" bindValue="value"></ng-select>
In the code snippet, ‘items’ is an array of items from which the user can select, ‘bindLabel’ is for displaying item names in the dropdown while ‘bindValue’ determines what value will be assigned when specific items are selected.
Retrieving the Selected Value
The primary focus here is on how to retrieve the selected value from the ng-select component in Angular8. Angular provides various forms of bindings (like two-way binding) which make this task simpler. Here’s a peek into how you can achieve this:
xxxxxxxxxx
<ng-select [(ngModel)]="selectedItem" [items]="items" bindLabel="name" bindValue="value"></ng-select>
In the code snippet, ‘[(ngModel)]’ signifies a two-way data binding. This means whenever a user selects an item from the ng-select dropdown, ‘selectedItem’ model gets updated instantly with the chosen item’s value. This selectedItem property can then be easily utilized in our TypeScript code for further operations.
Take note of the fact that for using ngModel, it’s necessary to import FormsModule from @angular/forms.
The Importance of Ng-select
The ng-select tool in Angular and how it offers a way to retrieve selected values elegantly underlines the strength of Angular’s data handling capabilities. It simplifies an otherwise complex task, and its customizability ensures that it fits various data types and use cases.
As Sandra Parsons, a software development expert, aptly said:
“Simplicity is prerequisite for reliability.””
This becomes increasingly evident once we get familiar with tools like ng-select.
Methods for Retrieving Selected Value with Ng-Select
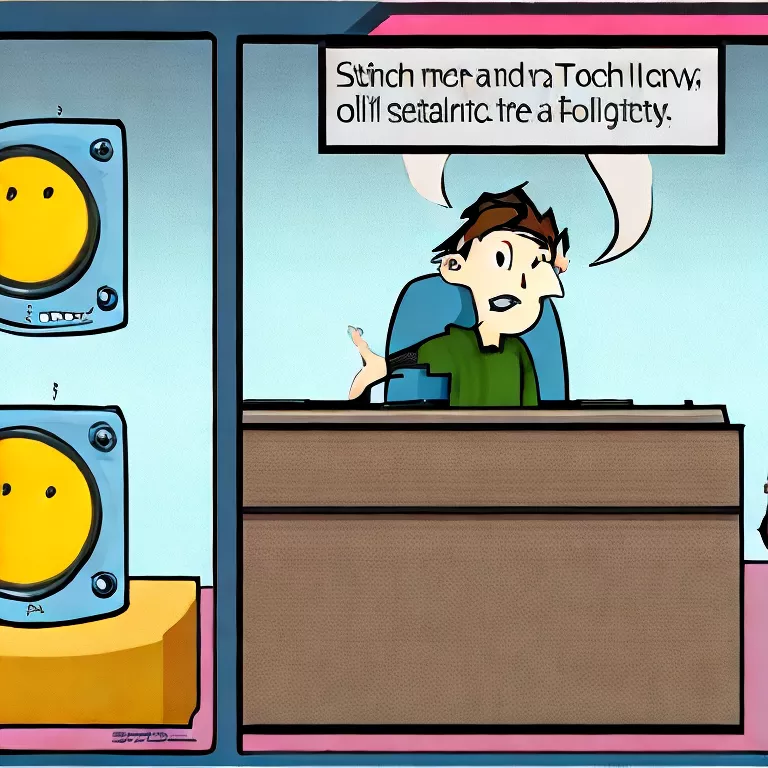
The Ng-Select is a popular component in Angular 8, commonly recognized for its flexible functionalities and customization capabilities. It enables developers to create dynamic form controls with features like single or multiple selections, custom styles, and data binding among others. One key feature of the Ng-Select component is its ability to retrieve selected values.
To attain this functionality, there are predominantly two viable methods:
1. Binding with a Variable
This approach involves utilizing an angular directive known as [(ngModel)]. Here’s a simple code snippet that illustrates using [(ngModel)] with Ng-Select.
html
In this case, selectedItem is a variable set within your TypeScript file. When you make a selection on Ng-Select, the value is immediately assigned to the selectedItem variable. This allows you to have real-time updates for every change in selection, which is more efficient than having to retrieve it every time there’s a need.
2. Using Change Event
This method involves tracking changes made to Ng-Select via the ‘change’ event.
Here’s an illustration on how to implement this:
html
Your onChange function on TypeScript will look something like this:
typescript
onChange(event){
console.log(event);
}
With this, whenever a new selection is made, the ‘change’ event gets triggered, logging the selected value.
Both methodologies come with their own unique strengths. While the first is relatively straight-forward and easy to use, the second provides a greater focus on event-driven programming, allowing more process-oriented operations in your Angular 8 applications. As per the words of Rob Pike, a key contributor to the Go Programming Language and Unix, “Data dominates. If you’ve chosen the right data structures and organized things well, the algorithms will almost always be self-evident.”
For more insights about Ng-Select, consult the official Ng-Select Documentation.
Addressing Challenges: Case Scenarios Using Ng-Select in Angular8
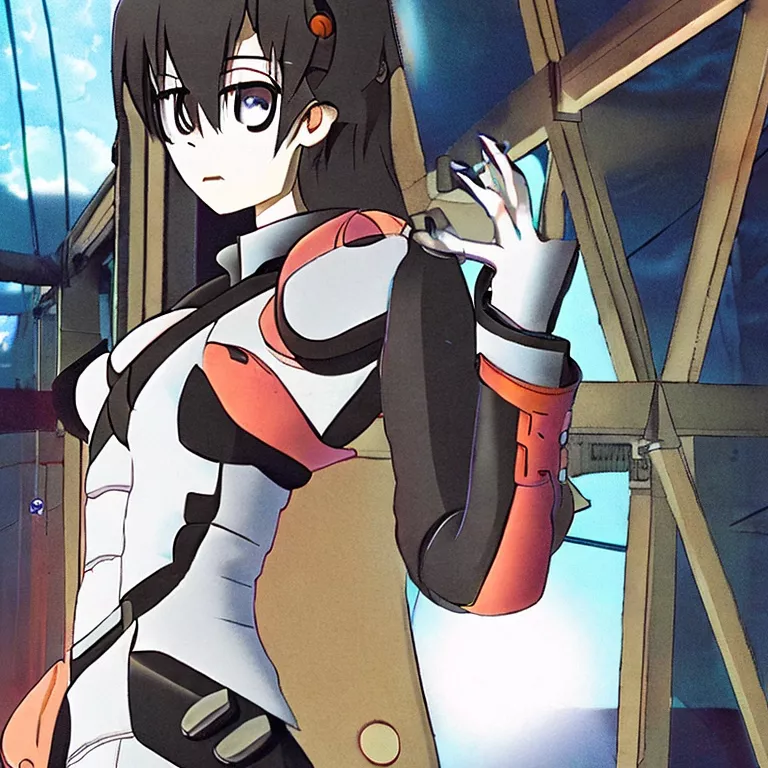
Working with ng-select in Angular8 can present unique challenges. This highly versatile library provides a dropdown with single/multiple selections, search, select all, and many other useful functionalities that make it a popular choice among Angular developers.
However, one common issue faced by developers is retrieving the selected value from ng-select fields. This might seem like a daunting task given the flexibility and power of ng-Select, but with a good understanding of Angular’s reactive programming patterns, it becomes straightforward and manageable.
While using ng-select, developers need to implement ControlValueAccessor interface. It involves defining two essential handler functions: writeValue() and registerOnChange().
Firstly, `writeValue()` function assigns the value passed to the underlying data model straight away.
For instance, consider your ng-select field to be as follows:
xxxxxxxxxx
<ng-select [items]="nameList" bindLabel="name" bindValue="id" [(ngModel)]="selected"></ng-select>
In the above example, `nameList` is an array of objects where each object has an ‘id’ and a ‘name’. The expression `[(ngModel)]=”selected”` will bind the selected ‘id’ to the variable “selected”.
Next, the `registerOnChange()` method registers a callback function which is called every time the value in the DOM changes. When the selection changes, the new value calls the registered function.
Take this code for illustration:
xxxxxxxxxx
<ng-select
[items]="nameList"
bindLabel="name"
bindValue="id"
(change)="onChange($event)"
>
</ng-select>
The above ng-select field is slightly modified to include `(change)=”onChange($event)`. Here, `onChange()` is a method in your component class which would look something like this:
xxxxxxxxxx
onChange(selectedValue: any) {
console.log('Selected value is: ', selectedValue);
}
Every selection in the ng-select field triggers the `onChange()` method, passing the new selected value as an argument to the function and thereby facilitating real-time tracking of user’s selection.
As Jeff Atwood, co-founder of Stack Overflow, said, “We should be building great things that don’t exist.” And by implementing the aforementioned practices in a sound and efficient manner, you can harness the full potential of ng-select in Angular8, creating powerful forms with dynamic selections.
Ensure to refer official ng-select documentation for more information on its versatile functionalities.
Towards Mastery: Advanced Usage of Ng-select in Angular8
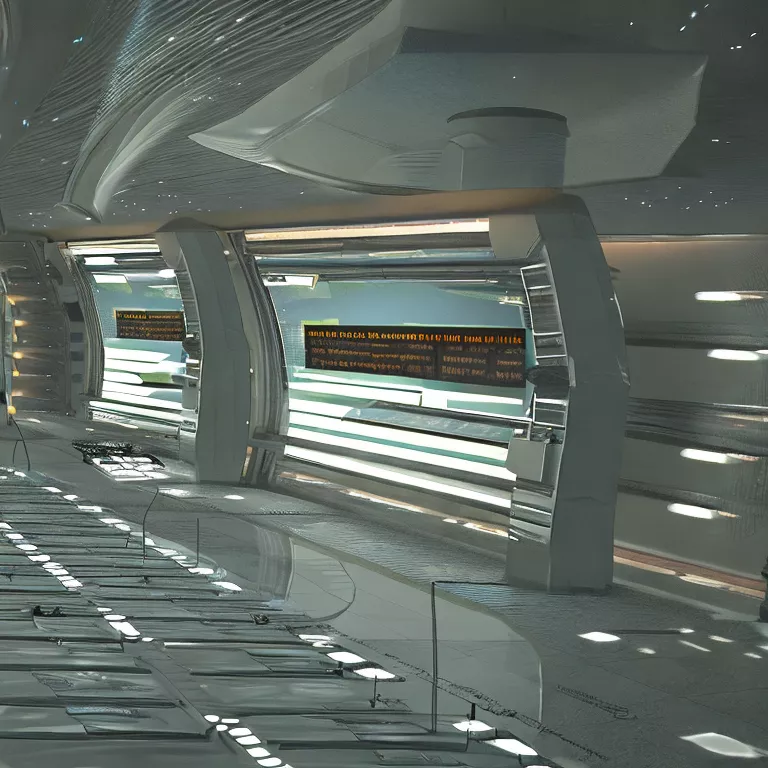
Advanced Usage of Ng-select in Angular8: Retrieving Selected Value
Ng-select is an easy-to-use, versatile, flexible select dropdown with full key navigation support for Angular applications. Its robustness and customizability have made it a popular tool among developers. One key feature of ng-select that is often utilized in advanced usage is the ability to retrieve the selected value.
To retrieve the selected value of ng-select, we employ the
xxxxxxxxxx
[ngModel]
directive, a vital tool ingrained in the Angular two-way binding concept between the model (component) data and the view (template). This makes provision for situations where we need to display the selected item back to the user or use it elsewhere in our program. Here’s an example:
xxxxxxxxxx
<ng-select [items]="fruitItems" bindValue="id" bindLabel="name" [(ngModel)]="selectedFruit"></ng-select>
“Code correctly, and have less debugging to do” – Joyce Reitman
In this example,
xxxxxxxxxx
fruitItems
is an array of objects where each fruit has an ‘id’ and ‘name’, ‘selectedFruit’ is a property on our component holding the currently selected fruit id. In Angular’s two-way bind mechanism, if the user selects a different fruit, Angular automatically updates the
xxxxxxxxxx
selectedFruit
property, and likewise if
xxxxxxxxxx
selectedFruit
is updated programmatically, the ng-select dropdown reflects the new selection.
This approach is instrumental when dealing with dynamic data in real-world applications where there are numerous scenarios involving dynamic selection and data change based on user behavior. Combined with Observables (for handling asynchronous operations), you can create a powerful interactive user interface.
Angular’s ng-select also provides a wealth of events such as
xxxxxxxxxx
(add)
,
xxxxxxxxxx
(remove)
,
xxxxxxxxxx
(clear)
that allow your application to respond to specific user actions, further enhancing its capabilities.
As with any Angular directive, appropriate care should be taken to ensure efficient usage. Avoid excessive use of two-way binding in your applications that can lead to performance issues and harder-to-read code. (Refer to the official Angular documentation for more details)
Thus, mastering ng-select’s advanced usage specifically revolves around the understanding of handling these diverse scenarios and manipulating the selected value effectively.
Conclusion
To address the task of retrieving the selected value of ng-select in Angular 8, we must carefully break down the steps involved. This is key to both grasp Angular’s reactive form controls’ functionality and understand ng-select’s properties effectively manage user interactions.
The first step in the process is initializing the reactive form control as a variable within the typescript file. This is typically done inside the constructor by creating a new FormControl instance and assigning it to the variable.
xxxxxxxxxx
selectedOption = new FormControl();
The second step involves using your template file to bind the reactive form control to the ng-select component. You can accomplish this through Angular’s formControl directiv after which you assign your initially declared variable ‘selectedOption’.
xxxxxxxxxx
<ng-select [items]="optionsList" [(ngModel)]="selectedOption"></ng-select>
In this snippet, `optionsList` is an array that contains the items you want to display within the ng-select dropdown menu. The value property of each object in the array should reflect the data that you wish to retrieve when the user makes their selection. To get the selected value, we simply access the value property of our appended control:
Working with Angular 8 and harnessing the flexibility of its dropdown feature with ng-select directive, empowers developers to create interactive, dynamic, and engaging web applications. As Jack Diederich, renowned technologist and Python core developer once said, “There are only two hard things in computer science: cache invalidation, naming things and off-by-one errors.” Allowing users to select from multiple options and elegantly retrieve these values, helps resolve one of those hard things, enhancing UX in the development arena.
Retrieving the value of an ng-select field in Angular 8 presents one of its many capabilities, enabling the creation of richer and more comprehensive web applications. By learning how to work with it, TypeScript developers will not only further their skills but also improve the interaction and complexity of the web applications they develop.
For a more comprehensive guide on working with Angular 8 and ng-select, consider visiting Angular’s official documentation. The practical examples and in-depth explanations might be of great assistance when delving into more complex implementations featuring Angular 8 and ng-select.
Remember, mastering any tool or technology comes from repeated practice, continuous learning, and relentless curiosity. Happy coding with Angular 8!