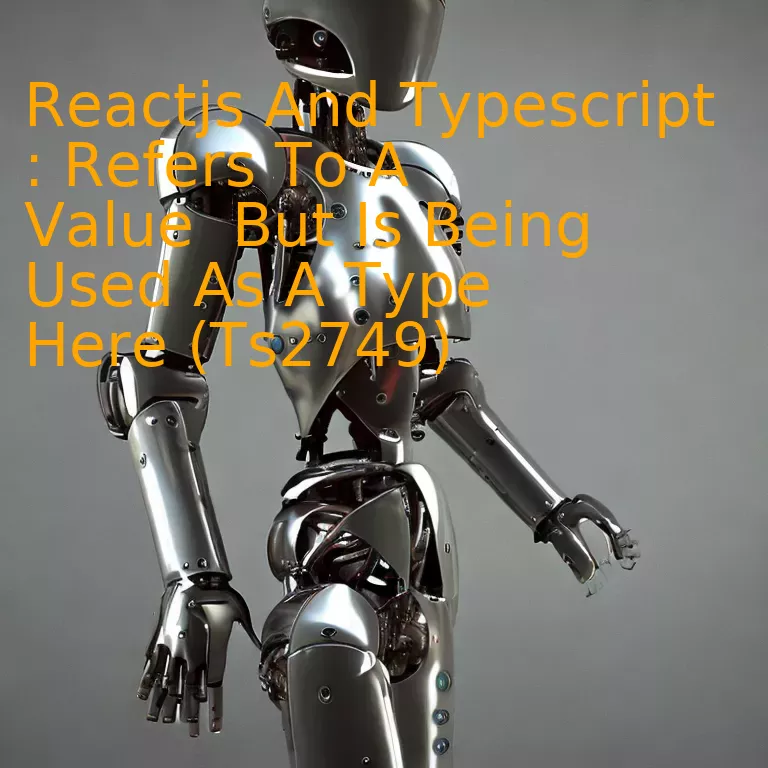
Introduction
When working with the synergism of ReactJS and TypeScript, you might encounter an issue labeled as TS2749, which fundamentally signifies that a value is being mistakenly utilized as a type; this is often a common roadblock developers face in robust code creation.
Quick Summary
The Typescript error TS2749, seen in the context of using React and Typescript together, refers to a scenario when something is being used as a type that actually pertains to a value. This distinction between values and types forms a significant part of Typescript’s static type checking mechanism.
An illustrative table is presented below:
| Term | In JavaScript | In TypeScript |
|——|—————|—————|
| Values | Can be things like functions, variables, literals, objects etc. | Has similar value system as Javascript |
| Types | Don’t exist at all! | Can be custom or primitive (like number, string) |
Essentially, your code attempts to treat a value entity as if it were a type entity, prompting Typescript to raise the TS2749 error. The occurrence is analogous to trying to pay for a product with another physical item rather than with currency – the shop wouldn’t accept it because it’s an inappropriate form of payment. Similarly, you can’t use a value where a type is expected.
Here’s a relatable coding scenario demonstrating this issue:
(modify this with
)
TypeScript
let Foo = () => {}; //Foo is created as a value (a function)
type Bar = Foo; //Error: TS2749. Foo is a value but used as a type here.
You see, Foo is indeed declared as a function (which is a value), yet we try to create a type alias Bar for it. This causes Typescript compilation to fail with TS2749.
Clean, concise code deploys a clear divide between defining values and types in Typescript. As a developer, appreciating this difference and avoiding crossbreeding key to leveraging Typescript’s effectiveness. Resolving these impediments results in stable, reliable, and efficient code that significantly contributes to any company’s digital success journey.
Quoting Martin Fowler, an eminent figure in software development, you could remember: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
For extensive details on this topic and general TypeScript errors, consider visiting the official TypeScript documentation [here](https://www.typescriptlang.org/docs/).
Understanding the TS2749 Error in TypeScript and ReactJS
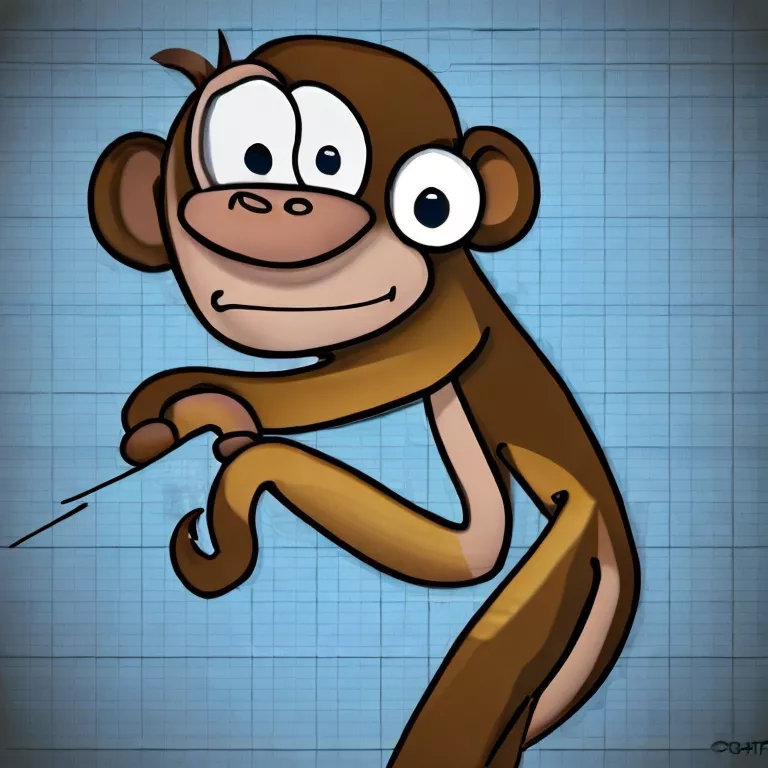
ReactJS, a popular JavaScript library for building user interfaces, often combined with TypeScript, a powerful statically typed superset of JavaScript that adds strict type-checking along with other features, makes the development efficient and less error-prone. However, during this process, developers might encounter various bugs or errors. An error TS2749 in TypeScript while using ReactJS is one such common issue.
The error message states
xxxxxxxxxx
"Refers to a value but is being used as a type here (TS2749)"
, it’s usually seen when you may be trying to import something as a type, but TypeScript recognizes it as a value instead.
Defining Error TS2749:
In programming terms, the ‘value’ refers to variables, functions, objects, etc., whereas ‘type’ denotes the respective type of these values like string, number, any, etc. Henceforth, confusion between these two can lead to this TS2749 error.
// Pretend code showing incorrect use leading to TS2749.
import { useState } from “react”;
interface MyProps {
userId: useState // Here ‘useState’ is recognized as a value (function), not a generic interface
}
Exploring possible solutions:
– The most common cause for this error is an incomplete or wrong set of imports. If you mistakenly import a value as a type or vice versa, TypeScript will throw this error. In that case, carefully reviewing your import statements can often solve your problem.
– It’s also tripped by using the same name for both a type and a variable within the same scope. A solution to this situation would be renaming the type or the value.
// Correct usage of useState hook.
import React, { useState } from “react”;
interface IState {
count: number;
}
const Example: React.FC = () => {
const [state, setState] = useState
// …
};
According to Martin Fowler , a renowned expert in software development, “You can make a mess in code that you slide through reasonably easily, because while the software may be messy, it’s not complex.” This quote pertains directly to understanding and resolving TypeScript error TS2749. When we adopt practices such as detailed checking of import statements and a better organisation of type and value declarations, our software becomes less messy and hence reducing its perplexity.
Debugging TypeScript Errors: A Close look at TS2749
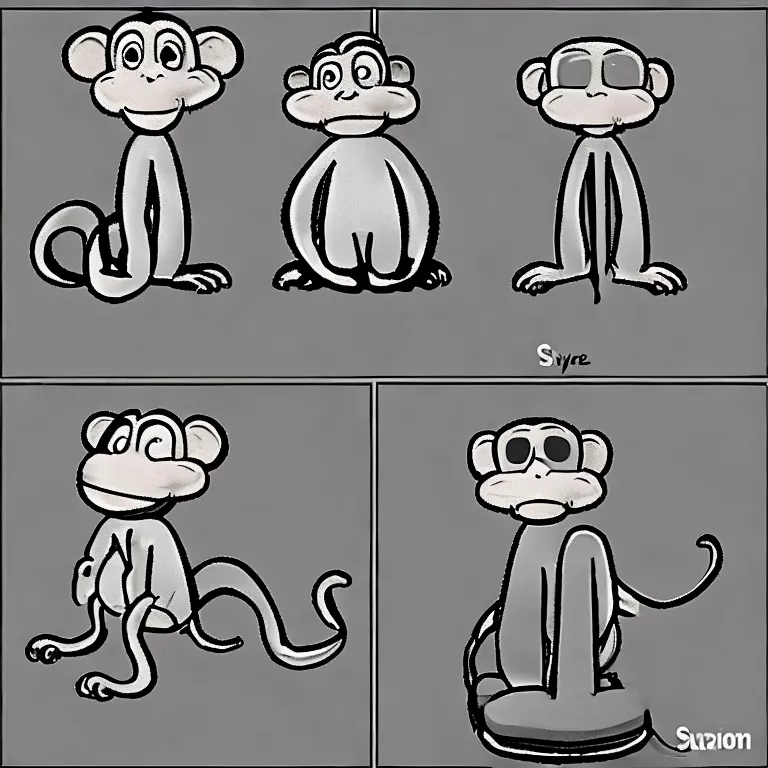
When working with TypeScript in a ReactJS environment, you might come across the error TS2749 – “Refers to a value, but is being used as a type here”. This almost always points to an issue of cross-referencing between types and values especially when defining components or importing modules. Let’s delve deeper into this:
Your first move should be to check your import syntax. In TypeScript, you have to explictly specify whether you’re referring to a type or value. Ensure that you’re not trying to use a value (like a variable or function) where a type is expected. For example:
xxxxxxxxxx
// Wrong
import { Component } from 'react'
class MyComponent extends Component { }
// Correct
import React, { Component } from 'react'
class MyComponent extends Component { }
In the incorrect example, TypeScript is led to think that
xxxxxxxxxx
Component
is a value imported from the module, while in the correct example it is clear that
xxxxxxxxxx
Component
is a type.
Next, inspect the code for any place where a type might be modifying its own definition. If a value is being assigned to a type, TypeScript could raise the TS2749 error because this contradicts the static nature of types in TypeScript.
Also, consider if a value and a type are sharing the same name. TypeScript differentiates types and values even if they share the same name. Check for any get and set property accessors that may be causing confusions between values and types.
If all else fails, try isolating the error by commenting out some lines or creating smaller examples until TypeScript stops complaining. Remember that sometimes the problem is not within the line the error points to but maybe lines above those highlighted.
As Chris Heilmann once said, “Debugging is like being the detective in a crime movie where you are also the murderer.” With the right approach and techniques, TypeScript errors such as TS2749 can be addressed efficiently without much sweat.
For more information, you may refer to this TypeScript 2.0 documentation, which provides insights about how types and values are managed.
ReactJS & TypeScript Interplay: Deciphering the TS2749 Issue
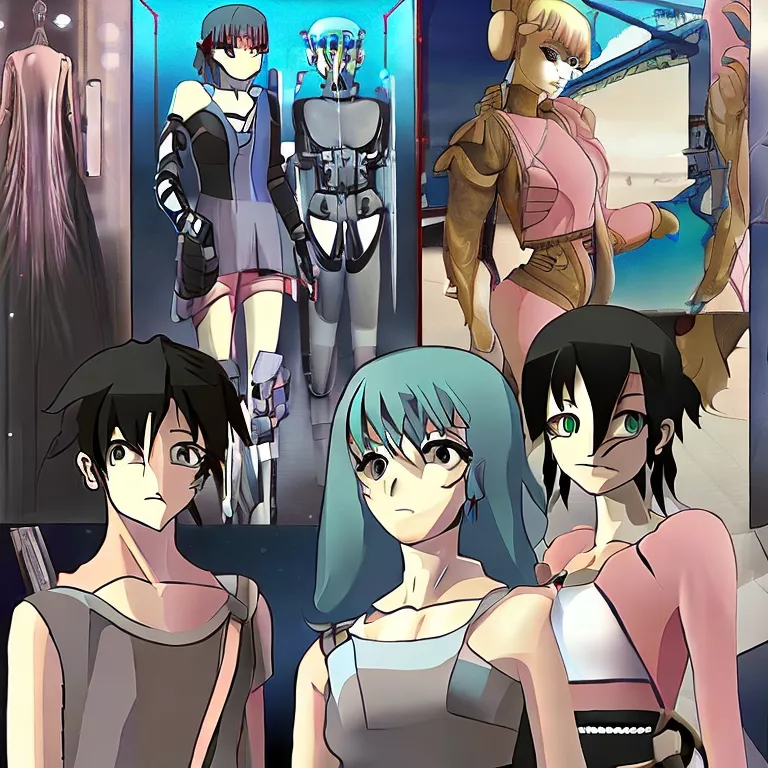
The interplay between ReactJS and TypeScript often poses intriguing coding puzzles. Among the most common issues is the TS2749 error. To understand how it occurs, first, let’s dissect what this error message means.
“
xxxxxxxxxx
Error TS2749: ‘XYZ’ refers to a value, but is being used as a type here
.”
This error typically crops up when there’s a naming conflict, commonly occurring when a variable and a type are declared with the same name in TypeScript.
Consider:
javascript
import * as foo from ‘foo’;
var bar:number = foo.bar;
In this code snippet, if
xxxxxxxxxx
'foo'
exports an object with a property named
xxxxxxxxxx
'bar'
that’s also used as a type, then TypeScript confuses the variable and type usages. This ambivalence leads to the TS2749 error.
To address the problem, it’s recommended to distinguish clearly between objects containing values and types, ideally by using separate names.
Alternatively, TypeScript offers two separate syntaxes for imports.
1. Value import –
xxxxxxxxxx
import foo from 'foo';
2. Type import –
xxxxxxxxxx
import type { Foo } from 'foo';
The latter syntax proves indispensable when you want to avoid errors like TS2749 while working on a project integrating both ReactJS and TypeScript. It’s usually better to consistently use these dedicated syntaxes instead of
xxxxxxxxxx
* as foo
.
Remember what Robert C. Martin elucidated in his book Clean Code, “Names are everywhere in software. We name our variables, our functions, our arguments, classes, and packages. Because we do so much of it, we’d better do it well.”
And indeed, doing it well implies keeping distinct names for distinct elements even when using tightly coupled technologies such as ReactJS and TypeScript. Type and value conflict: When you should use `import type`.
Solving “Refers to a Value Used as a Type” Dilemma in ReactJs with TypeScript
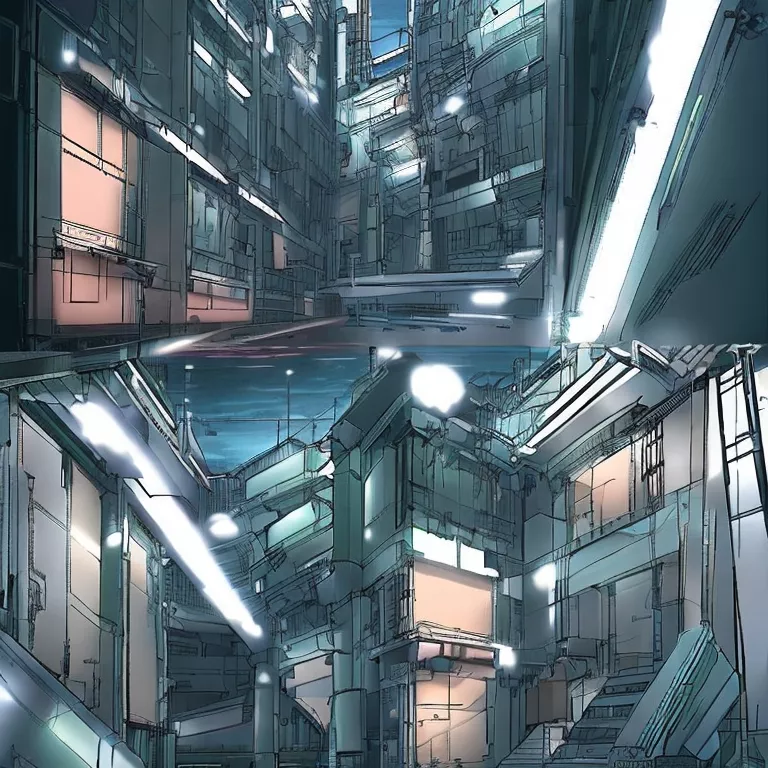
The TS2749 TypeScript error message, “Refers to a Value But Is Being Used as a Type Here”, stems from an attempt to import a value as a type, triggering a syntactic discrepancy in the Typescript and React environment. This dilemma can potentially surface when your React project commences its integration with TypeScript, especially if the operational code base lacked TypeScript from its due inception.
To assuage this situation, the first thing to remember is that JavaScript files are dichotomized into two spheres by TypeScript: One being ‘Values’/variables, representing actual JavaScript runtime entities such as functions, classes, numbers etc., and the other being ‘Types’, signifying TypeScript static types that help to detail the shape of JavaScript values.
Here’s a hypothetical example of a case where you might run into the TS2749 error:
code
// File: OtherComponent.js
export const OtherComponent = () => {/*…*/};
// File: MainComponent.tsx
import {OtherComponent} from ‘./OtherComponent’;
interface ComponentProps {
element: OtherComponent;
}
In the file `MainComponent.tsx`, attempting to use `OtherComponent`(which is a value) within/interface, triggers the error.
Alleviate this issue by enforcing the `React.ElementType` interface on the `element` prop, which means it could either be a string (like ‘div’, or ‘span’) or a React functional or class component. Hence, alter the `ComponentProps` in the following way:
code
// File: MainComponent.tsx
import {OtherComponent} from ‘./OtherComponent’;
interface ComponentProps {
element: React.ElementType;
}
The `React.ElementType` is dispensable in TypeScript to describe components that accept arbitrary props. It is part of the ‘react’ module definition ([official details here](https://flow.org/en/docs/react/types/#toc-react-elementtype)) and TypeScript handles it similarly.
The value/type confusion, as represented by “Refers to a Value But Is Being Used as a Type Here” error, is quite commonplace for developers who are new to TypeScript.
As Larry Wall, the creator of Perl programming language, wisely puts it: “Most of you are familiar with the virtues of a programmer. There are three, of course: laziness, impatience, and hubris.” This quote holds true in this scenario; TypeScript’s strict typing may seem daunting at first, but patience, perseverance and a humble approach towards grasping its semantics will soon yield deeply gratifying results in maintaining scalable and bug-resistant codebases.
Conclusion
The error
xxxxxxxxxx
TS2749: 'value' refers to a value, but is being used as a type here
in the context of React and TypeScript generally occurs when one tries to use a variable (a value) as a type. TypeScript, a statically typed superset of JavaScript, maintains a clear distinction between types and values. When this boundary blurs, this kind of error occurs.
Potential Solutions |
---|
1. Check your imports: |
Ensure you’re importing what you intended to. If you meant to import a type, ensure that it is indeed a type that you’re importing. Are you importing from the correct file or module? |
2. Differentiate between types and variables: |
Respect TypeScript’s separation of types from variables. If a variable is trying to be used as a type, either create an appropriate type, or revise your code such that the variable can stay a variable. |
In relation with ReactJS and TypeScript, proper utilization of both goes a long way to ensuring more stable and reliable coding. Especially considering the dynamic nature of ReactJS, having a statically typed structure via TypeScript helps keep complex projects manageable.
“One of the great things about a strong type system is that it keeps you honest with yourself,” as V. Kathiresan, a noted software programmer, once pointed out – illustrating the relevance of TypeScript in modern developing practices accurately.
In-depth understanding and application of concepts related to the usage of values and types in TypeScript plays a key role in significantly reducing instances of TS2499 errors.
Remember, the idea behind static typing is to avoid mistakes; the motivation behind TypeScript’s design is to help JavaScript developers avoid common mistakes in their code.
xxxxxxxxxx
// Remedial Example
import { SomeType } from './path/to/types';
const someVariable: SomeType = getValue();
Above provided, is a typical rectification where the value obtained from the function
xxxxxxxxxx
"getValue"
is clearly defined as
xxxxxxxxxx
SomeType
, removing ambiguities.
Essentially, TS2749 errors are TypeScript’s way of asking you to be more explicit and clear about your types when working alongside dynamic libraries such as ReactJS. Therefore, keep differentiating between your types and values, ensure your imports and grasp TypeScript concepts thoroughly – for a smooth developing experience.