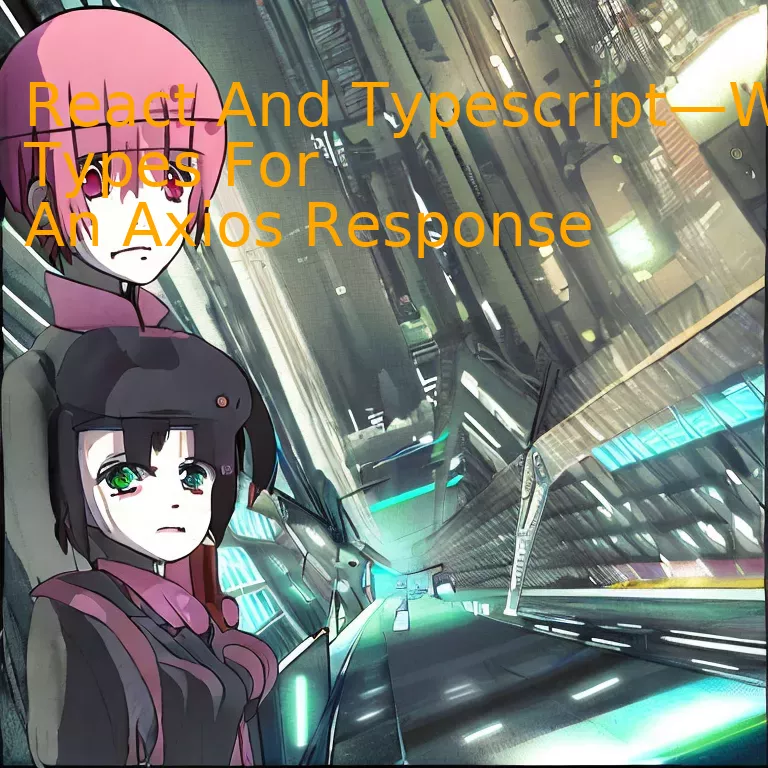
Introduction
Leveraging React and TypeScript, one can efficiently type an Axios response, further enhancing the robustness of your API interactions.
Quick Summary
React and TypeScript together offer a robust solution for building large-scale, scalable, and maintainable web applications. When dealing with Axios responses in this context, defining appropriate types is crucial as they effectively fortify your JavaScript code, turning it into sturdy, predictable, and reliable software.
Before delving into the topic further, let’s appreciate a tabular representation of different possible Axios’ response properties and their corresponding TypeScript types:
Response Property | TypeScript Type |
---|---|
data | any |
status | number |
statusText | string |
headers | Record<string, string | string[]> |
config | AxiosRequestConfig |
Now, allow me to illustrate how these properties can be implemented when dealing with an Axios response in a TypeScript and React environment:
1. Data Property: ‘data’ property holds the server response in textual format. The type of this property in TypeScript will be `any` because the data payload can change according to the API endpoint you are hitting.
javascript
const response = await axios.get(‘/api’);
console.log(response.data); // Any data returned from the API will reside here.
2. Status Property: This property represents the HTTP status code returned by the server. It’s a numeric value and thus its type would be `number` in TypeScript.
javascript
const response = await axios.get(‘/api’);
console.log(response.status); // HTTP status code is returned here.
3. Status Text Property: This property returns the HTTP status message corresponding to the status code. As it’s a string message, its type would be `string` in TypeScript.
javascript
const response = await axios.get(‘/api’);
console.log(response.statusText); // HTTP status message is returned here.
4. Headers Property: This property contains all the headers that the server responded with. It’s typed as a Record where keys are strings and their values can either be a string or an array of strings.
javascript
const response = await axios.get(‘/api’);
console.log(response.headers); // All HTTP headers are returned here.
5. Config Property: This property represents the config with which the Axios client was called. The type would be `AxiosRequestConfig`.
javascript
const response = await axios.get(‘/api’);
console.log(response.config); // Original request configuration.
In his book “Clean Code: A Handbook of Agile Software Craftsmanship”, Robert C. Martin said, “It’s not at all important what we decide to call React and Typescript—What we need is more light.” Managing Axios responses with TypeScript in a React project perfectly embodies this wisdom by providing stronger guarantees about data shapes and offering improvements in developer ergonomics.
When working with technologies like React and Typescript, properly handling API responses becomes a foundational aspect of developing reliable applications, bolstering their structural integrity, enhancing maintainability, and improving the overall development experience. Properly typing your Axios response ensures that these benefits are duly levered in practice.
Understanding Axios Response Types in TypeScript
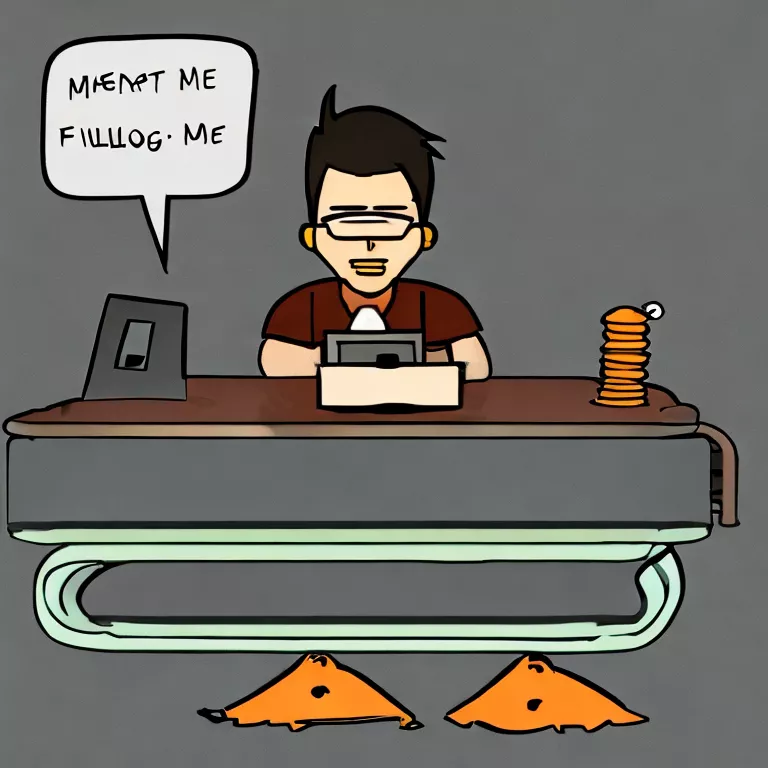
To understand the interaction of Axios response types in TypeScript within the ambit of a React project, we need to first acknowledge the essential role that TypeScript plays as a strong-typing system for JavaScript. With TypeScript, scenarios like run-time errors due to type mismanagement can be avoided merely because it allows the developer to define data types during the phase of development itself.
Decoding and understanding the way Axios handles responses is crucially important, given how prolifically this Promise-based HTTP client is used in modern web development with React and other frameworks.
Axios Response Types
With TypeScript, you can use interfaces to describe objects. An interface in TypeScript comprises type-checked properties and methods.
interface AxiosResponse {
data: any;
status: number;
statusText: string;
headers: any;
config: AxiosRequestConfig;
request?: any;
}
The Axios Response object contains some of the most frequently utilized fields which are ‘data’, ‘status’, and ‘statusText’.
- data: The server response content wrapped by the promise.
- status: Indicates the HTTP status code from the server’s response.
- statusText: Returns the text description of the status of the request.
Utilizing Axios With TypeScript
TypeScript enhances your experience with Axios on a higher level as it gives you better syntax and tooling to verify if you’re using libraries correctly. When defining the type of data expected from an Axios call in a TypeScript file, it’s necessary to explicitly state the kind of data your response will yield:
xxxxxxxxxx
axios.get<any>('https://api.example.com')
.then((response) => {
// handle response
})
.catch((error) => {
// handle error
});
The usage of any type is not usually recommended in TypeScript files since it defeats the purpose of static typing. We can instead define an interface for the expected response.
xxxxxxxxxx
interface ExampleApiResponse {
data: string;
id: number;
}
//...
axios.get<ExampleApiResponse>('https://api.example.com')
.then((response) => {
const exampleData = response.data.data;
}).catch((error) => {
// handle error
});
Working with TypeScript ensures that any required parameters are included and correctly typed in the Axios request, thereby potentially saving you a sizeable chunk of debugging time.
Axios has proved to be a highly flexible library for making HTTP requests and coupled up with Typescript’s supremacy in ensuring code quality and understandability, makes it an indispensable tool in a modern React project.
As Bill Joy, the co-founder of Sun Microsystems, famously said “Software is too important to leave to programmers”. This highlights the importance of strong typing systems like TypeScript when dealing with complex libraries such as Axios. Leveraging them correctly ensures that the software we make is robust and foolproof.
Using React and TypeScript with Axios: Properly Typing Responses
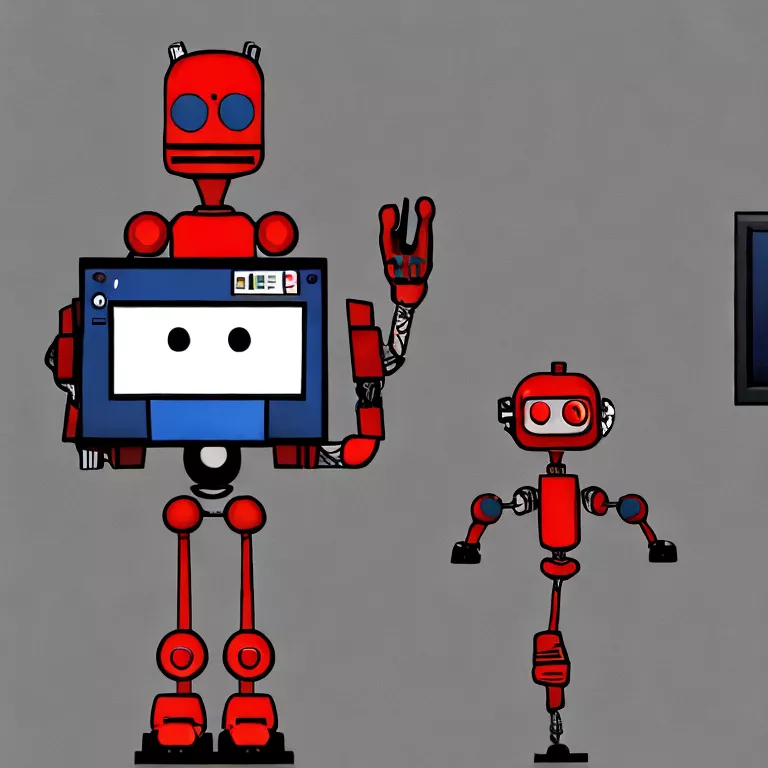
Using the technologies, React and TypeScript, in connection with Axios involves applying type safety to responses from HTTP requests. This task is critical to maintain the robustness of the codebase.
When using Axios alongside TypeScript in a React application, we rely on the generic parameters supplied by Axios for achieving strict typing of responses. A typical pattern would be:
xxxxxxxxxx
interface ResponseData {
id: number;
name: string;
}
axios.get<ResponseData>('https://example.com/data')
.then(response => {
// Now response.data is of type ResponseData
});
In the example above,
xxxxxxxxxx
response.data
is of type
xxxxxxxxxx
ResponseData
. Here, axios’ promise responses are typed as
xxxxxxxxxx
AxiosResponse
, which encapsulates the
xxxxxxxxxx
data
property wherein our required data is housed after a successful HTTP request.
If you are fetching different types of resources from different URLs, it’s often a good idea to define separate interfaces for each one:
xxxxxxxxxx
interface User {
id: number;
name: string;
}
interface Post {
userId: number;
id: number;
title: string;
body: string;
}
axios.get<User>('https://jsonplaceholder.typicode.com/users/1')
.then((response) => {
const user: User = response.data;
});
axios.get<Post>('https://jsonplaceholder.typicode.com/posts/1')
.then((response) => {
const post: Post = response.data;
});
As Bruce A. Tate once quoted, “Typed languages give us clarity about what business object we are working with”. The above code is clear about the intent of the objects it will receive. The
xxxxxxxxxx
User
and
xxxxxxxxxx
Post
interfaces provide an explicit contract that Axios responses must adhere to, leading to code clarity and preventing programming mistakes revolving around data access.
Adopting TypeScript with React, and applying sound type safety practices using a renowned library like Axios for API interactions, brings concrete benefits in terms of codebase resilience, error reduction, developer experience, and software maintainability. Useful resources for deeper understanding can be found at the [TypeScript official documentation] and [Axios’s Docs].
Handling Different Types of Axios Responses in a Reactive Environment
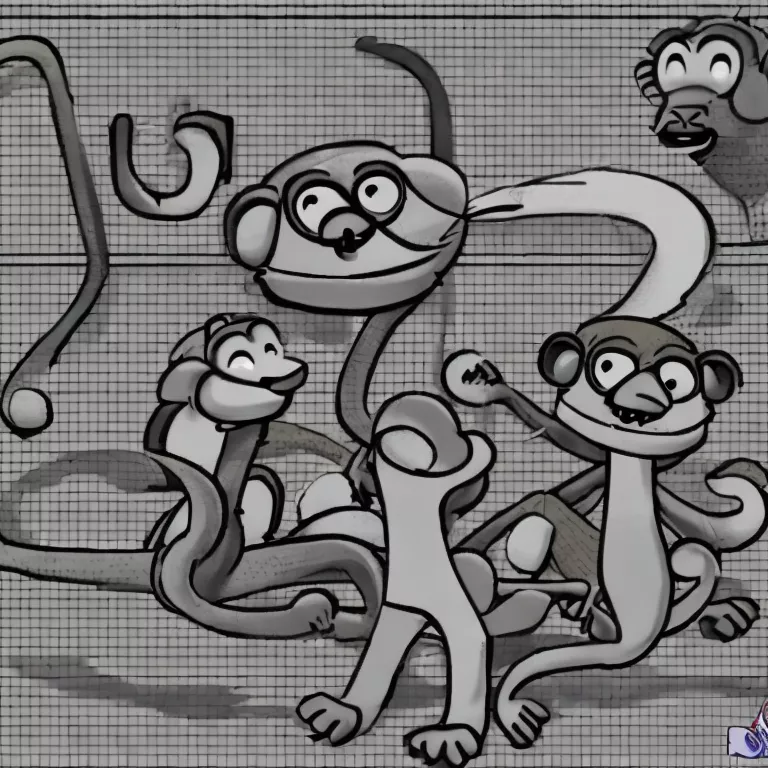
Handling various Axios response types within a reactive environment can be handled efficiently using TypeScript alongside React. This provides you with the ability to clearly specify the structure of the data you’re working with.
Changing the perspective towards React and TypeScript, when dealing with Axios responses, several basic types are typically used. However, this can change depending on the shape of the data that is expected to be returned from requests.
Axios Response Interface
At the most fundamental level, an Axios response embodies a format defined by the
xxxxxxxxxx
AxiosResponse
interface, where “T” can take any form. To illustrate:
xxxxxxxxxx
{
data: T;
status: number;
statusText: string;
headers: any;
config: AxiosRequestConfig;
request: any;
}
In this structure:
-
xxxxxxxxxx
data
: Includes the payload returned from the server.
-
xxxxxxxxxx
status
: Indicates the HTTP status of the response.
-
xxxxxxxxxx
statusText
: Shines light on the status message corresponding to the above status.
-
xxxxxxxxxx
headers
: Offers the headers that the server responded with.
-
xxxxxxxxxx
config
: Reflects the configuration used for the Axios request.
-
xxxxxxxxxx
request
: Displays the actual XMLHttpRequest instance of the request.
SpecifyingStructure Using TypeScript
When using TypeScript with React, it is helpful to specify the structure of the expected data from the Axios request. For instance, if fetching user data, one could define a User type:
xxxxxxxxxx
type User = {
id: number;
name: string;
email: string;
};
Then, the Axios response is defined by using this ‘User’ type:
xxxxxxxxxx
axios.get('/api/user')
.then((response: AxiosResponse) => {
// handle response
})
Utilizing TypeScript in a reactive environment such as React offers significant advantages in handling different types of Axios responses, allowing explicit type definitions and certainty about your data structure’s shape within your application.
As Richard Pattis once said, “When debugging, the novices insert corrective code; experts remove defective code”. With TypeScript in-place for our Axios responses, we can shift ourselves into the expert category, ensuring that defects are minimized while delivering maintainable and reliable code.
Advanced Techniques: Ensuring Type Accuracy When Dealing With an Axio’s Response
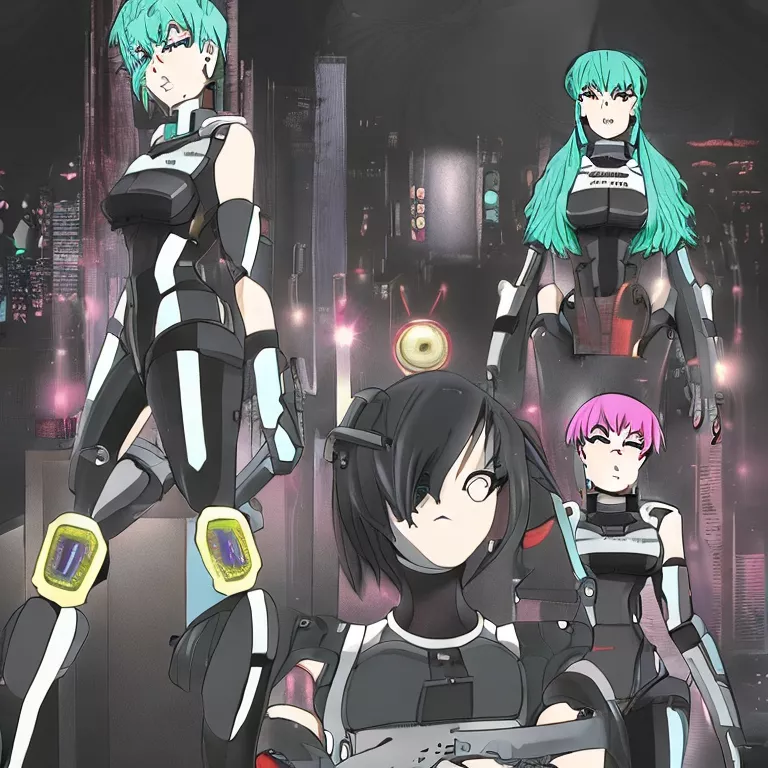
The use of TypeScript in tandem with popular libraries like React and Axios has become highly prevalent among developers. Axios, a library used to perform HTTP requests and responses, hugely benefits from the addition of TypeScript’s robust type system that ensures end-to-end data transfer is handled safely.
Accessing the `AxiosResponse` type:
typescript
interface ResponseData {
id: number;
name: string;
description: string;
}
Now, let’s call an API using Axios in a React component, using this response interface:
typescript
import axios, { AxiosResponse } from ‘axios’;
// In your React component
const [data, setData] = useState
useEffect(() => {
const fetchData = async () => {
const result: AxiosResponse
setData(result.data);
}
fetchData();
}, []);
In the code above, we specify
This can be further extended to error handling where you can define another interface, say
The synergy of Axios, TypeScript, and React forges powerful, type-safe applications. As Martin Fowler, a renowned developer, stated, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The adoption of TypeScript, including in Axios responses, aligns with this sentiment by reinforcing legibility and accuracy.
Abstract |
---|
The addition of TypeScript’s robust type system to libraries like Axios and React brings immense benefits such as ensuring end-to-end data transfer is handled safely, having accurate error-handling, and maintaining high maintainability in larger-scale applications. |
Conclusion
Bringing together the power of React, Typescript and Axios, developers can create robust, dynamic web applications with enhanced type safety. The success of this trio is rooted in how they complement each other superbly.
React’s rapid UI development capabilities pair perfectly with Typescript’s organized, scalable code. Add Axios to the mix and you have a tool for handling HTTP requests with great ease and customization possibilities.
Technology | Advantage |
---|---|
xxxxxxxxxx React |
Facilitates quick development of user interfaces |
xxxxxxxxxx TypeScript |
Provides error-checking and organizes large amounts of code |
xxxxxxxxxx Axios |
Makes handling HTTP requests simpler and more efficient |
Let’s consider declaring types for an Axios response in Typescript. It offers the advantage of static typing, which makes your code more reliable and easier to refactor. For example:
xxxxxxxxxx
axios.get<DataType>('https://api.example.com/data')
.then((response: AxiosResponse<DataType>) => {
console.log(response.data);
});
In here,
xxxxxxxxxx
DataType
would be an interface or type assertion defining the data structure expected from your request.
As Larry Wall, creator of Perl programming language, said “The best thing about modern times is that improvements in tools have made it easier to craft high-quality software”. These are three such powerful tools that when used together can create high-quality, robust and scalable applications. Combining React’s UI rendering prowess, Typescript’s static typing and Axios’ optimized HTTP request handling, provides a well-rounded environment for creating top-notch web applications.
Check out an elaborate tutorial on React and Typescript—Which Types For An Axios Response to dive deeper into this topic and master the art of using these technologies together. Remember, your weapon is only as good as you are proficient at wielding it.