Introduction
To ensure optimal efficiency while utilizing Next.Js Getstaticprops with Typescript, observe that careful configuration of your types in the function parameters contributes to seamless and search-engine-friendly operation.
Quick Summary
Below is a comprehensive guide providing insights into how you can make `getStaticProps` in Next.js work cohesively with TypeScript:
Process | Action |
---|---|
1. Create a new Next.Js project with Typescript | Create a brand new Next.js app and setup TypeScript by running
x create-next-app . --typescript |
2. Define the data type | In TypeScript, define a new interface or type for the data that will be returned from your API call or static file read. |
3. Use getStaticProps | Inside the exported function of your Next.js page, include the
xxxxxxxxxx getStaticProps method, which Next.js will call at build time on the server-side. |
4. Return the props object | After fetching your data, return an object from
xxxxxxxxxx getStaticProps that includes a property called ‘props’ which is an object containing your typed data. |
Looking closely at these steps, the first process is to set up Next.js with TypeScript, accomplished by employing the command `create-next-app . –typescript`. This creates a new Next.js project locally and initializes TypeScript.
The second step focuses on the essence of TypeScript – defining types. In this situation, it is essential to develop a new Type or Interface, essentially blueprinting the data that will result from the API call or static file read. This provides clarity over the structure of the data you’re maneuvering and promotes efficient error handling.
Incorporating `getStaticProps` method into your code represents the third step. This function is exclusive to Next.js and is executed at build time on the server-side. Particularly with TypeScript, it serves to fetch and validate static data according to predefined types.
Lastly, after successfully retrieving your data in the ‘getStaticProps’ method, you are required to return an object that contains a props property comprised of fetched typed-data. This object becomes accessible within your component as a prop. Therefore, the components can efficiently manipulate the statically generated data based on pre-defined types.
In a quote by Addy Osmani, a Google engineer: “Code has a cost. More lines of code often means more to maintain, more bugs and more time spent paying down technical debt.”. However, this process elegantly reduces the risk of bugs and enhances readability, thus promoting well-structured, accurate, and maintainable code. As a TypeScript developer using Next.js, executing `getStaticProps` alongside TypeScript is an optimal strategy ensuring the delivery of high-quality web applications.
Leveraging Next.Js GetStaticProps in TypeScript: A Detailed Approach
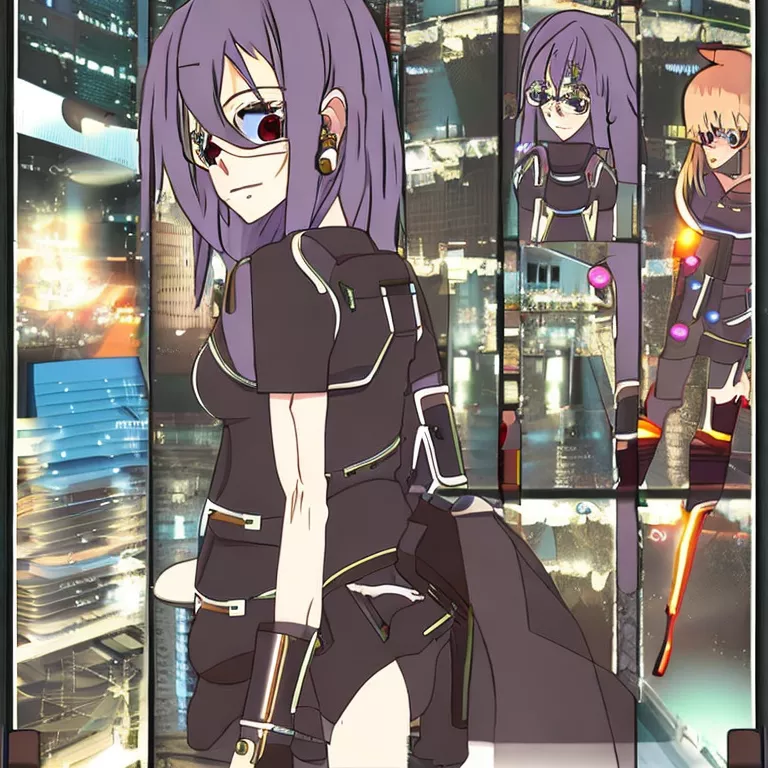
Undeniably, the union of Next.js with TypeScript equips developers with a high degree of predictability, scalability, and robustness. More so if you tap into the power of GetStaticProps—a data fetching method provided by Next.js. Here’s an analytical understanding of how to make GetStaticProps work with TypeScript.
To apply TypeScript to Next.js’ `getStaticProps` function, type interfaces could be defined. An interface in TypeScript serves as a way to define complex shapes of dynamic data types or functions, such as the props for a Next.js page.
typescript
import { GetStaticProps } from ‘next’;
interface HomePageProps {
content: string;
}
export const getStaticProps: GetStaticProps = async () => {
return {
props: {
content: “Your static content here”
}
}
}
const HomePage: React.FC = (props) => {
return (
)
}
export default HomePage;
In this example, we leverage TypeScript to predictably type our `getStaticProps` function. It helps secure that boilerplate fetching operation and ensures we meet data expectations else our code fails at compile-time rather than runtime.
`GetStaticProps` is a generic function from the `next` package which expects to receive the structure of the props that are passed to the component. By feeding it our custom interface — `HomePageProps` — it guarantees typing uniformity all through usage.
That said, consider the scenario where fetched data structure is intricate and mutable— like entries from a CMS or files from a directory. This situation demands an inherently unique approach which can validate the shapes against their correctness.
As Martin Fowler once counsely advised, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” And indeed, by leveraging the TypeScript union types or optional types features, we can anticipate such variance and still maintain understandable, scalable code.
Taking this a step further, JSON Schema is a particularly powerful tool that you might leverage for validating data fetched in `getStaticProps`. With packages like ajv – Another JSON Schema Validator, you can validate your received props against your schema. This strategy could serve not just as a dynamic typing mechanism, but also as a first layer of anti-corruption incentivizing data integrity and security.
Look at Next.js and TypeScript as friends whose relationship makes your data fetching logic easier to maintain, read, adapt and scale. In the journey of effective development experience, their collaboration undoubtedly serves as an efficient catalyst in nailing application’s predictability, reusability, and robustness.
Understanding the Key Concepts of GetStaticProps with TypeScript
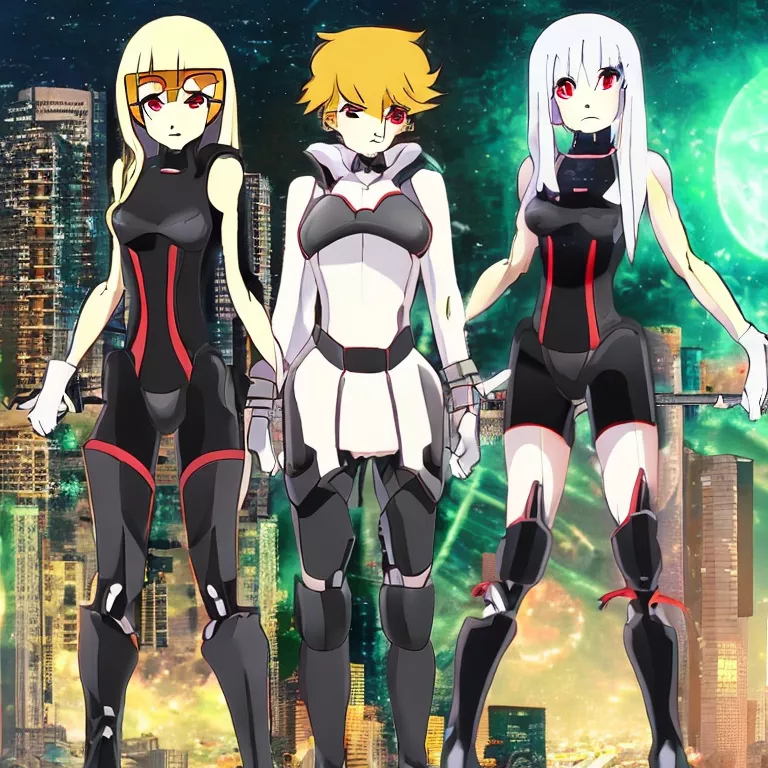
When leveraging getStaticProps feature in Next.js with TypeScript, there are key concepts and techniques that are crucial to comprehend.
TypeScript and getStaticProps
TypeScript provides static typing for JavaScript, which bolsters code quality and understandability while offering a seamless debugging experience. When used in tandem with the Next.js `getStaticProps` function, dynamic data can be fetched at build time, thereby facilitating statically generated pages even when dealing with varying items of data.
The role of
xxxxxxxxxx
getStaticProps
is fetching data during static generation and returning it as props. Given its importance, we will delve into the steps involved when implementing this method using TypeScript:
Exporting GetStaticProps
The `
xxxxxxxxxx
` TypeScript type from Next.js could be exported from your page component file, and it's meant to provide typings for `getStaticProps`. Thus, engendering stricter type checking and autocorrect features offered by most IDEs.
typescript
import {GetStaticProps} from ‘next’;
export const getStaticProps: GetStaticProps = async () => {
…
}
xxxxxxxxxx
GetStaticProps
Defining Typed Props
The implementation conundrum of
xxxxxxxxxx
getStaticProps
using Typescript generally arises while typing these props. Here is a succinct way to accomplish that.
Define an interface for your props at the top of your component file:
typescript
interface PageComponentProps {
property1: number;
property2: string;
…
}
Subsequently, the>
xxxxxxxxxx
GetStaticProps
should take a single type parameter which describes the returned props object:
typescript
export const getStaticProps: GetStaticProps = async () => {
const data = await fetchAPI();
return {
props: { …data },
};
};
Injecting Props to Page Component
On acquiring the expected props, make sure to inject them into your page component.
typescript
const PageComponent: NextPage = ({ property1, property2 }) => {
// use propertis here
}
“Good code is its own best documentation,” remarks Steve McConnell, author of Code Complete, underscoring the value of legibility and clear logic inherent in quality programming. Every aspect of this execution––typed props, the injection of these into page components, and the definition and exportation of
xxxxxxxxxx
getStaticProps
, resonates well with McConnell’s sentiment. With TypeScript added to the equation, coding in Next.js becomes an efficient and seamless endeavor where errors are caught early, while ensuring robustness and maintainability of applications.
Utilizing Typescript for Optimal Use of Next.Js’s GetStaticProps Functionality
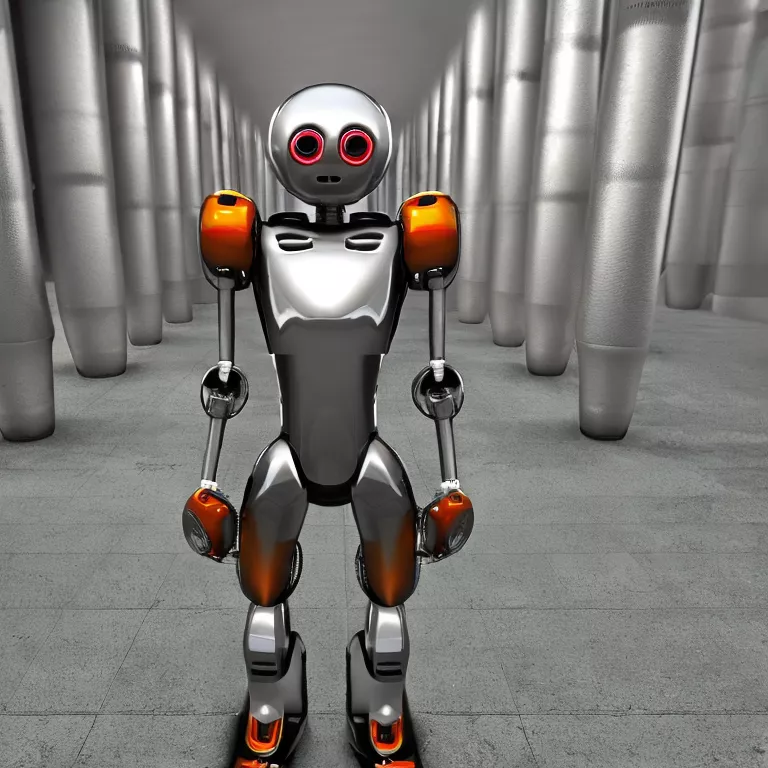
The Next.js framework’s `getStaticProps` function paired with TypeScript offers a robust platform for building highly optimized and statically generated pages. The use of TypeScript helps to assure type safety, enhancing the developer’s experience by catching errors early in development and helping to write clearer, more understandable code.
Use of getStaticProps with TypeScript
In a basic implementation within a Next.js application, the `getStaticProps` function leverages TypeScript’s static typing feature to fetch data at build time, generating static HTML which works perfectly even without JavaScript. This quality contributes greatly to loading speed and SEO ranking as search engine crawlers can better index your site’s content.
A simplified example of the `getStaticProps` usage:
xxxxxxxxxx
type Props = {
posts: Array
}
export const getStaticProps: GetStaticProps<Props> = async () => {
const res = await fetch('https://.../posts')
const posts: Post[] = await res.json()
return {
props: {
posts,
},
}
}
Note that the
xxxxxxxxxx
GetStaticProps
generic from Next.js is used, taking our defined type (Props). This allows us to explicitly define what props our page will receive, thereby leveraging TypeScript’s benefits.
The `getStaticProps` function is executed at build time on server-side. It will not be included in the JS bundle for the browser. That means you can directly include any secret keys or passwords in your `getStaticProps` function without them being exposed to the world.
The Various Forms of Pre-rendering in Next Js
There are two forms of pre-rendering: Static Generation and Server-side Rendering. The difference is when it generates the HTML for a page since each has its own merits:
- Static Generation is the pre-rendering method that generates the HTML at build time. The pre-rendered HTML is then reused on each request. This where `getStaticProps` comes in.
- Server-side Rendering is the pre-rendering method that generates the HTML on each request
Borrowing a quote by Kyle Simpson, renowned JavaScript expert and author of You Don’t Know JS, “Code is not just meant to be executed. Code is also a means of communication across a team, a way to describe to others the solution to a problem.” Applying this principle when building our Next.js applications with TypeScript, we would certainly boost both our developer experience as well as our website performance.
For more in-depth guidance, refer to the official Next.js documentation on Data Fetching.
Best Practices and Common Pitfalls When Implementing GetStaticProps Using TypeScript
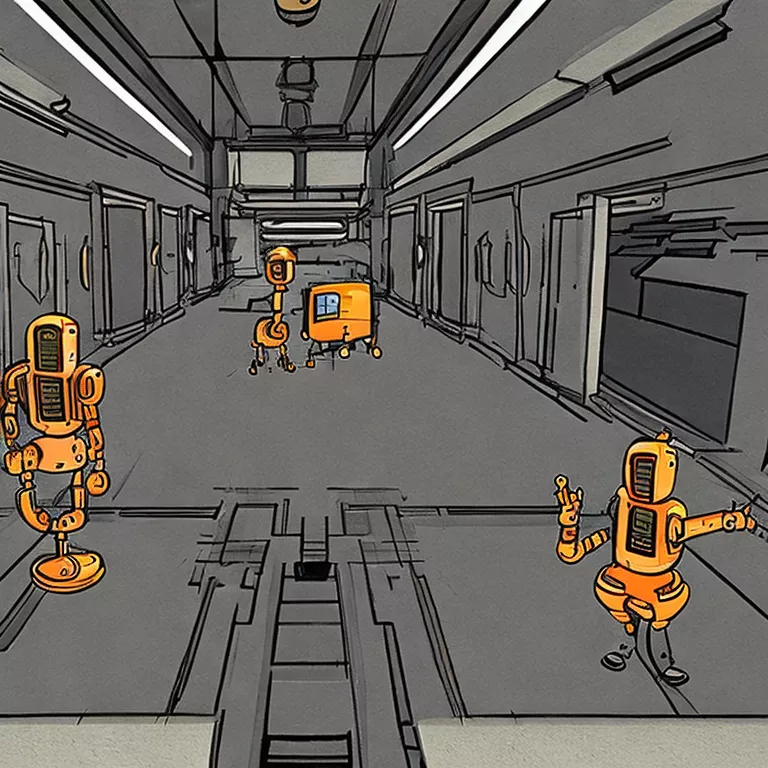
Implementing
xxxxxxxxxx
getStaticProps
in your Next.js project, using TypeScript, can greatly enhance the static generation of your application’s pages. However, it’s essential to follow best practices and be aware of common pitfalls to harness its full potential.
Defining Prop Types with TypeScript
Define types for the props you’ll be returning from
xxxxxxxxxx
getStaticProps
. TypeScript allows us to specify clear expectations about what data can and cannot be passed as props, providing a better understanding for ourselves and team members, and minimizing possible bugs.
xxxxxxxxxx
type HomeProps = {
posts: Array<{
id: string;
title: string;
}>;
};
export async function getStaticProps(): Promise<{ props: HomeProps }> { }
With this structure, props returned now adhere to typed parameters, ensuring greater code reliability.
Always Return an Object with Properties
An easy pitfall to avoid is trying to return anything other than an object within
xxxxxxxxxx
getStaticProps
. It must always return an object containing a ‘props’ property.
xxxxxxxxxx
return { props: { } }; // Correct
return undefined; // Incorrect
This ensures data gets correctly passed to your component, maintaining application stability.
Fetch Data at Build Time
One compelling feature of
xxxxxxxxxx
getStaticProps
is pre-rendering pages at build time with data fetched then. However, it’s essential not to mistake it for data-fetching at request time.
Avoid calling APIs or databases that update frequently inside
xxxxxxxxxx
getStaticProps
, as it would result in stale content until the next build process runs.
Error Handling
Just like any data-fetching operation, your code within
xxxxxxxxxx
getStaticProps
might throw errors. Always guard your data-fetching operations with appropriate try/catch blocks.
xxxxxxxxxx
try {
const response = await fetch('https://api.example.com/posts');
const posts = await response.json();
return { props: { posts } };
} catch (error) {
return { error };
}
Handling possible errors offers a more robust application and a better user experience.
James Gosling, the creator of Java, once said, “Security is not a feature, it’s a way of life”. So, the same goes for error handling in any coding practice, including TypeScript.
Testing GetStaticProps
When unit testing components that depend on
xxxxxxxxxx
getStaticProps
, supply mock data to mimic what getStaticProps would provide during actual runtime. Not doing this can lead to unexpected results because your component expects specific props to be present.
Implementing the above best practices should help you make the most out of Next.js features using TypeScript, ensuring smoother data-fetching operations, increased productivity, and better code maintainability.
For further details consider checking out NextJs official documentation on TypeScript usage of getStaticProps.
Conclusion
Digging deeper into the world of Next.JS and TypeScript reveals a fascinating interplay where
xxxxxxxxxx
getStaticProps
comes alive in multilayered applications. This feature offers an incredibly strategic and effective advantage to server-side rendering with Next.js).
Employing
xxxxxxxxxx
getStaticProps
with TypeScript involves certain thoughtful steps. First off, it is highly advisable to define an interface for the props that forms the basis of your function. A carefully established TypeScript ambient declaration file (.d.ts) becomes beneficial here. For instance:
xxxxxxxxxx
interface AppProps {
posts: {
id: number;
title: string;
}[]
}
Later on, this declared interface can be used in your
xxxxxxxxxx
getStaticProps
method:
xxxxxxxxxx
export async function getStaticProps(): Promise<{props: AppProps}> {
const res = await fetch('https://.../posts')
const posts: AppProps['posts'] = await res.json()
return {
props: {
posts,
},
}
}
This way, we successfully establish a high level of reliability and predictability within our codebase. As a result, by implementing TypeScript with Next.js’s
xxxxxxxxxx
getStaticProps
, the project gains both compile-time type safety and enhanced autocompletion functionality.
As Aaron Frost, a leading figure in the tech industry, put it, “TypeScript brings a level of agility to JavaScript development, providing advanced autocompletion and type safety”. This sentiment rings particularly true when applied in the context of using
xxxxxxxxxx
getStaticProps
in Next.js.
Lastly, the beauty of Next.js lies in its seamless compatibility with other tools, TypeScript included. These capabilities allow developers to build polished, high-performance applications.
From these points, it’s apparent that employing Next.js
xxxxxxxxxx
getStaticProps
with TypeScript is quite a game-changer. It isn’t just about a one-time solution to a problem, but a sustainable, long-term strategy for improving web application performance rooted in technology best practices.source.