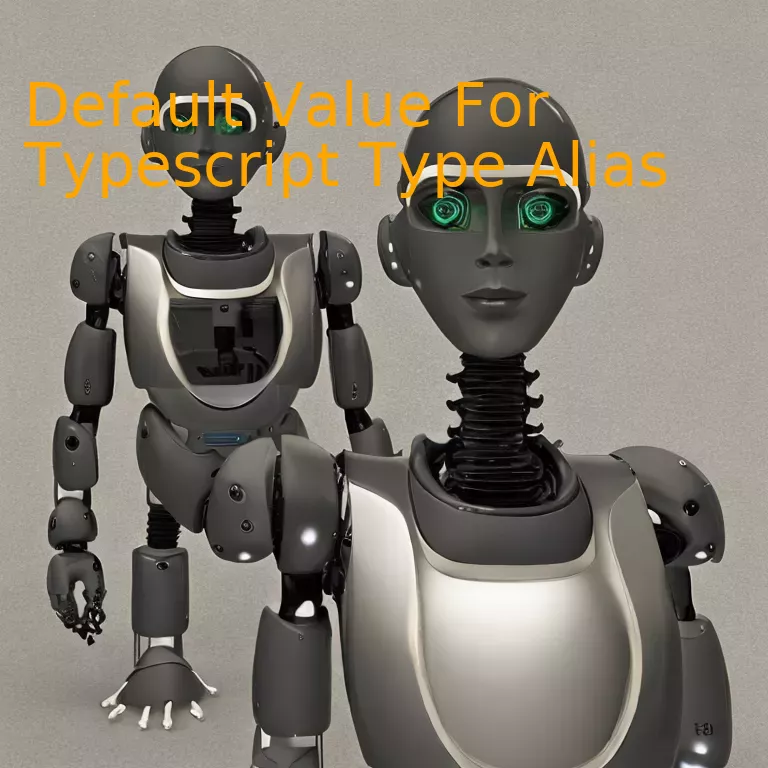
Introduction
Seamlessly integrating defaults into TypeScript Type Alias enhances programming efficiency by pre-setting values, minimizing potential for coding errors and streamlining web development processes.
Quick Summary
According to TypeScript’s type system, the concept of a default value for a type alias does not explicitly exist. Instead, developers define types that determine the values a variable may possess and assign default values during the variable’s initialization phase.
Before we delve deeper into this concept, consider reaching a better understanding through a structured informational representation:
Type Alias | Description | Default Value (Initialization) |
---|---|---|
x type StringAlias = string; |
An Alias for a primitive type ‘string’ |
xxxxxxxxxx let variable: StringAlias = ''; |
xxxxxxxxxx type NumberAlias = number; |
An Alias for a primitive type ‘number’ |
xxxxxxxxxx let variable: NumberAlias = 0; |
xxxxxxxxxx type BooleanAlias = boolean; |
An Alias for a primitive type ‘boolean’ |
xxxxxxxxxx let variable: BooleanAlias = false; |
TypeScript Type Aliases are simply names for any type, ranging from simple primitive types such as `string`, `boolean`, or `number`, to complex structurally defined types. You might ask, can you specify a default value when defining a type alias in TypeScript? The response is direct: No, you cannot directly specify a default value when defining a type alias.
However, when initializing a variable with a specific type (or type alias), it is perfectly acceptable – and often recommended – to assign a ‘default’ value. Although these aren’t “default values” in the traditional sense (e.g., as you might see in a function parameter), they do provide an initial value for those variables.
This practice does not only apply to TypeScript, but is also a common programming idiom used in many other languages (including JavaScript) to avoid issues such as `undefined` or `null` reference errors.
As Robert C. Martin rightly quoted, “Clean code is not written by following a set of rules. You don’t become a software craftsman by learning a list of heuristics. Professionalism and craftsmanship come from values that drive disciplines.”
Harnessing the Power of TypeScript Type Alias Defaults
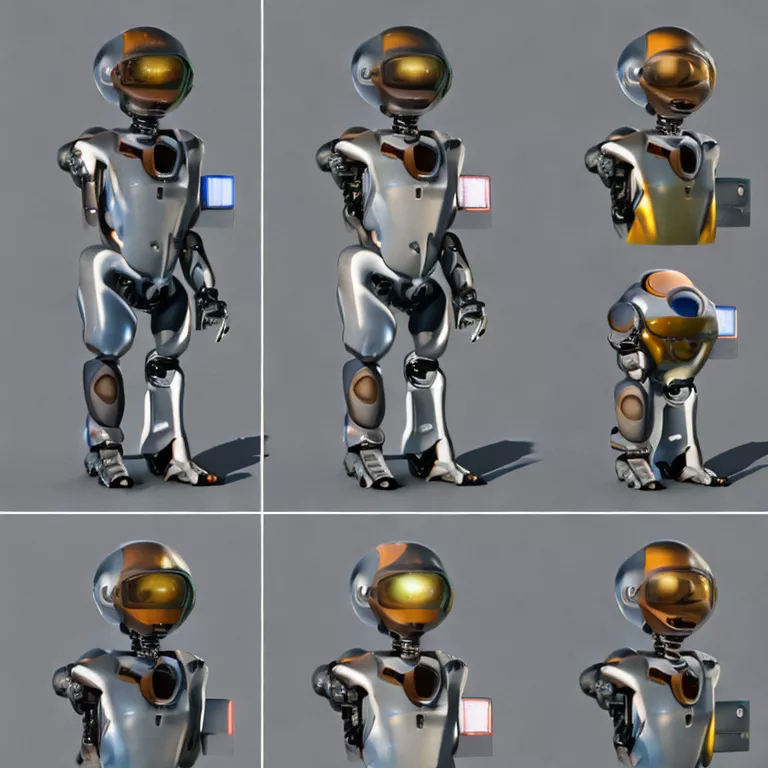
Leveraging the power of TypeScript Type Alias Defaults can significantly aid in streamlining coding practices and enhancing software development efficiency. When it comes to setting a default value for a TypeScript Type Alias, unfortunately, TypeScript doesn’t directly support this feature in its arsenal. However, practical solutions can be implemented to replicate similar behavior.
One effective approach is using Union Types in conjunction with Type Aliases. This methodology allows a variable to adopt a specific type from a set of predefined types, providing an implicit ‘default’ behavior.
Let’s look at an example:
xxxxxxxxxx
type AliasType = 'Option1' | 'Option2' | undefined;
function demoFunction(value: AliasType = 'Option1') {
return value;
}
In this code snippet,
xxxxxxxxxx
AliasType
is a type alias which can have either of the values –
xxxxxxxxxx
'Option1'
,
xxxxxxxxxx
'Option2'
, or
xxxxxxxxxx
undefined
. The function
xxxxxxxxxx
demoFunction
has an argument
xxxxxxxxxx
value
of this type alias with a default value of
xxxxxxxxxx
'Option1'
. So, if no argument is passed while calling this function,
xxxxxxxxxx
'Option1'
will be considered as the default value.
Note that the default value applied in the function parameters isn’t intrinsic to the type alias itself but offers a mechanism to leverage default behavior where needed.
It’s critically important to understand that each tool, language, or framework comes with a unique set of features, constraints, and best use-cases. TypeScript is no exception. Although Type Aliases in TypeScript do not have built-in support for providing a default value, intelligent usage of its robust characteristics like Union Types and type inference can mitigate these limitations.
As Steve Jobs once said, “Technology is nothing. What’s important is that you have a faith in people, that they’re basically good and smart, and if you give them tools, they’ll do wonderful things with them.” TypeScript is just one of those advanced tools, empowering developers to craft seamless, reliable and maintainable JavaScript applications.
Understanding Values in TypeScript Type Aliases
In TypeScript, a type alias is a way to refer to a type using a different name. Type aliases work with any kind of type: object types, intersections and unions, primitives, tuples, and any other types you define.
A significant aspect to discuss here is understanding the concept of values in TypeScript’s type aliases, and harnessing the power of default values in these cases. The key issue revolves around creating a TypeScript type alias that not only reduces redundancy but also ensures a default value. Here’s how it can be achieved:
In some scenarios, you may want to have a specific default or fallback type if no type is provided when derivating from a type alias. However, TypeScript does not natively support default values inside their type system.
Consider this code snippet:
xxxxxxxxxx
type AliasType = {
key: string;
value: string;
};
We have defined a type alias ‘AliasType’ for an object having properties ‘key’ and ‘value’, both being of type string. There isn’t a direct method to set a default value within the definition.
However, we can achieve a similar effect by utilizing the concept of Function Default Parameters in TypeScript. It means defining a function parameter with a default value.
Here’s a basic example:
xxxxxxxxxx
function createObject(key: string = 'defaultKey', value: string = 'defaultValue'): AliasType {
return {
key,
value
}
}
The `createObject` function returns an object adhering to the ‘AliasType’. If no parameters are passed while calling, they take up their respective default values.
Indeed, the TypeScript’s type system is designed to represent the shapes that JavaScript objects can take. Within that goal, “the concept of default values” is somewhat outside its domain. Having said that, utilizing such concepts – like shown above – ‘Function Default Parameters’, can certainly provide a way to set default values indirectly.
As Bill Gates expressed, “The function of good software is to make the complex appear to be simple.” In TypeScript, this concept becomes evident as we learn to handle complex scenarios with such simplicity and elegance.
For more in-depth details related to TypeScript’s type alias, refer to the official TypeScript Documentation.
TypeScript: Navigating Default and Optional Types
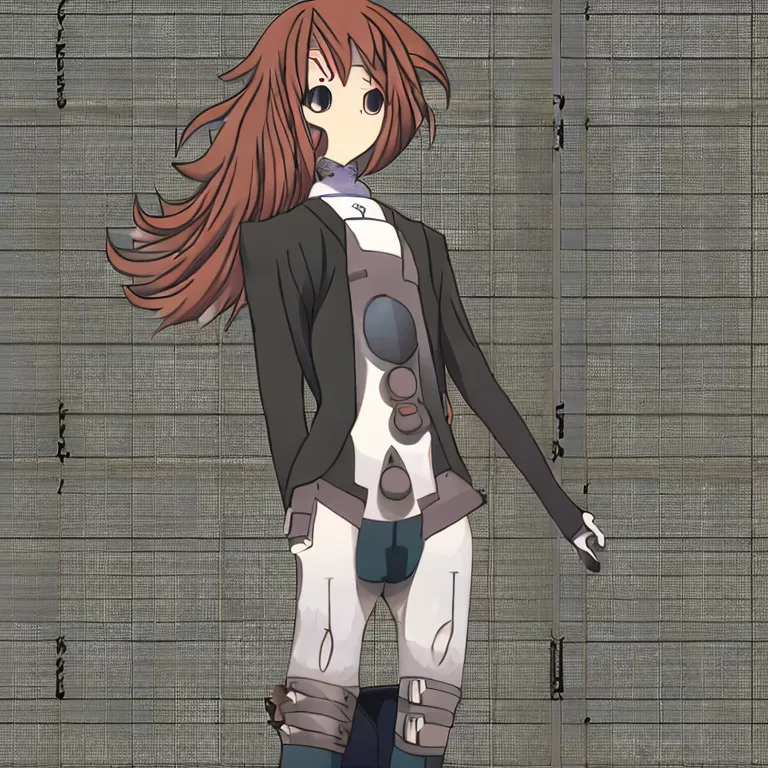
TypeScript, a super-set of JavaScript, introduces static typing, enabling developers to write more readable and robust code. Ensuring type safety makes the overall software development lifecycle much faster and less error-prone. Part and parcel of this are optional and default types which permit the assignment of default values and the avoidance of unnecessary complexity in defining object structures.
Talking about TypeScript’s default value for “type alias”, one should note that TypeScript does not inherently provide “default” values for “type aliases”. However, we have the luxury to manipulate function parameters to provide a sort of makeshift default value equivalent.
xxxxxxxxxx
type MyCustomType = {
name: string,
age: number,
}
function greet({ name, age }: MyCustomType = { name: 'John Doe', age: 30 }) {
return `Hello ${name}, you are ${age} years old.`;
}
In the preceding example, an object of “MyCustomType” is expected as the parameter for the “greet” function. If no arguments are provided, it will default to the provided default object.
TypeScript also provides us with the option (pun intended) to execute with optional types; this enables assigning undefined to properties that might not always be available. This takes advantage of TypeScript’s SomeType | undefined feature. An optional type can be defined by adding a question mark (?) after the property name.
xxxxxxxxxx
type OptionalType = {
requiredProp: number;
optionalProp?: string;
}
Here “requiredProp” is mandatory while “optionalProp” becomes an optional property and can be overlooked when creating an object of “OptionalType”.
Circling back to type alias defaults, although TypeScript does not directly allow defaults with type aliases, leveraging optional types alongside default parameter values ensures a similar level of code readability and stability.
As Yukihiro Matsumoto, the creator of Ruby, once insightfully put it, “I hope to see Ruby help every programmer in the world to be productive, and to enjoy programming, and to be happy. That is the primary purpose of Ruby language.” His words resonate with our discussion; TypeScript exists not to limit JavaScript programmers but to provide them with more structured, robust, readable, predictable, and hence happier software development experiences.
The interplay of default values in function parameters and optional types can aid many use cases in everyday development with TypeScript, enhancing code readability while keeping it DRY (Don’t Repeat Yourself) and bug-free.
Concrete Use Cases for Default Value in TypeScript Type Alias
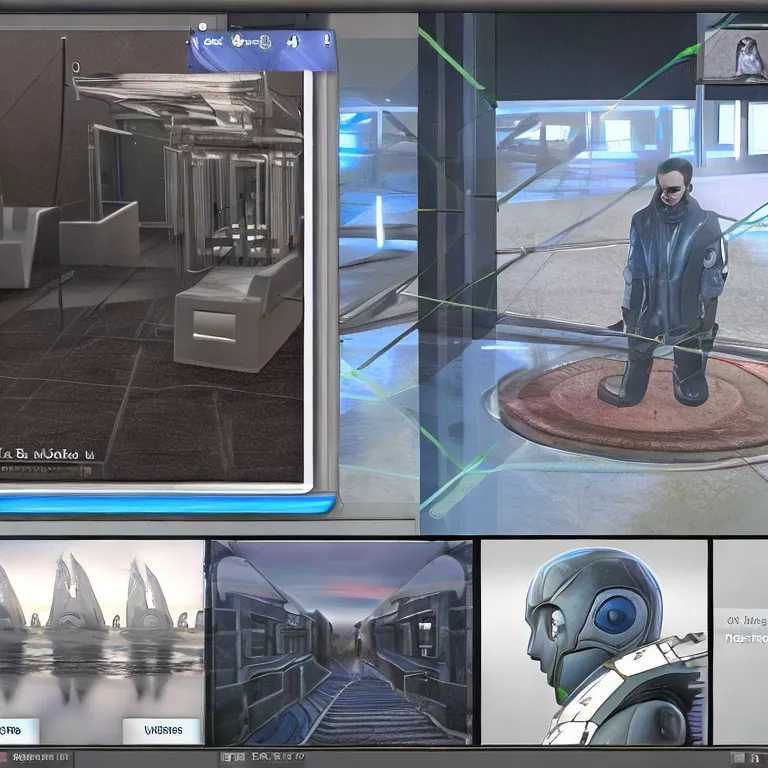
TypeScript is known for its strong typing system which enhances JavaScript’s flexibility and productivity. One exciting feature that TypeScript offers is the ability to assign default values to Type Aliases. Before diving into concrete use cases, let’s quickly understand what a Type Alias in TypeScript is.
A Type Alias allows you to create a new name for an existing type. We can then use this alias throughout our code wherever we want to use the existing type, enhancing code readability and maintainability.
xxxxxxxxxx
type Point = {
x: number,
y: number
}
Here, ‘Point’ is the type alias for our existing object type consisting of properties x and y. However, TypeScript doesn’t inherently support assigning default values to Type Alias.
But with ES6 destructuring assignment in TypeScript, we can couple your Type Aliases with function parameters to assign default values. This approach can be instrumental in specified use cases:
1. Optional Object Properties
In scenarios where some properties are optional but should take on standard values when not provided, default value assignments become advantageous. Let’s come up with a simple function `draw()` which uses our ‘Point’ type:
xxxxxxxxxx
function draw({x = 0, y = 0}: Point) {
// Drawing logic here using x and y
}
Without providing x or y, ‘draw’ function employs default zero values preventing possible undefines.
2. Simplifying APIs
By integrating default values, we can make user-facing APIs more straightforward by reducing mandatory parameters for functions. Consider an API route that fetches user data:
xxxxxxxxxx
type UserParams = {
userId?: string;
};
function getUserData({userId = 'defaultUser'}: UserParams){
// Fetching logic with userId
}
This method will continue working even if no userId is passed, catering to users who want a default behavior.
In TypeScript functional parameters can be deemed optional, and this with defaults form a formidable duo to simplify your code and handle edge cases wisely. John Papa, an American developer known for his work in the Angular community says, “Code readability improves when you lift the essential parts of the narration.”. Leveraging TypeScript’s type aliases with default values definitely echoes that quote.
Conclusion
With TypeScript’s advanced static typing features, it becomes possible to create more reliable and maintainable code. The Type Alias enables developers to declare a name for any kind of type whatsoever. This precludes the need to repeatedly write out complex object structures or union types, therefore ensuring readability and manageability within extensive codebases.
A significant advantage of using TypeScript Type Alias comes in the possibility of assigning a default value. In JavaScript, you might have assigned a default value for function parameters to handle instances where an argument is not passed during a function call. TypeScript takes this further and extends the capability to include Type Alias.
Let’s check a small example:
xxxxxxxxxx
type Person = {
name: string;
age: number;
address?: string;
};
// Here we provide default values when initialising a new object.
const johnDoe: TPerson = {
name: "John Doe",
age: 30,
address: "200 Main St.",
};
In this example, providing a default value for TPerson has several advantages:
– It aids in making your TypeScript code self-documenting.
– It prevents potential runtime errors from unexpected undefined values.
– It provides ease of use for functions that consume these objects. Functions can now operate with some assurance about the structure and expected data types.
Assigning default values to a TypeScript Type Alias significantly streamlines code development process. However, it must be noted that Type Aliases are statically analyzed and do not exist during runtime. Thus, providing a default value at the time of Type Alias creation doesn’t directly affect the runtime operation rather it acts as a guideline for future coding activities.
To comprehend TypeScript Type Alias better, exploring resources such as the [official Typescript documentation] can prove to be beneficial.
As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” In light of this quote, TypeScript’s Type Alias proves to be an indispensable tool for coders and developers aiming to achieve maximum efficiency through enhanced code readability and maintainability.