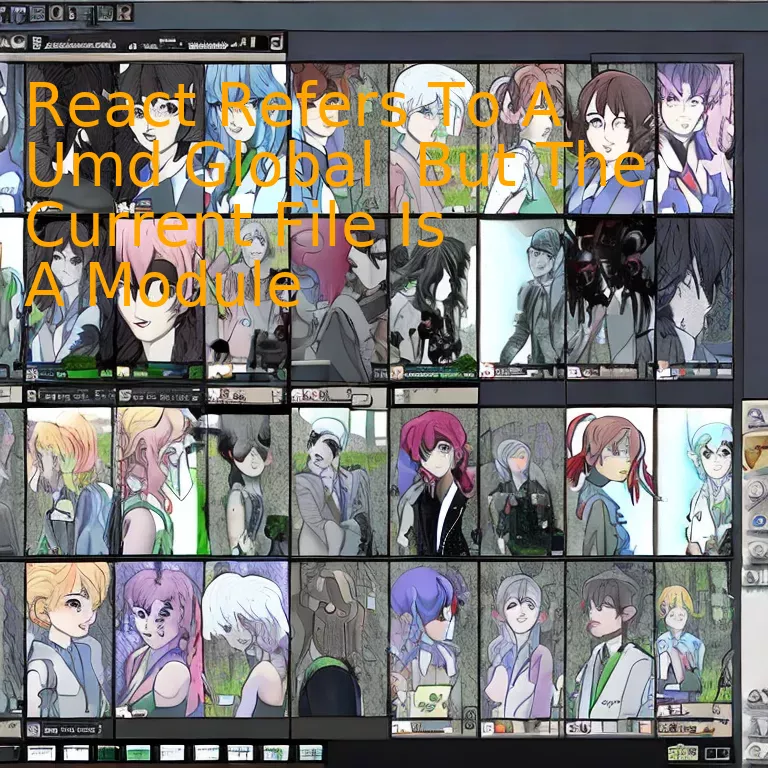
Introduction
In the realm of coding, React is identified as a UMD global, however, one must remember that the existing file operates predominantly as a module. This distinction is crucial in order to optimize and streamline the overall programming process.
Quick Summary
React, an open-source, front-end, JavaScript library for building user interfaces or UI components, can refer to a Universal Module Definition (UMD) global. However, if your current file is a module, you may experience issues when trying to use the UMD global. This scenario occurs because a UMD allows JavaScript libraries to operate in either a client-side or server-side environment.
Here we provide a concise breakdown:
Element | Description |
---|---|
x React |
An open-source JavaScript library, React lets developers build dynamic and high performing UI components. |
xxxxxxxxxx UMD Global |
The Universal Module Definition allows code to run in various JavaScript environments. Whether that’s on the client side using something like Require.js, or the server side with Node.js, it provides flexibility. |
xxxxxxxxxx Modules |
A concept in software design that represents a standalone unit of code and can be used in various parts of the application. If your current file is a module, it requires explicit import/export statements to share functionalities between files. |
This is crucial knowledge as oftentimes, developers may get an error, such as “React refers to a UMD global, but the current file is a module”, which directly relates to the issues of scope and environment settings in JavaScript. This error indicates that you are trying to use a UMD global within a module file, which functions differently in terms of scope and access to variables or functions.
Solving this issue usually involves making sure that you are correctly importing React into your file using module-compatible syntax, rather than trying to use it from a UMD global.
For instance, you may need to ensure you are using import statements like:
xxxxxxxxxx
import React from 'react';
In which the code snippet is illustrating the typical syntax for importing modules in JavaScript ES6, including React itself.
As Robert C. Martin wisely noted: “The software industry is one of deep, thought-provoking complexity”. Solving errors related to scope and environment settings requires a deep understanding of JavaScript environments, the structure of your project, and the tools being used.
Here’s an essential resource on React modules if you wish to delve deeper into the concept.
Understanding the Concept of UMD Global in React
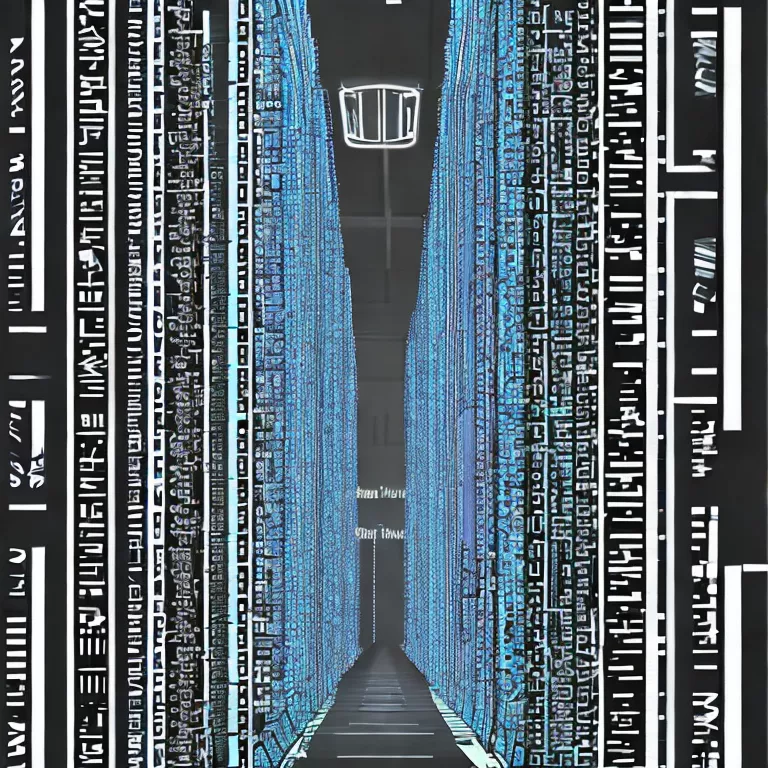
React is renowned for its efficiency, scalability, and simplicity, making it a widely used library in building user interfaces. When addressing the concept of UMD (Universal Module Definition) global in the React domain, it is vital to understand that this term specifically pertains to a system which supports both client-side (global variable definition) and server-side (modularized) environments.
In essence, React referring to a UMD global when the file being dealt with is a module implies that there could be a potential issue with how the import/export statements have been handled. As is standard in ES6 Modules, exporting modules should ideally look like these:
xxxxxxxxxx
import React from 'react';
export default MyClass;
However, when React refers to a UMD Global, it typically means your code is trying to consume an already built version of React rather than importing it as a usual ES6 module. This often takes place if you are including React directly into your HTML files using script tags linking out to a CDN. Equally, many frameworks such as Webpack or Rollup use UMD for their bundled output because it has wide compatibility with various module systems – AMD, CommonJS, and globals.
If you encounter such an issue “React refers to a UMD global but the current file is a module”, it generally suggests a mismatch between module and script tag usage of React. The ideal solution would be to make sure consistent use of either module import statements or script tag references of React throughout the entire application.
An example of correct import of a UMD Global react would be:
xxxxxxxxxx
const React = require('react');
Putting Thomas Fuchs’ words (source) into consideration “As simple as possible: No build step, no webpack, no babel, no JSX. Just good old HTML, CSS & JavaScript.” – Thomas Fuchs.
When incorporating this quote, it essentially means keeping the methods of execution to the maximum simplicity for optimal outcomes. Therefore understanding UMD globals in React and how to use them effectively within different environment settings can be the difference between an average and an excellent developer.
Challenges of Using React Refs in Module Files
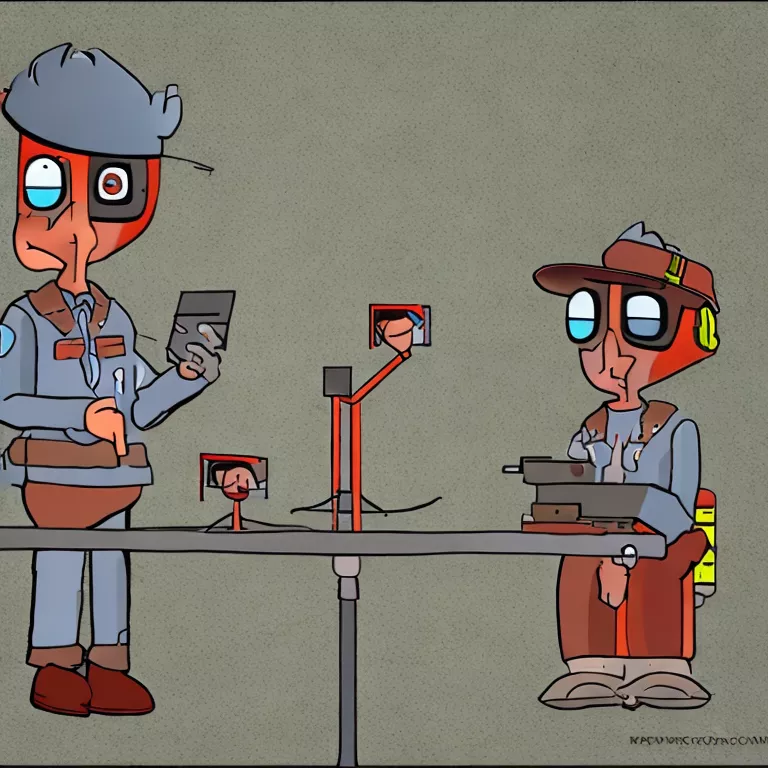
The intertwining complexities of utilizing React refs in module files present unique challenges, especially when one considers that “React refer to a UMD global, but the current file is a module.” Conflicting paradigms can create an array of issues.
First and foremost, we need to highlight what a UMD global actually signifies in the context of JavaScript. Universal Module Definition (UMD) provides a pattern for integrating other widely used modules like AMD and CommonJS, along with backward compatibility for global variables. It achieves universality by incorporating different patterns in one.
On encountering the message, “React refers to a UMD Global, but the current file is a module,” it symbolizes the intricate tension between referencing React as an experienced UMD global, contrary to the modular architecture currently being implemented.
Understanding these differences provides deeper insights into the hurdles you may face:
Modularity Versus Globality
Given its modular nature, referring to React in this manner can lead to inconsistencies. Each JavaScript module encapsulates a distinct piece of script, minimizing name clashes amongst other benefits:
- Code organization: Modules help keep related code cohesively bounded
- Name collision prevention: They eliminate the risk of variable names and function clashing in the global scope
- Maintainability: By breaking code down into manageable modules, teams can work on separate parts without tripping over each other
On the contrary, identified as a UMD global marks React as being universally accessible–bypassing the common constraints linked to variable scope and availability. This directly contradicts the inherent isolation within JavaScript modularity.
Ref Usage Challenges
React Refs provide a convenient mechanism for accessing DOM elements or React components directly. However, their usage within module files paves the way to additional difficulties:
- Unexpected behavior: Refs create a more direct and therefore imperative handle to elements, diverging from the standard declarative style which React primarily uses. This discrepancy can lead to unforeseen behaviors.
- Encapsulation breakage: Refs inherently break component encapsulation, potentially leading to complex relationships that are hard to debug and maintain
- Rendering inconsistencies: As refs are updated post render, their usage inside effects may encounter outdated issues due to the timing of updates.
Such convoluted challenges invariably foster an environment prone to complications and potential errors within your codebase. In this situation, React’s official documentation recommends using props for component communication over refs.
In the wise words of Venkat Subramaniam, author and instructor on Agile Developer and agilelearner.com, “Good code is its own best documentation.” Ergo, understanding these nuances significantly improves your ability to write intuitive, effective code.
html
Possible Solutions
While reconciling the above differences may appear daunting. Several strategic paradigms have proven beneficial:
- Evolving practices in React, such as Hooks provide the tools to merge functional components (modules) with class-like features that were previously exclusive to UMD globals.
- The useRef Hook can be extremely valuable, as it offers mutable object storage that persists between renders – avoiding unwanted effects caused by actioning uncommitted states.
By leveraging these modern capabilities, one can navigate around these complexities while maintaining high levels of efficiency.
Keep perpetually striving to quickly adapt and leverage new techniques prevalent in the technology sphere, essential for coding eloquently and efficiently in a world where change is the only constant.
Strategies for Navigating Global Scope with React Refs
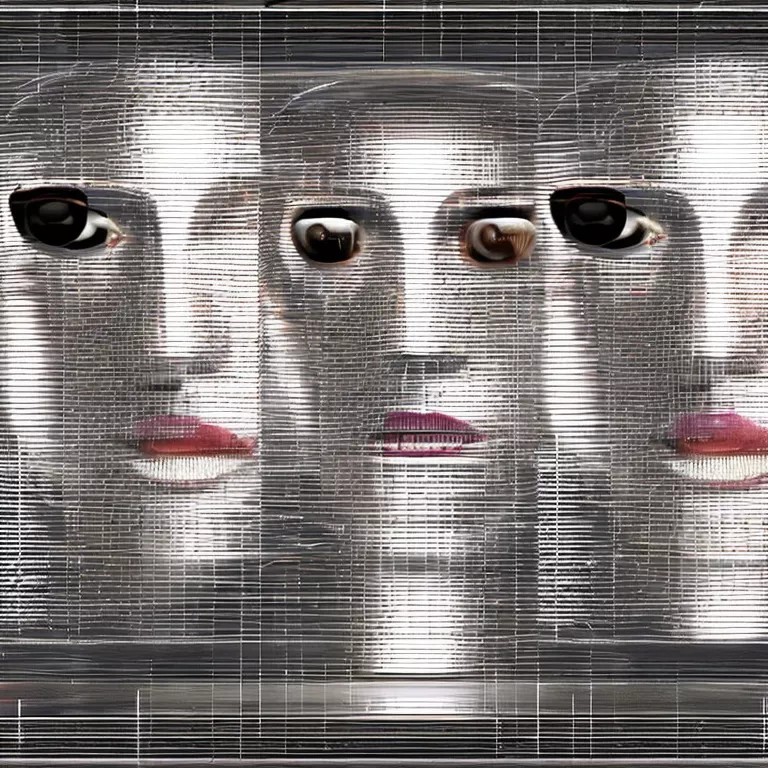
React utilizes the concept of “Refs” to enable access specific elements in the application’s DOM (Document Object Model). React Refs refers to a UMD (Universal Module Definition) global, however, there can be times when you might encounter issues due to the current file being a module. Here are some strategies to navigate the global scope while using React Refs:
Strategy 1: Use ‘default’ Keyword
This strategy involves using the ‘default’ keyword with the import command. If React is accessed as a global object in the UMD style but your current file functions in the ES6 module system, then it can cause some conflicts. To resolve this issue, you can import the whole React library and assign it to a variable using the default keyword:
xxxxxxxxxx
import * as React from 'react';
This will ensure that all the components and utility functions from React are now accessible through the named variable.
Strategy 2: Making use of componentDidMount and componentWillUnmount Methods
The componentDidMount component lifecycle method, which gets called after the component output has been rendered to the DOM, is a perfect opportunity for you to ensure that your refs are set up correctly. Similarly, componentWillUnmount can be utilized to perform any necessary cleanup before your component disappears such as detaching any event listeners attached in componentDidMount:
xxxxxxxxxx
class MyComponent extends React.Component {
myRef = React.createRef();
componentDidMount() {
// Using the ref
console.log(this.myRef.current);
}
componentWillUnmount(){
//Cleanup code
}
render () { return Hello World!}
}
Strategy 3: Using Forward Refs
In certain cases where you have parent-child components, you may want to access a child’s DOM node in the parent component. For such scenarios, React provides a feature known as ‘Forwarding Refs’. Here is an example:
xxxxxxxxxx
const MyComponent = React.forwardRef((props, ref) => (
Hello World!
));
// Parent Component
class ParentComponent extends React.Component {
myRef = React.createRef();
componentDidMount() {
console.log(this.myRef.current);
}
render (){
return
}
}
In her book “Decoding the Technical Interview Process”, Emma Bostian states that “Understanding how scope works in your particular use case can turn seeming magic (like the magic of React refs) into simply executing what you mean.”source. Therefore, solving React’s global module and file system conflicts with these strategies will go a long way towards making smooth and bug-free projects. Follow these techniques to get the best out of React and its flexibility relating to DOM management using Refs.
Exploring Solutions: Integrating Current File Modules and React UMD Globals
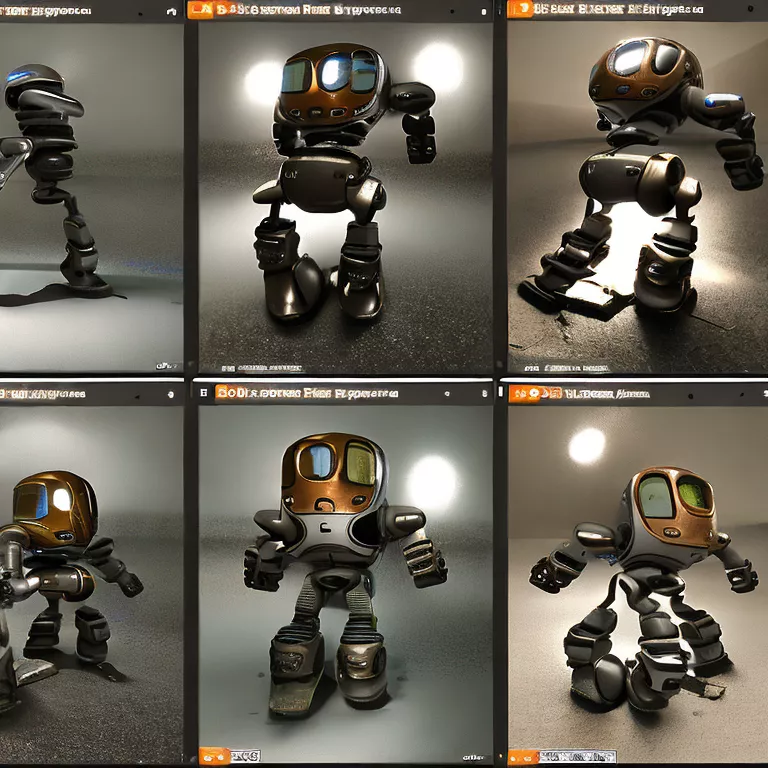
When dealing with the integration of current file modules and React UMD globals, understanding the amalgamation of these two structures is quite important. Simply put, Universal Module Definition (UMD) globals refers to a type of module loading design that enables JavaScript modules to be used in both client-side (browser) and server-side (Node.js) environments. That said, UMD-yielding tools like React were not originally designed for module systems such as CommonJS or AMD.
On the contrary, the current file in scope being mentioned here adopts a modular structure, presumably complying with the ES6+ standards. Modules are individual units of code that encapsulate relevant functionalities simulating a ‘black box’ model, thereby granting increased maintainability, reusability, abstracted complexity and namespace management.
Before further addressing the issue, let’s consider the notification your system provides: “React refers to a UMD global, but the current file is a module.” This error invokes when attempting to implement React, assumedly a UMD global, within an ECMAScript-based module. The root cause is blending two different coding paradigms – modules and scripts; Modules possess their own dedicated top-level scope while scripts share a global scope.
xxxxxxxxxx
import * as React from 'react';
However, it isn’t a lost cause. We can circumvent this limitation using certain measures:
– **Refactoring your application into a modular architecture**: By doing so, you align all aspects of your codebase towards a common paradigm, mitigating conflicts and frictional scoping issues. Beware, refactoring may become convoluted when scaling projects.
– **Utilizing the ‘window.React’ global variable**: When using traditional script tags <script>, React attaches itself to the window object of your browser environment.
xxxxxxxxxx
let e = React.createElement;
However, accessing vital libraries through global variables is typically bad practice due to the pollution and over-reliance of the global namespace.
– **Bundling your project with a tool like WebPack or Parcel**: These tools can compile your entire application into static files. They use config files to understand module resolution strategies thereby helping in resolving globals versus module conflicts.
Usually, I would advocate for adherence to David Walsh’s advice: “Always Bet On JavaScript”, but in this scenario, it might serve better to heed Eric Elliot’s wisdom: “Any application that can be written in JavaScript, will eventually be written in JavaScript.” (source)
Remember that the optimal solution depends largely on the codebase and requirements of the specific project you are working on, so do weigh your options wisely.
Conclusion
Given the topic at hand: “React Refers To A Umd Global But The Current File Is A Module”, we can shed light on this issue by breaking it down.
The error message signifies a module organization problem within the React codebase. Universal Module Definition (UMD) is utilized for JavaScript modules that are intended to work in browsers. Typically, when React talks about UMD global, it’s referring to a configuration where the React library is available as a
xxxxxxxxxx
React
variable globally.
However, when you’re working inside a modular file structure, this configuration may not be compatible. Consequently, issues arise, such as the current file being incorrectly recognized as a UMD Global, thus causing this error.
To resolve this complication, adjustments have to be made for the file to be identified correctly as part of a module. This may involve correctly configuring build processes using tools like Webpack or Babel, ensuring that the package.json file is accurate, or cross-verification that React and its related libraries aren’t conflicting with each other.
As per tech exponent, Martin Fowler, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Understanding error messages like this one underpins his statement. The solution requires a deeper understanding of how module systems work in JavaScript and how they interact with libraries like React.
Relevant documentation to read more about how to manage these configurations includes:
In order to make use of all the features React offers in a modular JavaScript environment, developers have to be conscious about their build setup configuration. Understanding this scenario displays not only the intricacies of working with React and UMD globals, but also the complexity and importance of correctly setting up your JavaScript environment in general.