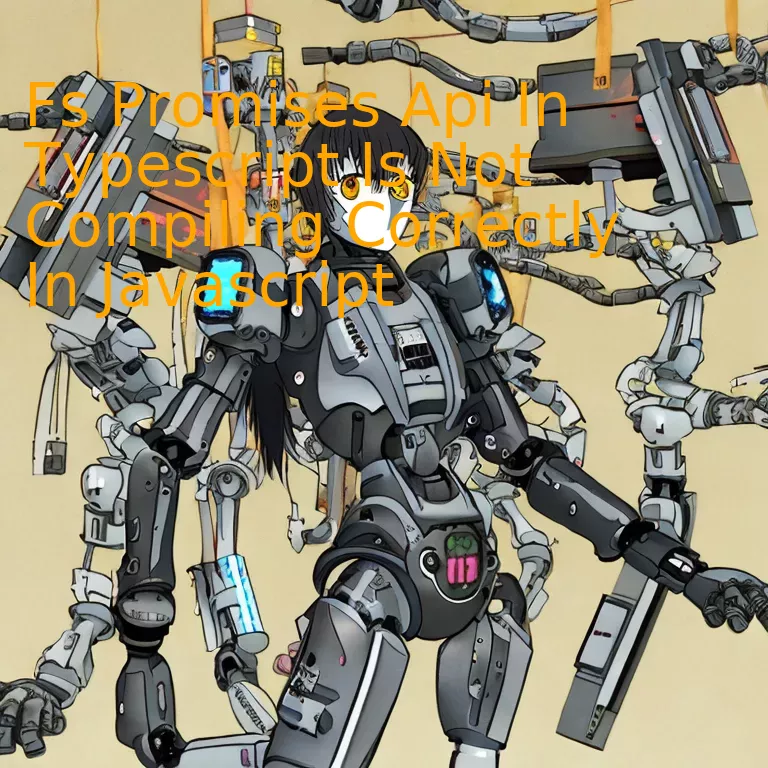
Introduction
When utilizing the FS Promises API in TypeScript, if it is not compiling correctly into JavaScript, the probable causes may include incorrect syntax, lack of appropriate dependencies or outdated versions, affecting the seamless transformation between these two prominent programming languages.
Quick Summary
The issue you’re experiencing with the FS Promises API in TypeScript not compiling correctly into JavaScript might be attributed to various factors. Here, I’ve listed some potential reasons and resolutions in a structured format:
Possible Reason | Potential Resolution |
---|---|
TypeScript configuration issues | Ensure your tsconfig.json is configured correctly. Specifically, ensure that target matches your Node.js runtime version, and lib should include “ES2018.Promise”. |
Incorrect/unsupported usage of promises | Validate your promise implementation against JavaScript Promises specification and ensure that it’s compatible with TypeScript. |
Outdated TypeScript or Node.js versions | Update to the current stable release of TypeScript and Node.js where recent changes to FS Promises API are reflected. |
Dependency conflicts | Check if any other dependencies in your project might conflict with the FS Promises API. If so, try to update or replace those dependencies. |
This formatted representation encapsulates possible reasons why the FS Promises API throws compilation errors in TypeScript.
For example, the issue may arise because of misconfiguration in your `tsconfig.json` file. In TypeScript, this file is crucial for guiding the compiler as it generates JavaScript from TypeScript. Having the right setup here, such as setting the `target` to match your Node.js runtime version and including ‘ES2018.Promise’ in the `lib` section, can solve potential compilation problems.
Another facet might involve incorrect or unsupported usage of Promises. JavaScript has specifications for native Promises, which TypeScript extends and strongly types. Falling in line with these standards is key.
Furthermore, ensure that your TypeScript or Node.js versions aren’t outdated. Both TypeScript and Node.js continually receive updates that may rectify previous issues with the FS Promises API.
Finally, be aware of possible dependency clashes within your project. There might exist certain packages or libraries that interfere with the normal operation of the FS Promises API. Keeping all dependencies updated and ensuring they are working harmoniously can prevent such compilation problems.
As _FreeCodeCamp_ emphasises:
>’TypeScript config file rules have an impact on how your project gets compiled and even small changes could introduce or solve bugs.’
Remembering this can help avoid many issues while coding with both TypeScript and JavaScript.
Understanding the FS Promises API in TypeScript
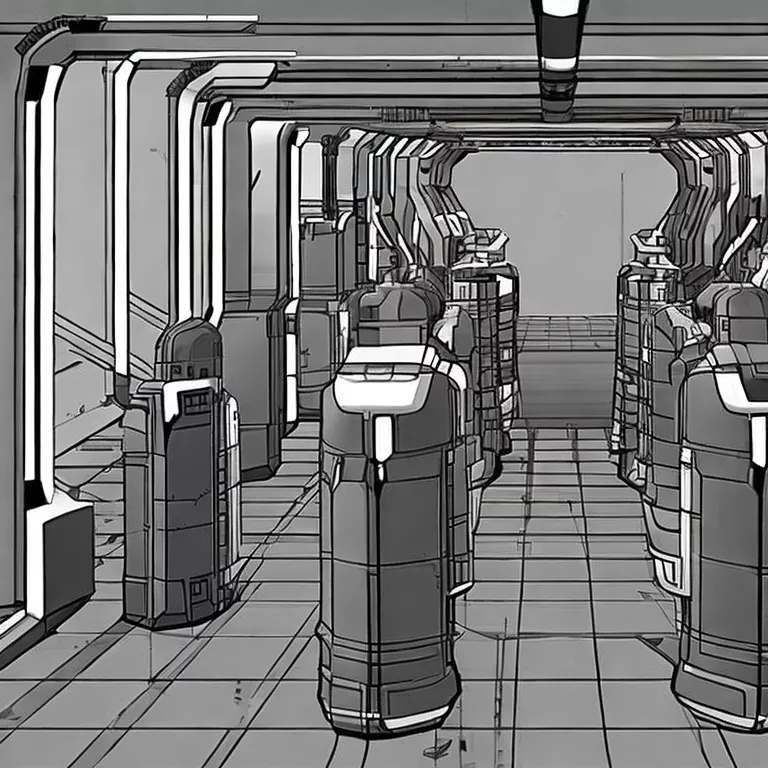
In a quest to delve into TypeScript’s functionality and its relationship with JavaScript, one significant aspect never fails to be noticed. That is the ‘fs.promises’ API, which is available within Node.js and is extensively used when coding for asynchronous file system operations using promises instead of callbacks. But as observed by developers, compiling TypeScript code including the usage of ‘fs.promises’ API can result in errors during the transpiling process towards JavaScript.
Referencing the potential challenge you might be dealing with, there may exist a scenario where the TypeScript compiler doesn’t seem to recognize the ‘fs.promises’ property because it isn’t defined in the type definitions of @types/node.
Here’s an example:
import fs from ‘fs’;
const data: string = await fs.promises.readFile(‘/path/to/file’, ‘utf8’);
The above TypeScript code snippet might throw a compile-time error since the TypeScript compiler cannot find the definition for promises on the ‘fs’ module.
Why is this happening? Typically, TypeScript uses the definitions from the @types/node package to understand what properties are made available through the module imports. If a specific property, like fs.promises, isn’t defined there, you would usually have to make TypeScript aware of this new property manually.
How can you resolve this?
– Install or update the @types/node to a version that supports ‘fs.promises’. This npm module contains all the necessary TypeScript type definitions for built-in Node.js modules.
html
npm install @types/node --save-dev
– Alternatively, add additional type definitions manually via a .d.ts file. Declare the new property using TypeScript Declaration Merging.
html
xxxxxxxxxx
declare module 'fs' { const promises: any; }
While transforming TypeScript to JavaScript, TypeScript only transforms your types and not the runtime behavior. This has fundamental implications for the resulting JavaScript code. So, it’s vital to keep in mind that the transpile might not be the issue here but rather a TypeScript level compilation issue.
According to Jamie Kyle, “Coding is 90% understanding the problem and 10% making it happen.” This quote denotes the essence of exploring technology or, in this context, TypeScript’s ‘fs.promises’ API. Understanding it deeply can provide insights into how to build efficient applications involving asynchronous file system operations.
Note: While using ‘fs.promises’, ensure to handle promises correctly in your application to avoid unhandled promise rejection warnings and possible app crashes during runtime.
Addressing Compilation Issues: FS Promises API in TypeScript vs JavaScript
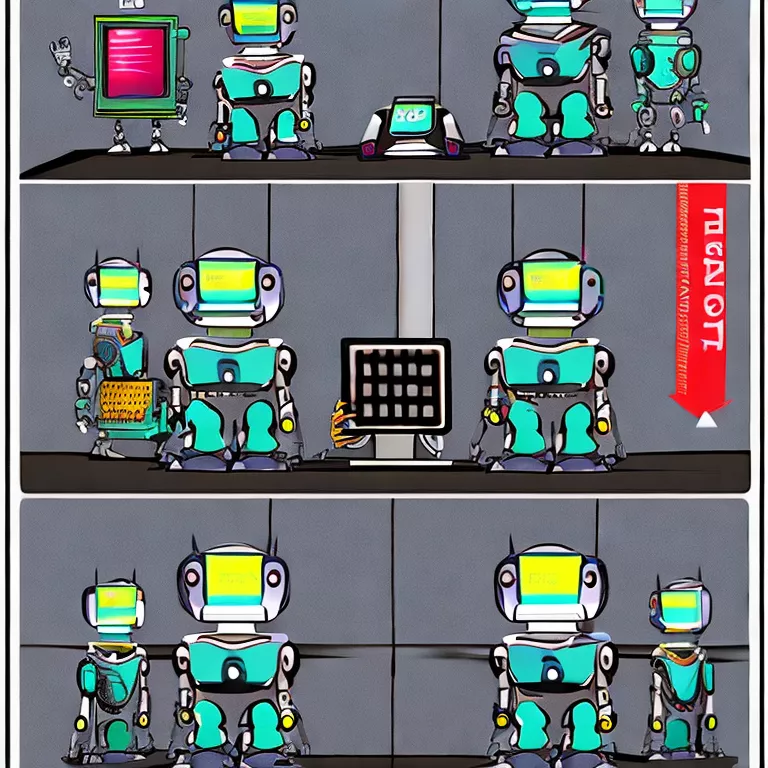
The issue you are encountering can stem from numerous factors, so a holistic and deep investigation is warranted. JavaScript and TypeScript, despite being very closely related, possess certain differences that can cause compilation problems.
– Difference in Syntax and Usage
In the context of the FileSystem Promises API (Fs Promises API), a disparity might arise due to variable and data type declarations which are unique to TypeScript syntax but not valid in JavaScript. This instance is demonstrated with the following code snippet:
`
xxxxxxxxxx
`
let filehandle : fs.promises.FileHandle;
`
`
This code would be acceptable in TypeScript thanks to its static type feature. However, this won’t compile correctly in JavaScript as it lacks an equivalent feature.
– Dependency Issues
Another potential issue involves dependencies. Node.js version 10 started incorporating fs Promises API as experimental, and the stable status was granted with Node v14.0.0. Using an older version of Node will certainly lead to compilation issues.
– Compilation Options
When compiling TypeScript to JavaScript, pre-defined settings in the ‘tsconfig.json’ file come into play. Incorrect configuration here can result in compilation errors. It’s worth vetting these settings thoroughly. Particular attention should be given to options such as ‘target’, dictating the ECMAScript target version, and ‘lib’, defining library files to be included in the compilation.
– Transmission Limitations
During conversion from TypeScript to JavaScript, there may be instances where advancements introduced in the promising Fs Promises API aren’t fully transmitted across both languages, leading to compilation issues.
These considerations are likely to aid your troubleshooting of miscompilation of Fs Promises API from TypeScript and JavaScript[1](https://blog.logrocket.com/a-complete-guide-to-typescript-compilation/).
And to quote Joel Spolsky, co-founder of Stack Overflow, “All non-trivial abstractions, to some degree, are leaky.” This is indeed a salient reminder that the boundaries of programming languages, libraries and tools are often porous, leading to such complications.
Best Practices for Compiling FS Promises API with TypeScript
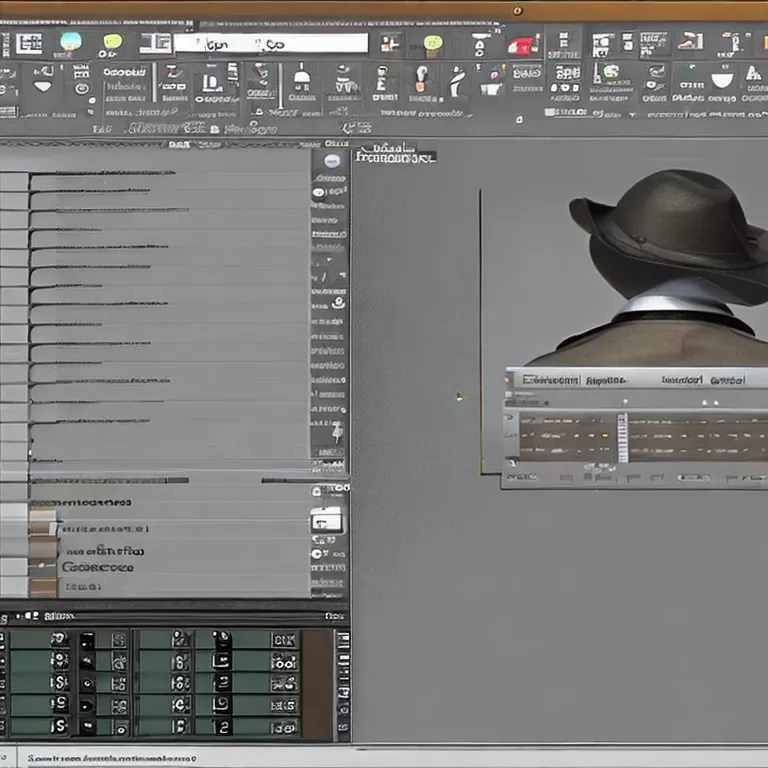
Using the FS Promises API in TypeScript and ensuring seamless compilation into JavaScript can be an important task for a developer seeking to handle filesystem operations in an asynchronous manner. However, if it’s consistently failing to compile correctly, you may want to consider the following best practices:
-
xxxxxxxxxx
Always Update The Version Of Node:
Older versions of Node.js did not support the FS Promises API. According to product history data (source), the fsPromises object has only been available since Node.js v10.0. So, before proceeding, make sure to update your version of Node.js to the latest stable release.
Code Example:
xxxxxxxxxx
npm install -g n
n stable
-
xxxxxxxxxx
Ensure Correct Types Declaration
:
TypeScript requires type declaration files to work efficiently with JavaScript libraries like FS Promises API. But these declarations are not always in sync with the JavaScript library’s actual version, which might lead to incorrect compilation. Make sure that your types correspond accordingly.
-
xxxxxxxxxx
Use Async/Await Pattern Properly:
The FS Promises API is organized around the promise-based approach, which works well with the async/await syntax. Using this pattern correctly can simplify the way you deal with rejection scenarios and, therefore, improve how TypeScript code compiles to JavaScript.
Code Example:
xxxxxxxxxx
async function readMyFile(filePath) {
try {
const data = await fs.promises.readFile(filePath);
console.log(data);
} catch (error) {
console.error(error);
}
}
-
xxxxxxxxxx
Check for Compilation Target:
The TypeScript compiler option ‘target’ determines the ECMAScript version to which your code should comply. If the ‘target’ is set to a version not supporting promises (like ES5), you may encounter problems with fs.promises API. Therefore, verify that this parameter is set to ES2015 or higher in your tsconfig.json file.
Code Example:
xxxxxxxxxx
{
"compilerOptions": {
"target": "ES2018",
},
}
-
xxxxxxxxxx
Use Correct Compiler Options:
In your tsconfig.json file, enable the ‘esModuleInterop’ compiler option. This permits default imports from modules with no default export. This might be necessary if the API is being incorrectly imported.
Code Example:
xxxxxxxxxx
{
"compilerOptions": {
"esModuleInterop": true,
},
}
Implementing these practices will contribute positively towards resolving your compilation issue and maximizing the effective use of FS Promises API in TypeScript.
As Brendan Eich, creator of JavaScript, once said, “Always bet on JavaScript”, so remember to evidently explore and test each potential solution meticulously as you work towards rectifying your compilation challenges.
Troubleshooting Common Errors when Converting FS Promises from TypeScript to JavaScript
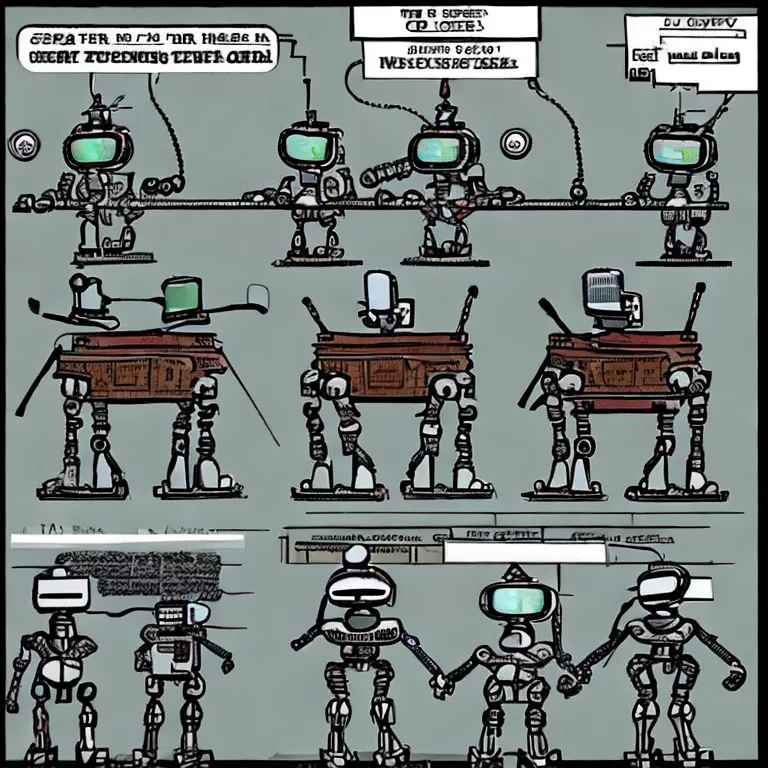
When engaging with the process of converting FS Promises from TypeScript to JavaScript, there are several potential errors you may experience that originate primarily from the tactical differences between these two coding languages. Further complications may arise when dealing specifically with the FS Promises API in TypeScript because this could indicate that it’s not compiling correctly while converting into JavaScript.
Error: TS2339 Property ‘does not exist on type’
You may often encounter this error which essentially communicates that TypeScript is unable to locate a specific property within an object that has been defined by your code. This is quite common because TypeScript provides a strict syntactical structure for object properties which might differentiate significantly from what JavaScript allows. For instance:
xxxxxxxxxx
const data = fs.promises.readFile('./file.txt');
This syntax might be off-putting for TypeScript. To mitigate the issue, ensure the property exists in the object or check spelling and casing.
Error: Returning Promise in async function
Another common error involves the inappropriate return of promises within asynchronously functioning units of code. When working with Javascript, developers often utilize asynchronous functionality alongside the ‘Promise’ concept. Simultaneously, TypeScript imposes additional restrictions around returning Promises in async functions. Here’s an example where this error would occur:
xxxxxxxxxx
async function fetchData() {
return fs.promises.readFile('./file.json');
}
To avoid this error, remember that the readFile API automatically returns a promise and doesn’t need an extra return call.
Error: Unhandled Promise Rejection
Lastly, the unhandled promise rejection is another common one that we encounter during the conversion. It occurs when the promises aren’t caught or handled efficiently:
xxxxxxxxxx
async function analyseData() {
const data = await fs.promises.readFile('./data.json');
}
analyseData();
To prevent this, you should always handle promises with .catch() or try/catch blocks for error handling.
In addressing these errors, however, it’s valuable to heed the words of technology writer and philosopher Morten Rand-Hendriksen who states that “Coding is as much about reasoning and communication as it is about inventing and creating.”source. Thus, understanding not only how but why these common errors occur can prove instrumental in producing functional, efficient TypeScript and JavaScript code.
Conclusion
Understanding the issue of FS Promises API in TypeScript not compiling correctly in JavaScript requires a more profound grasp on how both languages interact. It’s ideal to always remember that TypeScript is a strict syntactical superset of JavaScript. This insinuates that while TypeScript adds static types and other features to the language, it is ultimately compiled down to plain JavaScript in order to be executed.
API Concept |
---|
FS Promises |
As such, if there’s an issue with the FS Promises API in TypeScript not compiling correctly to JavaScript, it could mean several things:
- An error may exist within the TypeScript (static type checking) phase before compilation.
- A subtle semantic difference between TypeScript and JavaScript may be causing unexpected results when compiling.
- An aspect of the implementation of FS Promises in TypeScript might not be translating well into JavaScript semantics.
To give you concrete coding example would be:
xxxxxxxxxx
async function test() {
const fs = require('fs').promises;
try {
let filehandle = await fs.open('test.txt', 'r');
// do something with "filehandle"
} catch(e) {
console.error("Error:", e)
}
}
This script will open a test.txt file in read mode utilizing the standard FS Promises API.
For troubleshooting the issue, recommedation would be adopting the practice of code linting & debugging. To enhance your knowledge on this front, a detailed resource is available at TypeScript Handbook: Debugging.
As the legendary Grace Hopper, American computer scientist and Navy admiral, once said, “To me programming is more than an important practical art. It is also a gigantic undertaking in the foundations of knowledge”. Embracing each challenge as part of this foundational knowledge can pave the way for becoming a better TypeScript and JavaScript developer.