Introduction
Experience the resolution for Error Ts2322, where a type mismatch happens as Event is not assignable to type String in TypeScript, aiding smooth and efficient code execution.
Quick Summary
The context of “Error TS2322: Type ‘Event’ is not assignable to type ‘String'” from TypeScript, is a situation where you encounter an error during coding because a particular value or variable, which is expected to be a ‘String’ data type, is receiving an ‘Event’ type instead.
Consider the table below:
Data Type | Description | Example | |
---|---|---|---|
‘String’ | A sequence of characters used for textual data. |
x "Hello World" |
|
‘Event’ | An object that describes an action that has occurred, typically triggered by user interaction. |
xxxxxxxxxx new Event("click") |
The error mainly stems from TypeScript’s static typing feature which ensures that variables are always treated as a specific type. Quite often this stems from designing your code with a ‘String’ in mind, but then passing an ‘Event’ object instead.
Take for example the following incorrect chunk of TypeScript code:
typescript
let name: string;
name = new Event(‘click’);
The type check here will fail as ‘name’ was initially defined expecting a string and instead received an ‘Event’. To fix this error, you need to ensure that ‘name’ receives a ‘string’ type:
typescript
let name: string;
name = ‘Adam’;
In the grander scheme of web development, understanding the data types in TypeScript and how they’re assigned can amplify the productivity of one’s code. By ensuring correct data type assignment, developers leave less room for bugs and improve the overall maintainability of their code.
To quote Martin Fowler, prominent software developer and author, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”. Understanding the simple basics of data types can lead to more readable and comprehensible code – aligning with Fowler’s sentiment that quality code is written for humans first.
A poignant reference can be found here which delves deep into the basic types in TypeScript. The doc provides an overview of several types, their functionality, and how to use them effectively. The better a developer understands these types, the less likely they will encounter the aforementioned error.
Understanding Error TS2322: Deep Dive into Typescript Mismatch
Understanding the nature of this specific TypeScript error, TS2322, revolves around the fundamental aspects of TypeScript’s static typing system that requires variables to hold values of a specific type. The phrase “Error TS2322: Type ‘Event’ is not assignable to type ‘String’.” essentially means that a variable declared as a string is being assigned a value of type Event, which conflicts with TypeScript’s typing rules.
Let’s delve into some elements that lead to this issue:
Type Assignation in TypeScript:
In TypeScript, when a variable is declared with a particular type, it is expected that this type be maintained throughout the code. For instance, if we declare:
xxxxxxxxxx
let sampleVariable: string;
, TypeScript will enforce that “sampleVariable” is used as a string.
The ‘Event’ and ‘String’ Types:
The types involved in Error TS2322 in this context are ‘Event’ and ‘String’. A ‘string’ represents a sequence of characters while an ‘Event’ object represents an event taking place in the DOM – these two types don’t match. Attempting to assign an ‘Event’ type to a variable initially defined as a ‘String’ type invites this error.
Consider this example:
html
js
function handleClick(event: String) {
// Logic Here
}
The argument provided to the handleClick function,
xxxxxxxxxx
event
, is actually of type Event, but the function expects it to be a String.
Resolving Error TS2322:
When a function takes an Event as an argument but you want to handle it as a String, you can use properties of the Event object to obtain a string. One common property to provide a stringifiable value is
xxxxxxxxxx
target.id
, considering that your event target has an id attribute.
Here’s an example of how to modify the previous code snippet using this concept to avoid TypeScript error TS2322:
html
ts
function handleClick(event: Event) {
let targetElementId: string = event.target.id;
// Logic here using targetElementId which is ensured to be a string
}
As Robert C. Martin, a renowned software engineer, said: “A good software structure is essential for system longevity and straightforward maintenance.” TypeScript’s robust static typing system promotes structural integrity in our code by preventing potential ambiguities brought about by interchangeable data types. By understanding TypeScript errors like TS2322, it develops writing clear code that respects variable types, leading to more maintainable, bug-resistant applications.
For further reading on TypeScript, consult the official documentation.
Strategies to Tackle Error TS2322 in TypeScript
Tackling error TS2322 in TypeScript, specifically when it presents as “Error TS2322: Type ‘Event’ is not assignable to type ‘String'”, involves understanding the inherent system of TypeScript’s static typing and exploiting its flexibility for your benefit.
TypeScript Static Typing
The origin of error TS2322 lies in TypeScript’s core property as a statically typed super-set of JavaScript. Statically typed languages require type information to be specified and don’t permit interchangeability of data types without explicit type conversion or coercion.
Error TS2322 occurs when you try to assign an object of one type to a variable of another type. When it refers to a problem between ‘Event’ and ‘String’ types, it means you’re probably handling an HTML event, often involving user interaction such as button click or text input, and attempting to directly assign it to a string variable.
Strategies to Resolve Error TS2322
Proper Event Handling
Events in HTML contain various properties and methods that are useful depending on the scenario. If you’re interested in the value a user inputs into a text field, you would drill down into the ‘target’ property of the event which holds the HTML element triggering the event. From there, you can retrieve its ‘value’ property – a string.
Here is a TypeScript code snippet demonstrating this:
xxxxxxxxxx
handleInput(e: Event) {
const value = e.target.value;
}
Type Assertion
In scenarios where you are confident about the structure and content of your data, you can use type assertion to bypass TypeScript’s static type checking. It’s like telling the compiler “Trust me, I know what I’m doing”. You perform it with the syntax
xxxxxxxxxx
as
. But remember, with great power comes great responsibility – misuse can lead to runtime errors. Here is how to apply type assertion:
xxxxxxxxxx
handleInput(e:Event) {
const value = (e.target as HTMLInputElement).value;
}
Type Guard
Instead of skipping the compiler’s checks, you might want to work with them. Type guards allow you to branch your code according to specific type conditions or checks. If the variable is of a certain type, the type guard conditions allow TypeScript to infer its type within that branch.
Here’s an example using a User-Defined Type Guard:
xxxxxxxxxx
function isHTMLInputElement(obj: any): obj is HTMLInputElement {
return !!obj && typeof obj.value === 'string';
}
handleInput(e: Event) {
if (isHTMLInputElement(e.target)) {
const value = e.target.value;
}
}
Alan Turing, the famed computer scientist, said – “We can only see a short distance ahead, but we can see plenty there that needs to be done.” As a developer, it stands relevant to the dynamic situations you handle, including TypeScript’s error TS2322. By understanding the root causes behind this static typing error and using these strategies, you can ensure fluid data handling while maintaining TypeScript’s robustness.
For further understanding of dealing with Type errors in Typescript, you could refer to TypeScript Advanced Types documentation.
Real-world Examples of ‘Error TS2322: Type Event is not Assignable to Type String’
The TypeScript compiler error ‘TS2322: Type ‘”Event”‘ is not assignable to type ‘string” manifests when there is a type mismatch, and we are trying to assign an incorrect value to a variable. This particular error typically arises when dealing with browser events in the context of front-end web development.
Let’s dive deeper into this by considering some real-world examples.
Example 1: Consider an onclick event for a button in HTML.
And on the TypeScript side, let’s suppose we are adding an event listener like so:
xxxxxxxxxx
let submitBtn = document.getElementById('submit-btn');
let userInput: string;
submitBtn.addEventListener('click', (e) => {
userInput = e;
});
In the above case, you will see the TypeScript error ‘TS2322: Type ‘”Event”‘ is not assignable to type ‘string”. This is because we are trying to assign the entire Event object to a variable of type ‘string’. The TypeScript type system catches this as a mismatch.
Solution: To resolve this, we can instead assign the content of the field from the Event object that is actually a string.
xxxxxxxxxx
submitBtn.addEventListener('click', (e): string => {
userInput = e.target.value;
});
Example 2: Imagine using InputEvents in a form:
xxxxxxxxxx
let inputElement = document.getElementById('name-input') as HTMLInputElement;
let inputValue: string;
inputElement.addEventListener('input', (e) => {
inputValue = e;
});
Again, ‘TS2322: Type ‘”Event”‘ is not assignable to type ‘string” error would be raised here as ‘e’ resolves to an Event object and we are trying to assign it to a ‘string’ type.
Solution: Similar to the prior example, the correct field from the Event should be extracted to fit the string type.
xxxxxxxxxx
inputElement.addEventListener('input', (e): string => {
inputValue = e.target.value;
});
To quote Martin Fowler, an influential figure in the field of software development: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”[^1^] When writing or refactoring code, strive for clarity and correctness as the key guidelines rather than brevity. The TypeScript compiler is an incredibly strong tool that helps developers write more reliable, maintainable and understandable code by catching these kind of errors.
[^1^]: Martin Fowler
Best Practices and Troubleshooting Steps for Resolving “Type Event Is Not Assignable To Type String”
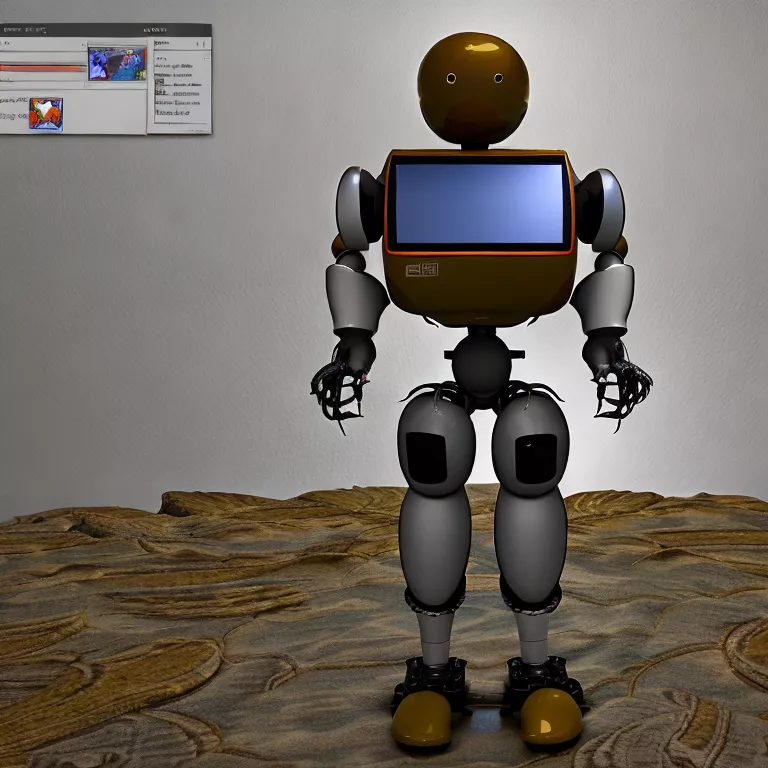
Understanding and resolving the TypeScript error “Type Event is not assignable to type string” is an important aspect of a TypeScript developer’s work. Generally, this error manifests in situations where there has been either incorrect typing or misuse of event handlers.
One might come across this issue typically when attempting to assign an event type (usually garnered from interactive DOM elements) directly to a variable typed as a string. To illustrate:
xxxxxxxxxx
let myVariable: string;
element.addEventListener('click', (event) => {
myVariable = event; // Error TS2322: Type 'Event' is not assignable to type 'string'.
});
The crux of the problem lies in the fact that event is not of type ‘string’. The TypeScript compiler is doing its job here, enforcing static typing rules.
To resolve this, there are several best practices that can be employed:
Use Target Properties: Persuasively, the best solution is to extract the desired string value from the target property of the JS event. For textual inputs such as input fields, the target value should correspond to the user’s input.
xxxxxxxxxx
let myVariable: string;
element.addEventListener('click', (event) => {
let target = event.target as HTMLInputElement;
myVariable = target.value;
});
Type Assertion: In some cases, you might know that the event will be a string object. If so, one could leverage TypeScript’s type assertion feature. However, it’s risky as it bypasses TypeScript’s type system.
xxxxxxxxxx
let myVariable: string;
element.addEventListener('click', (event) => {
myVariable = event as any as string;
});
Achieving success requires understanding and applying these methods thoughtfully. Kate Gregory, a renowned software developer, once said, “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.” Adopting this perspective, we can see the importance of comprehensively understanding data types when wrestling down TypeScript errors[1].
References:
[1] TypeScript: Basic Types
Conclusion
Deep diving into the realm of TypeScript, we often encounter various errors that challenge our problem-solving skills. One such common error is
xxxxxxxxxx
Error TS2322: Type 'Event' is not assignable to type 'String'
. As TypeScript is a statically typed superset of JavaScript, it offers superior type checking at compile time. If there’s a mismatch of data types in your code, TypeScript will promptly notify you with exceptions like this.
The error basically points out that an ‘Event’ type object has been erroneously assigned to a variable which was declared to be of ‘String’ type. This happens primarily due to confusion between static typing in TypeScript and dynamic typing in JavaScript. In TypeScript, once a variable is declared with a particular type, it cannot be assigned a value of another type, hence triggering this error.
The best solution for rectifying this error involves modifying the way variables are assigned; ensuring that the assignment aligns with the declared type of the variable. Appreciating TypeScript’s assistance in error identification during coding helps strengthen your debugging skills, allowing you to write cleaner, less-error-prone code. An effective workaround would be doing a proper type assertion or a conditional type check.
xxxxxxxxxx
let event: Event;
let str: string;
str = event as unknown as string; // correctly assigning 'Event' to 'string'
This example shows how you can resolve the problem by performing a double assertion: first, assert the ‘Event’ as ‘unknown’, then the ‘unknown’ as ‘string’. Essentially, you’re informing TypeScript to consider the ‘Event’ as a ‘string’ type variable to avoid the Error TS2322.
TypeScript documentation expands on different use cases of types and their handling aspect. TypeScript’s keen type-checking mechanism extends beyond the Type ‘Event’ is not assignable to the Type ‘String’ error, making it an incredible tool for scaling JavaScript development.
As Edsger Dijkstra once said, “If debugging is the process of removing bugs, then programming must be the process of putting them in”. However, by leveraging TypeScript’s static typing and understanding the mechanisms behind the errors, we can certainly reduce the time spent debugging and focus on coding functionality.