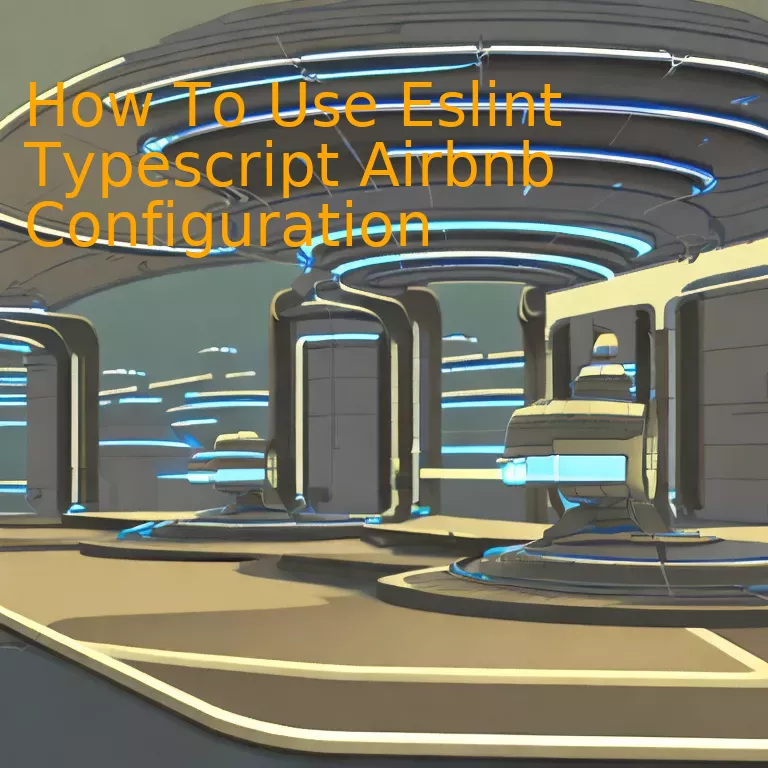
Introduction
To implement the ESLint TypeScript Airbnb configuration effectively, you begin by installing necessary dependencies, then create a .eslintrc file in the root directory and specify relevant rules, ensuring your code adheres to Airbnb’s coding guidelines which can enhance your projects overall quality and readability.
Quick Summary
To shed light on how to use ESLint Typescript Airbnb configuration, here is a simplified guide represented in a structured format and a step-by-step walkthrough:
Action | Result |
Installation of ESLint | This process requires running the command:
npm install eslint --save-dev . This initiates the installation of ESLint as a developer dependency in your project. |
Configuring ESLint with Airbnb’s TypeScript guide | Once you run
npx install-peerdeps --dev eslint-config-airbnb-typescript , this configures ESLint according to Airbnb’s TypeScript Style Guide. |
Adding ESLint config file | This entails creating a
.eslintrc.js or .eslintrc.json file at the root of your project then adding Airbnb’s configuration details. An example would be: { "extends": ["airbnb-typescript"] } . |
Running ESLint | You can easily run ESLint by using the command:
npx eslint '*/**/*.{js,ts}' --quiet --fix in the terminal. Any linting errors or warnings will show up here. |
The primary step involves setting up ESLint within your TypeScript project – this involves installing ESLint as a development dependency using npm (Node Package Manager).
Following installation, the next critical action is linking ESLint with Airbnb’s TypeScript Style Guide. You accomplish this by initiating a specific command that installs necessary peer dependencies for the Airbnb configuration.
Upon successful binding of ESLint and the Airbnb configuration, it becomes crucial to add an ESLint config file (either .eslintrc.js or .eslintrc.json format) at the root directory level of your project. It is in this file that you define rules based on which ESLint will scrutinize your codebase.
Once the ESLint configuration setup has been completed, all that remains is checking your TypeScript code for any linting errors. You can achieve this by running ESLint using an appropriate terminal command. This operation then inspects each JavaScript (.js) and TypeScript (.ts) file within your project while suppressing warnings and automatically fixing what it can.
As Linus Torvalds stated: “Talk is cheap. Show me the code”. Following this sequential approach ensures a smooth setup of ESLint with Airbnb’s TypeScript configuration, allowing developers to enforce consistent coding standards across their project, thus improving quality and maintainability of code.
Understanding the Basics of ESLint, TypeScript and Airbnb Configuration
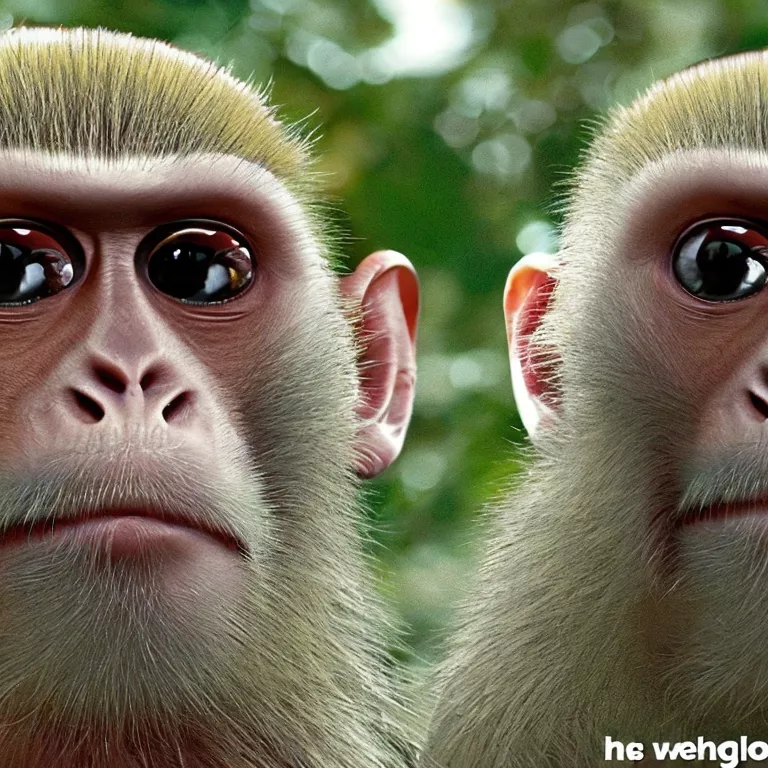
An understanding of ESLint, TypeScript and Airbnb configuration is pivotal when dealing with high-quality JavaScript code. With this trinity, you can bolster your development process through static analysis tools that improve readability, maintain consistency, and reduce the potential for error.
Understanding ESLint
ESLint
is an open-source JavaScript linting utility which facilitates developers to discover problems in their JavaScript code without running it. It identifies patterns that do not adhere to certain style guidelines thus enhancing the code quality.
Understanding TypeScript
TypeScript
is an open-source language created by Microsoft, that extends JavaScript by including types. It provides static type checking during compile time, thus reducing errors and making debugging effortless.
Understanding Airbnb Configuration
Airbnb, a globally recognized name, maintains its JavaScript Style Guide, one of the most popular coding styles for JavaScript. The
Airbnb eslint configuration
is a widely-accepted extension that includes rules for ECMAScript 6+ and React.
To utilize the
Airbnb eslint configuration for Type Script
, follow these steps:
1. To begin with, install necessary packages by using npm or yarn.
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
2. Once installed, make use of the Airbnb base config in the .eslintrc file. If the file does not exist, create one.
{ "extends": ["airbnb-base"] }
3. In case you need to override existing rules or add new ones, modify the rules object in the .eslintrc file.
4. Execute ESLint on any file or directory just like this:
./node_modules/.bin/eslint yourfile.ts
With this integration, developers reap the benefits of a strict linting tool offering better readability and consistency across projects thus enhancing their code quality.
As Alan Turing once said, “We can only see a short distance ahead, but we can see plenty there that needs to be done.” Building a strong foundation in ESLint, TypeScript, and Airbnb configuration is one such necessary step for any developer to ensure a productive coding journey.(source)
Implementing ESLint with TypeScript: A Step-by-Step Guide
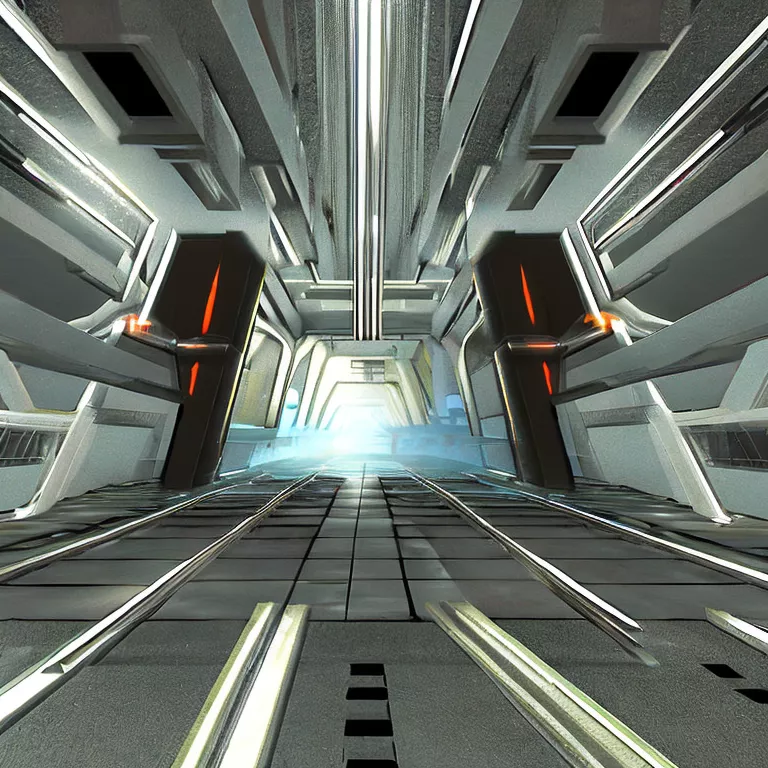
In the realm of TypeScript development, ensuring clean, consistent, and error-free code can be a challenging task. A solution to this predicament has arrived in the form of ESLint, a pluggable linting utility for JavaScript and, by extension, TypeScript. Paired with Airbnb’s popular style guide for TypeScript, ESLint provides an advanced workflow for quality assurance in TypeScript projects.
Firstly, it is important to understand what TypeScript, ESLint, and Airbnb configuration are:
– TypeScript stands as a typed superset of JavaScript, providing static definitions for implementing complex applications.
– ESLint, on the other hand, aids in identifying and reporting patterns found within ECMAScript/JavaScript code.
– Lastly, the Airbnb Style Guide offers comprehensive coding standards for JavaScript, including TypeScript. When combined with ESLint, this results in powerful optimization and cleanliness check flow for TypeScript codebases.
Here’s a step-by-step guide on utilizing Airbnb’s ESLint configuration with TypeScript:
- Installation: The first step involves installing a number of dependencies required for setting up the project:
- .eslintrc.js Configuration: After installation, you need to set up the ESlint configuration file—typically named ‘.eslintrc.js’. This provides ESLint with rules for checking our TypeScript codes:
- Running ESLint: With the ESLint configuration file in place, we can now use the ‘eslint’ command to check our TypeScript files:
- Fixing Errors Automatically: ESLint will identify problem areas in your TypeScript code. It can, in most cases, fix these issues automatically when you add the ‘–fix’ flag to your command:
npm install -D eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-config-airbnb-typescript
module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint'], extends: ['airbnb-typescript'], }
npx eslint '*/**/*.ts'
npx eslint '*/**/*.ts' --fix
By using ESLint with Airbnb’s TypeScript configurations, you ensure a robust and efficient workflow for handling TypeScript projects. In the words of Jeff Atwood, co-founder of StackOverflow, “The best code is no code at all.” With this setup, we can strive to make our written code as clean, straightforward, and efficient as possible.
Remember that the end goal of implementing ESLint with Airbnb’s configuration in a TypeScript project is to foster code consistency, attain better readability, prevent bugs, and enhance overall code quality. Take the time to understand the nuances of each rule mentioned in the Airbnb Style Guide, as this knowledge will contribute significantly to writing high quality TypeScript code.
Mastering Rules Customization in AirBnB’s ESLint Configuration
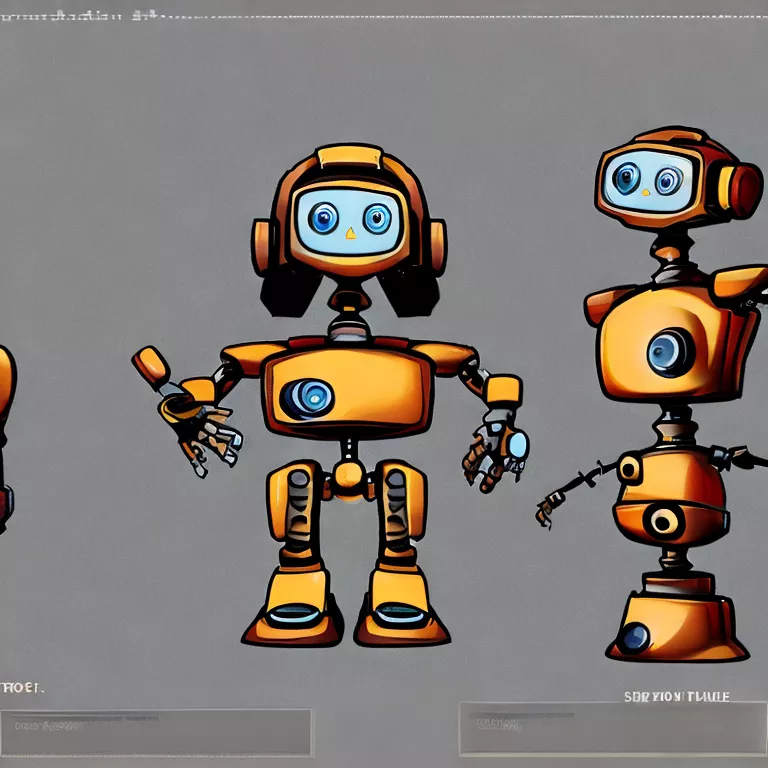
Mastering the rules customization in Airbnb’s ESLint configuration and utilizing it with Typescript, is akin to elevating your coding efficiency and practices up several notches. It’s like hiring a vigilant supervisor who ensures the quality of code keeps exceeding standards, mitigating many potential disasters before they manifest.
Airbnb’s ESLint Configuration provides us a wide range of rules that govern the style and structure of code. As a TypeScript developer—that often involves meticulously perusing thousands of lines of code—a personalized linting rule-set improves both production quality and speed. Utilizing Airbnb’s ESLint configuration:
– Enhances consistency throughout the codebase diminishing friction points
– Uproots potential mistakes and non-optimal code at earlier stages
– Paves for galvanized best-practice adoption among the entire team
Now, let’s delve into how we can use AirBnB’s Eslint settings with TypeScript:
Firstly, install all necessary packages by running the following command:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-config-airbnb-base
Create an .eslintrc.js file in your project’s root directory. Populate the file like so:
module.exports = { parser: '@typescript-eslint/parser', extends: [ 'plugin:@typescript-eslint/recommended', 'airbnb-base', ], rules: { // Place to specify ESLint rules. // Can be used to overwrite rules specified from the extended configs // e.g. "@typescript-eslint/explicit-function-return-type": "off", }, };
The above setup enables you to leverage TypeScript ESLint alongside Airbnb’s base ESLint configuration. Whatever overwrites or customizations are desired for the rules can be made inside the “rules” object in .eslintrc.js; each rule can be turned off, set as a warning, or an error creating a tailored linter that suits your project requirements.
In the well-articulated words of Linus Torvalds, “Talk is cheap. Show me the code,” let’s start linting TypeScript files with Airbnb ESLint.
Saving and then manually calling ESLint by running `eslint ./ –ext .ts`, you can now see any problems arising from the TypeScript code. For daily development use, routines can be improved by integrating this tool within your preferred editor/IDE, building automation scripts, etc.
Though a little learning curve while setting it up, the investment boomerangs back in experiential improvements that evolve with every productive use. With this knowledge, mastering customization rules in Airbnb’s ESLint has become a reachable ideal rather than a hyperbolic target.
Tackling Common Challenges with Eslint Typescript Airbnb Configuration
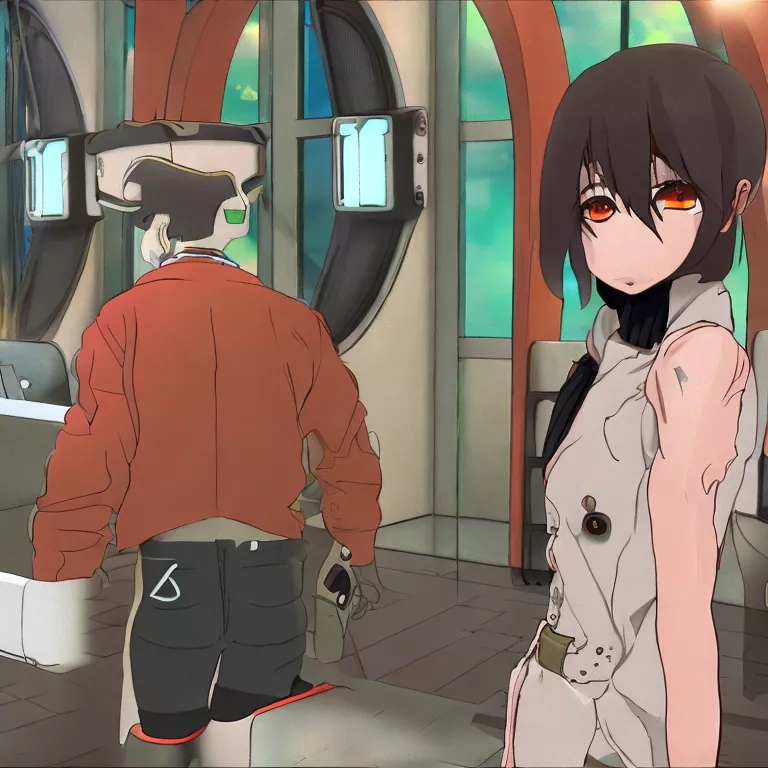
The challenges of configuring ESLint with TypeScript and Airbnb style guide can be overwhelming at first, especially for new developers. However, there are streamlined ways to successfully establish and use this configuration to improve your coding practices and enhance not just code readability but also maintainability.
Let’s start with navigating through the most common issues that might surface during the process:
1. Installing ESLint, TypeScript, and Airbnb Style Guide Correctly
The initial step is ensuring all three components are correctly installed and configured in your project. The installation process runs smoothly when performed in the correct sequence.
npm install eslint --save-dev
npm install @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
npx install-peerdeps --dev eslint-config-airbnb
Following the above steps not only installs the relevant packages but also handles their peer dependencies automatically.
2. Proper Configuration of .eslintrc.js File:
After successful installation, creating and correctly configuring your .eslintrc.js file is crucial for the ESLint to function right.
module.exports = { parser: '@typescript-eslint/parser', // Specifies ESLint parser extends: [ 'plugin:@typescript-eslint/recommended', // Uses the recommended rules from the @typescript-eslint/eslint-plugin 'airbnb-base', // Uses airbnb-base rules ], parserOptions: { ecmaVersion: 2020, // Allows for the parsing of modern ECMAScript features sourceType: 'module', // Allows for the use of imports }, };
Use the above configuration as a starting ground, modifying it according to your specific needs as you move forward.
3. Integrating with IDEs
Finding a setup that works well with your Integrated Development Environment (IDE) can also be a challenge. Most modern IDEs like Visual Studio Code or WebStorm provide an in-built linting support, but to get the most out of ESLint and TypeScript, a proper configuration is paramount.
WebStorm users can refer to this guide, while VS Code users can follow the instructions laid out in this documentation.
4. Resolving Conflicts between ESLint and Airbnb Rules
Sometimes, there might be conflicts between ESLint rules and Airbnb rules because of their different approaches towards certain aspects of coding style and best practices. In such cases, the “rules” section of the .eslintrc.js configuration file can be used to overwrite the default settings.
module.exports = { ..., rules: { 'no-unexpected-multiline': 'error', // Changes this rule's severity to 'error' 'semi': ['warn', 'always'], // Requires semicolons at the end of statements ... }, };
While going through these challenges, remember Linus Torvalds’ words – “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Embrace the process and don’t hesitate to ask for help from the community when needed. Remember, every programming obstacle you overcome strengthens your skills.
Conclusion
Through this discussion, one can ascertain the significance of ESLint in Typescript development, especially when combined with the Airbnb configuration. The overall benefits such as cleaner code, fewer bugs, and adherence to a universal style guide renders it an essential part of any professional developer’s toolkit.
Key Features | Advantages |
---|---|
Code Consistency | Leveraging ESLint with Airbnb Configuration allows for consistent code architecture across multiple projects which is beneficial in collaborative settings. |
Bug Prevention | ESLint identifies potential errors by analyzing code patterns. This contributes significantly towards reducing instances of bugs in applications. |
Enhanced Code Quality | The stringent rules enforced by the Airbnb style guide equipped with ESLint helps developers to maintain quality and readability in code. |