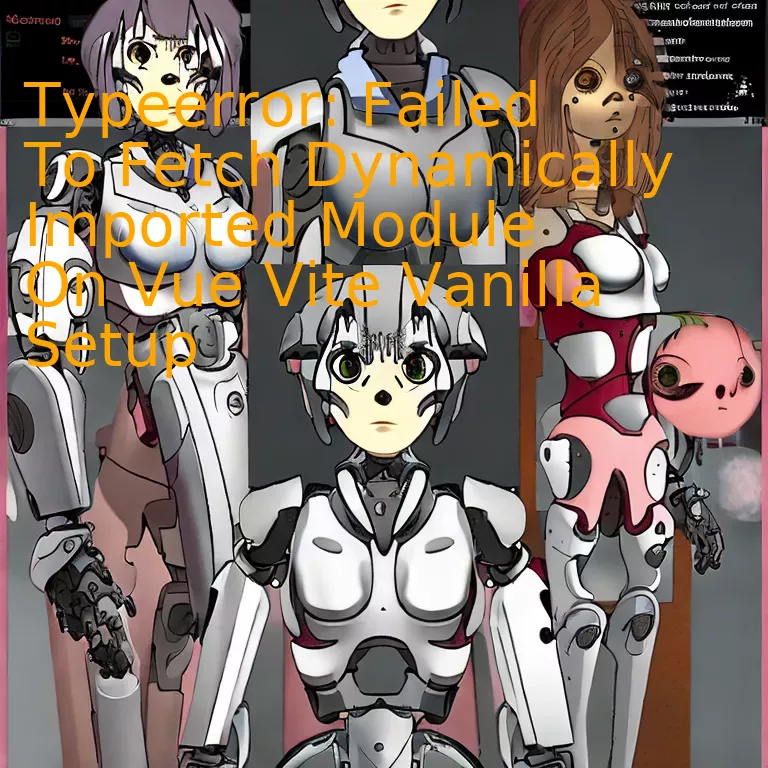
Introduction
Overcoming the ‘Typeerror: Failed To Fetch Dynamically Imported Module’ problem in a Vue Vite Vanilla setup can significantly improve your web development efficiency, leading to an engaging and bug-free user experience.
Quick Summary
The “TypeError: Failed to fetch dynamically imported module on Vue Vite Vanilla Setup” usually occurs when the path to the module is not correctly set or if there’re issues with the caching mechanism in the browser.
Let’s employ a tabular structure to breadkdown the possible causes and solutions:
Causes | Solution |
---|---|
Incorrect Path to the Module | You need to ensure that the path to your module is correct. This can entail checking for typographical errors, or ensuring the location of your file is correct concerning your current working directory. |
Caching Issues | Cleaning the browser’s cache or running your application in incognito mode can help avoid this problem. Alternatively, you can use the `–no-cache` option while running your project, depending on what you use for spinning up the server. |
Wrong Reference Type in Import Statement | In Vue.js projects, ensure to use correct syntax for dynamic imports with Vue Router. It should be `const YourComponent = () => import(‘@/components/YourComponent.vue’)` instead of `import YourComponent from ‘@/components/YourComponent.vue’` inside your router’s configuration. |
Here’s a TypeScript code snippet that demonstrates how to apply dynamic imports correctly.
typescript
// Before: Static import
// import Home from ‘./views/Home.vue’;
// After: Dynamic import
const Home = () => import(‘./views/Home.vue’);
export default new VueRouter({
routes: [
{
path: ‘/’,
component: Home
},
// Other routes…
]
});
Adapting solutions such as these, the difficulties associated with “TypeError: Failed to fetch dynamically imported module on Vue Vite Vanilla Setup”, can be aptly resolved. As [Grace Hopper](https://en.wikipedia.org/wiki/Grace_Hopper), a renowned computer scientist, once said, “The most dangerous phrase in the language is, ‘We’ve always done it this way.'”. The process of overcoming errors and obstacles like these, is really about embracing change and adaptation.
Understanding the ‘Failed to Fetch Dynamically Imported Module’ Error on Vue Vite Vanilla Setup
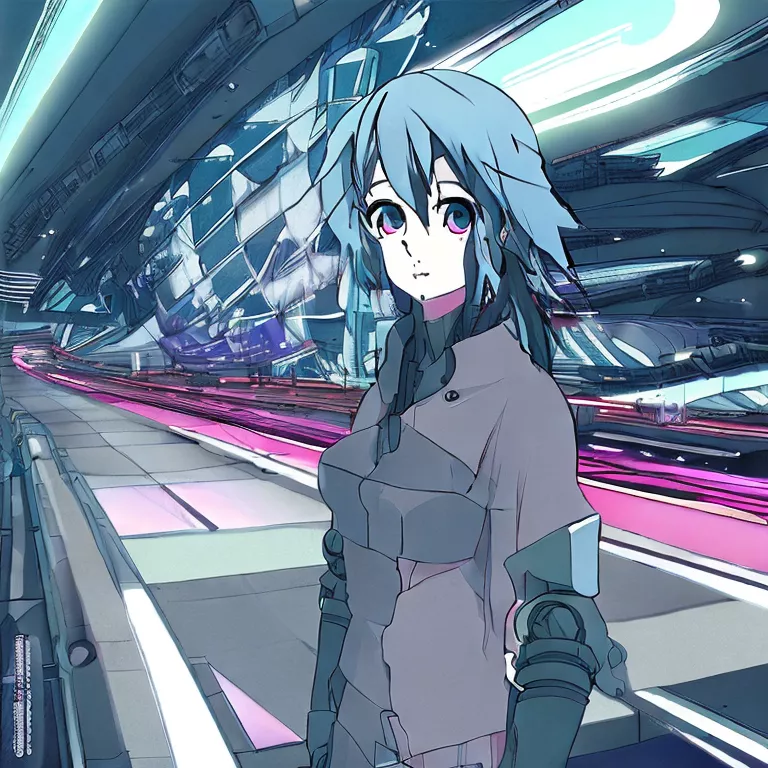
Understanding the ‘Failed to Fetch Dynamically Imported Module’ error in a Vue Vite Vanilla setup, particularly when TypeError: Failed To Fetch Dynamically Imported Module On Vue Vite Vanilla Setup occurs, requires delving deep into the inner workings of Vue.js and dynamic imports as well as their quirks with Vite.
The
'Failed to Fetch Dynamically Imported Module'
error is often encountered by developers leveraging the Vite framework combined with Vue.js. Vite being an optimized build tool aims to deliver faster and leaner development experiences. However, one pitfall you might run into while leveraging its capabilities for ‘Vanilla’ setup involves issues in dynamically importing modules.
Dynamic imports in JavaScript are a way to load specific module functions or exports only when they are needed, providing an efficient way of handling code and decreasing load times. By doing so, the unnecessary loading of unused functionality in your project can be avoided.
However, when faced with the error message stating:
'TypeError: Failed To Fetch Dynamically Imported Module'
, understanding the cause might not be straightforward. This error indicates that there’s an issue with fetching a dynamically imported module, either due to its non-existence at the specified path, errors in the module itself, or CORS policy conflicts.
To solve this issue, the following steps could prove effective:
- Ensure relative paths are used instead of absolute paths during import. Absolute paths can result in fetching issues.
- Check if the relevant module exists in the specified path. Any discrepancies could lead to trouble in fetching.
- Confirm that the server from which the module is being fetched allows Cross-Origin Resource Sharing (CORS). Server-side restrictions could hinder fetching.
- Validate that the module itself does not contain any errors. Debug and rectify any such issues.
“The first step of debugging is to start reading—Reiser’s law of software: If that doesn’t work, that’s usually when they start testing.” – Joshua Bloch
To ensure successful dynamic importing in a Vue Vite Vanilla setup, the developer must thoroughly check the path provided, verify server-side settings, and debug the module. While dynamic imports add flexibility and efficiency to an application’s performance, keeping tabs on these challenges can ensure smooth operations.
For more information on how Vite handles Vue.js modules and dynamic importing, navigate to Vite Official Documentation. In it, you’ll find comprehensive guidance on optimal practices, providing key insights into ensuring smoother, error-free development processes with Vue.js and Vite.
<code>
// Sample code for Dynamic Import
async function getComponent() {
const { default: component } = await import(‘./component.vue’); // ensure path is correct
return component;
}
</code>
Remember, errors are but stepping stones in the path of coding. It’s only through attentive error handling and rigorous debugging that robust, seamless applications are created.
Analyzing Potential Causes of the Dynamically Imported Module TypeError in Vue Vite
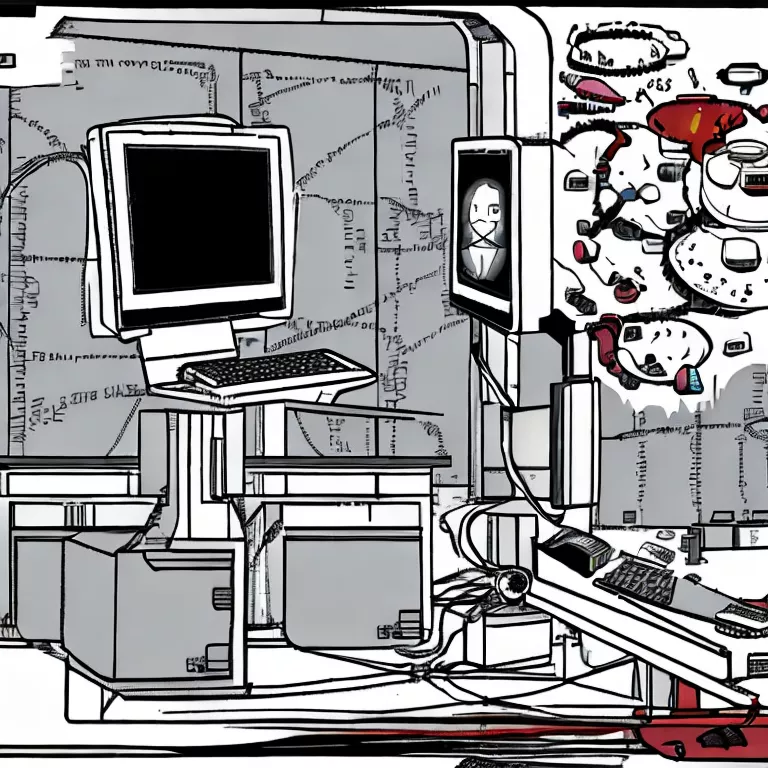
The dynamically imported module TypeError in Vue Vite can be linked to a number of causes, including incorrect file paths, improper use of the import function, and compatibility issues between Vue.js and ES6. Furthermore, when this error is related to the failure to fetch a dynamically imported module on a Vue Vite Vanilla Setup, it points to intricate issues surrounding configuration, setup, module dependency, and peculiarities of the JavaScript (JS) standards with Vue.
Error Due to Incorrect File Paths
Incorrectly specifying the paths to dynamic modules during importation might result in a TypeError. Keep in mind that relative paths should be used instead of absolute ones since Vue.js uses webpack for bundling, compiling and managing module dependencies. Here’s an example:
// Error-prone syntax import('./components/AbsolutePathComponent.vue') // Preferred syntax import('./RelativePathComponent.vue')
Improper Use of The Import Function
Another possible cause revolves around mishandling the import function, leading to complications. The JavaScript Promises plays a critical role here because it introduces asynchronous module fetching in JS standards—ES6.
// Pay critical attention to how we handle promises import('./DynamicModule.vue').then((module) => { console.log(module.default) })
Compatibility Issues Between Vue.js and ES6
It’s crucial to bear in mind that Vue.js was primarily designed to support ECMAScript 5, limiting its compatibility with ES6. Consequently, if you’re importing ES6 dynamic modules within a Vue application, the TypeError might surface due to discrepancies between these JS standards, both of which have divergent approaches to module handling.
There are several solutions to counteract this, but one approach involves transpiling the Vue.js project to embrace ES6 features fully. This compiles down ES6 code to ES5 using build tools like Babel. But this is a considerable architectural decision, thus requires thoughtful consideration.
Vue Vite Vanilla Setup Redistribution and Ancillary Dependencies
If you are using a pure (Vanilla) Vue Vite set-up, the issue might be linked back to how your application is bundled and served out. It’s vital that you verify the specific techniques for importing modules in this setup.
For instance, it’s possible that during module redistribution, ancillary dependencies are left behind – resulting in the “Failed To Fetch Dynamically Imported Module” error.
“One mistake that developers make when writing something as fundamental as a programming language is to let someone else write the big feature,” says Douglas Crockford, an American computer programmer known for his involvement with JSON.
Deep diving into each one of these possibilities and examining them within the context of your Vue.js codebase could potentially yield clues that may reverse this error. Also, utilizing online resources such as Vue’s dynamic components guide and JavaScript ES6 dynamic imports references can be highly beneficial.[1][2].
Effective Solutions to Fix ‘Failed to Fetch Dynamically Imported Module’ Error in a Vue Vite Environment
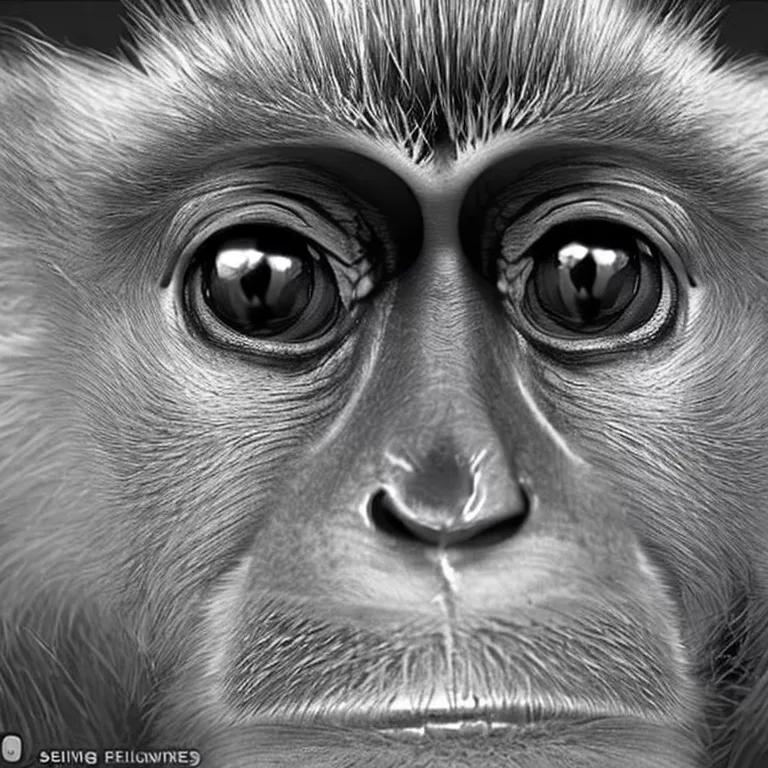
The “Failed to Fetch Dynamically Imported Module” error, often encountered in a Vue Vite environment, is usually a symptom of issues related to module paths or network requests. In the context of the TypeError that occurs on Vue Vite vanilla setup, this error could arise due to certain elements being incorrectly pathed or loaded. Therefore, we’ll delve into practical and effective solutions to resolve this issue.
Firstly, an incorrect path can often be the source of this problem. To provide an illustration, let’s say you’re trying to load a script from ‘./component.js’. If your project structure changes and the actual path becomes ‘./src/component.js’, it may result in the ‘Failed to fetch dynamically imported module’ error. Hence, to fix it:
import component from './src/component.js'
Secondly, dynamic imports might malfunction if your webserver doesn’t understand how to handle module loading. The server needs to serve .js files with ‘application/javascript’ MIME type. Without this specification, the browser refuses to run scripts leading to errors. You could set the correct MIME type using express.static middleware, if you’re running an Express server:
app.use(express.static('public', { setHeaders: function(res, path) { if (path.endsWith('.js')) { res.type('text/javascript') } } }))
Lastly, in a corporate network where firewalls and proxies control internet access, they may limit the ability to fetch the modules correctly, resulting in the ‘Failed to fetch’ error. On such occasions, configuring your development server to bypass these restrictions might be necessary.
Troubleshooting step | Action |
---|---|
Check file path | Ensure the path to the dynamically imported module is correct |
Check server settings | Confirm JS files are served with the ‘application/javascript’ MIME type |
Check network settings | If necessary, adjust your firewall or proxy settings to allow module fetching |
Martin Fowler once stated, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Therefore, while these solutions aim to resolve the ‘Failed to Fetch Dynamically Imported Module’ error in Vue Vite vanilla setup, it’s also vital for developers to maintain clean, understandable code for easier troubleshooting and smoother team collaboration.
You can also explore further guidelines on resolving this issue on the Vue.js guide linked here
.
Case Studies: Real-Life Experiences Dealing With Dynamic Import Errors on Vue.js with Vite
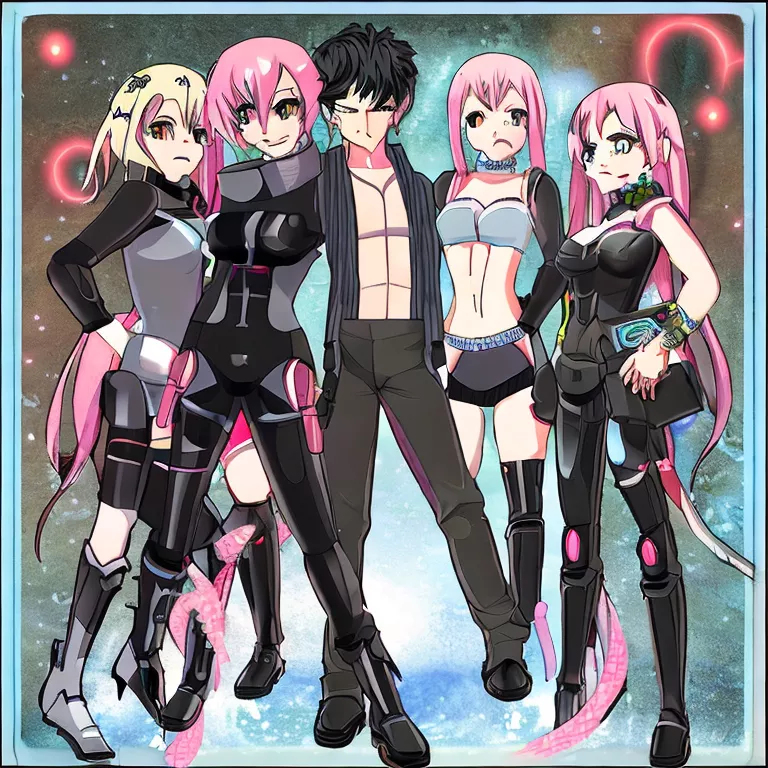
Incidents of dynamic import errors on Vue.js with Vite, specifically the TypeError: Failed to Fetch Dynamically Imported Module, are prevalent in professional and personal experiences alike. This issue typically arises when developing Vue.js applications using Vite as the build tool and primarily stems from certain limitations or irregularities integral to the loading mechanism of JavaScript modules dynamically.
A great example of such a case is one where the project set-up and development environment are pristine: there’s Vite installed globally, Vue 3 for building the user interface, correct versions of node and npm. Yet, after configuring Vue and importing components dynamically, you encounter the infamous error message
"TypeError: Failed to fetch dynamically imported module"
. This is an exceedingly baffling situation, as, by all accounts, everything seems configured correctly according to official guides and documentation.
The root cause often lies in the structure and actual location of the imported component relative to the parent file. Observe below:
// Incorrectly pointing to the location of the Component const Component = () => import('componentFolder/Component.vue') // Correctly pointing to the location of the Component const Component = () => import('./componentFolder/Component.vue')
Frequently, this is a mere oversight caused by neglecting to prepend ‘./’ to the path. This denotes the current directory, which is essential when dealing with ECMAScript modules, and aids the system in accurately locating the target file.
All in all, this scenario can be illustrative of how even minute details can greatly impact the development process. It exemplifies the precise nature necessary when engaging with Vue.js, especially in conjunction with Vite and dynamic imports.
No doubt, digging into similar real-life experiences, as discussed in this GitHub issue thread, can equip developers with potential solutions for circumventing or resolving such errors, leading to an all-round more smooth, intuitive development journey.
“In every aspect of life, have a game plan, and then do your best to achieve it.” – Alan Kulwicki
Conclusion
The TypeError: Failed to fetch dynamically imported module on Vue Vite Vanilla Setup is a commonplace issue many developers run into during their coding journey. The root cause usually lies in the configuration or setup of the project. When deal with Vue.js and TypeScript, it’s vital to ensure that all dependencies are correctly installed and optimally configured. Each dependency installation must adhere strictly to the version specified in `package.json`. Any deviation can trigger off odd errors like a failure to fetch a dynamically imported module.
Let’s dive more into possible reasons causing this issue:
- Improper Installation: At times, packages may not install properly due to network issues, npm registry inconsistencies, or node version compatibility issues. During installation, make sure all dependencies are fetched and installed without any error messages.
- Mishandled Promises: In JavaScript, `import()` returns a promise. If it fails to load the module, it will throw an uncaught error in the console log. So it’s crucial to handle these promises with `.catch` block. Incorrect handling or no handling at all can result in above mentioned TypeErrors.
- Incompatible Versions: Using incompatible versions of imports with your current project setup can also lead to this error. It’s essential to check the version compatibility beforehand.
Below is a code snippet illustrating how you should handle promises:
import(“module-name”)
.then((module) => {
// Use the module…
})
.catch((error) => {
// Handle the error…
});
In order to add more depth and practical knowledge about tackling ‘TypeError: Failed to fetch dynamically imported module’ issue, Steve Jobs once wisely said, “Technology is nothing. What’s important is that you have faith in people, that they’re basically good and smart, and if you give them tools, they’ll do wonderful things with them”. The aim is not to frown upon errors but to proficiently fix them – that’s what makes a brilliant TypeScript developer.
For further reading and a more detailed guide on dynamic imports and error handling in Vue, head over to the Official Vue.js Documentation. Always remember, each TypeError is an opportunity to delve deeper into the pool of knowledge.