Introduction
In dealing with Angular 9, a common complication that developers encounter is when ngcc fails due to an unhandled exception, which requires proactive troubleshooting and a deep understanding of the framework.
Quick Summary
Version | Problem | Solution |
---|---|---|
Angular 9 | Ngcc fails, culminating in an unhandled exception error message. | The ngcc issue is typically prompted by a problematic configuration or compatibility issue, which can often be solved using a combination of updating packages, adjusting the tsconfig.json file and revising scripts in the package.json file. |
The information displayed suggests that Angular 9 users could encounter errors stemming from the Angular compiler (ngcc). When this occurs, it manifests as a stumbling block that hinders progress, and presents as an unhandled exception error message – a circumstance that many developers might stumble upon.
Diving deeper, one key cause is rooted in a misconfiguration or an incompatibility issue. This could mean there is something awry within the dependencies being utilized by the developers or, at times, the tsconfig.json file contains some settings that are incompatible with Angular 9. This snares the Angular compiler into the error in question and disrupts smooth sailing.
In such contexts, software engineers can resort to several viable strategies aimed at confronting the error. These entail actions revolving around updating all the individual packages in the project, including both development and production dependencies. An up-to-date package environment minimizes potential for conflicts and inconsistencies that could trigger the aforementioned problem.
Secondly, adjustments to the project’s tsconfig.json file may also be necessary. Alterations to certain compiler options could resolve incompatible elements that precipitate the error. Finally, revising scripts found in the package.json file plays a crucial role in bridging any gaps causing the ngcc failure. Often, these scripts call for ngcc to execute tasks that it inadvertently stumbles on, culminating in the unhandled exception.
As [Nick De Cooman](https://twitter.com/nickdecooman/status/1148942953296039938?lang=en), a renowned software engineer once quipped, “Debugging is like being the detective in a crime movie where you are also the murderer.” This quote encapsulates the detective work needed when debugging. Given Angular 9’s open-source nature, complexities arise inevitably, and that necessitates developers to adapt a problem-solving mindset grounded in persistence and ingenuity.
Understanding the Ngcc Error in Angular 9
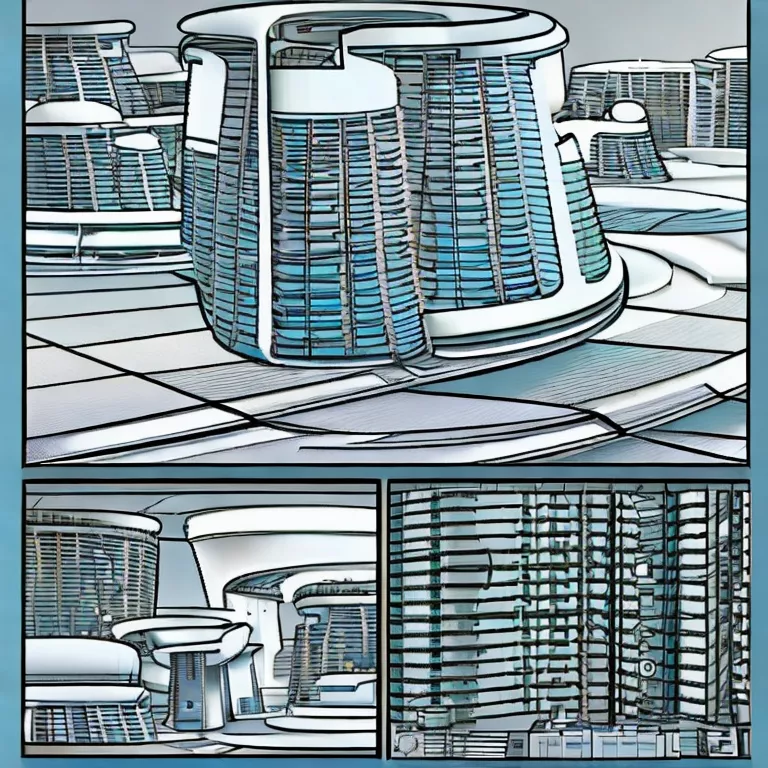
The Angular 9 Ngcc failure with an unhandled exception occurs due to a conflict in package compatibility. The Angular compatibility compiler (ngcc) is prone to encounter errors, especially when working on a project that has been migrated from earlier versions of Angular.
Let’s look at the factors and potential solutions:
Error Source Identifiers:
- Ngcc is unable to derive metadata for a non-Angular package. It can also occur if third-party libraries are incompatible with Ivy.
- Incorrect Node modules installation could cause discrepancies leading to ngcc failure.
Solutions:
- Re-installation of node-modules: Deleting the node_modules folder and executing
x
npm install
may resolve the issue.
- Use of compatible packages: Replace the current package with one that is compatible with Angular and adheres to the Angular Package Format (APF).
Since the error might arise out of a myriad of situations, it is crucial to understand the specifics of your project before applying the solution.
Error Scenario | Description | Potential Solution |
---|---|---|
Metadata error | This error occurs when ngcc encounters a non-Angular package. | Replace or remove the conflicting package. |
Node modules installation error | The node_modules folder itself is incorrect or incomplete. | Delete node_modules folder and run
xxxxxxxxxx npm install . |
Keeping your Angular development practices up-to-date and following good package management principles will reduce such issues significantly. Remember, as Kent Beck states: “Optimize later – first make it work, then make it right, and finally make it fast.” You should utilize this principle as a guidepost when handling unhandled exceptions in Angular 9 for ngcc.
References:
Angular Ivy,
Angular Ngcc
Troubleshooting Steps for Unhandled Exception Errors
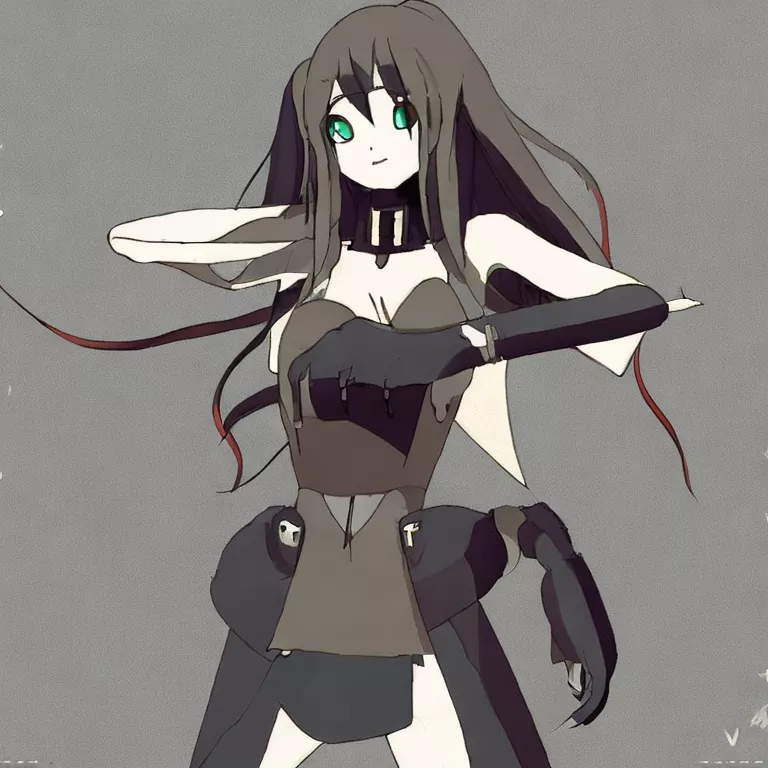
Troubleshooting “Unhandled Exception” errors in Angular 9, especially those related to Ngcc failure can be an arduous task. However, thanks to the vast Angular community and extensive documentation, we have been able to map out some effective steps that can be employed to address these problems efficiently.
To understand better, first, it’s necessary to know what ngcc is. The Angular Compatibility Compiler or ngcc is a tool that makes your node_modules compatible with the Ivy compiler. It comes by default with Angular 9 and helps you execute applications faster.
Step | Description |
---|---|
xxxxxxxxxx Clean install |
The first step to troubleshooting is ensuring all your installations are correct. Often, corruption or overlapping versions might cause an unhandled exception. Running
xxxxxxxxxx npm clean-install can help ensure everything is installed as expected. |
xxxxxxxxxx Delete node_modules |
If you still encounter issues, delete your entire node_modules directory by using OS-specific commands or manually and reinstall your packages via
xxxxxxxxxx npm install . Clearing cached data often solves many unexpected error issues. |
xxxxxxxxxx Upgrade or Downgrade |
Bugs can be version-specific. If certain versions of Angular or other third-party libraries have well-documented problems leading to an unhandled exception, considering upgrading or even downgrading could be helpful. |
xxxxxxxxxx Check Dependencies |
Examining dependencies is crucial in resolving the issue. Look out for unmet or conflicting dependencies and resolve them. |
xxxxxxxxxx Error Logs |
Angular creates comprehensive logs upon encountering errors. Having a careful glance at it can give insights into the line of code causing the issue. |
xxxxxxxxxx Check Online Resources |
Official Angular documentation, StackOverflow, GitHub or other coding forums might have relevant solutions for your unhandled exception error. |
As Grady Booch rightly said, “The function of good software is to make the complex appear simple”. Applying these steps could certainly assist in troubleshooting the unhandled exception errors related to NgCC in Angular 9 and bring back the simplicity that we yearn for in our code.
Addressing Common Issues with Ngcc in Angular 9
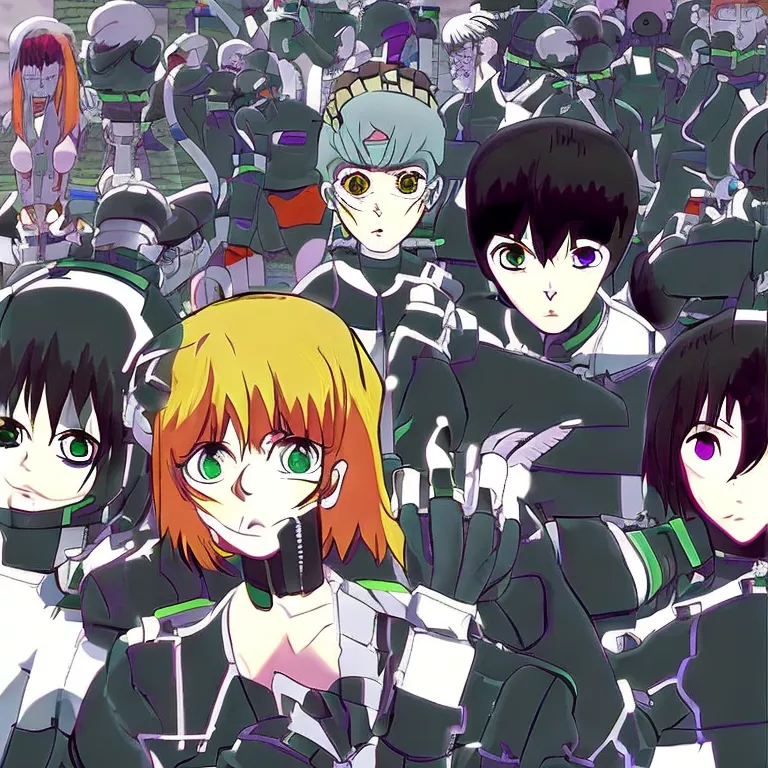
When working with Angular 9, you may sometimes encounter an issue where the Angular Compiler (ngcc) fails due to an unhandled exception. This problem can affect your development workflow significantly and can stem from several reasons such as conflicts with node modules, incorrect configuration, or compatibility issues.
Let’s break down some common causes of this issue and their respective solutions:
Issue With Node Modules
One probable reason for ngcc failing could be a conflict between node modules, especially if they were not installed correctly or are incompatible with Angular 9.
The solution is to remove the node_modules folder and reinstall it by running the following commands in your terminal:
xxxxxxxxxx
//Delete node_module folder
rm -rf node_modules
//Reinstall node_modules
npm install
Remember to stop your server before performing these actions, and then restart it once you’re finished.
Misconfiguration in Angular
Incorrect configurations are another reason that might cause the ngcc to fail. When setting up Angular, pay close attention to ensure each aspect is correctly set up. For example, check your “tsconfig.json” file and compare it with the official Angular guide to assure it’s correctly configured.
Compatibility Issues
Ngcc also tends to fail when there are compatibility issues between different versions of Angular or with non-Angular libraries. To overcome this, use compatible versions of libraries and Angular itself. Information about compatibility can usually be found in the documentation of each library.
As Martin Fowler, renowned software developer and author, once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Let’s remember this sentiment when debugging errors such as these, patiently tracing the cause of the error and resolving it in a way that makes the code clear and understandable.
If none of the above-listed solutions works, you may need to dig a little deeper. It might be worth checking the version of Angular in use, the scripts running in “package.json” or potentially even considering the migration to a newer Angular version if compatibility becomes an issue. Remember: software development is as much about problem-solving as it is about coding, so a patient and methodical approach will often yield results.
Implementing Best Practices to Avoid Fails With An Unhandled Exception
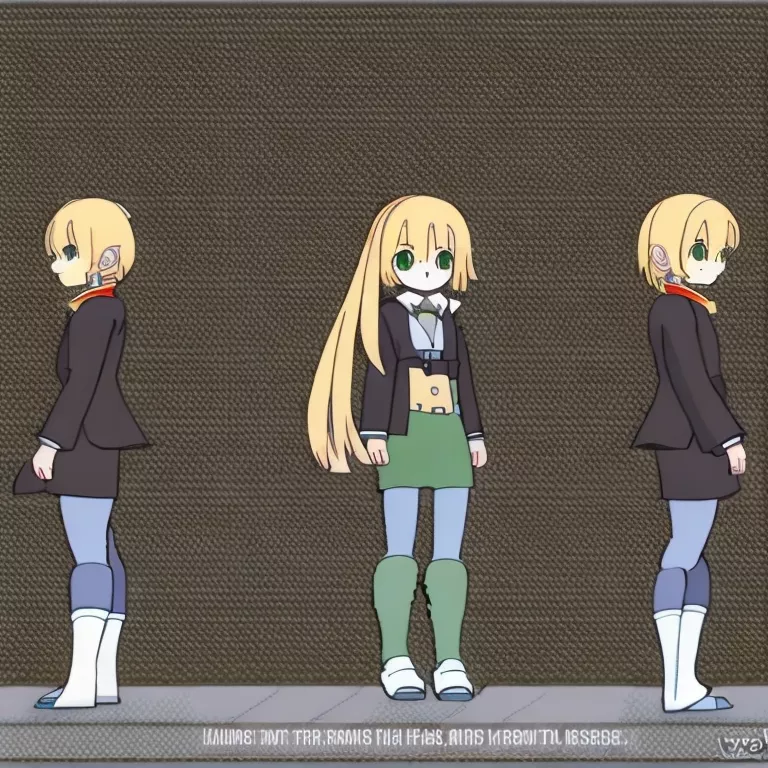
Handling exceptions effectively is a critical aspect of coding, and Angular 9 is no exception. When we’re dealing with the ‘Ngcc Fails With An Unhandled Exception’ error specifically in Angular 9, there are a few tried and tested best practices to follow.
Exception handling:
An ‘Unhandled Exception’ suggests an unexpected occurrence within the code that could have been intercepted or handled but was not detected in any try/catch block. This could potentially lead to an application crash if left unresolved.
Angular comes with its own Error Handling mechanism called ErrorHandler class.
A code snippet exemplifying how this can be used is shown below:
xxxxxxxxxx
export class AppErrorHandler implements ErrorHandler {
handleError(error){
//custom error handling logic goes here
}
}
Angular’s error handling philosophy encourages developers to write safer code by making potential pitfalls and unexpected outcomes apparent.
Meticulous Dependency Checks:
For ‘Ngcc Fails With An Unhandled Exception’, it is necessary to thoroughly ensure that all dependencies are up-to-date, as outdated packages could lead to these types of unhandled exceptions. Type ‘ng update’ in your terminal to automatically update all your angular dependencies.
Clean Reinstall:
It is also recommended to try a clean reinstall in some cases where npm packages might be corrupt or incorrectly installed. The process involves deleting your node_modules folder, package-lock.json file and then running ‘npm install’.
Ensure Correct Angular Compiler Version:
Sometimes, this kind of issue can arise when the Angular Compiler (ngcc) version clashes. Your compiler versions need to be in line with that of Angular’s, thereby confirming that every library and Angular are using the same TypeScript version.
As Brian Kernighan, a notable computer scientist once said, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” This insightful quote highlights why following best practices is crucial in avoiding unhandled exceptions. The above steps, therefore, provide an outline for effective error handling and prevention of failures with Angular 9’s ‘Ngcc Fails With An Unhandled Exception’.
Conclusion
Understanding the Angular 9 NGCC failure is crucial for any TypeScript developer. This exclusive error prompts when NGCC compilation process doesn’t come off as planned. It is a non-traditional obstacle that asks for our precise attention and comprehension.
Untreated exceptions, especially in a robust framework like Angular, often signify an underlying issue in your codebase or system configurations that requires immediate rectification. They break the standard mechanism by inducing unexpected behavior often leading towards application failure. Identifying the source of such exceptions can be complex, but close examination of error messages, log files & software documentation invariably provides key insights into resolution approaches.
When encountering
xxxxxxxxxx
Angular 9 - Ngcc fails with an unhandled exception
, one might need to delve into several possible solutions:
- Reinstalling node modules: Dependencies can get corrupt. Wiping out ‘node_modules’ directory & reinstalling dependencies often helps.
- Checking System environments: Sometimes, settings at the system level can cause these exceptions. A thorough check-up on the same is recommended.
- Enhancing Typescript Version: Higher versions of Typescript typically solve many known bugs.
- Altering Angular Compiler Options: At times, adjusting the ‘angularCompilerOptions’ setting within tsconfig.json can resolve this complication.
Being well-versed with effective debugging strategies and having a solid understanding of Angular’s architectural patterns can significantly help to rapidly address these unhandled exceptions thereby saving precious time and resources. As Alan Turing rightly said, “We can only see a short distance ahead, but we can see plenty there that needs to be done.”
It might also serve useful to follow discussions on the official Angular Github repository. Many developers share their firsthand encounter with similar issues and contribute solutions that have helped them successfully tackle such complications in the Angular ecosystem.
Furthermore, consulting official Angular documentation and enrolling in online educational resources like Udemy or Pluralsight courses that shed significant light upon complex topics such as Angular NGCC can be a powerful weapon to turn any roadblock into a learning opportunity.