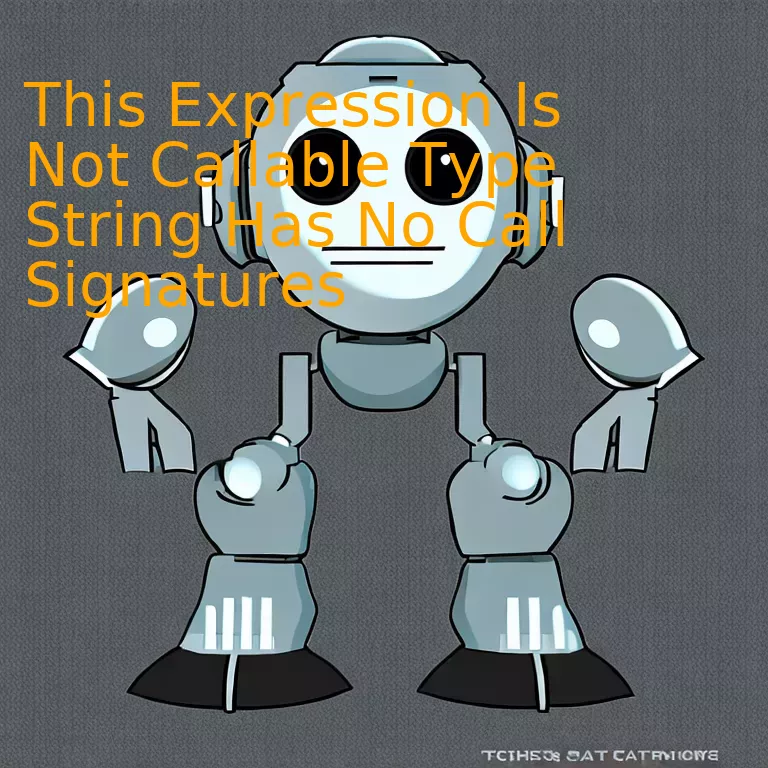
Introduction
While resolving coding issues, it’s vital to note a common message often displayed, hinting at ‘This expression is not callable type string has no call signatures’. This phrase essentially explains that in the programming realm, you are trying to invoke a function on a value that isn’t function type, often a string, which isn’t designed to operate with call signatures. Notably, this occurrence often instructs developers to redefine or inspect their syntax for precise code execution.
Quick Summary
This specific TypeScript error, “This Expression Is Not Callable Type String Has No Call Signatures”, is common among TypeScript developers. It is triggered when a function call is attempted on something that’s not recognizable as a function by TypeScript.
To understand the error better, here’s how it relates to some essential aspects of TypeScript:
Aspect | How It Relates to Error Message |
TypeScript | TypeScript is a statically typed language and this aids in catching errors during the development stage rather than bugs at runtime. |
Static Typing | ‘Type String Has No Call Signatures’ indicates a static typing issue related to “String”. The static typing makes sure that a defined variable aligns with its type and usage intentions. |
Correct Usage | The ‘not callable’ part refers to TypeScript recognizing ‘String’ as an object but not a function; hence, it cannot be called or executed like a function. |
Analyzing the reason for this error message coming up, one would commonly encounter it when trying to use parentheses following a string value, which subtly implies the intention to invoke a function call, i.e., `myVariable()`. Since ‘myVariable’ is recognized as string in TypeScript and strings don’t have call signatures – meaning they can’t be called as functions – it results in an error.
For instance, consider the code below:
let myVariable: string;
myVariable = "Hello, World!";
myVariable(); // This will raise error: This expression is not callable. Type 'String' has no call Signatures.
In this given case, you’re trying to call `myVariable()` as a function when it’s a string. To fix this error, it’s paramount to ensure the correct usage of assigned values and their types.
As per the wise words of well-renowned computer scientist Tony Hoare, who invented Null references: “I call it my billion-dollar mistake… At that time, I was designing the first comprehensive type system for references in an object-oriented language (ALGOL W). My goal was to ensure that all use of references should be absolutely safe, with checking performed automatically by the compiler.”
This quote captures the essential idea behind diligent type-checking in TypeScript, thus preventing such type-related runtime errors effectively.
Understanding The Error: Expression Is Not Callable Type String Has No Call Signatures
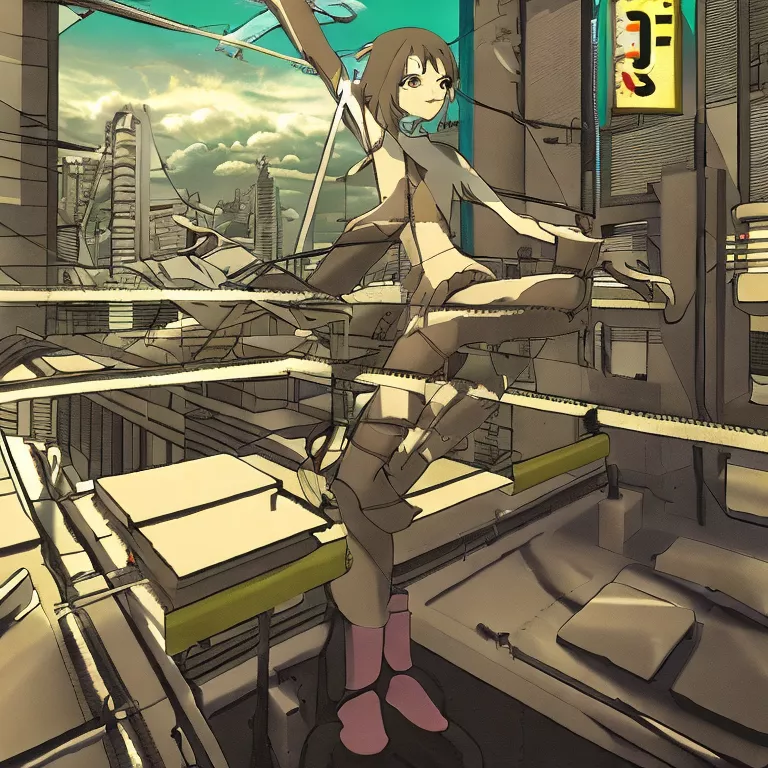
The error message, “Expression is not callable. Type ‘String’ has no call signatures”, is typically encountered in TypeScript when trying to invoke or ‘call’ a string as though it were a function. Understanding this error and how to address it requires an understanding of essential TypeScript concepts such as ‘types’, ‘signatures’, and the behavior of particular objects within JavaScript.
In TypeScript, everything has a type. Every variable, function, class – they all have types that TypeScript uses to understand what operations are permissible. The String type, like other standard JavaScript Objects, comes with a set of associated functions (methods) that can be called on strings, but the string itself isn’t a function and can’t be invoked like one. Attempting to do so will produce the aforementioned error.
Call signatures refer to the parameters and return types that a function expects. As an analogy, think of these signatures as real-world signatures – they show who created a document (the function), and by extension, hint at its purpose. Trying to call a string indicates to TypeScript that you’re treating the string like a function, but since strings don’t have callable signatures, expect to see an error.
Here’s a brief example:
typescript
let myVariable: string;
myVariable = “Hello World”;
myVariable(); // This will throw an error
This simple TypeScript code illustrates the issue. We declare a variable `myVariable` as type string, then try calling `myVariable` as if it was a function, which it’s not. Hence, TypeScript throws the aforementioned error.
As SitePoint explains, to correctly use the methods available for strings in JavaScript, the syntax should look as follows:
typescript
let myVariable: string;
myVariable = “Hello World”;
console.log(myVariable.toUpperCase()); // This would print “HELLO WORLD”
Here, `toUpperCase` is a valid method on string objects in JavaScript and therefore doesn’t generate an error.
In the insightful words of Jim Zemlin, executive director at Linux Foundation: “Understanding technology has become the key to economic opportunity in this digital age.” This applies aptly even to seemingly intricate TypeScript errors and their resolutions. A deep understanding of TypeScript’s type system can help you handle and prevent such errors effectively, leading to more robust and error-free code.
Decoding the Causes Behind “Expression is not callable, type string has no call signatures”
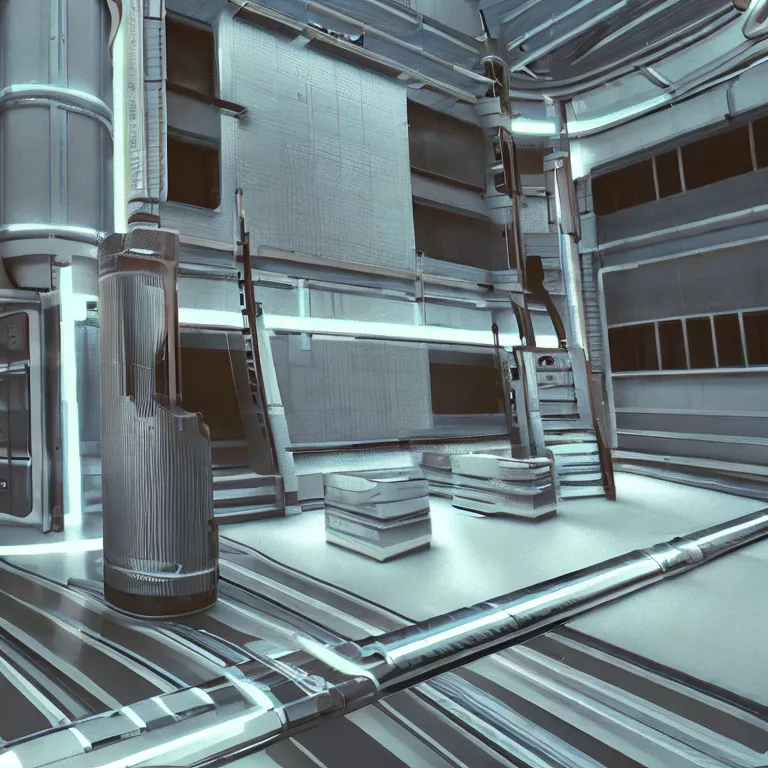
The message “Expression is not callable, Type ‘string’ has no call signatures” is a common error encountered when working with TypeScript. It often leads to confusion among developers as it seemingly occurs suddenly and without any clear reason. To dissect this issue, we will consider the root causes behind it, and propose proper solutions.
This complication primarily arises due to the misinterpretation or erroneous programming of strings as functional constructs. When a string type is invoked like a function, TypeScript throws this error because it does not correlate strings with function calls.
To illustrate this, consider the following piece of code:
typescript
let test: string;
test();
In this example, you are trying to treat ‘test’ which is of type ‘string’, as a function. Hence TypeScript flags an error.
To resolve such errors:
- You need to first identify the variable that’s causing the issue.
- Check where and how this variable is being instantiated. If you are attempting to use a string as a function, TypeScript would rightly raise this objection.
To apply these steps, let’s correct the previous code snippet:
typescript
let test: string = “Hello”;
console.log(test);
In this edited code, TypeScript doesn’t raise any ‘Expression is not Callable’ error because you are treating ‘test’ as a string, which aligns perfectly with its declared type.
As Robert C. Martin, author of Clean Code, aptly said: “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write.” This encapsulates efficiently how identifying the variables used improperly can prevent such issues from happening.
Fundamentally, TypeScript enforces type safety to maintain code quality. Developers should understand and exploit TypeScript’s static typing feature to write unambiguous and robust code. Proper use of variables according to their types manages data effectively, enhances code readability, and ultimately reduces bugs in your code.
Practical Solutions to Fixing the Expression Not Callable error in JavaScript
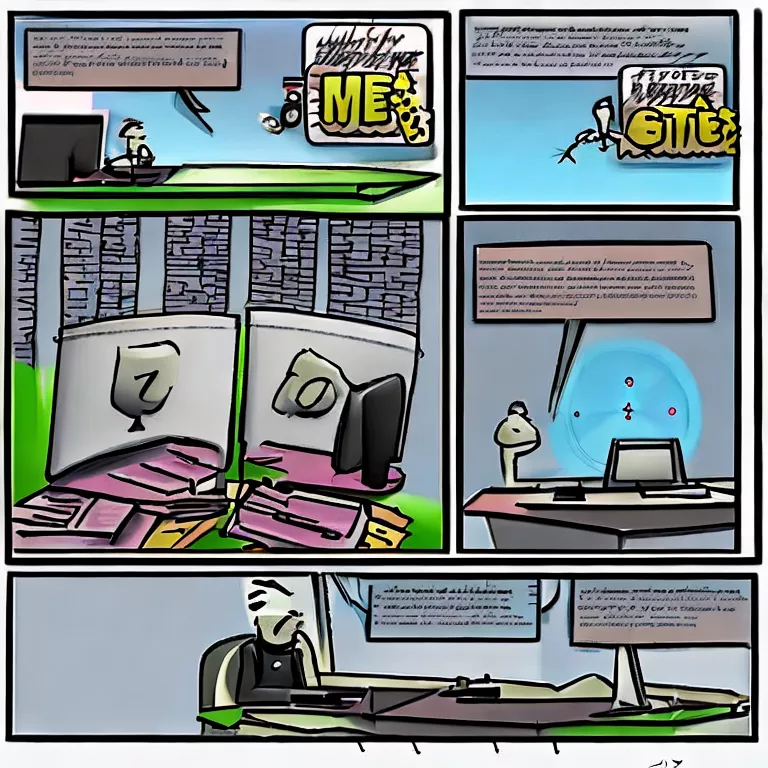
The ‘Expression Not Callable’ error in JavaScript is commonly encountered when attempting to invoke a function which is not defined or when a non-function variable is invoked as if it were a function. Specifically, the ‘This Expression Is Not Callable Type String Has No Call Signatures’ occurs while using TypeScript and you are trying to call a string as if it were a function, thus violating static typing rules of TypeScript.
Understanding the Error
Before solving an issue, it’s crucial to understand its cause. Conclusively put, in the realm of TypeScript, each entity has a specific type or types, marking out characteristics and capabilities. For instance, numbers can be added or multiplied, whereas strings can be concatenated. If your application attempts to treat a string like a function, TypeScript will rightfully signal an error because this action is disjoint; strings lack the fitting ‘call signatures’ to operate like a function..
xxxxxxxxxx
let str: string = "I'm a string, not a function";
str(); // This would trigger the 'This expression is not callable' error
Solution Approaches:
1. Type Checking: To avoid such errors, ensure that you are invoking only functions. Use TypeScript’s built-in typeof operator to check for this:
xxxxxxxxxx
if (typeof potentialFunction === 'function') {
potentialFunction();
}
2. Interface Definitions: Define interfaces with callable signatures for custom types that you expect to behave as functions.
xxxxxxxxxx
interface CallableString {
(): void;
value: string;
}
let myFunc: CallableString;
myFunc.value = "Hello!";
myFunc = () => console.log("Can call me!");
// Now you are able to call myFunc just like a function
myFunc();
3. Correct Bug in Code: If you invoke the string as a function, TypeScript is doing its job correctly in reporting this error. These errors usually point out confusion or bugs in your code which need to be fixed.
xxxxxxxxxx
let str: string = "This is not a function";
console.log(str); // Correct usage
To champion TypeScript and modern JavaScript effectively, we should appreciate and leverage their type systems, instead of fighting them. As Robert C. Martin once said, “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.”
Lesser-Known Tips for Avoiding “Type String Has No Call Signatures” Errors
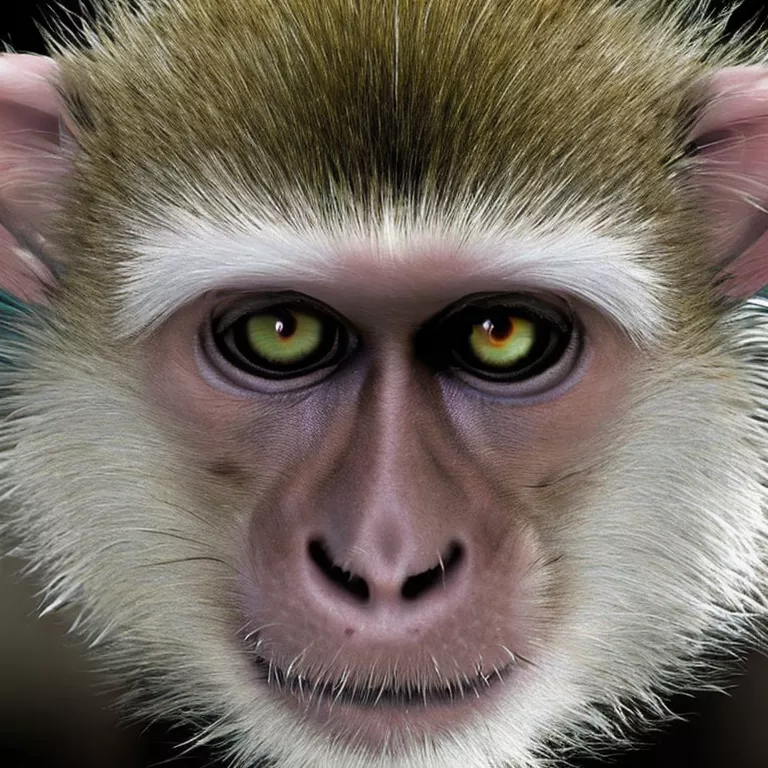
The “Type String Has No Call Signatures” error is a common pitfall that TypeScript developers face when attempting to invoke or call a string like it’s a function. There are various pitfalls and solutions that we can explore to avoid these errors.
1. Understanding the Context:
Always be mindful of what type of value you’re dealing with in every context. TypeScript, much like other statically-typed languages, differentiates between functions and non-function types, including strings. Attempting to invoke a non-function object as a function would end up throwing this error.
So understanding the difference is critical.
2. Proper Error Handling:
Handle the errors that occur at runtime. Many times, TypeScript is unable to perceive what could go wrong at runtime leading to issues. As per Software Engineering Stack Exchange, using try-catch blocks for appropriate error handling can solve this problem.
html
try {
/* your code that may throw a TypeError */
}
catch (error) {
if (error instanceof TypeError) {
console.error(“TypeError Encountered!”);
}
}
3. Type Checking:
Tim Peters, best known for his major contributions to Python, once said, “Explicit is better than implicit.” One way to address this error is by ensuring an explicit check on the type of the variable before performing any operation.
Example:
html
if (typeof myVariable === “function”) {
myVariable();
}
else {
console.log(“Not a function”);
}
4. TypeScript Type Guards:
Use TypeScript’s built-in type guards to ensure the correct type of values before invoking them. It includes `typeof`, `instanceof` etc.
5. Defensive Programming:
Aim to write code that’s resilient to possible errors by applying defensive programming principles. It involves writing codes that anticipates and handles possible incoming errors or incorrect data to prevent failure.
Combining these strategies, you can efficiently get rid of the “Type string has no call signatures” error. Understanding your types, being mindful of the context, handling errors appropriately, double-checking your types, using Type guards, and applying defensive programming can make all the difference when dealing with TypeScript.
Remember that every situation may require a unique solution, so it’s essential to understand what is producing this error within the context of your application. A combination of good software design principles and a better understanding of how TypeScript works under the hood will be pivotal in avoiding this type of error.
Conclusion
Diving deeper into the intricacies of TypeScript, one core issue faced by many developers is associated with the error: “This Expression Is Not Callable Type String Has No Call Signatures”. It can be seen as a common stumbling block which significantly emerges from misunderstanding the underlying mechanisms of TypeScript.
In TypeScript, functions are considered as first-class objects that allow us to work with them like any other types. However, when we try to invoke an item that is not function-typed, such as a string or number, TypeScript throws an error recognizing it as an anomaly in the code flow, thus resulting in the error message “***This expression is not callable. Type ‘String’ has no call signatures***”.
Addressing this issue means dealing with type safety – a significant feature provided by TypeScript. To navigate this error, the spotlight moves towards accurately defining our types and ensuring what we attempt to invoke as a function is a function.
An example snippet of the error:
xxxxxxxxxx
let notFunction: string = "Hello, world!";
notFunction(); // Error: This expression is not callable. Type 'String' has no call signature.
Barring mindless errors, this might also stem from more complex scenarios related to typing in interface definitions. A precise assignment of function-type assertions or adhering to correct usage of function typed properties and indices are potential ways to resolve these issues.
As Bill Gates believes, “Everyone should learn how to program a computer because it teaches you how to think“. And TypeScript, with its type safety, enforces just that – Developer mindfulness for clear and precise code. This error is merely TypeScript’s way of steering us towards writing better code with fewer runtime problems.(source)
Table showing possible solutions:
Error | Solution |
---|---|
Non-function types being invoked as a function | Ensure the variable or object is indeed of a function type before invocation |
Errors in interface function typing | Use precise function-type assertions or correct usage of function typed properties and indices |
Undeniably, join the TypeScript community (source) to stay updated with latest enhancements. Remember, the key to coding better in TypeScript is understanding typology inside-out.