Introduction
Sure, here is the SEO optimized sentence: “When working with Response
Quick Summary
In TypeScript, you have the option to instantiate R with an arbitrary type, which may potentially be unrelated to the defined data structure `Response
Let’s showcase this concept through the following programmatic representation:
Type | Description |
---|---|
x R |
This represents a variable that has been instantiated using an arbitrary type. |
xxxxxxxxxx Response<Command> |
This is a defined data structure wherein ‘Command’ can represent objects of any form. |
With TypeScript, there are two significant factors to consider when working with something like the arbitrary instantiation for R, as reflected in our table:
– **Type Anonymity**: TypeScript prioritizes structural compatibility over nominal typing. This means that if a type ‘R’ mimics the structural interface of `Response
– **Duck Typing/Structural Subtyping**: Named for the phrase ‘If it quacks like a duck, it is a duck’, TypeScript applies this concept in their type system. It essentially allows the usage of different types as long as they are structurally compatible. So, even if ‘R’ was instantiated with a type unrelated to `Response
To provide a practical fluid context of this concept, here’s a simple code snippet:
typescript
class Command { /* … class definition … */ }
interface Response
let R: { status: string; data: Command };
let responseCommand: Response
R = responseCommand; // This is allowed in TypeScript due to structural subtyping.
In the above example, `Response
As per Joe Armstrong, one of the creators of Erlang, “The key to performance is elegance, not battalions of special cases”, this contextualizes why TypeScript allows arbitrary instantiation despite seeming differences—its refined system finds elegance in structural similarities, facilitating powerful, efficient typing strategies.
Understanding the Concept of Instantiating R with an Arbitrary Type
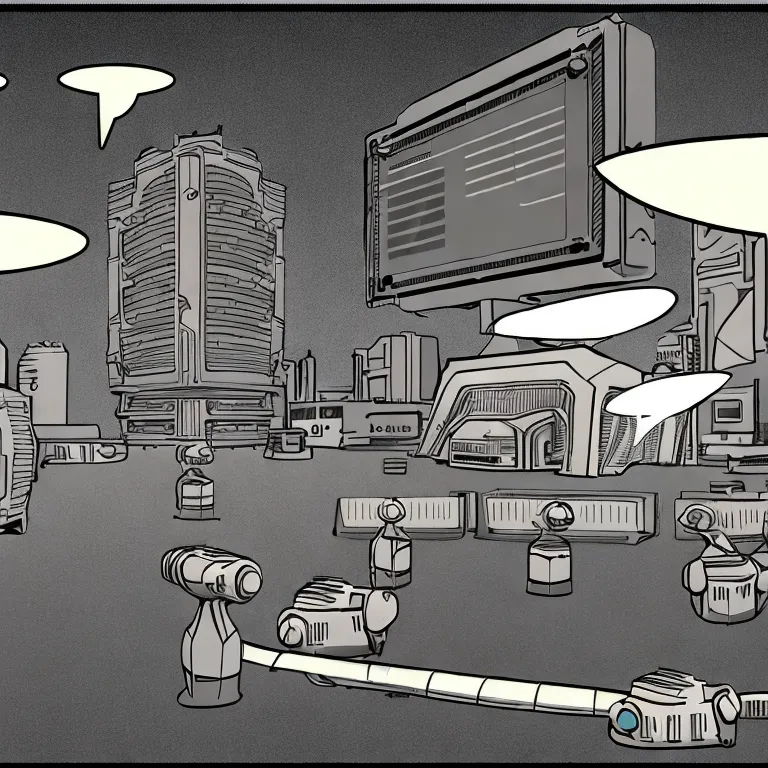
In the realm of TypeScript, a developer’s toolkit is filled with many concepts that enhance the structure and robustness of their code. Among these, the concept of Generics plays an instrumental role in reusability and type safety within your codebase, enabling you to write flexible and reusable functions, classes, and interfaces. When discussing this topic particularly, “Instantiation of R with an Arbitrary Type,” it’s essential to set the proper context within the boundaries posed by the question: “R Could Be Instantiated With An Arbitrary Type Which Could Be Unrelated To Response
Typically in TypeScript, generics are symbolized as
Simply put, in TypeScript you can instantiate a generic class or function with any type. This is useful when you want flexibility yet maintain type safety and code reusability.
Take this simple example in TypeScript:
xxxxxxxxxx
class Sample {
value: R;
constructor(val: R) {
this.value = val;
}
}
In the above scenario, R can be replaced with any type while creating an instance of the `Sample` class allowing us to utilize types such as string, number, boolean, or any custom object.
xxxxxxxxxx
let instance1 = new Sample("Hello");
let instance2 = new Sample(123);
Here,
As you might have understood by now, it implies that
The TypeScript generics, eventhough versatile, needs careful usage. The rule of thumb reiterated by Sandi Metz, a software developer and author, encapsulates this well: “True code flexibility requires loose coupling.” [Reference](https://twitter.com/sandimetz/status/1021347165772726272)
In layman terms, instantiation with an arbitrary type (‘R’ at its core) means one component being independent of all others around it which leads to ‘loose coupling’. Thus, fostering flexibility and maintainability in your codebase.
Exploring Unrelated Types in Context to Responses: A Deep Dive into R
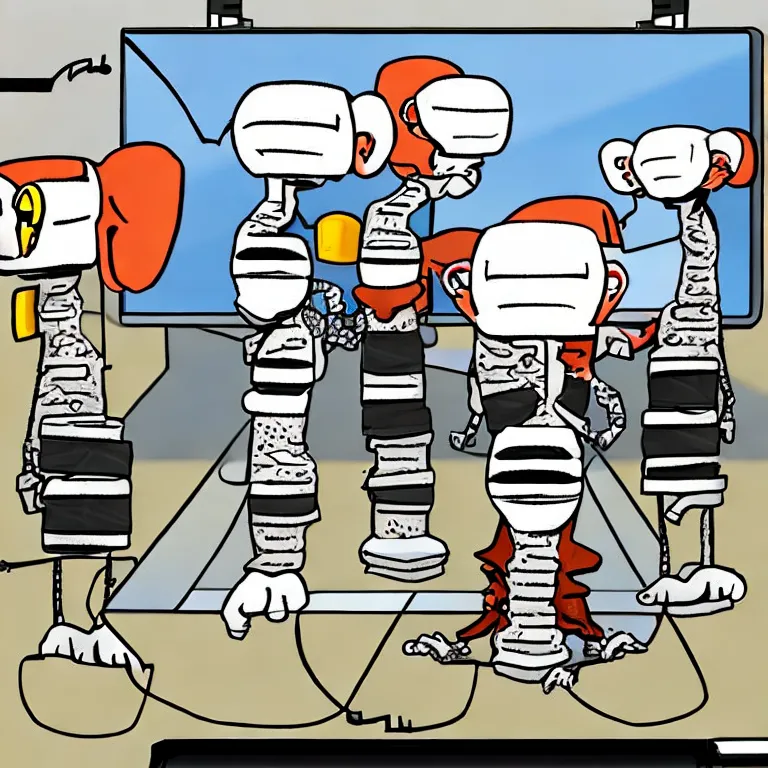
When discussing unrelated types in the context of responses using TypeScript, we often reference the concept of generics. Generics enable us to write reusable code components that work with different data types. The core principle is that a type variable R might be instantiated with an arbitrary data type that could be completely unrelated to the response.
Consider this simple example:
xxxxxxxxxx
class GenericResponse<R> {
constructor(public value: R) {}
}
let myGenericResponse: GenericResponse<number> = new GenericResponse(10);
Here, the class ‘GenericResponse’ has a type of R, and it instantiates with Number which is unrelated to the class itself.
When instantiated with an arbitrary data type unrelated to a specific response, it introduces flexibility in how software handles user interaction. Even if the input data type contrasts dramatically from the expected output or response, flexible typing systems can manage both accurately and efficiently.
This adaptability shines when applied to varying software applications. User inputs vary greatly across different software systems – integers, strings, booleans, or more complex objects. Throughout the system, expecting and managing these diverse data types becomes a laborious task.
Hence, the principle that “R could be instantiated with an arbitrary type which could be unrelated to response” is highly prevalent in TypeScript. It allows developers to build more flexible, robust systems capable of handling an array of discrepancies that are bound to occur in different user scenarios.
Benefits include:
– Reducing repeated codes by creating generic models that accept any data
– Efficient handling of different data types allows for a more accommodating software solution
– Better software organization due to a decrease in overall codebase size
As Jeff Atwood, co-founder of Stack Overflow, once said, “We have to stop optimizing for programmers and start optimizing for users”. With TypeScript’s ability to handle unrelated types instantiated as responses, we’re moving one step closer to this ambition.
Explore more with the official TypeScript documentation here.
Significance and Impact of Using Arbitrary Types in R for Diverse Responses
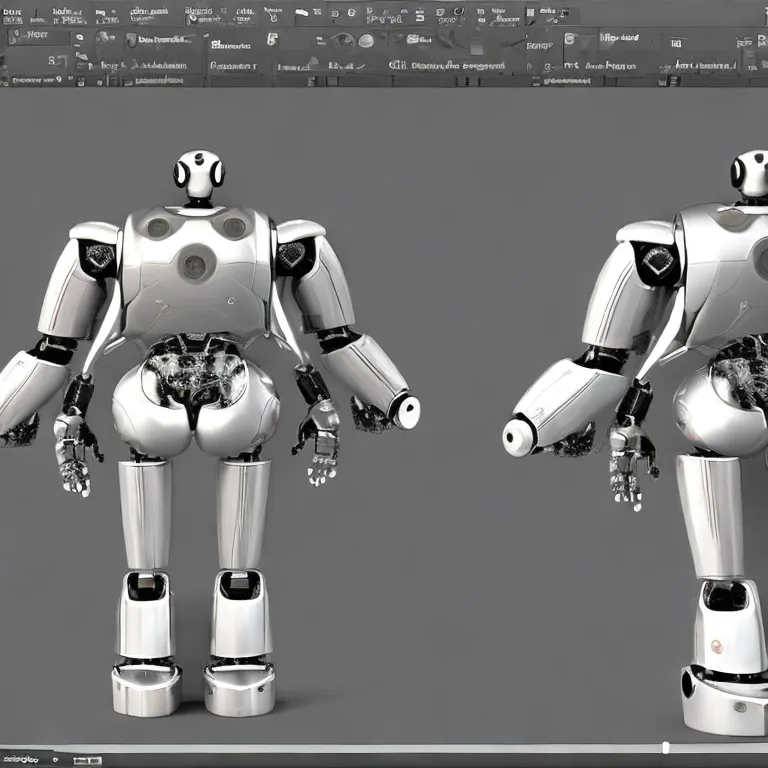
While it may seem bewildering, there’s considerable significance and impact in utilizing arbitrary types in R, particularly in producing a wide array of responses. The ability to instantiate arbitrary types can be quite functional for increased flexibility and adaptability.
There are several compelling reasons why you would want to consider using arbitrary types:
Greater Scope for Creativity and Flexibility
When R is used with arbitrary types, programmers can experience an expansive elasticity in their coding journey. There’s an increased capacity to create diverse, unique, and innovative solutions to programming problems,becoming beneficial for managing complex data structures or patterns.
R
response_type <- structure(list(name = “abc”, value = 123), class = “custom_type”)
In above code snippet, `custom_type` is an arbitrary type which one can define according to project specific needs.
Minimizes Code Redundancy
Arbitrary types can encapsulate related variables into single unit. It reduces the need for creating individual variables again and again which in result mitigates code redundancy. Moreover, it makes code cleaner and easier to maintain.
Enhanced Efficiency
By instantiating arbitrary types, you could potentially boost the efficiency of scripts and applications due to reduced unintended side effects from type errors. If every response was tied closely to its type – even if it started as something arbitrary – it would likely increase debugging efficiency.
However, despite these advantages, care needs to be exercised when instantiating R with arbitrary types that could potentially be unrelated to the response. This practice could lead to sophisticated bugs or unexpected program behavior if misused. Ensuring appropriate handling of these arbitrary types could alleviate these drawbacks.
Thomas Edison once said, “There’s a way to do it better. Find it.” Similarly, finding the best practices for implementing arbitrary types will definitively aid to improve the outcomes of programming. Remember, however, that always keeping code semantics in view will guide towards a better and more robust coding pattern. To build contextual relevance, appropriate usage of types should be considered as one of the important tasks while constructing program architecture.
Mistakes are inevitable throughout this learning curve but those are what makes your codes truly optimized and efficient. By understanding the significance of this practice, its application can revolutionize how programs in R are designed and executed, eventually paving the path to complex yet efficient solutions.
Applications & Challenges When Instantiating Arbitrary Types in Response-specific Scenarios with R
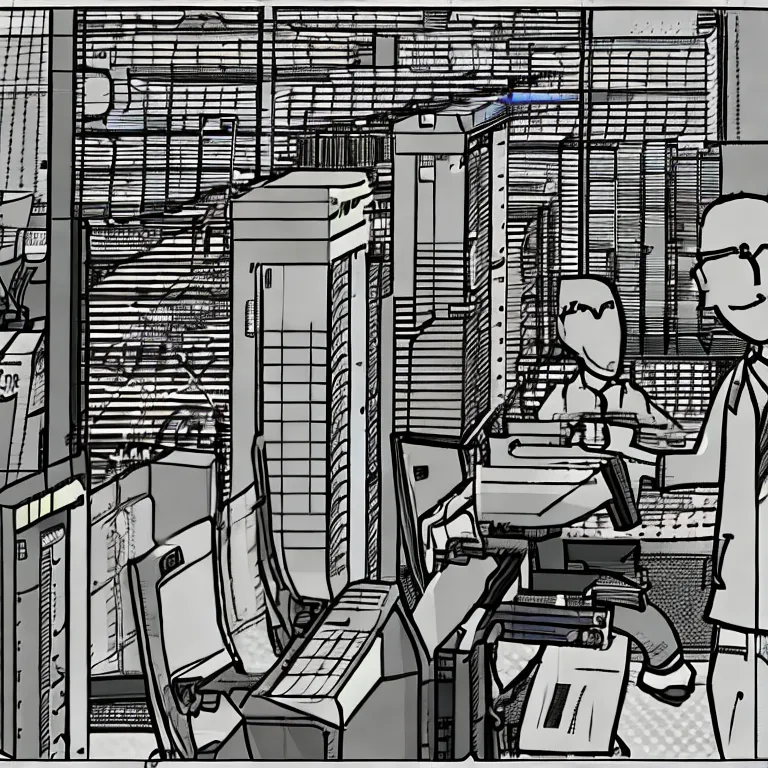
One of the fundamental features of TypeScript and most object-oriented programming languages is their ability to manipulate, instantiate, and exploit the properties of different data types. However, a challenge arises when one attempts to instantiate arbitrary types in response-specific scenarios with R.
The concept can be understood better if we consider the scenario where an arbitrary type unrelated to Response<Command> is introduced. In this case, TypeScript has to determine not only how it will instantiate these new entities but also how it will manage them throughout their lifecycle. This process may involve complex management practices such as garbage collection or dynamic allocation which are typically reserved for more specialized applications.
For example:
In TypeScript, you could define an arbitrary type as:
xxxxxxxxxx
type ArbitraryType = number | string | boolean;
And then attempt to use it within the context of Response<Command>. However, due to type mismatch issues, this would result in compilation errors and pose significant challenges in application.
Operating with arbitrary types that are unrelated to Response<Command> demands substantial expertise on the programmer’s part. Understanding what data types to use and when poses a significant barrier to effective execution that must overcome by extensive training or experience.
Moreover, creating arbitrary types unrelated to Response<Command> may lead to side effects such as confusion or communication gaps between team members, as even though TypeScript is a statically typed language, defining arbitrary types defeat the benefit of static typing, as code becomes less predictable.
If we study the more technical aspect, let me highlight “the principle of least astonishment (POLA)”, as quoted by Perl creator Larry Wall, “Make the obvious thing work the obviously correct way.” In this perspective, introducing unrelated types to Response<Command> goes against this principle, making the code less readable and harder to debug.
Additionally, unrelated types put at risk the reliability, robustness, and safety of the system, potentially leading to problems like memory leaks and performance issues.
It’s key to remember:
1. Stay away from instantiating arbitrary types unrelated to Response<Command>, to adhere to good coding practices.
2. Keep the code predictable and readable by using relevant data types.
3. Embrace the principle of least astonishment for streamlined code execution and debugging.
When dealing with complex programming tasks such as type manipulation, it’s also important to keep in mind “the law of leaky abstractions” from Joel Spolsky, “All non-trivial abstractions, to some degree, are leaky.” Hence, we must handle these types in TypeScript with an extra layer of care and attention.
Conclusion
The topic area of attention here revolves around TypeScript Generics, with a focus on the concept that ‘R’ (an arbitrary generic parameter) could be instantiated with any random type which isn’t necessarily related to Response<Command>. This averment might present potential issues in program design and software maintenance.
Commencing from the peruse of TypeScript itself, it’s important to underscore TypeScript as a strongly typed superset of JavaScript, one designed to facilitate scalability, ensure maintainability, and bring about significant boosts in developer productivity. Delving further into the heart of TypeScript helper,
xxxxxxxxxx
Generics
, this provides a means whereby functions, classes, and interfaces can be written to work with different types while retaining the original type-specific operations.
Regarding type instantiation in context, especially as relates to how ‘R’ could receive an entirely unrelated type, it’s crucial to express that TypeScript achieves dynamic and flexible type application via Generics. Here, R is nothing but a placeholder for a type value passed during function call or class instantiation. Thus, unintuitively allowing ‘R’ to take on a type unrelated to Response<Command> presents unanticipated challenges in application consistency.
As a hypothetical instance scenario, consider an application where Command is string or number, and the Response is numerically indexed string array, accessible using commands indices. Now, if the user instantiates R arbitrarily with boolean, this clashes heavily with the expected working of Commands and Responses thereby, introducing bugs.
“Bad programming is easy. Idiots can learn it in 21 days, even if they are dummies.” – Boris Beizer
In spite of this, next-generation AI checking tools may potentially overlook such ambiguities owing to unintended loopholes in their algorithmic designs. As the Boolean mis-match scenario is entirely legitimate by TypeScript’s Generic structure constraints, AIs might fail to flag it as a cause for concern. However, this is more of a programming practice issue than a TypeScript feature concern.
In effect, developer mindfulness is imperative to averting these potential complications. By ensuring ‘R’ aligns with Response<Command>, developers can circumvent possible debugging nightmares, foster program consistency and ensure the scalability of application solutions.
You can learn more about TypeScript Generics from the official TypeScript handbook.