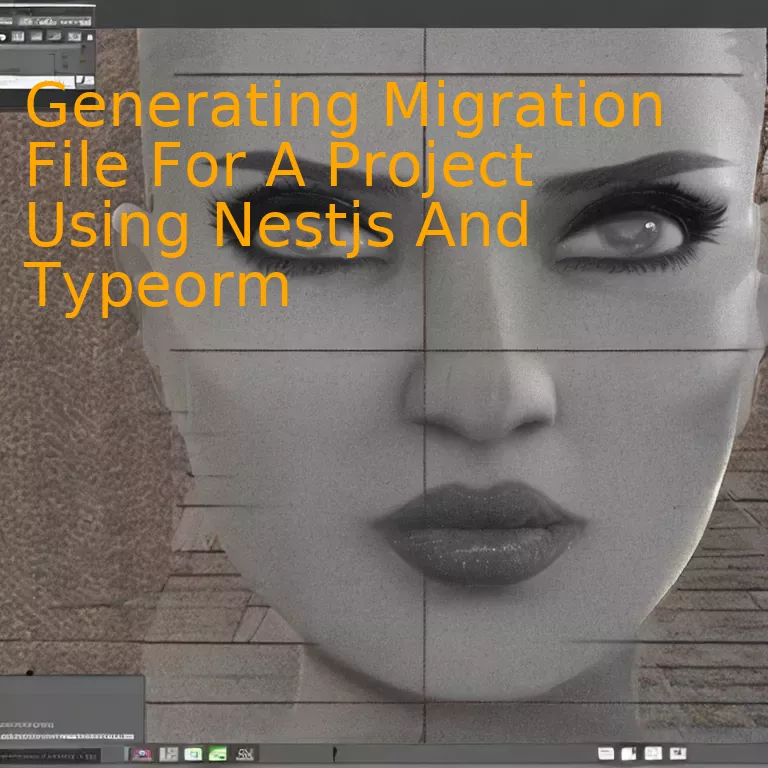
Introduction
When managing your project with Nestjs and Typeorm, generating a migration file can streamline database alterations, thus improving efficiency and maintaining data consistency.
Quick Summary
Your question revolves around generating a migration file using NestJS and TypeORM for your project. This process comes down to a few pivotal steps:
Steps | Description |
---|---|
Create Database Connection | Establishing a method of communicating with the database is a crucial first point of call. |
Generate Migration | This involves instructing TypeORM to read project entities and generate a migration file that reflects corresponding changes in your database schema. |
Run Migration | Last but not least, execute this migration file, applying all changes codified within to update your database structure. |
Let’s delve in, shall we?
The primary aspect is initializing a connection between our application and the database. NestJS combined with TypeORM provides a direct and efficient programming technique to configure this connection. Your TypeORM module could be designed to synchronize with a Postgres database utilizing specific environment variables like so:
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.POSTGRES_HOST,
port: +process.env.POSTGRES_PORT,
username: process.env.POSTGRES_USER,
password: process.env.POSTGRES_PASSWORD,
database: process.env.POSTGRES_DB,
autoLoadEntities: true,
synchronize: false
})
This configuration helps maintain secure and seamless communication with your database.
Next, comes the generation step. Once we have set up our ORM configuration, we can use TypeORM’s CLI to generate migration files based on our entities. The command `typeorm migration:generate -n MigrationName` does the trick.
Lastly, it’s time to implement this derived migration file. Again, our reliable friend TypeORM comes into play. The simple command, `npm run migration:run` promptly runs all pending migrations, reflecting changes on your database.
Katherine Johnson, a pioneering programmer once said, “The main job was to analyze the man-made environment of space.” In our case, the man-made environment is the database schema, and as developers, it’s our job to analyze it, adapt it, and evolve with our project. Generating migration files using NestJs and TypeORM provides us with the necessary tools to do that effectively.
Understanding the Role of Migration Files in NestJS and TypeORM Projects
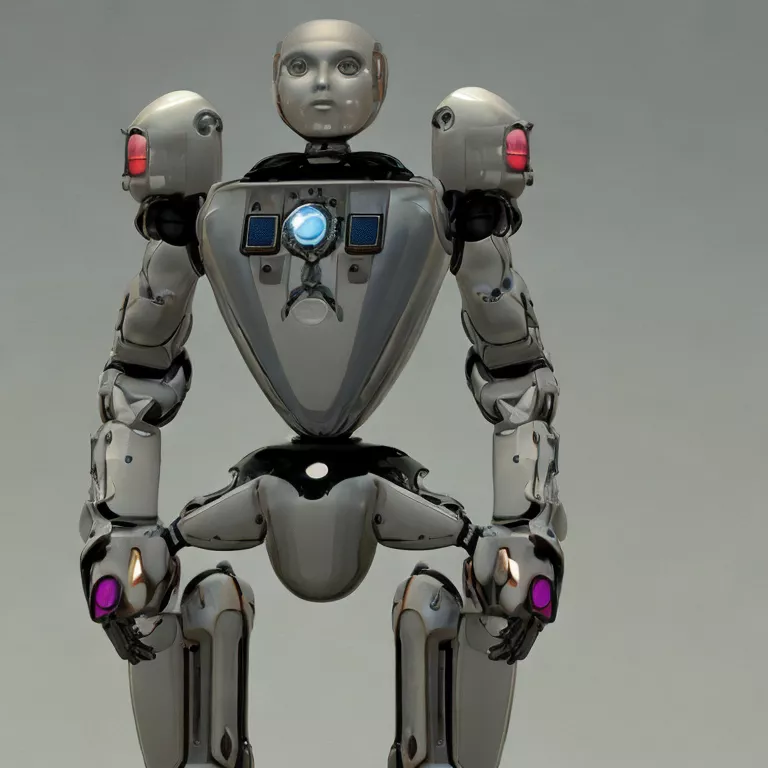
Migration files play a significant role in NestJS and TypeORM projects. Primarily, migration files aid in creating a sequential order of transformations that mold your database schema. These transformations include creating tables, adding columns, updating data, and more.
NestJS, being a powerful Node.js framework that supports TypeScript directly, pairs well with TypeORM- an Object-relational mapping (ORM) tool designed to work effectively with TypeScript. When using these two technologies concurrently, creating and maintaining structured and well-crafted database schemas become seamless.
To grasp this concept explicitly:
Realizing the Significance of Migration Files
Control Over Database Schema Evolution:
Migration files provide comprehensive control over the evolution of a project’s database schema. With migrations, developers can apply alterations to the database manually, allowing better supervision and management of changes in a systematic order.
Structural Consistency Across Environments:
It is crucial to maintain structural consistency across all environments. Migration files resolve the issue of manual database adjustmentsin different environments, ensuring that a unified structure is adhered to throughout development, staging, and production environments.
Version Controlling:
Creating a linear set of amendments that migrate your database scheme ensures that these files are version controllable. The ability to track modifications made to the database schema is a particular advantage when multiple teams or developers are involved.
A Guide to Generating Migration File for Project Using NestJS And Typeorm
When utilizing Nestjs along with Typeorm for project development, here’s a succinct guide on generating a migration file.
Firstly, make sure Typeorm is installed and setup. Then, generate a migration file using the Typeorm CLI:
xxxxxxxxxx
npx typeorm migration:create -n MyFirstMigration
This command creates a new file under the path specified in
xxxxxxxxxx
typeormconfig.js
.
Subsequently, implement the generated empty migration file using up and down methods from TypeORM MigrationInterface.
xxxxxxxxxx
export class MyFirstMigration implements MigrationInterface {
public async up(queryRunner: QueryRunner): Promise {
// Defining your table structure here
}
public async down(queryRunner: QueryRunner): Promise {
// Reverting any change you are making in up method here
}
}
The ‘up’ function is employed to run the migration, while the ‘down’ function cancels performed actions.
To execute the migration, use:
xxxxxxxxxx
npx typeorm migration:run
NestJS and TypeORM produce an efficient TypeScript ecosystem, and database migrations performed with these technologies can create a structured and effective solution for project development.
“We think of coding as a form of expression, laying out your thoughts and ideas into something tangible. It’s like building with virtual LEGO bricks, except that the bricks are tiny commands fired by your keyboard,” – Chris Wanstrath, Github Co-founder. Practice deliberate implementation of your thoughts through coding, ensuring that every line of code reflects your intents lucidly and effectively.
Strategic Approach to Generate Migration File Using NestJS
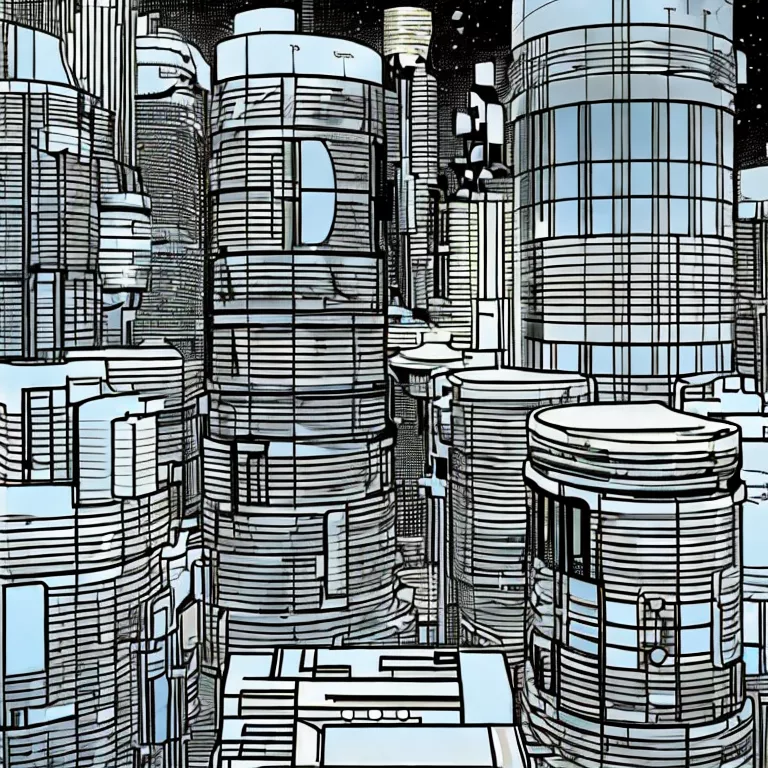
A strategic approach to generating migration files using NestJS begins with a proper understanding of how NestJS and Typeorm work together. NestJS, being a progressive Node.js Javascript framework, is built for developing reliable and scalable server-side applications. It supports TypeScript out-of-the-box, offering robustness and maintainability by incorporating strong typing.
Typeorm, on the other hand, is highly flexible; it can run in NodeJS and browsers, be connected to different databases, and support various JavaScript platforms like TypeScript. Its functionality includes migrations API which allows you to manage your database schema manually and automatically.
How to Generate a Migration File Using Typeorm & NestJS?
The process begins with defining your entities in your NestJS application. An entity is a class that maps to a database table. Each instance of an entity represents a row in the table. Once your entities are defined and mapped, running the migrations:generate command will lead to the representation of these entities as tables in the database.
xxxxxxxxxx
npm run typeorm:migration:generate -- -n CreatePosts
In this example, `CreatePosts` is the name of the migration we want to generate. You should replace ‘CreatePosts’ with the name appropriate for your use-case. After running this command, a new file should appear in the ‘migrations’ directory of your application. This file contains SQL queries written in JavaScript for both up (executing the migration) and down (reverting the migration).
Approaching This Strategically
When following a strategy for generating these migration files, consider these points-
• Always categorize each entity change as a separate migration. The notion is to have multiple small migrations instead of one big migration file; it keeps the changes manageable and traceable.
• Make sure you annotate all your migration classes; it aids in maintaining clarity about what changes they are implementing to your database structure.
• Always test the migration up and down scripts. Ensuring the ability to revert without any data loss or structure issue is exactly what makes using migrations so powerful.
For further understanding, follow the official NestJS documentation on SQL(Typeorm), and Typeorm Migrations.
Mastering Migration File Creation with TypeORM: Step-by-Step Guide
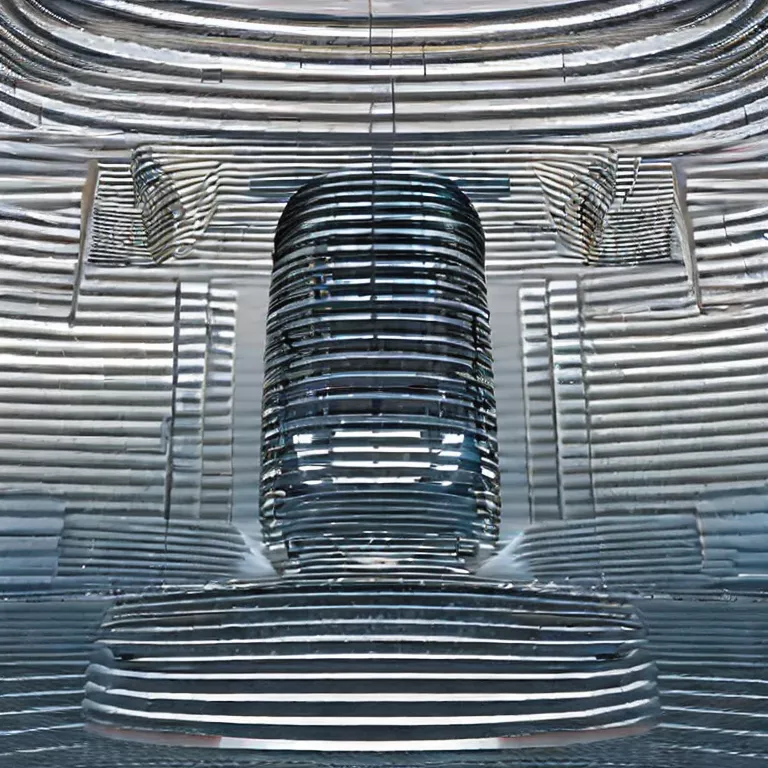
Migrating data and tables is an integral part anytime you aim to update or improve your application. TypeORM, a renowned Object-Relational Mapping (ORM) tool for TypeScript and JavaScript, provides powerful migration API that allows developers to perform this task in an efficient and developer-friendly manner. The focus of this discussion will be about the generation of a migration file for a project developed using Nestjs with Typeorm.
Before We Start: What is a Migration?
Migration describes the process of applying updates within the database schema without compromising any existing data. These updates can be anything from creating new tables, modifying existing ones, or even deleting them. It assures that your database schema remains in sync with your entity metadata defined in your TypeScript code, which enhances the overall efficiency.
TypeORM and Nestjs Overview
TypeORM is an innovative, feature-packed ORM designed for both TypeScript and JavaScript. It supports multiple databases and aids in working with databases abstractly.
On the other hand, Nestjs is a robust framework for building server-side applications that primarily utilize TypeScript. Using these technologies together combines high performance with ease of development.
Generating Migration Files
Firstly, ensure your project configuration is correctly set up to use TypeORM with Nestjs. Once properly configured, to generate a migration file, you need to run the TypeORM CLI ‘migration:generate’ command. The command format looks like this:
xxxxxxxxxx
npm run typeorm migration:generate -- -n MigrationName
The ‘-n’ flag indicates the name of the migration. ‘MigrationName’ should be replaced with the name you desire for your migration file.
Understanding the Generator Script
When you run the mentioned command, TypeORM compares the entities in your codebase with the current state of your database. It then generates a SQL operations script considering any differences found.
Nestjs projects with TypeORM configuration look something like this without usage specific details:
xxxxxxxxxx
<pre>
{
"type": "postgres",
"host": "localhost",
"port": 5432,
"username": "user",
"password": "password",
"database": "test",
"entities": ["src/**/**.entity{.ts,.js}"],
"migrations": ["migration/*.ts"],
"cli": {
"migrationsDir": "migration"
}
}
</pre>
The ‘migration:generate’ command creates a new .ts file in the ‘migrations’ directory, as defined in the configuration.
In the words of Steve Jobs, “One should not compromise on the quality of their work”. Ensuring top-notch quality during migration file generation is imperative to guaranteeing functionally efficient migrations. To that end, creating effective and comprehensive migration files using Typeorm and Nestjs aids in maintaining individual software component’s excellence.
With TypeORM and NestJS, you can take your project’s data handling capabilities to the next level. Mastering migration creation allows seamless application improvements while keeping the integrity of your database intact. Plus, it provides a transparent view of how these modifications happen over time, keeping every team member on the same page.
Troubleshooting Common Issues When Generating a Migration file with NestJS and TypeORM
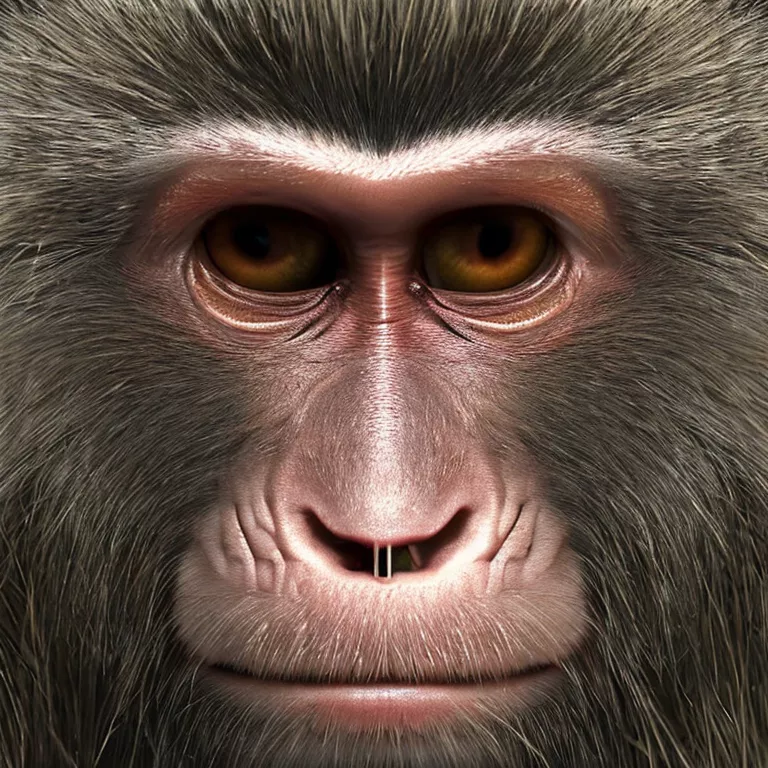
While working with NestJS and TypeORM for generating migration files for a project, developers may come across a few common problems that could hinder their progress. This section will detail the errors and offer solutions for restoring streamlined function. Understanding these issues and their resolutions can boost your efficiency in managing migration files.
1. **Incorrect Database Connection Configuration**
In scenarios where the configuration file (`ormconfig.json`) is not properly set up, or the environment variables are inaccurately configured, you’ll have trouble generating a migration file.
Typically, the `ormconfig.json` configuration should resemble something like this:
html
{
“type”: “mysql”,
“host”: “localhost”,
“port”: 3306,
“username”: “root”,
“password”: “root”,
“database”: “test”,
“entities”: [“src/**/**.entity{.ts,.js}”],
“migrations”: [“src/migration/**/*{.ts,.js}”],
“cli”: {“migrationsDir”: “src/migration”}
}
Importantly, ensure that you’ve correctly assigned entities and migrations fields. Entities must align with your database models, while migrations should point to your directory containing generated migration files.
2. **Failure to Install CLI Globally or Missing Dependencies**
Make use of TypeORM’s CLI, which provides commands for generating migration files. A common problem arises when developers attempt to employ the CLI without installing it globally or if there are missing dependencies. Operating without these key elements results in failure to generate the necessary files.
To resolve this situation, install the CLI globally utilizing npm with the following command line in the terminal: `npm i -g typeorm`, then validate all dependencies are there.
3. **Misuse of TypeORM Synchronization**
TypeORM’s synchronization feature automatically updates your database schema whenever an application is launched, which can imperil data during production. Relying on migrations instead of this feature gives developers more controlled alterations. To avoid any related issues, be mindful to keep the `”synchronize”: false` flag in your `ormconfig.json` or connection options.
4. **Inappropriate Declaring of Entities**
When entities are not annotated with decorators like `@Entity()`, TypeORM cannot detect them, generating empty migration files as a consequence. Make sure to use these essential decorators when declaring your entities.
Remember Rasmus Lerdorf’s words: “*Programming is breaking down a complex problem into smaller manageable parts and then expressing that solution in code*”. By understanding the hurdles mentioned above related to NestJS and TypeORM and tackling them one at a time, you will streamline the process of generating migration files for your project.
Conclusion
Generating a migration file for your projects utilizing NestJS and Typeorm is an essential skill to possess in order to manage the change in database schemas professionally. Good practice starts with understanding your project’s requirement, then follows up by leveraging tools like NestJS and Typeorm. These platforms simplify the entire process and enable developers to create seamless, efficient, and maintainable applications.
By leveraging these services, you can adhere to the natural development life cycle of applications without resorting to altering the production database manually. This not only enhances efficiency but also significantly reduces the risk of loss or corruption of data due to human error.
-
xxxxxxxxxx
NestJS
: A server-side application framework that uses TypeScript, empowering the creation of secure, scalable, and manageable applications. It brings in elements of object-oriented programming, functional programming, and functional reactive programming.
-
xxxxxxxxxx
Typeorm
: An Object-Relational Mapping (ORM) tool supports both Active Record and DataMapper patterns. It offers a broad range of database options including MySQL, Postgres, and MariaDB among others. This too, uses TypeScript, for managing relational databases.
There are key steps taken when creating a migration file:
- Installing necessary dependencies.
- Configuring the ORM.
- Running the generate command.
To illustrate, let’s consider an example where we have added a new column in our entity:
xxxxxxxxxx
@Entity('users')
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ length: 500 })
name: string;
@Column({length: 500})
email: string;
}
After altering your models, you would run the following command to auto-generate migration files:
xxxxxxxxxx
npm run migration:generate -- -n AddUserEmail
The `-n` flag is to specify the name of the migration.
In order to create a robust and scalable system, AI developers need to become proficient with such processes. Elon Musk once said, “If you are not making some notable mistakes along the way, you are certainly not taking enough business and technological risks.” The usage of tools like NestJS and Typeorm in managing migrations for your project reduces the risk and allows for growth by creating space for scaling and expansion while maintaining data integrity.
Finally, across every stage of an application’s development life cycle, it’s vital to consider optimal practices to enrich overall efficiency. By using powerful tools and frameworks like NestJS and TypeORM, developers can simplify the task of generating migration files for their projects. This enhances productivity, encourages scalability, and most importantly, guarantees preservation of data over time.
For further details, do review Nestjs’s official guidelines on integrating Typeorm for generating migration files.