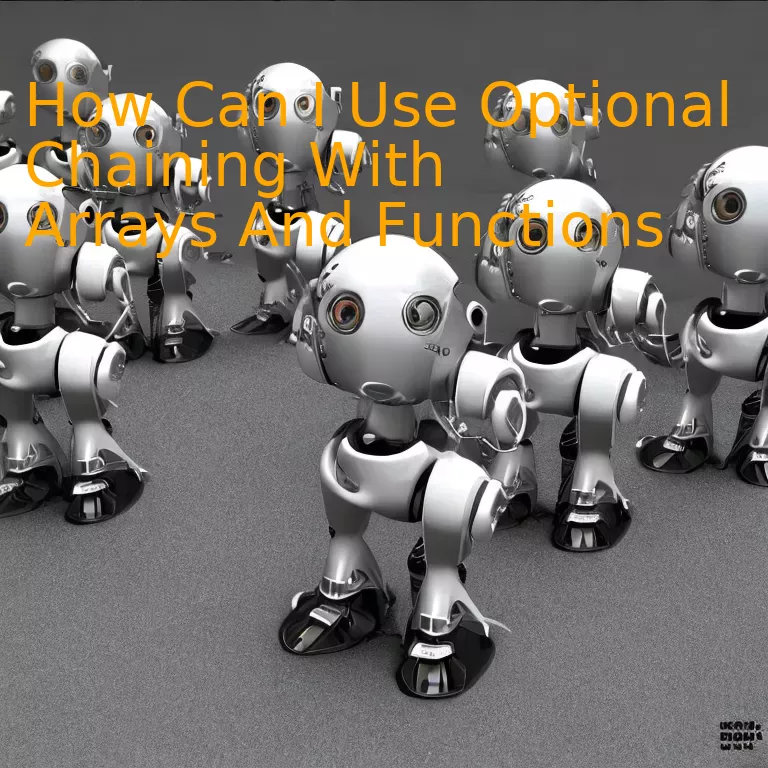
Introduction
To apply optional chaining effectively with arrays and functions, a developer must understand that it gives them the ability to read deeply into an array or function’s structure while avoiding errors from undefined/null properties and without the need for conditional statements. The syntax includes a question mark “?.”, which enables checks on whether the value before it is defined or not, making coding cleaner and clearer.
Quick Summary
Object | Chaining Element | With Optional Chaining | Without Optional Chaining |
---|---|---|---|
Arrays | Index | array?.[index] | array && array[index] |
Functions | () | functionName?.() | functionName && functionName() |
The table above delineates how to utilize optional chaining while working with arrays and functions in TypeScript. For understanding optional chaining, we first need to understand what it means and why it’s so beneficial.
Optional Chaining is a syntax that helps in reading the value inside an object chain regardless of whether the intermediate properties exist or not. This can be extremely convenient when dealing with nested objects where the existence of properties or keys can’t always be guaranteed. In JavaScript or TypeScript, trying to reference an undefined property or method typically results in either an error or `undefined`, which can create ripple issues throughout your code.
When you’re working with arrays, optional chaining provides a safe way to access an element by its index. With the help of optional chaining (`?.[]`), we can now directly attempt to read inner properties of the array without worrying about causing an error if the array is undefined or null.
Let’s observe the following example:
let arr = undefined;
console.log(arr?.[1]) // will output 'undefined' instead of causing an error
In this instance, rather than causing an error due to `arr` being undefined, optional chaining takes over and simply returns ‘undefined’.
The same principle applies to functions. Optional chaining facilitates in calling a function only if it exists, otherwise, it bypasses the execution altogether. Here’s a snippet illustrating how:
xxxxxxxxxx
let functionName = null;
console.log(typeof(fn?.())); // will output 'undefined'
Without optional chaining, we would need to manually verify the existence of an index for the array or the function before attempting any further operations.
As Steve McConnell, author of Code Complete, said:”Good code is its own best documentation.”
Indeed, optional chaining serves as a testament to good coding practices by not only making the code cleaner but also reducing the possibility of runtime errors.
Leveraging Optional Chaining with Arrays in Javascript
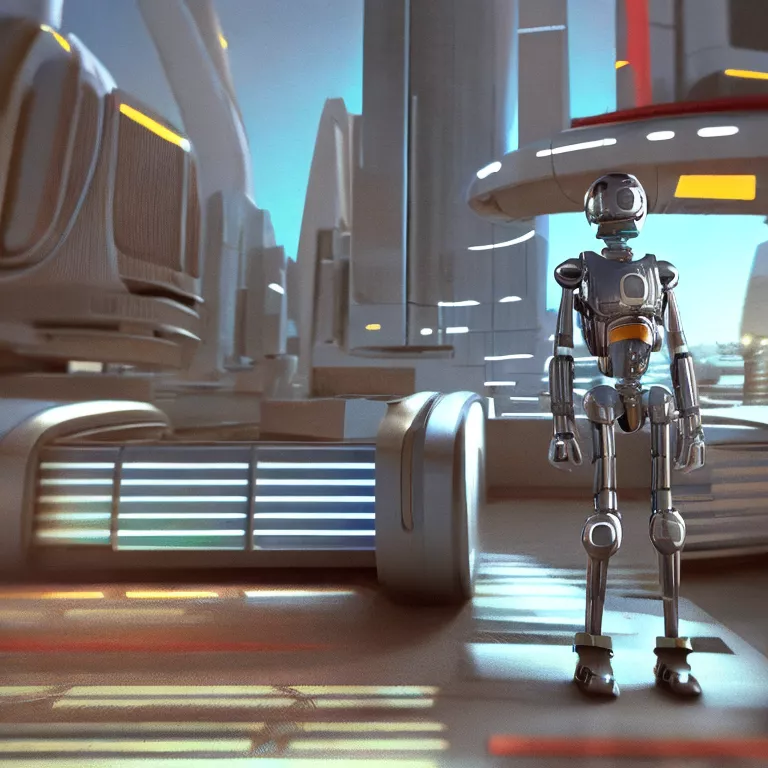
The Optional Chaining feature in JavaScript, which is a part of ECMAScript 2020, can be used to simplify how you access properties and call functions within arrays. This feature offers a way to get around errors due to undefined or null values with less hassle.
Optional Chaining with Arrays
You no longer need to litter your codes with repetitive conditional checks for every layer of objects just to avoid the TypeError: Cannot read property ‘x’ of undefined error. Here’s an example:
With optional chaining:
xxxxxxxxxx
let nestedProp = obj?.prop?.[0]?.nested;
Without optional chaining:
xxxxxxxxxx
let nestedProp = (obj &&
obj.prop &&
obj.prop[0] &&
obj.prop[0].nested) ? obj.prop[0].nested : undefined
From the above code snippet, it’s clear that optional chaining helps streamline your code, making it easier to read and maintain.
Optional Chaining with Functions
This works in similar fashion with functions. Accessing properties of potentially undefined values could trip your code but with optional chaining you can rest easy. Let’s look at an example.
With optional chaining:
xxxxxxxxxx
let result = obj?.func?.();
Without optional chaining:
xxxxxxxxxx
let result = obj && obj.func ? obj.func() : undefined;
Additional conditionals are no longer necessary. If
xxxxxxxxxx
obj
or
xxxxxxxxxx
func()
is undefined,
xxxxxxxxxx
result
simply retains an undefined value rather than throwing an error.
Kenneth Love, a renowned software engineer once said: “Code is readable by humans and writable by machines.” It’s clear that optional chaining aids in this regard by rendering clearer, cleaner code. Consider embracing this robust feature in your JavaScript coding today to optimize the handling of undefined or null values and for a snazzier JavaScript development experience.
Remember, by leveraging the power of optional chaining with arrays and functions, you’ll add an extra layer of efficiency to your coding process. For more information, consider checking out Optional Chaining on Mozilla Developer Network.
Utilizing Optional Chaining to Simplify Function Calls
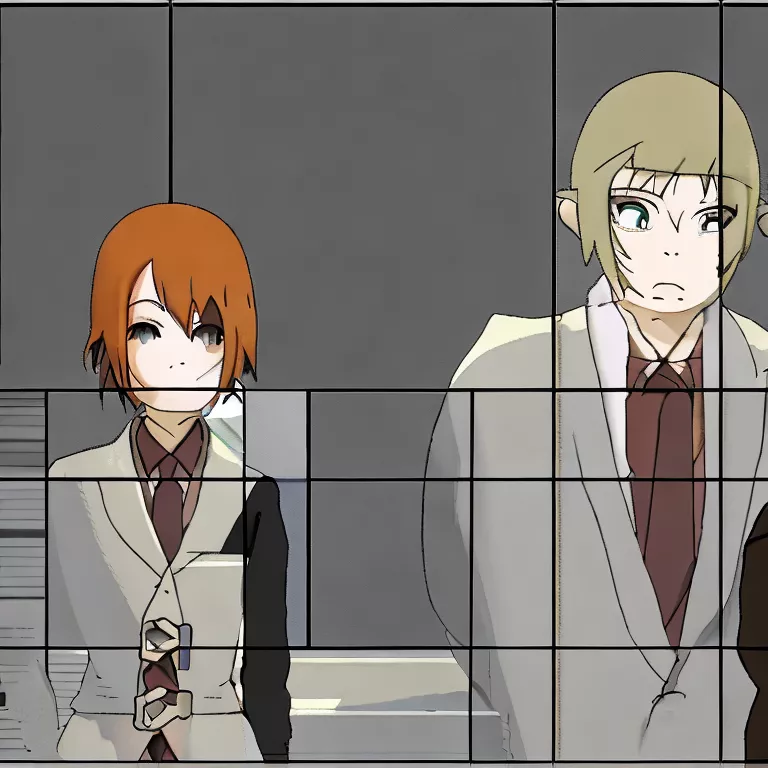
Optional Chaining is a relatively newer addition to JavaScript (and by extension TypeScript), which significantly simplifies accessing nested object properties. This is incredibly useful when it comes to working with optional values in arrays or invoking functions that may not always exist in an object.
Consider the scenario where you are dealing with an array of objects and want to access a particular property. Traditionally, to prevent “undefined” or “Cannot read property of undefined” errors, you would have to check each parent property if it exists before attempting to access the child property.
let person = {
name: ‘John Doe’,
address: {
street: ‘123 Main St.’,
city: ‘Anytown’,
code: 12345
}
}
if (person && person.address && person.address.street) {
console.log(person.address.street);
}
This can get tedious with deeply nested properties. However, with Optional Chaining, this task becomes a breeze:
let street = person?.address?.street;
console.log(street);
If at any point in the chain, a null or undefined value is encountered, evaluation stops, and the entire expression returns undefined. This goes a long way in offering you protection from unavailable properties in your data structure.
In case of functions, imagine calling a method that might not be available on the object at runtime. Without Optional Chaining, you might typically do something like:
if (person && typeof person.sayHello === ‘function’) {
person.sayHello();
}
With Optional Chaining involved, function calls become much simpler and cleaner:
person.sayHello?.();
The ?. operator functions in such a way that if the part before ?. is not defined or null, then it won’t attempt to call the function and just return undefined without throwing an error. This practice proves very beneficial when dealing with functions in objects that might or might not be defined.
Learning how to handle optional properties and methods with Optional Chaining can indeed help you write safer and more readable code. Steven McConnell, a noted software engineer and author once said, “Good code is its own best documentation.” With features like Optional Chaining, you’re well on your way to crafting cleaner and more understandable TypeScript code.
Unveiling the Potential of Optional Chaining for Array Manipulations
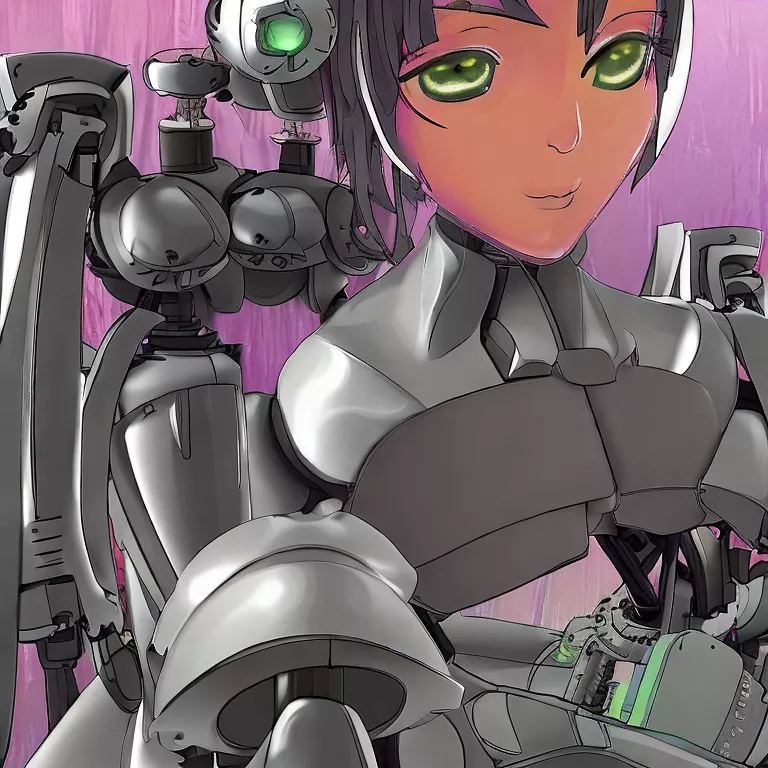
Optional chaining is a cutting-edge JavaScript functionality introduced in ES2020 as an efficient way to access deeply nested properties within structures. TypeScript, a superset of JavaScript, has also adopted this tool, which can greatly aid in handling and manipulating arrays. Nonetheless, it is crucial to consider both its capabilities and limitations in this context.
Optional Chaining’s Role in Array Interaction:
At its core, optional chaining is utilized to simplify lines of code by removing the need for conditional statements before accessing object methods or properties. This approach offers a cleaner, more readable syntax that developers often prefer, particularly when working with large arrays of structured data.
For a code example, let us assume we have an array of users, where each user object has certain properties:
typescript
let users = [{name: ‘Joe’, age: 55 }, { name: ‘Jane’, quote: ‘Coding is fun!’ }];
If you’re looking to access the property of an item that may not exist in the array (e.g., `quote` on the first user), using optional chaining can eradicate unwanted errors:
typescript
let firstUserQuote = users[0]?.quote;
In the above snippet, TypeScript would return `undefined` rather than breaking the entire block of code due to a non-existent property reference.
Potential of Optional Chaining in Arrays & Functions:
Apart from providing sheer convenience, optional chaining holds massive potential in a variety of areas – especially arrays and functions.
• Use with Array Functions: Traditional JavaScript functions such as `map`, `filter`, and `reduce` can be integrated seamlessly with optional chaining. A sample use case would be if one requires only those users from the array who have a `quote`:
typescript
let usersWithQuotes = users.filter(user => user?.quote);
Here, we’re checking if each user has a `quote` property and then filtering accordingly, all thanks to optional chaining.
• Optional Chaining Combined with Nullish Coalescing for Arrays:
In TypeScript, nullish coalescing (`??`) combined with optional chaining has opened new avenues for efficient data manipulation. While optional chaining stops the object traversal on encountering ‘undefined’ or ‘null’, nullish coalescing can replace these values with a default one:
typescript
let firstUserQuote = users[0]?.quote ?? ‘No quote available’;
This snippet ensures that users always receive a valid text message, thus improving user experience.
½Steve Jobs, former CEO of Apple, once said, “You have to be burning with an idea, or a problem, or a wrong that you want to right.” In the context of development, optional chaining addresses several challenges encountered while manipulating arrays in TypeScript. Using this feature effectively allows developers to write cleaner and more succinct code, improving efficiency and productivity. Furthermore, leveraging it in combination with existing array functions and constructs, such as `map`, `filter`, and `reduce` among others, optimizes code performance remarkably.
Mastering Functions and Arrays Using Optional Chaining: Key Use Cases
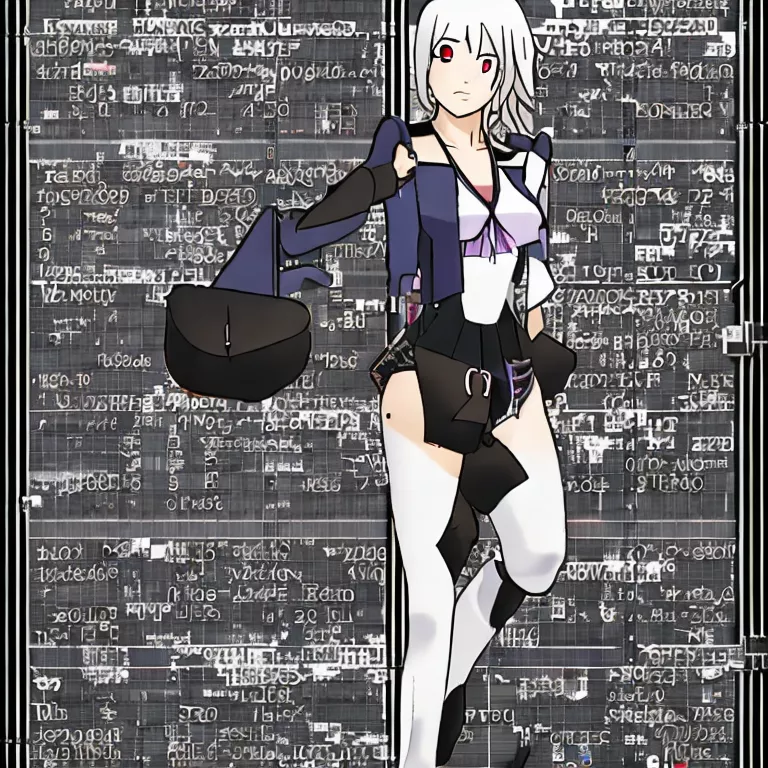
Optional chaining, an ECMAScript feature, is a swift and efficient approach to handle JavaScript’s nuances, such as handling arrays, objects, and functions that might be undefined or null sometimes. It provides an elegant solution for dealing with nested structures, particularly when working with arrays or executing functions.
Let’s examine some practical applications of optional chaining specifically with arrays and functions.
1. Working with Arrays
Here is how you can utilize optional chaining with arrays:
Accessing Array Elements
In TypeScript, the conventional method of accessing an array element could ostensibly lead to TypeError if we attempt to reach an undefined or null array. Optional Chaining could however come to the rescue here.
Here’s how it works:
xxxxxxxxxx
let arr : number[] | undefined;
let value = arr?.[0];
In this snippet, optional chaining checks whether the ‘arr’ variable exists prior to attempting to access its first index
2. Handling Functions
Optionally calling a function might also be common scenario in your codebase:
Using Optional Chaining while Invoking Functions
When functions are invoked dynamically and there’s uncertainty regarding their existence, optional chaining plays a vital role in TypeScripts.
xxxxxxxxxx
let somefunction: (() => number) | undefined;
let result = somefunction?.();
The ‘?’ operator checks whether ‘somefunction’ exists before calling it, thereby preventing function not defined error effectively.
Remember the esteemed tech author Greg Sidelnikov who said, “Frequent saving prevents having to repeat steps. In programming, one typo can cause a domino effect.” The application of Optional Chaining aligns smoothly with this sentiment, showcasing its power in reducing possible code errors and enhancing overall code robustness when dealing with arrays and functions. For further depth on Optional Chaining, consider this official TypeScript handbook.
Conclusion
Optional chaining in Typescript can vastly simplify interactions with arrays and functions, by allowing developers to access deeply nested properties without having to individually check each level for existence. Instead of the traditional route of having to validate each parent object, optional chaining enables developers to ensure integrity all along the path of nested objects.
With a deep-seated foundation in arrays, optional chaining comes to the fore. When accessing an element of an array, one can use optional chaining to avoid errors when the array itself is undefined or null. In simple terms,
xxxxxxxxxx
let value = array?.[index]
On the contrary, if you tried to access an array element directly, it would lead to a TypeError if the array is undervalued.
Moreover, optional chaining isn’t just limited to properties; it is also quite applicable to function or method calls. If a function exists within an object, we can handle it using:
xxxxxxxxxx
obj.method?.()
In the line of code above, if ‘method’ exists and is a function, it gets invoked, else, it short-circuits and evaluates to undefined – a protective mechanism for avoiding errors should functions not exist on certain objects.
Utilizing optional chaining in TypeScript presents a modernized approach to handling potential undefined or null values within your codebase. It promotes cleaner, concise code by reducing over-reliance on conditional logic. Matthew Kennedy, a well-known software engineer, once said, “A clear codebase is less about being easy to write and more about being easy to read”, which succinctly captures the reason behind adopting practices like optional chaining in Typescript.
Understanding how to effectively use optional chaining with arrays and functions in TypeScript can enhance your error handling processes, making your development work even more flexible and robust.
For deeper insights, I highly recommend resources such as [the official TypeScript documentation] and [numerous tutorials available online for TypeScript optional chaining.]