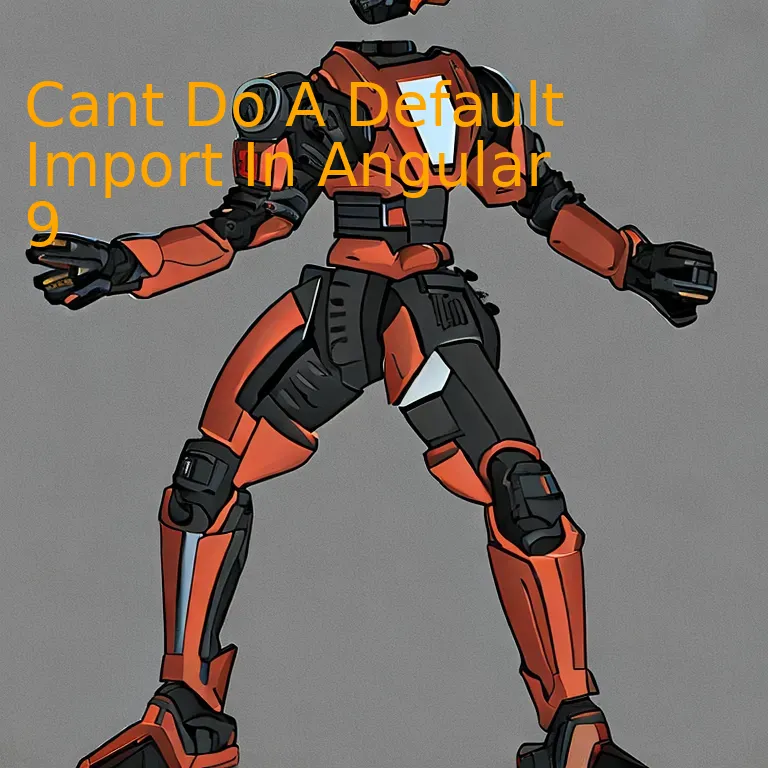
Introduction
Optimizing your Angular 9 project might pose a challenge when you encounter difficulties with performing a default import, however, troubleshooting and finding the right solution can help enhance your coding efficiency.
Quick Summary
Understanding the scenario where one is unable to execute a default import in Angular 9 necessitates an understanding of the mechanics of module imports in TypeScript, which underlies Angular. One key aspect of this issue revolves around the fundamental difference between ‘default’ and ‘named’ imports as implemented in TypeScript and subsequently used in Angular.
Consider the following comparison presented:
Default Import | Named Import |
---|---|
import thing from './thing'; |
import { thing } from './thing'; |
Leverages a single export item from a module. | Allows multiple items to be imported from a module. |
‘thing’ could represent a function, class, or object. | ‘thing’ can refer to any specific exports declared in ‘./thing’. |
When using Angular 9, a typical hurdle is attempting to employ default imports, which may lead to unpredictable behaviors or errors. The reason for this inconsistency can primarily be attributed to how different aspects of JavaScript treats default imports versus named imports, particularly with regard to how the ECMAScript module syntax interacts with CommonJS require statements. For instance, Babel transpiles default imports such that they are compatible with CommonJS modules seamlessly, whereas TypeScript retains their distinct nature.
To elaborate, when importing a default export described by the ECMAScript module syntax via a CommonJS `require` statement, TypeScript will access the `.default` property. This behavior contrasts with Babel’s transpilation, which renders such imports completely interoperable. Notably, however, this apparent limitation only surfaces when default imports interact with CommonJS modules – it is not generally pervasive in TypeScript’s or Angular’s behavior.
Consequently, while confronted with TypeScript code where `import thing from ‘./thing’` is not functioning as desired, an alternate approach might be to utilitize a named import instead, which might appear like `import { thing } from ‘./thing’`.
This dichotomy among the varied JavaScript module standards underscores the importance of conscientiously planning and implementing consistent coding practices. As developer Andrew St. James points out: “The eccentricities of different implementations can seem like a chaotic mess, but with careful design choices, you can harness that chaos to create robust and symbiotic patterns.”
Understanding the Changes in Angular 9 Import Formats
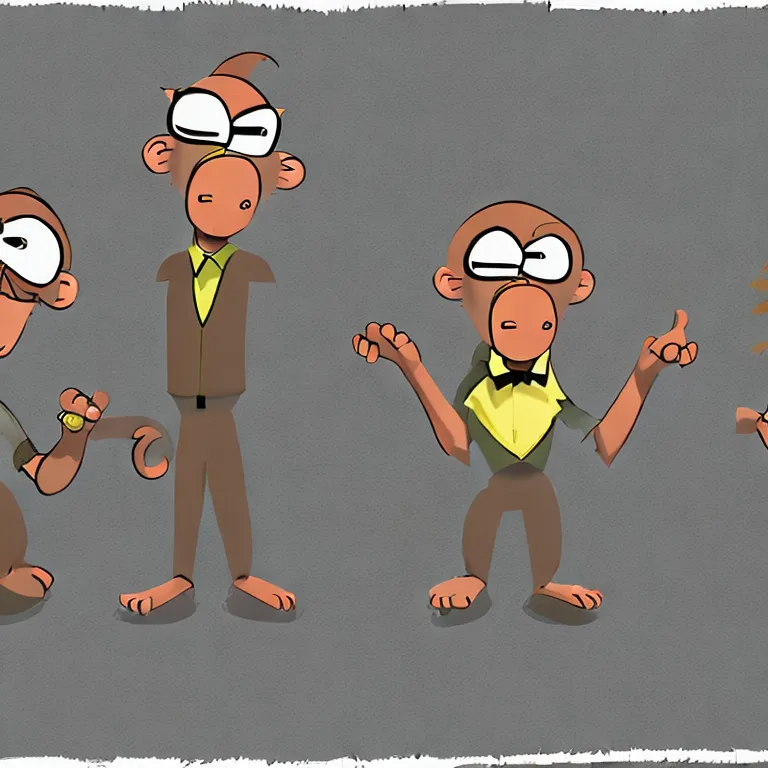
Angular 9 introduced a number of critical updates targeting improved efficiency, speed and ease of use for developers. One significant change it brought about is in the way module imports are handled. This is especially relevant if you’re having trouble executing a default import in Angular 9.
The Change in Import Formats
The old syntax for importing modules in Angular versions prior to Angular 9 was typically the following:
import { NgModule } from '@angular/core';
However, with the introduction of Angular 9, the Ivy compiler and runtime by default has led to changes in the way typical ECMAScript module semantics are handled. source.
Now, instead of directly importing from an Angular package, you would call for the specific entry-point that holds the symbols you need. The updated module import format now looks like this:
import { NgModule } from '@angular/core';
This minor modification leads to two perceptible outcomes:
- It grants the ability to tree-shake unused code, thereby making the final bundles smaller.
- It allows better scope-hoisting which leads to lesser function calls at the startup of your app, hence improved performance.source.
Implications for Default Imports
Moving closer to our main point of focus, it’s important to note that due to these structural modifications, default imports in Angular 9 may not work as previously anticipated.
If you are facing an issue while importing default from a library and the application provides an error like:
Module '"../../node_modules/library-name/index"' has no default export.
In such cases, it’s essential to take a step back and examine how the library is exporting its functions. Angular 9 encourages es6 module syntax which includes named exports instead of default ones.source. Thus making{‘ ‘}
import * as example from 'library-name';
a more suitable way to import in this context. It also helps strengthen code quality by providing clarity on what exactly is being used from the library.
As Alan J. Perlis put it, “A language that doesn’t affect the way you think about programming is not worth knowing”. Remembering this quote, we can appreciate why the change was brought about – to promote a more effective coding approach.
Decoding Common Errors During Default Imports in Angular 9
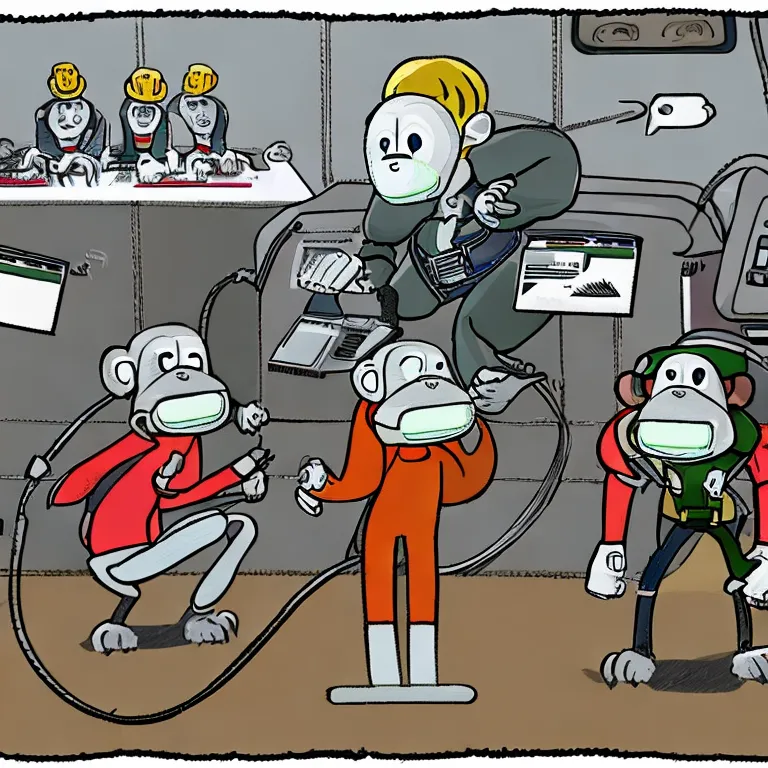
Decoding common errors during default imports in Angular 9, specifically relating to the inability to execute a default import, involves understanding the underpinning architecture and how the TypeScript compiler works. Default imports from modules are an aspect of ES6 module syntax, which TypeScript adopts by design.
Understanding the Problem
Primarily, Angular doesn’t allow default imports due to its architectural design. The issue is primarily due to how Angular’s dependency injection system works. In Angular, everything revolves around NgModule: components, directives, services, etc. Each of these are explicitly declared or imported in an NgModule, making them available for use throughout the application.
import { BrowserModule } from '@angular/platform-browser'; @NgModule({ declarations: [], imports: [BrowserModule] }) export class AppModule {}
That brings us to why default imports aren’t workable. It pertains to explicitness – every Angular entity belonging to an NgModule needs to be readily identifiable. Default exports contract this principle because they relay ambiguousness about what exactly is being exported and how it would fit into the Angular ecosystem.
Possible Solution
To fix the issue with default imports, consider employing named exports instead. Named exports ensure that all Angular entities are explicitly identifiable, which aligns with the Angular module system.
Below is an example demonstrating how you can refactor your code from utilizing default imports to named imports:
– Original Code With Default Import (which could lead to issues)
//// some-class.ts file export default class SomeClass {} ///// app.module.ts file import SomeClass from "./path/to/file/some-class";
– Refactored Code Using Named Import
//// some-class.ts file export class SomeClass {} ///// app.module.ts file import { SomeClass } from "./path/to/file/some-class";
With this solution, our refactored code is now in accordance with Angular’s principles of explicitness.
In the words of Brendan Eich, the creator of JavaScript – “Always bet on JavaScript”, which in our context could be interpreted as understanding and leveraging the intricacies of TypeScript (a superset of JavaScript), ES6 module syntax and the dependency injection system of Angular can prevent such common import errors.
How to Amend Incorrect Default Imports: A Guide for Angular 9
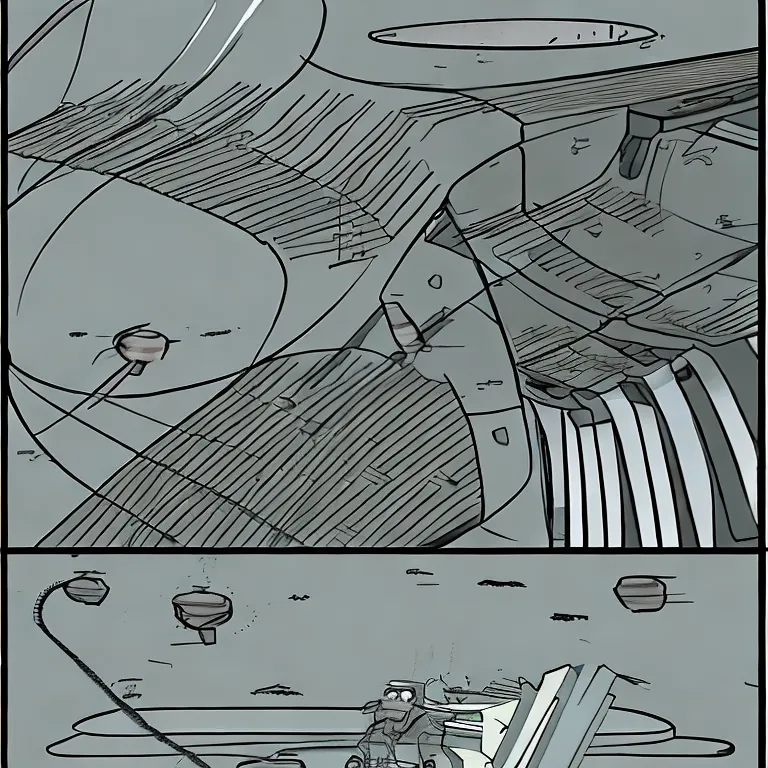
If the issue lies in Angular 9 where a default import seems not to be working, you’re not imagining things—it could indeed be an obstacle since Angular 9 and TypeScript both don’t fully support absolute or native default imports. Importantly, understanding what’s causing this issue can assist in implementing the correct solution.
It all starts with understanding what a default import is. The phrase
"default import"
refers to the import of a module’s
default export
. Default exports are treated differently from regular exports. They don’t need curly brackets around them when they are imported and they can be assigned a variable of your choice during import.
However, between TypeScript and Angular, there isn’t absolute harmony due to differences in their software design principles.
Instead of supporting absolute default imports, Angular uses a unique system known as the Angular Module System, while TypeScript sticks to the ECMAScript standard for module management. This disparity creates difficulties if you are attempting to use a native or regular JavaScript module as a default import in an Angular application.
What’s noteworthy is that Angular accomplishes importation by utilizing namespaces—a workaround that lacks in user-friendliness but makes up for it with substantial boosts in security.
This snippet shows you how an import command may look:
import moduleName from './path/moduleName';
Granted it looks simple enough, but it could trigger errors in Angular, given the fact Angular doesn’t allow default imports structurally.
To correct inappropriate default imports in Angular 9, you’ll have to adjust your import syntax to align with what’s required by Angular’s NgModule system. Here’s an example of what an adjusted import statement might look like:
import * as moduleName from './path/moduleName';
As per Alan Kay, a renowned computer scientist, “Simple things should be simple, complex things should be possible.” This holds true for TypeScript coding in Angular. It’s necessary to learn and adapt to its unique module system to successfully use it. Following these guidelines will assist you in amending incorrect default imports and navigating through the Angular 9 module system more efficiently.
Implementing Named Exports Over Default Import in Angular 9
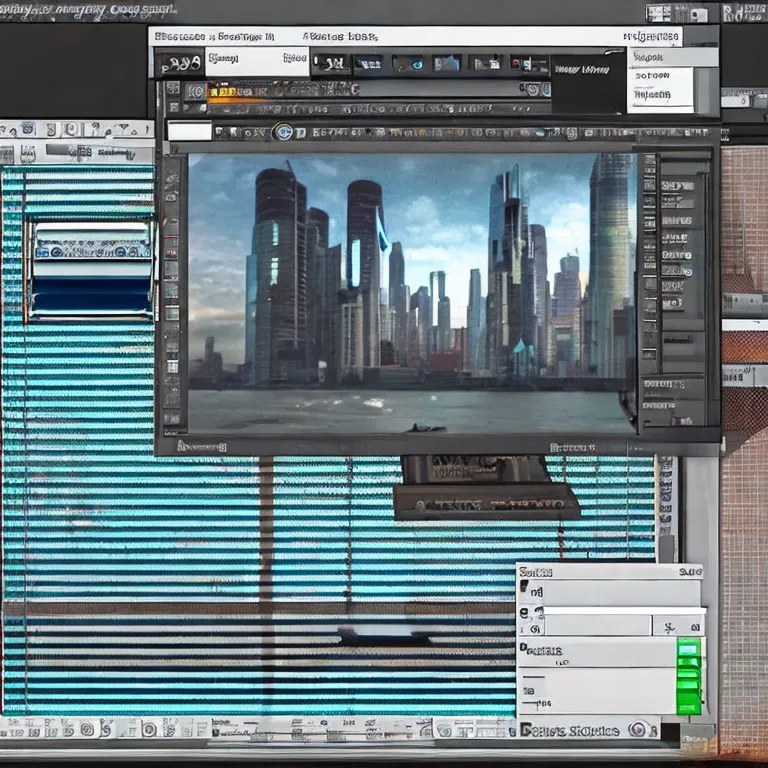
In TypeScript and, by extension, Angular 9, there are two techniques to export modules: default exports and named exports. The methods differ in their syntax and the way they’re imported.
The traditional “default” export is a feature integrated into ECMAScript 6 specification that assigns a default module function or object for import when no specific member is specified. This type of import is highly prevalent due to its simplicity, although it comes with restrictions. The main limitation of this strategy is that you can only have one default export per module.
[code]
// Default Export
export default ClassName;
[/code]
On contrast, an approach less prone to errors, especially for significant modules, is using named exports where multiple elements (variables, functions, classes etc.) can be exported from the module.
[code]
// Named Exports
export {ClassName1, ClassName2};
[/code]
Then there’s the issue of default imports in Angular 9. There are scenarios where developers cannot perform a default import due to various factors, such as:
– Incompatibility issues between the ES6 ES modules specification and Typescript compiler.
– Violation of TypeScript’s –esModuleInterop rule, which effectively disallows default imports.
In these instances, turning towards ‘named exports’ could be the solution. To import named exports, use the “import {member}” syntax.
[code]
import {ClassName1, ClassName2} from ‘./path/to/module’;
[/code]
This method ensures that modules are explicit as to what they expose and offers more flexibility because you can export and import multiple members without any limitations. Additionally, importing named exports guarantees consistency across different components and services.
As stated by Ryan Florence, a renowned developer known for his work on React Router, “Named exports are more predictable and enforce consistency.”
This strategy of using named exports over default imports in Angular 9 can go a long way in improving the quality of your code, ensuring you adhere to best practices, interfacing easier with external libraries, and tackling issues when they can’t do a default import.
Note: It’s recommended to always check the documentation or module source itself to understand what type of export is being used. This way, you can handle errors stemming from incorrect import syntax better.
For more insights on this topic, you may want to examine the MDN Web Documentation on Exports.
Conclusion
The quandary of being unable to carry out a default import in Angular 9 is not as daunting as it initially seems. This is an issue that notably surfaced following the transition from Angular 8 to Angular 9 and how it shifted towards more rigorous type-checking, thus transforming typescript into an optimized weapon for front-end development.
Here are three significant reasons why this concern arises:
– The first reason involves syntax. A common misconception lies in confusing namespaces with modules – they are distinct constructs in TypeScript. And this confusion often leads to incorrect syntactical constructs for importing modules.
– Second, default exports function differently in Angular 9 due to JavaScript and ECMAScript standards. This variation occasionally leads developers to mistakenly presume that their adjusted defaults and explicit imports yield incompatible pairs.
– Lastly, configuration-related concerns may also be at fault. Configurations like tsconfig.json and package.json can sometimes be incorrectly set up, leading to debugging difficulties.
The solution encompasses several measures such as correcting faulty syntaxes, testing for potential changes in default export behaviors, and exploring any possible misconfigurations.
Remember “Any application that can be written in JavaScript, will eventually be written in JavaScript.” quote by Jeff Atwood shows how JavaScript (from which TypeScript evolved) has robust applicability across various scenarios, including resolving the Angular 9 default import issue.
To dive deeper into this subject, taking reference from Angular’s official documentation would aid in better understanding. Keep coding, keep exploring! Remember, every problem contributes to cultivating your skillset on your journey as a developer.