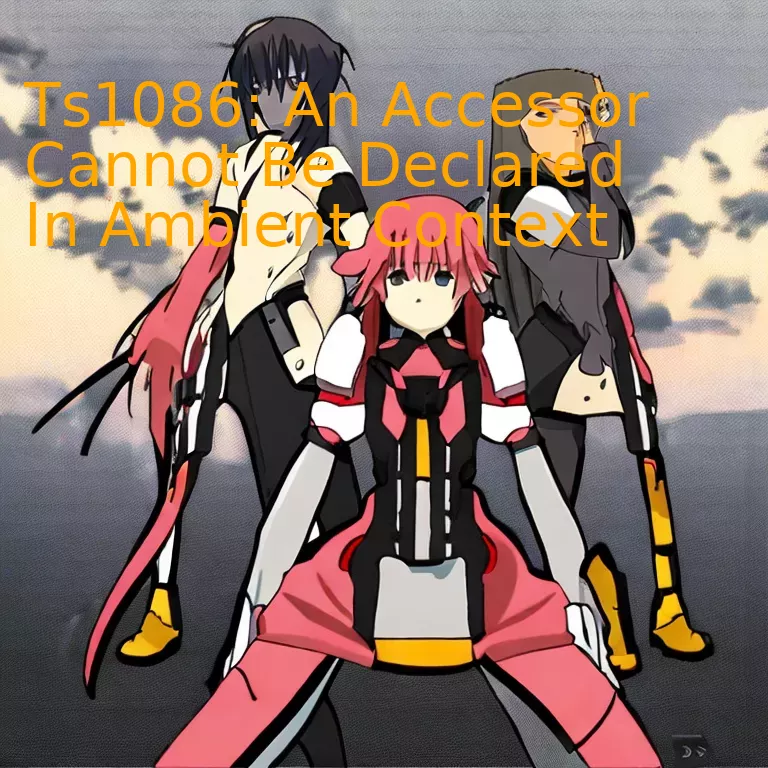
Introduction
In the sphere of TypeScript programming, a common issue that developers often encounter is the TS1086 error, signifying that an accessor cannot be declared in an ambient context, which underscores the necessity to understand the script’s environment and avoid this programming obstacle.
Quick Summary
Understanding TS1086, or “An Accessor Cannot Be Declared in Ambient Context”, involves delving into several key points.
Situation | You’re attempting to employ a ‘getter’ or ‘setter’ within an ambient context in TypeScript |
Error Code | TS1086 |
Mistake | Ambient contexts have many restrictions and accessors aren’t allowed |
Solution | Redefine the code outside of the ambient context or avoid using ‘getters’ or ‘setters’ directly |
To unpack the terminology, an **accessor** refers to a ‘getter’ or a ‘setter’. These are special types of methods in JavaScript (and thereby TypeScript), which help in achieving data encapsulation or controlled access to object’s property.
On the other hand, an **ambient context** is TypeScript way of handling existing JavaScript libraries. You’re saying you want to create a new global variable that exists at runtime, but because it’s not written in TypeScript, you need a way to tell TypeScript compiler about its type. That’s where the **ambient declarations come** in. They simply declare the existence of these already implemented objects so that TypeScript can type-check against them.
However, the ambient context isn’t equipped to handle more complex constructs like accessors. In other words, ‘getters’ and ‘setters’ cannot be declared in an ambient context because they require a genuine implementation, something which can’t exist in an ambient context.
To resolve this, you would have to rearrange your TypeScript code. This may involve moving the ‘getter’ or ‘setter’ outside of the ambient declaration, or even refraining from using an accessor method directly.
As Donald Knuth, an established computer scientist has aptly quoted – “Testing can show the presence of errors, but not their absence.”. It is therefore paramount to rigorously test your code to ensure that such errors do not arise in your TypeScript development phase.
Here is an example of how to implement a getter and setter outside of the ambient context:
class MyClass { private _myField: number; get myField(): number { return this._myField; } set myField(value: number) { this._myField = value; } }
In this example, `myField` cannot be used directly. Rather, you utilize the getters (`get myField(): number`) and setters (`set myField(value: number)`) to retrieve or modify `_myField`. The benefit of this approach is improved control over how `_myField` is manipulated, encapsulating access to it within predefined methods.
Understanding the TS1086 Error: An Accessor Cannot Be Declared in an Ambient Context
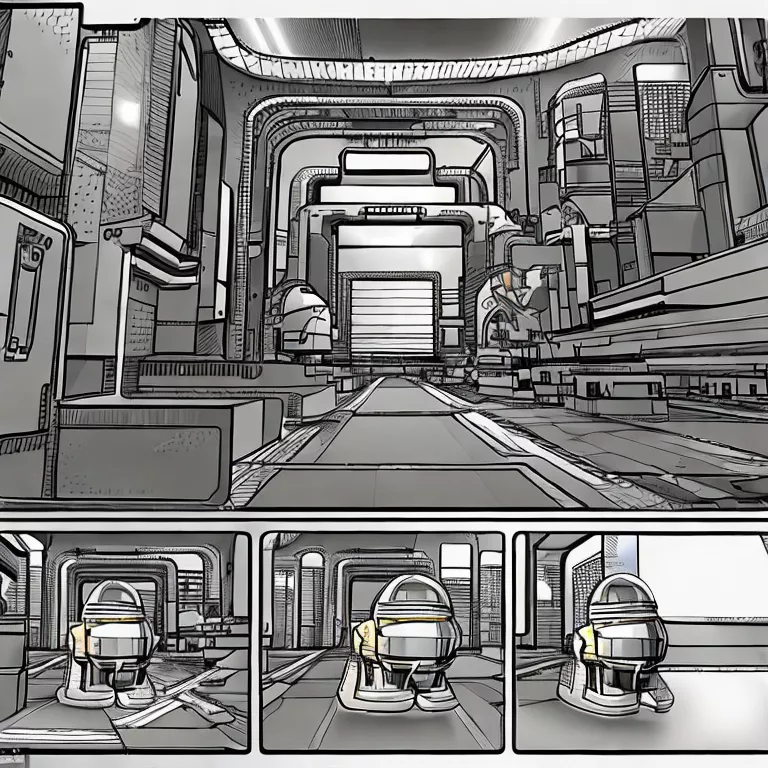
The TS1086 error, or “An accessor cannot be declared in an ambient context” is a common TypeScript pitfall that every developer should understand. This particular error typically occurs when trying to compile TypeScript definitions to JavaScript without the `–declaration` option.
Take for instance, the coding example below where we are attempting to declare getter and setter methods within an interface.
interface Test { get Prop(): number; set Prop(value: number); }
The above code will cause the compilation error: TS1086.
Let’s delve into why this issue arises:
– Involvement of Accessors: Accessors, which include `get` and `set` methods, cannot exist in type declaration files (.d.ts) without actual implementation details. As interfaces don’t contain implementations, this leads to conflict.
– Ambient Contexts: An “ambient context” refers to TypeScript .d.ts declaration files. In these files, all declarations represent types only, and there are no active executable codes, such as function bodies or initialized variables.[1](https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html)
– Compilation Options: As mentioned earlier, if you’re not using the `–declaration` (or `-d`) option while compiling the TypeScript file, it can result in this error, since TypeScript doesn’t generate declaration files by default.
To remedy this, there are a few workarounds. The simplest of which involves removing the accessors from the interface and moving them into a class:
class Test { private _prop:number; get prop(): number { return this._prop; } set prop(value:number) { this._prop = value; } }
Another solution entails settings your tsconfig.json correctly, with `”declaration”: true` in the `compilerOptions` object. This ensures TypeScript will generate corresponding .d.ts files when compiling your project.
In computer programming, technologies and languages hardly ever behave exactly as one expects. They tend to manifest with cryptic error messages and opaque behaviors. As Bill Gates once quipped, “I choose a lazy person to do a hard job because a lazy person will find an easy way to do it.” Unraveling these layers of intricacy certainly cultivates expertise and enhances problem-solving ability in developers. Once we comprehend the cause behind the TS1086 error, we can devise efficient solutions or sidestep potential issues in TypeScript development workflow.
Decoding Error TS1086: Technical Insights into Accessibility and Ambient Context
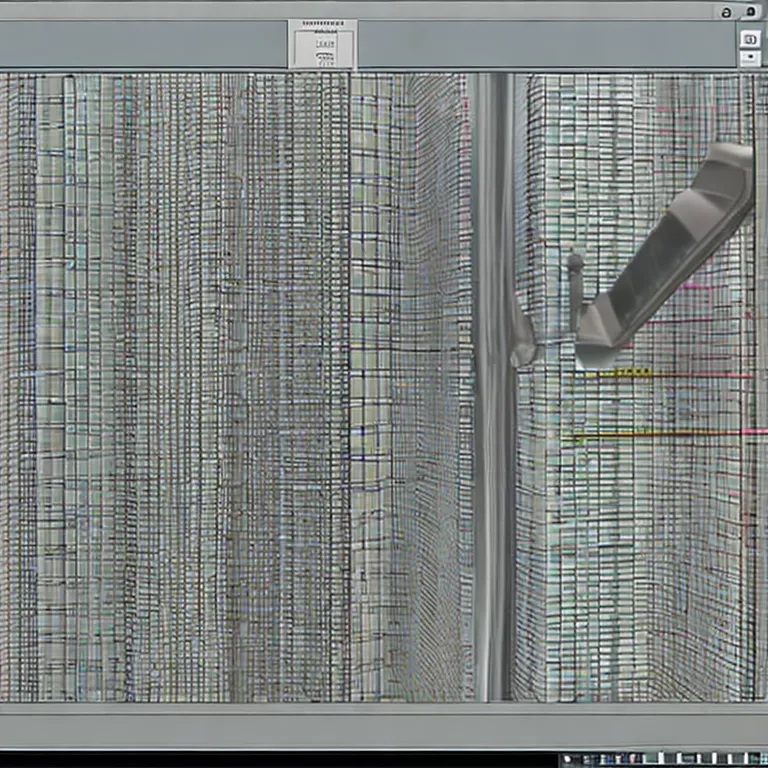
The TS1086 error, colloquially known as “An accessor cannot be declared in an ambient context”, is thrown by TypeScript when it encounters a get or set accessor within an ambient declaration. Ambient declarations are leveraged to signal to the TypeScript compiler that some specific elements – be they variables, classes, or functions – already exist in the global scope.
declare var message: { get text(): string; };
Notably, using a get or set accessor in an ambient context could lead to this error, as seen above. TypeScript does not allow accessors to be used in such cases because it assumes these already-manifested elements would have their value assignment handled externally.
With relevance to Web Accessibility and its importance in software development, we can parallel this error’s significance. In drawing this comparison, the prohibited use of accessors in an ambient context reminds us of how a web application can fail accessibility standards if it tries to tap into user-specific settings without clear consent or appropriate provision for adjustments.
As Aaron Gustafson, a web standards advocate, once said, “Just as our designs today must accommodate all sorts of browsing contexts … they also must be accessible in a broader sense, reaching out to users irrespective of their browsing technology or physical abilities.” This is reminiscent of our case with the TS1086 error: trying to tap directly into an environment beyond the boundaries set by TypeScript results in a failure, just like a web application tapping directly into user settings can violate accessibility laws.
To fix this error in TypeScript, one needs to provide concrete definitions outside the global scope or seek other means of manipulating these elements.
The error-free version for the previous snippet might look like:
var message = { _text: 'Hello, World!', // internally managed get text(): string { return this._text; } };
In this refactored example, the getter function is able to retrieve ‘_text’ from the object because it is no longer in an ambient context, thus avoiding the TS1086 error.
Exploring Solutions to TS1086 Accessor Errors in an Ambient Context
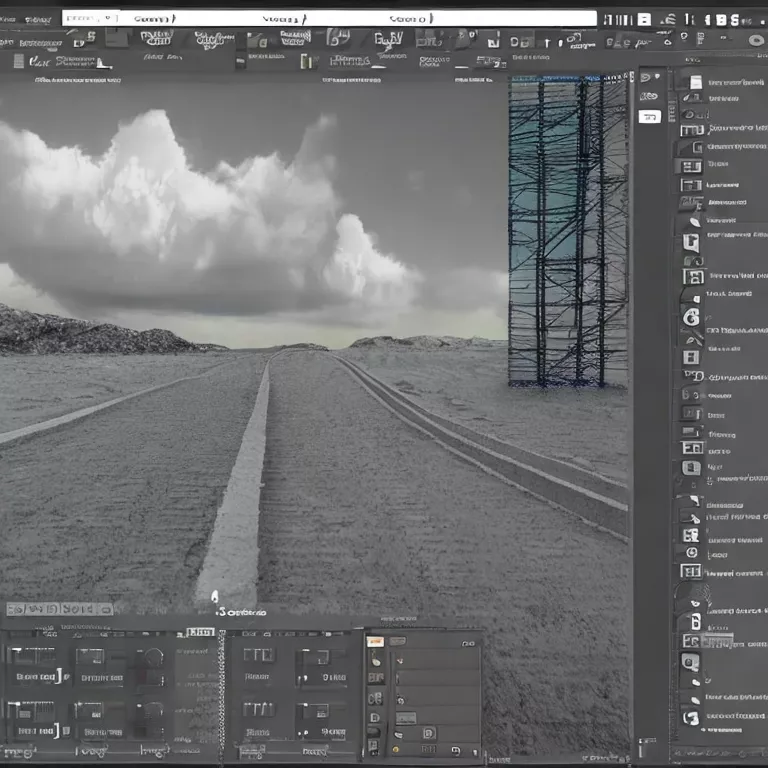
When it comes to addressing the TS1086 error in TypeScript, several solutions are viable. This troublesome message often appears due to a missing JavaScript library file, incorrect setup, or misuse of accessor functions. Understanding and applying these solutions may provide immediate relief, whether you’re a beginner or seasoned TypeScript developer.
Firstly, this error becomes apparent when there’s an attempt to declare an accessor in an ambient context. Ambient contexts in TypeScript are essentially means of including variables that exist in the runtime environment but aren’t part of your codebase.
// This is an example of ambient context. declare var document: Document;
In the above code, TypeScript will know that “document” exists in the global scope but isn’t defined by your Typescript files. However, trying to use accessor (get and set) functions in such a context doesn’t sit well with the compiler. So the primary solution involves avoiding accessor usage within an ambient context.
declare class NotFoundError extends Error { name: string; }
Secondly, TS1086 errors may emerge due to a missing lib in your tsconfig.json file, which can cause problems if your code relies on proposals from ECMAScript that were introduced after ES2015/ES6. Such an occurrence could be resolved by updating tsconfig.json:
{ "compilerOptions": { "lib": ["es6", "dom"], // ... }, // ... }
This will include built-in definitions for both ES6 and DOM APIs, potentially eradicating the TS1086 issue.
Lastly, notifying the TypeScript transpiler about these external dependencies that do not have type definition files via installation of DefinitelyTyped @types package might resolve the issue. Given that TypeScript supports new ECMAScript standards, missing libraries tend to catalyze errors.
As Steve Jobs once remarked, “Everyone in this country should learn how to program a computer because it teaches you how to think.” The solutions above offer a stepping stone to rectifying the TS1086 error, applying the essence of Jobs’ words by incorporating critical thinking into problem-solving.
Please review TypeScript’s Handbook for ambient context and Stack Overflow for more insights. It is also beneficial to visit the DefinitelyTyped GitHub repository for relevant DefinitelyTyped packages. Having these references can help in dissecting and comprehending such technical issues.
The Impact of TS1086 on Development Processes
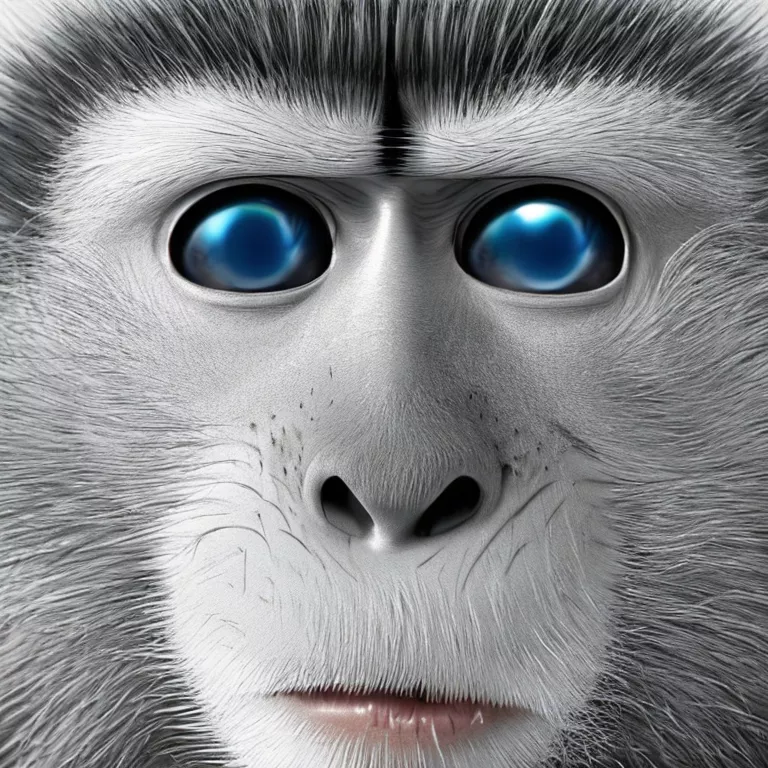
The TS1086 error in TypeScript can create notable effects on development processes that need to be understood and managed. Primarily, this error message appears when a developer tries to declare an accessor (getters or setters) within an ambient context without a body. This throws a wrench into the workflow as TypeScript requires explicit definitions in ambient contexts.
Animate with an example of where developers might encounter the TS1086 error:
declare class ExampleClass { get exampleProperty(): string; //This will cause TypeScript error TS1086 }
To overcome this while maintaining the overall accuracy and correctness of the code, the getter needs to be changed to a method declaration like so:
declare class ExampleClass { exampleMethod(): string; }
Impacts on the development process:
– Learning Curve: Developers unfamiliar with TypeScript may find it challenging to understand why they are experiencing this specific error. This results in increased time spent debugging and self-learning, affecting overall project timelines.
– Code Modularity: When avoiding ‘TS1086’, you may face constraints in organizing your code. Shifting from using Accessors to methods limit the structuring options which could potentially reduce readability of your code.
– Convergence: One primary advantage of TypeScript is that it provides better support for larger scale projects due to its statically typed nature. However, this error may hinder scalability because of extra time needed to deal with it.
As [Eric Elliot](https://ericelliottjs.com/), a JavaScript expert, said “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Despite being a minor error, consistent understanding and rectifying such TypeScript errors like TS1086 helps contribute towards better coding practices in the long run.
Relevant Online References
Understand Accessors: [MDN Web Docs – Defining getters and setters](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Working_with_Objects#defining_getters_and_setters)
Diving Deeper into TS1086 error – [StackOverflow link](https://stackoverflow.com/questions/37365277/how-to-declare-a-class-property-in-typescript)
Conclusion
The notorious “TS1086: An accessor cannot be declared in ambient context” error is a common stumbling point for many TypeScript developers as they navigate the complexities of the language and its type system. Often this error emerges as an obstacle when importing type definitions from certain libraries without included accessors, indicating that TypeScript’s compiler can’t infer the correct typings.
An “ambient context” in TypeScript refers to the environment where a module or script runs. TypeScript has a capability known as ambient declarations, through which we can define types that mirror runtime reality in an idealistic manner. However, doing so requires careful navigation of the TypeScript syntax and semantics to avoid inadvertently stepping beyond its bounds. Accessors (getters and setters) aren’t allowed in declare statements going by the TypeScript design principles – hence the TS1086 error. The parsing difficulty associated with having accessors in declaration ambients makes it unfavorable.
Straightforward solutions to the ‘TS1086’ error could entail either:
• Revising the TypeScript configuration file i.e., tsconfig.json to downgrade the library versions associated with the problematic accessors.
• Alternatively, if applicable, word around the advent by manually working on the accessor functions, therefore avoiding using the defective ambient declares.
// Let the User class look something like this initially: declare class User { get name(): string // This throws a TS1086 error. } // We can rewrite it as: declare class User { name: string }
This mitigates the problem at hand and can be referenced to achieve the desired result.
“The key to effective TypeScript code is understanding the environment you are working in and making full use of the language’s rich typing capabilities.” – Ethan Brown, Web Development with Node & Express