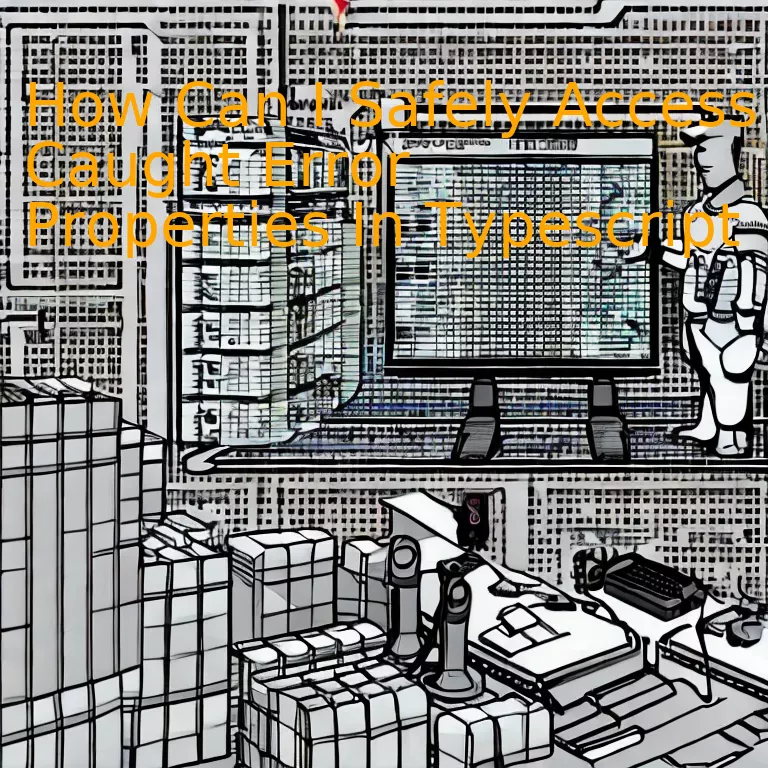
Introduction
To safely access caught error properties in TypeScript, you’ll need to exploit TypeScript’s error handling capabilities, which assist in identifying abnormal or unexpected events that may occur during code execution and provide a systematic way to capture and handle such instances for optimized performance and safe results.
Quick Summary
While working with TypeScript, one can encounter situations where it becomes crucial to access properties from caught errors safely. However, JavaScript and by extension TypeScript treats all thrown objects as errors, but not all of these objects have relevant features like `message` or `stack`.
Here’s a way to approach this:
html
Error Handling Case | Code | Description |
---|---|---|
Instance of Error Class |
x try { // code that throws error... } catch (error: any) { if (error instanceof Error) { console.error(error.message); } } |
The method checks if the caught ‘error’ is an instance of Error class. This allows us to safely access ‘message’ property on the error. |
User-Defined Error Type |
xxxxxxxxxx class CustomError extends Error { constructor(public extra: string, message?: string) { super(message); } } try { // code that throws CustomError... } catch (error: any) { if (error instanceof CustomError) { console.error(error.extra); } } |
If you’re working with errors of a specific user-defined type like ‘CustomError’, you can use ‘instanceof’ to determine its type and handle accordingly. Custom properties can then be accessed without type issues. |
This table comprises two error-handling Instances named *Instance of Error Class* and *User-Defined Error Type*. In the Code column, it demonstrates how to use TypeScript’s error-handling syntax to manage these errors. Descriptions offer deeper insights into what each code snippet is performing.
The former instance showcases how one must check if a caught ‘error’ is an actual instance of the Error class before proceeding to access properties safely such as `message`.
The latter instance example demonstrates how TypeScript developers handle custom errors using user-defined error types—where, again, correct identification using `instanceof` helps in handling different types and subsequently accessing their custom properties securely without issues associated with type.
As famously quoted by Martin Fowler: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This holds very true for error handling in programming. Understanding and implementing these approaches should effectively help in managing error properties in TypeScript.
Unveiling the Concepts of Error Handling in TypeScript
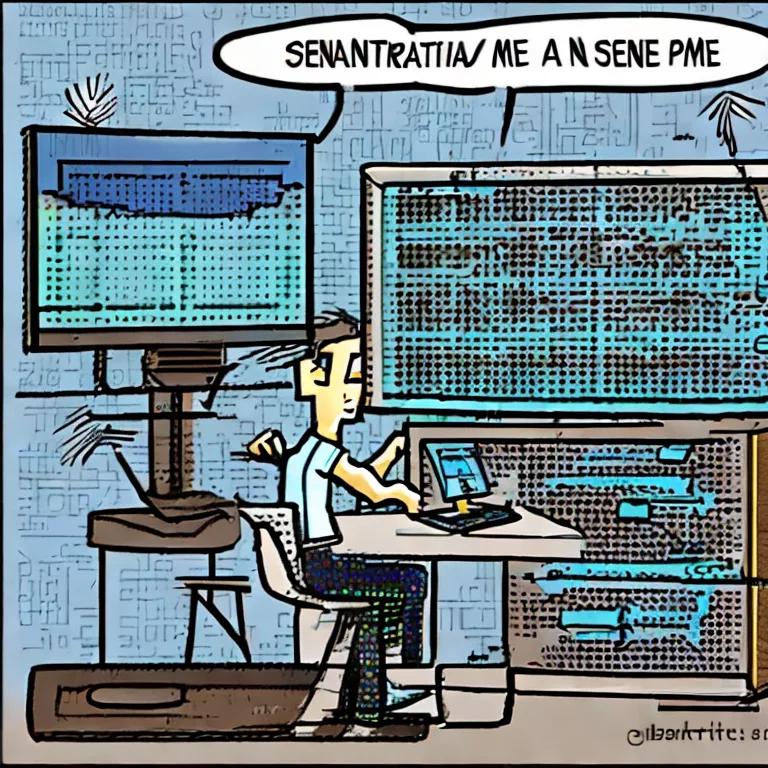
Handling errors in TypeScript introduces a method of dealing with unexpected or exceptional runtime conditions. For developers wanting an in-depth perspective on how to securely tap into caught error properties, understanding these concepts is vital.
For starters, let’s take a peek at the standard structure of a TypeScript error:
class MyError extends Error {
constructor(message?: string) {
super(message);
this.name = “MyError”;
}
}
Here, `this.name=”MyError”;` is key. This line changes the name property from ‘Error’ to the class name, ensuring you can easily identify the type of error that occurred by checking the name property during error handling.
Now coming onto safely accessing caught error properties, it is necessary to know that a generic catch clause will treat any caught exception as having the type `any`. Thus, TypeScript cannot make assumptions about what properties might be available.
One way around this, however, is type guards[1](https://www.typescriptlang.org/docs/handbook/advanced-types.html#type-guards-and-differentiating-types). Here’s an example:
typescript
catch (err: any) {
if(“name” in err) console.log(err.name);
}
In the above example, we are using the `in` keyword as a type guard – this helps us ensure that the `name` property exists on the error object before trying to access it, avoiding potential undefined references at runtime.
Another approach would be to implement a custom type guard function to help facilitate error type differentiation and alleviate error handling complexity:
typescript
function isErrorWithMessage(error: any): error is { message: string } {
return error && typeof error.message === ‘string’;
}
try {
// …code which throws an error…
} catch(e: unknown) {
if (isErrorWithMessage(e)) {
console.error(e.message);
}
}
In this example, isErrorWithMessage acts as a type guard and helps TypeScript recognize the object’s shape. Hence, we safely access the error properties.
However, do remember that while these methods help safely access properties on Error types in TypeScript, it’s best practice to always type check before trying to access any property[2](https://basarat.gitbook.io/typescript/type-system/typeguard).
As adorned computer scientist Edsger W. Dijkstra said, “Testing shows the presence, not the absence of bugs.” Do take heed when dealing with Error properties, ensuring you incorporate a combination of thorough testing and sound coding principles alongside TypeScript’s robust features for best results.
Understanding Property Access in Caught Errors: A Deep Dive into TypeScript
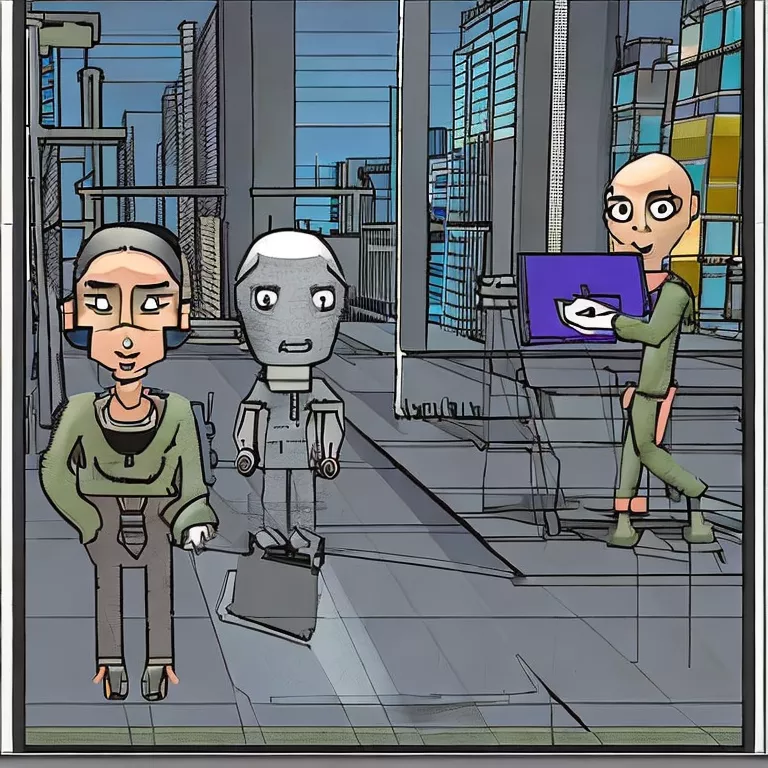
To safely access caught error properties in TypeScript, developers need to keep a few important concepts in mind.
One of the significant advantages TypeScript offers over JavaScript is its strict typing system and enhanced error handling features. It supports proper function and variable typing and throws compile-time errors whenever incompatible types are used.
But what happens when you interact with an error object? If your program execution is stopped due to an exception (a runtime error), TypeScript makes it possible for you to catch this error and handle it safely.
The common structure of handling errors in TypeScript embraces “
xxxxxxxxxx
trycatch
” similar to other programming languages:
Code Snippet |
---|
xxxxxxxxxx <script> try { // risky code } catch (error) { console.error(error); } </script> |
By default, the error object holds little type information that TypeScript can use, so accessing properties might lead to compile-time errors. Here’s where you must employ precise methods – Error casting or User-defined Type guards, enabling safe access to caught error properties.
Error Casting: This approach utilizes as keyword for explicit conversion of one type to another.
xxxxxxxxxx
try {
// risky code
} catch (error:any) {
console.error((error as Error).message);
}
It’s worth noting though, using ‘any’ diminishes the benefit of TypeScript’s static typing and hence seen as a bit antithetical to the spirit of TypeScript.
User-defined type guards: You could define a type guard to validate whether the caught error has the expected properties-
xxxxxxxxxx
function isErrorWithMessage(error: unknown): error is { message: string } {
return typeof error === 'object'
&& error !== null
&& 'message' in error;
}
try {
// risky code
} catch (error) {
if(isErrorWithMessage(error)) {
console.error(error.message);
}
}
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler[1].
Exploring these techniques, using TypeScript for robust exception handling and the safe accessing of caught error properties becomes more streamlined. By leveraging TypeScript’s static typing, we safeguard our program against various runtime complications.
Do keep in mind, that while TypeScript offers a level of compile-time safety, ultimately, it’s JavaScript that runs in your user’s browser. So, all TypeScript specific syntax, including type annotations and interfaces, will essentially be discarded upon compilation. Hence, using the techniques mentioned above would ensure your program remains secure from potential runtime errors upon deployment.[2].
Use proper error handling in your TypeScript codes to make it not just computer-readable but human-understandable as well.
Best Practices for Safely Accessing Error Properties in TypeScript
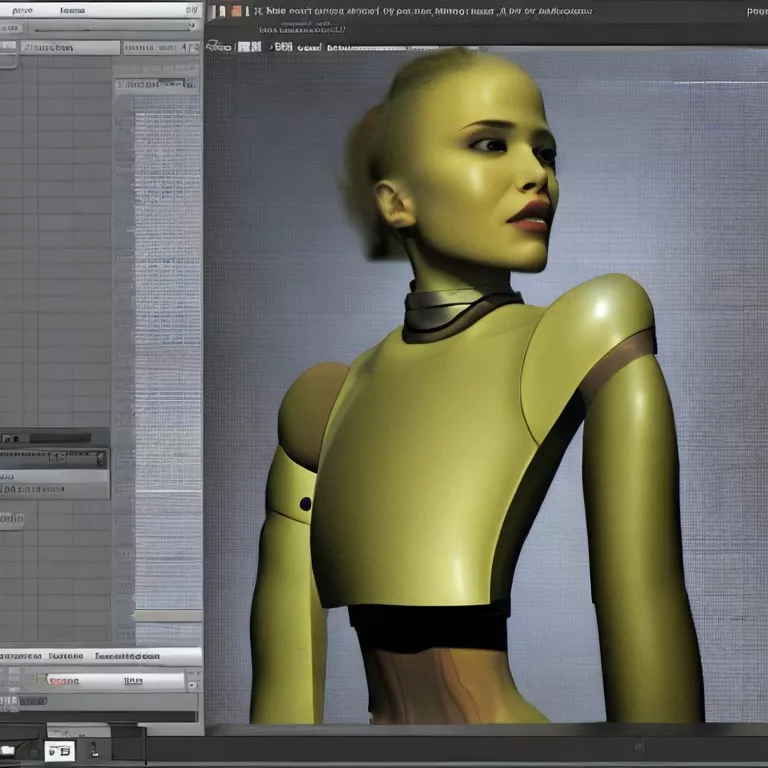
Accessing error properties in TypeScript can be tricky due to the existence of both built-in JavaScript errors and user-defined exception classes. Depending on the function, an error may be an instance of `Error`, or it could be a primitive value like null or undefined. To prevent run-time errors due to accessing non-existent properties, you’ll need to handle these scenarios safely.
Here are some best practices:
– **Never assume property existence**: Given the dynamic nature of JavaScript, any object including an Error object might lack certain properties. Always check for the existence of a property before trying to access it.
`
xxxxxxxxxx
`
if (typeof error === 'object' && error !== null && 'message' in error) {
console.error(error.message);
} else {
console.error(error);
}
`
`
– **Use type guards**: Type guards allow TypeScript to infer whether a particular type is a boolean, string, number, symbol, null, or undefined at compile-time. A common way to create a type guard is with the typeof operator. This can be expanded by including custom logic in the same function.
`
xxxxxxxxxx
`
function isError(value: any): value is Error {
return value instanceof Error;
}
if (isError(error)) {
console.log(error.message);
}
`
`
– **Leverage Union types and Type Discrimination**: TypeScript’s union types let you specify that a value can be one of several types; combined with type discrimination, this allows for safer handling of various possible types of errors:
`
xxxxxxxxxx
`
type ApiError = { status: number; message: string };
type NetworkError = { offline: true; message: string };
type ErrorType = Error | string | null | undefined | ApiError | NetworkError;
function handle(error: ErrorType) {
if (error instanceof Error) {
console.error(error.message);
} else if (typeof error === 'object') {
if ('status' in error) {
console.error(`API Error: ${error.status}`);
}
else if ('offline' in error) {
console.error(`Network Error: ${error.offline}`);
}
} else {
console.error(error);
}
}
`
`
– **Default and Fallback Values**: Even with thorough type checking, there’s always a possibility of unknown error types. A default or fallback value can prevent application crashes in such scenarios:
`
xxxxxxxxxx
`
function getErrorMessage(error: any): string {
if (error instanceof Error) {
return error.message;
}
if (typeof error === 'string') {
return error;
}
// Fallback message
return 'An unknown error occurred';
}
`
`
It’s important to remember Robert C. Martin’s quote: “A code is like humor. When you have to explain it, it’s bad.” Simple, clear, and defensible code is often the safest way to handle errors in TypeScript.
These are some of the ways to safely access caught error properties in TypeScript. High-quality coding practices will ensure that your programs deal robustly with different error scenarios, creating applications that recover gracefully from errors.
Ascertain Secure Approach to Retrieve Caught Errors’ Attributes with TypeScript
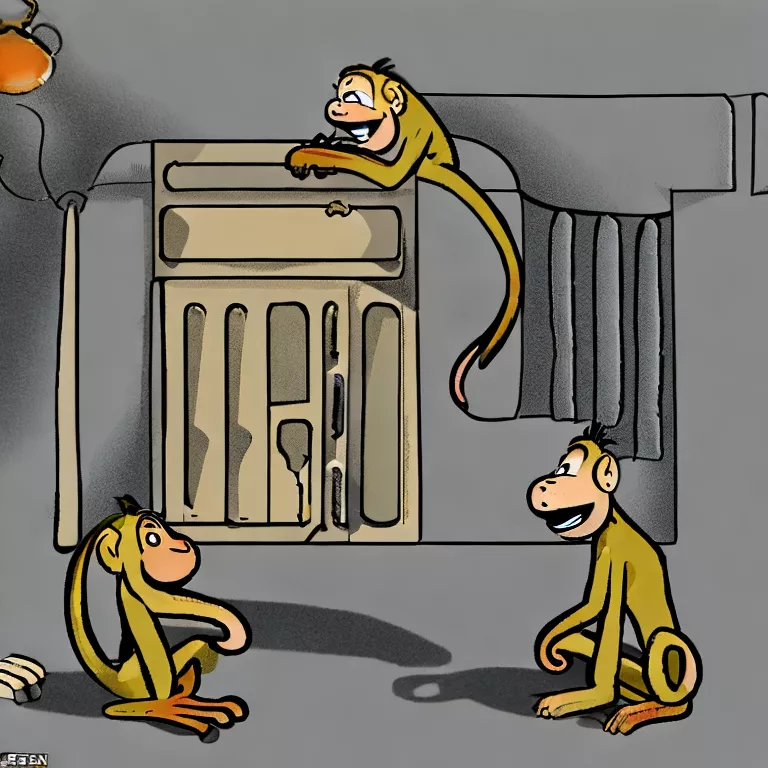
When designing software with TypeScript, it’s critical to address and manage exceptions properly. This will ensure the robustness of your application, prevent potential crashes, and provide useful feedback if any error does occur.
Accessing the properties of caught errors in TypeScript can often be a challenge due to the variable nature of JavaScript errors. Generally, when you catch an error in TypeScript or JavaScript, you have an object which may contain unique properties but is generally typed as just “any”. To tackle this, the modern version of TypeScript has introduced `unknown`, a new type that is more type-safe and secure than `any`.
Here’s how we can safely access caught error properties using the `unknown` type:
html
xxxxxxxxxx
try {
// insert code here where the error might be thrown
} catch (error: unknown) {
if (error instanceof Error) {
console.log(error.name);
console.log(error.message);
} else {
console.error('Caught throwing non-error');
}
}
In this example, we use the keyword `unknown`. When we catch an error, we don’t necessarily know what the structure of the error object is – whether it possesses certain properties or not. So instead of typing error as `any`, we type it as `unknown` to build a more secure and robust approach. Then, we verify if the caught object/error is an instance of the Error class before attempting to access the `name` and `message` properties.
It’s worth noting that we should carefully consider which attributes of a caught error we are accessing. Undoubtedly, consistently using a secure and type-guarded approach such as this for handling errors in TypeScript ensures that:
– The code remains resilient against unanticipated situations.
– The integrity of your application is preserved, leading to fewer unexpected crashes at runtime.
– User-driven actions can be reliably captured and processed.
– Enhances the overall quality and trustworthiness of the developed software.
As aptly highlighted by Jason Williams, a seasoned software developer, “Errors are not always negative. Sometimes they are your best guide. Handling them right is craftmanship.” Given this perspective, it’s clear why ensuring secure and proper error handling in TypeScript is considered critical.
Conclusion
Navigating the landscape of error handling and understanding how to access caught error properties in TypeScript is a rewarding, yet multifaceted topic.
– When an error is thrown in JavaScript, it becomes an object embodying nuances that includes pertinent properties such as
xxxxxxxxxx
message
,
xxxxxxxxxx
name
, and
xxxxxxxxxx
stack
. Leveraging these properties can augment our debugging process by conveying valuable contextual information about the error.
Error Property | Description |
---|---|
xxxxxxxxxx message |
Offers a human-readable description of the error event |
xxxxxxxxxx name |
Specifies the type of error that occurred |
xxxxxxxxxx stack |
Returns a trace that represents where the error happened |
– The beauty of TypeScript is in its utilization of static type-checking, including error objects. Unfortunately, TypeScript does not naturally discern the properties nested within a caught error; hence a type assertion may be required – essentially instructing TypeScript what type it should expect, facilitating safe access to those properties.
For instance, consider the following code:
xxxxxxxxxx
try {
// Code that may provoke an error
} catch (e: any) {
console.log(e.message);
}
Here, ‘e’ is declared with a type ‘any’, effectively silencing TypeScript’s inability to infer and verify its structure. Though this may seem counterintuitive given TypeScript’s emphasis on robust typing, it permits safe access to error properties (e.g., ‘e.message’).
To paraphrase Douglas Crockford – an influential figure in the field of JavaScript development – “TypeScript is a surprisingly well designed language. It’s more reliable, more comfortable to work with, and has broad implications for application reliability and scalability.”(source)
By way of understanding its sophisticated type system, one can fully enjoy TypeScript’s benefits. Crucially, although it may appear that we’re battling against TypeScript when handling caught errors, remember that the language features are ultimately there to protect us – ensuring, as far as possible, the safe execution of our code and the extraction of valuable debugging information from error properties.