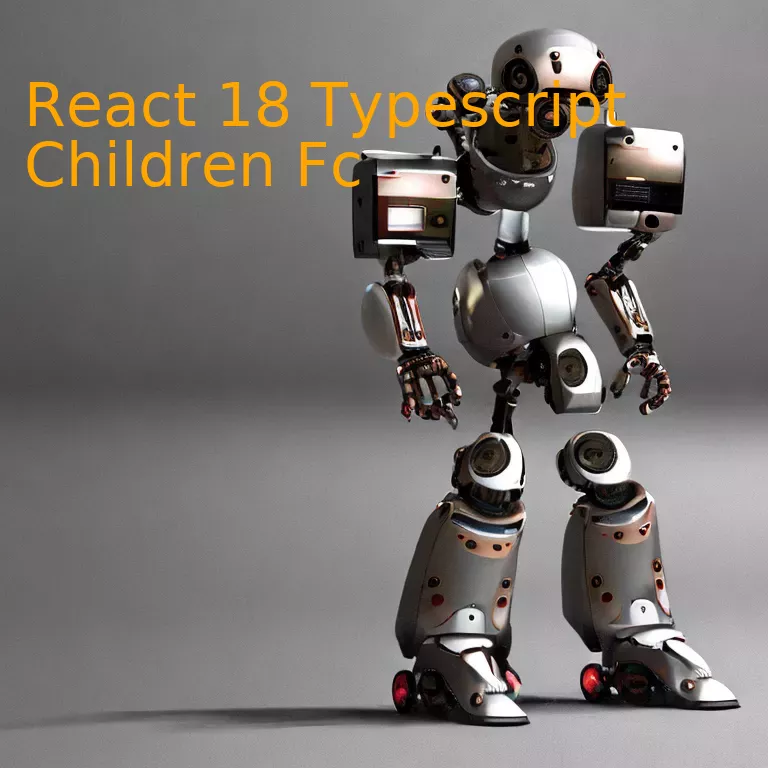
Introduction
Leveraging React 18 along with Typescript enhances the performance of Children Functional Components (Fc), thereby ensuring a user-friendly and efficient application.
Quick Summary
React 18 Typescript Children Fc, better known as Function Components for React library using TypeScript and specially built with a focus on handling children elements, showcases an incredible mixture of precision, clarity, and high functionality that developers need to explicitly define types for their props, including children.
Before delving into its nuances, it would be prudent to first provide an overview through a well-structured table. The underlying intent is to help users grasp the structural intricacies faster and foster a deeper understanding of every operation involved in this technology.
Element | Explanation |
---|---|
React 18 | The latest version of Facebook’s popular JavaScript library for building user interfaces. |
TypeScript | A typed superset of JavaScript that makes it easier to write reliable code by catching bugs early. |
Children | In React, ‘children’ refers to the arbitrary components passed to a parent component during invocation. |
Fc – Function Components | A function-based way of writing components in React using TypeScript; they often use hooks to maintain state and lifecycle methods. |
Now let’s focus on how React 18 handles TypeScript Children Function Components (Fc). When working with TypeScript, it’s essential to specify the type definitions for the various React components, including but not limited to the children prop.
For instance, examining a traditional TypeScript function component written for React, you might observe a component structured like this:
type Props = { children: React.ReactNode; }; const MyComponent: React.FC= (props) => ( {props.children});
In the code snippet, React.ReactNode is used to type the ‘children’ prop, meaning that it can receive any valid JSX, be it a string, a number, null or undefined, an array of these types, or even another React element. This approach provides a high level of flexibility.
The utilization of TypeScript with Function Components (FC) in React 18 offers a broad array of possibilities. As Steve Jobs once said, “Everybody in this country should learn how to program a computer… because it teaches you how to think.” Learning and understanding this technology can indeed reshape how we approach programming tasks systematically and logically.
Unraveling the Basics of React 18 with TypeScript
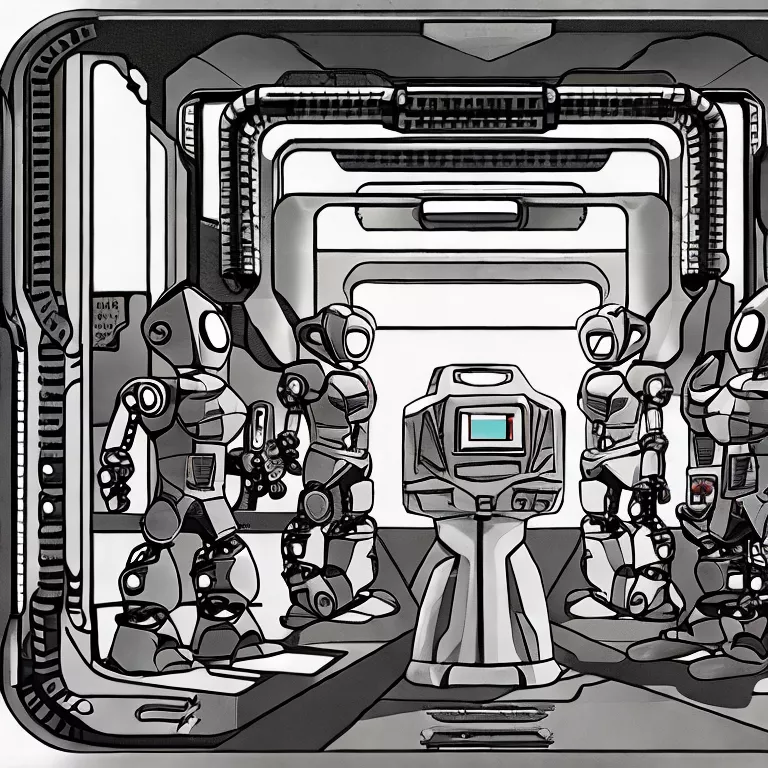
React 18 introduces several innovative features that enhance the performance and user experience of your application while coupling TypeScript with reactive programming, bringing static types and modern JS functionality to your coding efforts.
When talking about the relevance of React 18 with TypeScript specifically in relation to Children and Function Components (FC), we delve into the intricacies of writing functional components with children property in TypeScript.
Understanding Functional Components (FC)
Function Components are a simpler way to write components that purely take props and render. Suppose we have a ‘MyComponent’. Here’s how you could define your function component using TypeScript:
type Props = {children: React.ReactNode}; const MyComponent: React.FC= ({children}) => { return ( {children}); };
In this component, the children prop accepts any valid React child elements to be rendered within this
The use of TypeScript with FC
TypeScript facilitates robust type-checking for components’ properties and states, making debugging and code refactoring much easier. With this, you can strongly type the children props as React.ReactNode while defining your functional component.
When interacting with TypeScript and leveraging it within the framework of React 18, you’re enhancing your ability to manage intrinsic complexities associated within the larger application constructs, especially when dealing with nested children components.
Implications and Considerations
Traditionally, handling children within functional components could become an intricate task demanding immense caution; with TypeScript, however, you equip yourself with a type safety net.
React 18 introduces concurrent rendering capabilities, which affords your applications a smoother user interface without unnecessary blockages. It is important to know how to correctly type your functional components and their child components when leveraging these new features.
However, despite the myriad benefits, it’s crucial to remain cognizant of possible challenges. For example, TypeScript might somewhat steepen the learning curve, or, there can be some complications in typing higher-order-components.
As Kent C. Dodds, a renowned JavaScript software engineer and teacher puts it: “TypeScript can catch bugs before they even occur and guide you towards writing better quality code”. Considering the benefits TypeScript brings to the table, and with the greater context of maximizing new functionalities of React 18, endeavoring to master its interoperability becomes increasingly vital.
For more insights on this topic you can check the React+TypeScript Cheatsheets, These cheatsheets provide a comprehensive overview for using TypeScript with Functional Components in React.
Exploring Children in Function Component (FC) through React 18 and Typescript
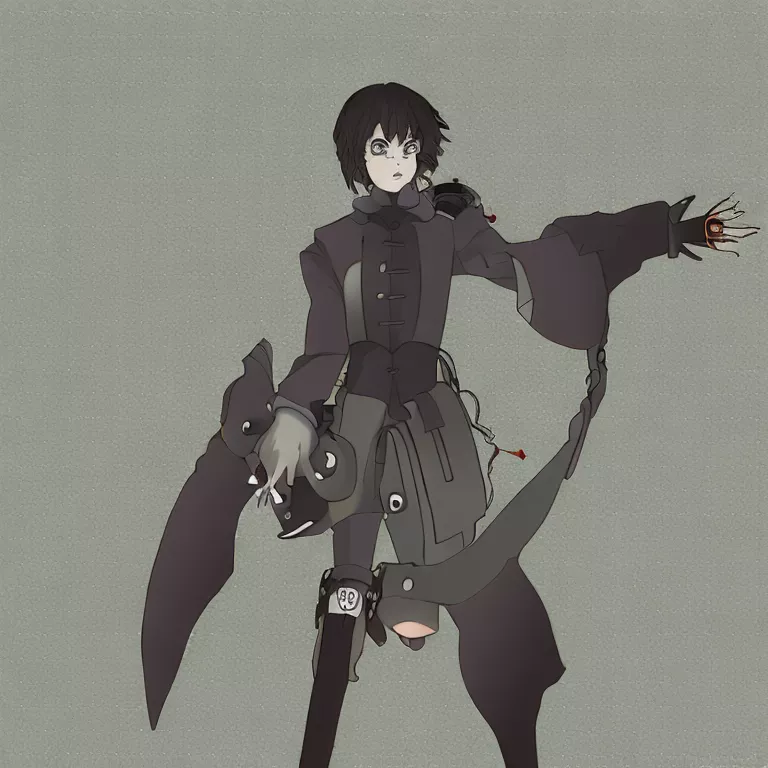
The exploration of children in Function Component (FC) with the developmental leaps created by React 18 and Typescript paves new paths regarding front-end development. The synergy from these computing technologies forms a critical aspect of modern web design applications that embraces seamless user experiences, adapting best practices, code maintainability, as well as integrating performance optimizations.
It is highly essential to conceptualize first the idea of “children” within the context of React. It refers to a unique property given to components which allows for data propagation between multiple levels or layers. In essence, they are mechanism to transmit data to components – an intrinsic attribute of “props”.
Let us delve deeper into what this means when implementing React development using the pattern: Function Component through Typescript.
The basic syntax of a function component can be detailed as follows:
const FunctionComponentExample: React.FC<Props> = ({ children }) => { return ( <div> {children} </div> )}
As seen above, we denote the usage of ‘children’ directly inside the functional component’s argument. This represents the content placed inside the tags of your custom JSX component, allowing programmers to nest additional components or elements as children.
One exceptional feature of utilizing ‘children’ in React is flexibility. For instance, consider creating re-usable layout components like ‘Card’ or ‘Container’. Here, styling and structure could be encoded into the parent function component and the ‘inner content’ could be passed as children.
In line with his philosophy on accessibility, Josh Comeau emphasized:
“Simplicity is prerequisite for reliability.”[1]
React 18 builds on this premise by the inclusion of advanced APIs such as *startTransition*. This feature immensely enhances rendering capabilities and UX in a React application, providing an augmented opportunity to build even more dynamic children props while using Typescript and React.FC.
Furthermore, Typescript empowers developers to create function components with type-checked ‘children’. Simply put, every child that passes through can be defined and checked with Typescript mechanisms – ensuring that only the expected elements appear as children.
type Props = { children: React.ReactNode }
This careful application of children in Function Component alongside annotations provided by Typescript, permits the creation of flexible and reusable functions/components. Therefore, it’s becoming increasingly ubiquitous in creating high-performance, exceedingly interactive web apps utilizing React 18 and TypeScript.
Keep in mind that these concepts must align with the goals of any project being undertaken – considering the efficiency of code maintainability, UX factors, and appropriate tools for task completions within the context of React 18 and TypeScript.
While React 18 and Typescript might present a high learning curve, mastering their use is a rewarding journey. The exploration of “Children” in FC via these technologies mirrors the vast range refreshingly adapting to the trends of our present digital space.
Learn More:
1.React+TypeScript Cheatsheets: This guide comprehensively covers a lot more on propTypes, including commonly used practices with TypeScript in the world of React.
2.JSX.ts Documentation: A more in-depth overview on how TypeScript works around JSX tags setup.
3.React ‘children’ API: Delve in deeper into the React library’s ‘children’ documentation.
—
[1] Josh Comeau, Front-end developer & Educator at Concordia bootcamps.
Harnessing the Power of FCs in TypeScript using React 18
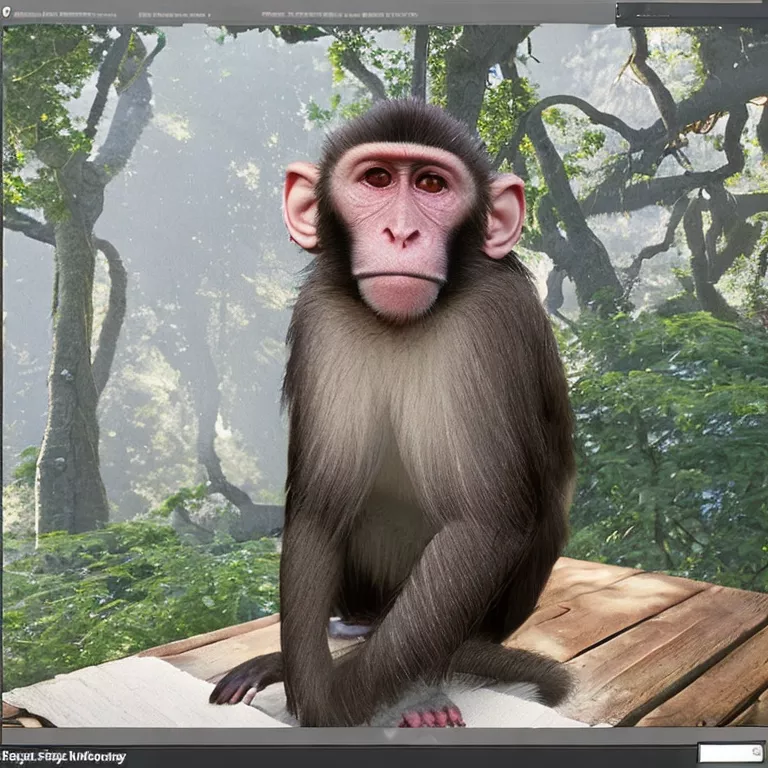
Harnessing the functional components (FCs) in TypeScript while using React 18 can enhance your app’s productivity and efficiency. Functional Components, widely known as FCs in the world of React, offer a simpler way to build components than using classes.
Understanding FCs in TypeScript
FCs represent a function that accepts props and returns a React element. They provide a simpler and cleaner syntax for building components.
const MyComponent: React.FC<{ text: string }> = ({ text }) => { return <div>{ text }</div>; };
In this code snippet, `React.FC` defines the component’s type. Inside <>, we define the props’ properties, and inside {}, we destructure these properties.
This clear construction promotes seamless integration with TypeScript, which is indispensable in enforcing types for props. So you get the power to ensure that the right kind of data is passed into the components for smoother execution. These advantages significantly ramp up the coding speed and quality.
Enhancements in React 18
With React 18, improvements have been made in terms of APIs, concurrent rendering, suspense, transitions etc. Developers will appreciate the fact that it allows for a more natural interaction between state management tools and UI states – all of which are quickly achievable due to the strong typing system present within Typescript.
Utilizing FCs with Children Prop
The type `React.FC` draws its strength from expressing the children’s type explicitly. The ‘children’ prop is unique and signifies any child elements passed into the component.
Let’s consider an example:
interface IProps { children: JSX.Element[] | JSX.Element; } const ComponentWithChildrenAsFc: React.FC= ({children}) => { return ( <div> {children} </div> ); };
In this code block, the `ComponentWithChildrenAsFc` is a functional component that accepts children. It represents a wrapper for ‘children’, thus leading to reusable and manageable code blocks as components.
Without a doubt, the interplay between TypeScript and React FCs can be truly beneficial in creating versatile applications with reliable, type-safe components.
As Martin Fowler, an accomplished software developer and author, rightly said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
This quote holds plenty of significance when it comes to leveraging TypeScript with React. The integration of strong types makes your code more legible, maintainable, and efficient. Moreover, when you apply these advantages in the context of functional components, especially with advancements in React 18, the results can be remarkably powerful and rewarding.
Implementing Child Components Effectively: An Exposé on TypeScripts, Functions & React 18
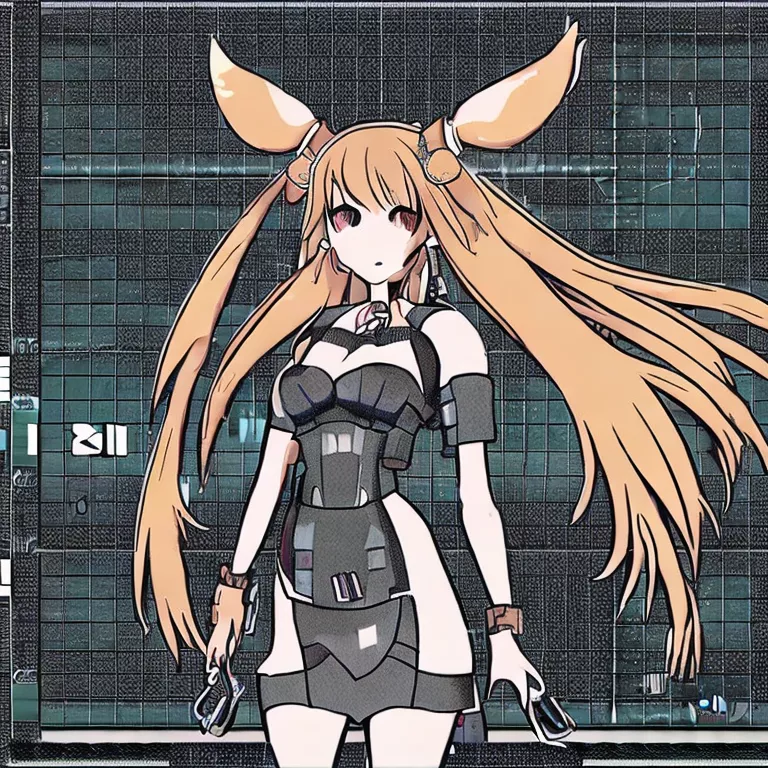
Implementing Child Components Effectively: TypeScript, Functions & React 18
With the release of React 18 and its forward-thinking features, there is a growing need for developers to optimize child components using TypeScript. Effective implementation of child components in React can significantly enhance application performance. This process can be achieved through Function Components (Fc) in TypeScript. So, how exactly does one go about this?
Firstly, it’s crucial to understand that when type-checking components, TypeScript requires you to define the types of properties your components receive.
type Props = { children: React.ReactNode; }; const Component: React.FC= ({ children }) => { return ( {children}) };
In the above snippet, a Function Component (`FC`) is used. The `ReactNode` type is all-encompassing, allowing any valid element that can be rendered including numbers, strings, JSX elements or arrays thereof, or even other React fragments.
Instances may arise where there are more specific requirements for children than just being “anything that can render”. In such cases, TypeScript’s powerful typing system steps in.
type PropsWithSpecificChildren = { children: React.ReactElement | React.ReactElement[]; }; const SpecificComponent: React.FC= ({ children }) => { return ( {children}) };
Above, we just created `PropsWithSpecificChildren`. We’ve tightened our `children` requirement to either be a single `ReactElement`(a JSX element) or an array of `ReactElements` — not just any renderable element.
Understanding the interaction between Function Components and types are indeed crucial to structuring child components effectively in React 18. Together, TypeScript and React provide various options, leaving the decision-making process on the developer’s shoulders.
Kent C. Dodds, a renowned software engineer in the JavaScript community, once said: “Any application that can be written in JavaScript, will eventually be written in JavaScript.”
It implies that as React 18 continues to evolve, more opportunities and challenges are being brought to the forefront in JavaScript /TypeScript world. Indeed, implementing child components effectively requires proficiency in TypeScript & Function Components.[source]
Conclusion
To encapsulate,
TypeScript Children FC
constitutes a key feature in using ReactJS, particularly now when React has launched its 18th version. TypeScript provides static types to JavaScript, reducing bugs and facilitating the construction of scalable applications. Dealing with Children in React TypeScript Function Components (FC), we achieve better code structuring and clarity.
Let’s probe deeper into this:
* One of the critical benefits is that it validates props passed to a component during compilation, reducing runtime errors. For instance:
type Props = { children: ReactNode; }; export const MyComponent: React.FC= ({children}) => { return ( {children}); };
This snippet demonstrates how children prop is utilized in a functional component, exhibiting the marriage between React and TypeScript.
* When you’re employing TypeScript with React, your components can have strong typing for props. This includes children. In case you pass something unexpected into the children prop, TypeScript will notify you about that misuse, potentially preventing an elusive bug in your application.
Tale as old as time tells us that there’s always been a disparity between uses of children in class-based components and function-based components in React. However, embracing TypeScript demystifies many of these deviations, making ‘children’ more usable regardless of the component type, which leads to cleaner, safer, and more maintainable code base.
As Jeff Atwood, co-founder of Stack Overflow once said – “Code is like humor. When you have to explain it, it’s bad.” The strength of TypeScript’s static typing system means less time spent explaining and deciphering your code and a bigger investment in crafting the features your users need most.
Nonetheless, technology continues to evolve, and so does React and TypeScript. It is pivotal to keep track of the ongoing development in their ecosystems and leverage the tools they provide, such as TypeScript Children FC, to tailor solutions that meet specific needs, thereby enhancing and improving user experience.
Every stone turned within TypeScript and React’s journey furthers the potential for better digital habitats, remembered fondly for their performance, and the way they respond to the life around them.