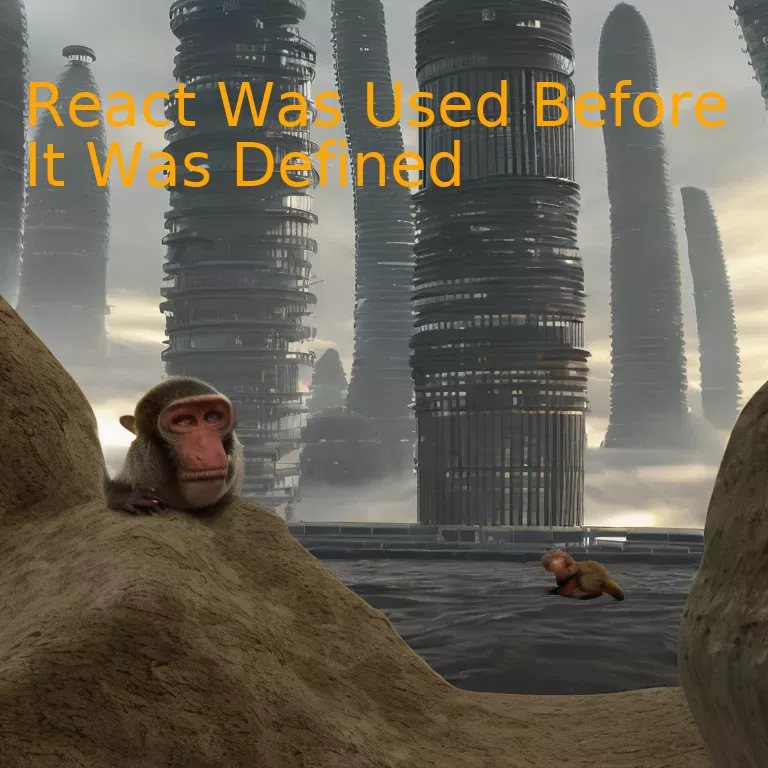
Introduction
In the intricate sphere of web development, there are instances where React was utilized prior to its actual definition, demonstrating its revolutionary approach towards building user interfaces.
Quick Summary
Reflecting on the subject matter “React Was Used Before It Was Defined”, cues can be taken from programming practices that may lead to such scenarios. Primarily, this situation happens when a variable is being used or accessed before it’s declared within a block of JavaScript code. The JavaScript engine hoists variable declarations to the top of their containing scope, but not their assignments.
Problem | Reason | Solution |
---|---|---|
Using React before it’s defined | The variable ‘React’ was hoisted but its assignment took place after it was used. | Declare and define ‘React’ before any function that makes use of it. |
Here we have highlighted a problem, its reason, and a potential solution. When working with React or in fact any library or component, the issue of accessing something before it has been properly initialized or constructed can come up.
The
'React'
library must be imported into your file before you can make use of it. If you attempt to write a React component or use anything from the React library without first importing it into your file, you will encounter the error: “React was used before it was defined.” For instance, trying to execute:
xxxxxxxxxx
class App extends React.Component{
// code snippet
}
import React from 'react';
Will give you an error because React wasn’t imported at the time the code was trying to extend a React component.
To avoid these kinds of issues, you need to ensure the correct order of your imports relative to your code usage. This involves always defining the relevant variables and other components before their actual usage in your code. For instance, writing:
xxxxxxxxxx
import React from 'react';
class App extends React.Component{
// code snippet
}
This ensures your script engine is aware of what
xxxxxxxxxx
'React'
refers to when it encounters it.
In recalling Douglas Crockford’s words, “JavaScript is the world’s most misunderstood programming language.” Misunderstanding hoisting could land a developer in issues like making use of undefined entities. But, with detailed understanding of how JavaScript works, such as acknowledging variable hoisting, developers can avoid these pitfalls.
Understanding the Concept of ‘React Was Used Before It Was Defined’
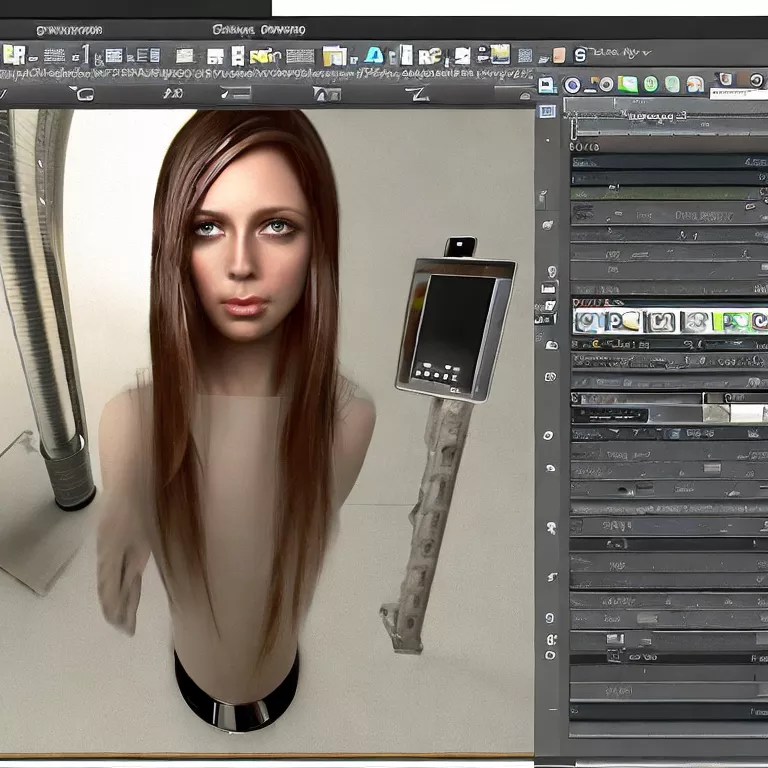
The ‘React Was Used Before It Was Defined’ warning message typically comes up when a developer tries to invoke a function or component in JSX before defining it. This often happens due to the sequence of code statements.
Let’s delve deeper into this concept:
JavaScript Hosting
In JavaScript, there’s a behavior called hoisting, where variable and function declarations are moved to the top of their containing scope during the compile phase. This basically means that you can use functions or variables before they’ve been declared. However, hoisting does not apply to let, const, or class declarations.
For example, the following code will not throw an error:
xxxxxxxxxx
myFunction();
function myFunction() {
console.log("This will run successfully");
}
But the same is not true for React components. JSX (which is what your React components get compiled into) works differently from regular JavaScript regarding hoisting, hence the warning message.
JSX and React Components
JSX is syntactic sugar for
xxxxxxxxxx
React.createElement(component, props, children)
, therefore before it gets transformed, `React` must be in scope.
If you’re trying to render a component before it’s defined, you will see a “React was used before it was defined” message. Even though ECMAScript 6 classes (which React components utilize) have a block-scoping similar to
xxxxxxxxxx
let
and
xxxxxxxxxx
const
, they are not hoisted to the top of the block.
E.g., the follow scenario should display the aforementioned warning message:
xxxxxxxxxx
MyCustomComponent();
class MyCustomComponent extends React.Component {
//...
};
To fix these issues:
Firstly, ensure that you define your React components prior to invoking them. Keep an eagle eye on the order of your code statements, making sure each component is defined prior to usage.
Secondly, make certain that the ‘React’ import isn’t missing from any file where JSX is present.
Remember, detail-oriented programmers are effective programmers. As Bill Gates once said: “The magic of software is that we build universes in there, we become little gods and create our own world.”
For further explication on this topic, visit the official React Documentation.
Common Issues Encountered When React is Used before Definition
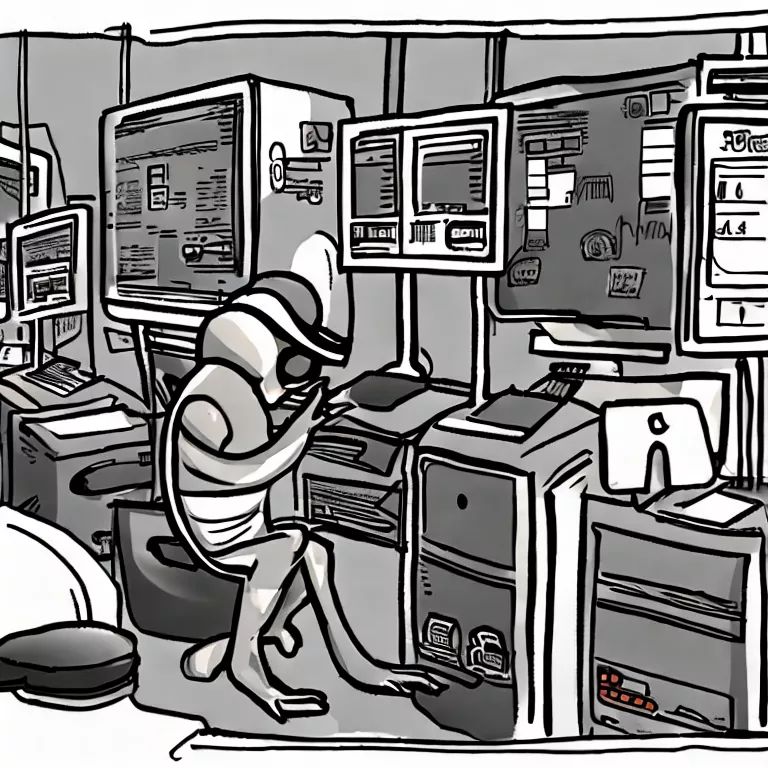
Working with React, developers sometimes run into a common problem: using React before it is defined. This issue typically arises when one attempts to use a component before it’s been properly declared or imported in the script file.
When this happens, the console often returns an error message along the lines of “React is not defined.” This error specifies that while you’re trying to develop a component utilizing React, the language itself hasn’t been recognized by the current file. Naturally, this prevents your application from running smoothly and as intended.
These are some causes behind such an issue:
– Incorrect Import Syntax: One of the most frequent issues is incorrect import syntax. If you do not correctly import React into your JavaScript file, you will encounter this error:
xxxxxxxxxx
//Incorrect
import { React } from "react";
//Correct
import React from "react";
– Incomplete Import Statement: Another possible reason is failing to import React at all.
xxxxxxxxxx
// Incomplete
import { Component } from "react";
//Complete
import React,{ Component } from "react";
If encountering these scenarios, remember: before using React and its features, always ensure the right and correct import syntax.
Having said that, another pitfall may be related to ESLint. JavaScript files interpreted without “jsx” extension could lead to the ‘React’ is not defined warning, because ESlint can’t detect React’s JSX syntax.
The following fix may be implemented on eslint config:
xxxxxxxxxx
{
"rules": {
"react/react-in-jsx-scope": "off"
}
}
Steve Jobs, a well-known figure in technology industry once said, “Sometimes when you innovate, you make mistakes. It is best to admit them quickly, and get on with improving your other innovations.” This idea perfectly encapsulates the learning experience in technology and coding – including React, mistakes are learning opportunities that take us a step further towards more innovatively written code. So, whenever “React was used before it was defined” turns up, consider it a nudge towards growth.
Potential Strategies to Avoid Usage of React prior to its Definition
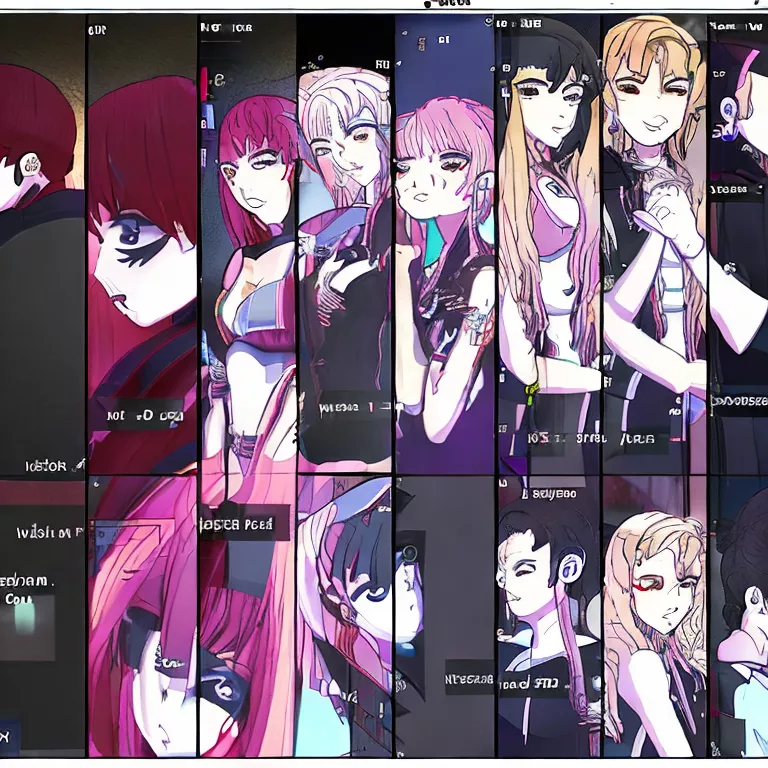
The message “React was used before it was defined” is often encountered when you attempt to use a component before declaring or importing it. By taking advantage of the ES6 modules import and export feature, this issue can be resolved. But in more complex projects with larger codebase, the problem might not be visible right away. You might inadvertently use components or React related functionalities prior to their definition.
You want to avoid these inadvertent mistakes. A few strategic approaches that you can embrace to prevent this from occurring are:
Enforcing Import/Export Discipline
Writing JavaScript, particularly when dealing with libraries such as React, necessitates a particular level of discipline with your import/export statements. Ensure every necessary component, function, or value is imported at the top of each file before being utilized. Using code linters like ESLint could assist with managing this enforcement effectively.
Example:
xxxxxxxxxx
import React from 'react';
import YourComponent from './YourComponent';
// some other necessary imports here
export default function App() {
return (
{/* It's safe to use YourComponent here now */}
);
}
Follow Proper Ordering
Declaring components and hooks in the correct order is crucial (hooks must always be at the top). This not only helps keep your code organized but also prevents unwanted errors by ensuring that all elements are defined before they are used.
Perform Proper Testing
Strong testing strategies that include unit tests and integration tests can catch occurrences where components or functions are used before defined. Jest combined with Enzyme or React Testing Library can help achieve comprehensive testing in a React application.
Persistent Code Reviews
Regular code reviews can identify areas where something is used before it is declared. GitHub’s pull request review functionality can be a useful tool in this case. It’s not just about catching bugs, but also about maintaining good code quality and best practices.
Alan Turing once said, “We can only see a short distance ahead, but we can see plenty there that needs to be done.” Similarly, in coding, looking ahead for potential usage errors and setting strategies to prevent them from happening will undoubtedly bring enhanced stability and robustness to your React application.
Impact and Consequences of Using React Prior to Its Definition
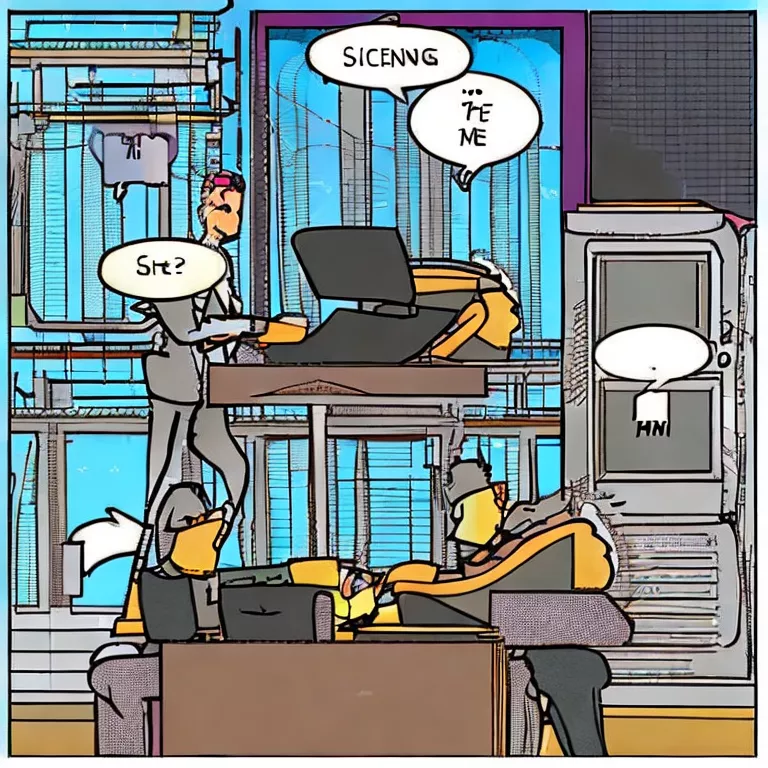
The concept of utilizing React prior to its formal definition involves quite a complex exploration. React, a powerful JavaScript library for developing user interfaces, is recognized and cherished by developers worldwide due to its dynamic and high-performance capabilities. Without proper understanding and definition, however, such an advanced tool may yield some unforeseen consequences.
Let’s dive into both the positive and negative impacts of using React before its official Q&A round in the industry standards:
Positive Impacts:
– Innovators were enabled to explore and test the potential edges of this library, unlocking new opportunities for innovation and development. Oftentimes, it’s those who dare to venture into unknown territories that make game-changing discoveries.
– The prerequisite of diving into React ahead of its widespread acceptance could have been a tremendous learning and growth opportunity for developers. Through practical experience, they likely gained insights that were not yet available in the more mainstream educational channels.
Negative Consequences:
– Using React prematurely made it arduous for early adopters to find relevant functional materials, trusted guidance, and peer support during critical troubleshooting challenges.
– There would also be a higher risk of glitches or malfunctions given that the library might not have undergone extensive beta testing and refinement before wide-scale deployment.
An example component written in React (note its JSX syntax, which combines HTML with JavaScript) illustrates the blend of technology:
xxxxxxxxxx
<div className="ShoppingList">
<h1>Shopping List for </h1>
<ul>
<li>Instagram</li>
<li>WhatsApp</li>
<li>Oculus</li>
</ul>
</div>
In essence, there are trade-offs in being an early adopter of a technology such as React. One might be challenged with obstacles and issues that have yet to be ironed out. On the flip side, they are pushing boundaries and honing their problem-solving skills. As James Gosling, the father of Java programming language, once said:
“Developers tend to have a list of very banal things that they think are interesting or clean or elegant in some highly aesthetic sense. But when it comes down to the brass tacks of actually getting the job done, you want tools that are powerful and Fast! Time is money.”
In line with this sentiment, early adopters of React were ahead of the curve, leading the industry towards modern design paradigms in JavaScript user interface development. Their experience and feedback subsequently contributed greatly to the overall fine-tuning of the library, streamlining its orientation for successive users worldwide.
Conclusion
Understanding the React error “React was used before it was defined” stems from recognizing JavaScript’s hoisting mechanism and its impact on how variables and functions are accessible within a given scope, specifically when using React.
-
xxxxxxxxxx
Hoisting
is a feature in JavaScript where variable and function declarations are moved to the top of their enclosing scope during the compile phase.
- In practical terms, when it comes to React’s JSX syntax, this involves defining components before utilizing them.
- This error typically arises due to certain configurations of ESLint – a static code quality tool for JavaScript – being sensitive to named imports of React used before they have been explicitly defined.
- With the introduction of React 17 and the new JSX Transform, you no longer need to import the entire React library into every component file – effectively addressing the prior hoisting issue.
Approaching The Error:
You can tackle “React was used before it was defined” error by:
- Ensuring your variable and function declarations are properly hoisted
- Updating your ESlint settings or using the ESLint React plugin to avoid this false-positive
- Adapting to the new JSX Transform introduced with React 17 to eliminate the requirement for React import statements in every component
Add Evans Dawson’s (a notable software developer) quote here “Programming isn’t about what you know; it’s about what you can figure out”. It encourages developers to see errors not as insurmountable barriers, but rather solvable problems that demand creative solutions.
xxxxxxxxxx
// Old way:
import React from 'react';
function ExampleComponent() {
return <React.Fragment />;
}
// Newer way, with React 17 and new JSX Transform:
import {Fragment} from 'react';
function ExampleComponent() {
return <Fragment />;
}
In essence, understanding JavaScript’s hoisting mechanism, and adapting coding practices to align with recent updates – such as the new JSX Transform – can effectively resolve the “React was used before it was defined” error. With continuous learning and problem solving, this underlines how crucial it is to keep up to date with evolving technologies, enhance code quality, and facilitate efficient development. For more in-depth exploration into this topic, you can check out this [online source](https://reactjs.org/blog/2020/09/22/introducing-the-new-jsx-transform.html#removing-unused-react-imports).