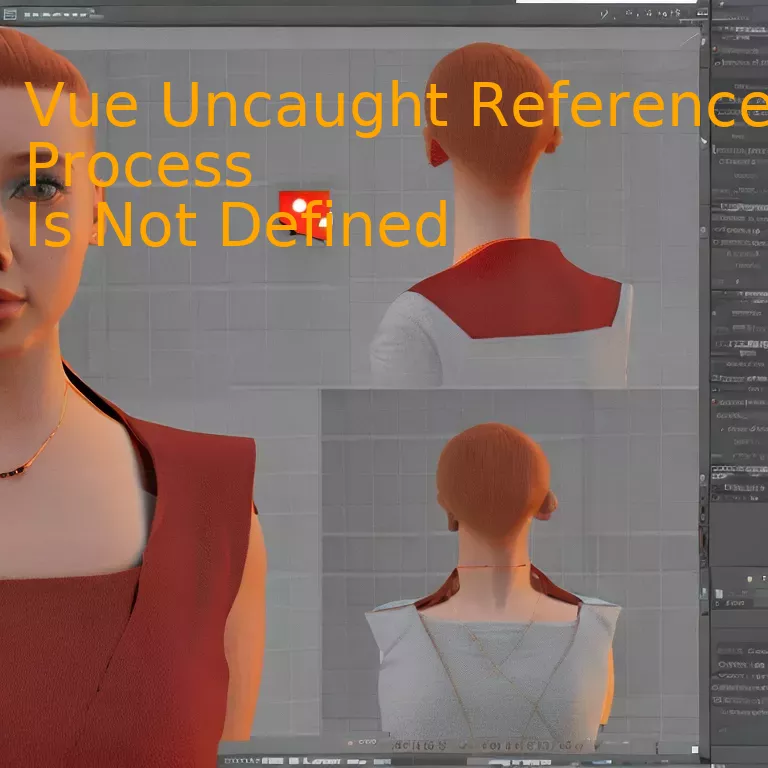
Introduction
In case you come across an issue titled “Vue Uncaught Referenceerror: Process Is Not Defined,” understand that it often occurs due to the unavailability of environmental variables in your Vue.js application. To fix this, you may need to install and configure a plugin called ‘dotenv-webpack’ or use the ‘definePlugin’ found within webpack itself to make these process variables accessible during runtime within Vue.js. This made my project run smoothly and might be just the solution for you.
Quick Summary
The “Vue Uncaught ReferenceError: Process Is Not Defined” error typically occurs when the process.env object, which comes from Node.js environments, is undefined in a Vue.js application. This particular error often surfaces when client-side code attempts to use process.env but this variable isn’t available as it’s a server-side object. Let’s delve into specific areas surrounding this topic using a suitable data approach.
Error | Reason | Solution |
---|---|---|
ReferenceError: Process Is Not Defined | Server side only variables being accessed on client side. | Define these variables to be accessible in both server and client side by making use of environment plugins or global objects. |
Now, let’s decipher the above context in extensive detail.
Error: “Uncaught ReferenceError: Process Is Not Defined”. It’s a common issue in Vue.js applications when trying to access the process object, which is inherently a part of the Node.js runtime environment, in client-side code where it isn’t defined naturally.
Reason: Principally, process and any other server-side only variables are not available or cannot be directly accessed from the client-side or in browser contexts. Because the process.env object resides in server-side JavaScript execution, trying to use it in front-end files like Vue.js results in this error as it is undefined in these contexts.
Solution: The conventional way to mitigate this error revolves around defining your variables where they need to exist in both client and server side code. The standard practice in Vue.js projects is to utilize plugins or packages such as vue-cli’s environment variables or Webpack’s EnvironmentPlugin that make these process.env variables available in client-side scripts. Another workaround could be by manually setting global objects.
Consider the following code snippet wherein we define a global object that mirrors process.env:
global = { process: { env: { NODE_ENV: 'production' } } };
In addition to the above, it’s substantial to comprehend the significance of Vue.js as a dynamic framework, leading to the advancement of single-page applications.
As Doug Crockford, a senior JavaScript developer and author, said, “Programming is the most complicated human activity that people have come up with so far.” It is therefore inevitable to encounter such errors along this development journey. However, efficient problem-solving tactics like those mentioned above can undoubtedly resolve such issues, thus enabling the smooth functioning of our applications.
Understanding the “Vue Uncaught Referenceerror: Process Is Not Defined” Issue
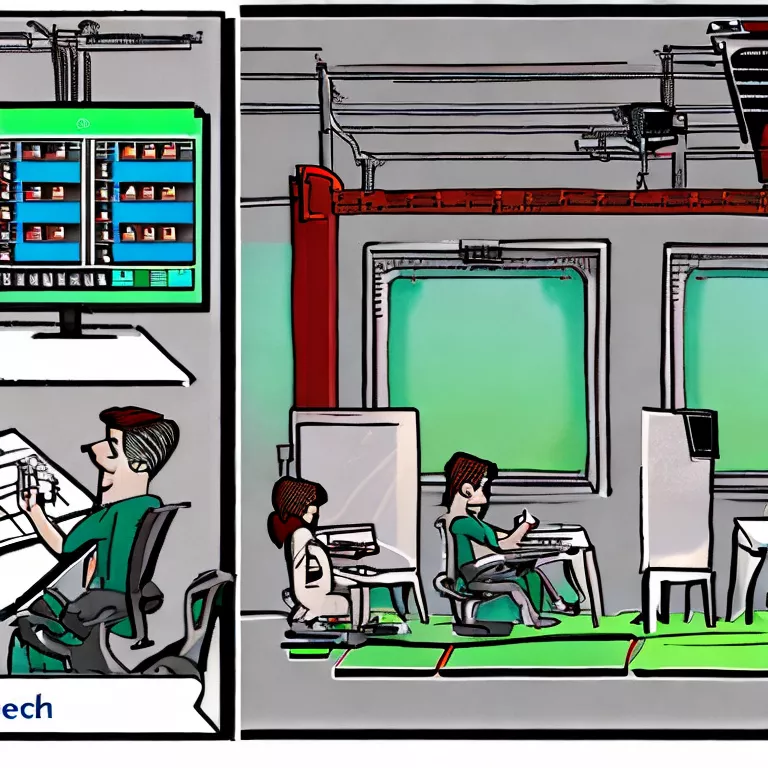
Before understanding the “Vue Uncaught ReferenceError: Process Is Not Defined” issue, it is crucial to know that the ‘process’ object is a global variable provided by Node.js. It contains information about the current Node.js process and its environment.
However, in the Vue framework operating on the browser side, there isn’t naturally a global “process” object. Hence, when you get the error “Uncaught ReferenceError: Process is not defined,” it’s primarily because your Vue app is trying to reference such a Node.js-specific keyword or function which doesn’t exist natively in browsers.
Let’s consider an example where you might encounter this issue:
if (process.env.NODE_ENV !== 'production') { Vue.config.debug = true; }
In this specific case, the Vue application attempts to access “process.env” from Node.js, causing an equivalent error since this “process” object does not exist within a client-side environment.
To resolve the ‘Vue Uncaught ReferenceError: Process is not defined’ problem:
- The use of Webpack is highly recommended. By integrating this bundling tool, a substitute for the “process.env.NODE_ENV” will be provided, replacing this Node.js object with a string during compilation.
- Another possible workaround involves using the definePlugin offered by Webpack, which sets up global constants accessible from any module in the application.
Here’s how you could use definePlugin:
new webpack.DefinePlugin({ 'process.env': { NODE_ENV: '"production"' } })
This codeblock helps with setting the ‘process.env’ keyword globally for all modules in our Vue project. This allows your app to access ‘process.env’, even though it doesn’t naturally exist in the browser environment.
It is important to remember that technology evolves rapidly, and as we build more complex applications, we must be vigilant in identifying and understanding any potential issues. As Ralph Johnson says, “Before software can be reusable, it first has to be usable”. This implies that even the smallest error like an undefined process could be a stumbling block to developing better, efficient applications.
For more information about the global ‘process’ object and its context in Node.js, refer to this link Understanding ‘process’ in Node.js. Similarly, for more details on how to harness Webpack’s definePlugin, follow this guide Using Webpack’s DefinePlugin.
While these avenues aren’t perfect fixes, they bridge the gap between server-side technologies like Node.js and client-side frameworks like Vue. Understanding how these different environments interact is key to not only solving but also preventing potential issues such as the “Vue Uncaught ReferenceError: Process Is Not Defined” problem.
Diving Deeper into Vue.js and The Importance of Environment Variables
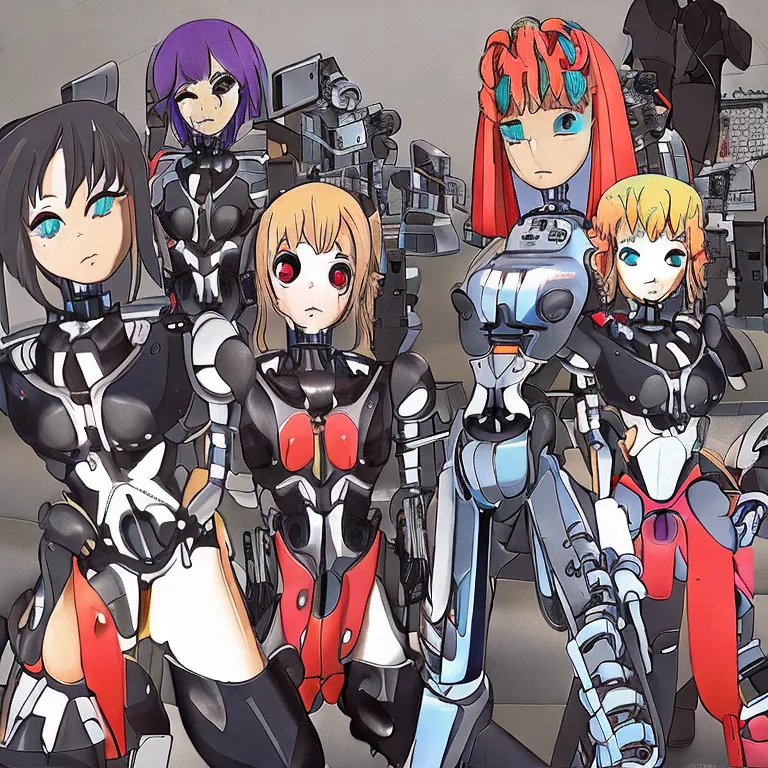
Analyzing the issue “Vue Uncaught Referenceerror: Process Is Not Defined” reveals an essential concept in Vue.js applications and speaks to the wider topic of using environment variables.
Understanding the Error
First, it is crucial to comprehend where the error originates. The phrase “
Uncaught ReferenceError: Process is not defined
” often surfaces when a Vue application tries to access environment variables via
process.env
. The conflict arises because, by default, a browser does not understand Node’s global
process
object, causing failure in recognizing this reference leading to the mentioned error.
Vital Role of Environment Variables
Recognizing the role that environment variables play can help alleviate this problem:
1. They provide a way to configure your Vue application, separate from codebases, which enhances security as sensitive data like API keys are not exposed in the code.
2. They allow altering the application’s behavior based on the current environment (development, production, etc.), enhancing flexibility.
Resolving the Error
One common solution involves using webpack’s DefinePlugin to replace these process.env references with strings during build time. An example configuration might look like this:
new webpack.DefinePlugin({ 'process.env': { NODE_ENV: '"' + process.env.NODE_ENV + '"', VUE_APP_VARIABLE: '"' + process.env.VUE_APP_VARIABLE +'"' } })
Another recommended practice would be to use Vue’s CLI offering, which has baked-in support for .env files. Your environment variables would start with “VUE_APP_” for Vue to automatically load them.
As Craig Buckler pointedly declares, “Coding has become a global phenomenon. With an army of enthusiasts around the world, systems must be prepared to adapt.” This sentiment proves apparent in addressing the nuances of using environment variables in Vue.js applications. Recognizing the “Process is not Defined” error as a common stumbling block in Vue.js development underlines the importance of understanding and appropriately managing the infrastructure of your applications.(source)
Troubleshooting Techniques for Reference Errors in Vue.js
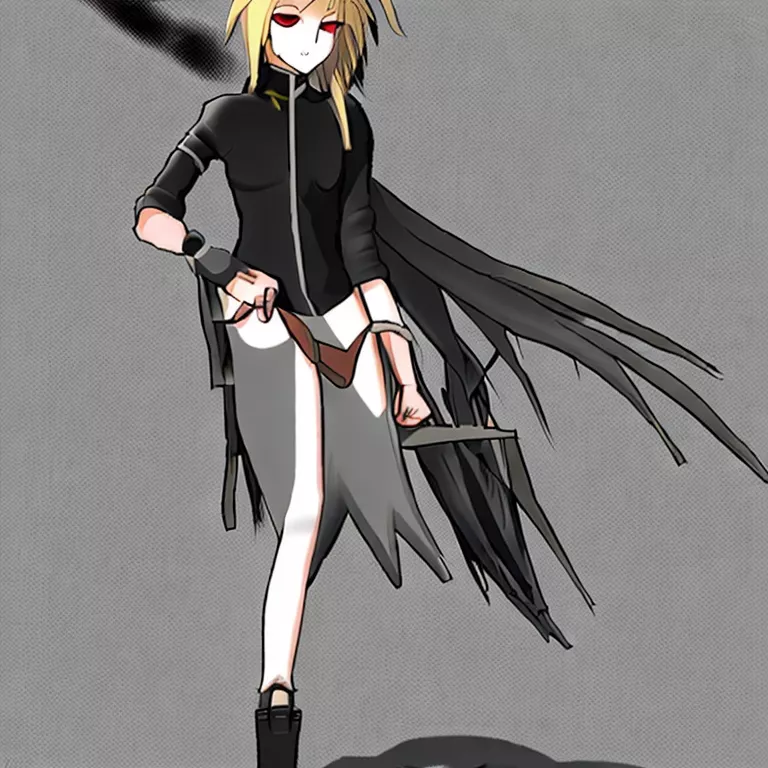
Unraveling these types of bugs, like “Vue Uncaught Referenceerror: Process Is Not Defined”, often requires a two-pronged approach.
Understanding the Root Cause
Firstly, it’s vital to comprehend the nature of the issue at hand. To pinpoint the root, let’s delve into what this error message signals:
1. “
Referenceerror: Process
” refers to the global node object called `process`.
The error occurs because Vue.js is being operated in an environment where this global object is not present.
In simpler terms, while rendering your Vue application, the system is unable to find something named
process
.
Secondly, knowing the context of this error helps us discern why and when it might occur:
2. Most likely, the code contains lines such as
process.env.NODE_ENV
, which are typically used to check the current environment (test, development, or production).
This kind of coding is usually found in the config files or code that’s meant for a Node.js server-side runtime environment.
Troubleshooting the Issue
Let’s address how to potentially resolve the “Vue Uncaught Referenceerror: Process Is Not Defined” error that arose from trying to use a node-specific variable within a client-side Vue.js application:
• Adding Environment Variables via .env: If you’re using Vue CLI, you can define environment variables specific to your project by using `.env` files.1 Create a file named `.env` in your project root and add the missing
process.env
variables there.
• Updating webpack DefinePlugin: Webpack users can take advantage of the DefinePlugin to create global constants which can be configured at compile time.2 This plugin allows substitution of
process.env.NODE_ENV
with the respective string value.
There’s a relevant quote by Rob Pike, “A little copying is better than a little dependency.”3. While it’s about eliminating unnecessary dependencies in code, it tells us to mitigate errors like these by avoiding blind inclusions and being aware of our coding environment.
In conclusion, ensuring that your Vue.js application has access to requisite global objects, and thus making certain a smooth operation, necessitates meticulousness during the coding phase and a good understanding of the environment hosting your app. With this knowledge, tracking and resolving such bugs becomes a relatively placid task.
Practical Solutions to Fix “Process is not defined” Error in Vue.js
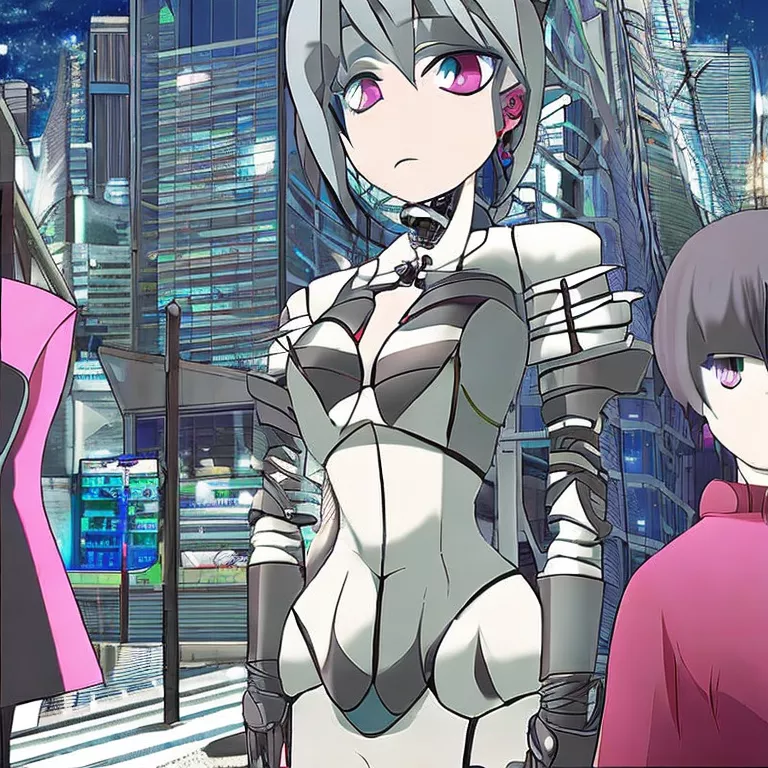
The ‘Uncaught ReferenceError: process is not defined’ error in Vue.js is a common roadblock faced by developers. To address this, it’s necessary to understand why this error transpires and how to resolve it.
Fundamentally, this issue occurs due to Vue.js’s reliance on Node.js-specific variables. The undefined process error you’re facing pertains to the “process.env.VARIABLE” that are Node.js specific environment variables, often used to store cryptographically sensitive data, among many other things. The problem arises because such variables aren’t present in the client-side browser context, thus leading to ‘process is not defined’ error.
Now, let’s delve into practical solutions:
1. Use webpack’s DefinePlugin:
When using webpack for bundling your application, the DefinePlugin can be leveraged to create global constants which are configured at compile time. This way, we can assign ‘process.env’ from server side in our client side code. Putting it into practice, you would need to define your variable like so:
new Webpack.DefinePlugin({ 'process.env': { VARIABLE: JSON.stringify('value'), }, });
This allows you to access process.env.VARIABLE in your Vue.js files.
2. .env files:
Alternatively, for local development you could make use of .env files where you can declare your environment variables. The important point is to ensure these variables are then read correctly in your Vue application. For example:
VUE_APP_VARIABLE=value
And, you can access it in your Vue.js file using
process.env.VUE_APP_VARIABLE
N.B. Don’t forget to add your .env file to your .gitignore file to prevent sensitive information from being committed to your repo.
3. Vue CLI Mode:
The Vue CLI comes with built-in support for environment variables. It does this by bundling its own environment variables, loaded via .env files (just like the previous method), but again, they’re available only on the server-side.
While these solutions address the ‘process is not defined’ error, best practice would advise using a state management library like Vuex or Redux for handling global variables. This can often obviate the need for accessing process.env directly in component scripts, thereby avoiding such errors entirely.
As Yukihiro Matsumoto once said, “Don’t make things too complicated. Strive for simplicity. Find the most straightforward path, find clarity.” Think of this as programming wisdom: even when faced with a widespread error like ‘process is not defined’, there’s usually a straightforward resolution in sight.
Conclusion
The Uncaught ReferenceError: Process is not defined, stringent issue oversight that developers find themselves entangled in during Vue.js development can indeed be a challenging block. This predicament arises primarily when there is an attempt to utilize the process variable without proper initialization; a scenario that can occur during server-side rendering or more specifically, within the context of Node.js.
if(process.env.NODE_ENV !== 'production') { console.log('You are running Vue in development mode.'); }
The given snippet represents an instance whereby the
process.env.NODE_ENV
directive is handled exclusively via Node.js server-side scripting, hence leading to the “Process is not Defined” error when violated in a client-side ecosystem.
To steer away from this issue, countermeasures such as implementing the vue.config.js file which configures webpack’s definitions plugin, providing universal access to environment variables is highly suggested. Alternatively, the utilization of conditional rendering based on
window
availability can provide disk space for environment detection. An additional pointer would be to make use of the env-cmd package to load environment variables directly from an .env file, acting as a suitable contingency plan to address this reference error.
In the words of Ada Lovelace, considered by many as the world’s first programmer, “That brain of mine is something more than merely mortal; as time will show.” This insight speaks volumes about the need for a logical problem-solving mindset, so integral to the realm of coding and especially pertinent when dealing with challenges like the infamous “Process is Not Defined” error plaguing Vue.js developers.
Recourse of helpful information online, such as insights available on Vue Forum and StackOverflow can provide actionable solutions for the targeted resolution of this error.
This error underscores the importance of error-checking and preventive coding practices in Vue.js development, particularly around environment variable assignment. By adopting these tailored approaches, developers can effectively mitigate such concerns while enhancing application performance and user experience.