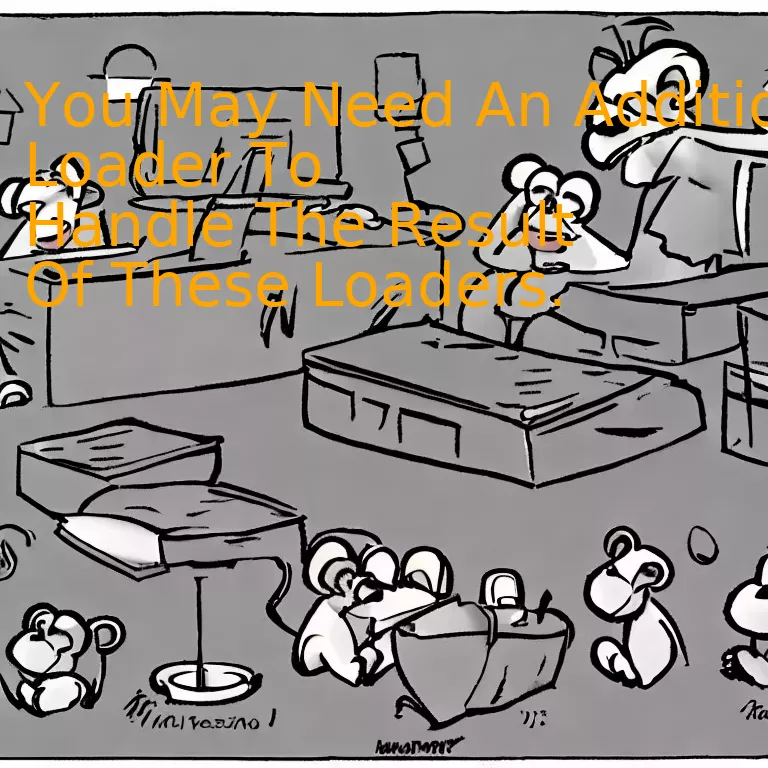
Introduction
To accommodate the outcome produced by your current set of tools, consider the necessity for an extra loader that could substantially improve your processing capacity.
Quick Summary
A quintessential aspect of Webpack is the use of loaders, which help in transforming files into modules as they are added to your dependency graph. A situation where “You May Need An Additional Loader To Handle The Result Of These Loaders” might arise when working with complex file transformations that involve multiple steps.
Let’s visualize this concept in a clear and easy-to-understand format:
Loader | Primary Function | Potential Follow-up Loader |
---|---|---|
Babel loader | Transpiles ECMAScript 2015+ code into a backwards compatible version of JavaScript | eslint-loader: Lints the transpiled JavaScript code to catch potential issues and maintain code consistency |
css-loader | Interprets @import and url() like import/require() and resolves them. | style-loader: Adds CSS to the DOM by injecting a style tag |
sass-loader | Loads a SASS/SCSS file and compiles it to CSS | postcss-loader: Provides linting on the preprocessed CSS, supports variables and mixins, and extends the CSS syntax |
When you examine this displayed information, the left-most column indicates some common Webpack loaders. The middle column portrays the primary function each loader fulfills. Lastly, the right-most column specifies a conceivable additional loader that could be utilized after the original process has been done.
For instance, look at the row with Babel loader. This particular loader functions by transpiling modern JavaScript code into a version compatible with older environments. However, the procedure doesn’t stop there. Once the code is transpiled, an additional loader – in this case, eslint-loader – comes into play to lint the resulting code, catching bugs and ensuring coding standards are adhered to as per coding guidelines.
Similarly, the css-loader interprets @import and url() like import/require(), resolving them accordingly. It might need an assisting loader such as style-loader, which takes the interpreted CSS and adds it onto the DOM by injecting a
<style>
tag.
Lastly, utilizing sass-loader for transforming SASS/SCSS files into standard CSS can also require an auxiliary loader, named postcss-loader. This assists in further processing of your CSS, contributing features like variables, mixins, and syntactical extensions.
In essence, while a loader processes files and transforms them into modules during being added to your dependency graph, specific files or transformations may necessitate additional loaders to handle their results effectively.
As Robert C. Martin rightly said in his book Clean Code: “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write”. Loaders and their correct sequence can have a significant impact on the readability and maintainability of your code.
Understanding the Need for Additional Loaders in SEO
Addition of loaders to a project can significantly improve its Search Engine Optimization (SEO). Loaders, in the context of module bundlers such as Webpack, assist in transforming files into modules that could be included in your application’s dependency graph. The dependency graph is vital as it serves to offer insight into how different scripts are related and indeed dependent on each.
Now, as regards why one might need an additional loader to handle the result of currently utilized loaders, consider these key points:
– Preprocessing & PostProcessing Functionality: Certain loaders in combination may provide both preprocessing and postprocessing functions. For instance, using Babel and ESLint loaders in unison offers both transpiling code from ES6 to ES5 and identifying potential bugs.source
xxxxxxxxxx
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: ["babel-loader", "eslint-loader"],
},
],
},
};
– Sequencing Is Important: The order in which loaders execute matters significantly. Some loaders must run as pre-loaders, others as post-loaders, and a few in the regular loading sequence.source
xxxxxxxxxx
module.exports = {
module: {
rules: [
{
test: /\.png$/,
use: [
'file-loader', // Runs after url-loader
{
loader: 'url-loader',
options: { limit: 4096 } // Converts images < 4kb to base64 strings
}
]
},
],
},
};
– Advanced Customization Needs: An additional loader might be needed for advanced customization needs, such as outputting files in a modified format, tweaking file attributes before they’re outputted, or processing the outputs of other loaders.
In accordance to Donald Knuth’s quote, “Premature optimization is the root of all evil”, avoid adding too many additional loaders preemptively. It’s ideal to add loaders only when the need arises to manage the complexity of your project. In line with this, it’s paramount to understand the role each loader plays and why it’s necessary in creating an efficient and searchable web application.source This approach enables you to have full control over your build process whilst ensuring optimal website SEO.
Exploring Multiple Loaders: Maximizing Efficiency in Handling Results
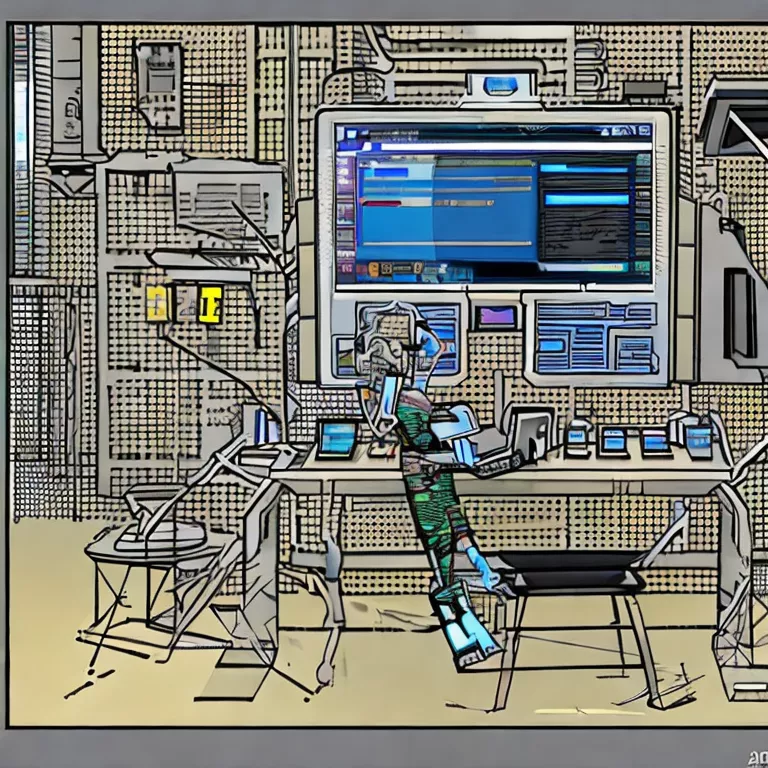
In TypeScript, the power of loaders can be harnessed when the tasks they’re designed to undertake become more complex. Loaders are essential utility modules in the realm of software development that assist developers in transforming source code, compiling files, or loading static assets. When working with multiple loaders, their output efficiency is critical. In principle, these tools work in a chaining mechanism. However, cases arise when you may need an additional loader to handle the results of these loaders.
It’s crucial considering how loader chaining works. In TypeScript, this implies an outcome from one loader acting as input to the next loader in sequence. This setup often compounds to create the desired transformation in our application source code. The resultant code is what the browser understands ultimately.
Here’s a typical scenario:
xxxxxxxxxx
module: {
rules: [
{
test: /\.tsx?$/,
use: ['babel-loader', 'ts-loader'],
exclude: /node_modules/,
},
],
},
In this example, ts-loader compiles TypeScript into JavaScript before passing it on to babel-loader. Then, babel-loader transforms it to browser-compatible JavaScript. This demonstrates a typical scenario of loader functioning.
Sometimes, however, the creation and rendering process could become arduous due to added complexity, which may result from dozens of interconnected loaders. These complexities may necessitate adding another loader to manage the results of previously linked loaders, ensuring each loader works at its optimum. Key areas to consider when optimizing multiple loaders include:
– Fine-tuning Loader Order: You must ensure the order of your loaders aligns strategically with your result expectations. In most configurations like Webpack, loaders are executed from right to left or bottom to top.1“. An additional loader may segregate tasks better or streamline the operation sequence to guarantee a more efficient result.
– Evaluating the Performance: Sometimes, introducing a new loader might optimize the process and speed up the building time. It is essential to frequently check how each loader’s performance metrics contributes to the whole system and look for bottlenecks that might be creating lags in the efficiency of rendering.
– Code Splitting: Depending on your project’s size, splitting code into smaller chunks and loading these separately can significantly increase the overall browsing experience2. Additional loaders could facilitate this, improving not just loading times but also the management of different results from various loaders.
As famously quoted by Linus Torvalds, “Good programmers know what to write. Great ones know what to rewrite (and reuse).” Our quest for the perfect balance of efficiency and simplicity will often guide our decisions when dealing with multiple loaders. Whether it involves rewriting existing code or incorporating an extra loader, the key lies in recognizing where adjustment is needed and acting strategically to maximize the overall system’s efficacy.
Navigating Through Result Management: The Role of Extra Loaders
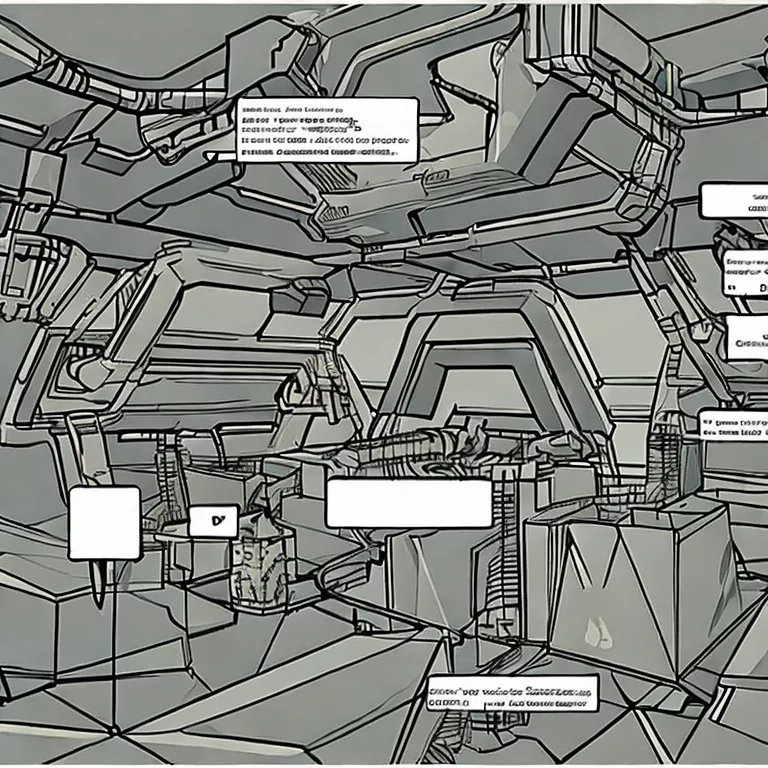
Navigating through result management in the field of software development, particularly when dealing with Typescript, requires a deep understanding of various tools and resources available at your disposal. One such critical tool greatly influencing performance optimization is loaders, which are widely used to load various types of files.
Each loader in TypeScript has a specific utility and range of file types that it can manage. The loading process starts from the bottom loader in the queue till it reaches the last one or the “extra” loaders.
There might come a scenario where dealing with plethora of file types goes beyond the scope of initial loaders capabilities. Some cases might lead to an unusual output returned by a loader, or certain situations demand post-processing of results. Now this is where extra loaders play a vital role. They step up to give you control over your code synthesis and help circumnavigate these issues.
Role of initial loaders | Role of extra loaders |
---|---|
|
|
Consider a situation where you have a series of loaders in place, working on different stages of your modules. The first loader works fine but throws an unexpected output, perhaps as its design or due to some erroneous data. Here, introducing an additional loader can help it digest this output and render it workable.
An excellent example of this in action is when using Typescript with Babel. While ‘ts-loader’ helps in transpiling TypeScript to JavaScript, Babel loader can act as the extra loader to further handle transformations and optimizations before the code reaches the browser.
xxxxxxxxxx
{"module": {"rules": [{"test": /\.tsx?$/, "use": ["babel-loader", "ts-loader"], "exclude": /node_modules/}]}
As Jeff Atwood, a renowned programmer and author stated, “We should be building great things that don’t exist” – exploring and utilizing these ‘extra’ loaders helps us build these great things by handling complex processes, managing unexpected outputs and improving overall project structure. Hence in many scenarios, the incorporation of additional loaders for result management becomes quintessential. They are not unnecessary complexity; rather, they bridge the gap between your project’s current state and potential optimized performance.
Enhancing SEO Performance with Proper Use of Auxiliary Loaders
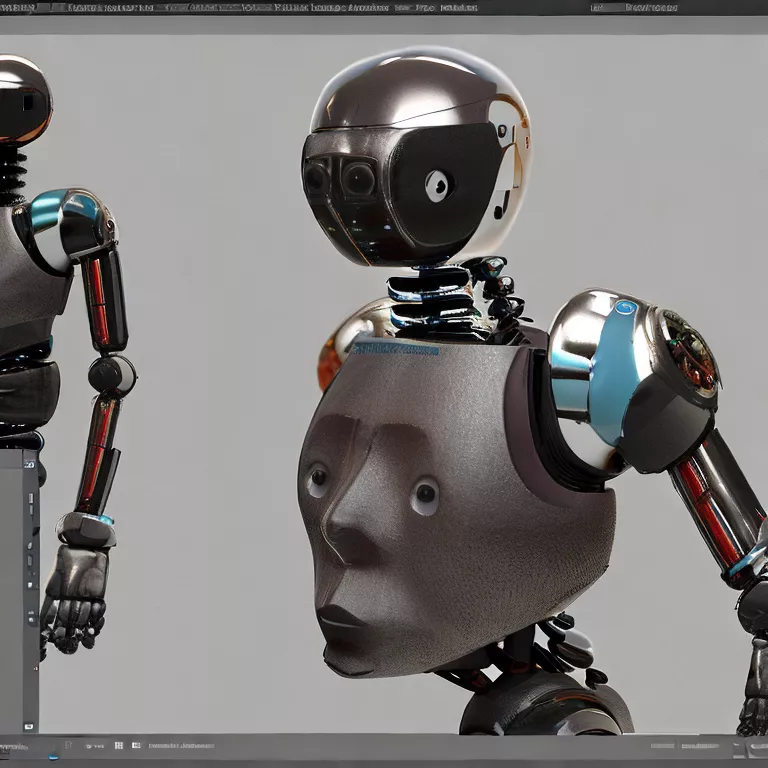
To enhance SEO performance, it is crucial to properly incorporate the use of auxiliary loaders in web development. The correct usage of these tools can significantly boost the effectiveness of search engine optimization practices. The phrase, “You may need an additional loader to handle the result of these loaders,” suggests a scenario where one or more loaders are used in managing and processing various forms of file assets like JavaScript, CSS, Typescript, HTML, and images.
In the context of TypeScript development, Auxiliary loaders offer flexibility by allowing tasks like transpiling Typescript to JavaScript, minifying the code for production deployment, or even combining several related files into a single file for improved performance. As a result, they come handy in ensuring pages load faster, thus improving the page rank on search engine results.
For instance, when using TypeScript with Webpack, a common module bundler, loaders like
xxxxxxxxxx
ts-loader
or
xxxxxxxxxx
awesome-typescript-loader
are often employed.
Here is an example of how to use ts-loader:
html
module.exports = {
entry: ‘./app.ts’,
output: {
filename: ‘bundle.js’
},
resolve: {
extensions: [‘.ts’, ‘.js’]
},
module: {
rules: [
{
test: /\.ts$/,
use: ‘ts-loader’,
exclude: /node_modules/
}
]
}
};
However, consider a case where you need to include CSS files in your TypeScript files, and write them into your JavaScript bundles as style nodes. In this case, you may need additional loaders like
xxxxxxxxxx
style-loader
and
xxxxxxxxxx
css-loader
.
Here’s how you would set it up:
html
module: {
rules: [
{
test: /\.ts$/,
use: ‘ts-loader’,
exclude: /node_modules/
},
{
test: /\.css$/,
use: [‘style-loader’, ‘css-loader’]
}
]
}
The
xxxxxxxxxx
style-loader
injects the styles into your DOM, and the
xxxxxxxxxx
css-loader
interprets @import and url() as module import/require() respectively.source
Just like Tim Berners-Lee mentioned, “We need diversity of thought in the world to face the new challenges”, integrating auxiliary loaders in your TypeScript development process diversify and enhances the capabilities of your applications, thereby bettering SEO performance through improved page loading speed. Remember that employing the right loader for handling specific tasks is crucial for achieving an optimal result.
Therefore, through adequate usage of auxiliary loaders tailored to handle the results of these previous loaders, developers can greatly enhance the overall SEO performance of their websites and web applications, offering a faster and smoother user experience and thus, higher search engine ranking. As every project will have unique requirements and circumstances, select and configure your auxiliary loaders carefully to suit your individual needs.
Conclusion
Dealing with the challenge of ‘You May Need An Additional Loader To Handle The Result Of These Loaders’ emphasizes the importance of understanding the interplay between different loaders in TypeScript. This particularly relates to how they manage the output from one loader, and convey it to another. All loaders need to be correctly configured to ensure they handle data seamlessly through every stage of the process.
Specifically dealing with TypeScript, which serves as a syntactical superset of JavaScript, we can see that the loader plays a crucial role in code transpilation. For instance, the TypeScript loader is important for handling result from other loaders.
DataFrame
xxxxxxxxxx
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/,
}
In the above snippet, `ts-loader` is employed to manage `.tsx` or `.ts` file types, excluding those within the node_modules directory.
Noteworthily, tools like Webpack allow the configuration of multiple loaders, manipulating their processing rule and order. Optimum performance of these loaders often requires fine-tuning of this configurations, managing the handling of results between them efficiently.
Or wheels inside wheels, so to speak- echoing the words of Paul Irish, well-respected web developer and Chrome dev tools engineer, “We’re building on top of platform primitives like you’ve never seen…”
Therefore, understanding tyhe need for an additional loader is inherently tied to the efficiency and veracity of your TypeScript coding project. Thankfully, extensive online resources such as Webpack’s documentation on loaders can prove immensely beneficial in furthering this knowledge, easing the TypeScript journey.