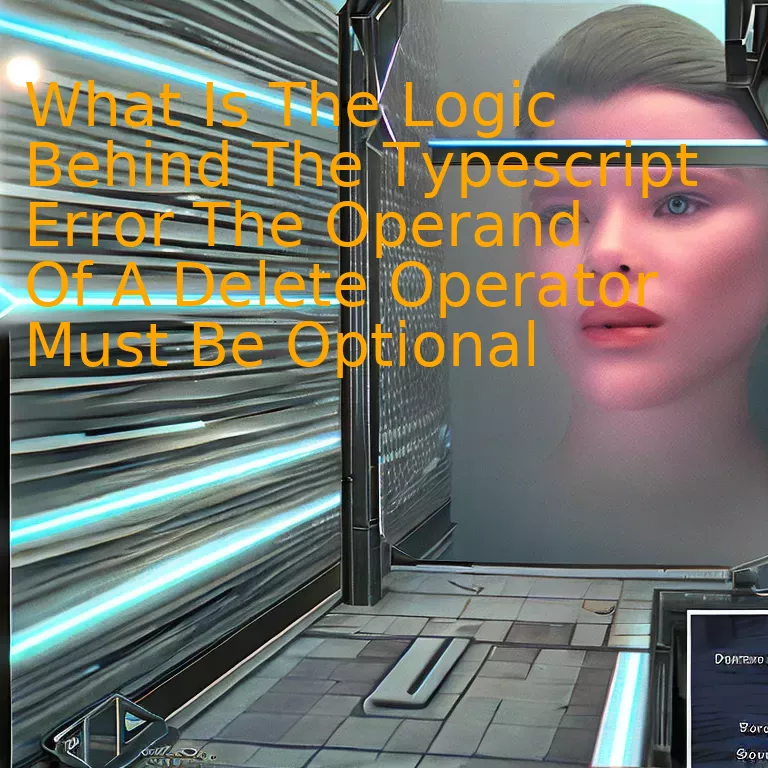
Introduction
In TypeScript, receiving the error “The operand of a delete operator must be optional” is based on the language’s strongly-typed structure aimed at preventing potential runtime errors. In other words, it ensures that you don’t accidentally try to remove a property that may not exist or could be essential for your program’s operation, thus reinforcing code safety and accuracy.
Quick Summary
Understanding the underlying reason behind the Typescript error: “The Operand of a Delete Operator Must Be Optional” is fundamental in enhancing your coding skills and pinpointing potential programming pitfalls. To systematically decode this phenomenon, we’ll commence with an organized dataset represented below.
Error Aspect | Detail / Explanation |
---|---|
Error Message | The Operand of a Delete Operator Must Be Optional |
Probable Cause | Attempting to use delete operator to remove a non-optional property from an object |
Solution | Use ‘delete’ operator on optional properties only or refactor the code to avoid its usage. |
When you receive an error stating, “The Operand of a Delete Operator Must Be Optional,” it is mostly because you’re trying to implement the ‘delete’ operator against a non-optional property within a Typescript object. To elaborate more, let’s illustrate an instance in code:
interface Example { prop1: number; prop2?: string; } let obj: Example = { prop1: 1, prop2: 'test' }; delete obj.prop1; // TypeScript will throw an error here. delete obj.prop2; // This is fine.
In the above example, prop1 is a non-optional property, whereas prop2 is optional (indicated by the ‘?’ symbol). As Typescript requires that all non-optional properties exist in all instances of the interface, the ‘delete’ operator shouldn’t be used on them. On the contrary, since optional properties don’t have to exist in all instances, they are safe for deletion. This explains the ‘delete’ command triggering an error when applied on prop1 but not on prop2.
To resolve this error, you should only use the ‘delete’ operator on optional properties. If it is essential to remove a non-optional property, you may need to refactor your code to avoid such necessity.
As Sheldon Cooper, the renowned theoretical physicist character from sitcom “The Big Bang Theory”, quotes: “Programming today is a race between software engineers striving to build bigger and better idiot-proof programs, and the universe trying to produce bigger and better idiots. So far, the Universe is winning.” Recall this quote whenever you encounter programming pitfalls; approach them as opportunities to hone your skills!
For more insights on TypeScript and its operations, you can refer to the website of TypeScript Documentation.
Understanding the Operand ‘Delete’ Operator in TypeScript
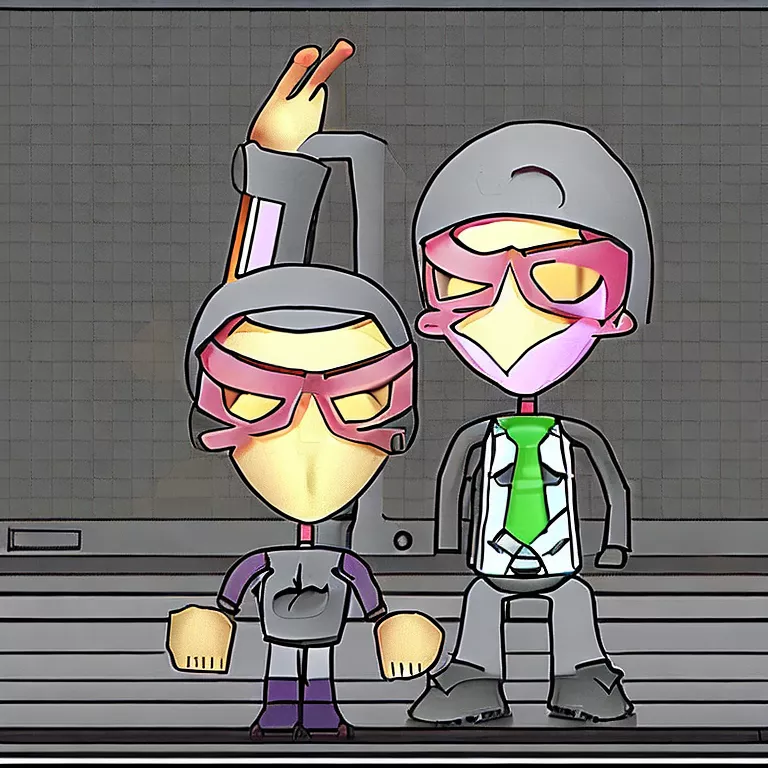
The TypeScript language builds upon JavaScript by adding syntax for type declarations and annotations. This extra layer promotes code reliability and easy maintainability. However, developers occasionally bump into unique error messages such as ‘The operand of a delete operator must be optional.’ Understanding this intriguing typescript error begins with comprehending the significance and operation of the ‘delete’ operator.
To begin with, let’s define what is a ‘delete’ operator. At its core, the
delete
command in TypeScript (as well as in JavaScript) is used to remove a property from an object. Here’s a simple code snippet to illustrate this:
let sampleObject = {prop1: "hello", prop2: "world"}; delete sampleObject.prop2;
Running this code would eliminate the prop2 property from the sampleObject, leaving only prop1.
Then comes the poignant question – Why does TypeScript require that an object’s property be optional before the delete operator can be applied to it?
This all ties back to strictness & strict null checking in TypeScript. Strict Property Initialization in TypeScript ensures variables are explicitly initialized in constructors. It expects every path through the code to either not construct an instance, or assign a value to each instance variable before it constructs the instance.
When you are coding in TypeScript with strict checks, signaling a property as ‘optional’ implies that such a property may at some point have an ‘undefined’ value, or may not exist at all on the object. The optional mark is denoted using “?” as follows:
interface SampleInterface { mandatoryProp: string; optionalProp?: string; }
In the code above, mandatoryProp is always required, whereas optionalProp, paddled with “?”, is optional.
Therefore, if you wish to use the delete operator on an object’s property in TypeScript, said property should be optional – essentially meaning it may or may not exist. This can save from future potential pitfalls where programmers might try to access properties that have since been eliminated.
The late computer scientist Edsger Dijkstra once said: “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” This highlights the value of understanding the intricacies of a language and its rules, and how communities create best practices to aid each other in writing better, more efficient code. So, understanding this logic behind the operand of a ‘delete’ operator strengthens your capability in leveraging TypeScript’s strictness features.
Decoding Errors: Why Must the Operand of a Delete Operator Be Optional?
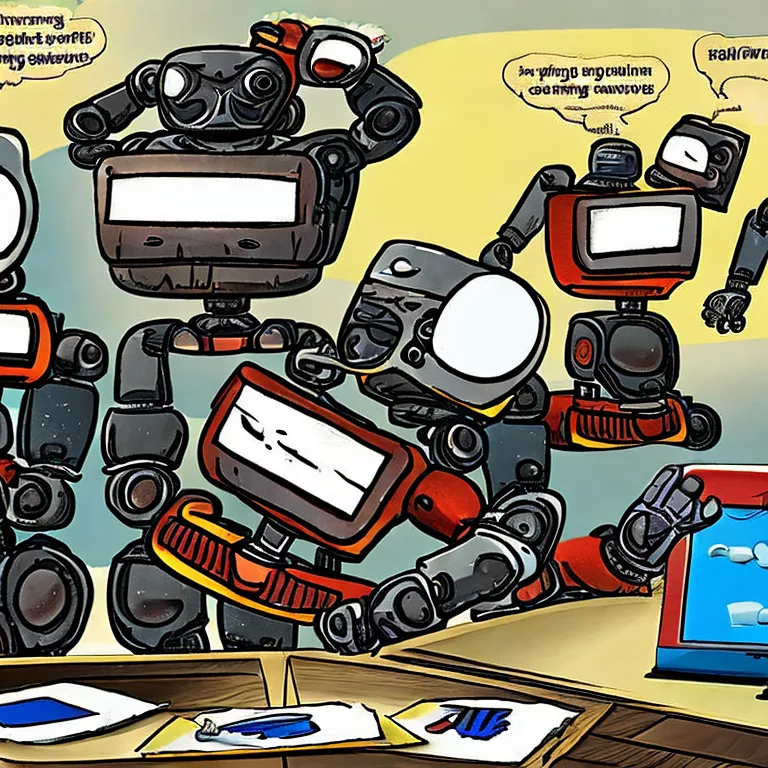
The necessity for the operand of a delete operator to be optional in TypeScript can be understood through its compatibility with specific JavaScript behaviors and the type safety provided by this language.
Understanding Delete Operator’s Operand
In JavaScript, when you delete an object’s property which is not writable, it does not throw any errors. Hence, TypeScript has to accommodate this behavior as it is a strict superset of JavaScript. For example, consider the code below:
const obj = {prop: "value"}; delete obj.prop;
Even though ‘prop’ is not stated to be optional within ‘obj’, the deletion operation does not trigger an error in JavaScript.
The TypeScript’s Check
However, the primary objective of TypeScript strictly checks types to ensure that any manipulations are handled appropriately. When making an attempt to delete properties that are not explicitly set as optional, TypeScript throws an error. This is explained by the following code:
type MyType = { mandatoryProp: string; }; const obj1: MyType = { mandatoryProp: 'I must exist!' }; delete obj1.mandatoryProp; // Error: The operand of a delete operator must be optional.
Here, trying to purge “mandatoryProp” from ‘obj1’ raises a TypeScript error because the property has been defined as non-optional within ‘MyType’.
Optional Properties
Conversely, when we annotate a property as optional, this signifies that, at some point – regardless of whether during runtime or codewriting – there may well be an absence of such a property. Therefore, considering these semantics into account, TypeScript allows the deletion of an optional property without causing any error. As an example:
type MyOptionalType = { optionalProp?: string; }; let obj2: MyOptionalType = { optionalProp: 'Maybe I exist, maybe not.' }; delete obj2.optionalProp; // No error
Here, the property “optionalProp” within ‘MyOptionalType’ is defined as optional demonstrated by the question mark following its name. This makes it acceptable to delete this property from ‘obj2’.
Essential Takeaway
Languages are built under certain specifications. For TypeScript, type safety lies at its core. Therefore, ensuring the operand of a delete operator must be optional adheres to this idea. As Richard P. Feynman stated, “For a successful technology, reality must take precedence over public relations, for nature cannot be fooled.” In essence, while TypeScript aims to make writing JavaScript smoother, it doesn’t shy away from critical checks and balances necessary for maintaining code stability and interoperability. Understanding such nuances helps developers write more robust, less error-prone code.
Typescript’s Logic Explained: The Mandatory Optionality of Delete Operands
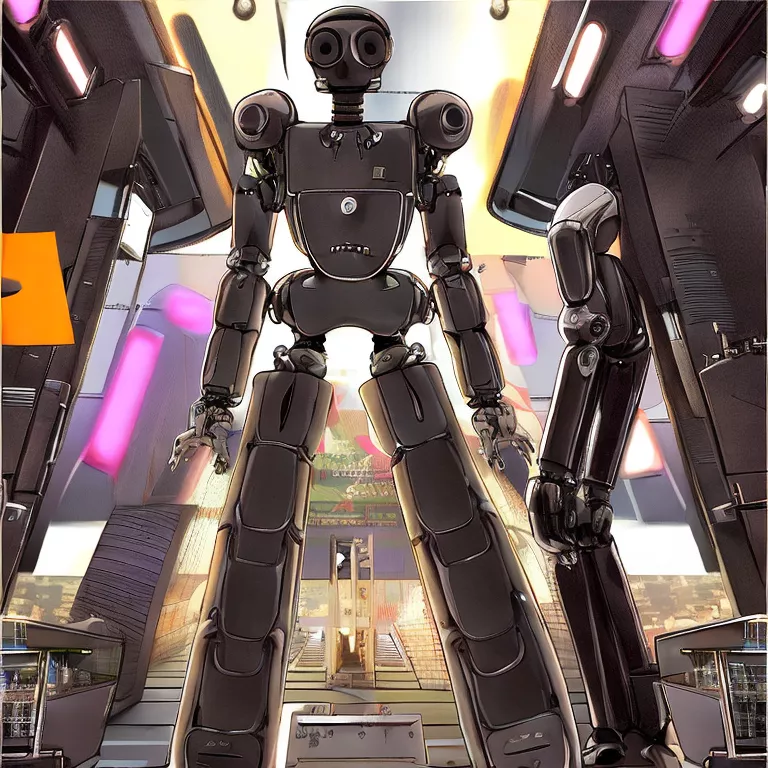
The operand of a delete operation in TypeScript is mandatory to be optional. The logic behind such a rule lies in the very essence of JavaScript’s delete operator and TypeScript’s static typing system. In light of this, discussing the lexical syntax form it takes, its runtime behavior, and implications in TypeScript, further enlightens this point.
In JavaScript,
delete
is an operator that removes a property from an object, and has a largely unique unary prefix notation:
delete obj.property;
The runtime behavior of the delete operator immediately reveals its peculiarity: if you try to delete a property that does not exist in an object, no error is thrown. This is, however, in stark contrast with TypeScript’s core principle of raising compile-time errors when attempting to access potentially non-existing properties.
When transposing JavaScript’s flexible runtime dynamics onto the strong, statically-typed skeleton of TypeScript, it may seem paradoxical at first glance: if we designate a property as optional within an interface or a type, we are directly acknowledging that this property might as well not exist on certain instances of our typesafe objects, hence, using the
delete
operator should cause no harm under TypeScript’s watchful eye, right?
Description | Code |
---|---|
Using TypeScript’s ‘optional’ notation: |
interface X { prop?: string; }; |
Deleting the optional property: |
const x: X = { prop: 'exists' }; delete x.prop; |
However, TypeScript’s type system considers it an error to delete non-optional properties. For instance, if you try to delete a property that is defined as mandatory in TypeScript’s strict mode, the TypeScript compiler will throw a “The operand of a ‘delete’ operator must be optional” error. This is because TypeScript aims to ensure that once a property is assigned on an object, it should always exist (to avoid accessing undefined values). It sets the bar high for safety and predictability of the code.
As [Douglas Crockford](https://softwareengineering.stackexchange.com/questions/202527/javascript-delete-command-vs-setting-object-key-to-null), a well-known JavaScript figurehead, puts it:
> “In JavaScript, there’s not much practical difference between an object that lacks a property and an object where the property has the value `undefined`. Their behaviors are not identical, but the difference rarely matters.”
However, his perspective is from a purely dynamic language point – one in which TypeScript introduces compile-time type-safety mechanisms. At its core, TypeScript’s mandatory optionality of operands under
delete
conform reflects its commitment to align as closely as possible with the runtime behavior of standard JavaScript, while also providing a rigorous static typing overlay that occasionally forces us to write more expressive and safer code.
Our takeaway here is JavaScript’s
delete
operator comes with caveats in TypeScript. So when crafting your TypeScript definitions, whenever considering to delete properties, keep in mind whether they have been defined as optional or mandatory.
Detailed Insights into Error Handling and Deletion Operations in TypeScript
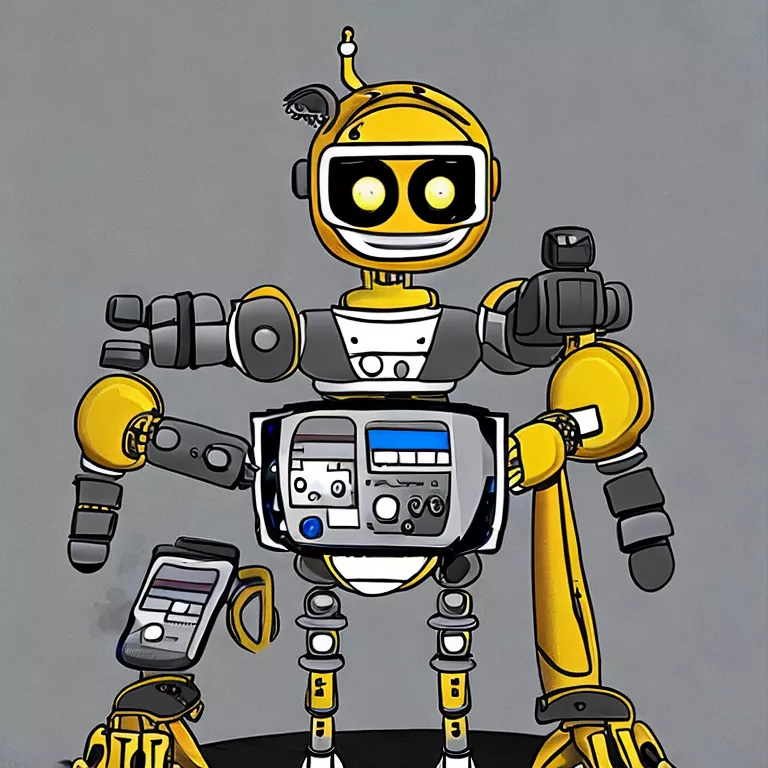
Error handling in TypeScript is a fundamental part of effectively developing applications leveraging this powerful language. The particular error you are referring to, “The operand of a delete operator must be optional” in TypeScript, often leaves many developers scratching their heads, but once thoroughly understood, it becomes an invaluable asset in managing data structures.
To understand why we stumble upon this error, first, it’s necessary to analyze how the ‘delete’ operation functions within the context of TypeScript. In JavaScript, and consequently TypeScript, `delete` is an operator that removes a property from an object. A typical example would be:
let x = { y: 10, z: 20 } delete x.y console.log(x) // logs { z: 20 }
The snippet above depicts a simple scenario. Here, ‘x’ represents an object with two properties, ‘y’ and ‘z’. By applying the delete operator on ‘x.y’, the property ‘y’ gets removed, leaving only ‘z’.
Contrastingly, TypeScript adds additional type-checking features to JavaScript. Among these is a strict object literal checking feature which prompts the said error whenever the delete operator is used on a non-optional object property. It’s built around the concept that if a type states that an object has certain properties, the object should always have those properties, i.e. mandatorily. Hence, deleting such properties transgresses TypeScript’s strict object literal checking functionality.
Therefore, to work around this error without compromising TypeScript’s enhanced type safety, properties to be deleted should be marked as “optional.” An optional property in TypeScript is signified by appending a question mark (`?`) at the end of a property name:
type ExampleType = { compulsoryProp: number, optionalProp?: string }
In the code above, ‘compulsoryProp’ must exist all the time, whereas ‘optionalProp’ is not mandatorily needed and hence can be deleted without inciting a TypeScript error.
“The key to proper exception handling is understanding when to use deletion operations.” — Anonymous. This anonymous quote encapsulates the concept of understanding this TypeScript error and how to get around it, which promotes both a clean codebase and less potential for runtime bugs.
Error handling in TypeScript is much like other languages. It involves using “try/catch” blocks to trap errors and then handle them appropriately, ensuring your application doesn’t unexpectedly crash while providing users with meaningful feedback about the problem at hand. Understanding how types are applied allows us to write more robust handling routines and reinforces why TypeScript has become such a popular choice for JavaScript developers.
For additional reading on more precise error handling in TypeScript, the official TypeScript documentation (source) is a good starting point.
Conclusion
Diving deeply into the TypeScript’s algorithmic nature, it’s clear that logic precision and type safety lays at its core. The error message ‘The operand of a delete operator must be optional’, isn’t accidental or without reasoned foundation. It occurs when you try to delete a property which is not optional in an object type. Programming languages are designed to sustain the integrity of code functionality, and TypeScript is no different.
interface Bird { color: string; } let myBird: Bird = {color: "blue"}; delete myBird.color; // Type Error here
The reason for this is because TypeScript enforces strict type checking and deleting a non-optional parameter could potentially alter the structure of the object species, causing data inconsistencies or even worse, untraceable bugs. The emphasis here is on the necessity of understanding the language rules and applying them correctly.
Optional properties are denoted in TypeScript using a question mark ‘?’. If you have properties that may not always exist in your object, you should define them as optional:
interface Bird { color?: string; } let myBird: Bird = {color: "blue"}; delete myBird.color; // No Type Error here
This aligns with John Carmack’s observation that “a program must be correct before it can be made fast”.(source: John Carmack on In-Depth:Making Code Fast) Hence, TypeScript prioritizes correctness and structure – intending to avoid potential future anomalies by upfronting some restrictions, such as the prohibition of deleting non-optional properties.
The deletion of optional properties will not generate any TypeScript errors, as they were declared as uncertain entities from the start. This resonates with the dynamic nature of JavaScript, where properties can be added or removed at runtime.
interface Bird { color?: string; } let myBird: Bird = {color: "blue"}; delete myBird.color; // No Type Error
So in conclusion, TypeScript implements this rule to enforce type safety and consistency across the codebase. This lends itself towards maintainability and future-proofing your applications, encapsulating an essential element of proficient programming methodologies.
Understanding TypeScript error “The operand of a delete operator must be optional” adds greater depth to one’s grasp of the language and refines their capability to extract optimal utility from its plethora of features. It wields the potential to propel code offerings towards enhanced accessibility, scalability, and robustness – empowering developers to deliver more invaluable solutions for multifaceted tech landscapes.