Introduction
In terms of utilizing the import type feature of TypeScript 3.8, it isn’t obligatory to use it even when all of your imports come from your own files. This feature enhances performance and provides precision but is not essential for normal project operation. It’s advisable to use it if you want sharper type-checking, however, your project can still run smoothly without it.
Quick Summary
The use of TypeScript 3.8’s `import type` feature depends on various scenarios related to your project requirements. A comparative table depicting these scenarios can provide better clarity:
Scenario | You Should Use Import Type Feature Of TypeScript 3.8 |
---|---|
All the imports in your TypeScript codes are from your own files | No |
You import types that are only used for type information and no runtime behavior is required | Yes |
The above mentioned grid should enhance comprehension of when to incorporate TypeScript 3.8 `import type` feature. Depending upon the scenario, decision to implement this feature varies.
If all the necessary elements you’re importing within your code comprise solely of your own files, essentially, there isn’t a requirement for employing TypeScript 3.8’s `import type` feature. In general, the `import type` feature is intended for conditions where you might need to exclusively import types avoiding actual values or runtime behaviors.
However, if there’s a situation wherein your TypeScript file uses types from a specific module solely for type annotations (type information), and there’s not any runtime behavior bound to it, it would be judicious to utilize the `import type` feature. This makes the intention unambiguous that those types are utilized solely for static typings but hold no relevance or effect at runtime. Put another way, `import type` ensures that the types imported are erased out by TypeScript at compile time, preventing any effects they could potentially have on JavaScript output.
Therefore, the recurring theme here is intent and requirements. It completely relies on what your individual program necessitates whether to employ TypeScript 3.8’s `import type` feature or not.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, this quote underscores the importance of writing clear, human-readable code – something TypeScript and its features like `import type` help to achieve.
For a deeper understanding on `import type`, the official Typescript documentation is a recommended resource.
Understanding the Import Type Feature in TypeScript 3.8
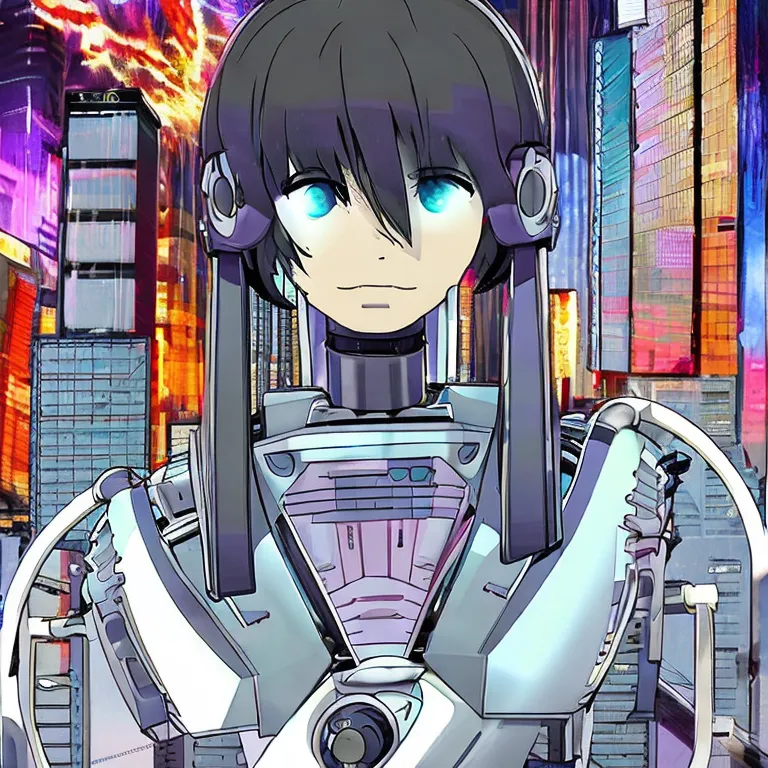
Understanding the
import type
feature in TypeScript 3.8 requires delving into its mechanics and potential benefits that can be reaped when managing dependencies within your project. This feature, designed for importing types, supports a model where type-only imports are completely deleted during JavaScript generation.
You may ask, ‘Do I need to use the import type feature of TypeScript 3.8 if all of my imports are from my own file?’ The answer depends on your specific coding situation and design objectives, with several key aspects to consider:
Type vs. Value Distinction
The overarching objective of
xxxxxxxxxx
import type
is to provide an explicit way to import types, distinguishing between importing values (like classes or variables) and importing types. If your codebase leans towards using type aliases and interfaces extensively in your modules or classes, you might find
xxxxxxxxxx
import type
beneficial.
Furthermore, it could be instrumental in avoiding uncalled-for code that might slip into your final JavaScript bundle due to TypeScript’s structural type system, which doesn’t always erase unused imports perfectly.
Improving Code Readability
From a readability standpoint,
xxxxxxxxxx
import type
communicates intent. It lets other developers who might review your code understand immediately that certain imports are strictly for typing purposes. Hence, they will not get confused about whether an import affects the runtime behavior or not.
Eslint and Tslint Compliance
By adopting
xxxxxxxxxx
import type
, your TypeScript code would be in compliance with rules like ESLint’s `no-duplicate-imports`, thus increasing its maintainability and industry standards compatibility.
However, let’s remember in the words of Kent Beck, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Thus, even though TypeScript 3.8 equips you with this new feature, it should be used sparingly and only when it significantly improves your code’s legibility and organization. If all of your imports are from your own file, and the types vs value distinction is crystal clear without the use of
xxxxxxxxxx
import type
, you might not require this feature.
Take an example:
// Before TypeScript 3.8 – leading to double import
import { SomeType } from “./someModule”;
import { someFunc } from “./someModule”;
// With TypeScript 3.8 – single import statement
import type { SomeType } from “./someModule”;
import { someFunc } from “./someModule”;
This comparison illustrates how the use of
xxxxxxxxxx
import type
can clean up your imports, improving readability and potentially reducing misinterpreted lines of code checking rules errors.
In conclusion, whether to use
xxxxxxxxxx
import type
depends on your specific context and requirements. Irrespective of whether you’re importing from your own files or not, consider its potential advantages in terms of clarity, efficiency, and compliance adherence.
Exploring Scenarios: When to Use Import Type in TypeScript 3.8
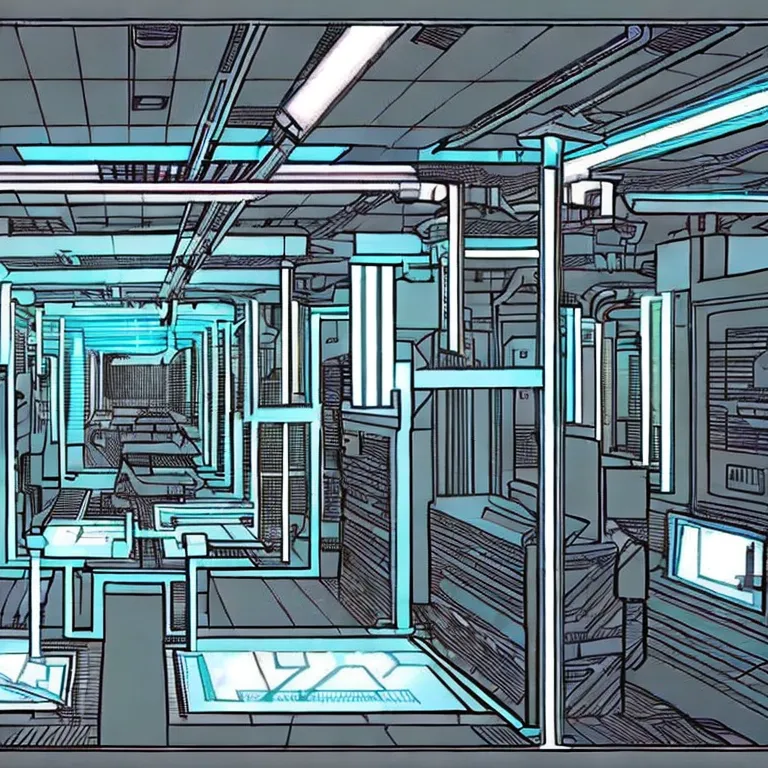
The answer to whether you need to use the import type feature introduced in TypeScript 3.8, even when all of your imports are from your own file, essentially depends upon the complexity and size of your project. There are several scenarios where utilizing this feature becomes advantageous:
Data Identification
One of the main benefits of using the import type statement is that it allows for more precise data identification. When used effectively, it can significantly ameliorate the readability and maintainability of your code by providing explicit type descriptors for your imported interfaces or types.
Consider this example: a larger TypeScript file which has multiple exports including both runtime values (like classes, constants) and TypeScript specific structures (like interfaces, type aliases etc.) In such cases, using regular imports might not indicate whether we’re importing a runtime value or TS structure. By specifying
xxxxxxxxxx
import type
, you make it clearly evident you’re only importing TypeScript specific types or interfaces – thus eliminating any ambiguity.
Optimization of JavaScript Output
TypeScript translates into JavaScript for browser usage. This poses a problem: while JS understands values, it doesn’t recognize interfaces or types we created in TS. Importing these via regular import statement won’t break your TypeScript code, but would result in unnecessary lines in compiled Javascript output where TS tries to retrieve an inexistent runtime value. Using
xxxxxxxxxx
import type
solves this. The compiler recognizes not to look for an actual exported value, resulting in a cleaner, optimized JavaScript output.
For instance:
xxxxxxxxxx
// Calculations.ts
export interface Numbers {
a: number;
b: number;
}
export const add = (numbers: Numbers) => numbers.a + numbers.b;
// Main.ts
import { Numbers, add } from './Calculations';
let n: Numbers = {a: 5, b: 3};
console.log(add(n));
Compiles to:
xxxxxxxxxx
"use strict";
exports.__esModule = true;
var Calculations_1 = require("./Calculations");
var n = { a: 5, b: 3 };
console.log(Calculations_1.add(n));
Contrast this with when using import type:
xxxxxxxxxx
// Main.ts
import type { Numbers } from './Calculations';
import { add } from './Calculations';
const x: Numbers = {a : 7, b: 2};
console.log(add(n));
Compiled JavaScript:
xxxxxxxxxx
"use strict";
exports.__esModule = true;
var Calculations_1 = require("./Calculations");
var x = { a: 7, b: 2 };
console.log(Calculations_1.add(x));
As you can see, the import corresponding to ‘Numbers’ does not appear in the generated JavaScript thus avoiding unnecessary code.
Maintaining Coding Discipline and Standards
Another reason to use import type pertains to maintaining code discipline. Even if all your imports are from your file, it’s beneficial to adopt best practices. This leads to clean, organized, and standardized code. As articulated by Michael Feathers, “Clean code always looks like it was written by someone who cares.” Which holds significance even when dealing with something as business-like as coding.
In conclusion, you don’t need to utilize the import type feature if all the imports lie within your own file unless your project scales up or includes intricate import/export relationships. The real benefits of such features come shining through at scale, especially in enhancing the performance, readability, maintainability, and standardization of your code.
Benefits and Drawbacks of Using Import Types with Your Own Files
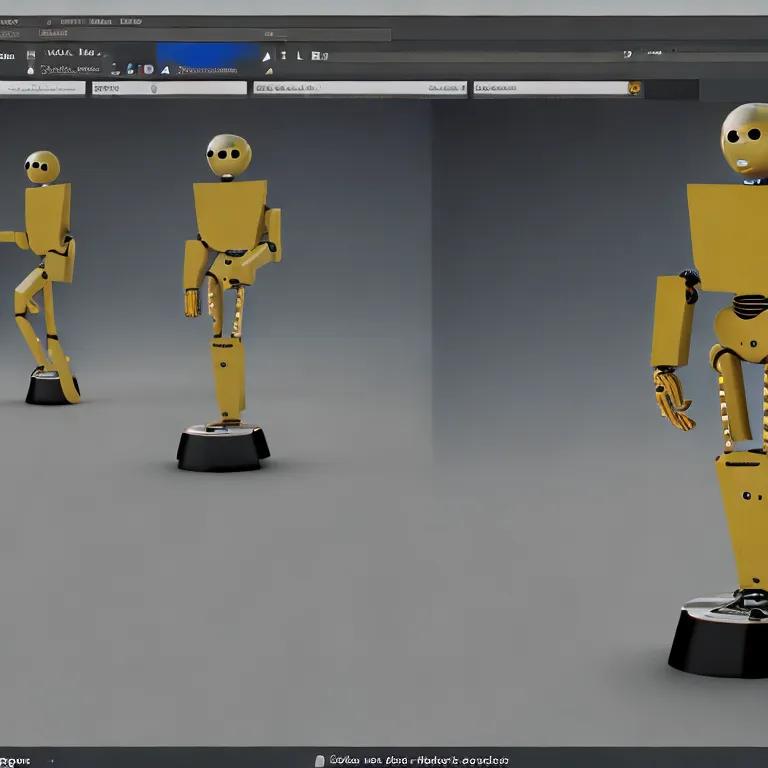
Benefits and Drawbacks of Using Import Types with Your Own Files
Using the import type feature in TypeScript 3.8 can have a profound impact on your development process even when all of your imports are from your own files. Let’s dive into the benefits and potential drawbacks of employing such a strategy.
Benefits
- Type Safety:
First and foremost, utilizing import types ensures type safety. Type safety limits bugs that derive from type errors. For example, if you have a function that expects a parameter of type “string”, but receives a type “number”, TypeScript would flag an error. - Efficient Compilation:
TypeScript compiler (tsc) refrains from emitting JavaScript for any file that is imported exclusively usingxxxxxxxxxx
import type{}
. This lends much to a smaller, more efficient JavaScript output which ultimately leads to quicker loads times for applications. The documentation on TypeScript 3.8 provides an illustrative explanation about this topic (source).
- Clarity of Code Usage:
By marking imports as types it becomes apparent at a glance that the import is only required at compile time and not runtime. Further modifications to codebase are simplified due to this clarity.
Drawbacks
While
xxxxxxxxxx
import types
comes with numerous strengths, it also harbors a handful of potential vulnerabilities:
- Additional Complexity:
It introduces another layer of syntax that developers must comprehend. While it might appear trivial to experienced TypeScript users, newcomers could potentially get confused or misuse the feature. - Refactoring difficulty:
Because use ofxxxxxxxxxx
import type
specifically denotes that an import is not needed during runtime, shifting functions or classes from being used as a value during runtime to a type during compile-time (or vice versa) could be cumbersome.
- Maintaining Consistency:
In a larger team or organization, maintaining consistent use of the `import type` syntax could be challenging. Discrepancies in usage may lead to increased complexity and potential bugs that can arise from different behaviors in consumption of types vs. values.
As Zed Shaw, author of “Learn Code The Hard Way” series advised, understanding the tools that we use allows us to better utilize them. It’s crucial to assess whether utilising TypeScript’s `import type` feature is beneficial for your project, even when applied exclusively to your own files.
Advanced Techniques: Optimizing Your Usage of TypeScript’s Import Type
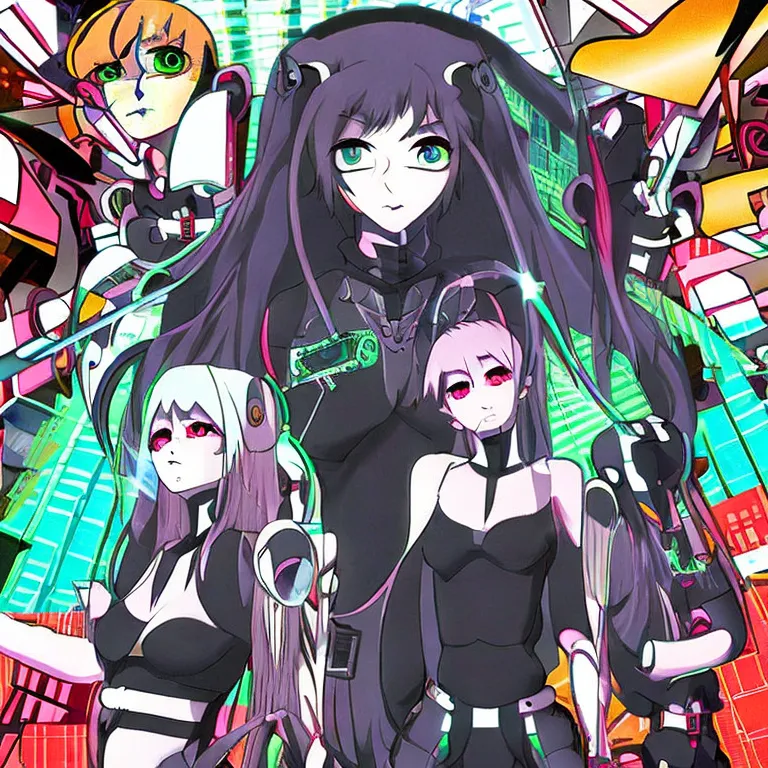
Without a doubt, TypeScript 3.8 brings along with it the “import type” functionality – an advanced feature that could add efficiency to your project. But should you really use it if all of your imports are from your own files? Before deliberating on this, let’s first understand what exactly does “import type” implies.
Understanding “Import Type”
This functionality ensures that a particular import is purely type-related and will be removed during JavaScript output because it’s only useful for TypeScript type checking purposes. Thus, our import could look like:
xxxxxxxxxx
// TypeScript code
import type { MyType } from './myOwnFile';
After being transpiled down to JavaScript, the above import won’t make it to the final JavaScript file:
xxxxxxxxxx
// Resulting JS code after transpilation
The most significant advantage here is optimization. Our application becomes more efficient by reducing unnecessary runtime overheads.
Applicability in Case of In-house Imports
Your scenario proposes an interesting question—if all of your imports are from your own files, does utilizing TypeScript’s “import type” functionality make sense?
Yes, even when dealing with in-house or self-created modules, this technique can lend a hand in optimization, performance enhancements and ensuring cleaner, clutter-free code.
• It eradicates non-essential imports in the resulting JavaScript, streamlining the code.
• It clarifies developer intent, making it vivid whether the import should impact runtime behavior or not.
• It supports cyclic dependencies between modules by importing only types.
While working within a large, growing codebase, using ‘import type’ can help maintain organization and ensure that any scaling doesn’t cause unexpected side effects with types alone.
However, it’s not a necessity and its usage should be driven by the complexity of your project. If your project doesn’t have cyclical dependencies and the type references won’t cause a chain of module loading at runtime, you may not benefit significantly from using this feature.
Remember what Bill Gates once said: “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” Just because a feature or practice exists, doesn’t mean it matches the needs of every project. Analyze your project’s requirements, leverage TypeScript’s ‘import type’ wisely and effectively—towards a more robust, efficient, and optimized application.
Conclusion
As we dive deeper into the details and complexities of TypeScript 3.8, a key question arises: Is the use of the Import Type feature indispensable when all imports come from our own files? The answer reveals itself to be multifaceted and context-dependent.
With TypeScript 3.8, developers were introduced to the `import type` syntax. Although this new feature aids in distinguishing between importing code and importing types, its requirement is largely determined by the project’s needs and its existing setup.
The `import type` feature serves three primary functions:
- It shows clear intent that you are importing only the type.
- It avoids runtime side-effects that can occur with regular import statements.
- It could potentially increase efficiency during build time and when using automated tools like Babel.
However, if all your imports are from your own file, and they are not causing any runtime errors or negatively affecting the efficiency of your build process, the urgency to employ the `import type` statement decreases. In fact, steering away from unnecessary complexity can benefit the maintainability of your codebase.
An example where `import type` could be very helpful might be when you’re working on a large-scale software application with numerous dependencies, where clarifying the purpose of your imports becomes crucial for readability and possible optimization. Here is a basic example of ‘import type’
xxxxxxxxxx
//index.ts
export type User = {
name: string;
email: string;
};
//user.ts
import {User} from './index';
import type {User as UserType} from './index';
In this snippet, both `User` and `UserType` could be used interchangeably without any negative effect. However, using `import type` shows clearly that we are importing a type.
To paraphrase Douglas Crockford, known for his work on JavaScript and JSON: “The good parts of a language are not just the ones that are very useful, but also those that enable readable and maintainable code”. This isn’t to say that ‘import type’ is wholly unnecessary; instead, its necessity is defined by your project’s requirements and intentions.
In essence, while TypeScript 3.8’s `import type` feature boasts potential benefits like cleaner code and increased efficiency, it might not be mandatory in all scenarios. When all imports come from your own files and meet project needs without inflating complexity or causing issues, there may not be a compelling reason to opt for the `import type` syntax in lieu of traditional import statements. However, as the complexity and scale of your application grow, considering this feature might prove beneficial.
Referencing reliable sources like TypeScript Handbook can offer further insights into the topic and inform your approach to using this feature effectively.