Introduction
The primary reason that catch clause variable type annotation must be “any” is due to the vast array of potential errors that may arise in programming, necessitating a broad, encompassing declaration to effectively handle and resolve them.
Quick Summary
When working with TypeScript, it’s essential to understand the peculiar nature of catch clause variable type annotation. The rationale behind this can be summarized in a table:
html
Characteristic | Description |
---|---|
Dynamism in Errors | The error thrown could be any value; not necessarily an instance of Error. |
Type Safety | TypeScript aims to provide static types for safety but errors are inherently dynamic. |
Compatibility | Preserving compatibility with JavaScript is vital, where any value can be thrown. |
Analyzing the presented information helps us comprehend the nature of this requirement in TypeScript.
Firstly, one needs to appreciate JavaScript’s dynamic nature, from which TypeScript evolved and around which it is constructed. Thus, while TypeScript aims at introducing static type checking, there are certain aspects, like error handling, where this dynamism cannot be neglected due to inheriting JavaScript’s characteristics. Therefore, the error caught in a `catch` clause can essentially be any value as in JavaScript, justifying why the type to always be `any`.
Secondly, this observance facilitates type safety. TypeScript’s core principle pivots around establishing a safer coding environment by flagging potential issues at compile-time rather than run-time. By mandating that the catch clause variable type annotation must be `any`, the language ensures that developers don’t make any presumptions about the type or structure of the error caught therein.
Lastly, maintaining compatibility with JavaScript plays a serious role in this design choice. Any constraints put on the error type in TypeScript would impede upon its overarching principle of being a strict syntactical superset of JavaScript, possibly introducing insurmountable difficulties for developers migrating from JavaScript to TypeScript.
As Gilbert Landras correctly stated, “A language that doesn’t affect the way you think about programming is not worth knowing.” The manner in which TypeScript manages error handling indeed calls for an adjustment in how we approach our code, and this phrase perfectly illustrates why these changes are meaningful.
Understanding the ‘Any’ Type Annotation in Catch Clause Variable
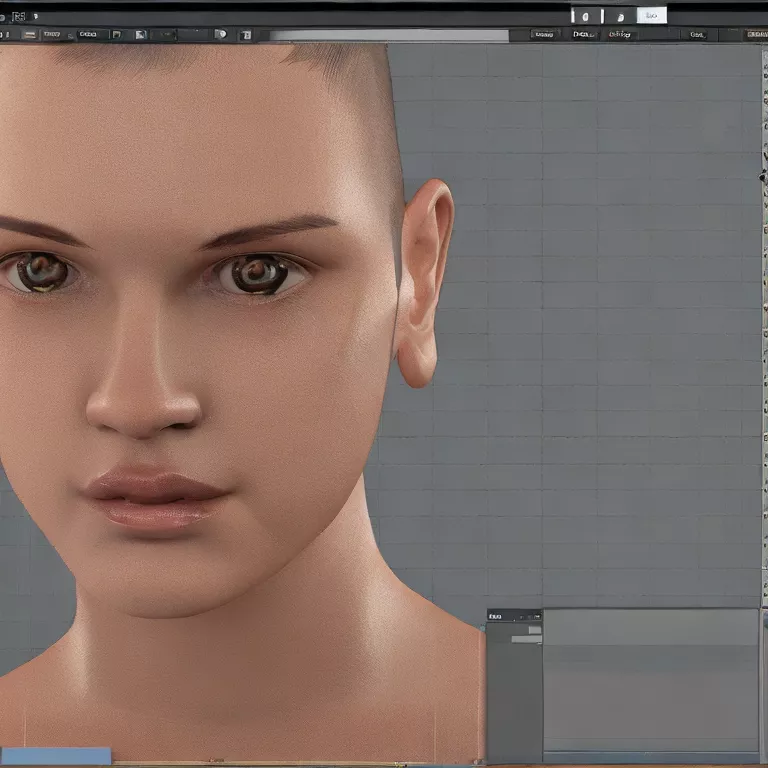
Understanding the ‘Any’ type annotation in a catch clause variable is essentially understanding TypeScript’s flexibility and robustness in handling errors. This is particularly relevant with respect to why typescript insists on the type annotation ‘any’ for catch clause variables.
Now, let’s delve into the details:
What’s TypeScript ‘Any’?
The ‘any’ type in TypeScript is a powerful way to work with existing JavaScript, allowing you to opt-in and out of type-checking during compilation. It’s essentially a supertype of all other types and it represents any kind of values, making it capable of performing any operations.
let notSure: any = 4;
notSure = "maybe a string instead";
notSure = false; // okay, definitely a boolean
Why Should It Be Used in Catch Clauses?
As per the ECMAScript standards, an error object that can be thrown as an exception might be an object, a primitive, null, or undefined. Hence, the true nature of what could possibly be caught in a catch clause is unknown till runtime.
xxxxxxxxxx
try {
// ...
} catch (error) {
console.error(error);
}
In this scenario, TypeScript applies the ‘any’ annotation to the catch clause variables. This is to ensure that developers can handle exceptions of ‘any’ type without being hindered by TypeScript’s static type checking mechanisms.
The Reasoning Behind It
Anders Hejlsberg, the lead architect of TypeScript, once said, “JavaScript is really quite flexible and will allow you to throw any kinds of things. Therefore, representing those exceptions as any kind of special specific type isn’t necessarily accurate.”[1](https://github.com/microsoft/TypeScript/pull/20006#issuecomment-346233325)
This aligns with TypeScript’s approach to provide error resilience, and ultimately the rationale behind why catch clause variable type annotation must be ‘any’. With this design decision, even when an unknown error is encountered at runtime, the system avoids cascading failures and the program remains robust.
Dealing with ‘Any’ in Catch Clauses
Despite the ‘any’ implication on catch clauses, good practices can be applied using type guards and conditional checks within the catch block.
xxxxxxxxxx
try {
// .....
} catch (error: any) {
if (typeof(error) === 'string') {
console.log('Caught a string error:');
} else if (error instanceof Error) {
console.log('Caught a generic error object:');
} else {
// handle unexpected case here
}
}
In essence, understanding ‘any’ in catch clauses uncovers one of TypeScript’s gateways for ensuring code resilience and flexibility during execution.
Reference:
1. [Hejlsberg Github Comment](https://github.com/microsoft/TypeScript/pull/20006#issuecomment-346233325)
Experimenting with Different Types in a Catch Clause
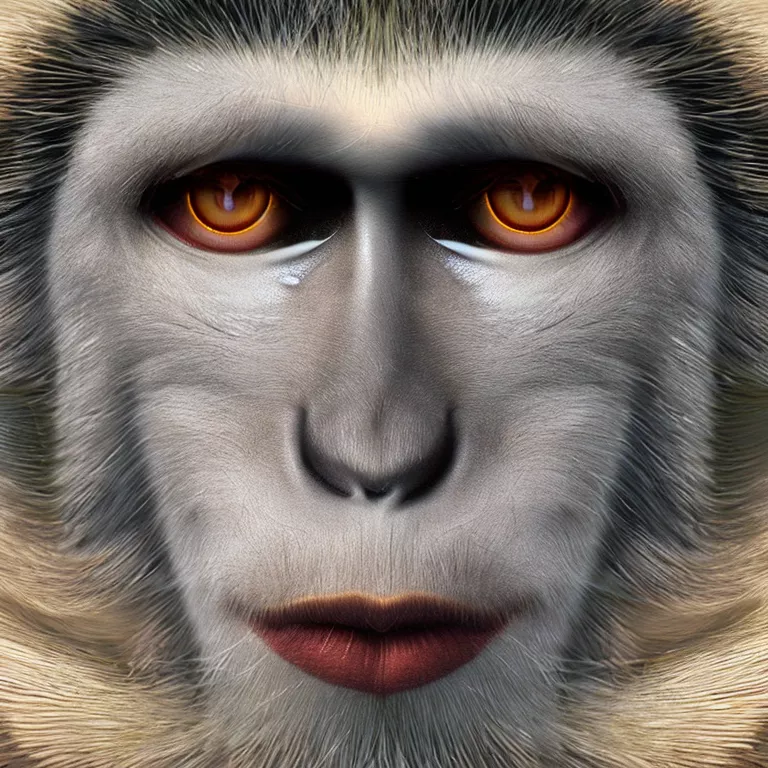
In TypeScript, the `catch` clause of a `try-catch` statement provides a way to handle exceptions or errors gracefully during the execution of your program. However, while implementing this error-handling mechanism in your TypeScript code, there are important points worth considering in relation to the type annotation for catch clause variable.
Understandably, one might think that, given TypeScript’s robust static typing feature, we could assign explicit types to the catch clause variables just like other typical variables. Let’s take a look at how it might appear:
typescript
try {
…..
} catch (error: Error) {
console.error(error.message);
}
However, while working with TypeScript, if we try to provide a specific error object type to the catch clause variable, we come across a compilation error. To understand why this is infeasible let’s dive into two significant reasons:
1. **Uncertainty of Error Types**: In TypeScript, an error might not always be an instance of the built-in JavaScript Error class. It could potentially be anything – a string, a number, an object or even undefined. If we specify a particular type within the catch clause, TypeScript would only allow operations that are valid against that particular type but it does not conform the reality and thus creates limitations.
2. **Compatibility with ECMAScript**: TypeScript always aims to be highly aligned with ECMAScript standards. According to these standards, any value can be thrown as an exception in JavaScript. Consequently, forcing the error to be of a certain type can break the code behavior when migrating between TypeScript and JavaScript.
Given these reasons, the TypeScript team decided to opt for the type ‘any’ implicitly for the catch clause variables. Here’s how it would really look:
typescript
try {
…..
} catch (error: any) {
console.error(error.message);
}
This enforces that the error object in the `catch` clause is effectively of type any, granting you the flexibility to handle all sorts of errors by ensuring that your catch block will work for exceptions of every conceivable type.
As Kernighan and Ritchie, gods among programmers and authors of ‘The C Programming Language’, remarked, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” So, using ‘any’ in TypeScript’s try-catch error handling strategy seems wise as it broadens our horizon, enabling potentially smoother debugging processes and indeed code that isn’t too ‘clever’.
For more details about TypeScript’s take on this ECMAScript specification, I recommend looking at the TypeScript 2.6 release notes.
Remember, while coding, catching an error is a process that is intrinsically flexible by its nature. This implicit ‘any’ gives us the license to be flexible when warranty and insurance against unexpected outcomes are what we need the most.
Implications of Using Specific vs. ‘Any’ type in Error Handling
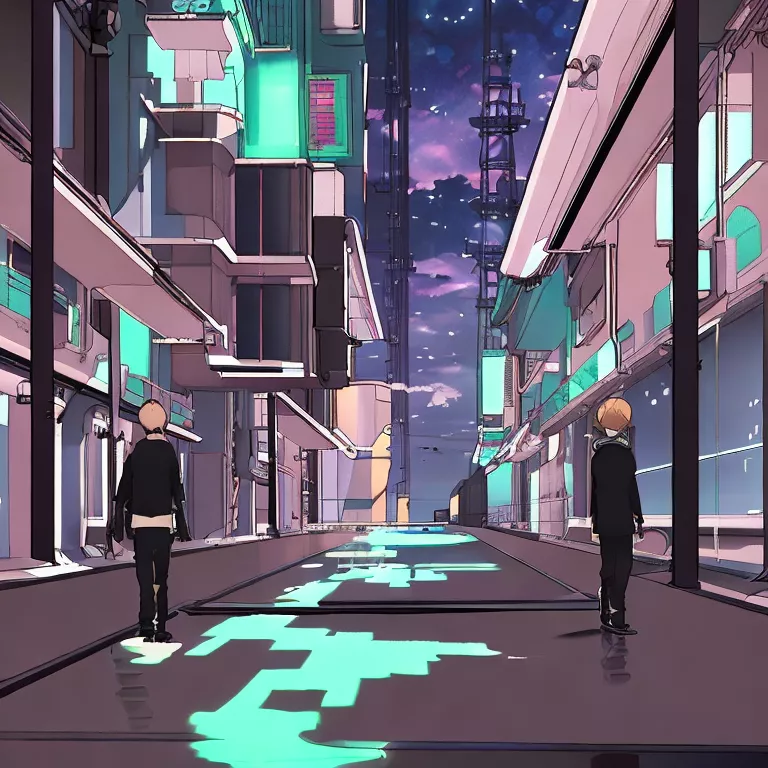
Error handling is a critical aspect of software development and Typescript offers multiple type annotations that developers can leverage. However, it is worth noting that the ‘any’ type annotation in a catch clause becomes handy when we don’t have prior knowledge of the probable error types to be handled.
Consider the example below:
xxxxxxxxxx
try {
// code prone to errors
} catch (error: any) {
console.log(error.message);
}
Here,
xxxxxxxxxx
error: any
suggests that the catch block isn’t certain about the type of error it might have to deal with. Thus, it has its advantages and drawbacks.
Benefits of using the ‘Any’ type are:
- Flexibility: Since ‘any’ signifies all possible Typescript types, it doesn’t restrain the catch clause to handle only designated error types.
- Better Debugging: Irrespective of the error nature, the catch clause will always have the ability to access properties or methods on that error object, which are sometimes crucial for debugging.
On the other hand, potential risks include:
- Poor Type Safety: Type safety is one of the main reasons why developers opt for Typescript over JavaScript. Using ‘any’ undermines this advantage to some extent because if an error has a property that’s wrongly assumed to exist, it may lead to unforeseen bugs.
- Inaccurate Error Reporting: Much like earlier points, if a catch clause erroneously tries to access a non-existent error property, it might throw extra exceptions, complicating error reporting and tracking.
A Large chunk of JavaScript library and APIs represent errors with standard-error or custom-error objects; therefore, it’s often impossible to circumscribe errors within known types. Hence Typescript declares that catch clause variables in the likes of TypeScript 4.0 could be annotated with types other than ‘any’. But this does not eliminate the necessity of ‘any’ type; instead, it provides more flexibility for developers dealing with predictable error scenarios.
In the light of discussing errors and types, famous developer Joel Spolsky once said, “Every program will expand until it can read mail.” This signifies the inevitability of errors as software increases in complexity. Therefore, carefully managing exceptions by understanding when to use an ‘any’ type versus specific types contributes to robust software. Typescript’s flexibility for variable type annotations is a beneficial tool to achieve this, but it should be wielded judiciously to prevent potential coding pitfalls related to error handling.
Case Study: Effectiveness and Limitations of the ‘Any’ Type in Catch Clauses
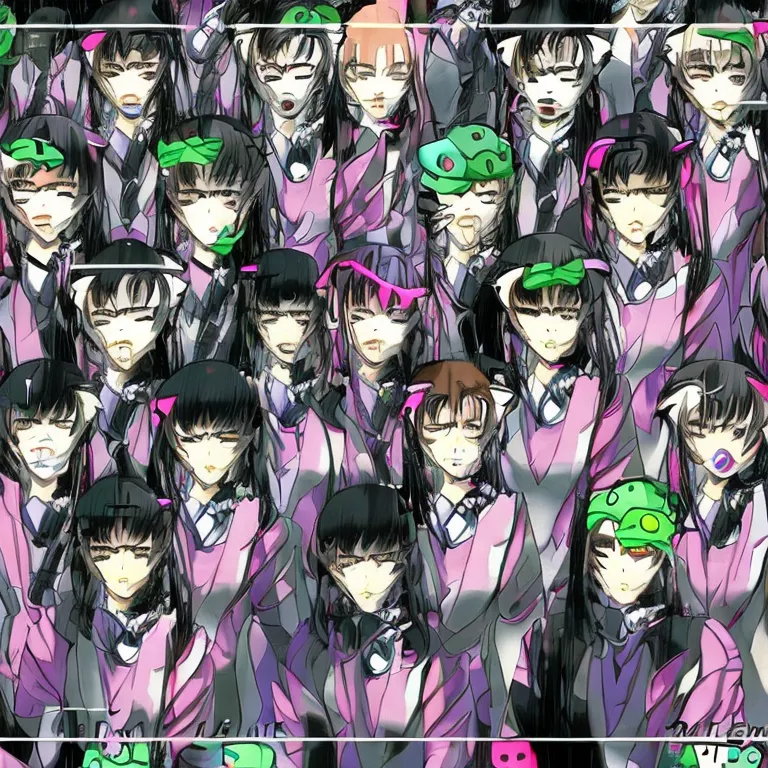
In TypeScript, a catch clause handles exceptions (errors) that are thrown during the execution of your code. When you wish to handle these exceptions in TypeScript, it’s typical to see the ‘any’ type used for the variable that represents the error object within the catch clause.
The ‘any’ type has quite a significant role and impact on the outcome of using catch clauses. Understanding its effective use and limitations require us to take an analytical approach by examining the details related to this matter.
Effectiveness of the ‘Any’ Type in Catch Clauses
– The primary benefit of using the ‘any’ type in a catch clause is flexibility. Since TypeScript adopts static types, it auto-infers type information during compilation. However, exceptions can be pretty dynamic – they might include user-defined errors, system errors, network-related errors, etc. Hence, implying a specific type to an unexpected error becomes virtually impossible. Herein lies the effectiveness of ‘any’. It accepts any kind of value and allows handling of diverse errors.
xxxxxxxxxx
try {
// Some code throws an error
} catch (error: any) {
console.log(error.message); // Access arbitrary properties or methods without TypeScript throwing a compile-time error
}
– Using ‘any’ in catch clauses also removes strict checks and allows developers more leeway when working with it. There’s no need for type checking every time you’re dealing with the error. This enhances the convenience of handling anomalies and exceptions throughout the program flow.
Limitations of the ‘Any’ Type in Catch Clauses
Despite its prevalent use, the ‘any’ type comes with certain constraints within catch clauses:
– Atypical to TypeScript’s ideology: One of TypeScript’s core benefits is boosting developer confidence via static typing. While socially accepted, using ‘any’ defeats the purpose of employing a statically typed language in the first place. Overuse of the ‘any’ type could lead to unsafe code since TypeScript can’t help you with type-related errors.
– No IntelliSense support: With ‘any’, TypeScript loses its capability to provide automatic code completion or popups, offering potential hits while coding (referred to as IntelliSense). This lack of assist might add a bit of difficulty when programming, especially for larger projects with more complex error handling scenarios.
xxxxxxxxxx
try {
// Assume we throw an instance of a custom error class "MyError"
} catch(e: any) {
console.log(e.message); // We assume MyError has a message property, but no IntelliSense assist here.
}
– Leads to silent failures: Although using ‘any’ offers convenience, it is also risky. For instance, if you develop a bug involving an erroneous property on your error object, TypeScript will not warn you. Your mistake will pass unchecked, and your exception-handling may fail silently.
As famously quoted by Satya Nadella, CEO of Microsoft, “The true measure of empathy is not just to listen, but to understand.” As TypeScript developers, it becomes our responsibility to understand and wisely choose whether to use ‘any’ in our catch clauses or counter these limitations via other methods such as user-defined types or unknown type for error handling.source
To sum up, while convenient, the usage of ‘any’ for catch clause type annotation needs careful consideration given the potential downsides. A balanced mix of ‘any’ with other type-safe options could possibly be the most pragmatic approach under this scenario.
Conclusion
The fundamental constraints of TypeScript compile-time type checking drive the requirement for a Catch Clause variable to possess a Type Annotation of ‘any.’ These constraints curtail the inherent flexibility attributed to JavaScript, causing a TypeScript Catch Clause to lose valuable type information. Consequently, a Catch Clause Variable Type Annotation transgresses into an ‘any’ type.
To further delve into this, let’s dissect these reasons in detail:
Reason | Explanation |
---|---|
TypeScript Compile-Time Type Checking | TypeScript is strict in nature due to its compile-time type checking functionality which makes TypeScript differentiates it from JavaScript. This rigidity helps evade runtime errors, but ironically also forces a Catch Clause Variable Type Annotation to inherit an ‘any’ type to be able to handle all possible exceptions that can occur. |
Loss of Type Information | To comply with these stringent rules, a Catch clause variable loses type-specific information during a TypeScript compile-time error. To amend this, TypeScript developers suchness a default fallback to ‘any’, thus allowing for generic manipulation of data irrespective of data type. |
The Any Type Safety Net | The heart of a Catch Clause Variable Annotation is to provide a secure environment for exception handling. The ‘any’ type safeguards code snippets within catch clauses by introducing a type safety net, capturing undetected anomalies or mishaps which could compromise application security & performance. |
Consider this piece of code as an example:
xxxxxxxxxx
try {
// Code prone to throw exceptions
} catch (error: any) {
console.error(`Oops! Something went wrong: ${error}`);
}
Here, the ‘any’ type accounts for all types of data including exceptions potentially thrown by the block in the try clause. This universal nature of ‘any’ provides an exhaustive safety net.
As American software engineer Kent Beck said, “For each desired change, make the change easy (warning: this may be hard), then make the easy change”. This embodies the rationale of adopting ‘any’ type for Catch Clause Variable Type Annotation. By making it an ‘any’ type, we can easily catch and handle any error thrown our way, trivializing what could have been a complex problem-solving task.
The importance of type safety should not subvert the necessity of complete coverage when handling potential exceptions. Thus, TypeScript adopts an out-of-the-box approach to maximize its robustness via the ‘any’ variable type annotation for catch clauses.