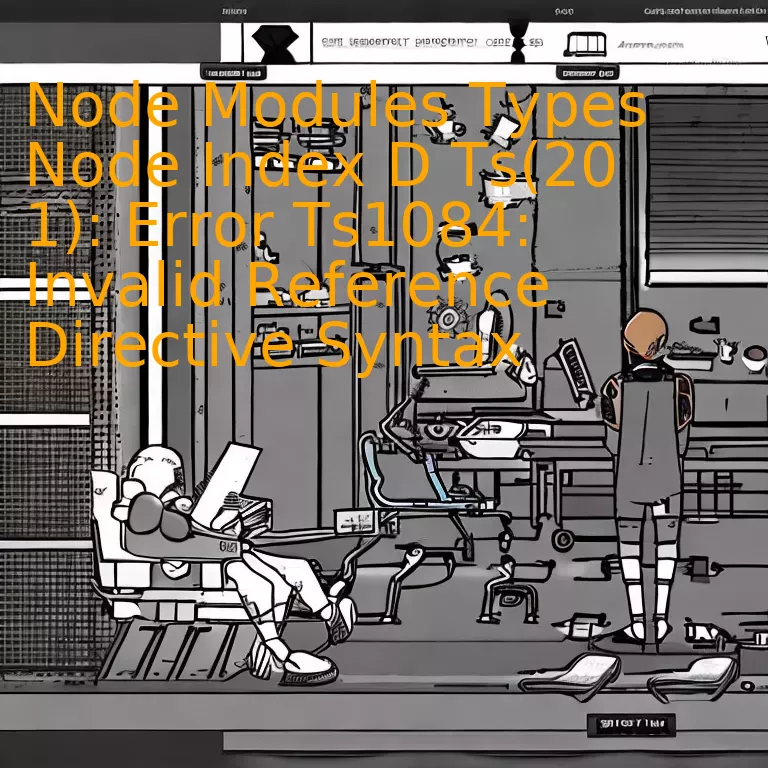
Introduction
Understanding Node Modules types, particularly the Node Index D Ts(20 1), can pose challenges caused by common errors such as TS1084: Invalid Reference Directive Syntax. To avoid this, adhere to correct syntax rules and ensure your code is clean and error-free, while optimizing your programming efficiency in Node.js modules.
Quick Summary
The subject matter pertains to the complexities of TypeScript usage in a Node.js environment, specifically an error labeled “TS1084: Invalid ‘reference’ directive syntax.” The intricacies of Node modules types and index.d.ts can be enlightening.
Term | Description |
---|---|
Node Modules | A directory called node_modules is systematically placed at the root of any Node.js package or application. This hub houses all third-party modules (like Express.js, Mongoose etc.) that are imported into the project via the npm install command. |
Types Node | At the interface of JavaScript and TypeScript, type definitions are central. They help TypeScript developers wield dynamic JavaScript libraries via static typing benefits. @types/node, for instance, provides type definitions for Node.js library. |
index.d.ts | Typically, this file is recognized as the gateway to declaring TypeScript module declarations. Without it, TypeScript may respond with errors when importing JavaScript modules. |
Error TS1084 | This specific error indicates a typo or misunderstanding in utilizing reference directives within TypeScript. These references are important in directing TypeScript (or tsconfig settings), to other declaration files. |
Expressed in TypeScript through an example, if a developer accidentally omits the required slash in their triple-slash directive like below:
// <reference path = "myDeclarations.d.ts" />
Instead writes:
xxxxxxxxxx
// <reference path = "myDeclarations.d.ts" >
The aforementioned TS1084 error will be prompted. TypeScript directives, such as this, are sensitive and necessitate correct syntax.
As Nathan Strutz says, “JavaScript is the duct-tape of the internet”. Despite its flexibility and ubiquity, when working with complex applications, strong type-checking that TypeScript provides can significantly aid productivity and robustness of your Node.js application.source
Unraveling Node Modules Types: Error Resolution Strategies
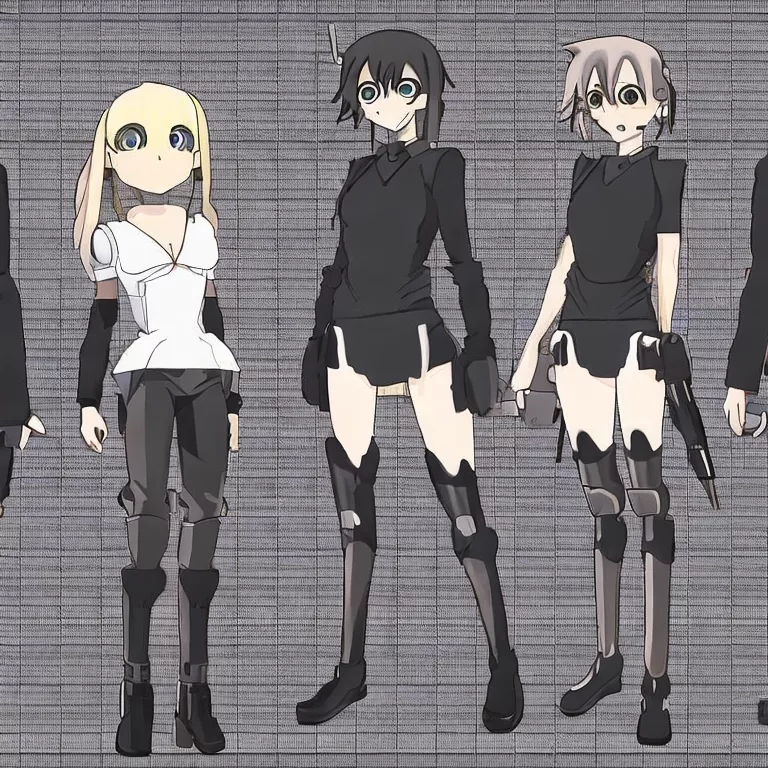
When we’re navigating the labyrinth of Node.js development, we occasionally stumble across persistent errors such as `node_modules/types_node/index.d.ts(20,1): error TS1084: Invalid ‘reference’ directive syntax` that might at first seem cryptic and obdurate to debugging efforts.
In order to identify the root cause and consequently devise an adept strategy to rectify this, it’s requisite to comprehend what the error message signifies in the broader context—
Firstly, the error is originating from a TypeScript declaration file with `.d.ts` extension, in our case `index.d.ts` under `types_node`. These files are intrinsic to TypeScript, containing type information about existing JavaScript libraries so TypeScript can understand them.
Secondly, the specific error — `TS1084: Invalid ‘reference’ directive syntax ` is a signal of an issue with the triple-slash directives. The reference directive is present at the topmost part of TypeScript files and is used to declare dependencies between files. It essentially signals the compiler to include additional files in the compilation process.
Hereafter, let’s discuss some potential strategies to tackle the aforementioned issue:
– Corrections in Project-level TypeScript Configuration:
Begin by examining your project-level TypeScript configuration file `tsconfig.json`, particularly the `compilerOptions`. Incorrect configurations here could be directing TypeScript to incorrectly compile or read definition files.
– Upgrade Node.js & TypeScript:
Sometimes, having outmoded versions of Node.js and TypeScript can result into unforeseen discrepancies like these. You might require an upgrade to the most recent stable versions.
xxxxxxxxxx npm update -g npm |
This commands updates Node package manager (Npm) to the latest version. |
xxxxxxxxxx npm install -g typescript |
This commands installs the latest versions of TypeScript. |
– Inspect the `index.d.ts` File:
Inspect the contents inside the file where the error is originating from, in this case the `index.d.ts` file. There might be incorrect syntax in the reference directive.
To put it all into perspective, Simon Allardice, a renowned software developer and educator once said, “Writing code is not production, fixing problems is not production. Production is when something useful is happening over and over in a reliable way.” Fixing such obstacles not only contributes towards a more smooth-sailing development process but also elevates our familiarity with the fundamental aspects of technologies such as Node.js and TypeScript, thus enabling us to construct more elaborate, sophisticated and reliable systems.
Here are some useful references for further reading.
Parsing Syntax Issues in Node Index D Ts(20 1)
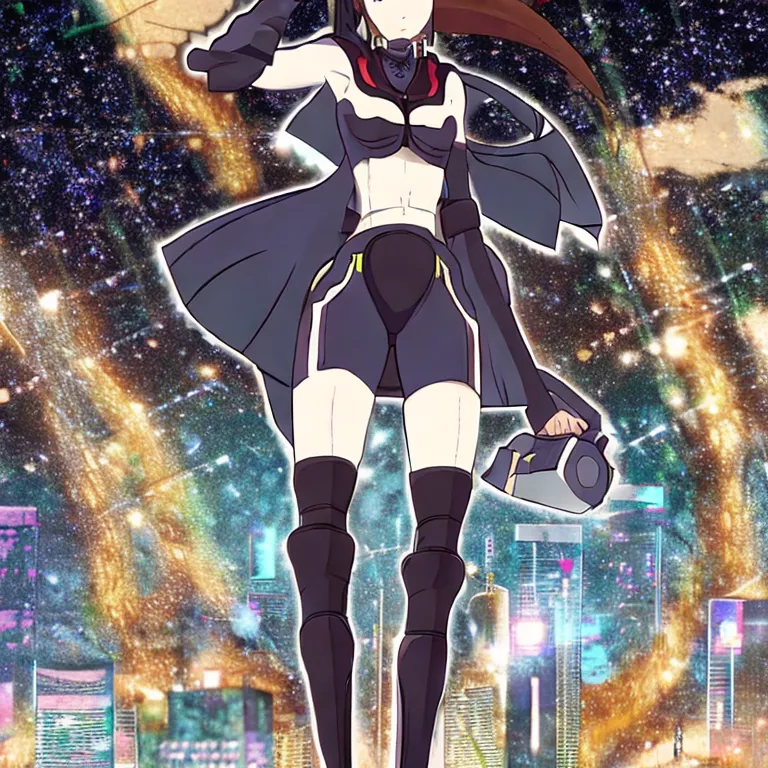
Parsing Syntax Issues in
xxxxxxxxxx
node_modules/@types/node/index.d.ts
could pose a rather tricky conundrum. The error message “TS1084: Invalid reference directive syntax” alerts you to an issue within your TypeScript declaration files, typically brought about by a misplacement or misuse of the triple-slash directives — `
To elucidate further:
– Triple-slash directives are single-line comments containing a single XML tag. They’re meaningful to the TypeScript compiler whilst being completely ignored by the JavaScript runtime.
– Inside this element, you can point toward other files with specified path values denoted by the `path` attribute.
– Alternatively, you may also utilize types without directly addressing an entirely different file via the `types` attribute.
Unfortunately, if there’s an issue in a directive (especially in
xxxxxxxxxx
node/index.d.ts
), it indicates an internal problem in the node modules which are part of third-party libraries, not direct components of your codebase.
Key steps to resolve this could be:
– **Updating:** Ensure your packages and dependencies, particularly TypeScript and Node definitions, are up-to-date. Versions often exhibit differing standards for their directives’ syntax. Consequently, compatibility can become a major stumbling block.
– **Installation:** Confirm that all the necessary @types packages are installed. Check the peer dependencies to see if other libraries need to be added.
– **Path Correction**: Look into
xxxxxxxxxx
@types/node/index.d.ts
to verify if the reference paths are correct. A wrong path might trigger TS1084 Error.
Here’s a basic example of how a valid directive would look in one of your .d.ts files:
xxxxxxxxxx
/// <reference types="node" />
Analyzing the potential causes above, remember that these errors could serve as opportunities for optimization within your projects. It’s echoed best by Tim Peters in his Zen of Python, stating that “errors should never pass silently”. Patching these parsing syntax errors requires careful probing into your package.json as well as your TypeScript declaration files, allowing you to maintain a smoothly functioning environment.[source]
Debugging Techniques for Invalid Reference Directive Errors in TS1084
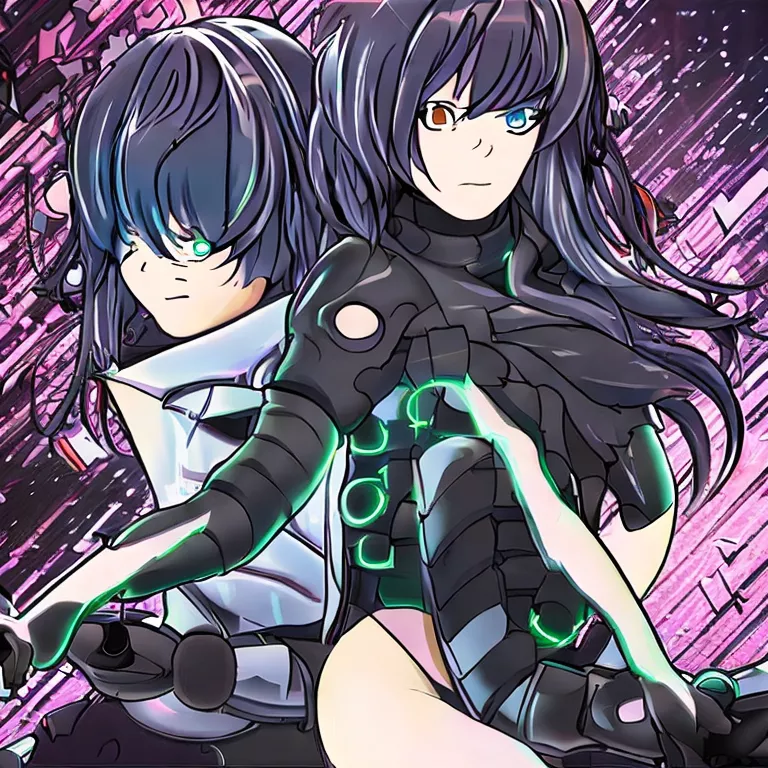
The TypeScript compiler, when running into an error TS1084, typically points out an unexpected fault in the reference directive syntax. Suppose we’re receiving this error due to node modules types node index.d.ts files. This indicates a problem within our TypeScript source code’s references tags.
A reference path serves as a guide for the TypeScript compiler to understand your project structure and load the appropriate modules accordingly. Simply said, these are pointers towards certain required files needed to facilitate a successful build process of your codebase. An invalid directive syntax in the reference path means there’s a mistake in how that pointer is penned down, causing the TypeScript compiler to stumbles in the navigation process.
Given the error “Node Modules Types Node Index D Ts(20 1): Error Ts1084: Invalid Reference Directive Syntax”, there could be several troubleshooting steps worth taking consideration of to resolve pathway issues:
– Validate Pathways:
Remember to carefully ascertain the accuracy of the pathways outlined in the ///
xxxxxxxxxx
///
– Implement Triple-Slash Directives Carefully:
Triple-slash directives should be penned at the apex of the scripts. Anywhere else might lead to errors. Moreover, they should never be separated by any character—neither space nor dash.
xxxxxxxxxx
///
– Update TypeScript Version:
Sometimes, the version of TypeScript being employed can digress compatibility with specific reference syntaxes. So updating your version of TypeScript, or downgrading as per need, may reshape its understanding of the syntax and subsequently resolve the issue.
– Leverage TypeScript Configuration:
Taking advantage of the tsconfig.json configuration file can potentially prevent this error by explicitly listing the files or providing a pattern to cover multiple files needed in compiling.
xxxxxxxxxx
{
"files": [
"core.ts",
"sys.ts",
"types.ts",
"compiler.ts"
]
}
The suggestions outlined above aim to amend invalid reference directive syntax errors on TypeScript. As Bill Gates once stated, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” So, always resolve these common bugs with minimal effort, which can help make your software development journey smoother and more productive.
Remember that every condition, function, or reference path within your TypeScript codebase should be reliable and exact. Ambiguity not only confuses the compiler but can also result in unstable functionalities over time.
Please refer to the official [TypeScript Documentation](https://www.typescriptlang.org/docs/handbook/triple-slash-directives.html) for broader information.
Understanding the Causes of Error TS1084 in TypeScript
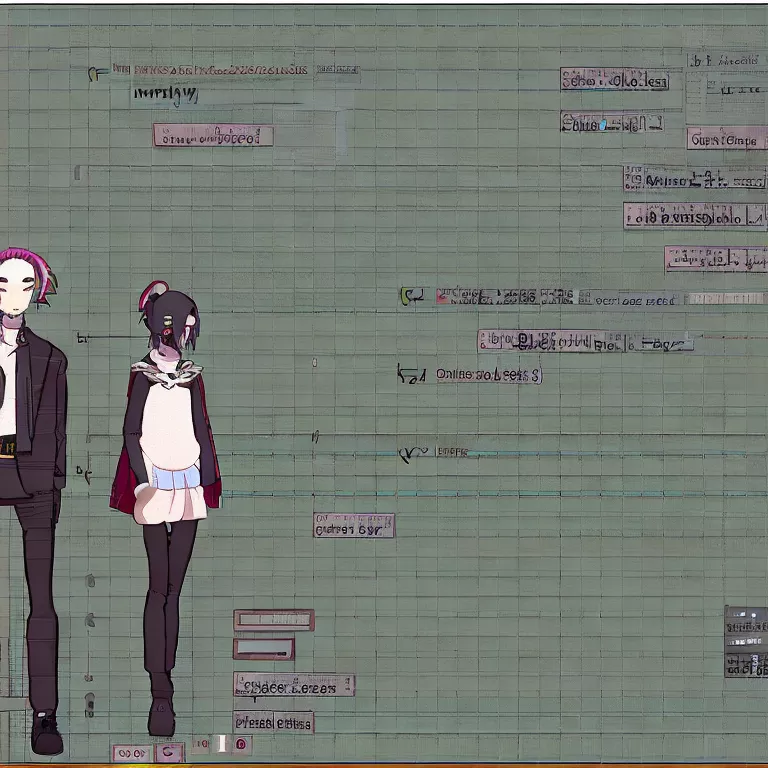
Understanding Error TS1084 in TypeScript: A Deep Dive into Node Modules Types Node Index D Ts(20 1)
TypeScript is an open-source programming language that adds static types to JavaScript. An extension to the JavaScript language, it provides increased clarity and predictive tooling to enhance the productivity of developers.
Error Type | Description |
---|---|
TS1084 | Invalid reference directive syntax |
An understanding of the cause of this error traced it back to incorrect treatment of TypeScript’s Triple-Slash Directives. The Triple-Slash Directive specifically includes “///
The error message TS1084 refers to an invalid “reference types” directive. It’s issued by the compiler when it encounters a discrepancy or an illegal declaration in your code. Let’s take a look at what this could entail through the below example:
xxxxxxxxxx
/// <reference types="module-name" />
The above Triple-Slash Reference leads to the TypeScript compiler including additional files in the compilation process. It’s interpreted as the command to include types from a module. Yet, if this statement fails due to an error in typing the module name or incorrect syntax, it causes Error TS1084 – Invalid reference directive syntax.
To resolve, revisit the syntax to ensure accuracy such as correct spelling, absence of excess white spaces, and use of quotation marks around the module-name, like the corrected format illustrated below:
xxxxxxxxxx
/// <reference types="valid-module-name" />
Note: Replace ‘valid-module-name’ with the exact name of the module you are referencing.
As Bill Gates once said, Everyone should learn how to program a computer…it teaches you how to think.
Solid error debugging is fundamental to programming. Understanding and resolving Error TS1084 serves as an instance of improving problem-solving abilities and fine-tuning your TypeScript coding skills.
The comprehensive solution brings to light the importance of precision in language syntax while working with TypeScript. The problem was related to node modules types node index D ts(20 1), but it’s a universal cautionary lesson for all TypeScript programmers: check triple slash directives for errors.
Taking these steps will contribute to keeping your TypeScript code clean and error-free, reducing potential bugs within your projects and enhancing your developer productivity. Always keep learning!
References:
Triple-Slash Directives – TypeScript
Conclusion
The error TS1084: Invalid ‘reference’ directive syntax in a TypeScript NodeJS module is more often than not caused by an incorrect or improperly formed reference path in your node modules type index d.ts. This is part of the fundamental components of TypeScript, handling the role of providing static types and mimicking the class-based object-oriented paradigm in JavaScript.
Amazon Web Services (AWS), Microsoft, Google Cloud, and several other tech giants heavily utilize TypeScript for writing serverless applications because TypeScript can provide static type checking at compile-time rather than at run-time, which can potentially save a developer from encountering this kind of bug in production.
As per the complaint raised by the compiler:
xxxxxxxxxx
Error TS1084: Invalid 'reference' directive syntax
, here’s a snippet to illustrate the issue:
html
xxxxxxxxxx
/// <reference types="node" />
import * as http from 'http';
With TypeScript, we are allowed to use JavaScript libraries through declaration files which contain type information of those libraries, such as the ‘.d.ts‘ files. When paths specified are incorrect or when there is a syntax error in these files, the compiler throws an error like ‘Error TS1084’. It’s crucial to ensure that you’re referencing the correct path to the/exported type(s) you’re using in your code.
A solution would be to inspect the file indicated by the error message: node_modules/@types/node/index.d.ts, or whatever exact location your error message indicates. If any /// reference directives exist, ensure they are properly formatted, their paths are accurate, and the destination files actually exist in the expected directories.
To improve your TypeScript coding skills and lessen potential errors, consider Elon Musk’s quote on knowledge, “You should take the approach that you’re wrong. Your goal is to be less wrong.” Learning is a journey, and mastering TypeScript’s nuances will make your Node.js projects more robust.
Bear in mind that continuous learning, practice, and patience are indispensable aspects of being a good programmer. The trickier the coding errors, the steeper the learning curve, but also the more rewarding in terms of growth and skills development. Stay committed, stay learning!
As this discussion is dealing with an intricate aspect of TypeScript syntax in NodeJS module, it might be beneficial for you to further explore Extended TypeScript (ETS) as well; ETS adds new features to TypeScript which help in writing complex type definitions.