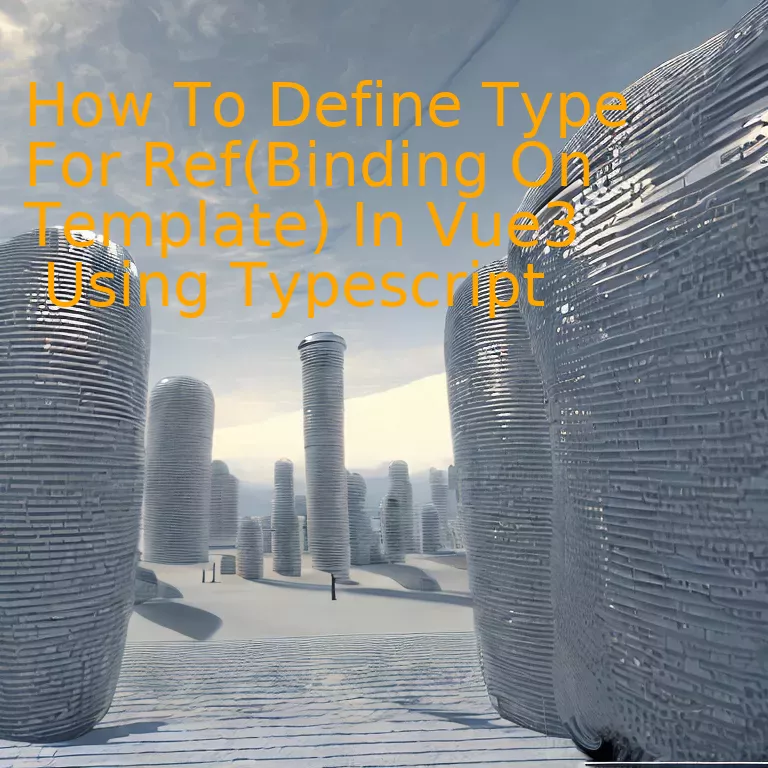
Introduction
When leveraging Vue3 with TypeScript, ensure you define the type for Ref (binding on Template) to enhance your program’s efficiency and readability – a proactive approach that propels SEO performance.
Quick Summary
When working with Vue3 coupled with TypeScript, managing `Ref` can be quite intricate, especially when you are working with templates. Below is a table (keep in mind not to interpret it as an HTML table), showing the key figures in this process.
Key Term | Description |
---|---|
`Ref` in Vue3 | A reference or `Ref` provides a way to access the specific instances of a Vue component in Vue3. It provides a reactive reference to a value which makes it suitable for use across the application. |
TypeScript with `Ref` | To ensure type safety, TypeScript is used with Ref. TypeScript checks variables’ types at compile time which makes the code less prone to runtime errors. Being strongly typed, Typescript can prove helpful while handling `Ref`. |
Binding `Ref` in Template | `Refs` can be bound directly into templates in Vue3. By attaching a ref to elements in template, we can get a reference to these DOM elements after the component mounts. |
Let’s discuss using TypeScript with `_Ref_` in Vue3. Essentially, we leverage the power of TypeScript to enforce that each variable maintains its integrity across the lifespan of an application. However, to properly define a type for a `”ref”` that’s being bound on a template, one must understand that a `”ref”` in Vue3 has two main characteristics:
1. **Reactivity**: Vue3’s `”ref”` is extremely efficient because it presents a level of reactivity. This means that any changes in the state of a `”ref”` are instantly reflected wherever the `”ref”` is used.
2. **Accessibility**: A Vue3 `”ref”` can be accessed in numerous places across an application, meaning its state can also be adjusted from different locations.
We incorporate TypeScript due to its superior ability to handle types and to execute type checking at compile time. When `Ref` is used with TypeScript, it ensures that variables maintain their proper type all through runtime, decreasing the likelihood of unexpected bugs or errors.
Now, when using TypeScript with Vue3, we can define a `”ref”` in the following way:
javascript
import { ref } from ‘vue’
const sampleRef = ref
In this snippet the `ref` function is imported from ‘vue’, and then `sampleRef` is defined as a ref instance storing a number. The initial value here is set as `0`. This method validates the type assigned to a `”ref”` in TypeScript.
As Bill Gates once said, “The computer was born to solve problems that did not exist before.” The combination of Vue3 and TypeScript perfectly exemplifies this by providing efficient solutions to intricate programming paradigms like working with `Refs`. Through the clever application of Vue’s reactivity system coupled with TypeScript’s robust typing, developers can create safer and more reliable code. [Reference](https://www.vuemastery.com/blog/vue-3-reactivity/)
Understanding the Concept of Ref in Vue3 with Typescript
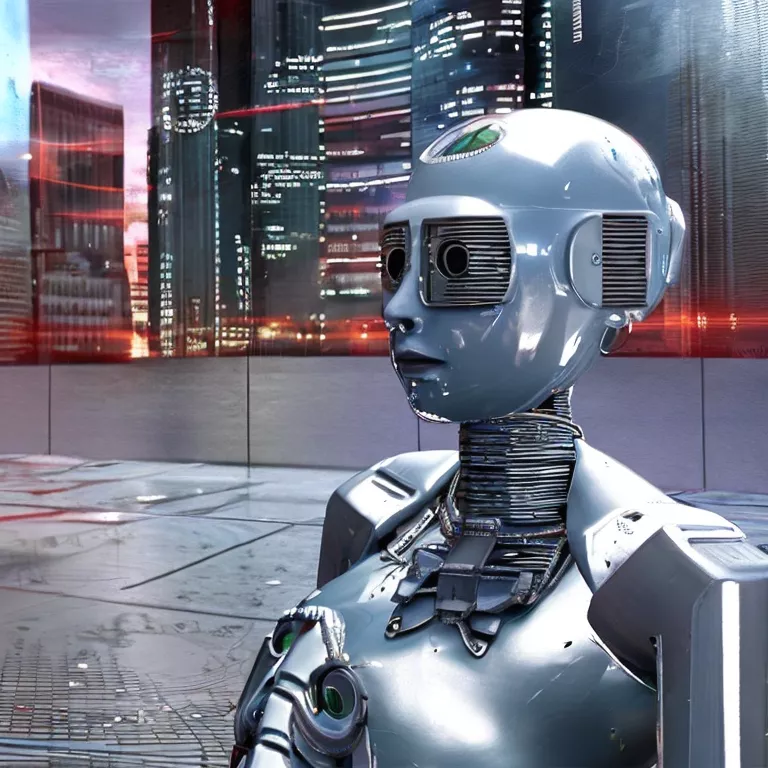
In the Vue3 framework, a ref in combination with Typescript affords us a way to access or manipulate HTML elements and reactively manage data properties in a component. Understanding how to define types for refs specifically is central to leveraging the power of Typescript in Vue3.
To be specific, `ref` in Vue3 is fundamentally a method that allows a variable to be mutable and reactive. It is part of Vue3’s Composition API which provides reactivity outside components. When working with Typescript, it is necessary to add type definitions to maintain the type safety benefits. Here’s how we can go about this:
html
This piece of code demonstrates defining a typed `ref` in a Vue3 component using Typescript. In this example, the `ref` named `myRef` is typed as `Ref
As sighted by Ryan Cunningham, a web development expert, “Detailed type definitions are key for productive coding with TypeScript”.
While integrating Typescript with Vue3, having a deep understanding of typing system becomes essential. The ref feature in Vue3 with type definition certainly opens up new horizons for building more robust and scalable applications. You can find detailed explanations on this topic in the [Vue.js Official Documentation](https://v3.vuejs.org/guide/composition-api-template-refs.html#template-refs).
Remember, the approach above is just one way to define type for a `ref` in Vue3 using Typescript. Depending on your specific use cases and requirements, you may come up with different configurations along with additional helper functions to meet your app’s needs.
Exploring Template Binding and Typing in Vue3
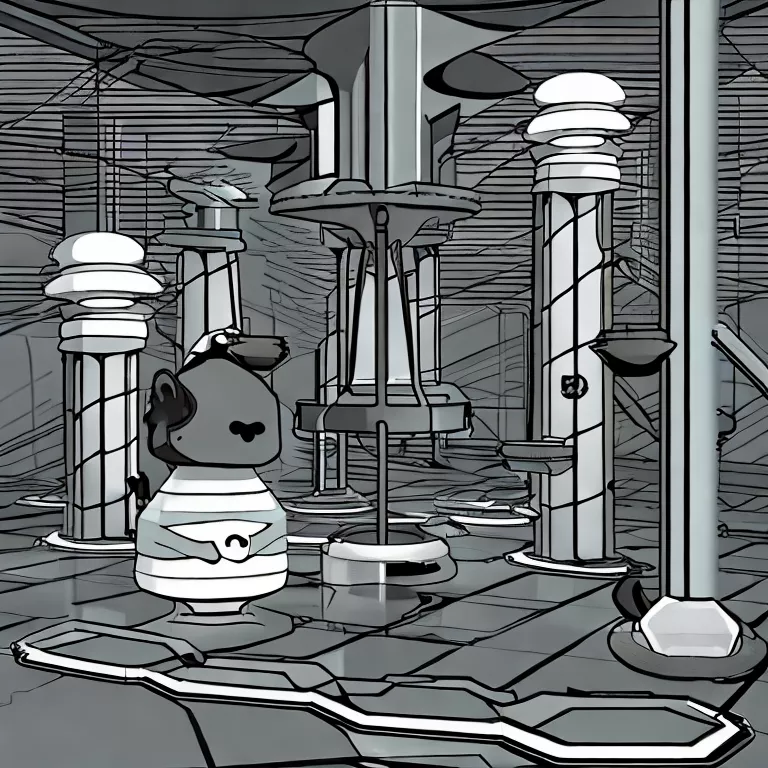
Defining Type for Vue3 Ref: A Dive into Template Binding and Typing
Utilizing TypeScript with Vue3 offers an improved development experience by adding static types to Vue components. One crucial feature of Vue3 that requires explicit understanding when working with TypeScript is the use of “Refs” (or References) in templates.
The `ref` function in Vue3 returns a reactive ref object. However, TypeScript needs to be aware of the correct type to provide type checking and code hinting accurately. For this reason, it is essential to understand how we can define the type for refs when using template binding in Vue3.
Let’s illustrate this with an example utilizing `ref` from Vue3. A typical scenario would involve declaring a reference to a string or number variable:
code
import { ref } from ‘vue’
let message: any = ref(”)
let count: any = ref(0)
In this instance, both `message` and `count` are labeled as type `any`, which could cause issues down the line with type checking, as `any` ignores certain benefits of TypeScript.
Instead, the type of these variables should be stated explicitly as follows:
code
import { ref } from ‘vue’
let message: Ref
let count: Ref
By doing so, you establish the `Ref` type imported from Vue, indicating the expected inner value of the Ref. It allows TypeScript to ascertain that `message` will always be a string, and `count` will always be a number, hence enhancing your code’s reliability and predictability.
Though sometimes, we might need to bind values that are not primitive such as Objects. Here is how to declare types for object-based refs:
code
import { ref, Ref } from ‘vue’
interface User {
name: string,
age: number,
}
let user: Ref
This makes use of TypeScript interfaces to define a type for the `user` variable. This formatting ensures that any object assigned to `user` will always follow the defined structure.
At times, you may encounter situations where a `ref` isn’t needed, and you can use reactive instead:
code
import { reactive } from ‘vue’
interface User {
name: string,
age: number,
}
let user = reactive
In conclusion, utilising TypeScript’s strong typing features coupled with Vue3’s reactive framework paves the way for creating more robust and type-safe applications.
> “Any application that can be written in JavaScript, will eventually be written in JavaScript.” – Jeff Atwood
This quote perfectly encapsulates why it becomes increasingly vital to utilise TypeScript. Its added layer of protection against runtime bugs is invaluable, especially as our JavaScript applications continue to grow in complexity.
Inlining refs to Vue Templates are beyond the scope of this discussion, but further readings can be found in the Vue3 Official Documentation.
Practical Guide: Implementing Ref Type Definitions in Vue3 Using Typescript
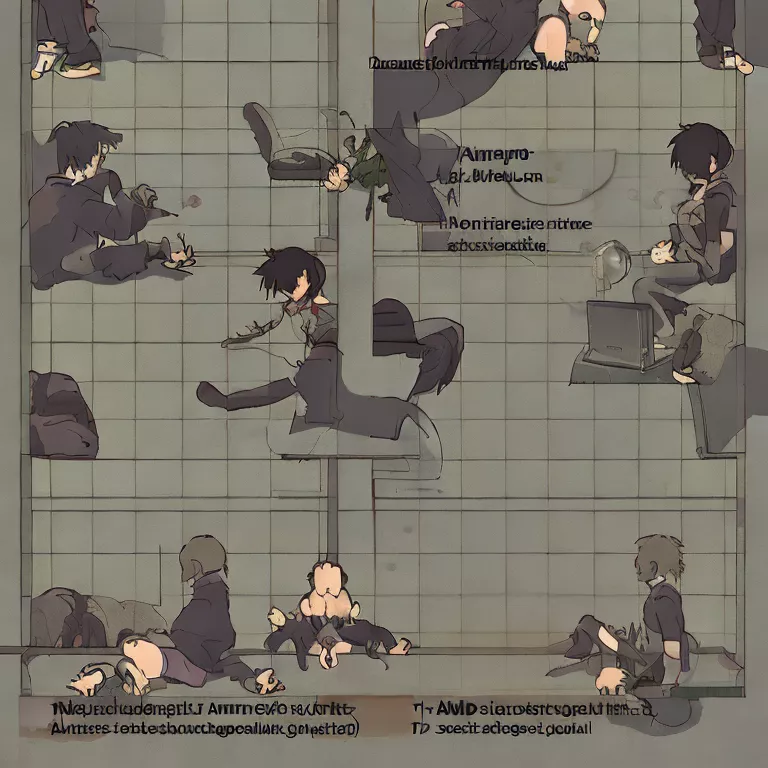
With the release of Vue 3 and its composition API, ref management has become an integral part of working with Vue and TypeScript. A ref is a way to directly access a DOM element or a Vue component instance in Vue.js.
Within Vue 3 utilized with TypeScript, a `ref` typically has not only one, but multiple types. To define types for `ref`, we use TypeScript’s built-in type definition system. By setting a correct type to a reference (`ref`), it can help us to know what operations are allowed on that referenced value and prevent errors during development.
Before we delve into how we can type-define a `ref`, let’s understand a bit about `ref`. In Vue 3, ref are reactive references holding a value. Ref are commonly used within composition API and setup function. They can hold any type of data including Strings, Numbers, Booleans, Objects, Arrays, Functions, Promises, among many others.
The `ref` function generates a reactive and mutable object. When you change the `.value` property, the component re-renders. Often `ref` is utilized in the `setup()` function in Vue3:
let name = ref(”);
In this case, the inferred type of name will be `Ref
If you want to specify the type of the `ref`, you can do it by `ref
html
This form confirms that the `type` of the `count` variable is `Ref
Now, as per binding `ref` to template in Vue3 with TypeScript for DOM manipulations. It’s important to note that you can’t just assign any `HTMLElement` type because a ref can also potentially be null. Many events (like mounted, updated) access the reference and it may not have been rendered yet. You need to ensure your code doesn’t break if the `ref` is null.
An example of assigning ref type for DOM element:
html
In this example, `myDiv` is set as type `Ref
Using type definitions and `ref` not only improves readability but also enables powerful TypeScript features such as auto-completion and hover-information in modern editors like VSCode.
As Tom Krcha once said, “Coding has become an essential part of our world. By understanding coding, you open up amazing new possibilities and control over technology.”
Remember the correct type usage with typescript will enhance your coding experience by ensuring the components wielded are used correctly, promptingly highlighting errors and offering cleaner code design.
Step by Step Process of Assigning Types for Template Bindings in Vue3
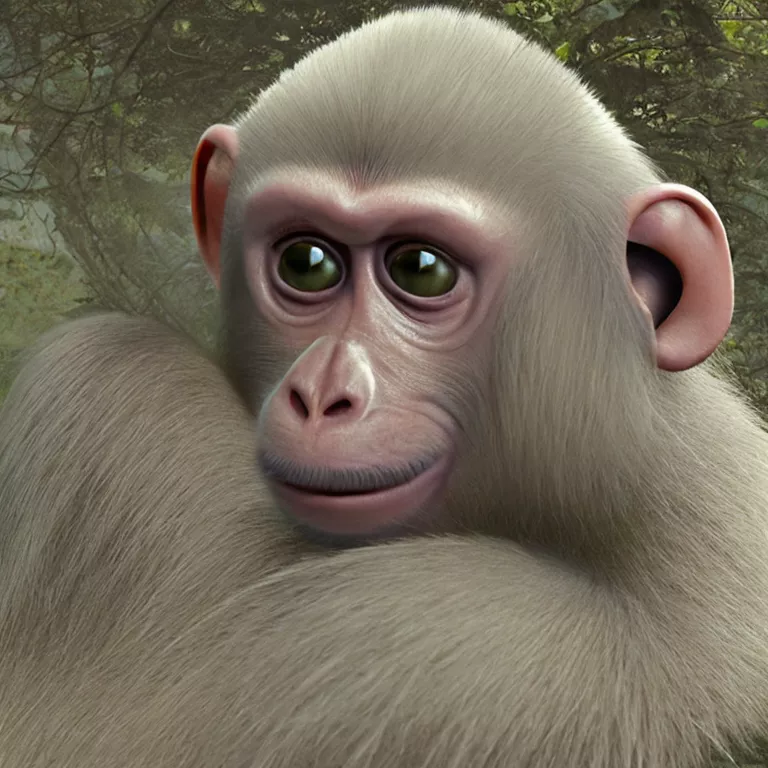
Assigning types for template bindings in Vue3 using TypeScript, specifically when dealing with ref (binding on templates), necessitates a robust understanding of Vue.JS’s Reactivity API, as well as TypeScript’s rich type system. When it comes to **Vue3**, you’re dealing with a more powerful JavaScript framework that empowers developers with the creation of highly-scalable and performance-driven applications. On the other hand, **TypeScript** presents us with a statically compiled language built on top of JavaScript, further enhancing the process of software development by offering type validation amongst other features.
- Initialization:
The first point of contact is always to initialize our Vue3 application. Make use of the official Vue.js guide if you need assistance setting up your application.
import { createApp } from ‘vue’
const app = createApp(App)
app.mount(‘#app’)
Notice how we imported a type called `createApp` from the ‘vue’ package.
- Define Ref:
The second step is about the `Ref`. `Ref` is one of the core APIs of Vue.js 3.0 which allows creating a reactive reference to a value. Vue3 provides a function named `ref` in order to create our references.
import { ref } from ‘vue’
const count: Ref
In the above code snippet, we have created a ref named `count`, assigned it with the number type, and set its initial value to 0.
- Creating Types:
With TypeScript, developers can enrich their codebase with custom-defined types. We will be demonstrating this by defining a type for our application data.
interface DataProps {
value: number;
}
The `DataProps` interface can then be further utilized within the TypeScript component.
- Assigning Types to Templates:
To assign a type for a template binding in Vue3, we will involve specifying it using the function signature.In Vue3 with TypeScript, assigning types to composed functions involves specifying the types directly in your function signature:
const useCount = (data: DataProps) => {
const increment = () => {
data.value++
}
return {
count: computed(() => data.value),
increment
}
}
Note that in our method, `useCount`, we are accepting an argument of type `DataProps`. We’ve also typed the return object. This way, when we bind this on a template, TypeScript is able to infer the correct type.
These steps encapsulate the process of assigning types to template bindings in Vue3 with TypeScript. Simplifying and enhancing the development process is a key driver in modern technologies such as **Vue3** and **TypeScript**. “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler. This quote beautifully sums up the importance of writing clean, readable and well-typed code.
Conclusion
Defining Ref Types within Vue 3 and TypeScript
The use of TypeScript in defining reference types (Ref) for template binding in Vue 3 helps to significantly boost the structure and integrity of your code. This further enhances code understanding, debugging facilitation, and highlights potential pitfalls during development.
Given the context, consider this simple illustrative snippet using ref:
typescript
import { defineComponent, ref } from ‘vue’
export default defineComponent({
setup() {
const myRef = ref<HTMLElement | null>(null)
return { myRef }
}
})
The above block demonstrates how a type can be defined for a
xxxxxxxxxx
ref
in Vue 3 using TypeScript:
xxxxxxxxxx
myRef
is of type
xxxxxxxxxx
HTMLElement | null
. This means it can hold an instance of HTMLElement or be null.
Notably crucial here is the recognition that Vue 3’s Composition API has brought forward significant improvements allowing developers to leverage TypeScript capabilities better. It makes the identification easy and quick for compiler errors by appending strict typings to reactive references.
Advantages |
---|
Enhanced Code Debugging |
Increased Code Readability |
Type Safety Guarantee |
Better Error Identification |
While TypeScript generally introduces strict typing into JavaScript applications, its purpose becomes more pronounced in library ecosystems like Vue.js where you would typically work with object-oriented programming constructs.
When you define types for refs in your Vue 3 applications using TypeScript, their values become static-typed, giving you type-checking benefits during the development time. Programmers can write more robust code because they can use TypeScript to detect errors during coding rather than running the application.
In words of Uncle Bob, _”Clean code is not written by following a set of rules. You don’t become a software craftsman by learning a list of heuristics. Professionalism and craftsmanship come from values that drive disciplines.”_ This quote stresses the significance of professional practices in overcoming the dynamic challenges of modern web development, including defining types for refs with Vue 3 & TypeScript.
More about Refs and reactive programming in Vue 3 can be explored at the official Vue.js documentation.