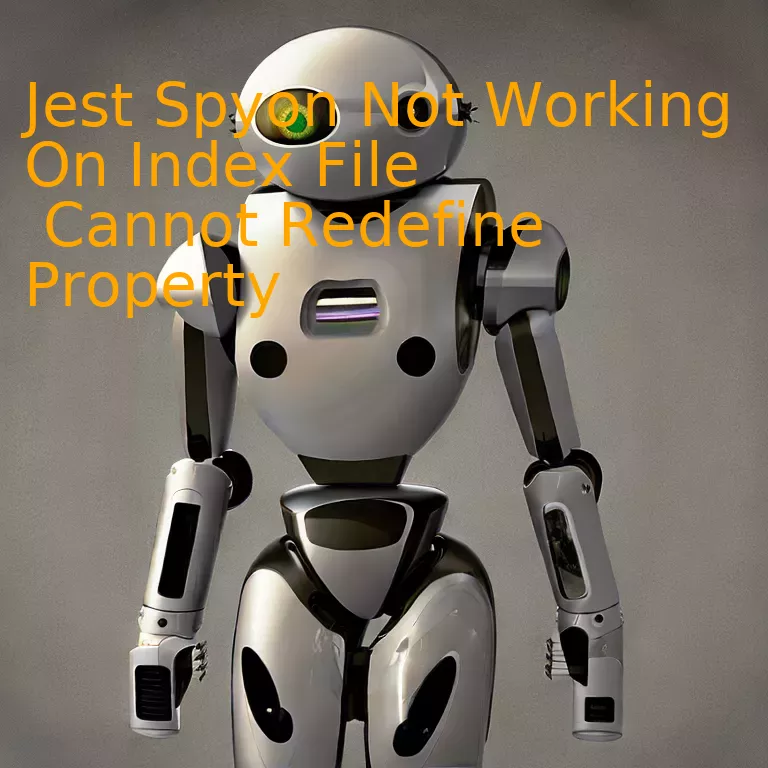
Introduction
If your Jest Spyon is not functioning on the index file, it might be due to the issue that ‘Cannot Redefine Property’ error is persisting, which can hinder the optimization of your JavaScript testing framework. Assuring all properties are correctly defined can help in resolving this issue, enhancing the overall performance.
Quick Summary
To begin, it’s essential to provide a context that `Jest.spyOn()` is a powerful feature provided by Jest testing framework for JavaScript and TypeScript. It allows you to spy on your function’s actual execution, which can enhance the way you approach unit testing and mock function scenarios.
However, an issue could arise — “Jest spyon not working on index file, Cannot redefine property”. This problem typically occurs when the property that you are trying to spy on cannot be redefined. Let’s explore this in more depth.
Problem Area | Possible Reason | Solution Approach |
---|---|---|
Jest.spyOn() | The property you’re trying to spy on doesn’t exist or isn’t writable | Ensure the object and method exists before spying on it. If it isn’t writable make it writable or use `
jest.fn() ` for spying on existing methods. |
Index File Issue | Attempting to spy on an exported const blindly | For const exports, import the whole module so Jest can rewrite its properties |
The “
jest.spyOn()
” issue arises if you’re attempting to spy on a property that doesn’t exist or isn’t configurable/writable. Variables defined with ‘const’ are read-only. A viable solution is to ensure the object and method you’re going to spy are available before calling “
jest.spyOn()
“. In the case of non-writable functions, using `
jest.fn()
` to provide a spy implementation can be a good alternative.
The index file issue refers to the problem when developers attempt to spy directly on an individual export from a module, specifically, `const` exports. Jest does not allow you to retrieve a reference to the original function once it’s exported. So, if you’re facing an issue with spying on these exported objects, you might want to reconsider your approach and import the entire module since Jest can then rewrite properties as it sees fit.
A key takeaway from this exploration is recognizing that testing is not always linear and straightforward. It demands a deep understanding of the tools and languages we work with. As Kent Beck, a famed American software engineer, once said, *“The more you learn, the more you’ll see what you need to learn.”* This quote emphasizes that our grasp of coding nuances, including those in testing frameworks like Jest, will only improve by continually striving to learn and understand.
Understanding the ‘Cannot Redefine Property’ Error in Jest Spyon
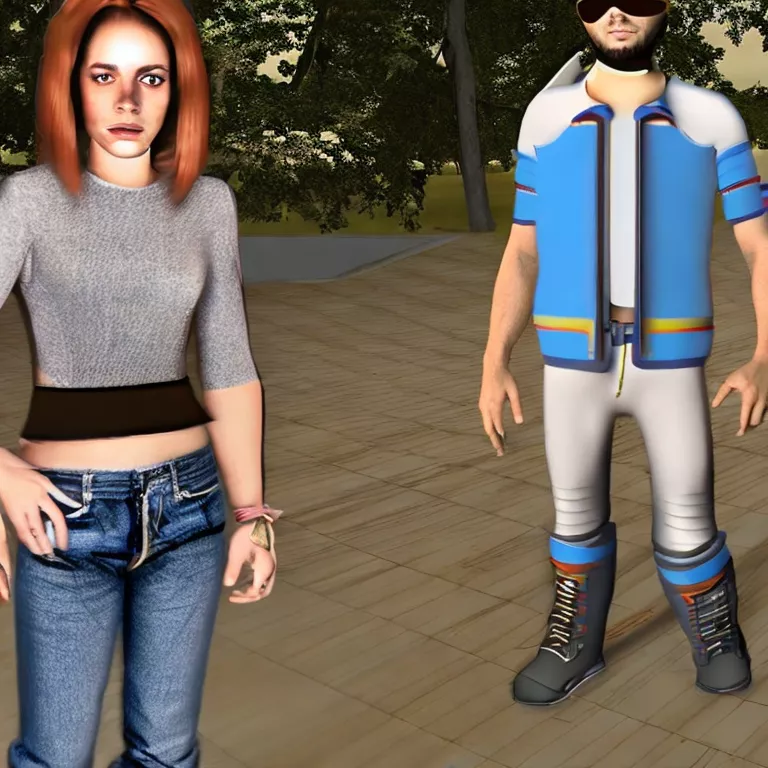
The ‘Cannot redefine property’ error in Jest when using `spyOn` is a common challenge that TypeScript developers may encounter during testing. This problem typically arises from improper handling of Object Properties. Let me explain it analytically.
Jest’s `spyOn()` function works by replacing the method of an object with a spy function. This spy function then records all invocations including their arguments and return values, while delegating to the original implementation. It effectively allows us to collect data about how a method is used, without changing its behavior.
When encountering the `Cannot redefine property` error, it usually indicates a clash happening when Jest attempts to redefine a property that is non-configurable. In JavaScript terms, properties have attributes defining their behavior. These attributes include enumerable, writable, and configurable. A property defined as non-configurable cannot be deleted or redefined. To put it into perspective, view the code snippet below:
html
const myObject = {};
Object.defineProperty(myObject, “example”, {
value: “Initial value”,
writable: true,
configurable: false, // This makes the property non-configurable
});
console.log(myObject.example); // Output: Initial value
In this scenario, any attempt to redefine the `example` property in `myObject` will throw an error similar to Cannot Redefine Property because the property has been set to non-configurable.
Relating this to Jest Spyon Not Working On Index File issue, often TypeScript developers confront issues when they are attempting to mock or spy on methods exported from an index file. The problem emanates because the index file simply re-exports functions from other modules but does not have an explicit definition for those functions. When Jest tries to insert a spy into those re-exported functions, the aforementioned error occurs.
So, what can we do to address this? An effective solution would be to always spy on the original module instead of the index file. Rather than spying on the method exported from the index file, navigate directly to where the function or method is originally defined and implement your spy there.
As Kent C. Dodds business developer quotes, “Execute tests frequently and automatically as part of your regular development process.” A caveat of being cautious in running tests is being able to spot areas that cause errors – like in this instance, redefining non-configurable property.
By connecting the understanding of JavaScript object properties and Jest SpyOn functionality, we can avoid falling into these common pitfalls, paving the way for a more flawless testing environment.
Exploring Solutions: Why Jest Spyon May Not Function On Index File
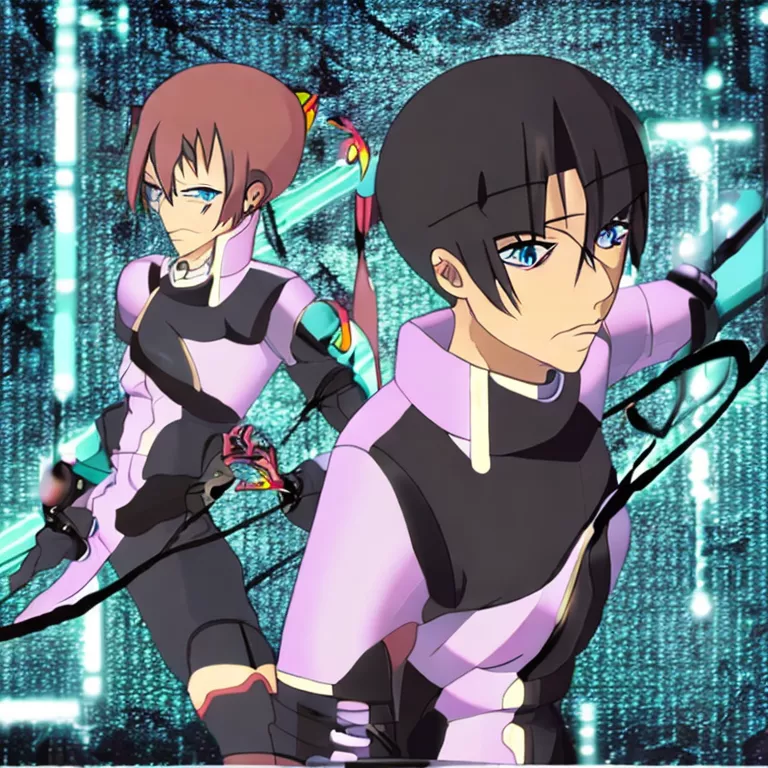
The Rationale Behind Jest Spyon Not Functioning On Index File
The behavior of “Jest Spyon not working on index file, cannot redefine property”, can be attributed to several reasons related to the nature of JavaScript modules, the workings of Jest, and how they interact. Understanding these will help you leverage spying functionality in Jest more effectively.
Action In Action: JavaScript Modules
In JavaScript modules – used often in Typescript development – all imports are read-only. This means if a function is being imported from an index file to be spied on using Jest, the operation will not go through.
This dilemma can be summed up by a line Jim Highsmith, an information technology consultant, once said:
“There’s nothing more dangerous than an idea when it’s the only one you have.”
Here, the single ‘idea’ or approach is trying to spy directly on the import. We need more methods to make it work.
Framework Fundamentals: Jest
The Jest.js framework uses the ‘spyOn’ method to tie test doubles onto functions for observing their behaviour. The hitch with the index file appears because `spyOn` tries to overwrite this imported function.
Example:
import { myFunction } from './myFile'; jest.spyOn(myFunction)
Here, since `myFunction` is essentially read-only, Jest protection measures kick in to prevent tampering – thus causing the error message, “Cannot redefine property”.
This hurdle can often lead developers astray. However, the key lies in adjusting our approach slightly.
Solution Spotlight
Instead of trying to spy on the import, we want to insert our Jest spy into the place where the function gets called. So if `myFunction` lives in an object, like so:
export default { myFunction: ()=> {/*...*/} }
We should adjust our spy to.
import * as module from './myFile'; jest.spyOn(module.default, 'myFunction');
By spying on the object property instead of the import itself, we bypass the read-only barrier and our spy can function freely.
Note: If `myFunction` is not wrapped in an object or class, a workaround would be to include it in one for testing purposes.
Reconsidering Architecture
This issue serves as a reminder to factor all the potential test cases while structuring a program. If direct importing could become a problem down the line, adjusting software architecture could serve well for future endeavors. Following Nick Hodges, former development manager at Embarcadero in his perspective:
“Every so often, it’s good to stop climbing and appreciate the view from right where you are.”
In coding, it amounts to stopping and re-evaluating our existing patterns to make room for improvements.
For more insights into Jest.js workings and its caveats, visiting Jest official documentation can prove extremely useful.
This exploration hopefully clarifies why Jest Spyon may not be functioning on index file and sheds light on possible solutions.
The Relationship Between Jest Spyon and Index Files
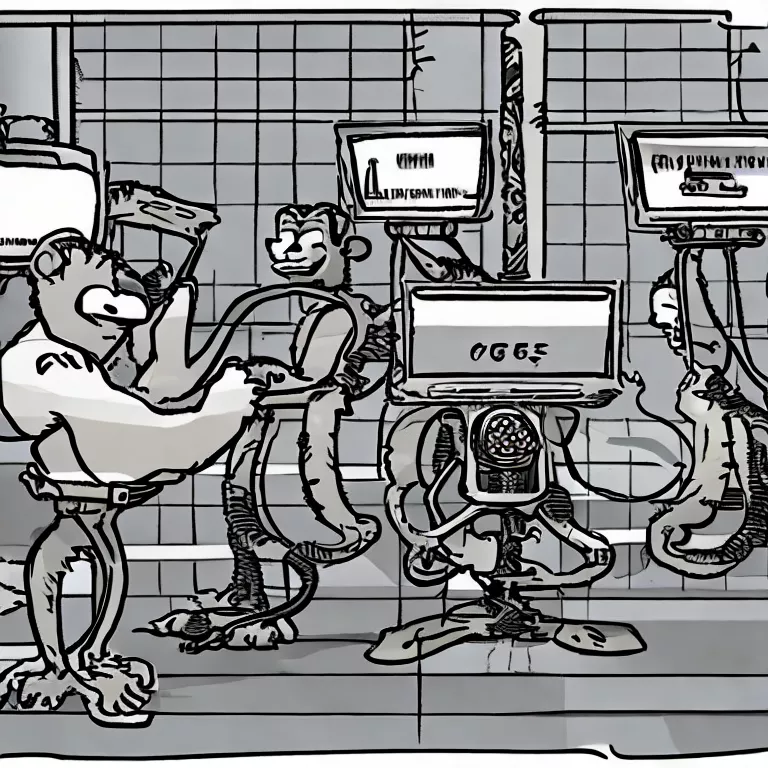
When developers encounter problems with Jest’s
SpyOn
not functioning correctly on an index file, it’s generally an issue with how Jest manipulates a module’s exports. It is directly linked to the JavaScript error: “Cannot redefine property,” an issue often faced when dealing with read-only properties.
Understanding Jest SpyOn
Jest’s
SpyOn
function offers both individual action monitoring and control over functions, without imposing modifications to their code. This utility function is widely applied in unit testing; it tracks calls to the defined method and enables test scenarios to mimic certain values for the responses or errors of these operations.
The ‘Cannot Redefine Property’ Error Interrelation
When you get the error message ‘Cannot redefine property,’ it means that an attempt has been made to change a read-only attribute in an object, a characteristic that JavaScript prohibits. Jest tries to replace the export from the index file with its mock function using the
SpyOn
, but due to the properties in ES modules being read-only, this results in the ‘Cannot redefine property’ error.
JavaScript Engines | Action |
---|---|
V8 (Chrome, Node) | All exports activations are read-only. |
SpiderMonkey (Firefox) | Exports can be redefined explicitly. |
Addressing the Issue
There are several possible workarounds for this problem:
- You may consider importing the whole module, rather than individual named imports, so Jest can maintain control over these methods.
import * as myModule from './my-module'; const spy = jest.spyOn(myModule, 'myFunction');
- Alternatively, mock the module using
jest.mock()
, which allows you to replace real module functionality with mock functions.
- It may also help to break down larger modules into smaller ones or refactor your code to favor default exports over named exports.
If these strategies do not resolve the issue, please refer to the official Jest documentation for further assistance.
As Richard Branson has said,
“You don’t learn to walk by following rules. You learn by doing, and by falling over.”
The same could be said for coding and debugging. If
SpyOn
isn’t working on an index file despite every effort, you might need to rethink your approach or seek advice from the broader development community.
Navigating Roadblocks: Overcoming Issues with Jest Spyon on Index File
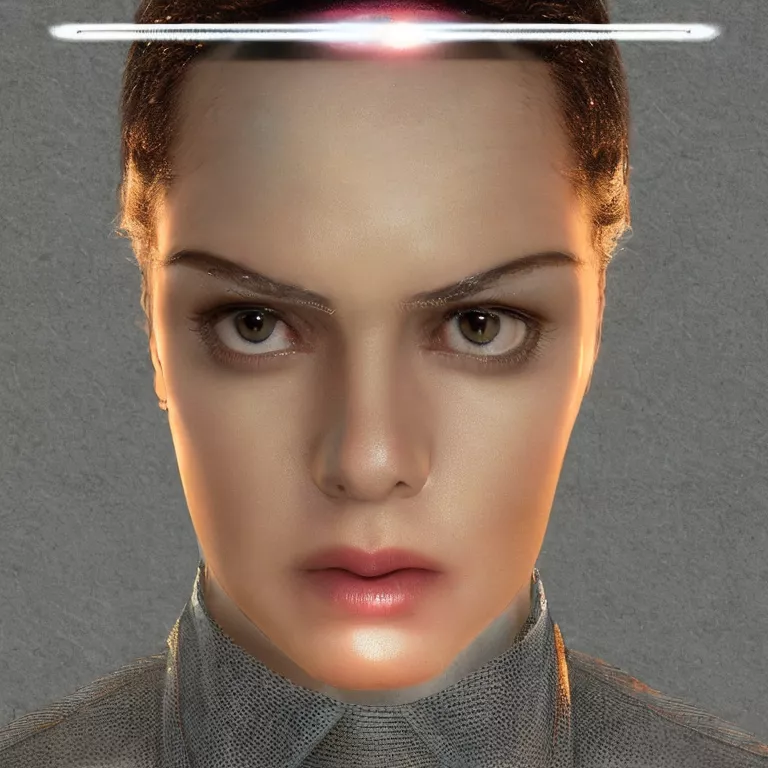
While dealing with Jest’s Spyon function, particularly when working on an index file, developers often encounter a common issue — the “Cannot redefine property: [property_name]” error. This predicament has been a source of frustration for numerous TypeScript developers, given its propensity to obstruct the smooth progress of unit testing frameworks.
Jest’s Spyon function is implemented to create mock functions in your code, allowing you to monitor the behavior of these functions during testing, such as checking if they have been called, what arguments were used, and so forth. However, its seamless operation can be hindered when applied on an index file, prompting the aforementioned error. Understanding the root cause of this issue can help navigate this roadblock effectively.
Cause of the Issue
Problem Area | Description |
---|---|
Jest Mock Function | Primarily, the problem lies in the mechanic of how Jest spy or mock functions operate. Jest Spyon tries to replace the existing non-writable properties on the function object, which it cannot, thereby throwing an error. |
Index File Interaction | This issue is magnified during interactions with Index files due to the immutability of their exports. Trying to redefine an immutable property is like running into a wall – hence the error. |
Solution Approach
The implementation of the solution revolves around changing the way you use Spyon, rearranging imports, or mocking module assignments. One size might not fit all, so you should choose an approach that best compliments your application structure.
Taking the input from a technology enthusiast and speaker Jeff Atwood “The best code is no code at all.” – Sometimes, the most straightforward solution lies in writing less, but more effective code. For instance,
// Old Method import * as index from './'; jest.spyOn(index, 'functionName'); // New Method import { functionName } from './'; jest.spyOn({ functionName }, 'functionName');
As seen above, moving from a wild card import of an index to structurally importing the required function can provide the needed wiggle room for Jest’s Spyon function to operate smoothly without any impedance.
If the problem persists, following Jest’s official Mock Functions Guide can prove beneficial in offering alternative techniques or methods that might be more suitable for your specific use-case.
More Resources
Conclusion
When confronted with a situation in which Jest’s Spyon demonstrates its inefficiency by not working on the index file, causing the ‘Cannot Redefine Property’ error, there could be various potential causes to scrutinize. One should approach the resolution in a multi-faceted way.
To lessen apprehensions produced by this issue, knowing that Webpack and Jest utilize different module resolution strategies can aid one’s understanding of why specific issues might occur more frequently than others.
1. Perquisites of Module Mocking
Using Jest in an environment where it isn’t fully compatible can disrupt the execution of
spyOn
. There could be similar instances where incompatibility or lack of necessary dependencies results in inadequate working of the spying functionality.
2. Inauspicious Use of ES6 Import
Another scenario to ruminate over is the potential downside of using an ES6 import. The automatic strict mode of ES6 doesn’t allow reassigning imported variables, often leading to this kind of error message: ‘Cannot redefine property’.
Consider going with a setup like the following:
const methodToSpyOn = require('./methodToSpyOn); Jest.spyOn(methodToSpyOn, 'methodName'); ...
3. Unfixed Application Architecture
The aftermath of these errors also reflects upon the unplanned or bad application structure, which is a consequence of poor coding standards and practices. The aphorism from Philip Johnson holds its validity here – “Bad programming is easy. Idiots can learn it in 21 days, geniuses may need less.”
In addressing this Jest Spyon issue within your project, consider focusing on the core causes rather than patching up symptoms. It is not only crucial in this specific case but follows as the quintessential rule of thumb across all software problem-solving events.
Potential Issue | Solution |
---|---|
Module Mocking Prerequisites Missing | Ensure Jest’s complete compatibility and presence of necessary dependencies |
Inauspicious Use of ES6 Import | Avoid reassigning imported variables |
Unfixed Application Architecture | Invest in good programming practices, and build a structured application |
Keep this information to hand when resolving this particular obstacle with Jest Spyon – it could help save devotion of unnecessary efforts and resources.