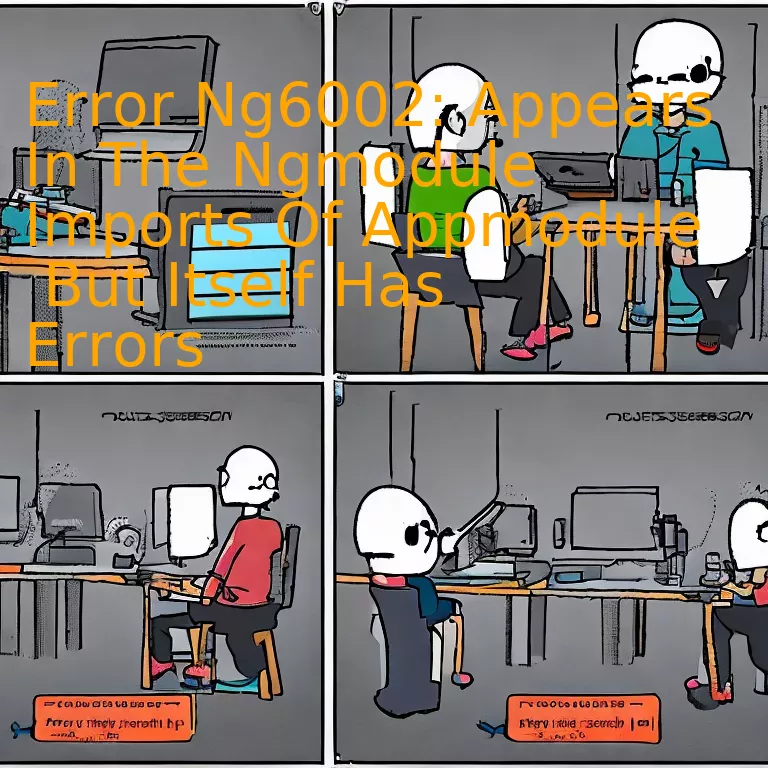
Introduction
When configuring the AppModule, an issue of Error Ng6002 can occur which denotes that a component or module being imported into the NgModule has internal errors. This error might be hindering the seamless functioning of your application and needs rectification through bug fixing or proper configuration.
Quick Summary
Given your query regarding the error “NG6002: Appears in the NgModule imports of AppModule, But itself has errors,” let’s delve into clarifying this complex issue. Typified as a common TypeScript, or more specifically, an Angular error, such glitch often arises during the process of importing modules that themselves contain certain unresolved errors.
It might prove insightful to first glance at a simplified representation of how this error is triggered:
Module | Error Trigger |
---|---|
NgModule | Attempt to import problematic AppModule |
AppModule | Presence of unresolved issues/errors within the module |
The `NgModule` indicates a core Angular module that functions by bundling together related code. This assists in efficient organization and separation of concerns in an Angular app. The `AppModule`, on the other hand, usually establishes the fundamental building block for several angular applications. Being the root module, your app launches by bootstrapping this particular module, which makes it essentially crucial.
Considering this, when `NgModule` tries to import `AppModule` while the latter contains unresolved errors, the NG6002 error is generated.
Investigating such scenarios requires systematic problem-solving, primarily focusing on determining and resolving the existing bugs within the `AppModule`. Java-based errors could range from incorrect syntax, null values, array index violations, to class casting issues. Once identified and resolved, your Angular application should operate more seamlessly, steering clear from the NG6002 error message.
As software pioneer Grace Hopper once pointed out, “To me programming is more than an important practical art. It is also a gigantic undertaking in the foundations of knowledge.” Similarly, understanding Angular and TypeScript isn’t just about mastering a programming language or a framework; it’s about fundamentally comprehending the layered intricacies of the digital, building a strong foundation for problem-solving across any obstacles that might pose in front.
Understanding the NG6002 Error in Angular
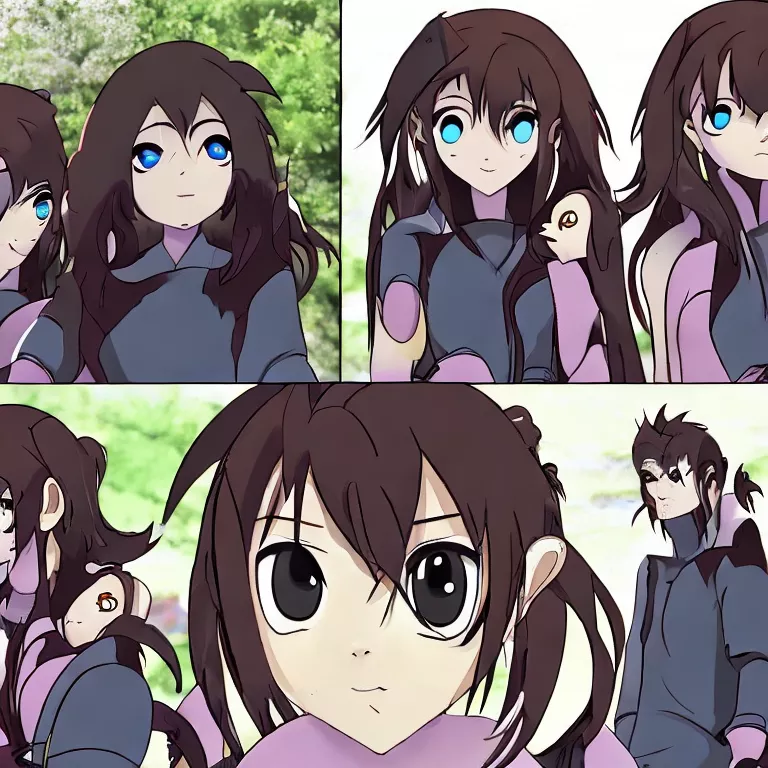
The NG6002 error in Angular points to an issue with the NgModule, specifically a problem with how some components or modules are imported. The error message “NG6002: Appears in the NgModule imports of AppModule, but itself has errors” suggests that the module which you tried to import into your AppModule is suffering from some problems.
What Is An NgModule?
An NgModule can be viewed as a fundamental block for building applications using Angular. It serves to assemble components, directives, and pipes into cohesive units of functionality. Each Angular application is required to have at least one root module (AppModule) allowing for bootstrapping, and often has more feature modules.
In essence, NgModules provide a compilation context for their components. A component along with its associated template forms a compilation unit. Before a component is instantiated, Angular brings together the corresponding template within the context of Angular Module, which in turn encapsulates a range of dependencies.
Causes Of The NG6002 Error
There are many possible causes for the NG6002 error. It could be due to:
- Misconfigured or missing imports in your application module.
- Mistakes in the NgModule’s declaration.
- Overlooking importation of an NgModule from another.
Solution For The NG6002 Error
To troubleshoot this error, meticulous revision of each import will be necessary. Be sure to go through the following checks:
- Verify that each import in the NgModule matches with the actual location and name of the imported element.
- Ensure every component/directive/pipe declared inside the NgModule is imported correctly.
- Confirm if you might’ve forgotten to import a necessary NgModule into another.
import { BrowserModule } from '@angular/platform-browser'; ... @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, ... ], ... })
Note that in the Angular structure, each specified import should point to an NgModule. The example here, from the official Angular guide illustrates this principle.
Quotation
To quote Grace Hopper, a brilliant computer scientist and Navy rear admiral, “The most damaging phrase in the language is, ‘It’s always been done this way'”. In the context of dealing with coding errors like NG6002, the reflex should be to think outside the box, revisiting and questioning all previous assumptions, rather than rigidly holding on to pre-established conceptions.
Common Causes of Error NG6002 in AppModule Imports
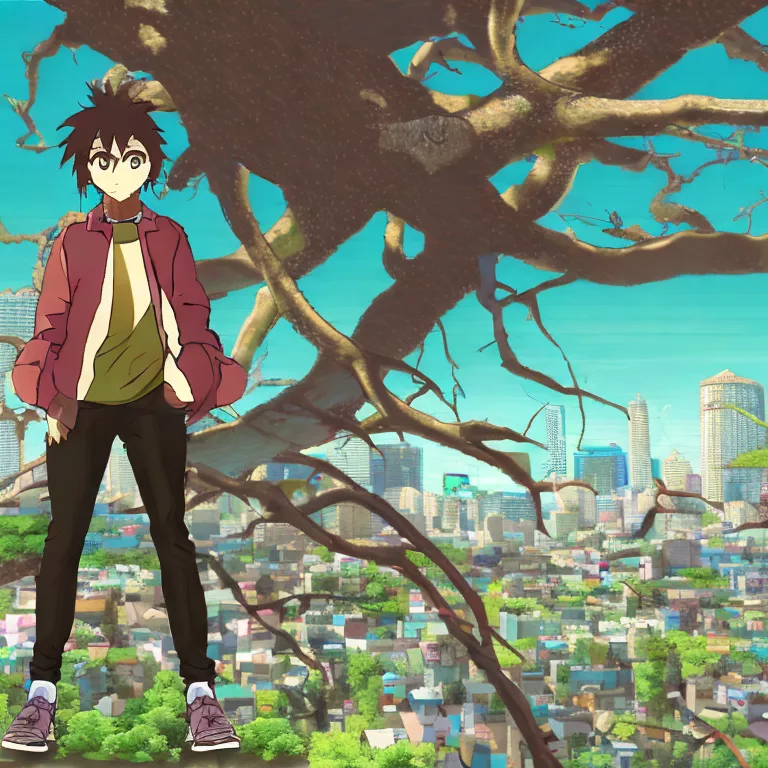
The NG6002 error is a common occurrence with Angular projects and essentially indicates that the module you’re trying to import into AppModule has thrown an error. This error message typically pops up when TypeScript files are not compiled correctly or there are discrepancies in the project’s dependencies.
Here are some of the major reasons why the NG6002 error may appear:
- Incorrect Compilation of TypeScript Code: The Angular compiler performs ahead-of-time (AOT) compilation, converting your TypeScript code into JavaScript before it runs in the browser. If there are compilation errors in your TypeScript code, such as typos, incorrect syntax, or ignoring TypeScript rules, the Angular compiler could fail to complete the process, leading to NG6002 error.
- Inconsistencies in Dependencies: Angular projects often depend on numerous external libraries. If a required library is missing from the node_modules folder or the package.json file hasn’t been updated correctly after installing a new package, Angular could report an NG6002 error. Similarly, conflicts between different versions of libraries can create similar issues.
To resolve these issues, you might want to explore the following strategies:
- Correct any TypeScript Compiler Errors: Check the compiler log for specific hints about what went wrong during the compilation process. These details often point directly to the problematic piece of code.
- Validate Your Project’s Dependencies: Ensure that all the necessary libraries are properly installed. Reviewing the package.json file and reinstalling the node modules may help resolve the issue.
- Update Dependencies: Sometimes, an update to the latest versions of Angular or other dependencies fixes the problem. But remember, performing updates without understanding the implications can introduce new issues, so careful consideration and testing are needed.
“Understanding TypeScript compiler errors is crucial to maintain clean and maintainable code.”
– Caleb Ebiware, Software Engineer
// Run this command in your terminal npm install
// Run these commands in your terminal npm update ng update
A consistent approach to debugging, like the one detailed above, often leads to identifying the source of the NG6002 error and resolving it effectively. Remember that every software project is unique – the solutions listed here may not cover every situation, but they provide a good starting point.
Troubleshooting Techniques for Resolving the NG6002 Error
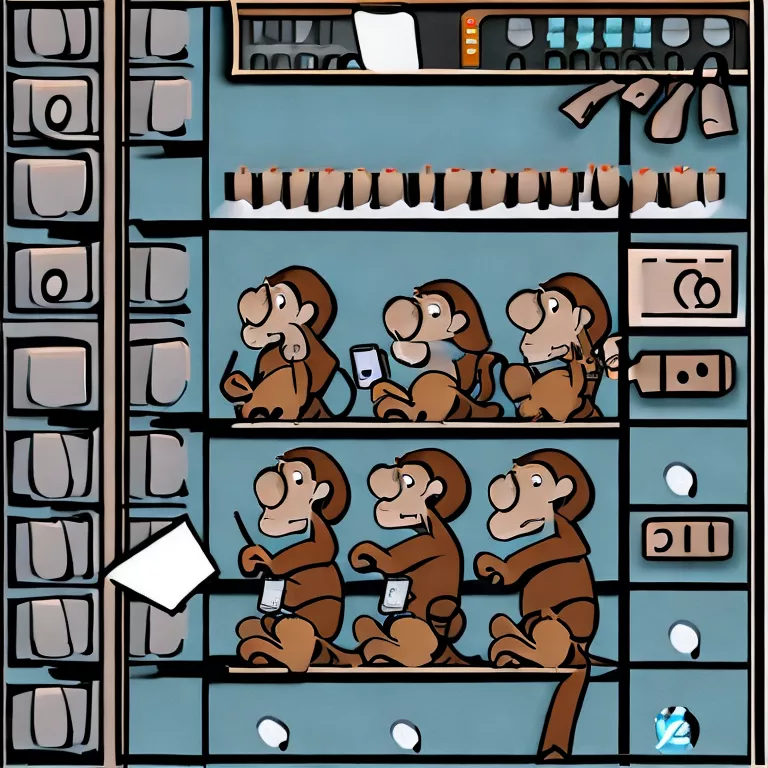
The ‘NG6002: Appears in the NgModule imports of AppModule, but itself has errors’ is an error you may encounter when executing an Angular application. This error commonly occurs due to a misconfiguration or improper import/export management within your code and can be quite a hurdle to overcome. However, fear not, there are several trouble-shooting procedures to resolve this issue proficiently.
Common Troubleshooting Techniques
- Finding and Fixing Import/Export Misconfigurations: The ‘NG6002’ error often signals that an import or export statement may not be correctly configured. It’s essential to examine your NgModule imports diligently in AppModule or other modules where error arising components or modules are being imported. Ensure that imported entities are correctly exported from their original modules and that no cyclic dependencies are leading to these anomalies.
- Resolving Inconsistencies with Lazy Modules: If you’re using lazy-loaded modules, ensure that they’re not simultaneously loaded eagerly. Simultaneous eager and lazy loading typically result in ‘NG6002’errors. If a module is indeed required to be available for both lazy and eager loading, consider creating shared modules and correctly distributing your components, directives, and pipes amongst these shared modules.
- Clarifying Angular CLI Versions: Interestingly, amidst dealing with ‘NG6002’ errors, StackOverflow discussions indicate that altering the Angular CLI version can serve as an effective resolution tactic. It may seem unexpected, nevertheless, ensuring that your local Angular CLI version matches your global Angular CLI version can help resolve conflicts instigating this error.
- Rebuilding The Project:Often referred to as a “nuclear” option, completely rebuilding your project can occasionally serve as an effective, albeit a tedious resolution method. Delete your ‘node_modules’ directory, clear your package-lock.json file, and reinstall your packages via the npm install command.
An Elementary Code Example
The misconfiguration of eager and lazy-loading modules is a common cause of the ‘NG6002’ error. To circumvent this problem, you should adhere to Angular’s best practices for eager and lazy loading. Here is an example of how to properly configure these modules (extracted from the Angular Official Documentation):
<!-- app-routing.module.ts --> const routes: Routes = [{ path: 'customers', loadChildren: () => import('./customers/customers.module').then(m => m.CustomersModule) }];
Remember the words of Edsger Dijkstra: “If debugging is the process of removing bugs, then programming must be the process of putting them in”. Understanding the nature of the errors we encounter in software development such as this NG6002 error, not only equips us with the skills to solve them but also prevent them from arising in the future.
Preventive Measures to Avoid Recurrence of the NG6002 Error
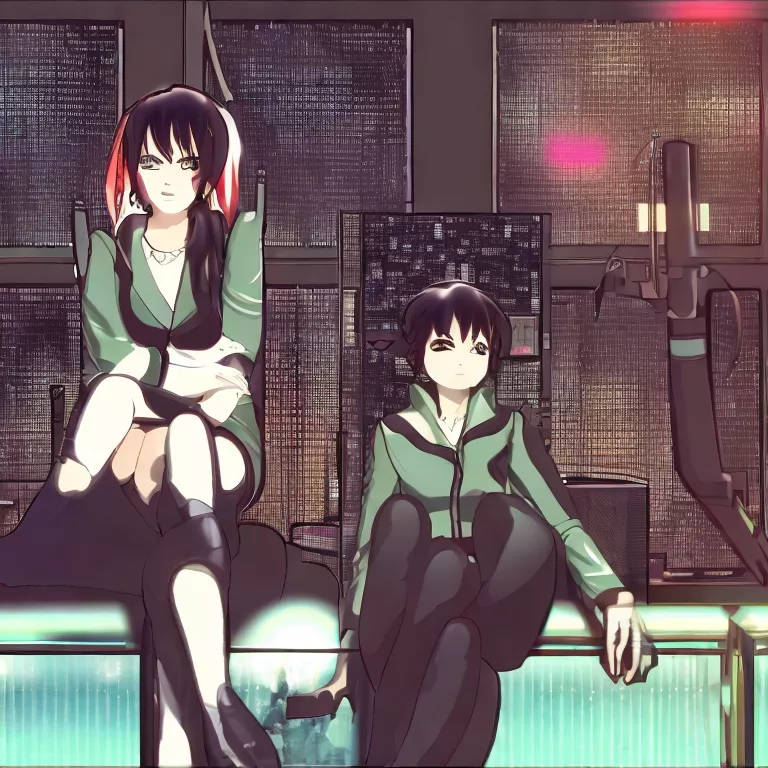
The NG6002 error often appears in the NgModule imports of AppModule, commonly due to inconsistent metadata information in the Angular library files or importing modules incorrectly. To prevent this error from reoccurring, we must adopt several measures:
Thorough Module Checking
Verify each module imported into your NgModule. It is easy for inconsistencies to creep in, for instance, importing a component instead of a module. Ensure that you are using the correct import method, as demonstrated below:
import { BrowserModule } from '@angular/platform-browser'; // Correct way to import BrowserModule
Rebuild Project Libraries
Metadata discrepancies can occur during codebase updates, which could possibly trigger an NG6002 error. Rebuild all libraries in the project to ensure their metadata matches what’s present in the current codebase and resolve any outstanding issues.
Proper Dependency Management
An inconsistent dependency tree can also result in an NG6002 error. Modules should strictly respect their dependencies, which means cyclic dependencies should be avoided at all costs. Use npm or other package management tools for effective dependency management.
Clear Cache Regularly
Sometimes, leftover build cache can interfere with the Angular CLI causing unexpected behaviour such as triggering NG6002 errors. Consider periodically clearing the cache and deleting the node_modules folder, then running
npm install
again to have a fresh set of modules.
“Anyone who stops learning is old, whether twenty or eighty.” – Henry Ford
Remember that these measures serve not just to fix, but more importantly, to prevent future NG6002 errors. Further reading on efficient Angular error handling can be found on the official Angular documentation site.
Conclusion
Error NG6002 is a common challenge encountered while using Angular, pertaining to module importation. The error often arises when AppModule, or any other Angular module, attempts to import an external module that contains issues within itself. Failing to rectify these internal errors only compounds the problem, and subsequently, the application fails to compile as it should.
The intricacy of Angular’s modular architecture is often hailed for its ability to separate concerns and enable cleaner code management. However, this unique design pattern also presents challenges like the NG6002 error whenever nonconformities exist within modules imported by your AppModule or a similar entity.
Implications:
- An understanding of Error NG6002 is crucial for maintaining the structural integrity of your Angular applications.
- Led by a deeper insight into the interplay between modules in Angular, you can map out more effective strategies for importing modules devoid of internal errors.
To manage Error NG6002 and keep your code running seamlessly:
ErrorNg6002FixModule
, being the problematic module bearing internal errors, should be cleaned up before importing into AppModule. This can come in the form of:
- Identifying and rectifying syntax errors within it.
- Ensuring all dependencies are properly established and not creating circular dependencies.
- Checking on the compatibility of the versions of Angular and the modules in use.
Adopting a meticulous approach towards module imports particularly will ensure that Error NG6002 becomes less of an impediment while building Angular applications. In essence, there is a substantial need for routine checks and fixes in advance of importing modules into our main AppModule or similar entities.
This principle was candidly put by Linus Torvalds – the creator of Linux and Git – who said, “Good programmers know what to write. Great ones know what to rewrite (and reuse).” A strategy as such not only mitigates recurring errors like NG6002 but also enhances programmer productivity in the sphere of Angular development.
If more depth is required on this issue, I recommend checking out this article on Angular’s Official Documentation which provides broader insights into Angular modules and their workings.