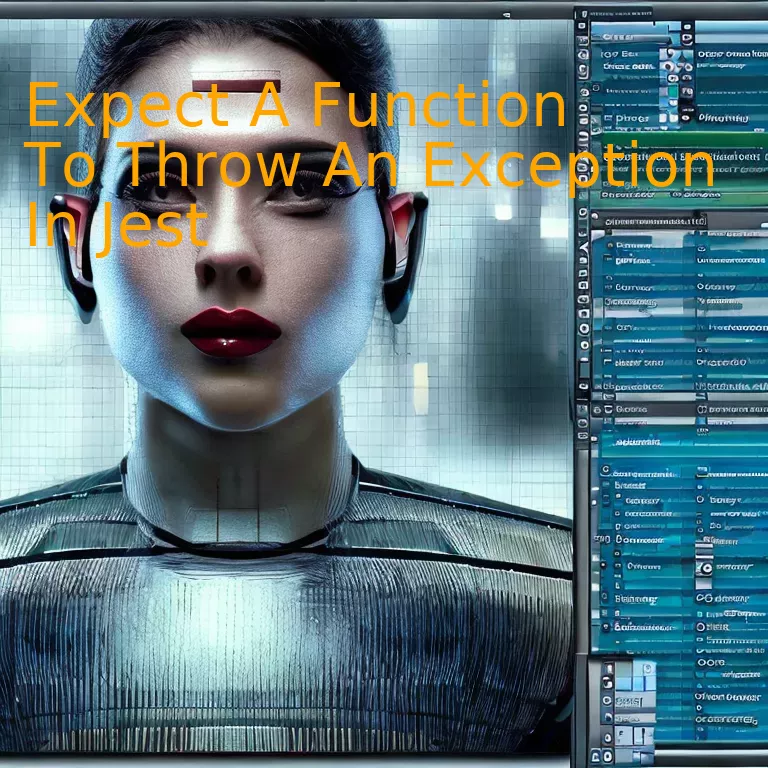
Introduction
In Jest testing, anticipate the occurrence of an exception through specific function execution using the expect keyword for efficient and error-free code operation.
Quick Summary
Steps | Use | Description |
---|---|---|
x throw new Error |
To simulate the occurrence of an error in a function. | You can instruct a Jest mock function to throw an error if it’s called by using
xxxxxxxxxx mockImplementation() . Inside this function, write the JavaScript error statement ‘ xxxxxxxxxx throw new Error('error message') ‘ with your custom error message. |
xxxxxxxxxx expect |
Instructs Jest to expect a certain outcome. | This keyword prepares Jest to verify whether the subsequent function behaves as expected. It’s like saying to Jest, ‘Hey, I predict that the next piece of code will behave like this.’ |
xxxxxxxxxx toThrow |
Evaluates if an exception was successfully thrown. | This is a Jest matcher that checks whether a function throws an error when it’s executed. This matcher validates your expectation about an erroneous runtime condition or failure scenario in your function. |
xxxxxxxxxx not.toThrow |
Evaluates if no exceptions were thrown. | This is another flavor of Jest matcher. The antithesis of
xxxxxxxxxx toThrow , xxxxxxxxxx not.toThrow verifies if the function under test doesn’t hurl an error at execution time. |
In the realm of JavaScript and TypeScript testing, Jest’s ability to handle exceptions in the application under test is a critical aspect. By incorporating the use of keywords such as
xxxxxxxxxx
throw new Error
,
xxxxxxxxxx
expect
,
xxxxxxxxxx
toThrow
, and
xxxxxxxxxx
not.toThrow
, developers can architect comprehensive and detailed test cases.
Here’s an illustration using these Keywords:
typescript
function riskyBusiness() {
throw new Error(‘Very risky!’);
}
test(‘Test that riskyBusiness throws’, () => {
expect(riskyBusiness).toThrow(‘Very risky!’);
});
This code equates to check if the function `riskyBusiness` throws an exception with the message, ‘Very risky!’. It’s a fundamental way to ensure that failures in your software are handled gracefully.
Disguising expectations for exceptions not only improves the quality of software tests but also leads to better application robustness over time. As Martin Fowler famously stated, “Good code is its own best documentation.”[1]
Understanding the Fundamentals of Exception Handling in Jest
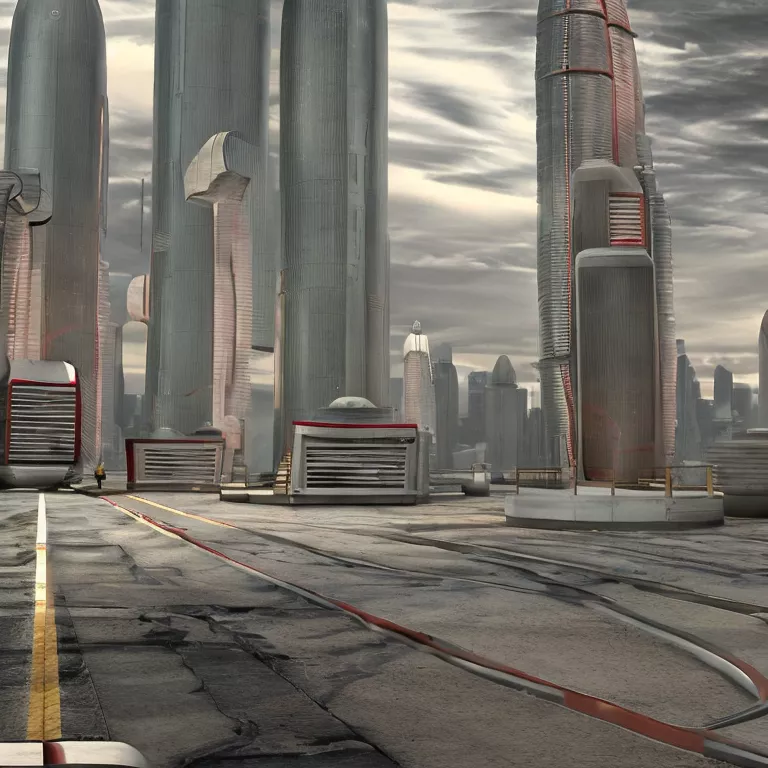
Jest, a JavaScript testing framework, offers an elegant system to deal with exceptions. This approach ensures that tests can effectively examine scenarios where an application might trigger an error condition, proving the robustness of an application’s error-handling mechanism.
Let’s start by examining the fundamental concept, which is directly related to your query regarding using Jest to check if a function throws an exception.
Exception handling in Jest primarily materializes via Expect and its method
xxxxxxxxxx
.toThrow()
. This servile utility allows developers to anticipate an exception as an outcome from a given function invocation within their tests.
With this in mind, let’s consider an example:
xxxxxxxxxx
const throwAnException = () => {
throw new Error('Exception occurred!');
};
test('function throws exception', () => {
expect(throwAnException).toThrow();
});
In the above code snippet, the
xxxxxxxxxx
throwAnException
function has been designed to throw an exception every time it’s executed. With Jest, this execution scenario is captured in our test case, where we use
xxxxxxxxxx
expect
along with the
xxxxxxxxxx
.toThrow()
matcher to verify if the function indeed throws an error when invoked.
Citing [Brian Brennan](https://brianbrennan.gg/), an eminent figure in tech industry, “Great software always begins at home.” In the context of Jest, his words echo the resonating idea that building quality into your applications starts with thorough testing. Anticipating exceptions forms part of the testing rigor, validating the sturdiness of your code’s defensive programming efforts.
Remember, Jest offers options to become even more specific with expected errors. You can assert not only if a function throws an error, but also what type of error gets thrown:
xxxxxxxxxx
test('specific exception type is thrown', () => {
expect(throwAnException).toThrow(Error);
});
Even more specifically, you can check if a function throws an error with a particular message:
xxxxxxxxxx
test('exception with specific message is thrown', () => {
expect(throwAnException).toThrow('Exception occurred!');
});
As per [Jest’s official documentation](https://jestjs.io/docs/expect#tothrowerror), the aforementioned strategies would further fortify your test cases and ensure they handle every edge case.
In essence, by harnessing these cultivated features of Jest, developers can not only anticipate exceptions but also ladle out an additional layer of sophistication to their test scripts. Testing for exceptions in Jest is hence a nuanced exercise, one that explicitly helps guarantee less catastrophes occur for end-users of the software. This enactment underlines Jest’s rigor as a testing tool and its objective in comprehensively assessing a function’s performance across different scenarios, be it triumph or exception-driven meltdown.
Utilizing jest.fn() and jest.spyOn() for Expected Exceptions
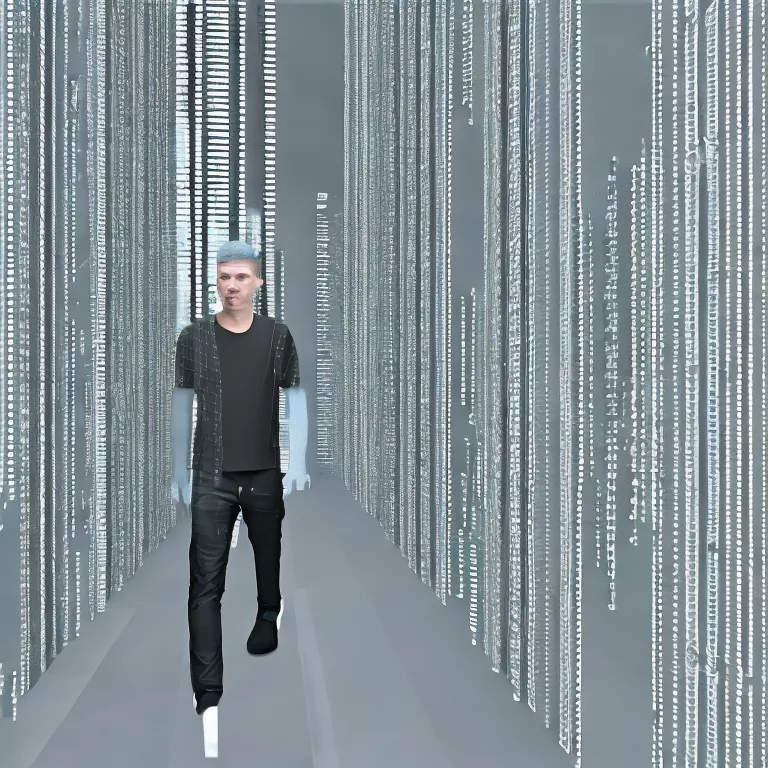
Jest provides several elegant ways for testing expected exceptions and monitoring behaviors of functions, with two paramount methods namely `jest.fn()` and `jest.spyOn()`.
The function `jest.fn()` creates a new mock function that can be used to test run-time behavior of a script. For instance, one could track how many times this mocked function is called, or the specific arguments it was invoked with.
html
xxxxxxxxxx
const mockFn = jest.fn();
mockFn();
console.log(mockFn.mock.calls.length) // Returns 1
However, in the event you’d like to wrap an existing function with a mock function to observe and keep track of calls made to it, then `jest.spyOn()` comes into play.
html
xxxxxxxxxx
const obj = {
myMethod: () => 'hello'
};
const spy = jest.spyOn(obj, 'myMethod');
obj.myMethod();
console.log(spy.mock.calls.length); // Returns 1
On the topic of expecting a function to throw an exception in Jest, both `jest.fn()` and `jest.spyOn()` can be employed to enforce specific conditions before utilizing the `.toThrow()` matcher, perfect for scenarios where we anticipate errors.
For example:
html
xxxxxxxxxx
// Using jest.fn()
const mockFn = jest.fn(() => {
throw new Error("Test Error");
});
expect(() => mockFn()).toThrow("Test Error");
// using jest.spyOn
const obj = {
myMethod: () => { throw new Error("Spying error"); }
};
const spy = jest.spyOn(obj, 'myMethod');
expect(() => spy()).toThrow("Spying error");
In both cases, the error messages are wrapped in an anonymous function because a direct invocation (without the function wrapper) would immediately execute and throw the error, leading to a test failure.
As ESLint suggests it’s important to wrap the throwing code into a function else the `toThrow` assertion could pass for the wrong reasons!
To paraphrase Martin Fowler – Testing code is just as important as writing it. Not rigorously testing exceptions and errors can lead to unexpected defects and user dissatisfaction; hence it’s advised to optimize usage of `jest.fn()` and `jest.spyOn()`, ensuring a top-notch application development.
Comprehend The ‘toThrow’ Matcher: Anticipating Exceptions in a Jest Environment
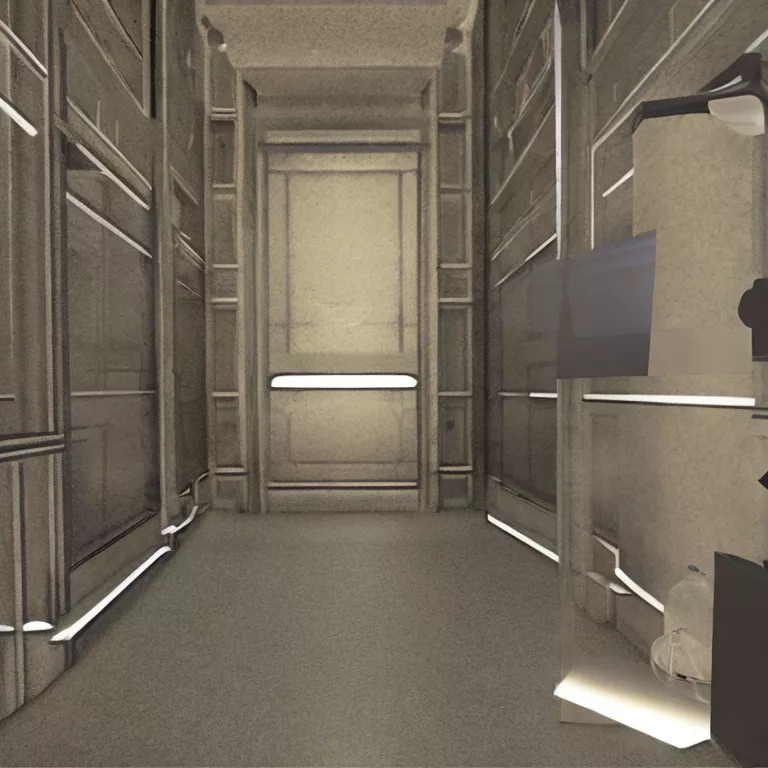
Understanding and implementing exception handling is an indispensable aspect of robust software development. When using Jest, a widely adopted JavaScript testing framework, the ‘toThrow’ matcher plays a vital role in predicting exceptions. This assertion provides developers with an efficient means to verify if a particular function throws an error under certain conditions.
Notion Behind ‘toThrow’
The ‘toThrow’ matcher embedded within the Jest environment helps software developers predict and handle errors by expecting them during the execution of a piece of code. It checks whether a function, when invoked, will throw an error — that is, it leads to an exception.
xxxxxxxxxx
function myFunction() {
throw new Error("Error occurred!");
}
expect(myFunction).toThrow();
If ‘myFunction’ does call an error upon invocation, this test would pass or else it would fail.
Specific Error Messages with ‘toThrow’
The ‘toThrow’ method can also adapt to specific error messages, augmenting its capability to deal with detailed expectations regarding exceptions. This can be extremely helpful in creating more precise tests.
xxxxxxxxxx
function myFunction() {
throw new Error("Error occurred!");
}
expect(myFunction).toThrow("Error occurred!");
In case the error message doesn’t match the anticipated one, the test will not succeed, offering even more detailed control over exception handling in your test suites.
Efficiency of ‘toThrow’
Asserting anticipated errors using the ‘toThrow’ matcher in Jest infuses efficiency and predictability into the testing phase. By anticipating probable exceptions, you pave the way for more resilient and error-proof code, thereby enhancing overall application stability and performance.
As renowned programmer Martin Fowler pointed out, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The ‘toThrow’ matcher contributes substantially to realizing this outlook by making error handling transparent, predictable, and humanly understandable.
On the whole, embracing ‘toThrow’ in Jest is a crucial step towards efficient error anticipation in automated testing. It not only helps software developers detect errors at an early stage of development but also contributes to creating strong, resilient, and maintainable JavaScript applications.
Applying Async/Await with Error Handling in Jest Tests
In JavaScript, async/await simplifies asynchronous operations, making them look and behave like synchronous code. But with this simplification comes a new set of challenges, particularly when working with Jest, a popular JavaScript testing framework.
A common requirement in tests is to check if a function throws an exception. With Jest, it’s straightforward to test a synchronous function that throws an error using
xxxxxxxxxx
toThrow()
. Just wrap the function call inside a function expression and pass it to
xxxxxxxxxx
expect().toThrow()
.
However, when you are dealing with an asynchronous function using async/await, the process becomes a bit more complex. Async functions return promises and exceptions thrown inside these functions cause the promise to be rejected. Therefore, when testing, we should wait for the Promise to resolve or reject.
Here’s how you can do this:
javascript
test(‘throws an error’, async () => {
async function flawedFunction() {
throw new Error(‘Not cool’);
}
expect.assertions(1);
try {
await flawedFunction();
} catch (e) {
expect(e.message).toMatch(‘Not cool’);
}
});
Breaking down the code:
– Async function: defined with the keyword `async`. When it’s called, it returns a Promise. When the function throws an error, the Promise gets rejected.
– Using `await` in front of the flawed function call makes JavaScript wait until the Promise settles, and then resumes the execution while returning the resulting value.
– The exception is captured in the `catch` block where Jest’s `expect().toMatch()` is applied on the error message to check if it matches the expected value.
– `expect.assertions(1);` is used to ensure that one assertion is called within our test case. This especially safeguards against cases where the promise might not get rejected at all leading to false positives in tests.
A nugget of wisdom from Addy Osmani, an engineering manager at Google working on Chrome: “Write tests. Not too many. Mostly integration.” Despite speaking in context of designing test coverage strategies for applications, this applies here too. While we aim to write comprehensive unit tests, a thorough approach with error handling ensures our async functions and components behave as expected under varying conditions.
This approach underlines the importance of structured test cases in software development, especially when dealing with complex concepts like asynchronous operations. By using Jest for testing and by correctly applying async/await alongside error catching, developers can ensure high-quality, resilient JavaScript code.[Source]
Conclusion
Leading the way in JavaScript Testing Frameworks, Jest offers you the ability to robustly test your functions by expecting them to throw an error or exception under specific circumstances. This advanced testing approach ensures that your function’s error handling is performing as expected, and aids in delineating a precise contract for your functions.
To expect a function to throw an error in Jest, one can wrap it in another function and use the
xxxxxxxxxx
toThrow
method. The syntax is fairly simple and reads logically.
typescript
it(‘throws an error’, () => {
expect(() => {
myFunction();
}).toThrow();
});
In this context, `myFunction` is the function being tested, which we’re expecting to throw an error. This example will catch any error thrown, but Jest gives us the capability of catching specific errors with the same method as well.
Understanding how to expect a function to throw an error in Jest doesn’t just prepare you for dealing with unexpected issues; it empowers you to write more secure, reliable and professional code, leading to a more dependable application.
Remember the words of famous programmer Linus Torvalds: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program”. So, let’s make our way towards effective programming by mastery of skills like testing with Jest.
Let your code not just pass the tests but tell its own story, a story of efficiency, precision, and reliability.