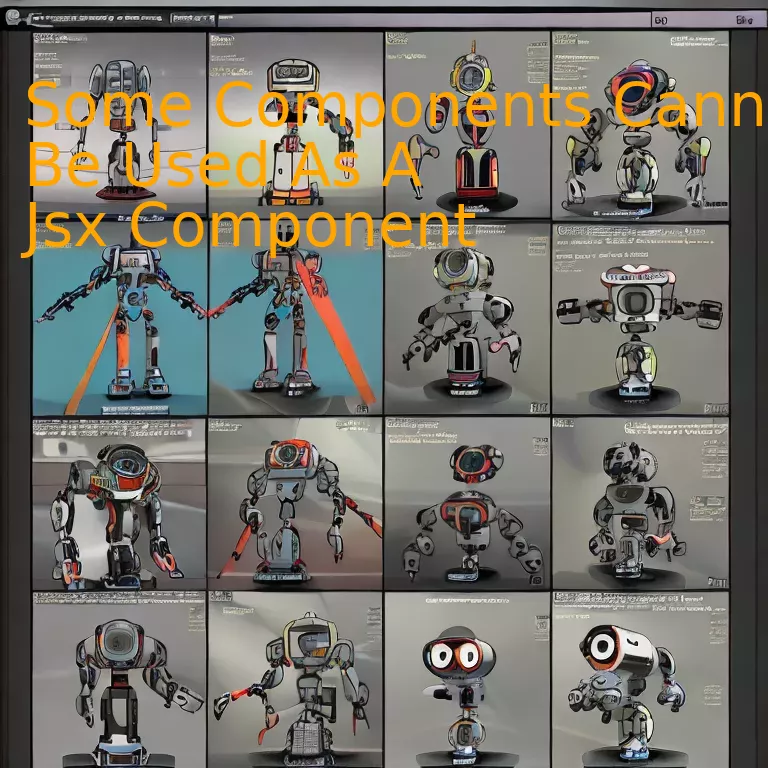
Introduction
In certain situations, not every component can function as a JSX component in React’s extensive library due to coding limitations and software compatibility issues.
Quick Summary
In the context of JSX (JavaScript XML), it must be understood that not every component can be utilized as a JSX component. This limitation arises from the nature of both JSX and JavaScript where specific components are exclusively reserved for certain functionalities and attributes. Examples of those ineligible for use as a JSX component include:
JavaScript Component | Reason |
---|---|
x <div> |
This cannot be used as a JSX component because it’s an inherent HTML tag, rather than a JavaScript or JSX entity. |
xxxxxxxxxx Date() |
This is a built-in JavaScript object, hence it lacks required life-cycle methods of a React component which prevent its usage in JSX. |
xxxxxxxxxx window |
It represents the browser’s window object and does not contain the properties needed to render JSX elements. |
JSX converts these components into calls to
xxxxxxxxxx
React.createElement(componentType)
, but vanilla JavaScript objects like
xxxxxxxxxx
window
and functions such as
xxxxxxxxxx
Date()
lack the properties DOJ Sports Directory expects on its prototype that assist in rendering JSX elements. Consequently, when attempted to use these as a JSX component, it yields a runtime error.
As Martin Fowler, an influential author in software development said: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This applies to JSX and JavaScript components too – correct understanding helps write understandable, functional, and structurally sound code.
Understanding JSX Component Limitations
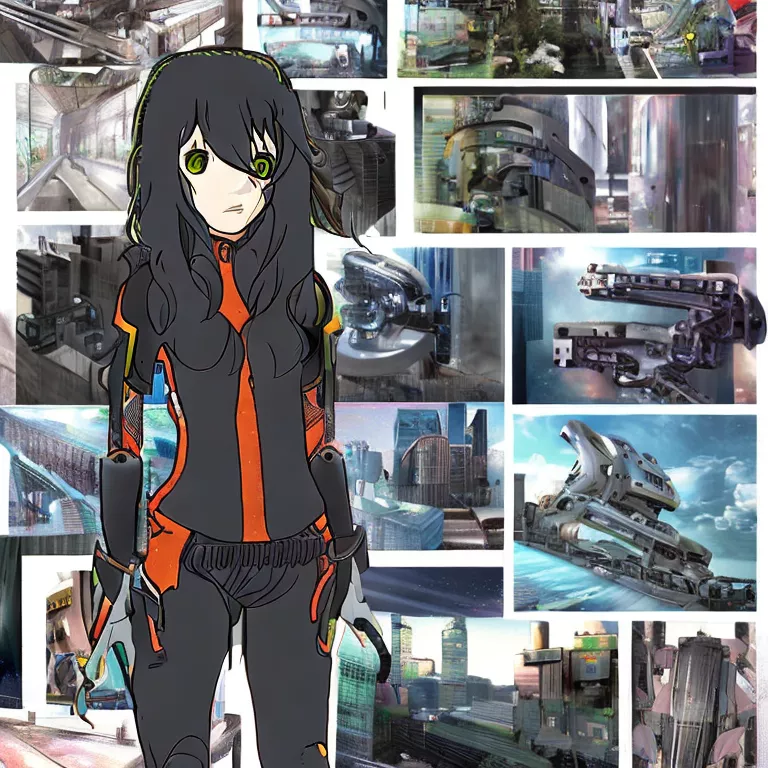
JSX components are central to developing applications in libraries and frameworks such as React. They enable developers to use custom elements, beyond the built-in HTML elements, that can hold complex logic or represent reusable parts of an application’s user interface. Nevertheless, there are certain types of components that cannot directly be used within JSX.
Non-React Components
While JSX is largely associated with React, it is beneficial to clarify that not every JavaScript object or function can serve as a React component, thus being usable in JSX. The main reasons that certain JavaScript objects and functions may not work as JSX components include pure JavaScript functions, array methods like `map()` or `filter()`, Promise objects, and proprietary object methods.
For instance, consider the case of attempting to use a pure JavaScript function within JSX:
xxxxxxxxxx
function greet(name) {
return 'Hello, ' + name;
}
<greet name="John" /> // This will not work
Here, the `greet` function is a plain JavaScript function and not a React component, and hence you cannot use it as a JSX component. To rectify this, you could convert it into a proper functional React component:
xxxxxxxxxx
function Greet(props) {
return <p>Hello, {props.name}</p>;
}
<Greet name="John" /> // Now it works!
DOM Element Constructors
Using the constructors for DOM elements directly as components in JSX is also problematic. For example:
xxxxxxxxxx
<HTMLElementConstructor prop="value" /> // This doesn't work
These element constructor functions aren’t designed to act as React components and do not meet the requirements React expects from a component.
JavaScript Classes
Despite React supporting class-based components, not all JavaScript classes can be used as JSX components. Only those extending from `React.Component` suit the pattern:
xxxxxxxxxx
class NotAComponent {
render() {
return <p>Hello world</p>;
}
}
<NotAComponent /> // Doesn't work
In this situation, you need to extend your class from `React.Component` or `React.PureComponent`.
Functional Components without Return Statement
Functional components that do not return either null or a valid React element will also cause issues if attempted to be rendered directly in JSX.
To conclude, limitations certainly exist on what can and cannot be used as a JSX component. Therefore, understanding these limitations is crucial for developers looking to maximize their effective use of JSX while avoiding potential stumbling blocks.
As Mark Zuckerburg once said, “The biggest risk is not taking any risk… In a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” This quote is memorable for programmers, encouraging them to explore beyond these component limitations for novel solutions, thereby enhancing their coding experience and application performance.
Exploring Why Some Components Can’t Be Used as JSX
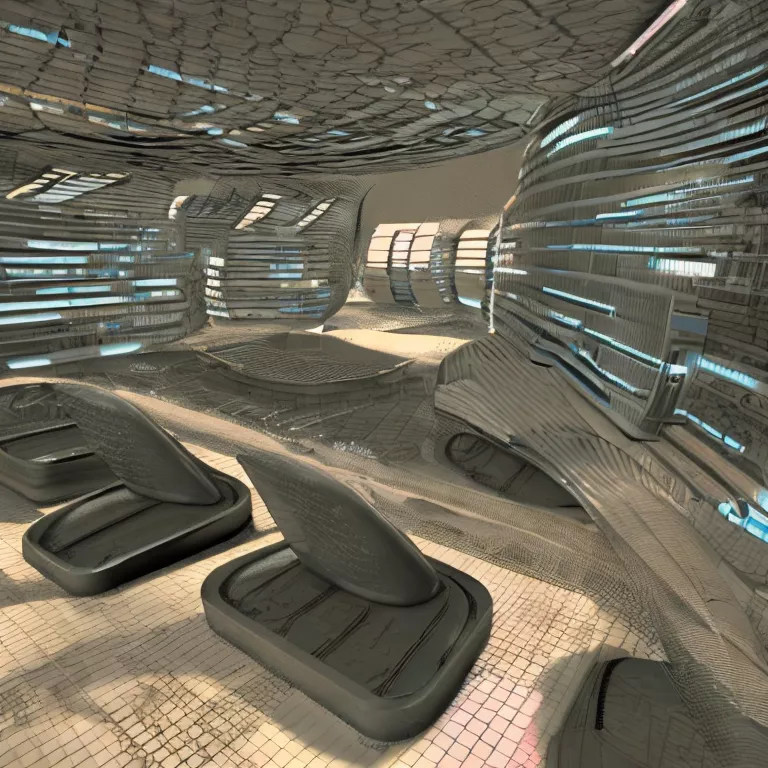
In the context of TypeScript and React, JSX (JavaScript XML) is a popular syntactical extension used to write user interface elements in JavaScript. While it allows developers to interactively create UI components with a syntax closely resembling HTML, it’s crucial to be aware that not all components can be applied as a JSX component.
Function vs Class Components
The nature of a component, i.e., whether it’s a function or a class component, has a significant bearing on its usage as a JSX component.
- Function Component: It’s the simplest form of a component and must return a single React element. Function components are typically expressed as functions returning JSX. However, if the function doesn’t adhere to this requirement, it cannot be utilized as a JSX component.
typescript
function Welcome(props) {
return
xxxxxxxxxx
{props.name}
;
}
- Class Component: A class component should extend from React.Component while defining a method called render(). The render() function should return a single React element. Object methods not adhering to these criteria do not qualify as valid JSX components.
typescript
class Welcome extends React.Component {
render() {
return
xxxxxxxxxx
{this.props.name}
}
}
Invalid Components
On occasion, one might encounter situations where certain components have not been imported correctly or defined without following the precise structure required by React. Such components will invariably fail when treated as JSX components.
Component Name Convention
In React, only components starting with a capital letter can be rendered as a JSX element. This rule stems from JavaScript’s distinction between built-in and user-defined elements. According to this rule, lowercase names are reserved for HTML tags, while capitalized names indicate custom (user-defined) components. Therefore, any component that does not follow this convention cannot be utilized as a JSX component.
Consider the words of Kent C. Dodds, a renowned software engineer – “In JSX, the naming conventions for components are strict. A component’s name must start with an uppercase letter; otherwise, it may lead to very unpredictable results.”
Ultimately, the inability of some components to act as JSX components hinges on these critical parameters. Any deviation from established norms and rules can prevent a perfectly valid component from being used as a JSX element.
Decoding HTML vs. JSX: Key Differences to Know
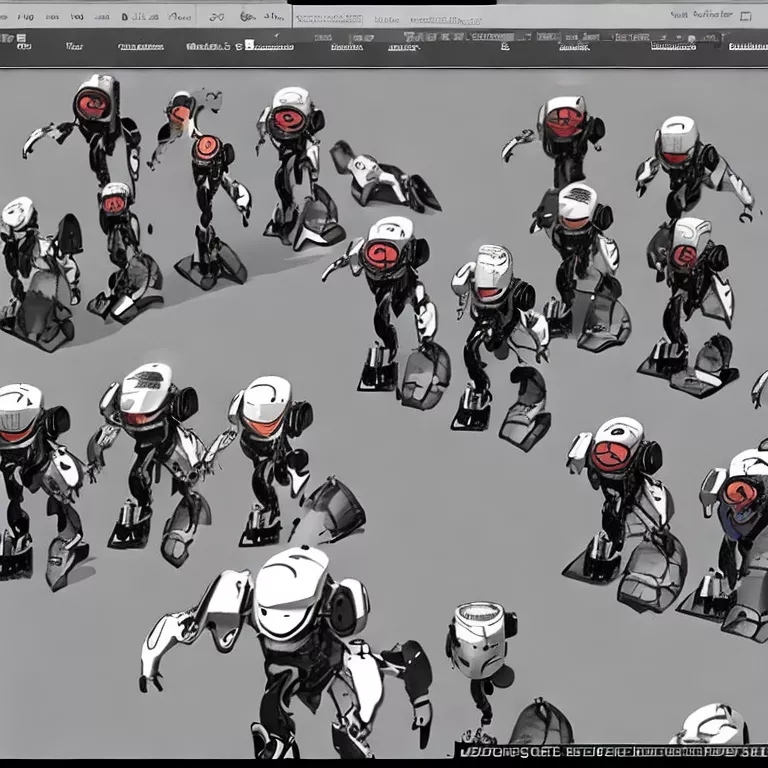
Let’s delve into the marked contrast between HTML and JSX, specifically addressing why certain components are incompatible with a JSX component. Understanding the key differences between these two could go a long way in sculpting your TypeScript application and improving your dynamics with ReactJS.
Perhaps you might be aware that ReactJS uses JSX, an XML-like syntax extension to JavaScript, vastly different from regular HTML that we use for building static web pages. The conflicting structure between HTML and JSX is precisely why not every HTML construct can serve as a JSX component.
For starters:
– HTML and JSX handle expressions differently: HTML doesn’t possess any built-in capabilities to interpret JavaScript logic while JSX allows incorporation of JavaScript logic via curly brackets
xxxxxxxxxx
{ }
.
Consider this example wherein an HTML element is unable to interpret JavaScript:
xxxxxxxxxx
<div>
&{msg}&
</div>
Contrastingly, the equivalent JSX segment would seamlessly execute this task:
xxxxxxxxxx
<div>
{msg}
</div>
Here,
xxxxxxxxxx
msg
is a JavaScript variable. JSX and HTML differ fundamentally in their approach to handling variables, logic, or expressions. That asserts the point that not all HTML components can fit like a glove into the JSX coroutine.
– Closure of tags: There’s an essential rule in HTML that not all tags need closure, such as
xxxxxxxxxx
<img>, <br>, <input>
. This rule does not hold when you move into the territory of JSX. JSX demands every tag to close properly – a requirement testers often overlook, leading to runtime errors.
Return to Southwind (n.d.) emphasized correctly on coding, stating that “Good code is its own best documentation.” Herein, the proper, documented structure of JSX is a good coding standard ensuring components are built without syntax discrepancies, thereby preventing erroneous outputs. [1]
“Good code is its own best documentation”
– Classes and inline styles: In an HTML component, classes and inline styles are defined as
xxxxxxxxxx
class
and
xxxxxxxxxx
style
. In contrast, JSX takes a different route, requiring the terms
xxxxxxxxxx
className
and
xxxxxxxxxx
style
(as an object) instead. If you try to use an HTML component that contains a
xxxxxxxxxx
class
attribute, it can’t be used directly as a JSX component.
To conclude, it’s essential to understand these fundamental differences when transitioning between HTML and JSX. Not all HTML elements can serve as JSX components owing to their dissimilar trait to handle expressions, closure of tags, classes, and styles differently. Equipping oneself with this understanding aids in reducing common gotchas while writing React components.
Solutions for Working Around Unsupported JSX Components
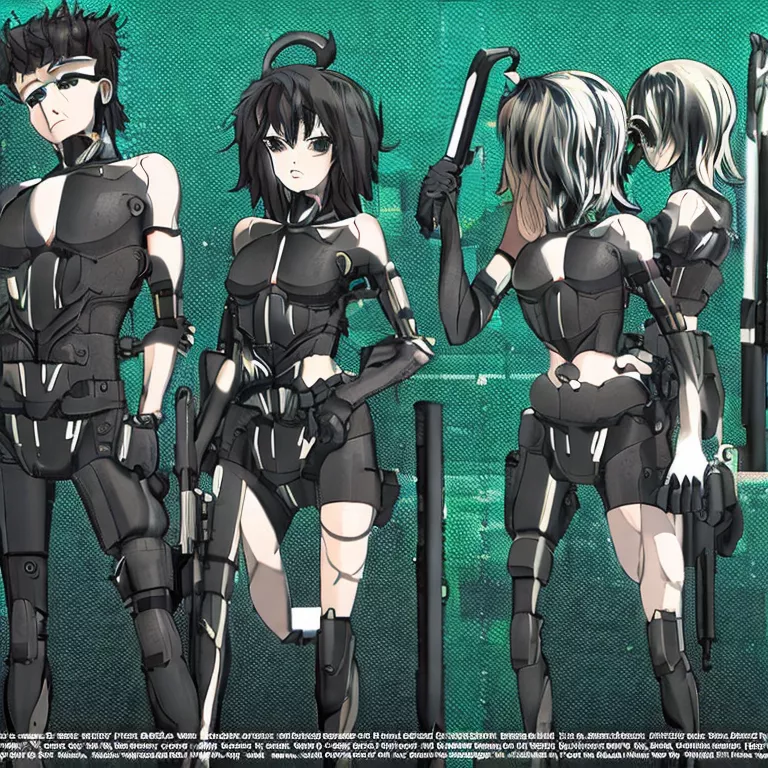
Working around unsupported JSX components can often seem like a daunting task. However, there are effective strategies to circumvent this issue, even for the components that are not designed to be used as a JSX component.
Typically, developers encounter difficulties when attempting to integrate third-party libraries into their projects without knowing if such libraries support React’s JSX syntax or not. Fortunately, several workarounds will allow these libraries’ functionality to incorporate seamlessly.
Using React’s createRef Element
CreateRef is an often overlooked but powerful part of React.1 It generates a ‘ref’ (reference). Refs are commonly used in React to access and interact with DOM nodes directly. You’ll want to use it to assign a ref to the element containing your non-JSX-supportive component.
For instance:
xxxxxxxxxx
typescript
class UnsupportedComponentWrapper extends React.Component {
myRef = React.createRef();
componentDidMount() {
const unsupportedInstance = new unsupportedComponent(this.myRef.current);
}
render() {
return
}
}
The above code shows how you would wrap your unsupported JSX component within a React ref.
Using Non-JSX Syntax
If the component doesn’t support JSX, you may need to revert to a traditional HTML approach. Instead of using JSX, you can use regular JavaScript and manipulate the DOM directly using built-in methods like createElement or appendChild.
xxxxxxxxxx
typescript
const parentElement = document.getElementById(‘parent’);
const newElement = document.createElement(‘unsupported-Component’);
parentElement.appendChild(newElement);
In the code snippet above, we generate an instance of our unsupported component and append it to a parent DOM node.
Adopting the Higher-Order Component (HOC) Pattern
The HOC pattern is a great tool for abstracting reusable functionality in React applications.2 It is particularly useful in this context because it lets you wrap the unsupported component within a supported one, thus gaining more control over its life cycle events.
React’s flexibility is one of its strengths, enabling developers to work smartly and effectively around potential obstacles. As Alan Kay once said about programming, “Perspective is worth 80 IQ points.” Having a clear understanding of these workarounds can revolutionize your approach to problem-solving in dealing with unsupported JSX components.
Always remember to validate if third-party libraries are compatible with React’s JSX syntax. If they’re not, there are several possible options you may take, such as using CreateRef, direct DOM manipulations, or employing the HOC pattern. At last, this should provide a well-rounded solution that caters to the complexities posed by certain components that cannot be used directly as a JSX component.
Conclusion
With regards to Javascript XML (JSX), a syntax extension for scripting languages like JavaScript, its utilization is not without limitations. Intriguingly, JSX cannot be applied to every component within its ecosystem. The demarcation between components that can be used and those that can’t lies in the realm of HTML native elements, regular JavaScript functions, and TypeScript’s distinct component types.
Type | Description |
---|---|
xxxxxxxxxx HTML elements |
JSX shines when it comes to working with HTML elements as it can directly translate them into actual DOM elements. Utilizing JSX with these elementary building blocks of web content perfectly fits its design philosophy. |
xxxxxxxxxx JavaScript functions |
Navigating towards JavaScript functions, things become slightly complicated. Regular classified or arrow functions aren’t JSX compatible, but they can turn into viable options if their return value results from a JSX element. |
xxxxxxxxxx TypeScript component types |
At a glance, TypeScript components appear perfectly adapted to JSX. But, due to how JSX measures equality – object identity rather than structural matching – TypeScript provides various formats for defining JSX components, not all of which are suitable to every situation. |
As Sandi Metz once said: “The only way to go fast, is to go well!” This wisdom holds true when aiming to optimize code design in JSX. An understanding of what components can and cannot be used will guide developers in writing efficient code, thereby aiding to maximize software performance while minimizing resource usage.
Visit the official ReactJS page to gain a detailed understanding regarding JSX elements and their effective use.
Hopefully, this exploration into the use of JSX components serves as a sincere guide towards maximizing its potential by understanding its limitations. Mindful utilization of these tools can make all the difference in app development projects.